Overriding methods is a central concept in object-oriented programming that allows you to implement specific behavior for derived classes. Especially in Python, it is important to understand this concept well, as it enables you to reuse and adapt many functionalities of your code through inheritance from parent classes. In this guide, you will learn how to effectively override methods to meet individual requirements in your programs.
Key Insights
- Method overriding allows you to customize functionalities in subclasses.
- It is important to pay attention to overriding initializers to avoid redundancy.
- A good understanding of inheritance will help you write efficient and clear code.
Step-by-Step Guide
Let's start with the basics of overriding methods in Python. We will use an example of a simple database connection.
The first thing you need to do is define a base class, e.g., DBAdapter, that describes general database operations. This class could include a connect method to establish a connection to the database.
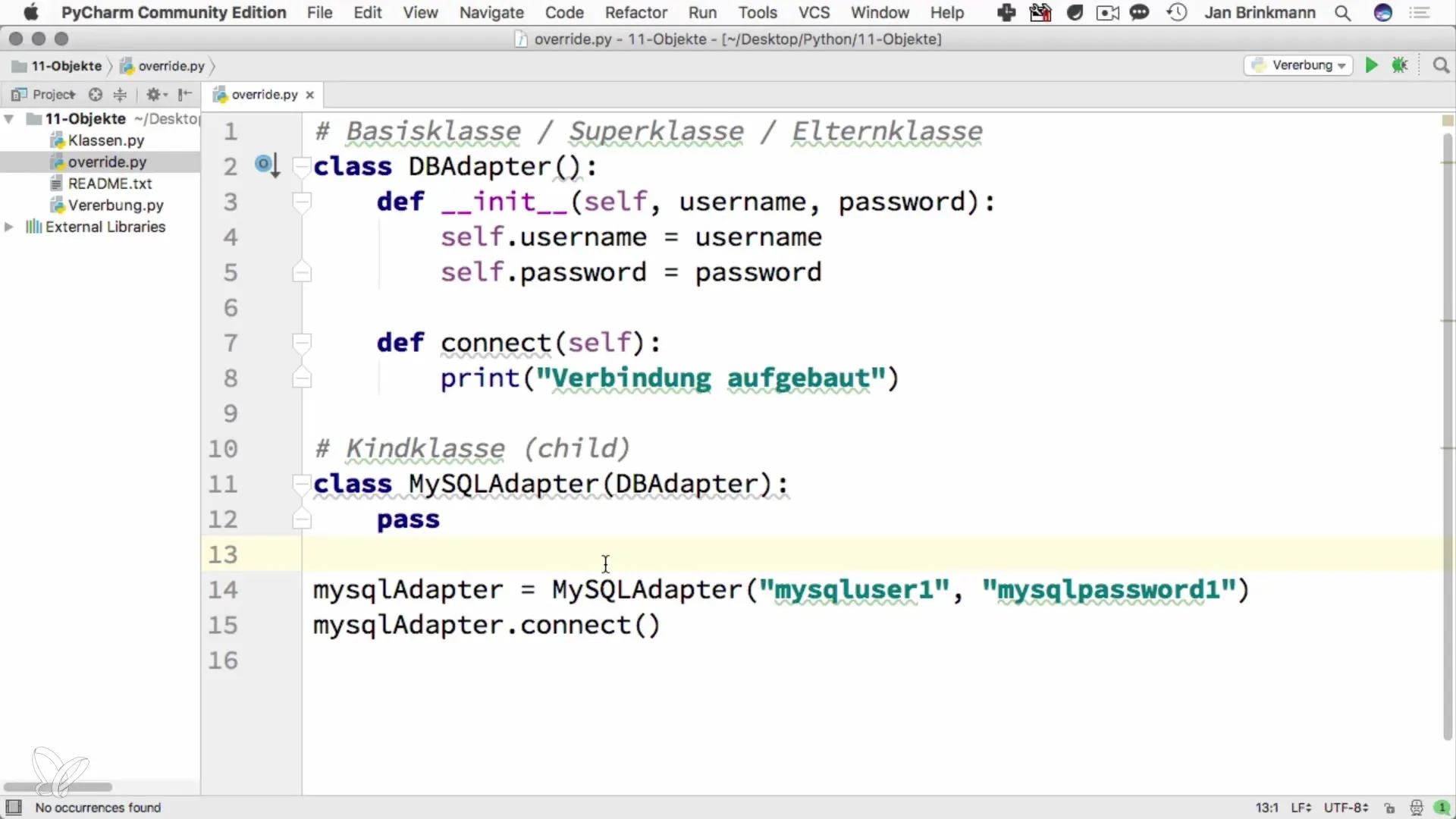
For our specific case, if you are working with MySQL, you would want to create a derived class, e.g., MySQLAdapter, that inherits from DBAdapter. In this class, you will override the connect method to account for specific MySQL connection parameters.
This is where you implement the specific requirements for the MySQL connection. You can reference specific libraries or connection types that are suitable for your database environment. By overriding the connect method, you ensure that the execution is appropriately tailored so that your MySQL connection is established correctly.
After you have overridden the method, it is important to test whether the implementation works correctly. Start the program and check if the MySQL connection is successfully established. In our example, we will see that the connection was successfully made without needing to remain in the base class context.
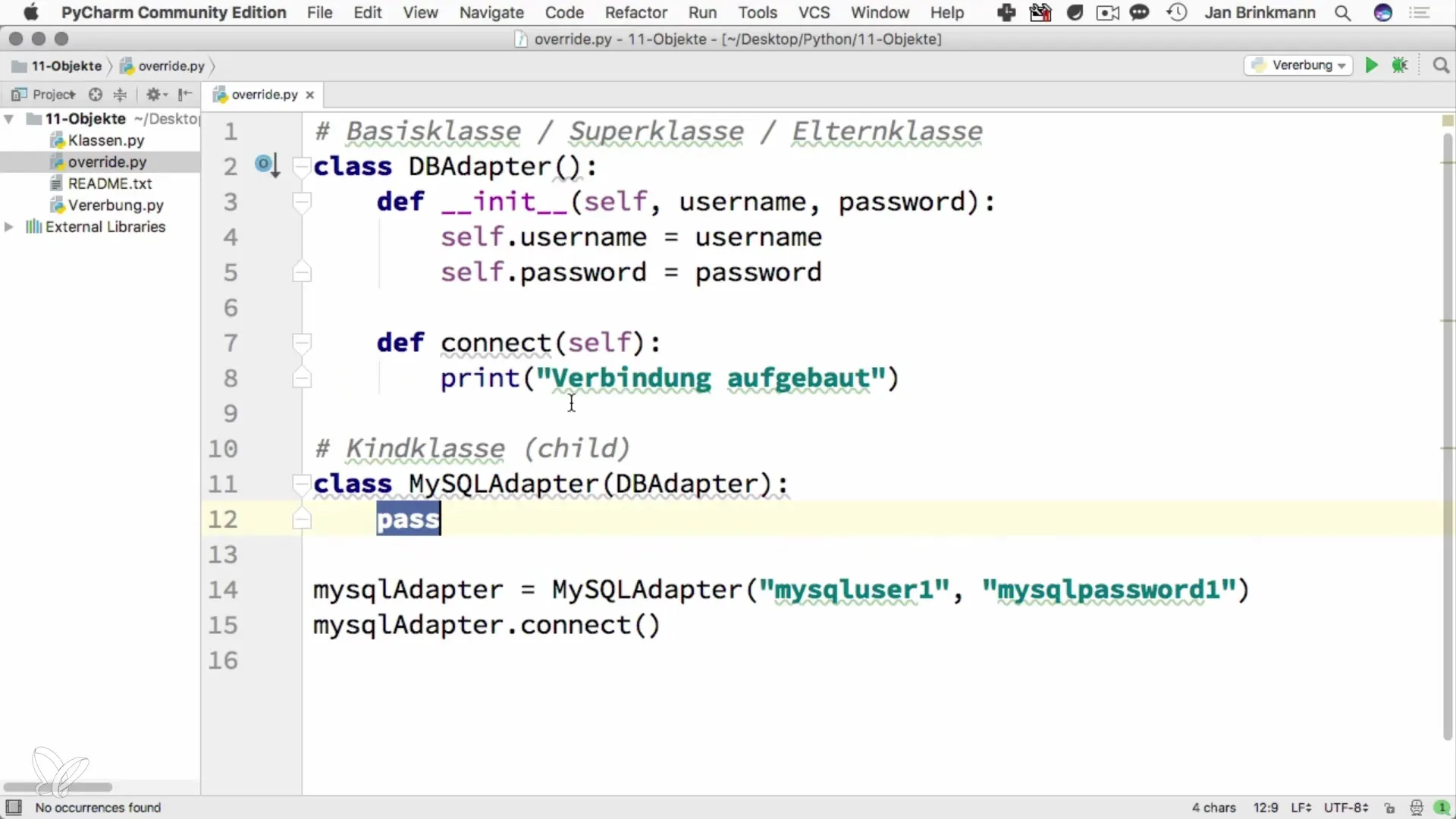
Another important aspect is overriding the initializer (__init__). Suppose your base class DBAdapter passes the username and password. In the MySQLAdapter class, you need to ensure that these parameters are processed in the derived class as well. Implement the initializer so that you pass the parameters and, if necessary, establish the connection directly.
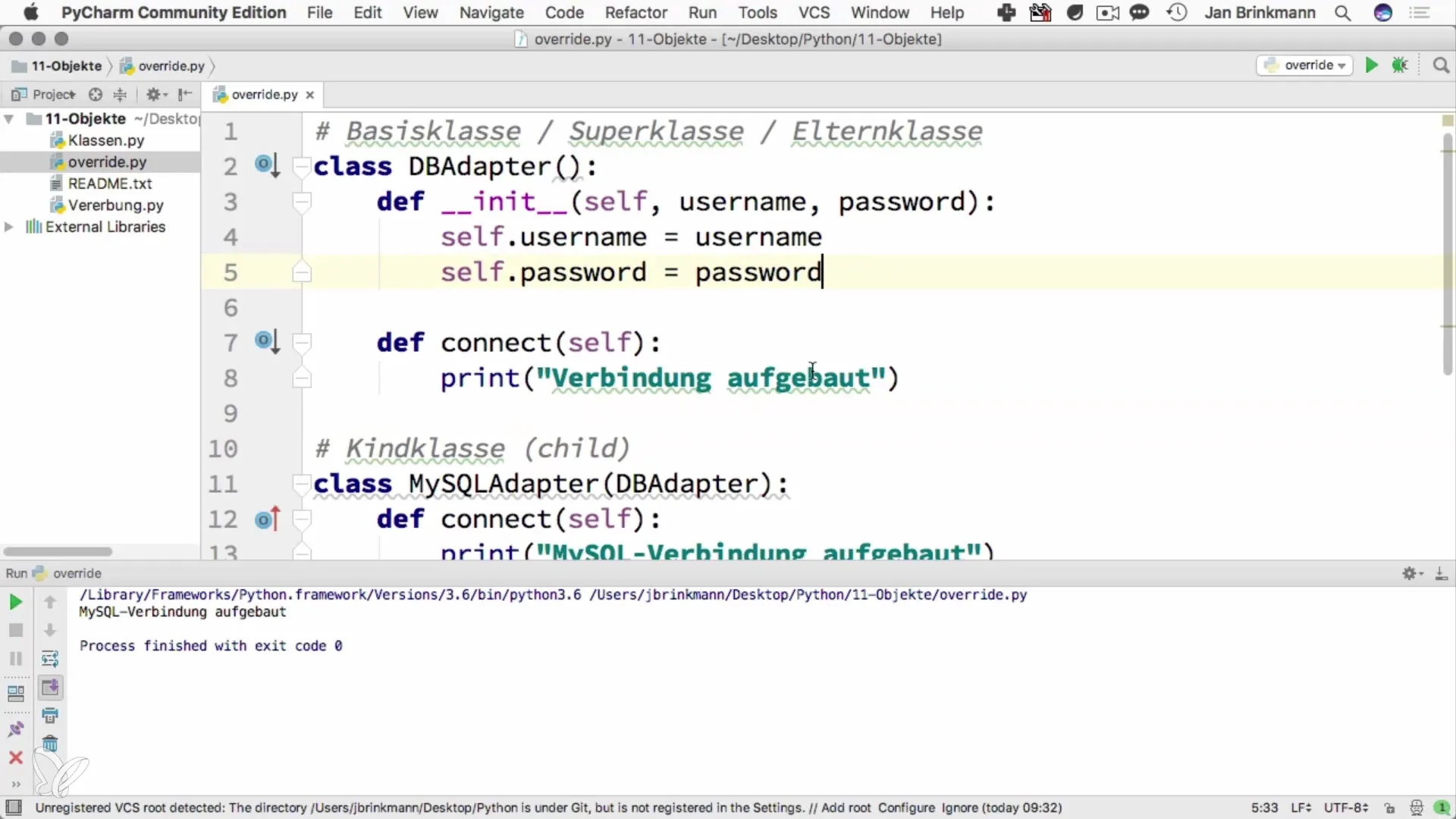
The new initializer would then look like this: You could receive the parameters username and password and call the connect method within the initializer to establish the connection right when creating an object. This saves you the need to call the connection separately. For example:
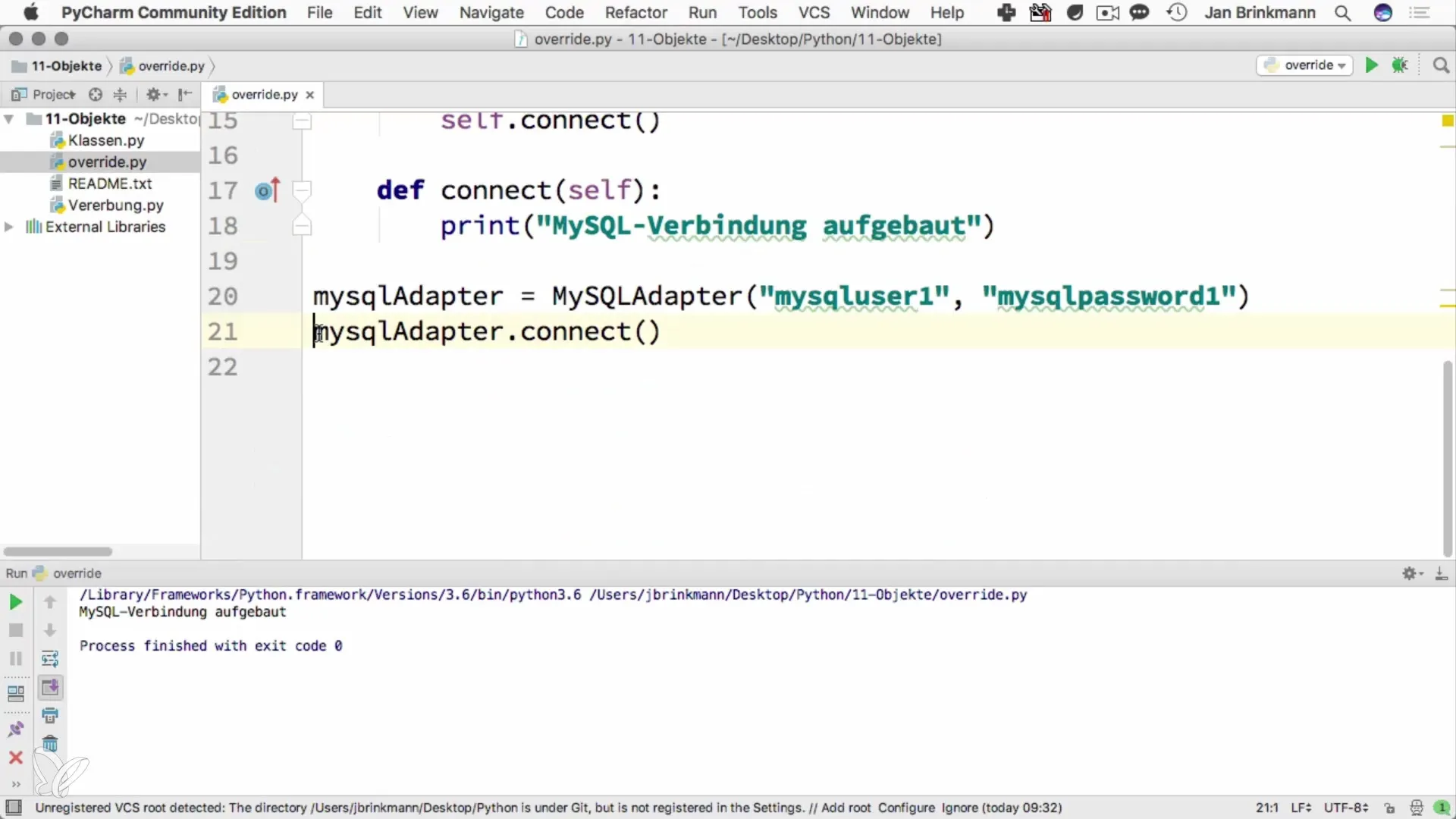
When you run your program again, you will find that the same result as before is achieved, as the new initializer now initializes everything necessary and automatically handles the connection establishment.
Now an important point comes into play: You should watch out for duplications in the code. Sometimes, code sections can become duplicated through copy and paste, which may indicate underlying issues. In such cases, you can consider how to design reusable classes or methods to improve code quality and eliminate redundancies.
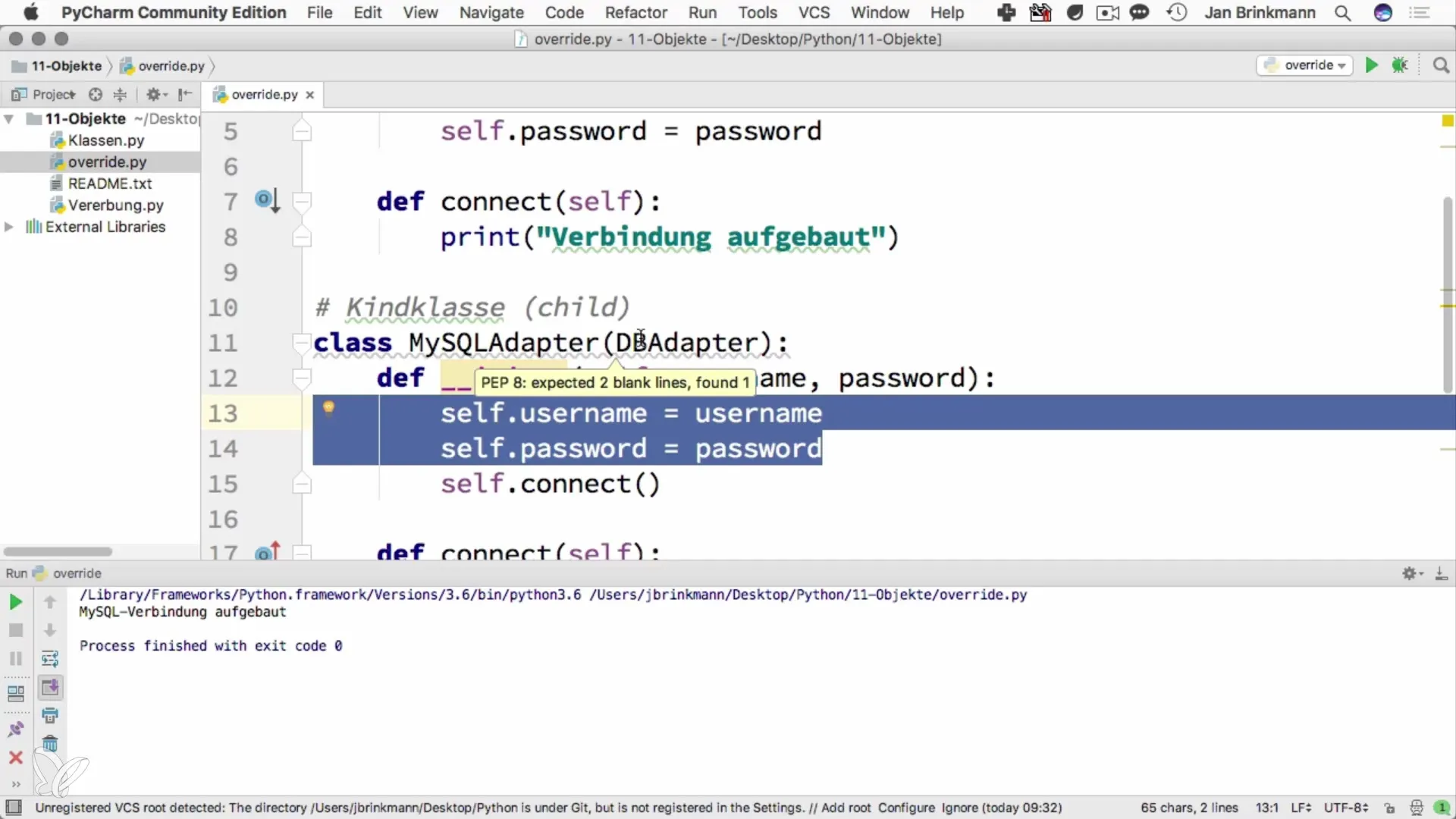
In the next video, you will learn more about solutions to these challenges. But for now, you have worked through the basics of overriding methods in Python and understood how to effectively implement specific requirements.
Summary – Method Overriding in Python
Overriding methods in Python allows you to manipulate the behavior of derived classes to meet specific requirements. By implementing the connect method in a derived class and adjusting the initializer, you can write clear and maintainable programs. Be cautious to avoid redundancies and to utilize reusable code structures.
Frequently Asked Questions
How does method overriding work in Python?Method overriding allows you to change the functionality of a method in a derived class that inherits from a base class.
Why is it important to override the initializer?By overriding the initializer, you can perform specific initializations, such as establishing a database connection directly when creating an object.
What is the danger of code duplication?Code duplicates can affect the maintainability and readability of a program as well as increase the likelihood of errors.
How do I test the overriding of a method?You can run the program and check if the expected behavior occurs, e.g., by creating an object of the derived class and verifying that the connection is successfully established.
Where can I learn more about the topic?In the next video, you will receive additional information and solutions to common issues that may arise when overriding methods.