Object-oriented programming (OOP) is a powerful paradigm that helps you design complex software architectures efficiently. In this guide, we focus on the application of OOP with PHP using a practical example: a boat rental service. The goal is to define methods that manage the renting and returning of boats. This structure will help you deepen your understanding of working with classes, constants, and arrays in PHP.
Key Insights
- Use of constants for frequently used values
- Use of associative arrays to store rental information
- Automation of time tracking
- Definition of custom methods for handling rental and return processes
Step-by-Step Guide
1. Create the Boat Rental Class
Start by defining the Boat Rental class. Within this class, you will set the constants and variables necessary for the functioning of the boat rental service. It is helpful to define the hourly rate as a constant that is used throughout the system.
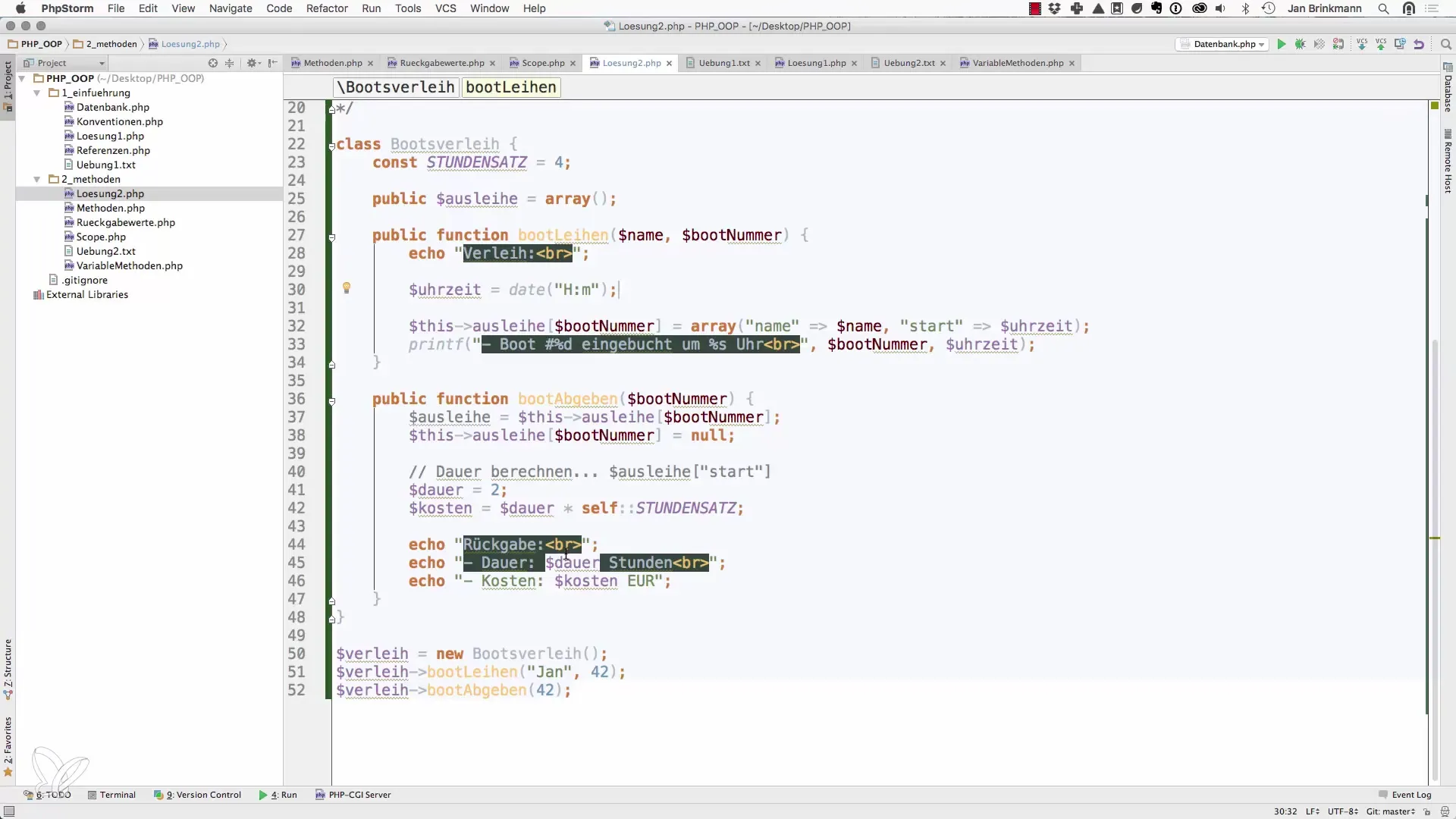
2. Declare Constants and Variables
Add the constants and a temporary array to store rental information. For example, the hourly rate could be set to €4. The array serves to store data as long as the instance of the class exists.
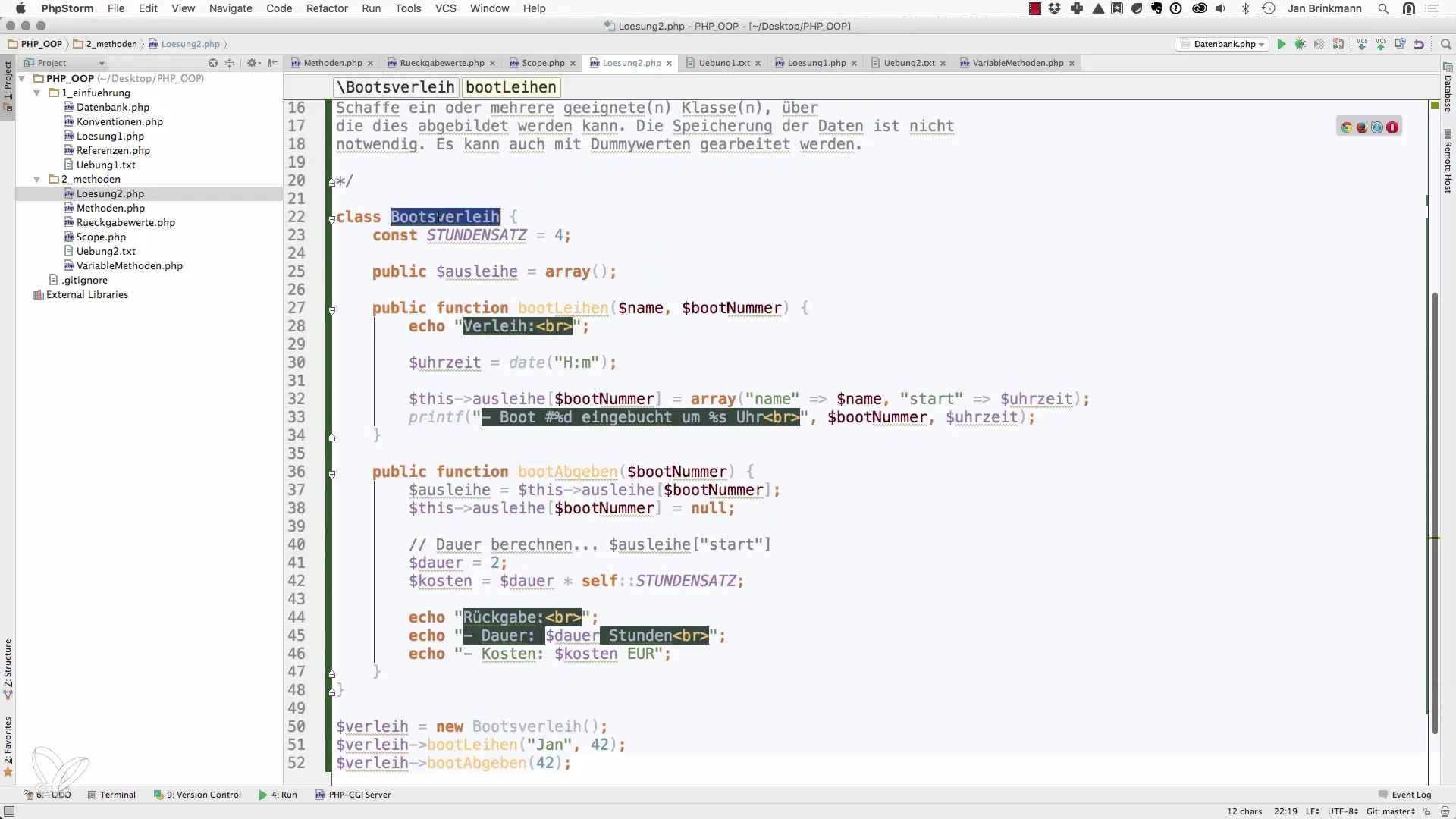
3. Implement the Method for Renting a Boat
Define a method that allows the renting of a boat. This method should take the boat number and the customer's name as parameters. You can automatically capture the system time instead of entering it manually.
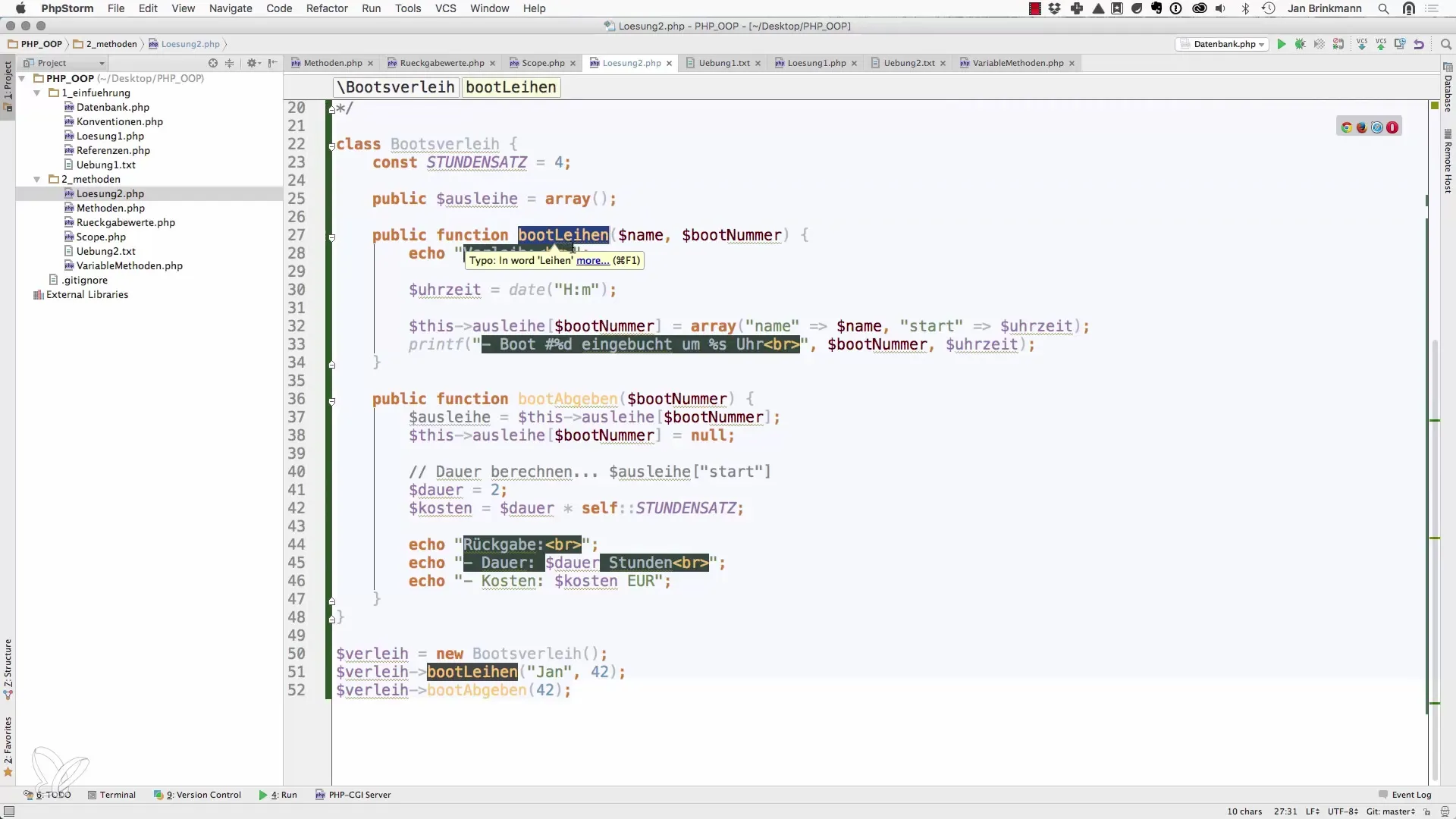
4. Store Rental Information
In the renting method, you will add the rental information to the associative array. Each boat number serves as a key that stores the customer's name and the rental time.
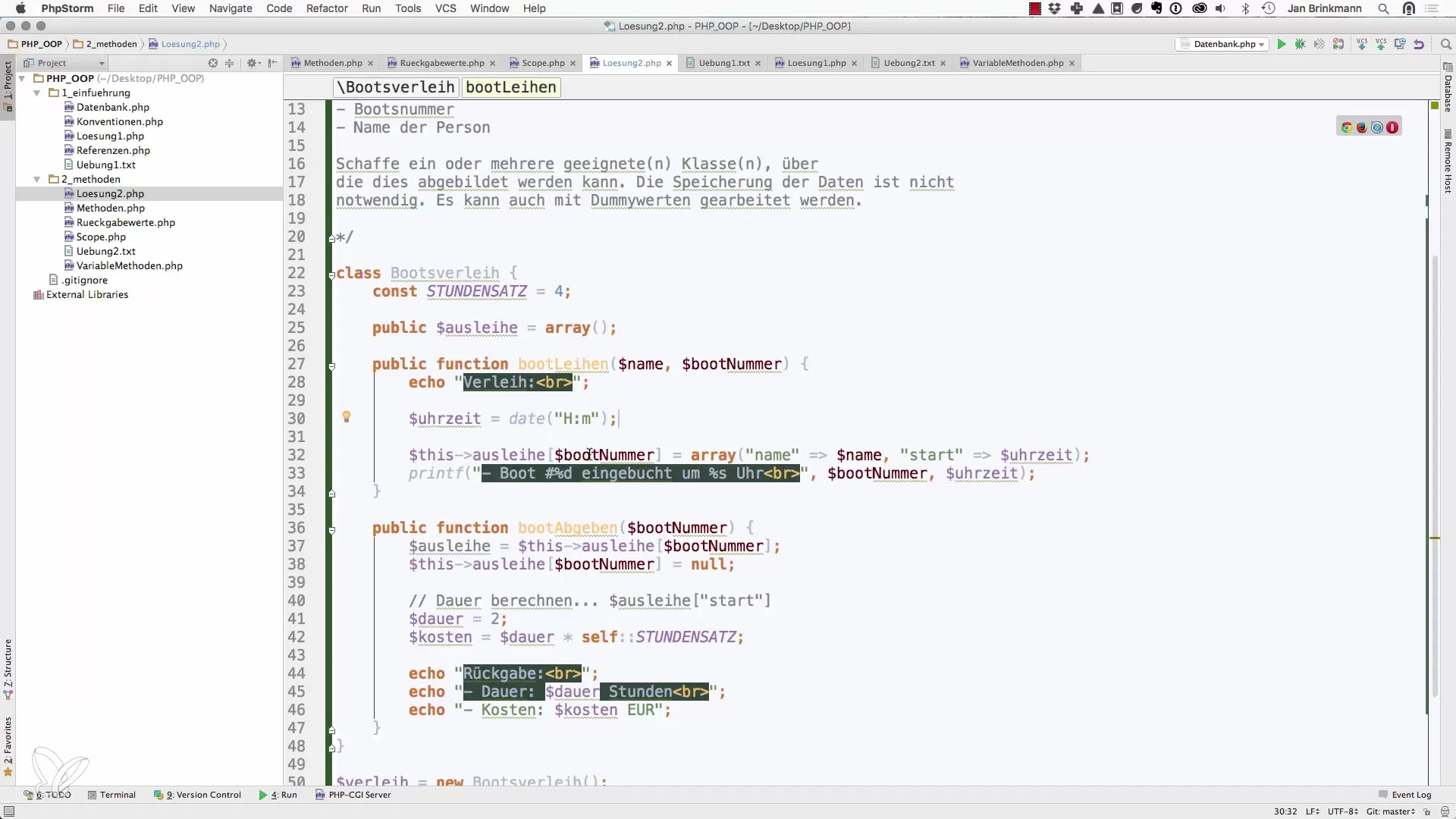
5. Create the Return Method
In the next step, you will create a method that manages the return of a boat. This method should record the return time, remove the rental information from the array, and calculate the duration.
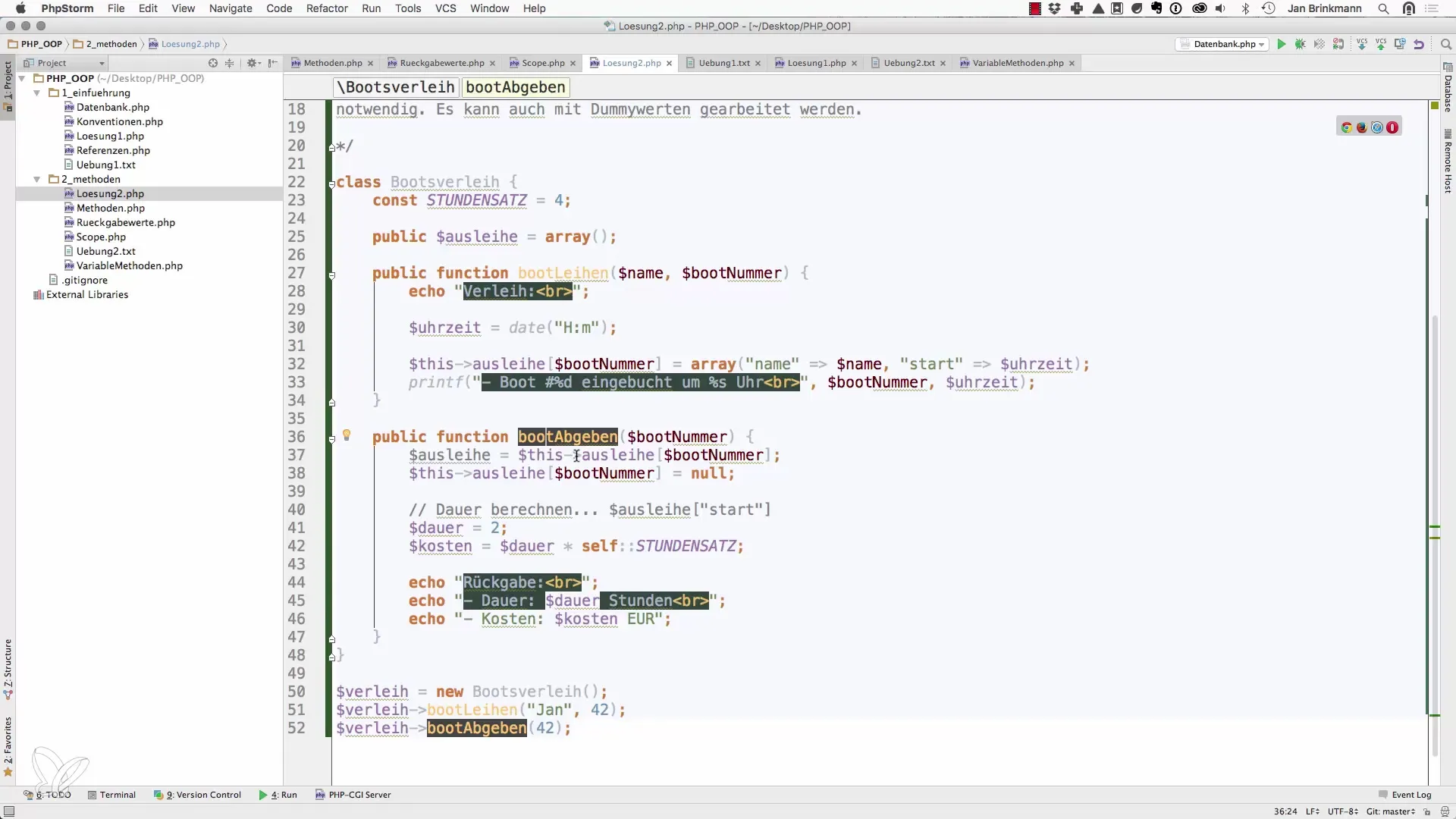
6. Implement Cost Calculation
Within the return method, you implement the logic for calculating the cost. This is done based on the elapsed time and the constant hourly rate. In this example, it is assumed that the duration is 2 hours, so the cost amounts to €8.
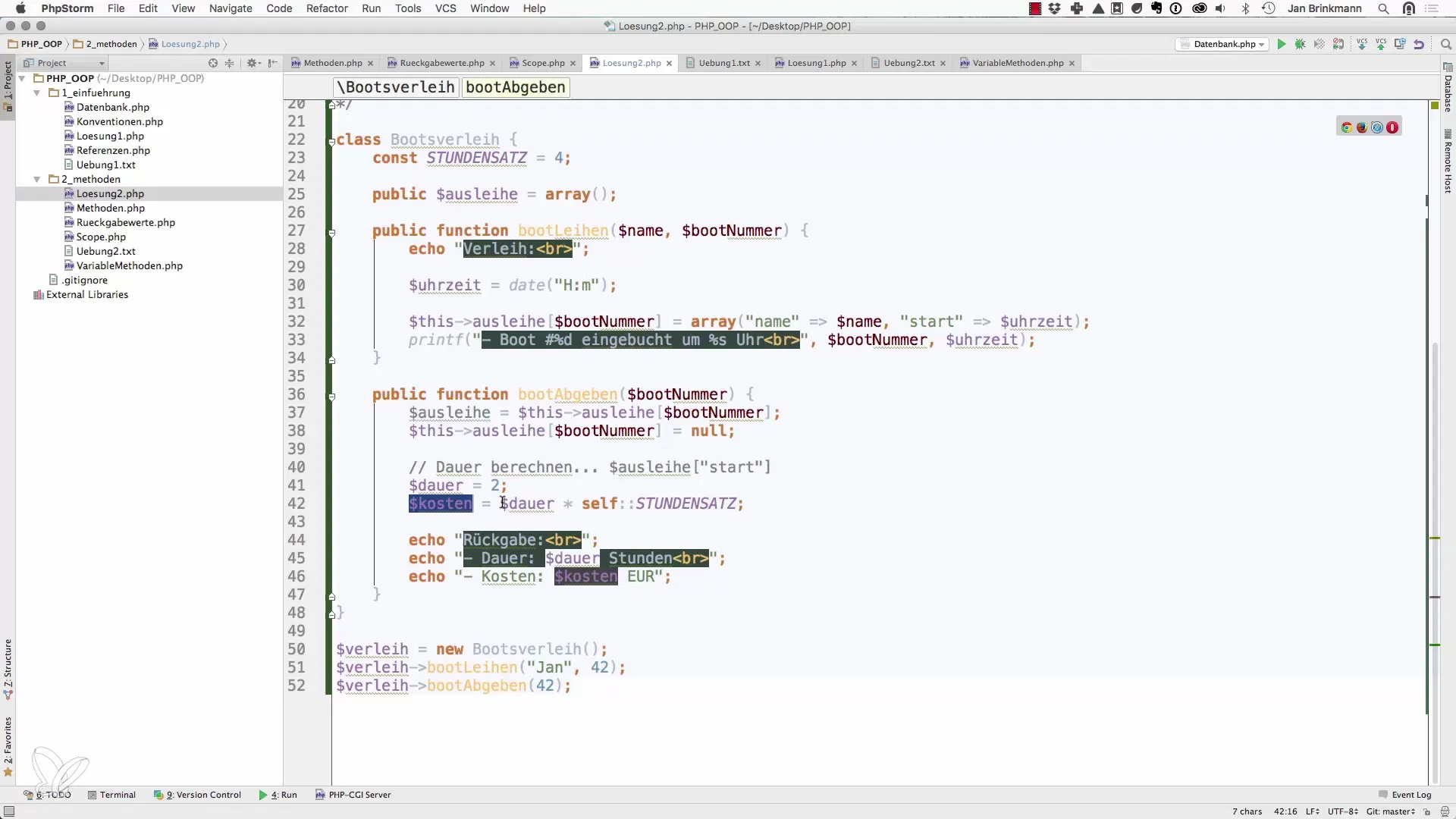
7. Output Results
Finally, you will output the results in the browser. The return values should display the boat number, time, and the calculated costs. You can use the printf function to format the output.
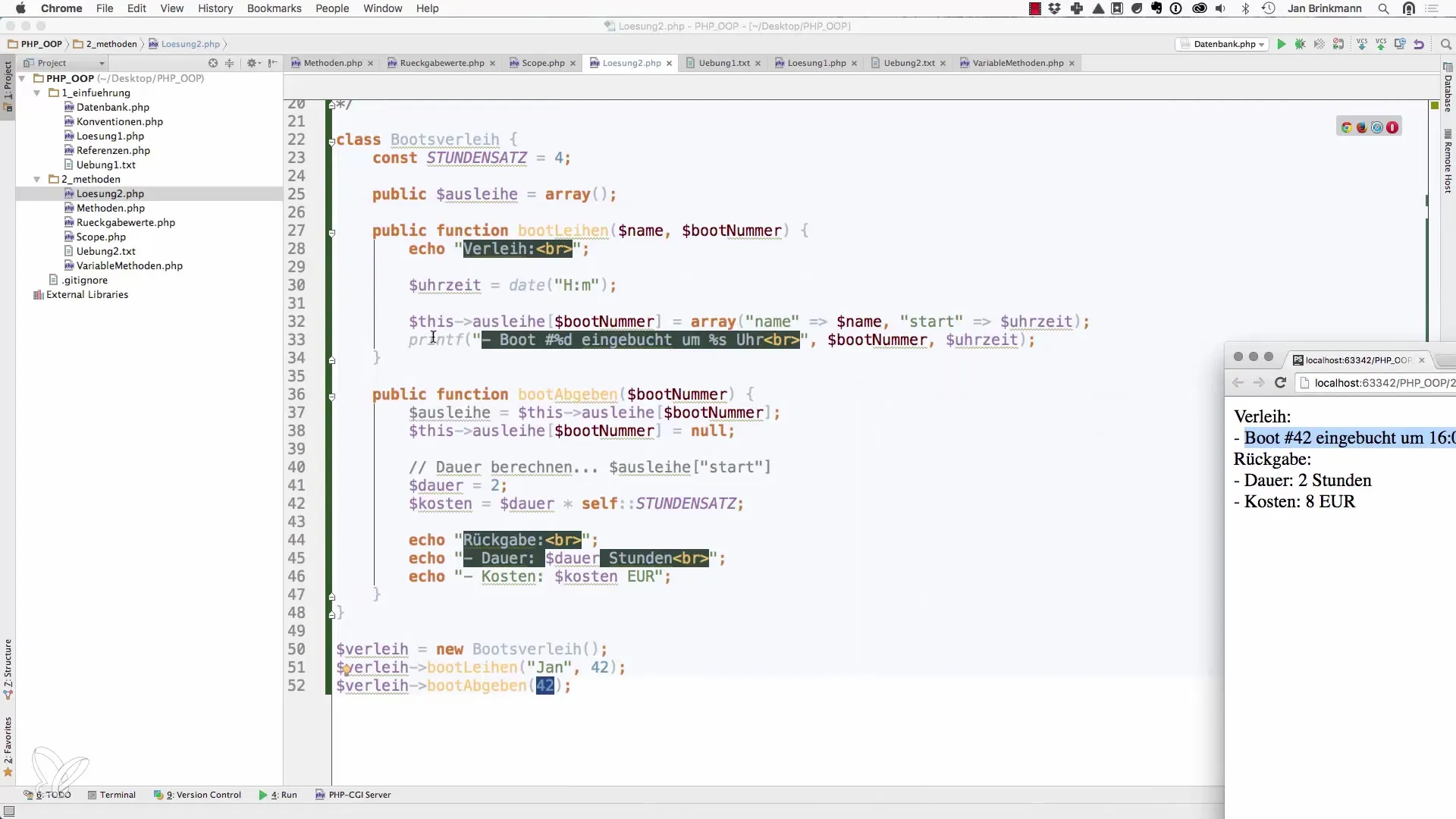
8. Method Usage and Best Practices
Overall, it is important to define methods for specific tasks in your class and consider the use of constants for immutable values. This contributes to the maintainability and readability of the code.
Summary – Object-Oriented Programming with PHP
In this guide, you learned how to create a class for a boat rental service using PHP. By defining methods and utilizing constants, you have laid a solid foundation for managing rental and return processes.
Frequently Asked Questions
What role does a class play in PHP?The class defines a blueprint for objects and contains methods and variables for handling information and processes.
Why should one use constants?Constants help keep frequently used values centralized and immutable, making the code more readable and maintainable.
How does cost calculation work?The costs are calculated based on the duration of the rental and the constant hourly rate.
What does the use of associative arrays allow?Associative arrays allow flexible storage of data by using keys (e.g., boat number) that contain information related to the respective values.
What is the significance of the printf function?The printf function allows formatted output by replacing placeholders with values, which improves the readability of the output.