The understanding of strings is fundamental for anyone programming with Python. These character sequences allow you to store and manipulate text. In this guide, you will learn how to effectively use strings in Python, including creation, nesting, handling special characters, and concatenating strings.
Key Insights
- Strings can be created using single or double quotes.
- The use of escape characters allows for handling special characters within strings.
- Multiline strings can be written using special syntax.
- Strings can be combined through concatenation.
Step-by-Step Guide
Creating Strings
To create a string, you can simply place text in double quotes. For example, to represent your name, simply write:
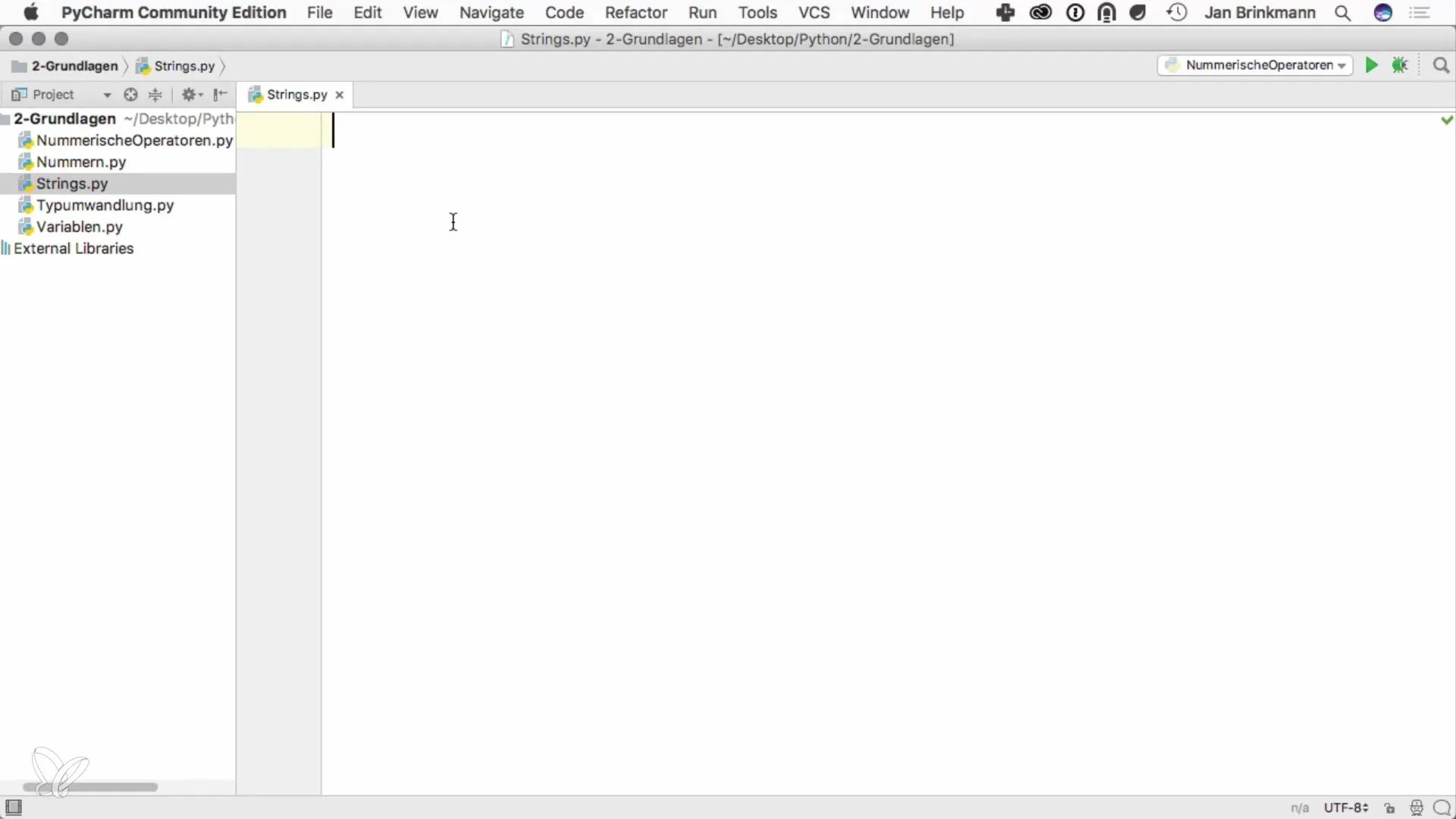
Similarly, you can also use single quotes. Both variants essentially have the same meaning but using single quotes helps avoid conflicts with double quotes when you want to use nested strings.
Nesting Strings
If you want to use a string within another string, you can cleverly combine the quotes. For example, if you want to create an HTML link with a nested string:
Here you will see that the problem arises when the inner string is not defined correctly.
A simple trick is to use single quotes for the inner string. This allows you to work seamlessly within the outer string.
Escape Characters
Sometimes you need to insert special characters into your text, such as quotes themselves. For this, we use an escape character, which in Python is the backslash (\).
For example, if you want to represent quotes within a string, write it like this:
Additionally, you can also create line breaks using the escape sequence \n. This can be useful when you want to produce formatted output.
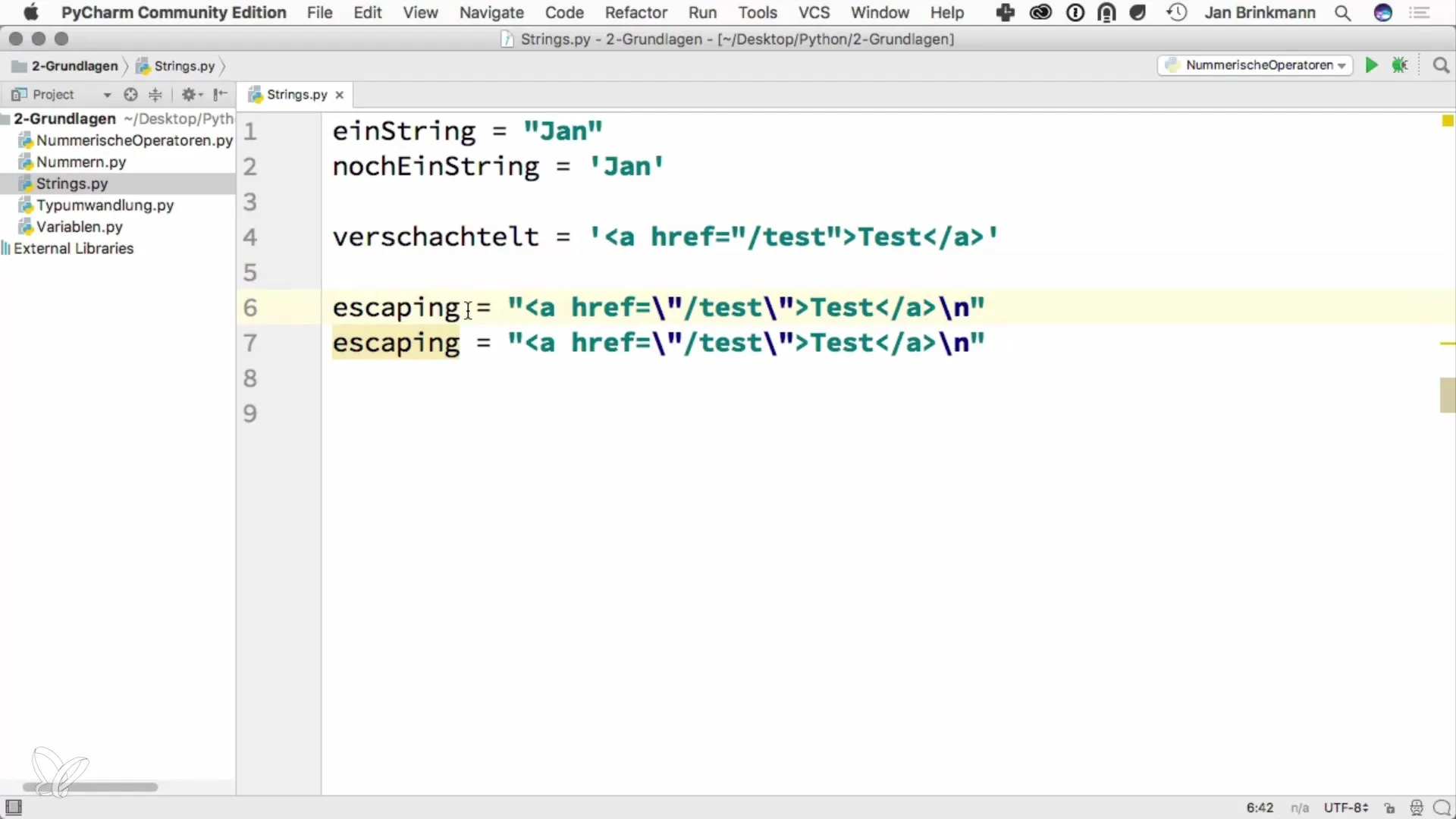
Multiline Strings
For multiline strings, there is also the option to use triple quotes (''' or """). With these, you can enter text over multiple lines without needing to use escape characters.
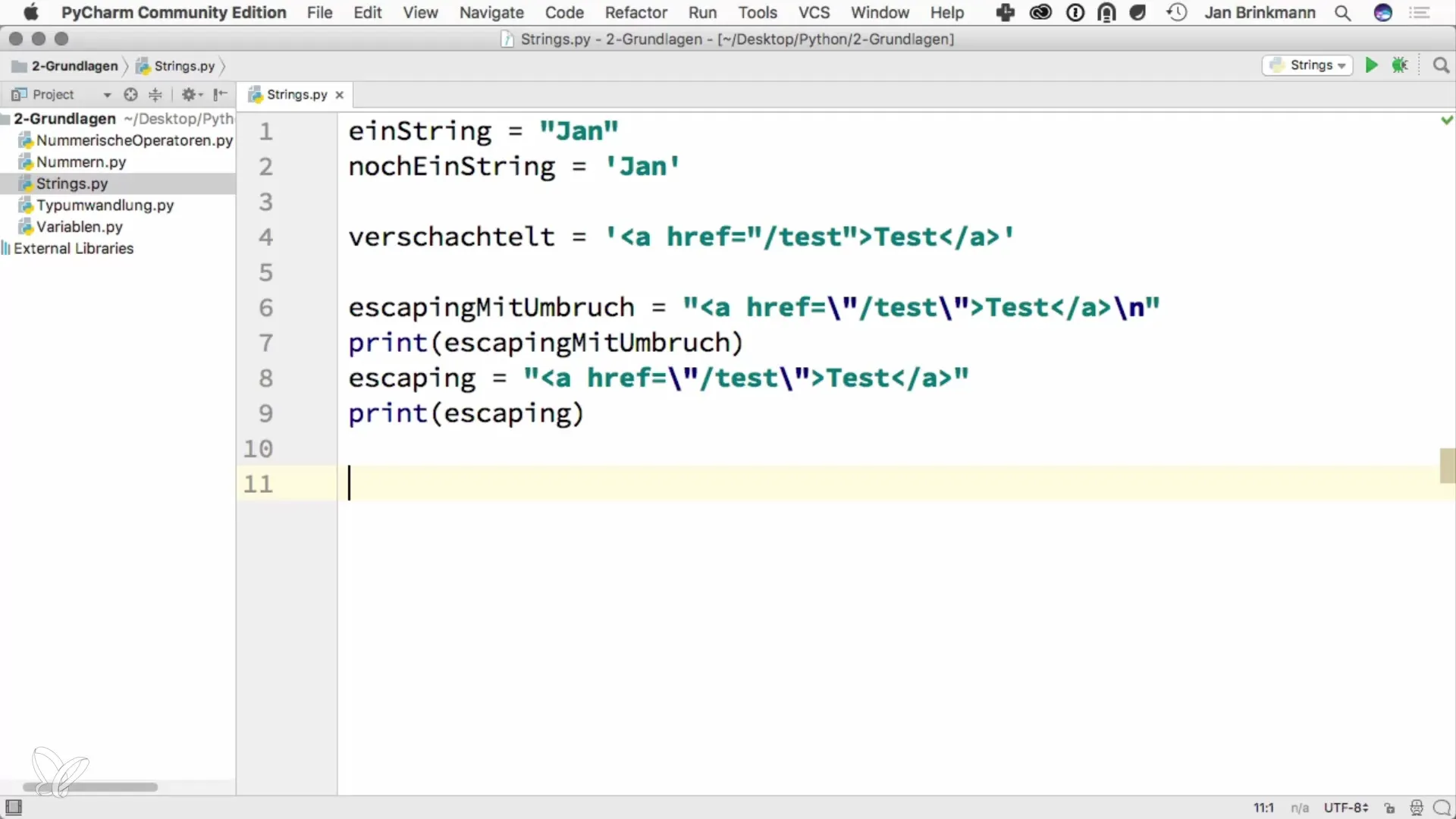
If you insert a multiline string without this syntax, Python will show you a syntax error because it expects everything to remain on one line.
Concatenating Strings
A common scenario when working with strings is concatenating multiple strings. This is done using the plus sign (+).
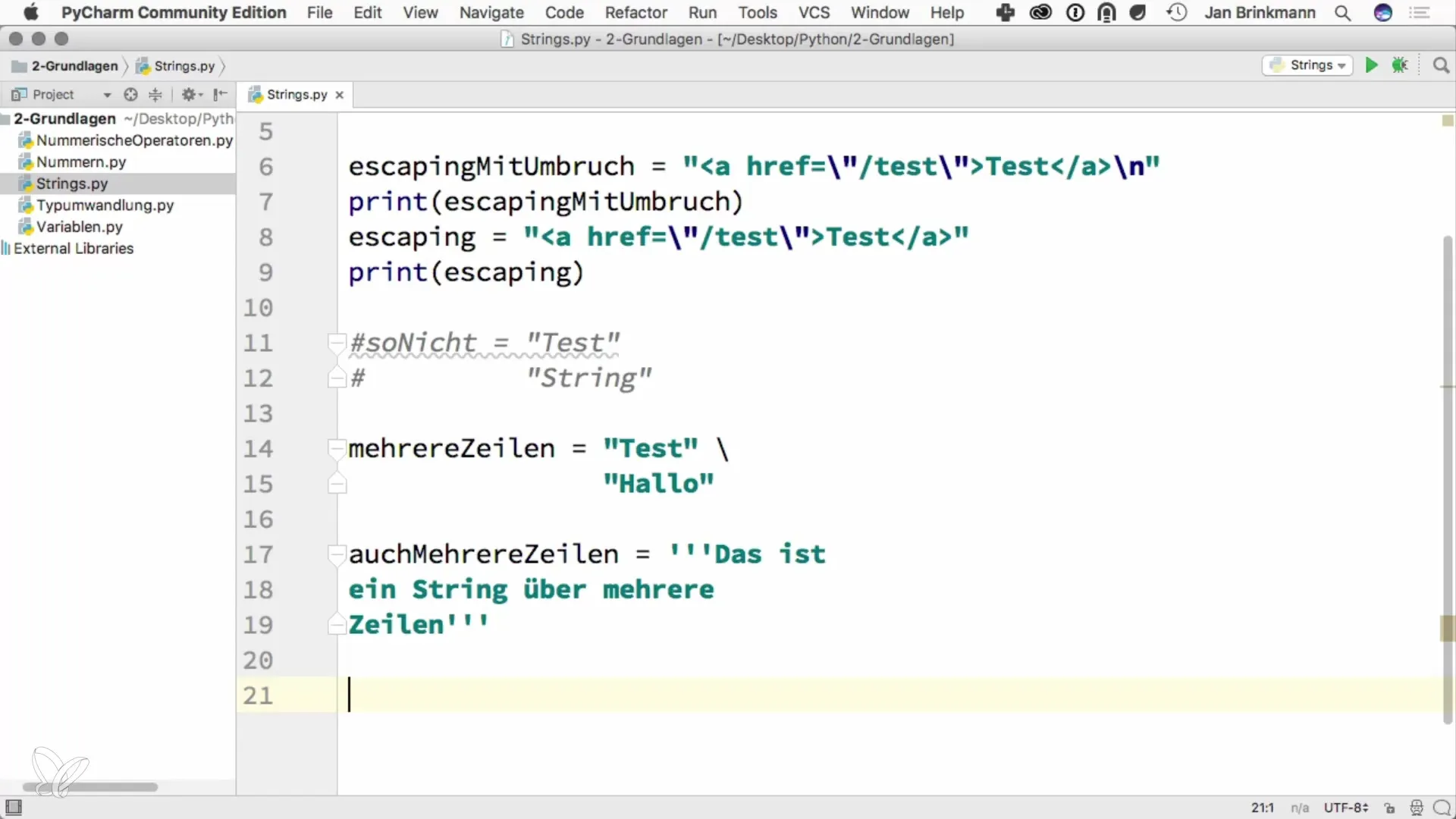
Let’s say you have the strings "Hello" and "World". You can simply combine them:
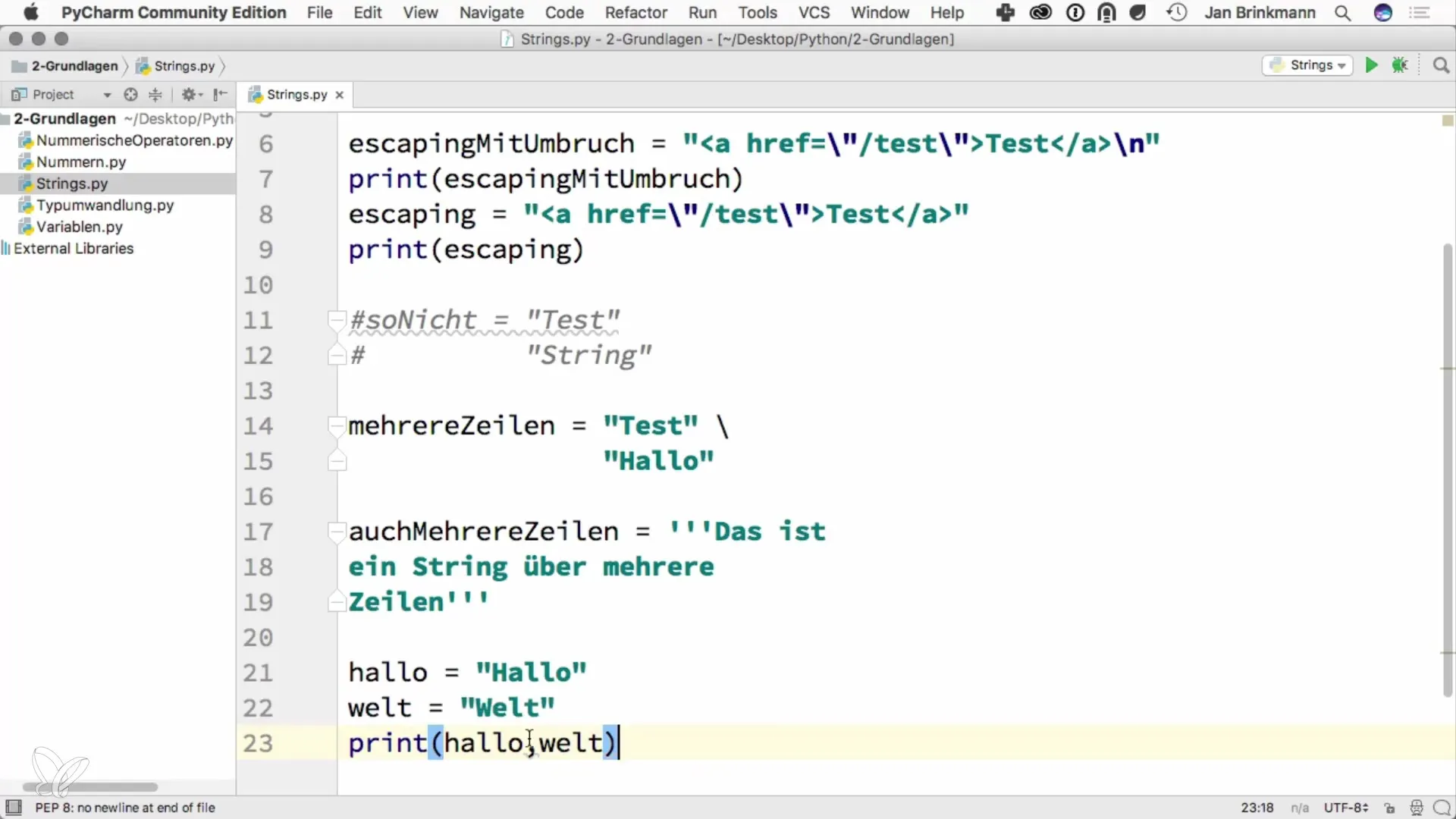
Note that there is no space between the strings. If you want a space, you must explicitly add it.
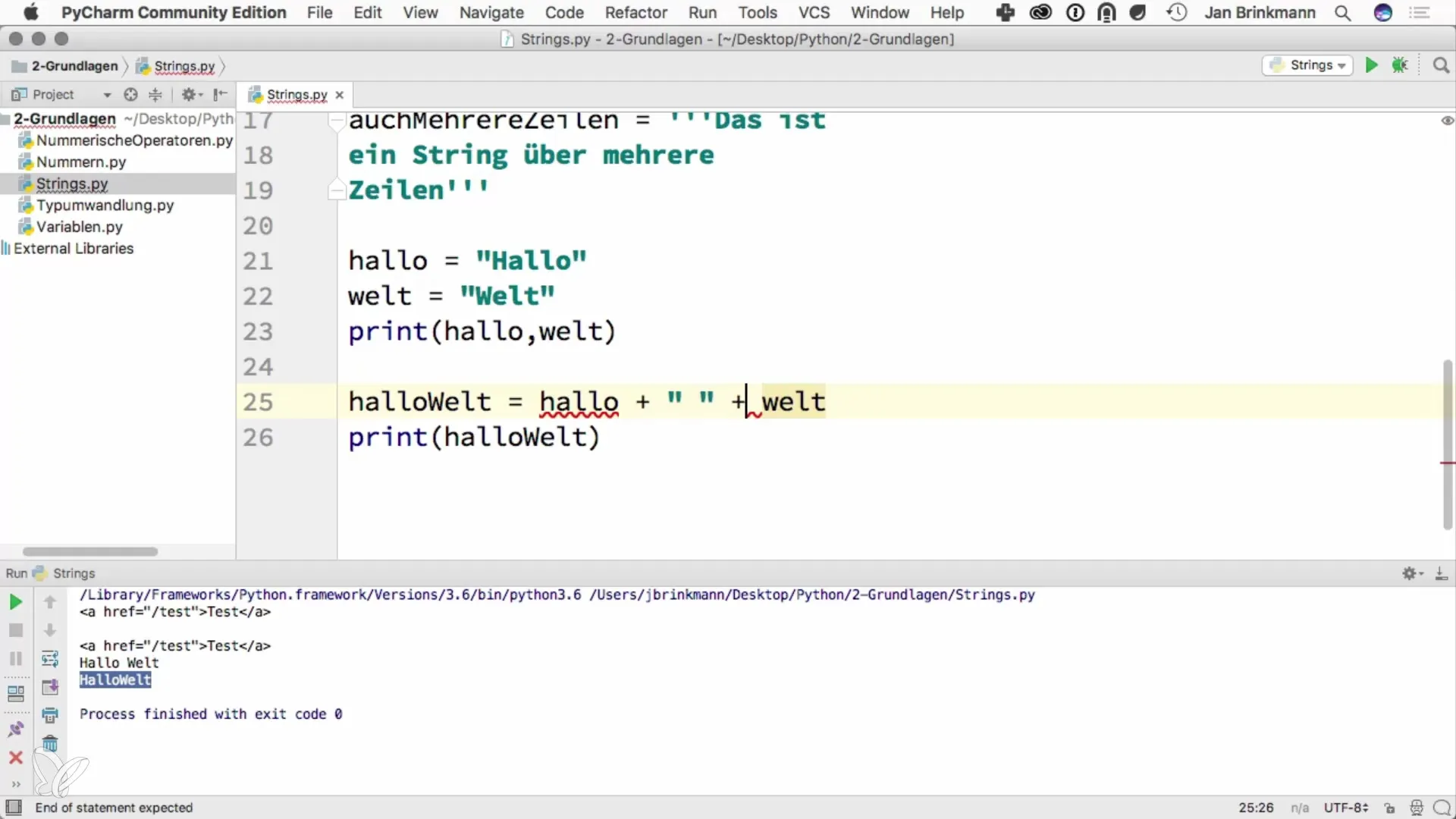
Keep in mind that concatenation creates a new string. Modifications to the existing string do not change the original variable.
Summary – Strings in Python – Basics and Applications
In this guide, you have learned the basics of working with strings in Python. You now know how to create strings, handle special characters, use multiline texts, and concatenate strings. These skills are essential for your programming projects.
Frequently Asked Questions
How do I create a simple string in Python?You can create a string with double or single quotes, e.g., "Hello" or 'Hello'.
What is the purpose of escape characters?Escape characters allow the use of special characters within strings without terminating the string.
How can I use strings over multiple lines?This is done with triple quotes, either ''' or """.
How do I concatenate multiple strings?You can combine strings with the plus sign (+); however, note that no automatic space is added.
How do I output a string with line breaks?Use the escape sequence \n to create desired line breaks.