Choose the right way to organize your modules to optimize the clarity and maintainability of your code. In this tutorial, you will learn how to combine your modules into packages to create a structured and customizable programming environment.
Key Insights
- Packages serve to group modules and improve the structure of a Python project.
- The __init__.py file plays an important role in defining packages.
- With special lists and the import of modules, you can control the visibility and accessibility of your functions.
Step-by-Step Guide
Step 1: Create a Directory
First, create a directory where you can store your modules. For example, you could name your directory DBAdapter. Be sure to make it clear that it is a directory where your submodules will be stored later.
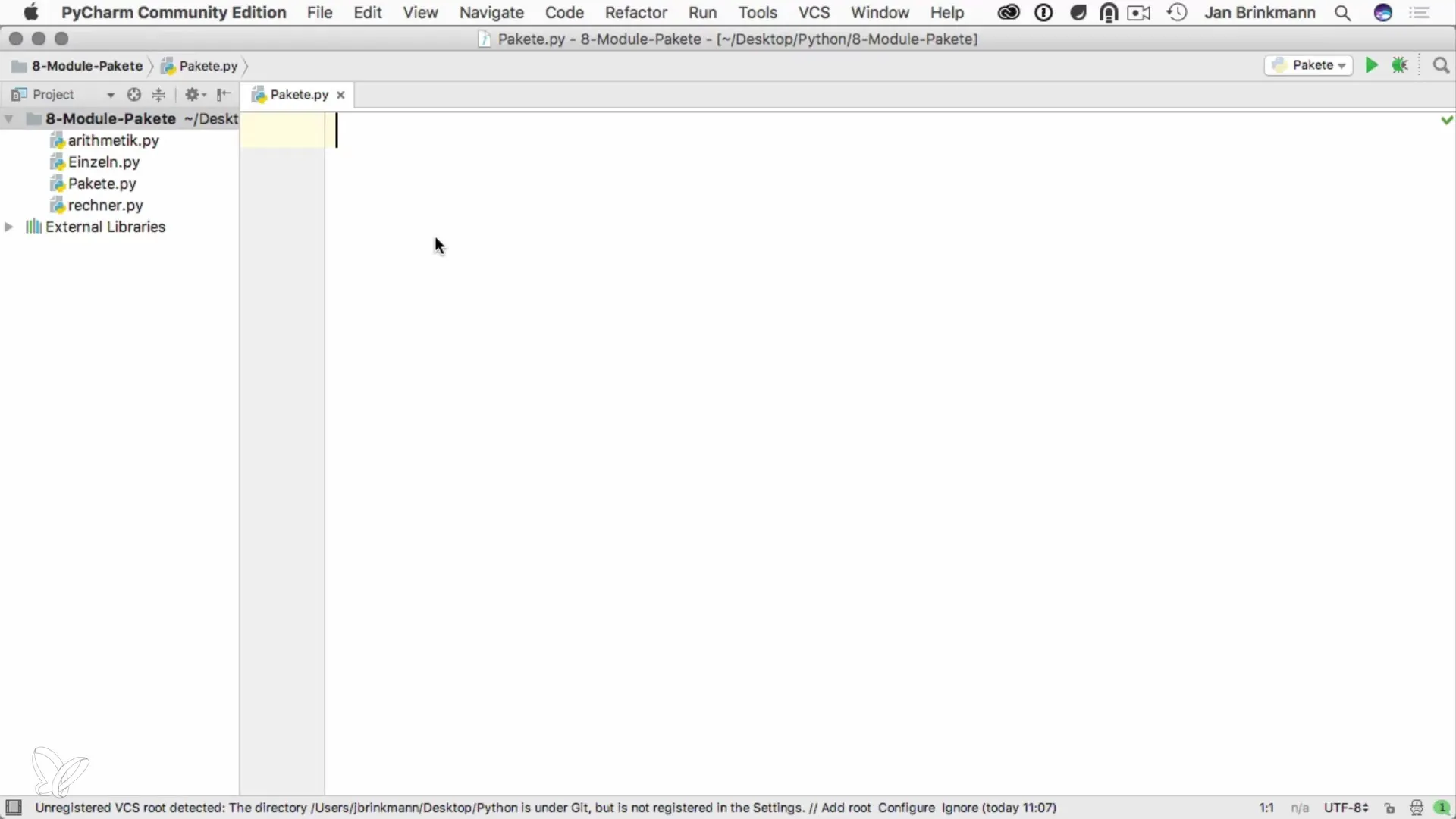
Step 2: Create Python Files for Modules
Now, create a separate Python file for each database module. For instance, create one file for MySQL and another for SQLite. These files will contain functions specific to each database connection, allowing you to keep the code clear and organized.
Step 3: Prepare Module Imports
After you have created your modules in files, you can start with the import. Similar to simple modules, you can also work here with the command from DBAdapter import MySQL. However, seeing that the import is not currently in use and is therefore grayed out will help you keep your code clean.
Step 4: Define Functions
In your Python files, define specific functions. For example, you could create a connect() function in the MySQL file that establishes a connection to the database. A similar function should also be present in the SQLite file.
Step 5: Interpret Packages
To actually use your packages, you need the __init__.py file in the main directory. This file is crucial because it tells Python that this directory should be treated as a package. If you use the directory without this file, it will not be recognized as such.
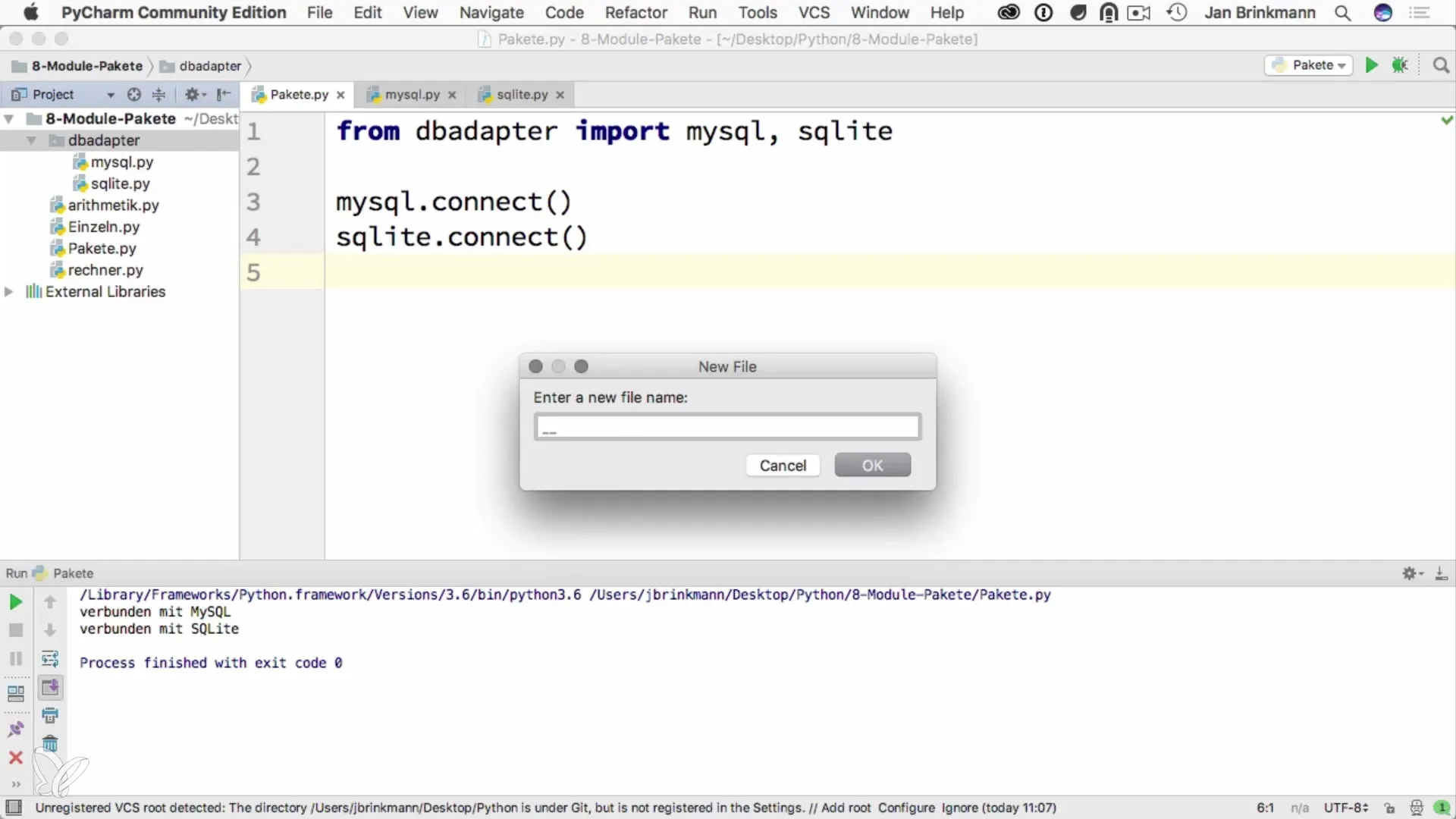
Step 6: Selective Importing of Modules
You have the opportunity to control all modules that should be imported through a special variable. Define a list named __all__, in which you specify the modules that can be imported. For example, you could include __all__ = ['MySQL', 'SQLite'] in your __init__.py file to control what gets imported.
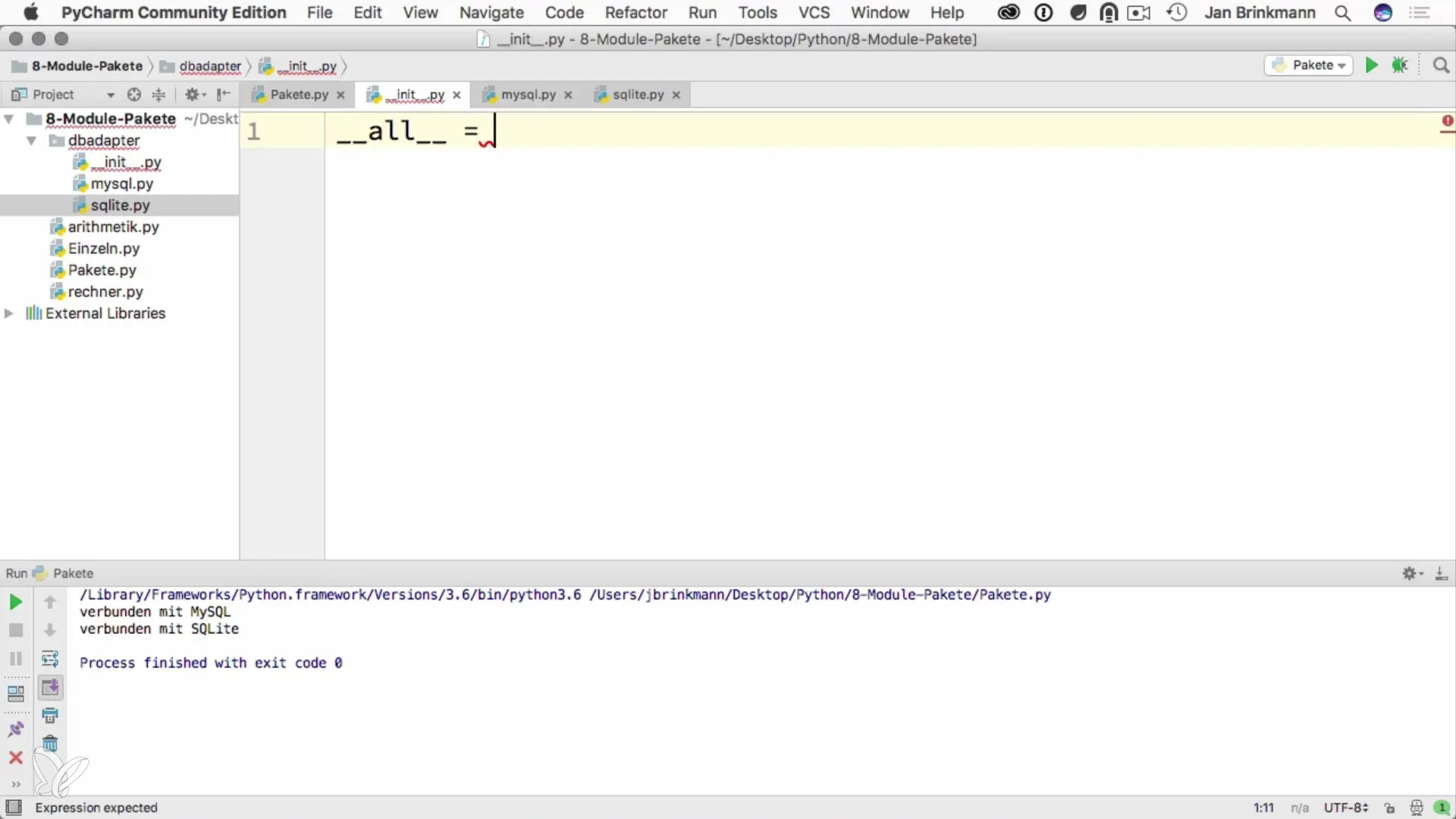
Step 7: Troubleshooting and Optimization
If you try to import all modules with a star import (from DBAdapter import *) and receive an error, it's because Python does not know which modules should actually be imported. Make sure your __all__ list is correctly set to ensure the error-free operation and functionality of your code.
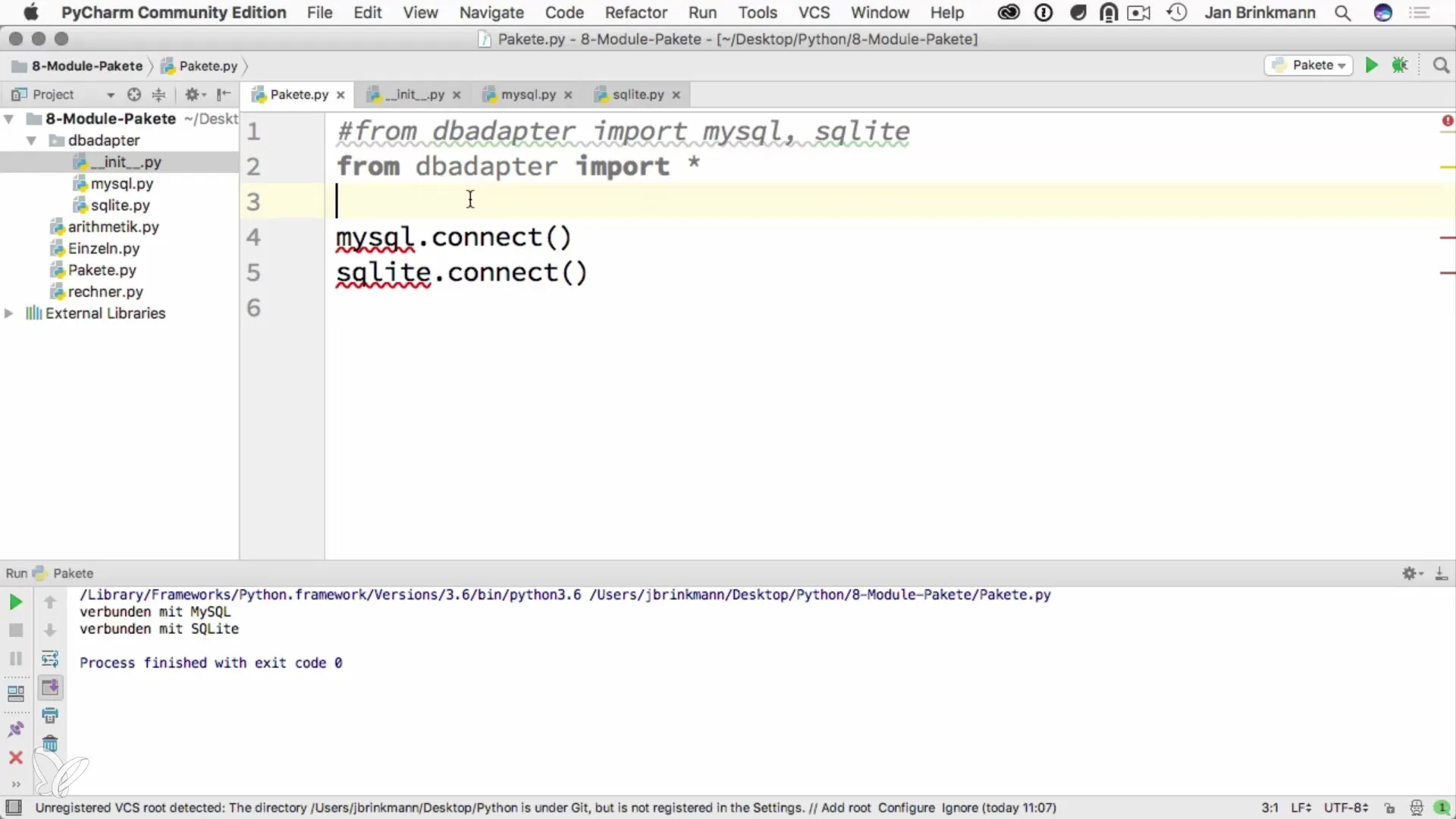
Step 8: Test the Structure
After you complete all the necessary steps, test your structure. Try to establish both MySQL and SQLite connections to ensure that both modules function independently of each other. This guarantees that your packages are correctly set up and that the desired functions work properly.
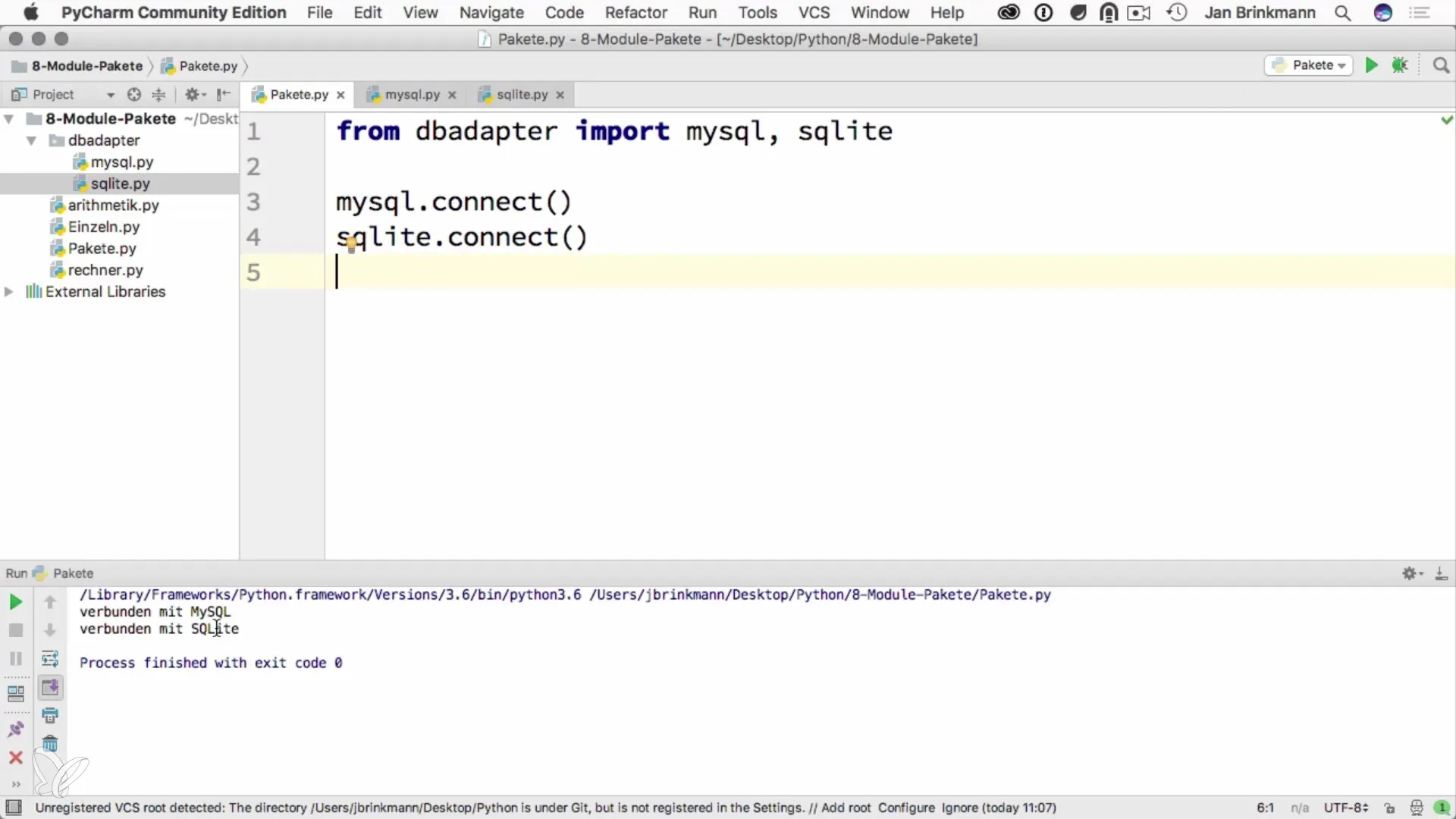
Summary - Packages in Python - Professionally Organizing Modules
By using packages in Python, you can effectively organize your modules and improve the clarity of your code. By utilizing the __init__.py file and specialized lists like __all__, you control the import of modules and minimize potential conflicts.
Frequently Asked Questions
What are packages in Python?Packages are a way to group modules and optimize the structure of a Python project.
How do I create a package in Python?A package is created by creating a directory with an __init__.py file and the modules contained within it.
Can I import multiple modules from a package?Yes, you can import multiple modules by using the star symbol (*) or a list named __all__.
What does the __init__.py file do?The __init__.py file informs Python that it's a package and allows for specific control over the imported modules.
What do I do in case of import errors?Check your __all__ list to ensure it is set correctly and lists all required modules.