Sets are an essential concept in programming, based on the mathematical definition of sets. This data structure allows you to store unique elements and perform various mathematical operations. In this guide, we will show you how to work with sets in Python so you can take full advantage of this structure in your applications.
Key Insights
- A set is a collection of unique elements and can generally store any data types.
- There are basic operations like adding, removing, union, intersection, and difference that facilitate working with sets.
- Sets provide an easy way to check if an element exists.
Step-by-Step Guide
To use sets in Python, you should first learn the basic operations. Here are the steps:
Creating a Set
To create a set, you can use curly brackets or the set() function. You can also create empty sets.
Set with Values
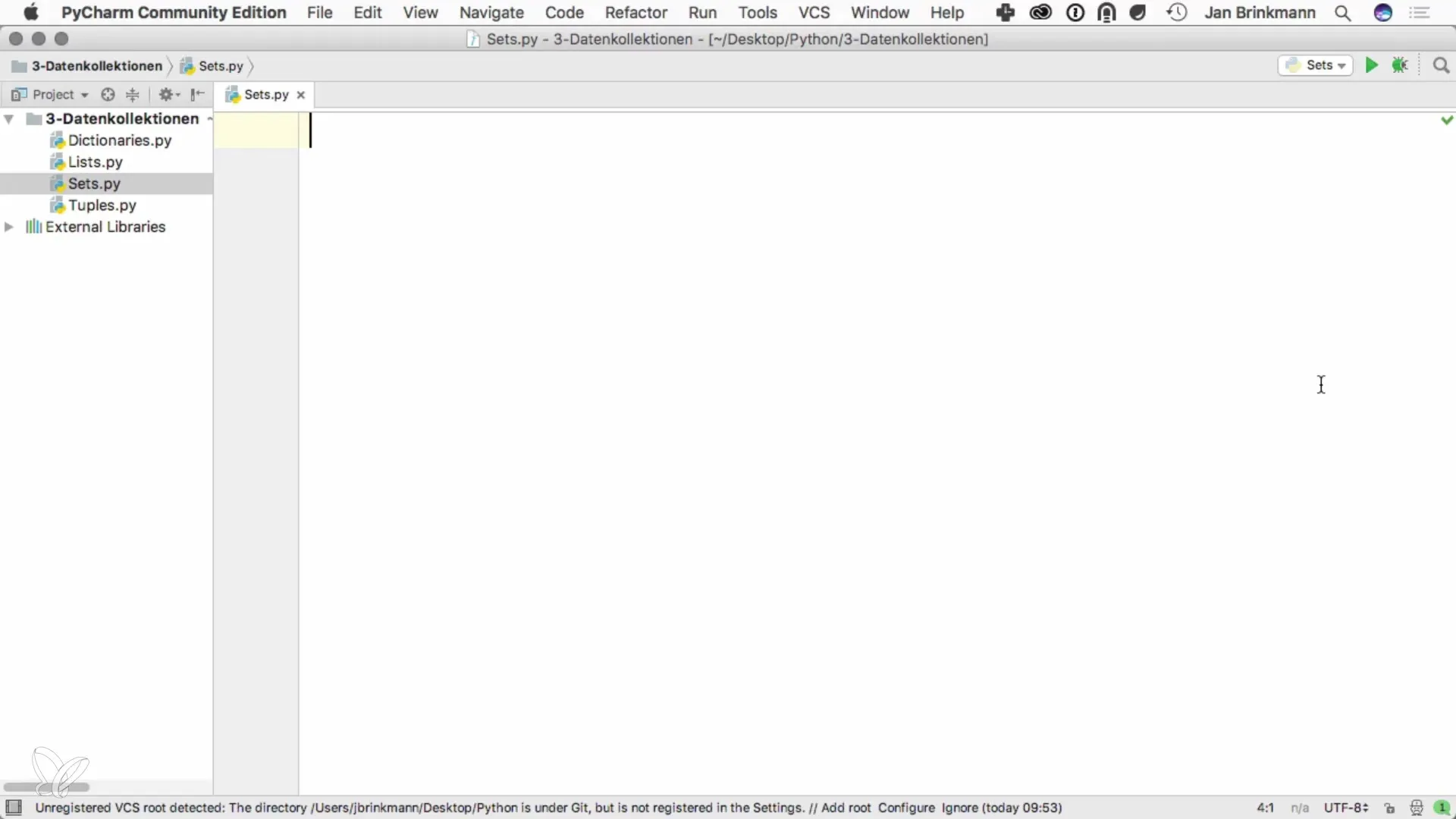
Adding Elements
To add elements to your set, you use the add() method. Note that if you add a value that is already in the set, you will not get duplicates.
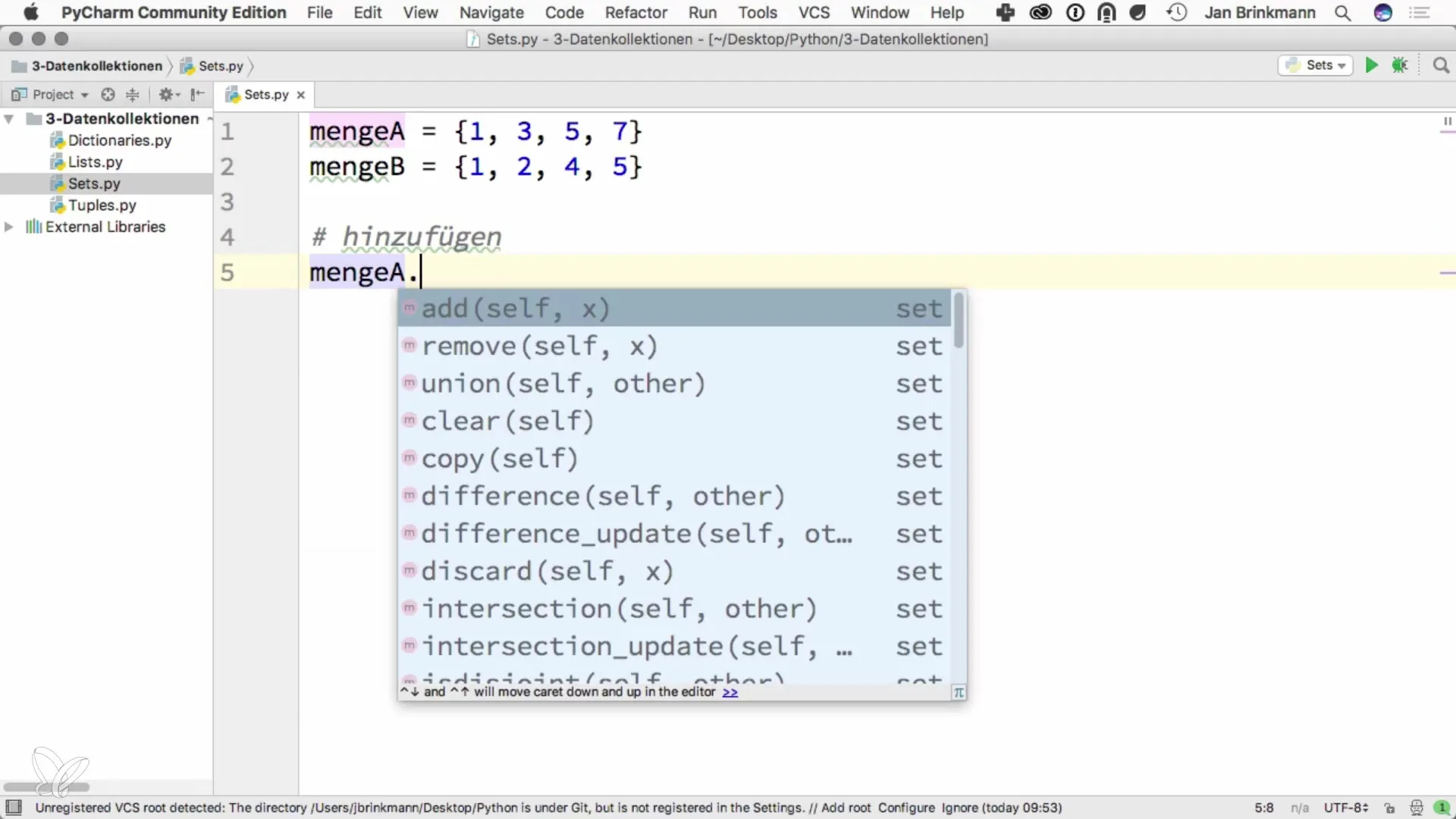
Removing Elements
You can remove elements from your set using the remove() method. If the element is not present in the set, this method will throw an error.
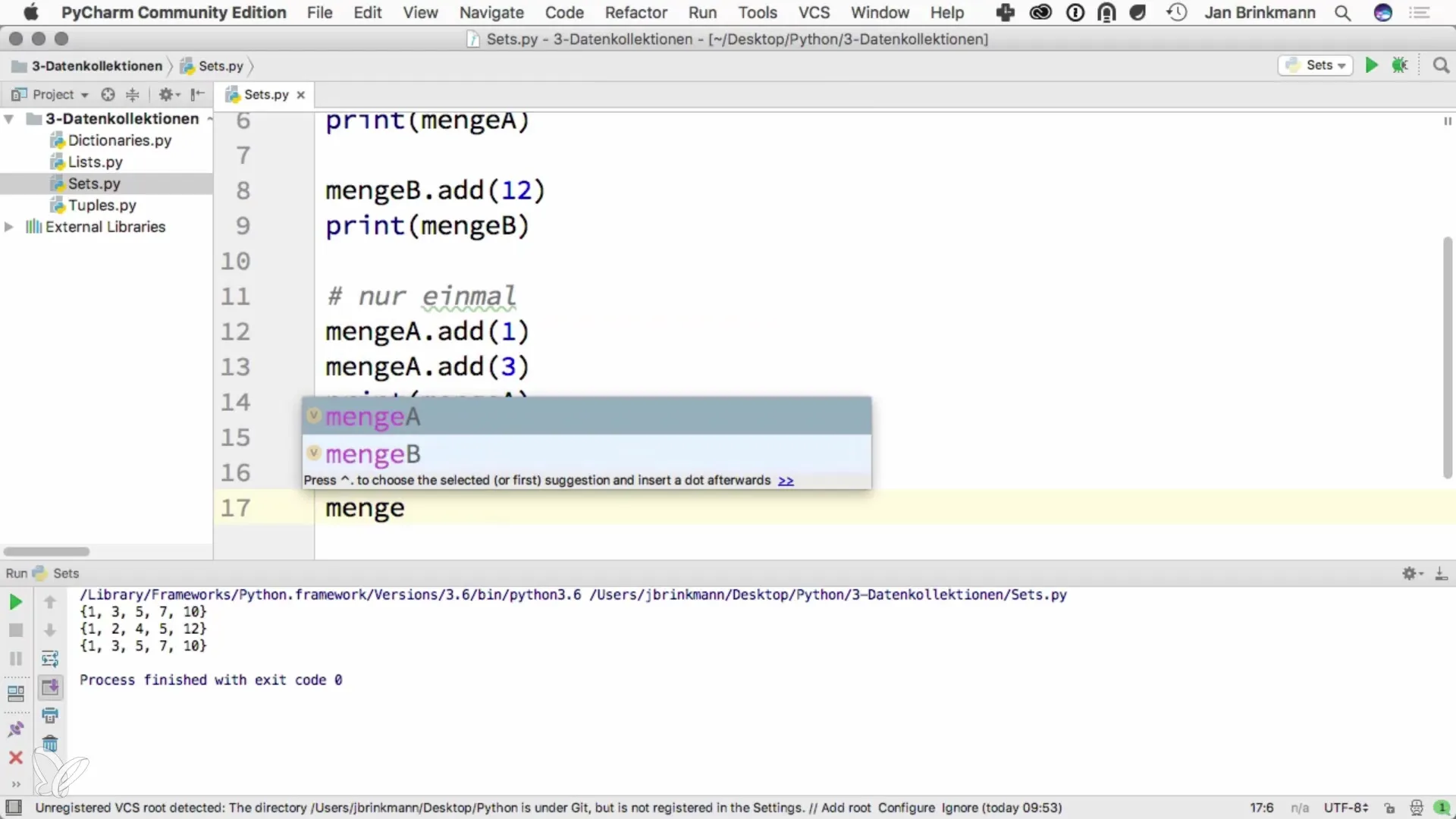
Checking if an Element Exists
You can check if a certain value is contained in your set by using the in operator.
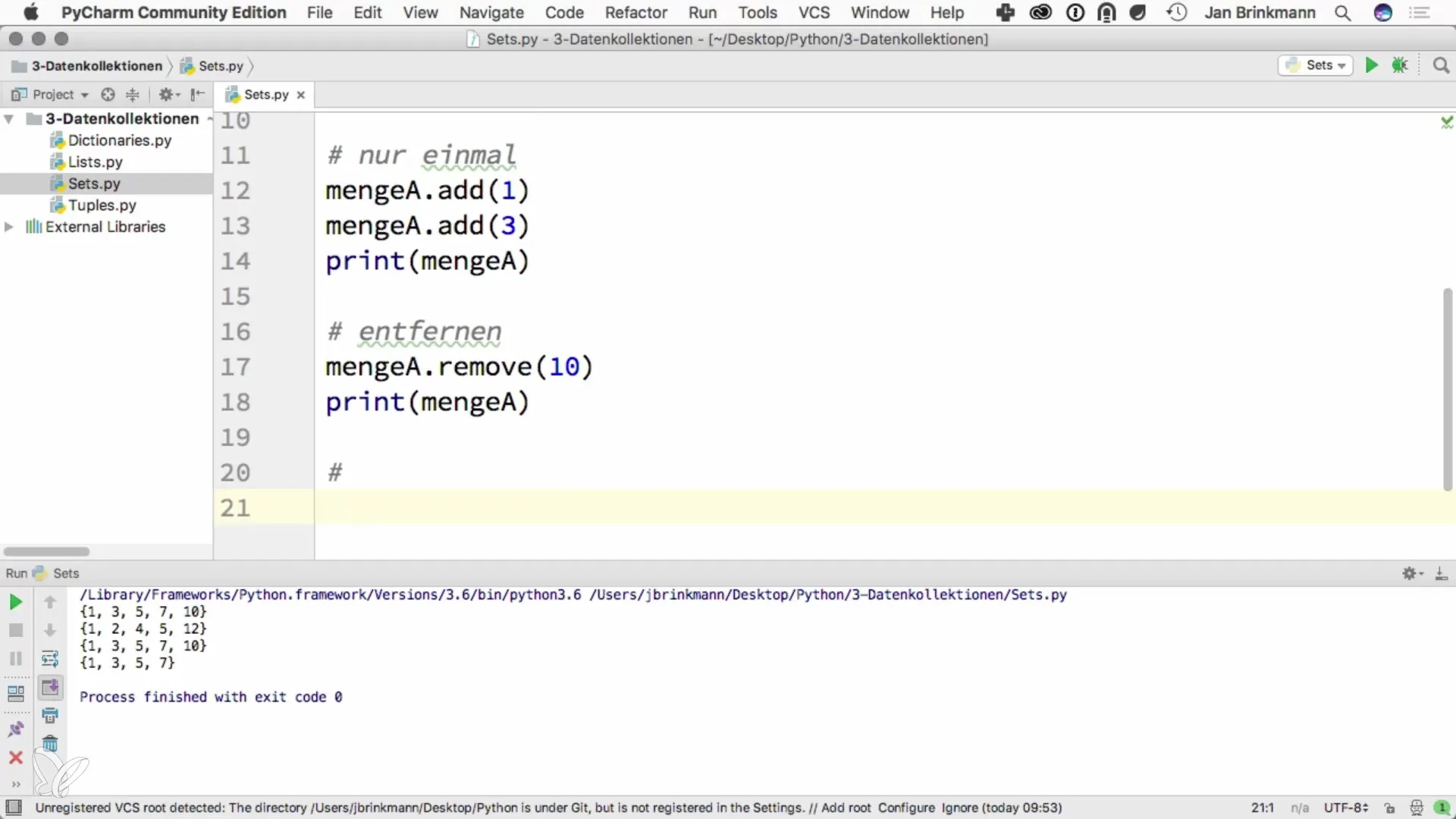
Union Set
The union set is a fundamental operation that combines all elements from two sets. You can use the union() method or the | operator for this.
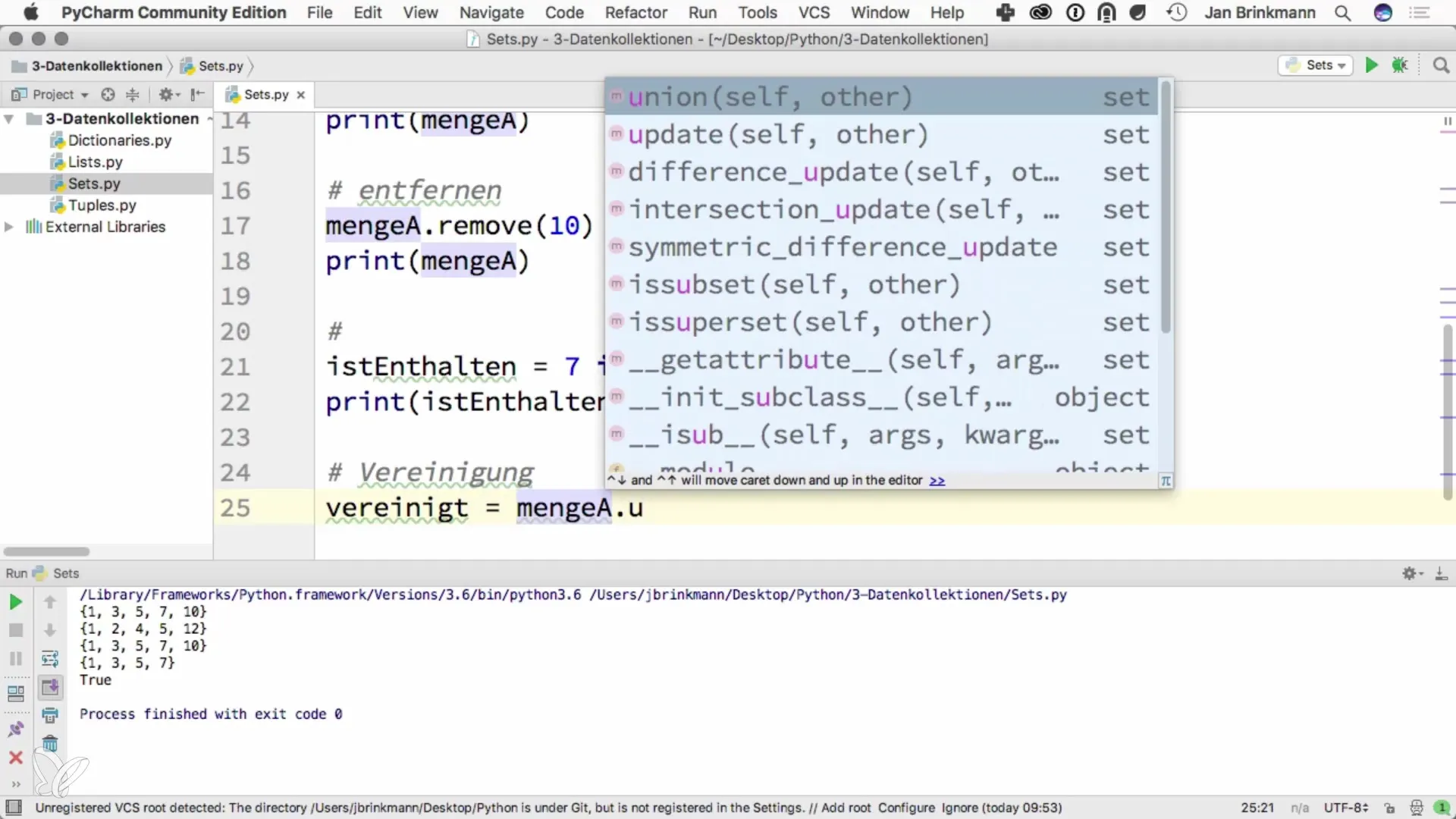
Intersection
The intersection identifies the common elements of two sets. You can use the intersection() method or the & operator for this.
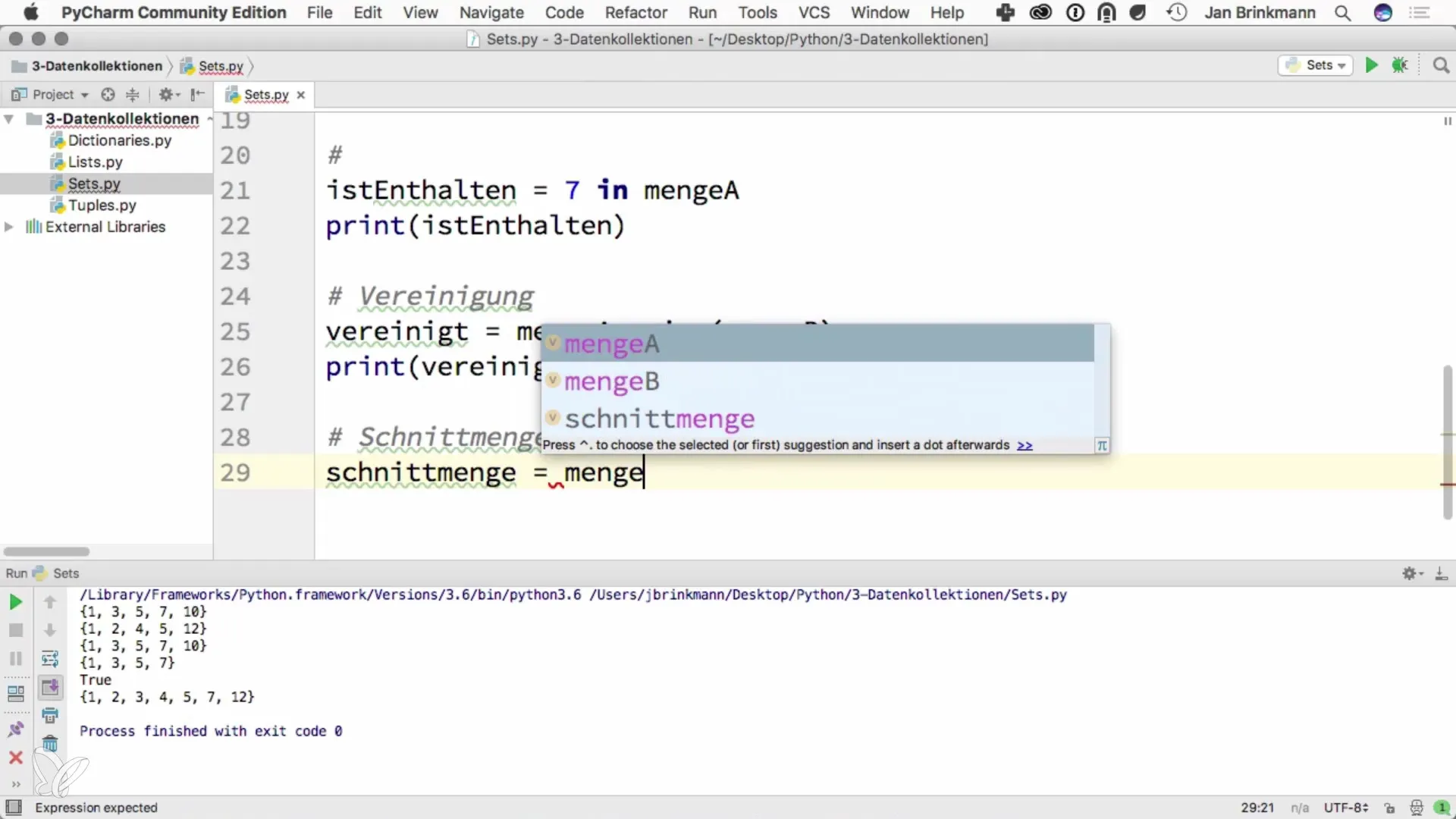
Difference Set
The difference set shows the elements from one set that are not in the other. This is achieved using the difference() method or the - operator.
Additional Operations
In addition to the operations mentioned above, there are many other mathematical possibilities for working with sets, including:
- Symmetric difference (symmetric_difference())
- Subset check (issubset())
These are less commonly used but are very useful in certain applications.
Reference: It is advisable to consult the official Python documentation to learn more about all available set operations.
Summary – Programming with Python – Working with Sets
Sets in Python are a powerful tool that allows for easy handling of unique elements and a variety of mathematical operations. With the capability to add, remove, and determine both the union and intersection through various operations, you have the opportunity to effectively work with data in your programs.
Frequently Asked Questions
What is a set in Python?A set is a collection of unique elements used in Python.
How do I add an element to a set?Use the add() method.
What happens if I add an already existing element?The set remains unchanged as duplicates are not allowed.
How can I calculate the intersection of two sets?Use the intersection() method or the & operator.
What is the difference between union and difference?Union combines all elements of both sets, while difference shows the elements that are in one set but not in the other.