Python as a programming language offers an outstanding feature: the comprehensive standard library, which provides developers with a wide range of modules and packages. This collection is known as "batteries included," which means that in many cases you are not reliant on external packages to accomplish common tasks. In this guide, you will learn how to effectively use the standard library and what valuable modules are available to you.
Main Insights
The Python standard library is a collection of modules and functions that cover many everyday programming tasks. It includes useful tools for data manipulation, file management, and network communication. By using these standard modules, you can work more efficiently and complete your projects faster.
Step-by-Step Guide
Overview of the Standard Library
The Python standard library provides a wide array of modules. These modules are organized into various categories. When you first access the documentation of the standard library, you will find that everything you need is already available.
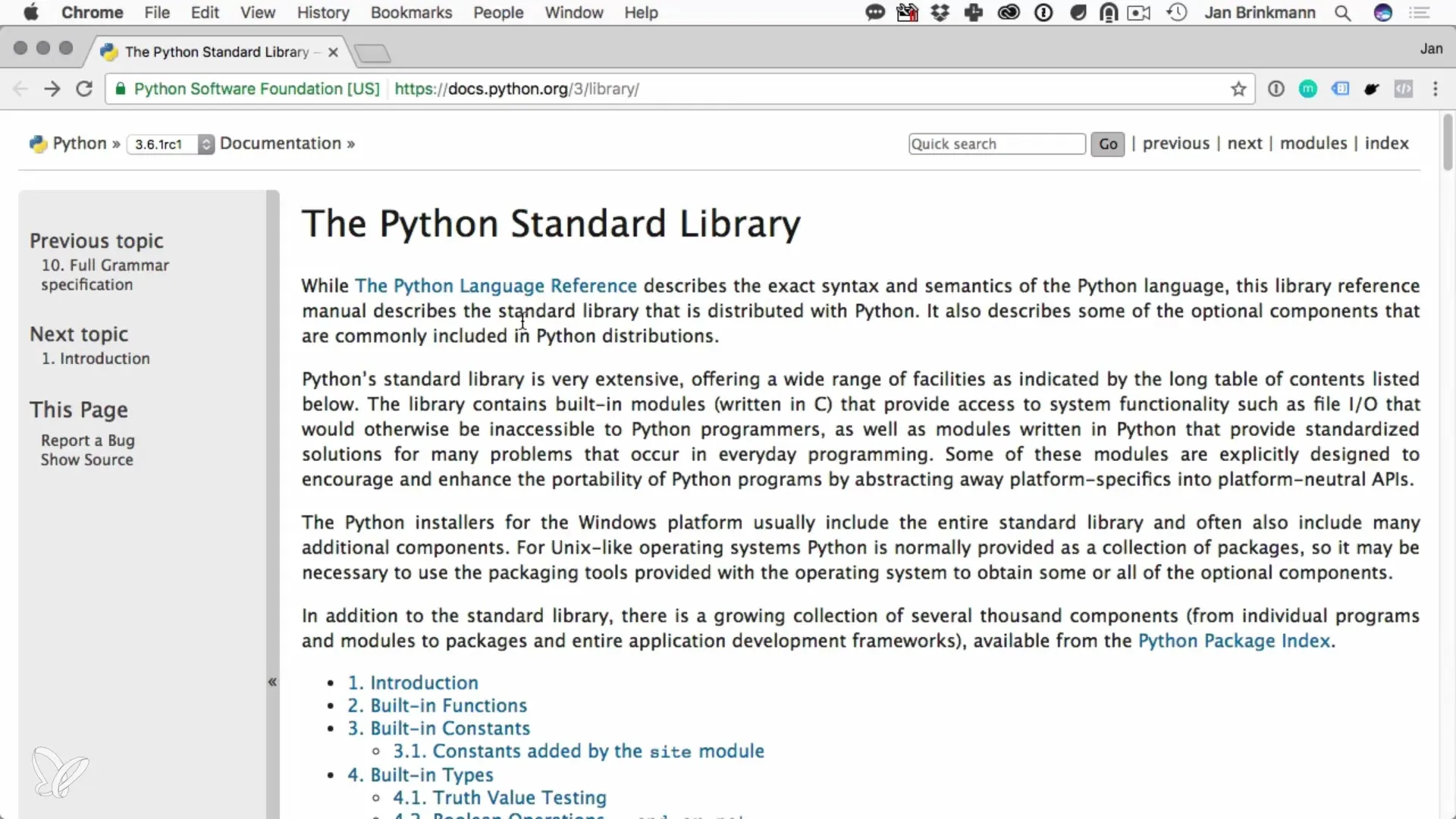
This collection includes basic data types, mathematical functions, file functions, network and internet protocols, as well as many other modules that you can use in your applications. It is advisable to familiarize yourself with the structure and categories of the library to quickly find the suitable modules.
Using Modules
To utilize the various modules, you must first import them into your project. For example, you can use the csv module to work with CSV files. When working with such modules, importing is the first step.
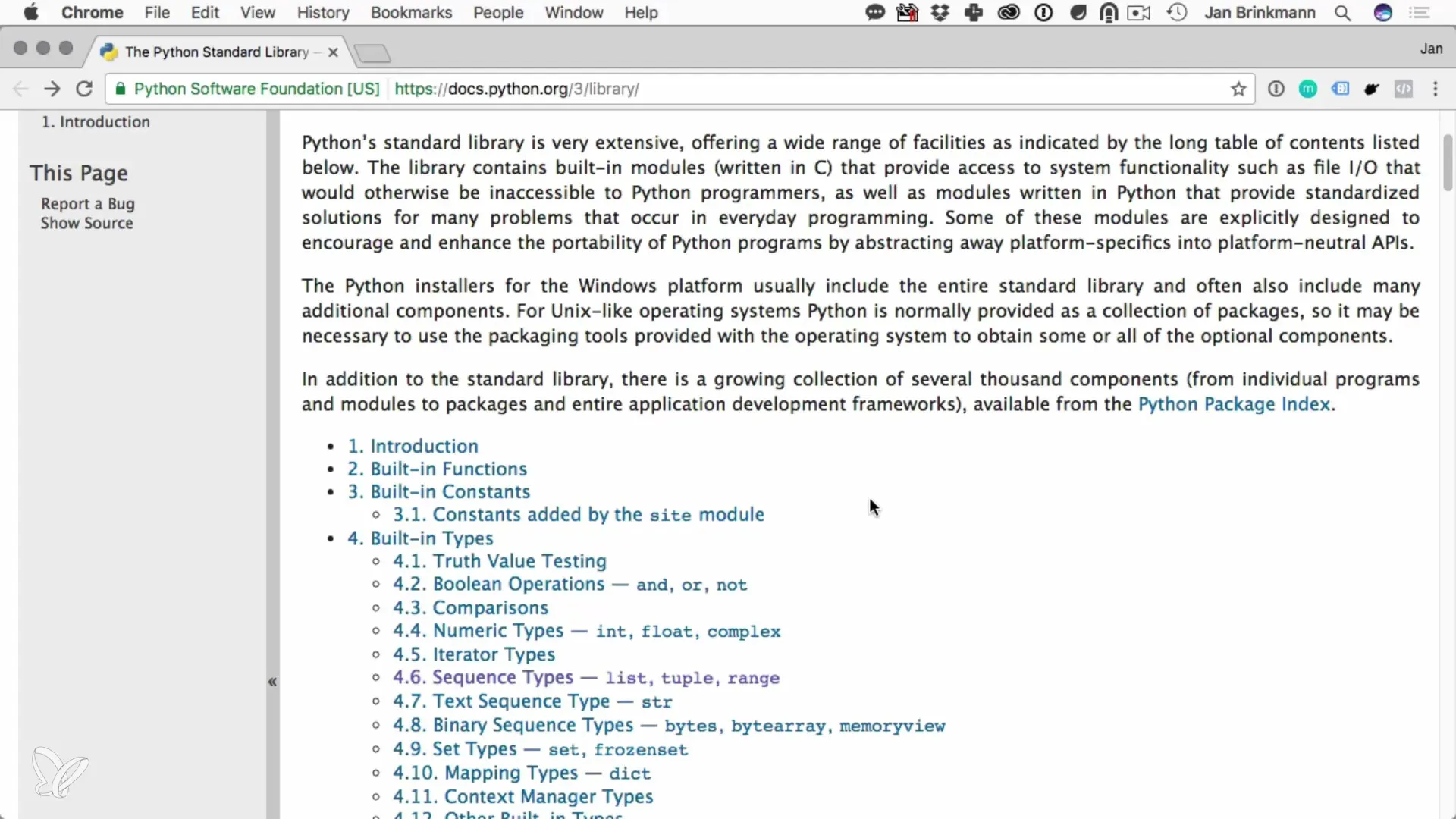
With a simple command like import csv, you can use the functions of the module in your code. It is important to choose the right modules for your specific needs. Python ensures that most of the functionality you require is already integrated.
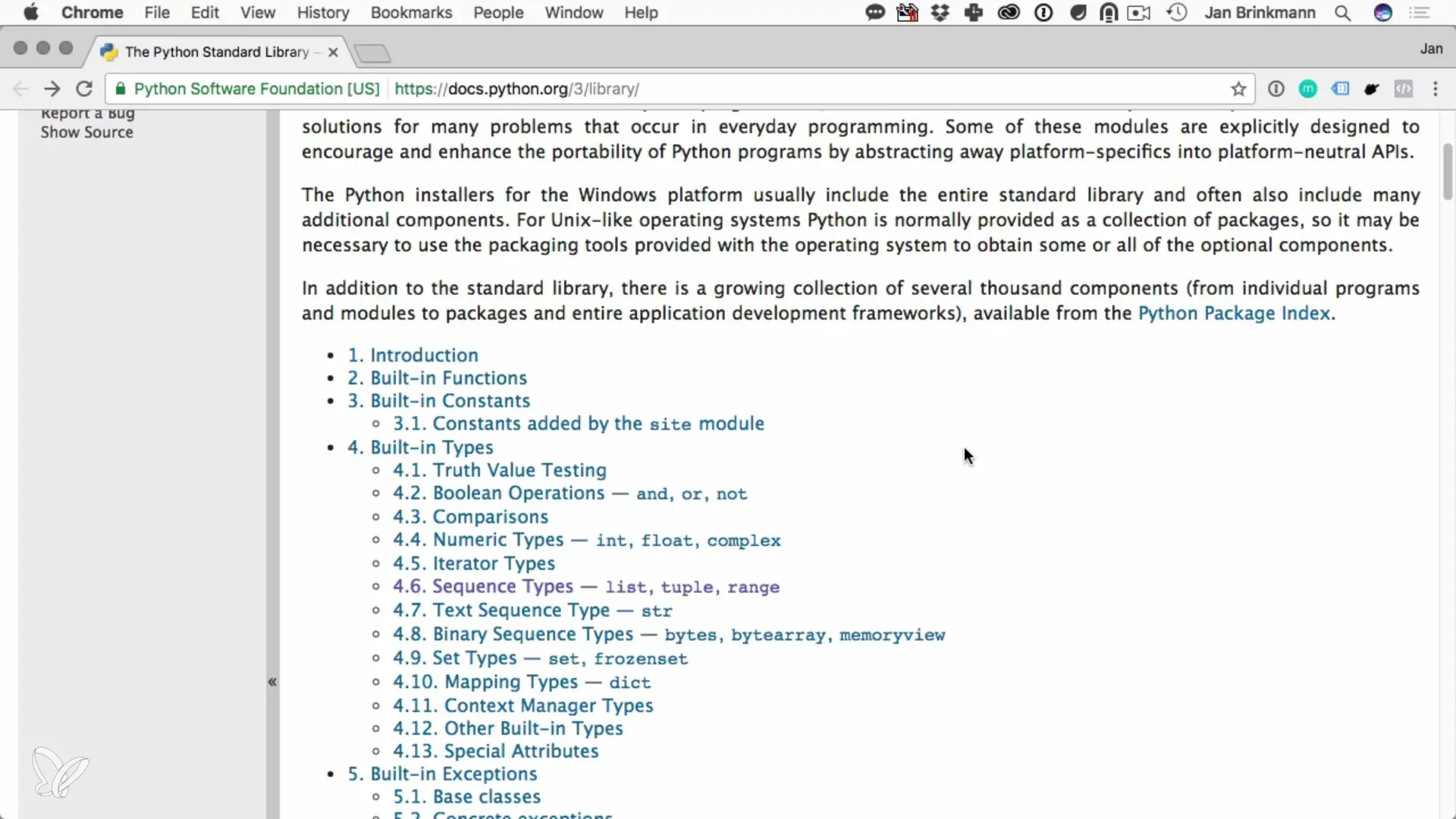
Example: Working with the CSV Module
Suppose you want to work with CSV files. The csv module provides you with an easy way to read and write this type of file. After importing the module, you can use the function csv.reader() to read a CSV file.
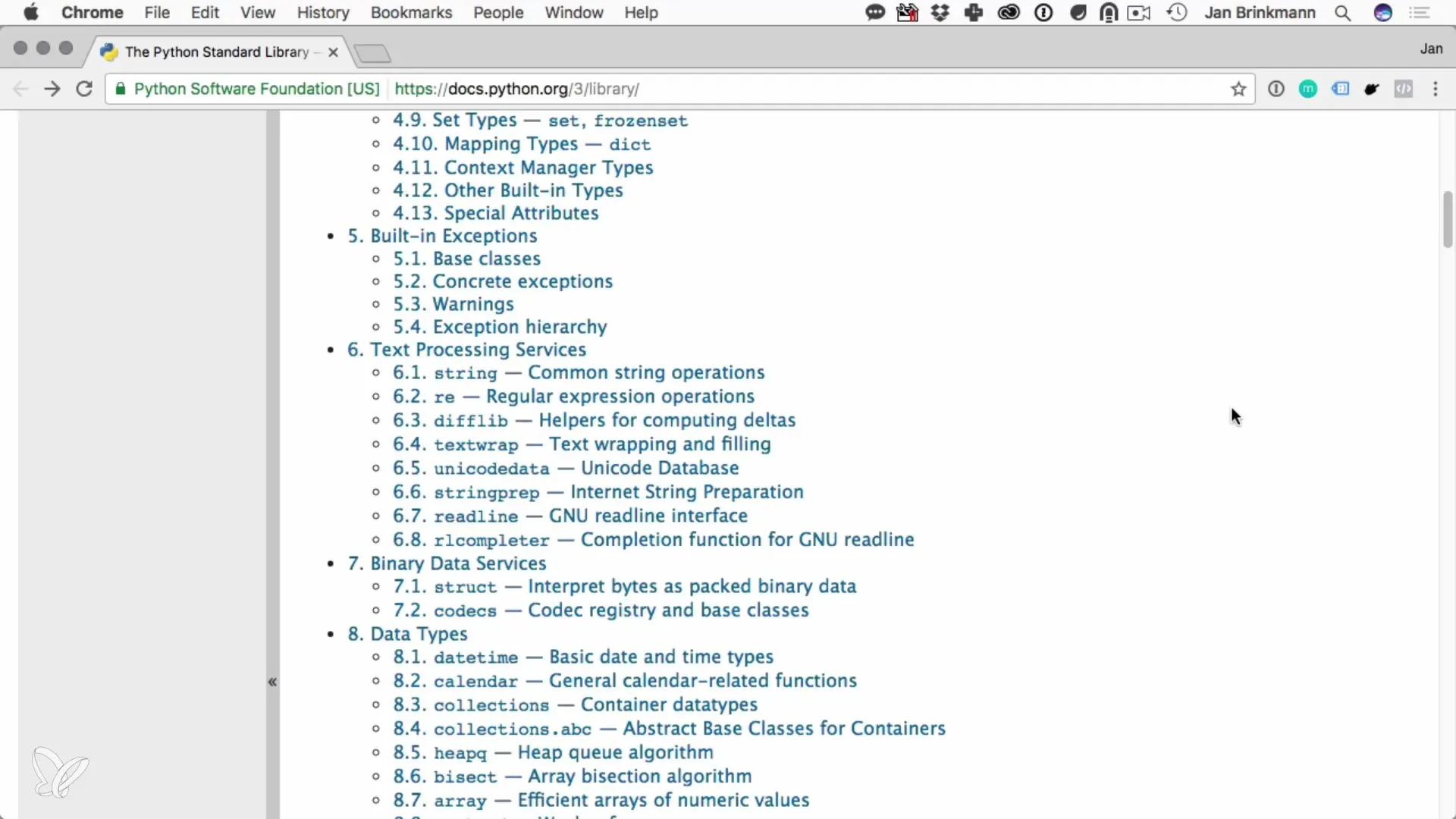
It is important to understand the structure of the CSV file. Since CSV files typically contain values separated by commas, you can easily convert the data into Python lists and process it further.
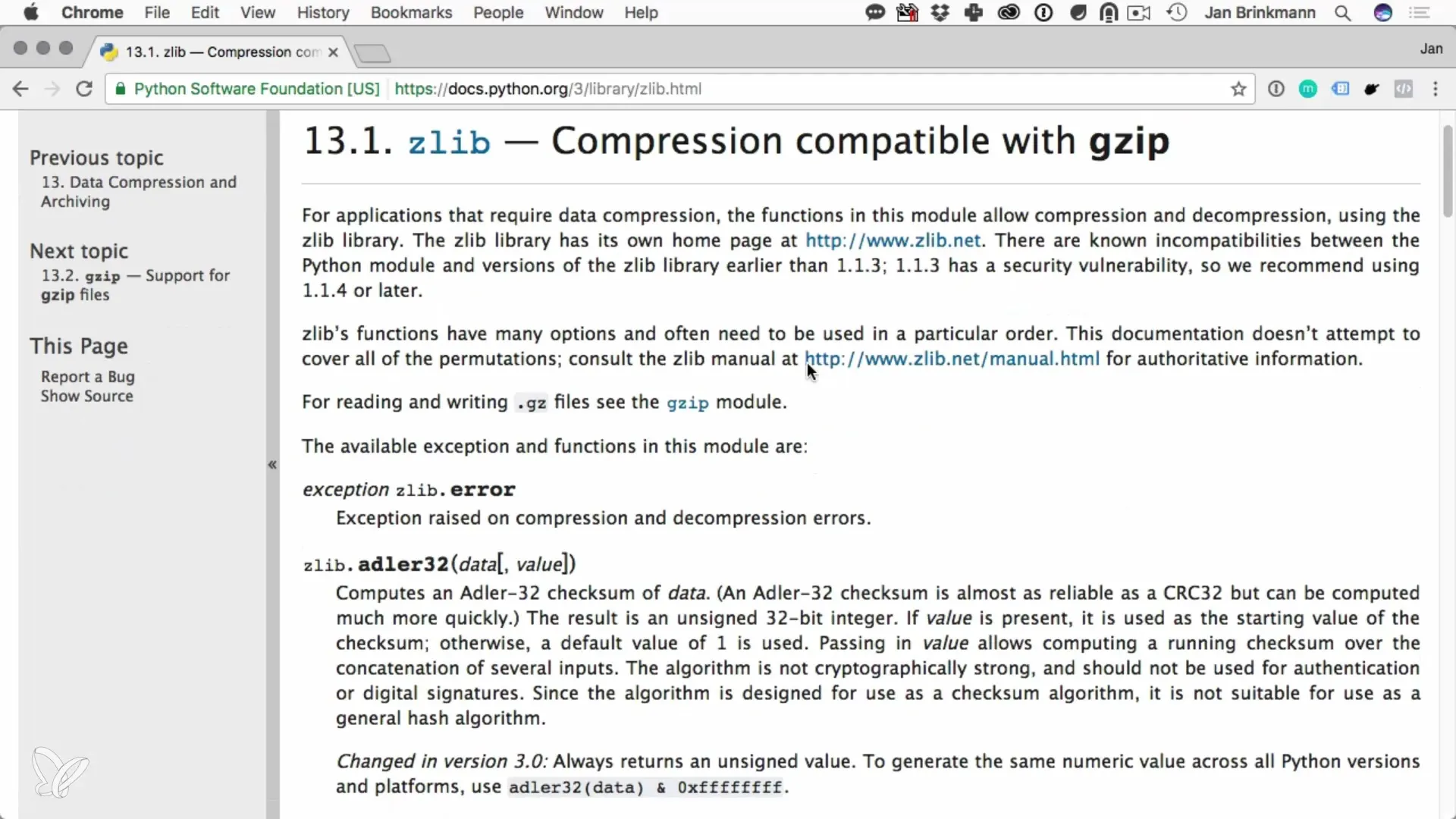
For writing CSV files, the csv module offers the function csv.writer(), which allows you to create and fill new files. This gives you the opportunity to export data efficiently.
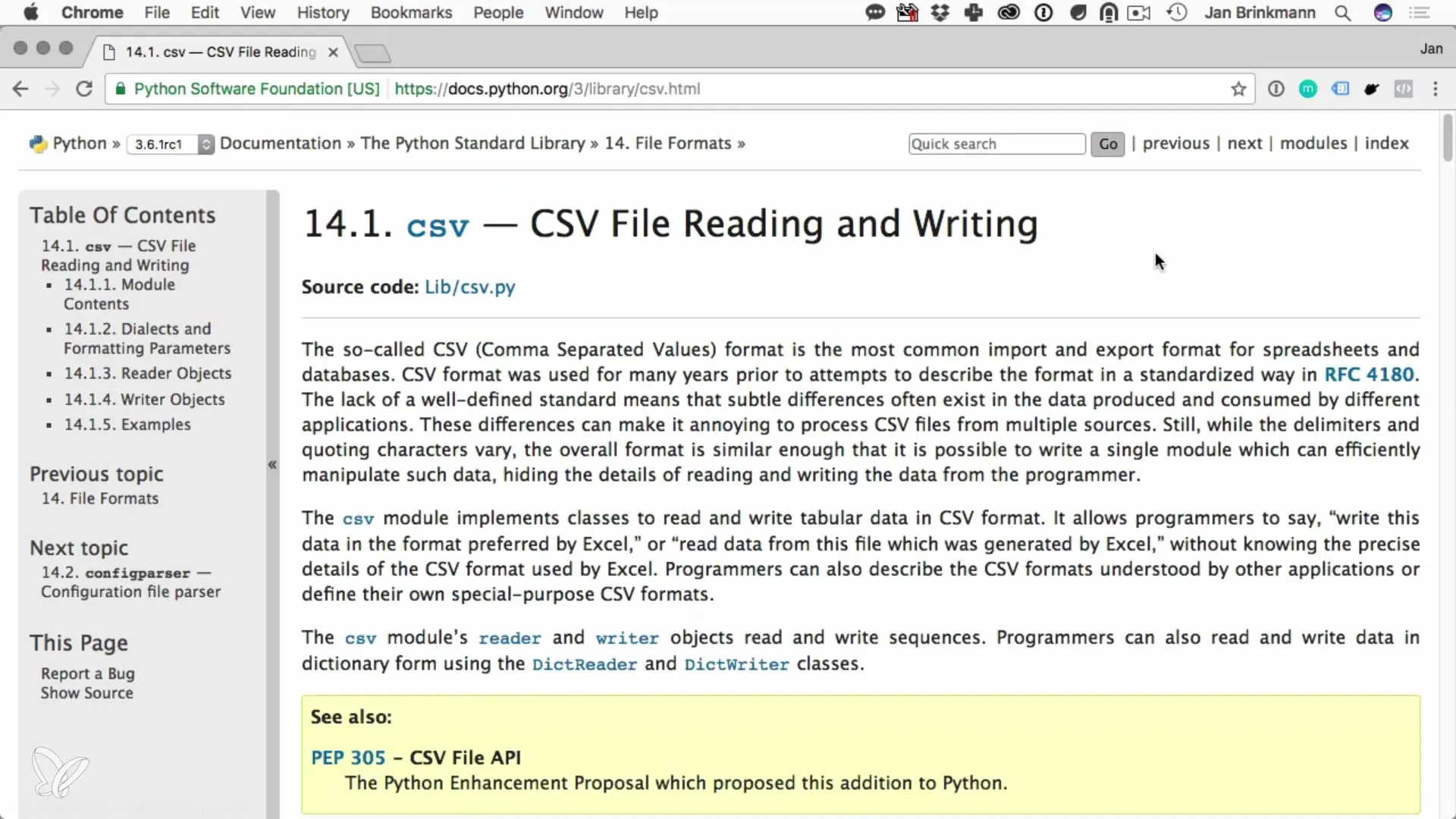
Discovering Additional Modules
The csv module is just one of many available to you. You can also use other modules like zlib for compression or json for working with JSON data.
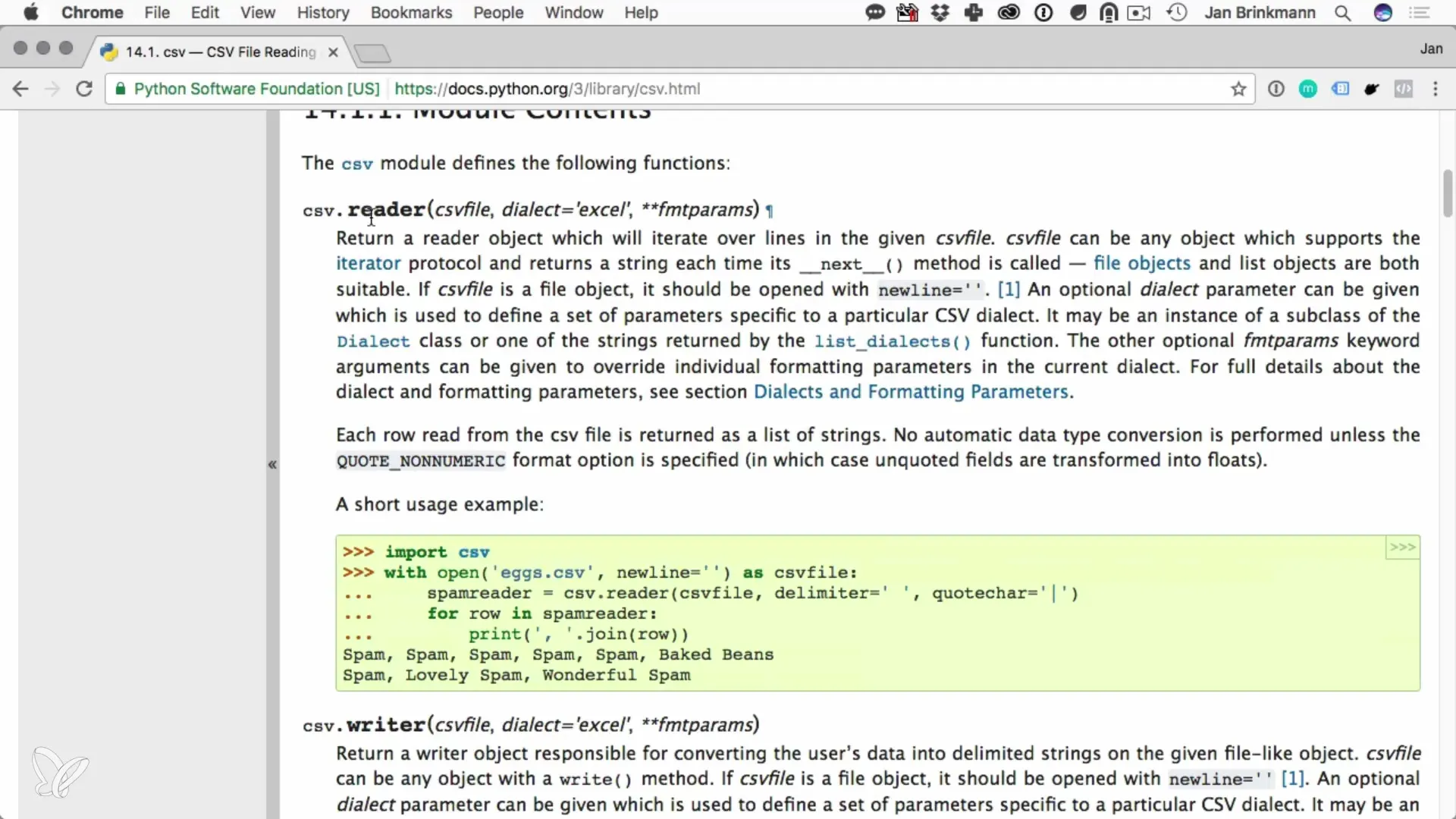
To learn more about the available modules, it is advisable to consult the official Python documentation. There, you will find not only a list of all modules but also their functions, usage examples, and much more information.
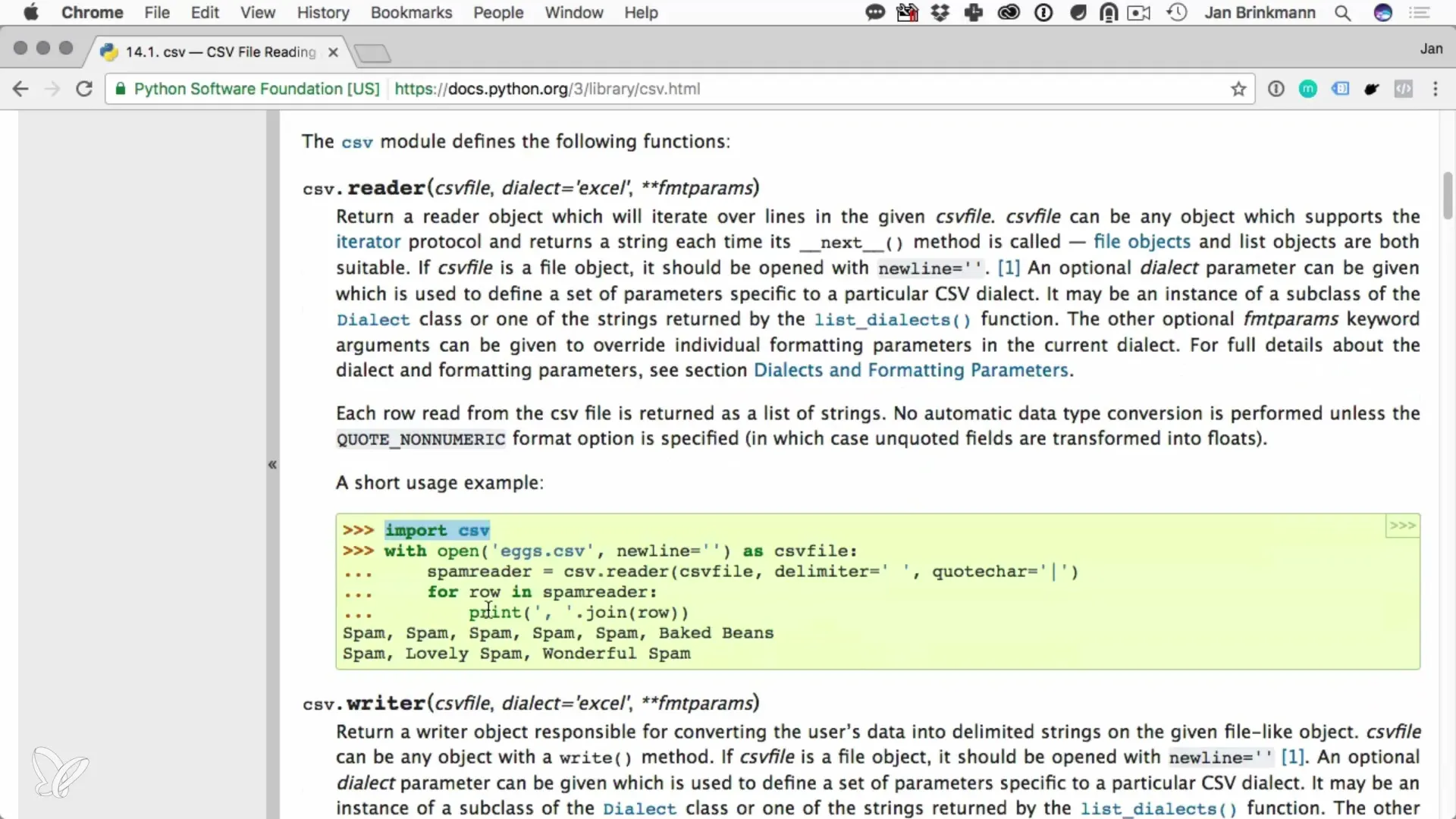
The number of modules can seem overwhelming, but it is advisable to proceed step-by-step and focus on the modules that you need for your current projects.
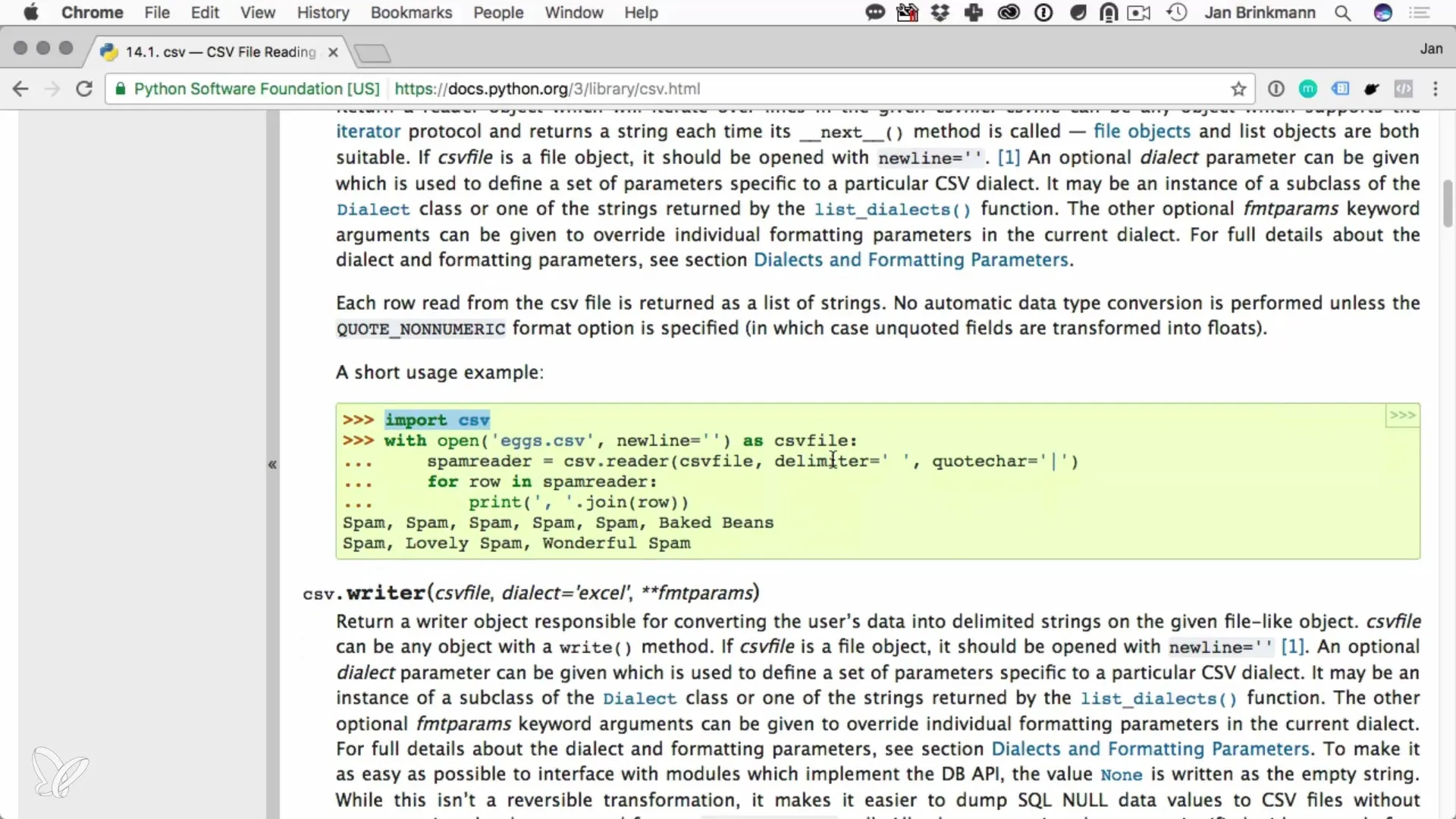
Summary – Programming with Python: The Python Standard Library in Detail
In this guide, you have learned that the Python standard library is a vast collection of useful modules that provide you with a variety of functions for different tasks. Accessing these modules is done by simply importing them, allowing you to work efficiently with common file formats like CSV or compression services. With knowledge of the available modules, you can program more effectively and speed up your projects.
Frequently Asked Questions
What is the Python standard library?The Python standard library is a collection of modules and functions that cover basic programming tasks.
How do I import a module in Python?You can import a module into your script using the command import modulname.
Which module can I use for CSV files?For working with CSV files, the csv module is recommended, which facilitates reading and writing.
Where can I find more information about the modules?The official Python documentation provides comprehensive information about all available modules.