Variables are the cornerstone of any programming language and play a central role in Python programming. They allow you to store, process, and access data dynamically. In this section, I will show you how to work with variables in Python, what data types exist, and what you need to consider when naming your variables.
Key insights
- Variables are references to memory areas where values are stored.
- In Python, everything is an object, including primitive data types.
- Variable names are subject to certain rules that must be followed when programming.
Step-by-step guide
To understand the concept of variables in Python, we will start with the Python console.
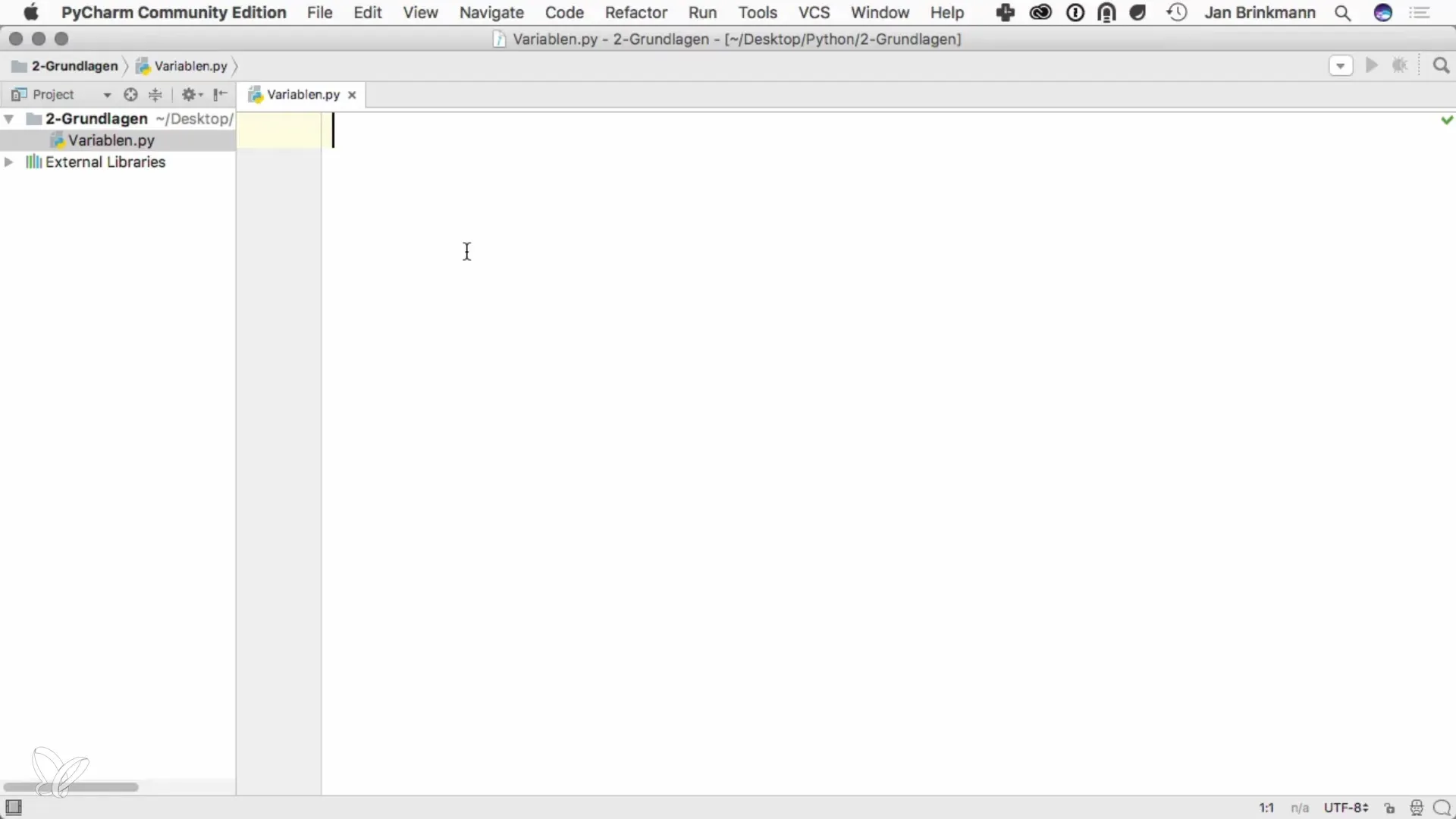
The Python console or interactive environment is an excellent tool to try out various programming statements and calculations immediately. When you open the Python console, you can interact directly and, for example, input numbers to see what happens.
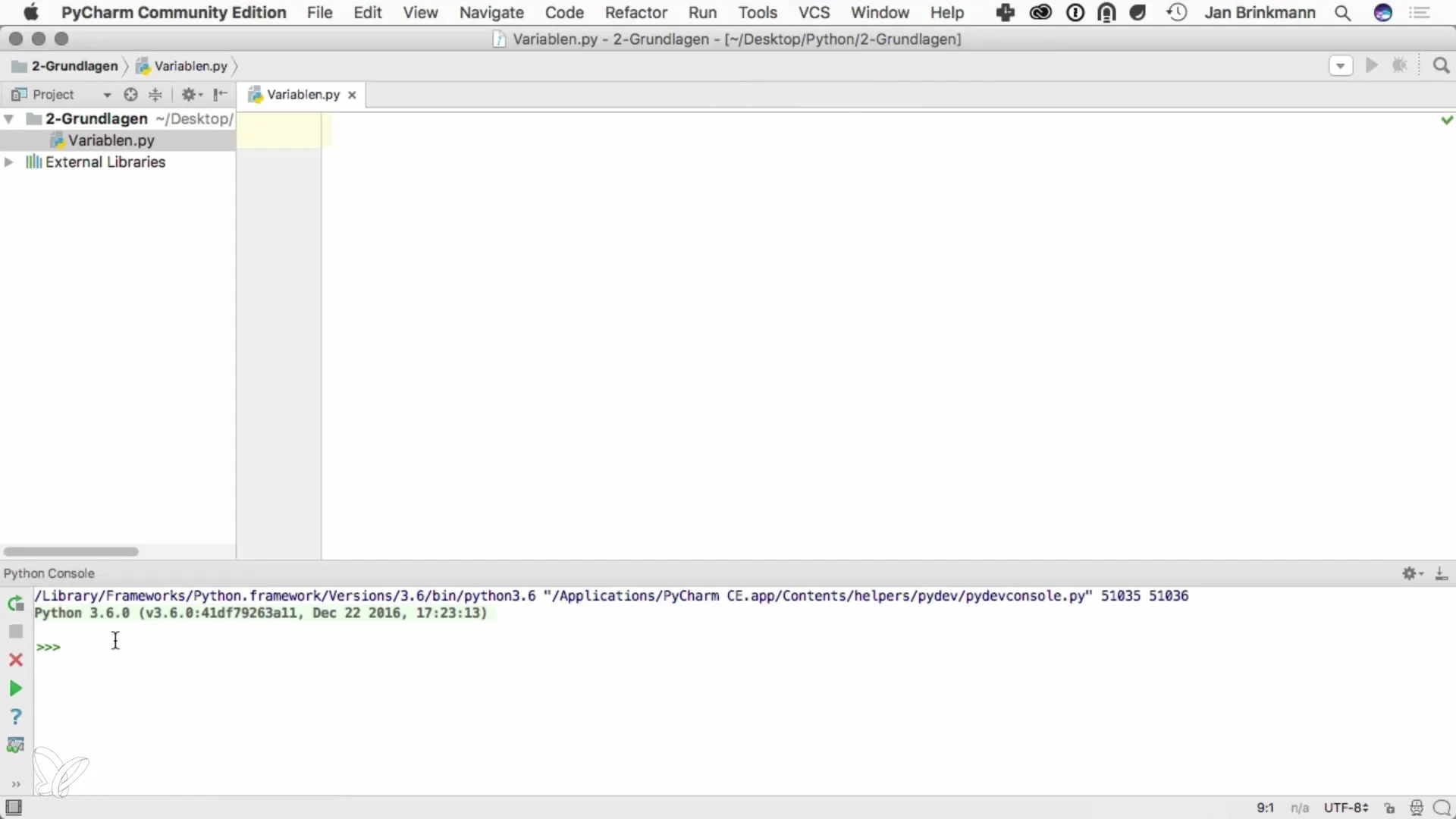
Here you can now enter a value, say the number 7. When you confirm the input, the value is immediately output. This shows you that Python has processed the input and displays the result directly.
Furthermore, you can perform calculations. For example, if you calculate 7 times 7, the console returns the result of 49. Thus, you have the opportunity to carry out both simple outputs and more complex calculations.
Now we come to an important aspect: working with variables. A variable is essentially a storage location that we can access to store and retrieve values. To create a variable, you simply assign a value. For example: x = 10. This means that the value 10 is stored in memory, and the variable x refers to it.
If you now type print(x), Python will show you the value 10. The great thing about it is that you can change the value of the variable at any time simply by assigning a new value to the variable, like x = 15. If you now execute print(x) again, you will get the value 15 back.
Variables can also be multiplied or used in other calculations. For example, you can say x * 3, which will give you 45, and then store this value in a new variable like y by writing y = x * 3. At this point, you can see how flexible working with variables is.
Now let’s look at how variables behave in code. You can not only use numbers, but also text in the form of strings. A string is a sequence of characters that you can define like this: name = "Jan Bringmann". With this assignment, you can store different texts and change them at any time by reassigning the value, e.g., name = "Joe Average".
If you want to work with other data types as well, you can define variables like x = 7 or pi = 3.14159 to store different values. There are some rules to keep in mind when it comes to naming variables.
Among the basics of variable naming is that they should always start with a lowercase letter. For example, x or xy is fine. If you want, you can also use uppercase letters in the name, such as in the name MyName.
A typical style is CamelCase, where you write multiple words together and start the first word with a lowercase letter. Another method is to use underscores like my_name, which is also quite common.
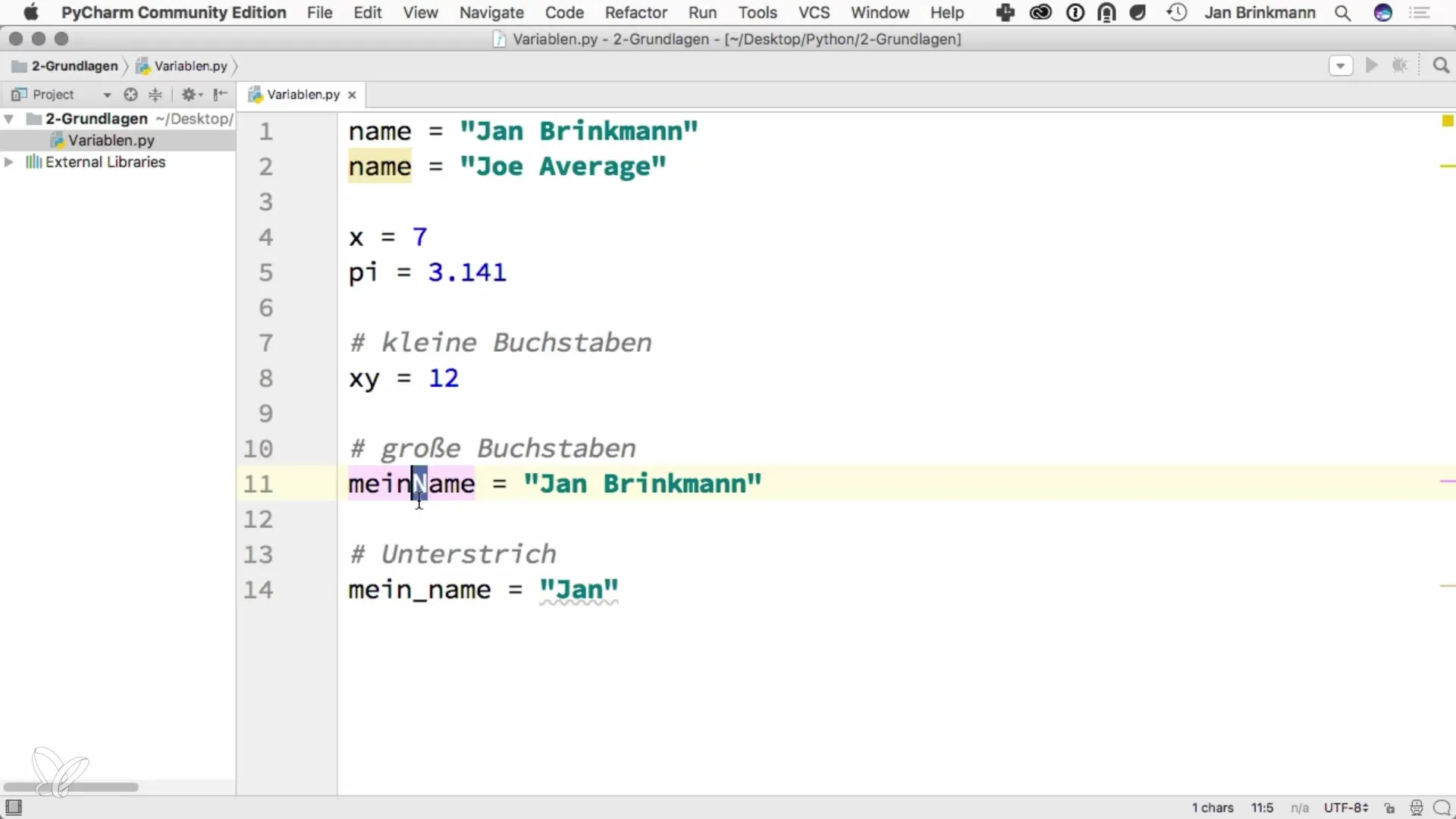
However, you must not use numbers at the beginning of your variable. For example, 1x = 5 is not considered a valid assignment and will cause an error. Additionally, you should be careful not to use reserved keywords like if or break, as these have special functions in Python.
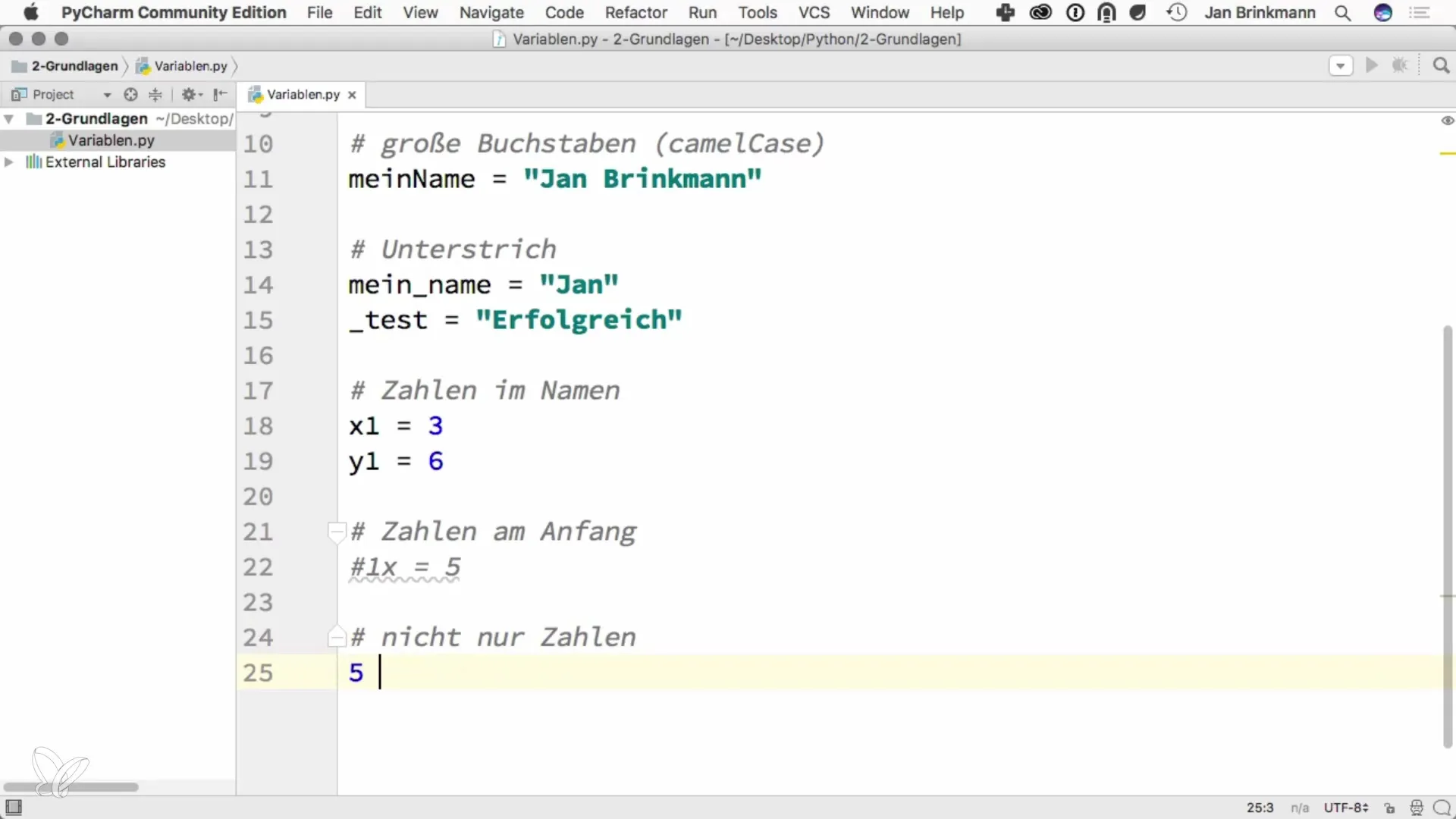
Also, remember that the data in Python should remain well-structured and readable. This includes ensuring that you always end your code files with a blank line to enhance readability.
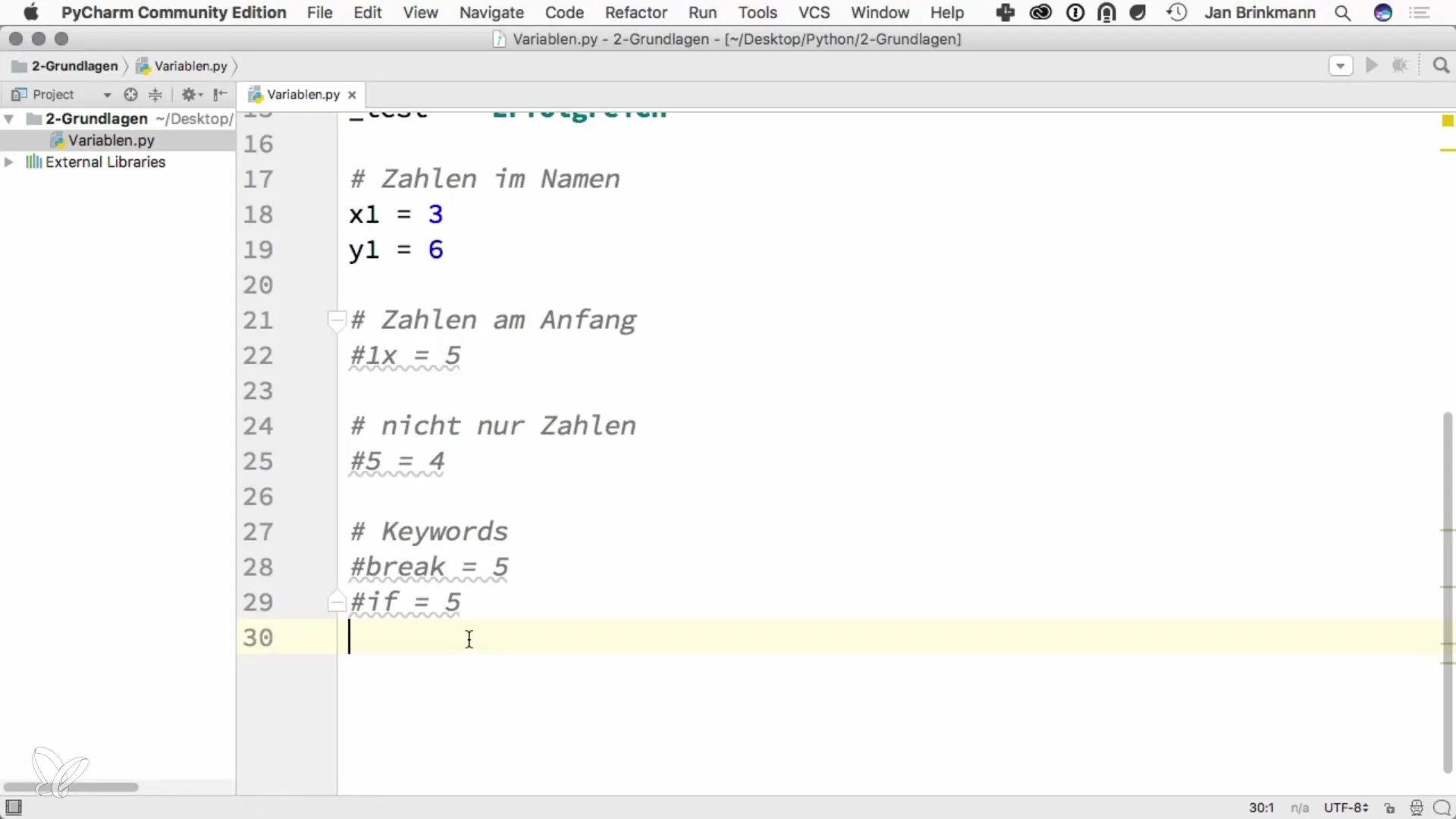
In summary, I have shown you how to work with variables in Python, what common data types exist, and what to pay attention to when naming. You are now ready to dive deeper into the world of Python and the various possibilities of programming.
Summary – Programming with Python: Understanding and Using Variables
Variables are the basic building blocks for storing data in Python. With the right knowledge of using and naming variables, you are well-equipped for your programming journey.
Frequently Asked Questions
How do I define a variable in Python?You can create a variable by simply choosing a name, followed by an equals sign and the value you want to assign, e.g., x = 10.
Can I assign multiple values to variables?Yes, in Python you can assign multiple values to variables even after they have already had a value.
What are the rules for variable names?Variable names should start with a letter, case can be used, and numbers are allowed but not at the beginning.
Can I use reserved words as variable names?No, reserved keywords like if, for, or break must not be used as variable names.
What is a data type in Python?A data type is a classification of data that determines its type and what you can do with it, e.g., Integer, Float, or String.