Establishing a connection between Python and MySQL is an essential skill for anyone who wants to develop database applications. In this guide, you will learn how to access the MySQL database from Python. This includes installing the necessary library, setting up a project, and creating a class that manages the connection to the database.
Key insights
- Installation of the MySQL Connectors
- Creation of a Python package for a clear project structure
- Implementation of a class to handle the database connection
- Use of cursors to execute SQL commands
Step-by-Step Guide
Step 1: Installation of the MySQL Connector
To establish a connection to MySQL, you first need to install the MySQL Connector for Python. Open the terminal or command prompt for this. Use the following command to install the package:
If the connector is already installed, you will see a corresponding success message.
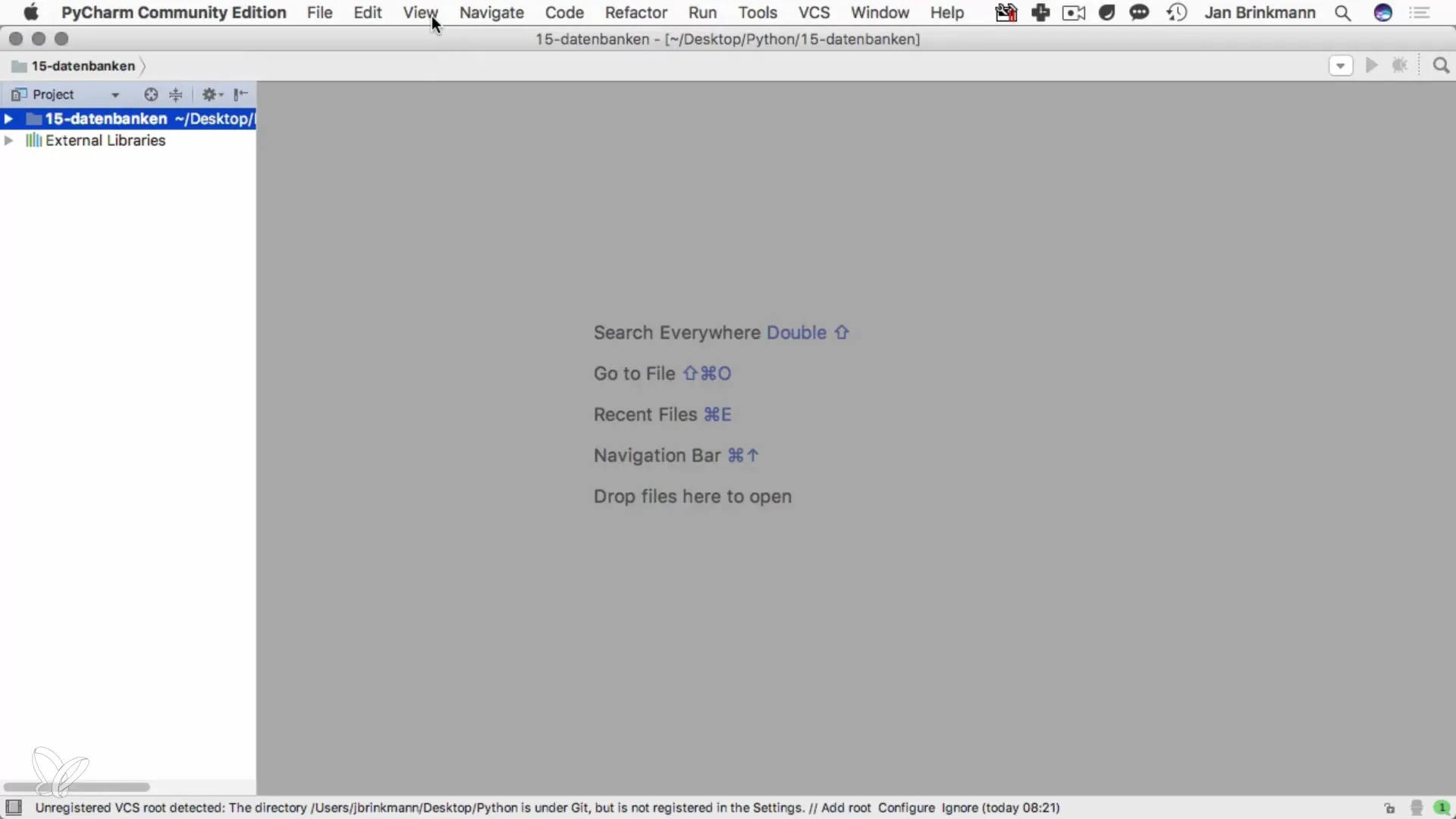
Step 2: Creating a New Python Project
Now it’s time to create your Python project. Create a new Python file, for example, book.py. This step ensures that you can implement your logic in a clearly structured format.
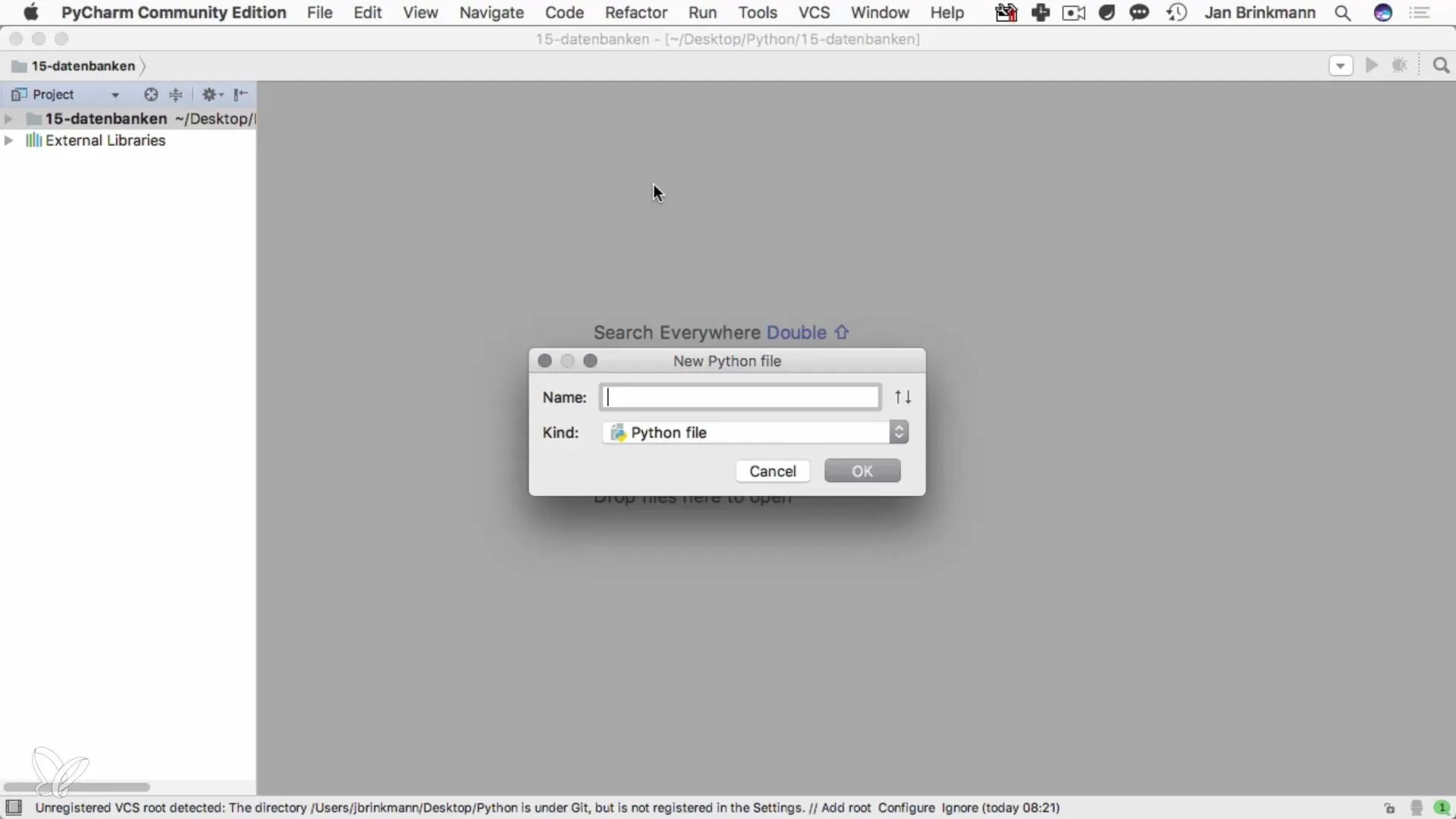
Step 3: Preparing the Python Package
To further refine the structure of your project, create a new package that we will call booklist. Within this package, you will create a file named bookDB.py. This file will be responsible for managing your database connection.
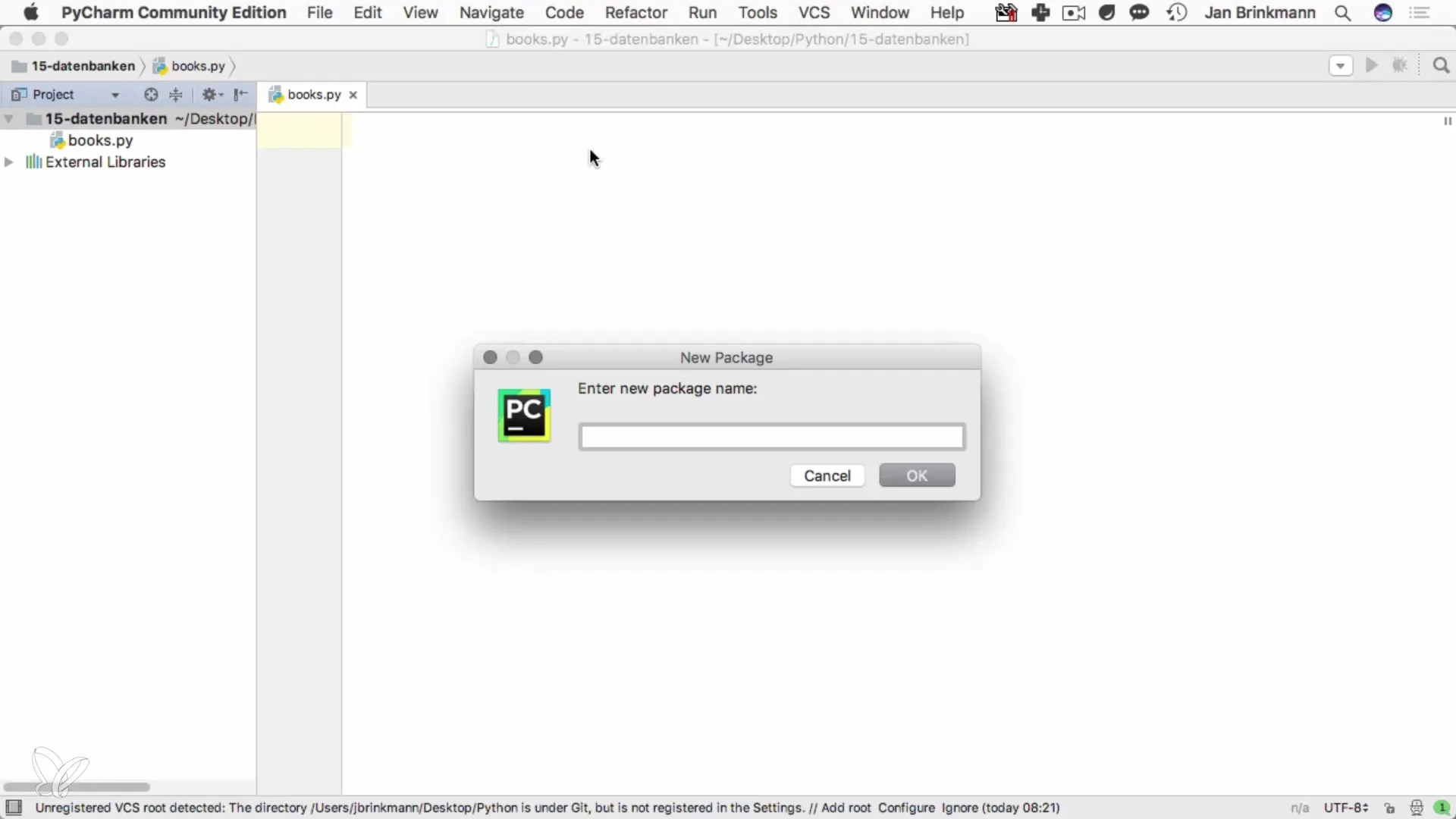
Step 4: Implementing the Database Class
In bookDB.py, you start by defining a class that is responsible for resource management. Name the class BookResource. It will contain the methods for establishing and handling the database connection.
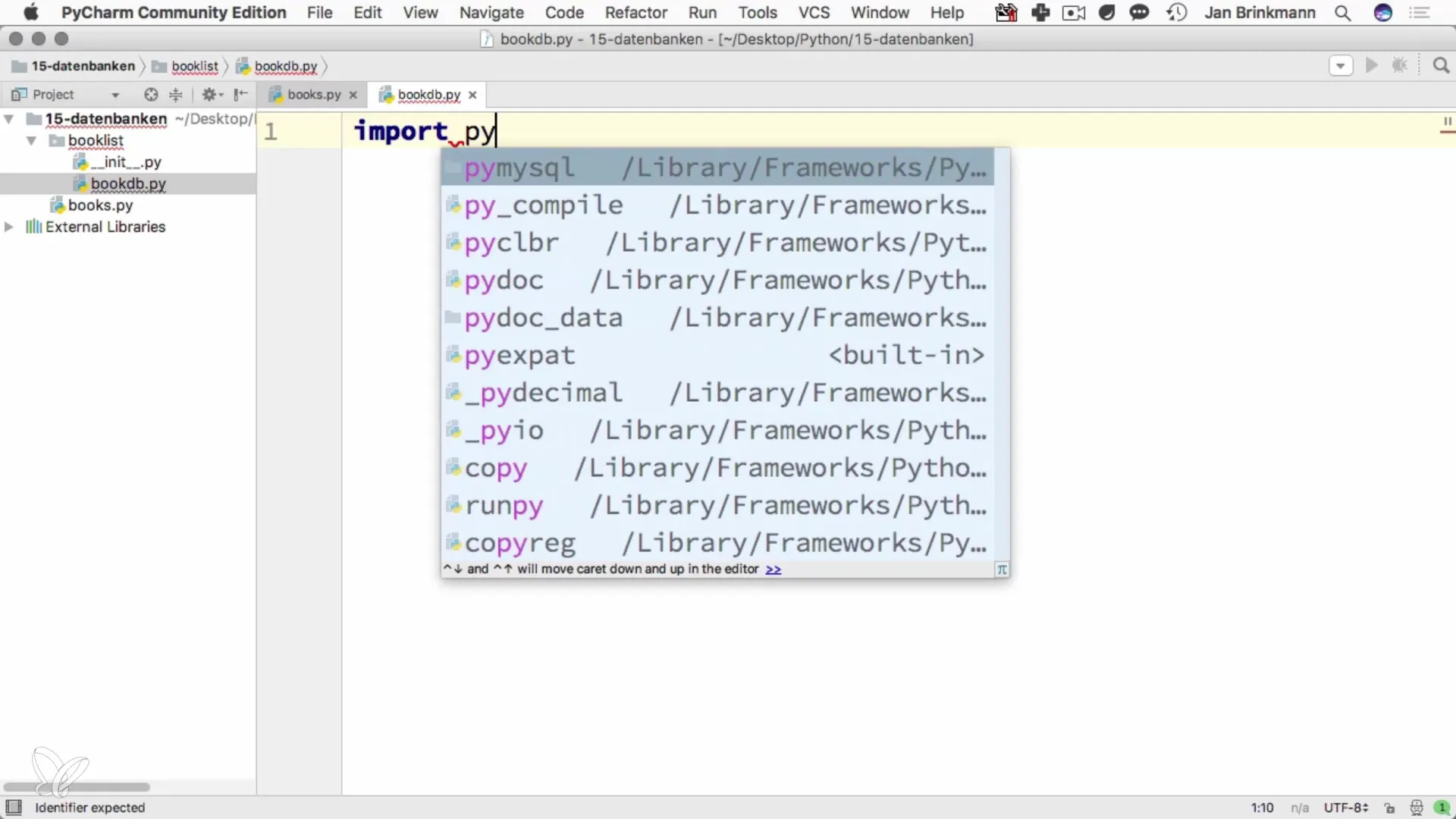
Step 5: Establishing the Connection
Within your class, you define a method __init__ that gets automatically called when an instance is created. In this method, you implement the logic to connect to the database. The parameters you need are the username, password, and database name.
Here, you can call the connect method to establish the database connection.
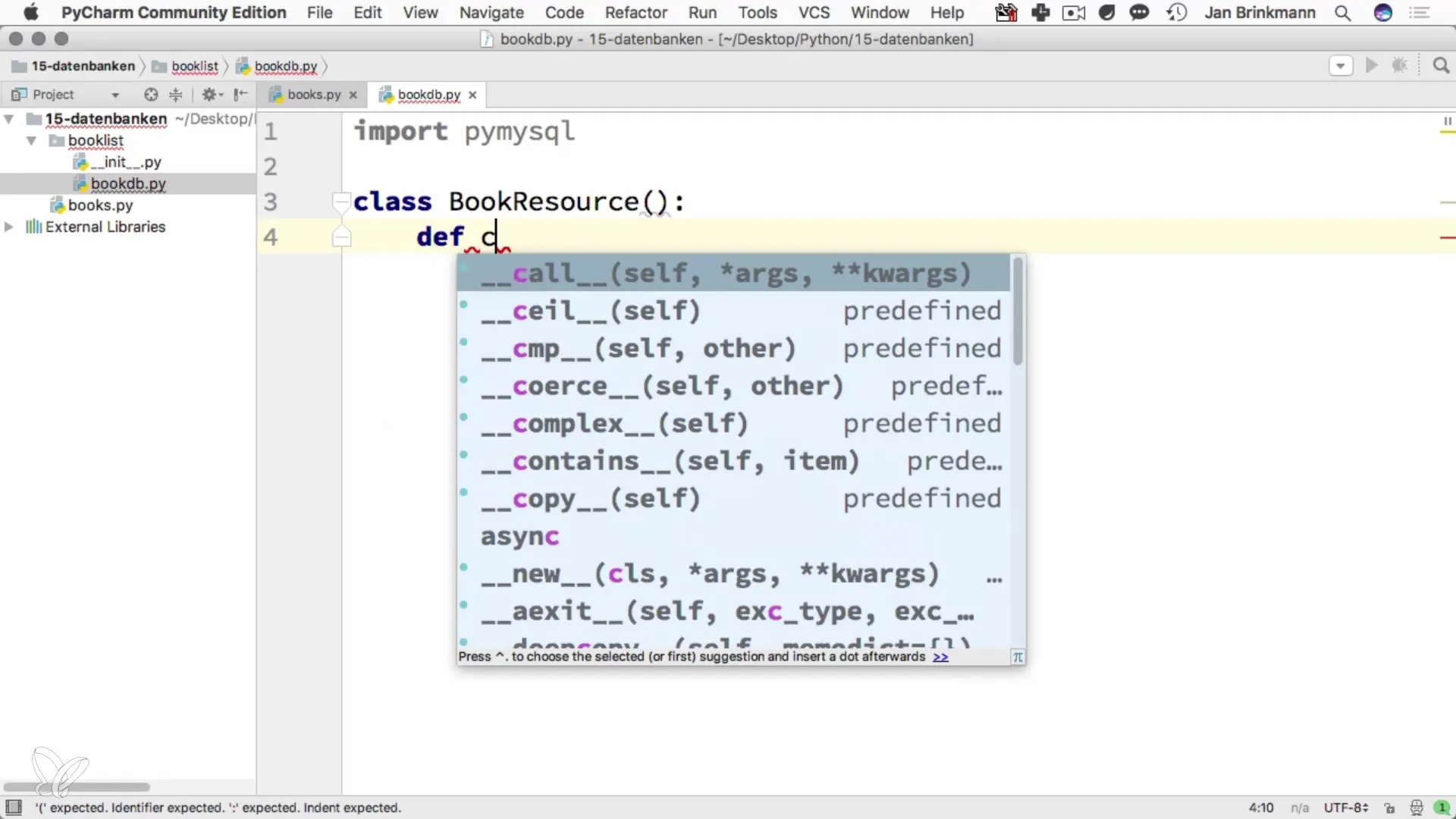
Step 6: Configuring the Connection Parameters
Use the mysql.connector.connect() method to set the connection parameters. In this method, you pass the host, username, password, and database name.
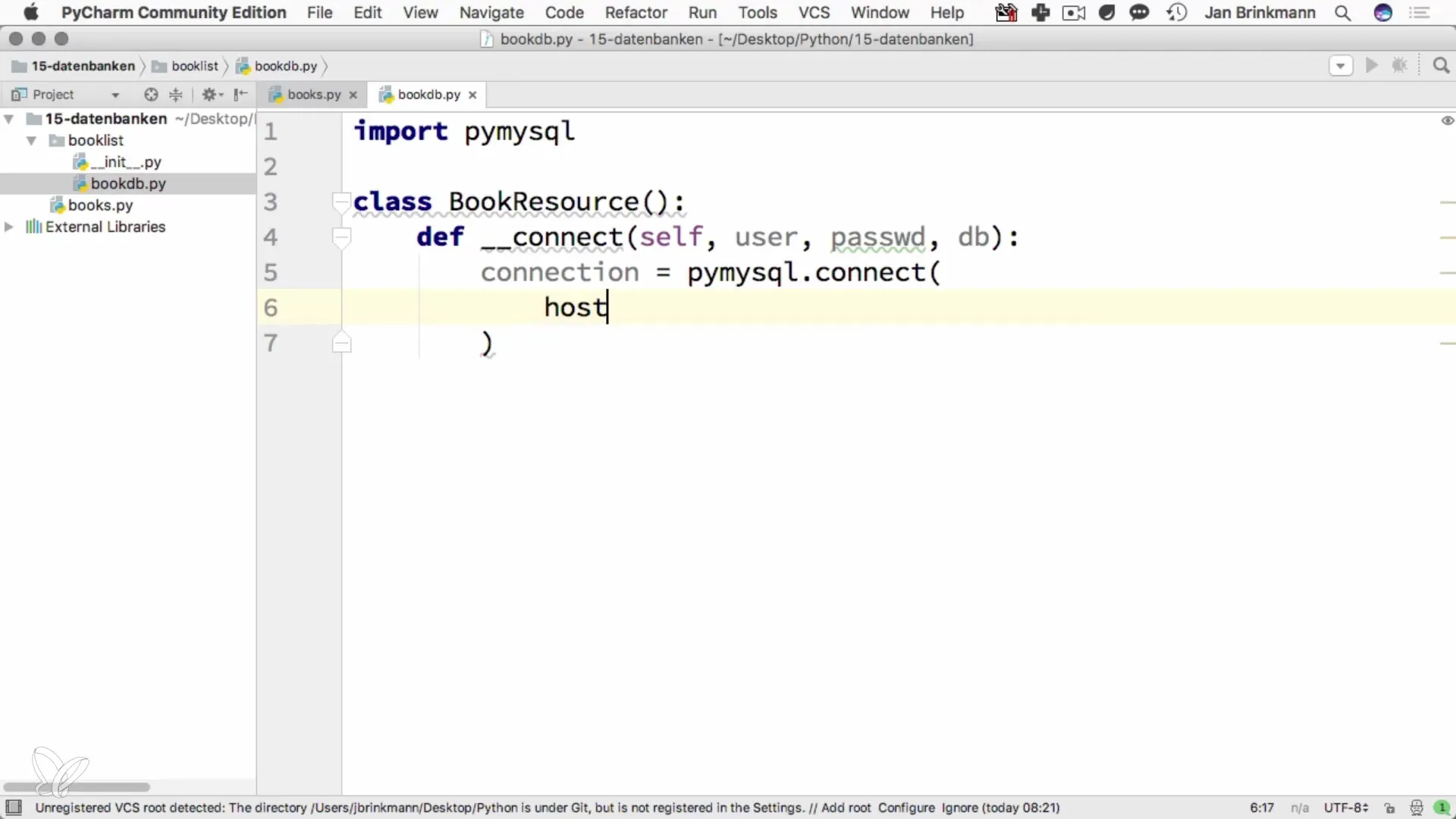
Step 7: Implementing the Cursor
To execute SQL statements, you need a cursor. This is derived from the existing connection. You can simply create it using the cursor() method of your connection.
Now you can execute SQL commands with the cursor.
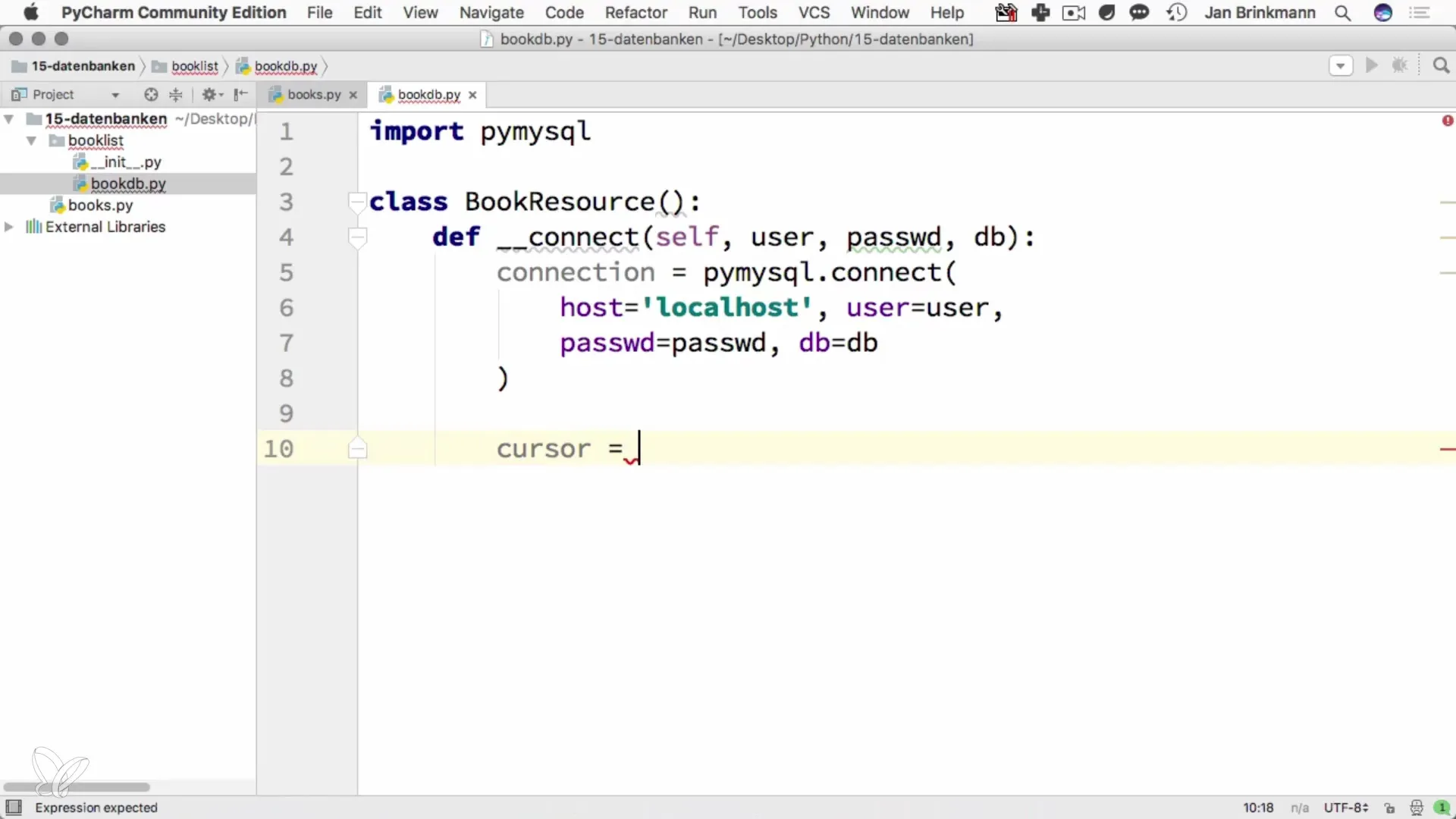
Step 8: Selecting the Database
To work with a specific database, use the SQL keyword USE. Set this in your method to select the correct database. This ensures that all subsequent SQL queries are executed in the right place.
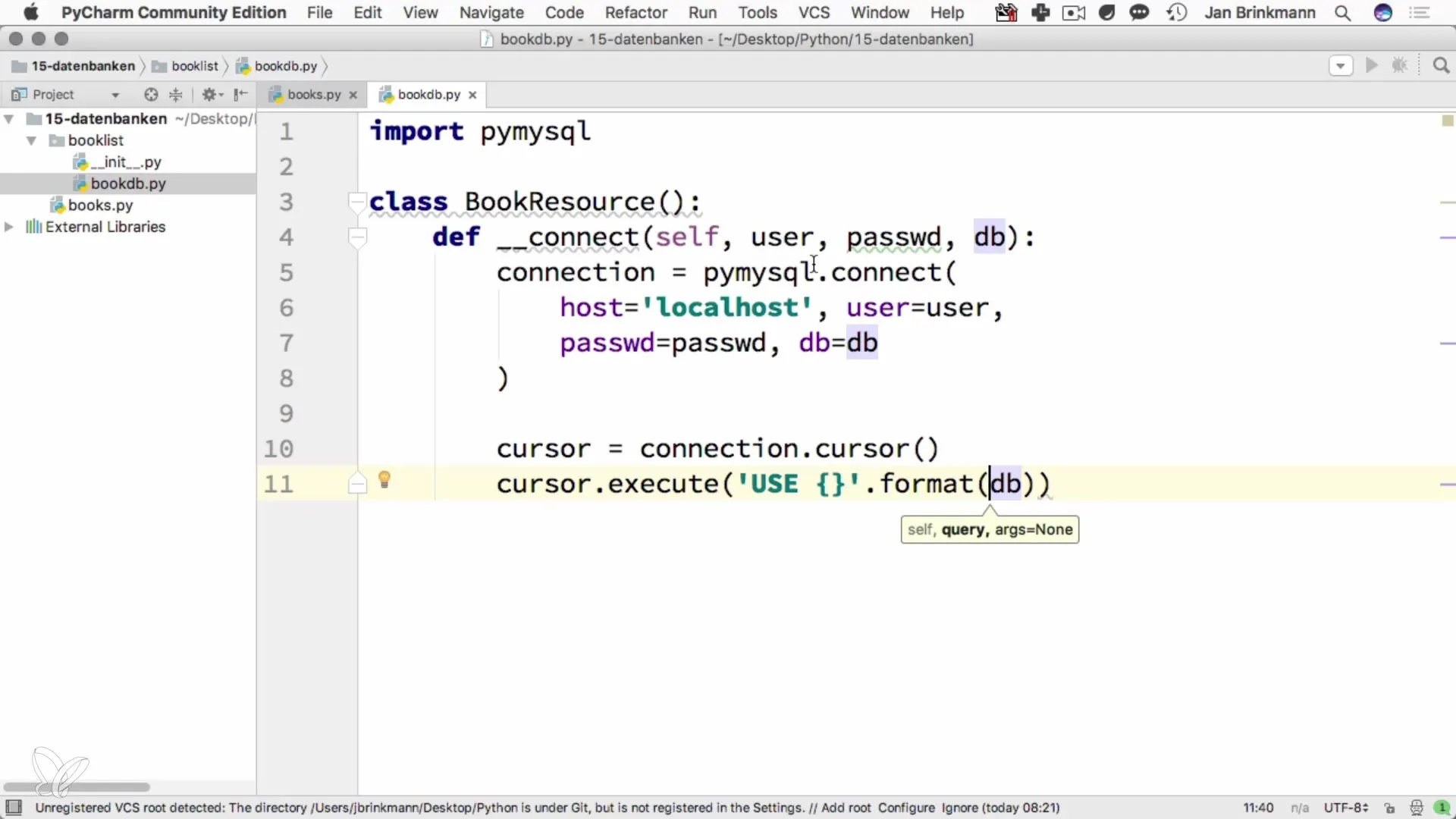
Step 9: Testing the Connection
Finally, you test the connection to your MySQL database. You can achieve this by simply querying a minimal dataset or executing a test INSERT statement. This ensures that all components work together.
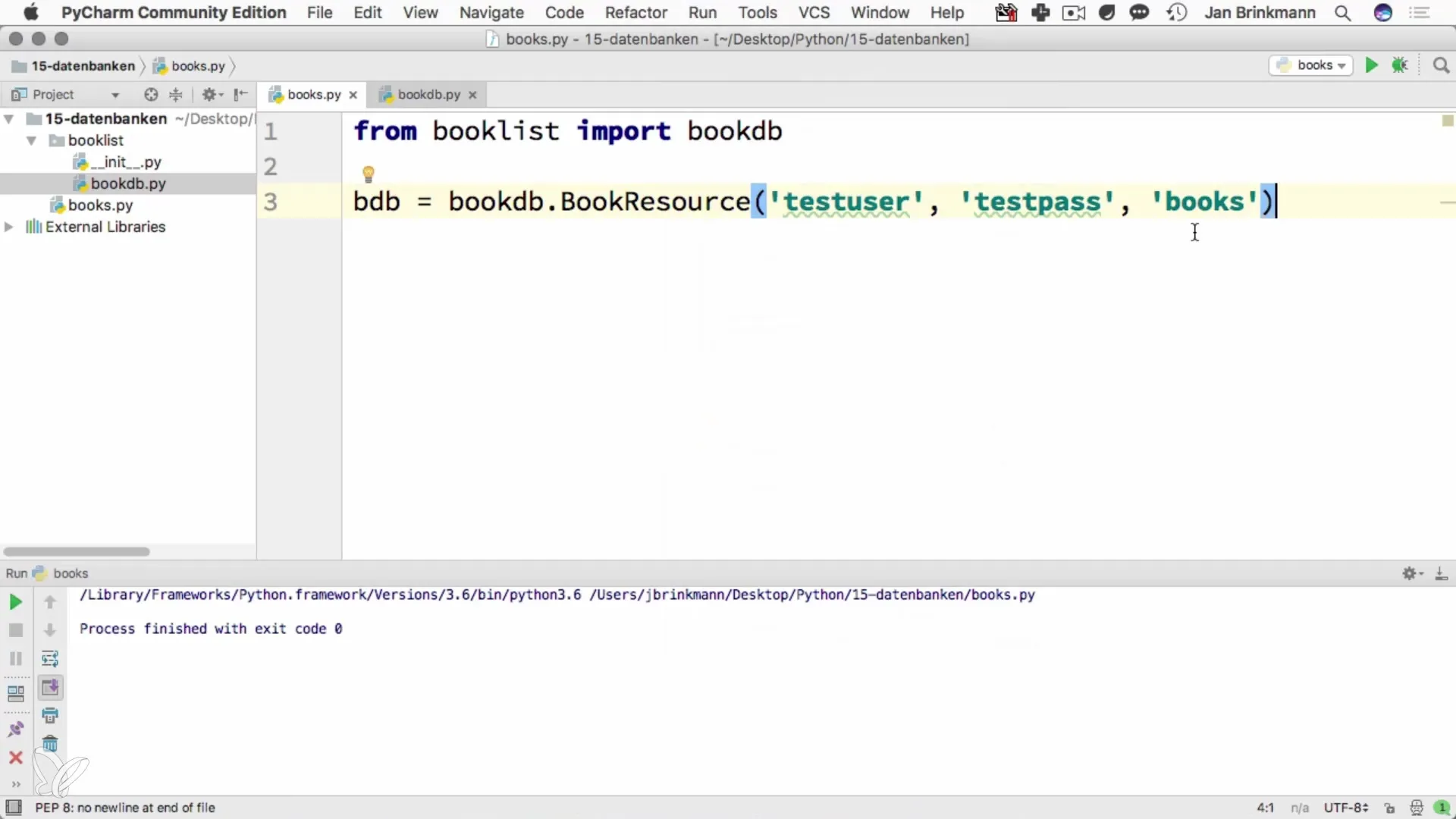
Summary - Establishing a Connection from Python to MySQL
In this guide, you learned how to establish a connection between Python and MySQL. You learned the necessary steps for installing the MySQL connectors, creating a project structure, and implementing a database class. With these basics, you can now develop further functions such as inserting and retrieving data.
Frequently Asked Questions
What prerequisites do I need for the MySQL connection?You need Python, pip, and a functioning MySQL database.
How can I uninstall the MySQL Connector?Run the command pip uninstall mysql-connector-python in the terminal.
How do I test if the connection was successful?You can execute simple SQL commands and check for errors.
How can I start querying data?Use the cursor to execute SELECT statements and retrieve data.