Dictionaries, also known as associative arrays or associative lists, are a fundamental data structure in Python. They allow you to efficiently organize and access data in the form of key-value pairs. This flexibility makes them particularly useful for many programming tasks. In this guide, you will learn how to work with dictionaries in Python and what basic functions they offer.
Key Insights
- Dictionaries use key-value pairs to store data.
- Accessing values is done via keys, not numeric indices.
- Error handling is important when accessing non-existent keys.
- Dictionaries allow adding, updating, and deleting entries.
Step-by-Step Guide
To work with dictionaries in Python, follow these steps:
1. Creating an Empty Dictionary
To create an empty dictionary in Python, use curly braces.
At this point, you have created an empty dictionary.
2. Adding Key-Value Pairs
Now you want to add values to your dictionary. This is done by assigning values to keys. For example, you could store ages of characters from a movie or book.
These lines create the corresponding key-value pairs for the characters Legolas, Aragorn, and Frodo.
3. Accessing Values
To access the values in your dictionary, use the key. You can do this with the print function.
This will display the age of Legolas, which you stored earlier.
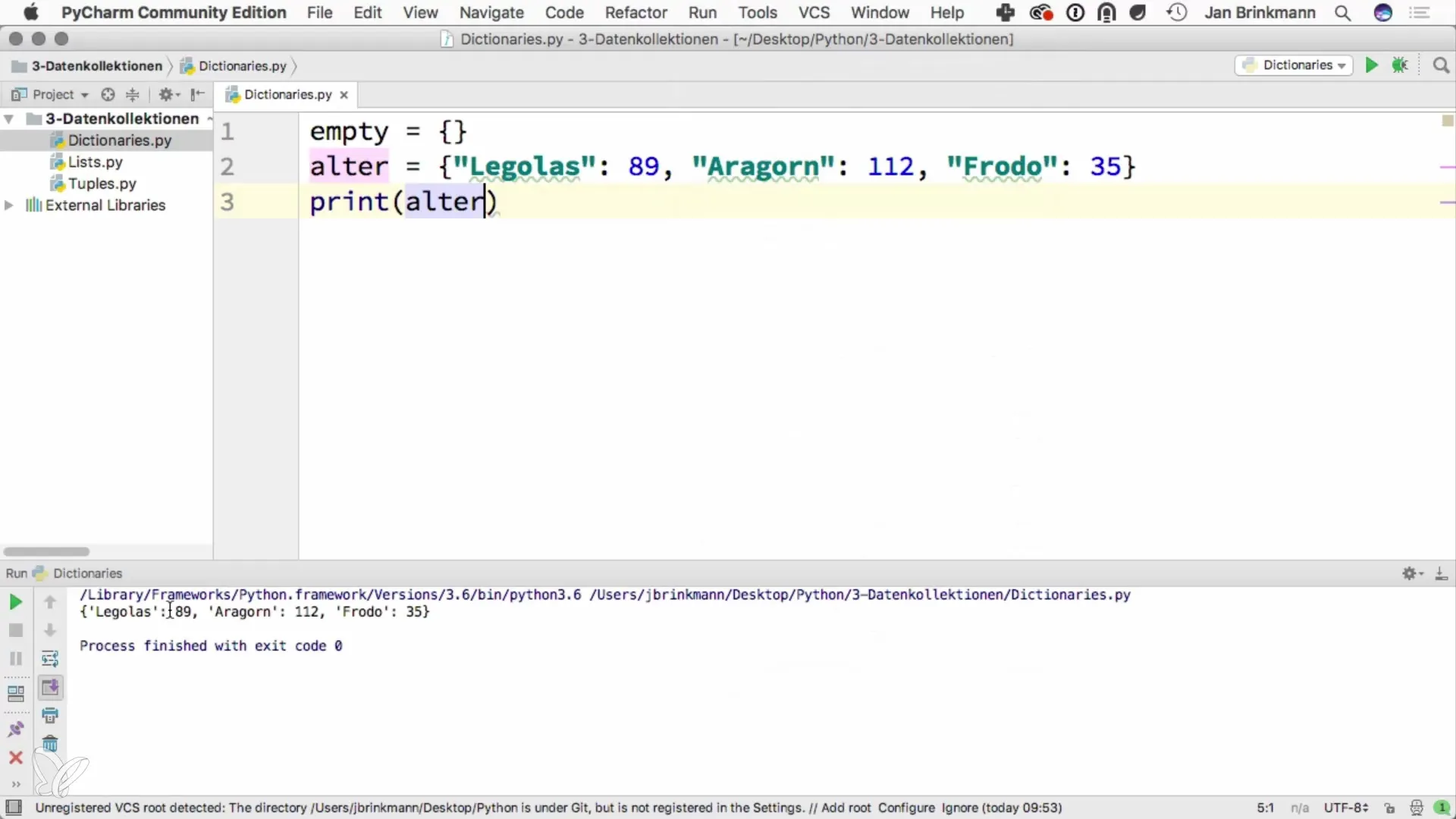
4. Error Handling
It is important to ensure that you only access keys that are actually present in your dictionary. If you try to access a non-existent key, you will get a KeyError.
This code will produce an error because 'Sam' is not contained in the dictionary.
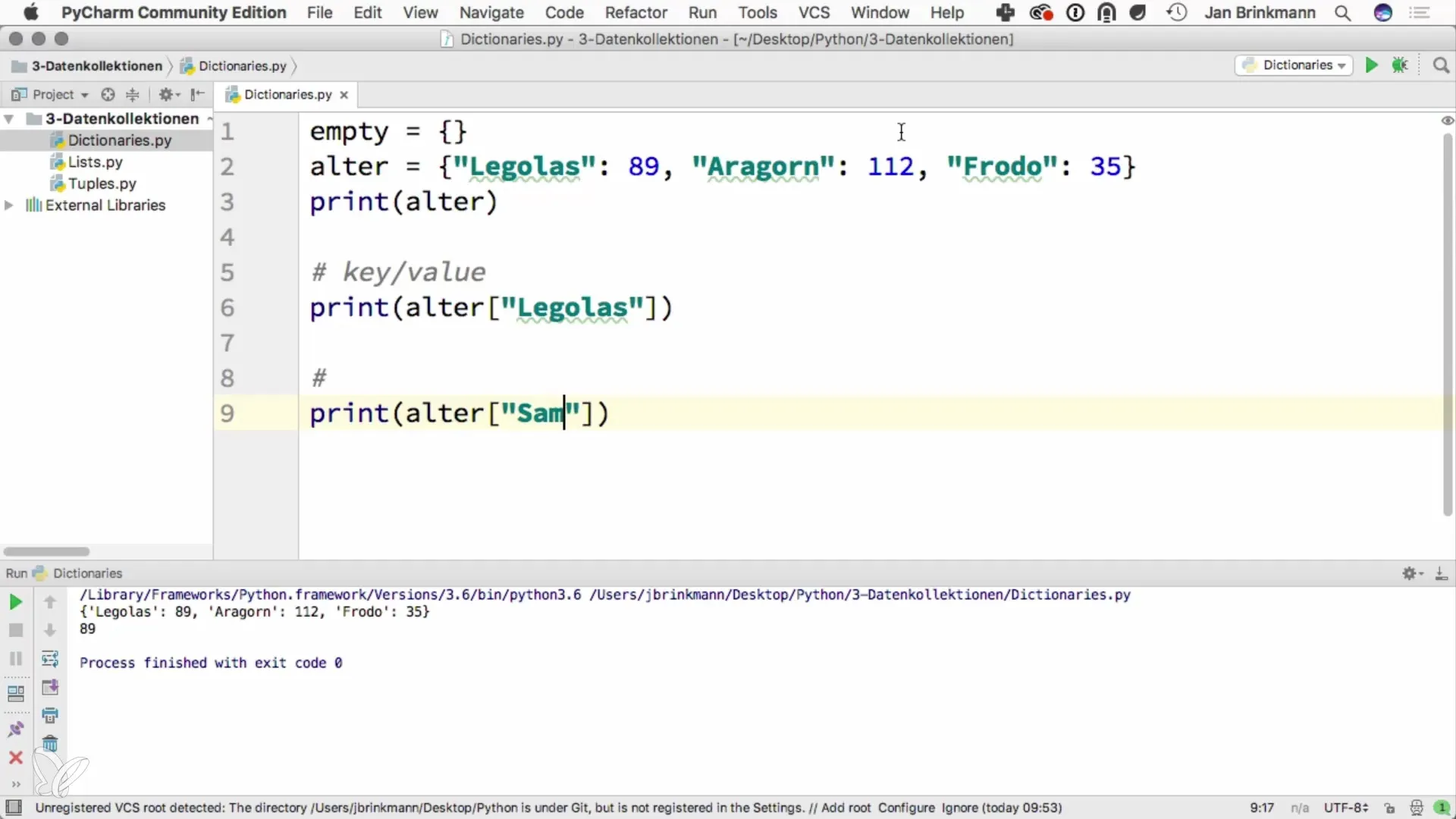
5. Using the get Method
To safely access values without causing an error, you can use the get method. This returns None if the key is not found, instead of throwing an error.
If 'Sam' is not present, it displays "Not found".
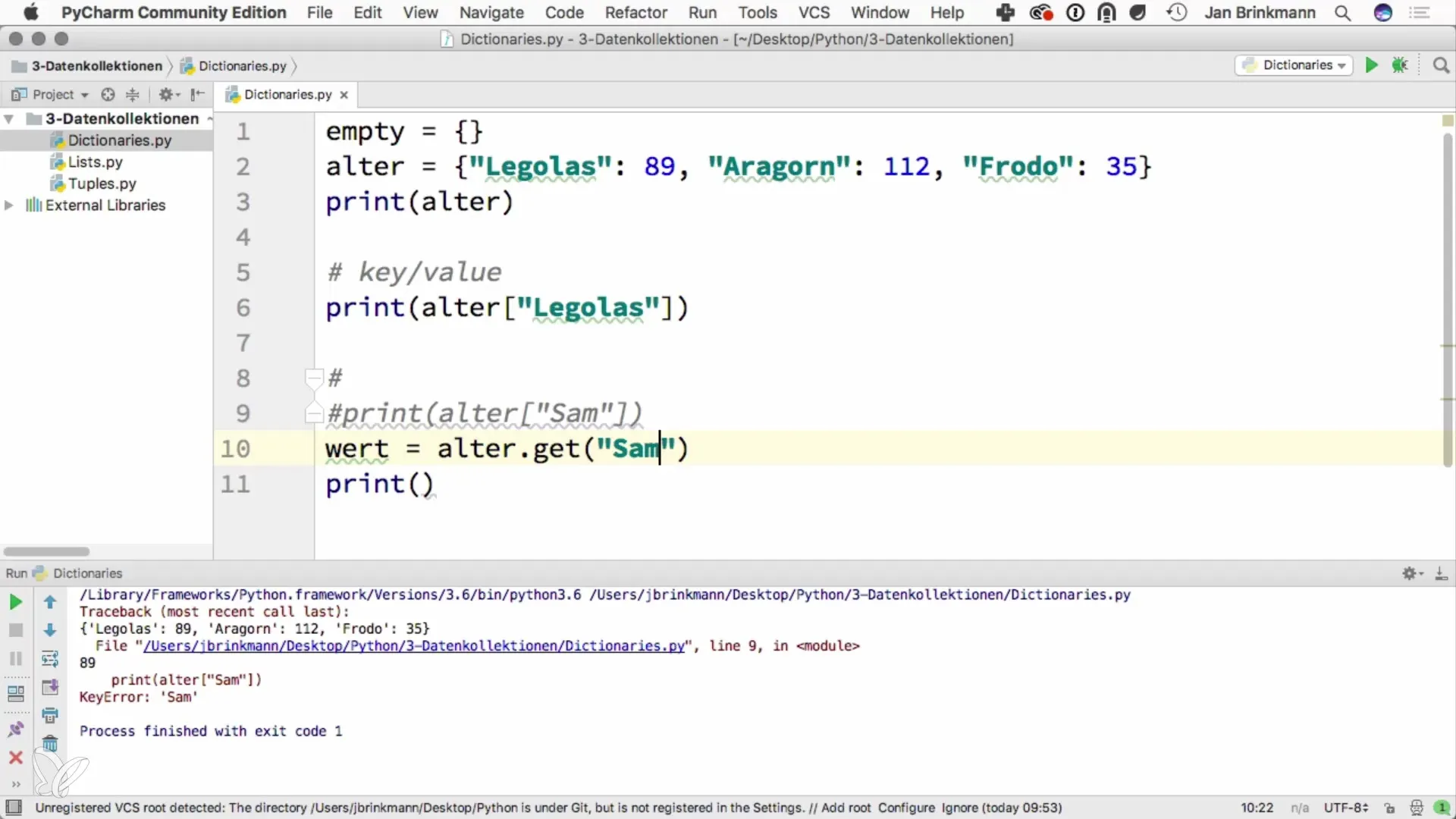
6. Updating Values
It is easy to update values in a dictionary.
Now Frodo has a new value.
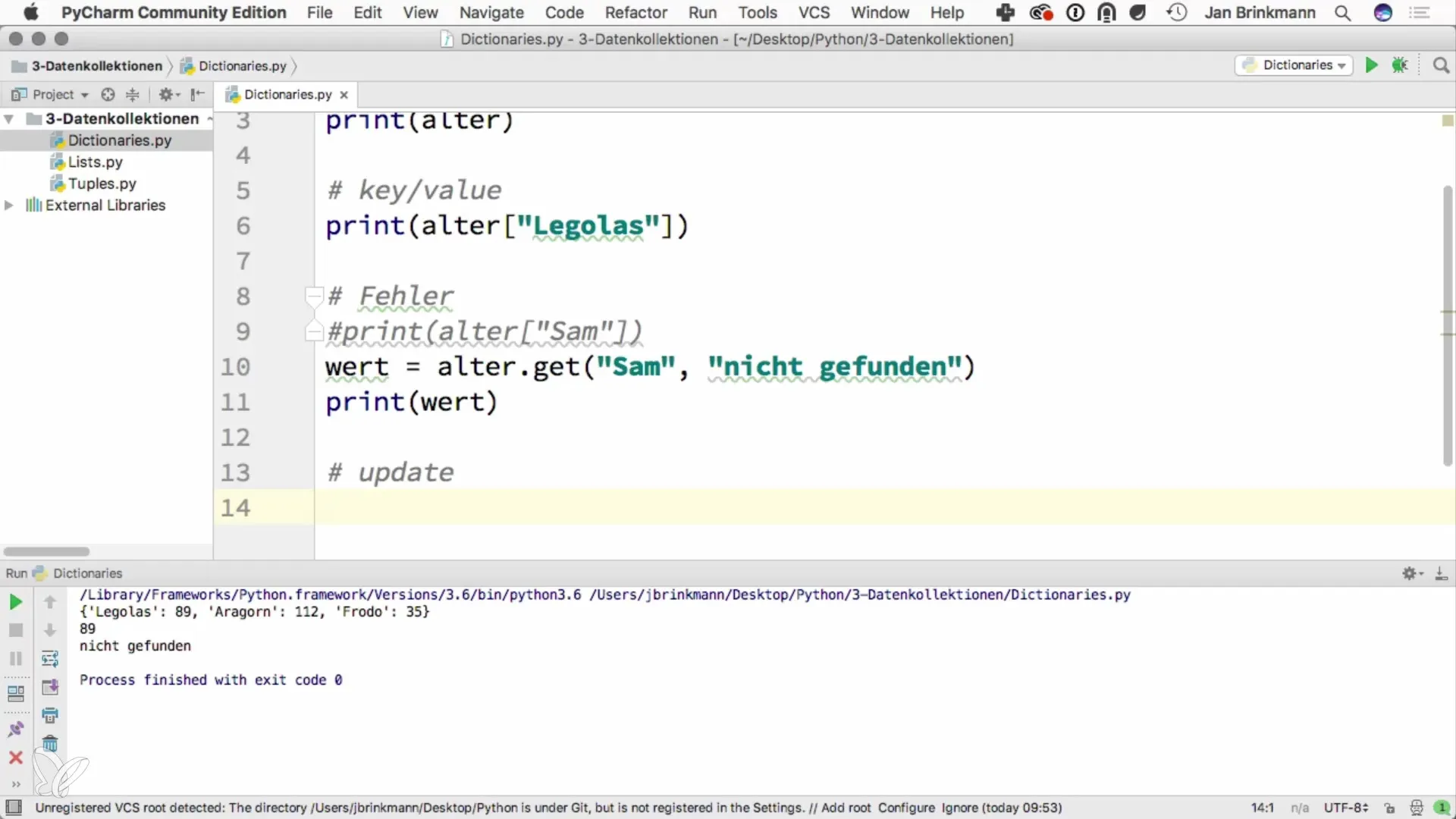
7. Adding New Entries
Adding new entries works in the same way.
Now Yoda is an additional entry in your dictionary.
8. Deleting Entries
If you want to remove an entry from your dictionary, you can use the del operator.
Yoda will be removed from the dictionary.
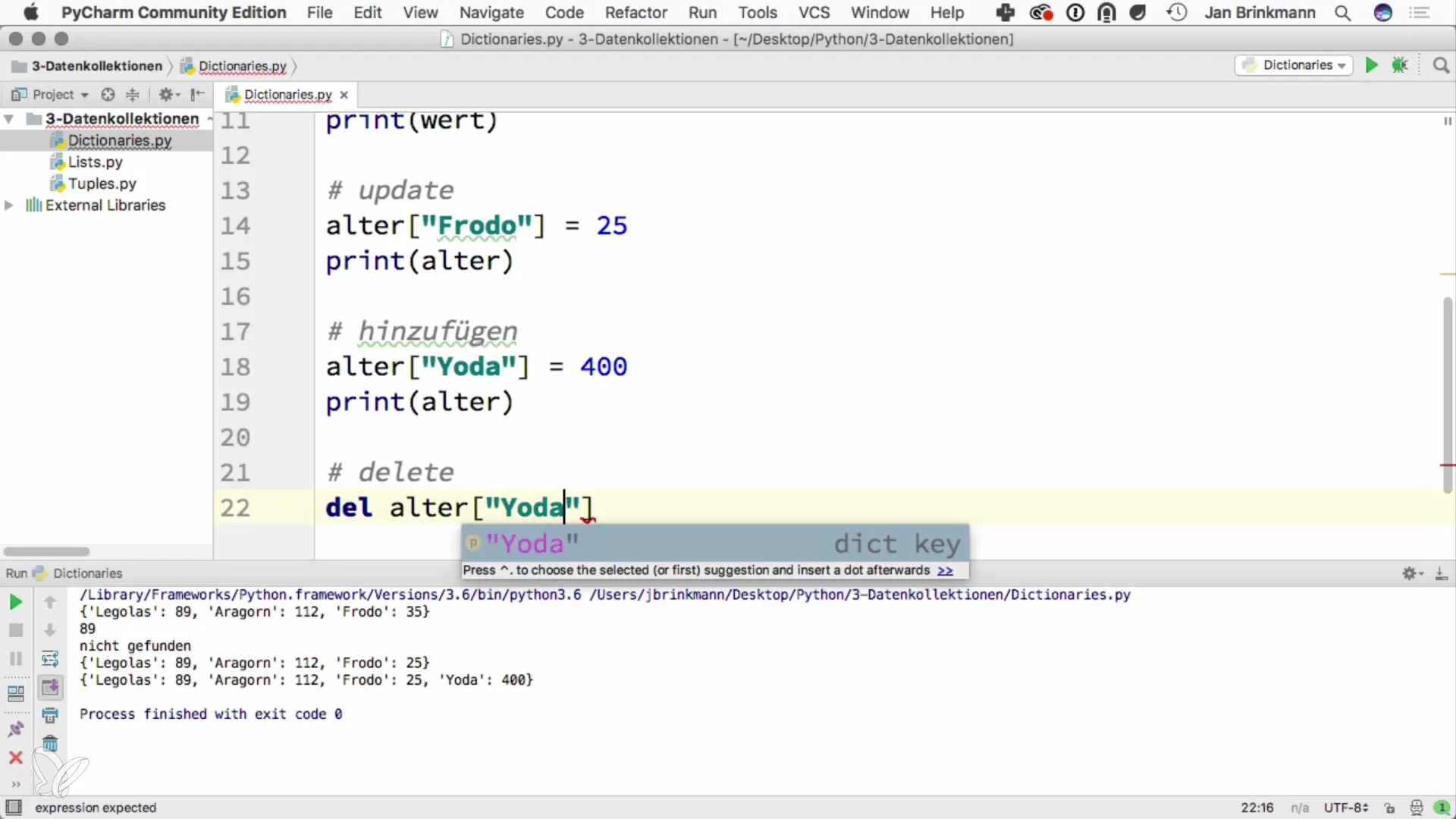
9. Clearing All Entries
If you want to clear all entries in your dictionary, you can use the clear method.
Now your dictionary is empty.
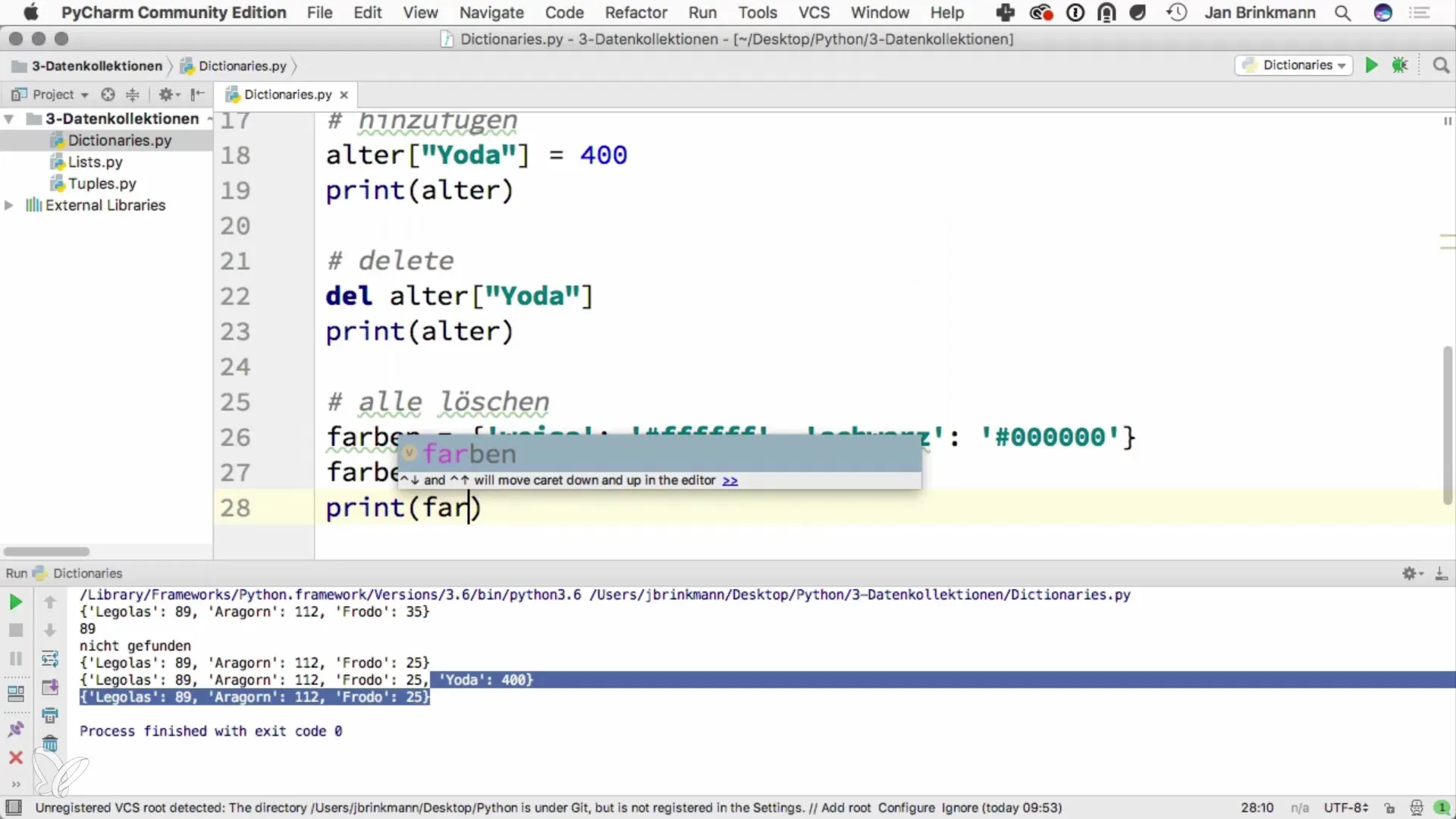
10. Checking if a Key Exists
Sometimes it is useful to know if a key exists in your dictionary.
This query will return whether Frodo is present in the dictionary or not.
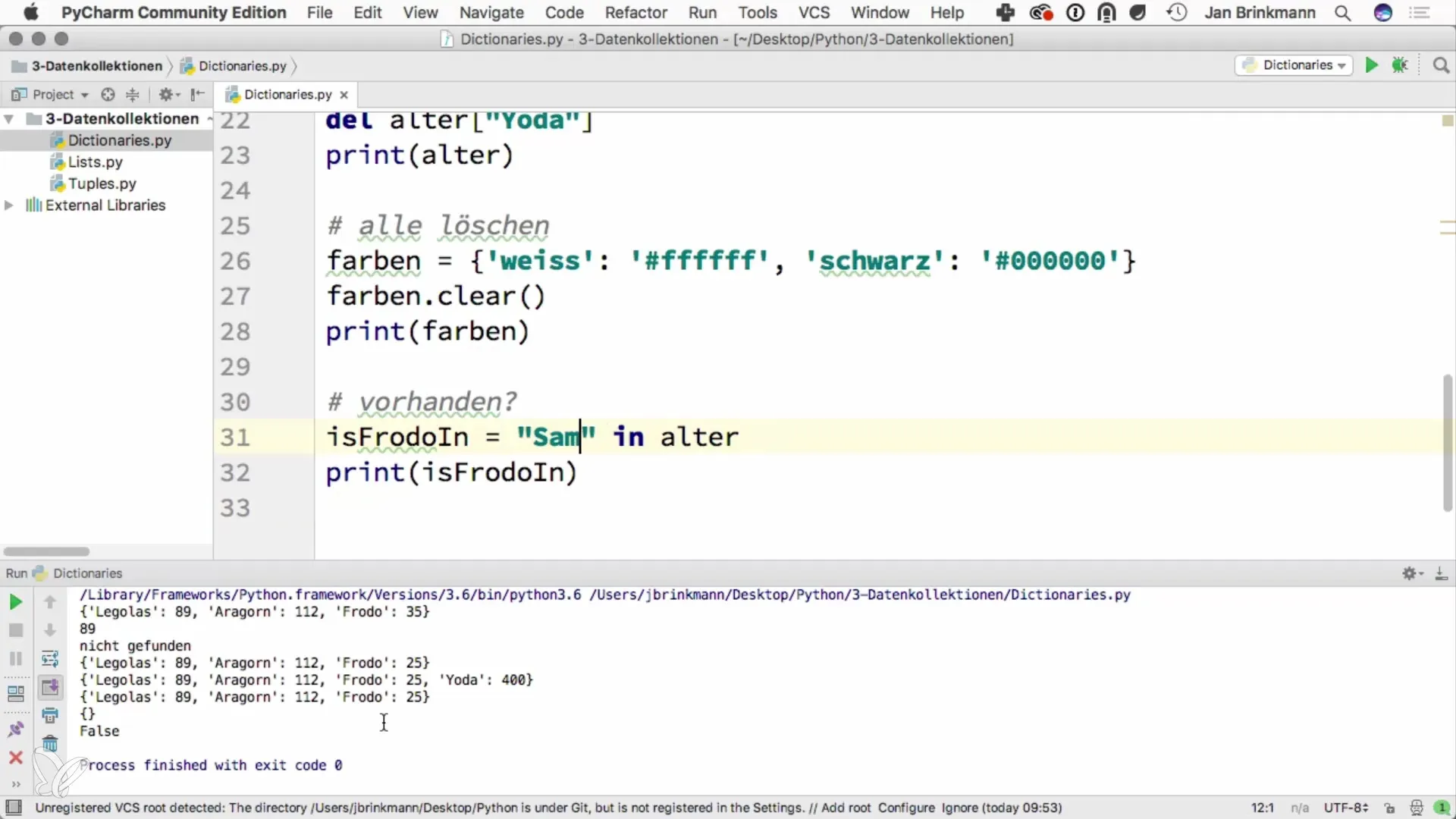
11. Listing All Keys
If you want to retrieve all the keys in your dictionary, you can use the keys() method.
This will give you an overview of all keys in the dictionary.
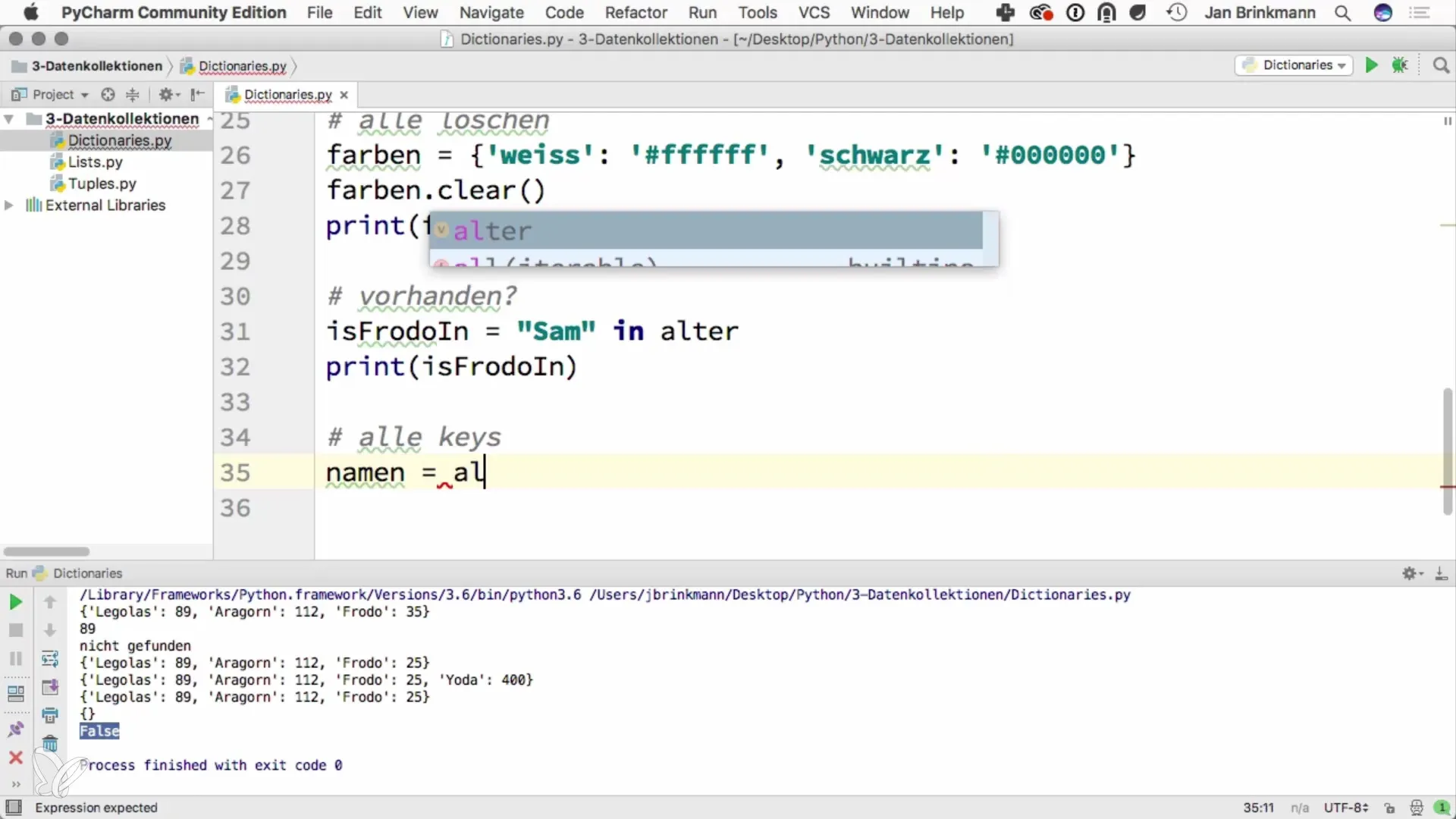
Summary – Introduction to Python Dictionaries: Understanding Associative Data Structures
In this guide, you have learned the basics of dictionaries in Python. You now know how to create them, access values, update them, and delete them. Additionally, you have learned how to avoid errors and ensure the integrity of your code.
Frequently Asked Questions
How do I create a dictionary in Python?Use curly braces: D = {}.
Can I initialize a dictionary with values?Yes, by specifying key-value pairs like D = {'Key': 'Value'}.
What happens if I access a non-existent key?A KeyError will be raised.
How can I list all keys of a dictionary?Use the keys() method: D.keys().
How do I delete an entry from a dictionary?Use the del operator: del D['Key'].