XML, or Extensible Markup Language, has established itself as an indispensable format for data exchange on the web. If you work with large datasets or process API responses, you will often encounter XML. In this tutorial, I will show you how to effectively process XML data with Python. By the end, we will read an XML-based e-commerce catalog together and extract the information contained within.
Main insights
- XML is a flexible markup language for describing and structuring data.
- Python offers an easy way to process XML data through the xml.etree.ElementTree module.
- Reading XML data requires understanding the hierarchy concept of nodes and sub-nodes.
Step-by-step guide
Step 1: Understanding XML data
First, you should take a look at an example of the structure of an XML file. XML uses nodes (tags) and attributes to represent information.
Here is the catalog of the main node, under which several product nodes are arranged. Each product node has its own child nodes such as ID, name, price, and stock.
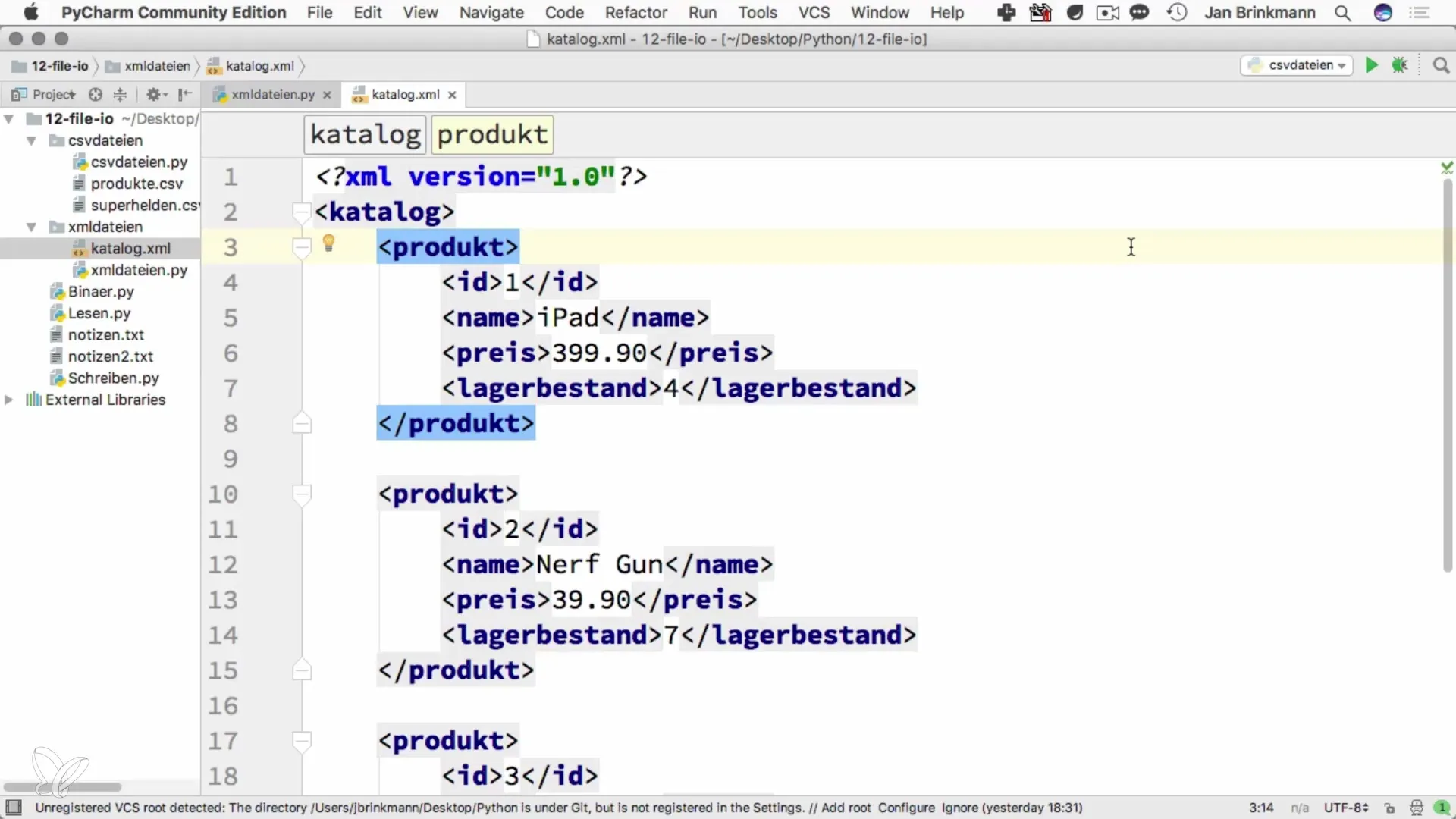
Step 2: Importing the XML module
To work with XML in Python, you need to import the xml.etree.ElementTree module. This allows you to read and manipulate XML data.
Once you have added the import statement to your code, you are ready for processing.
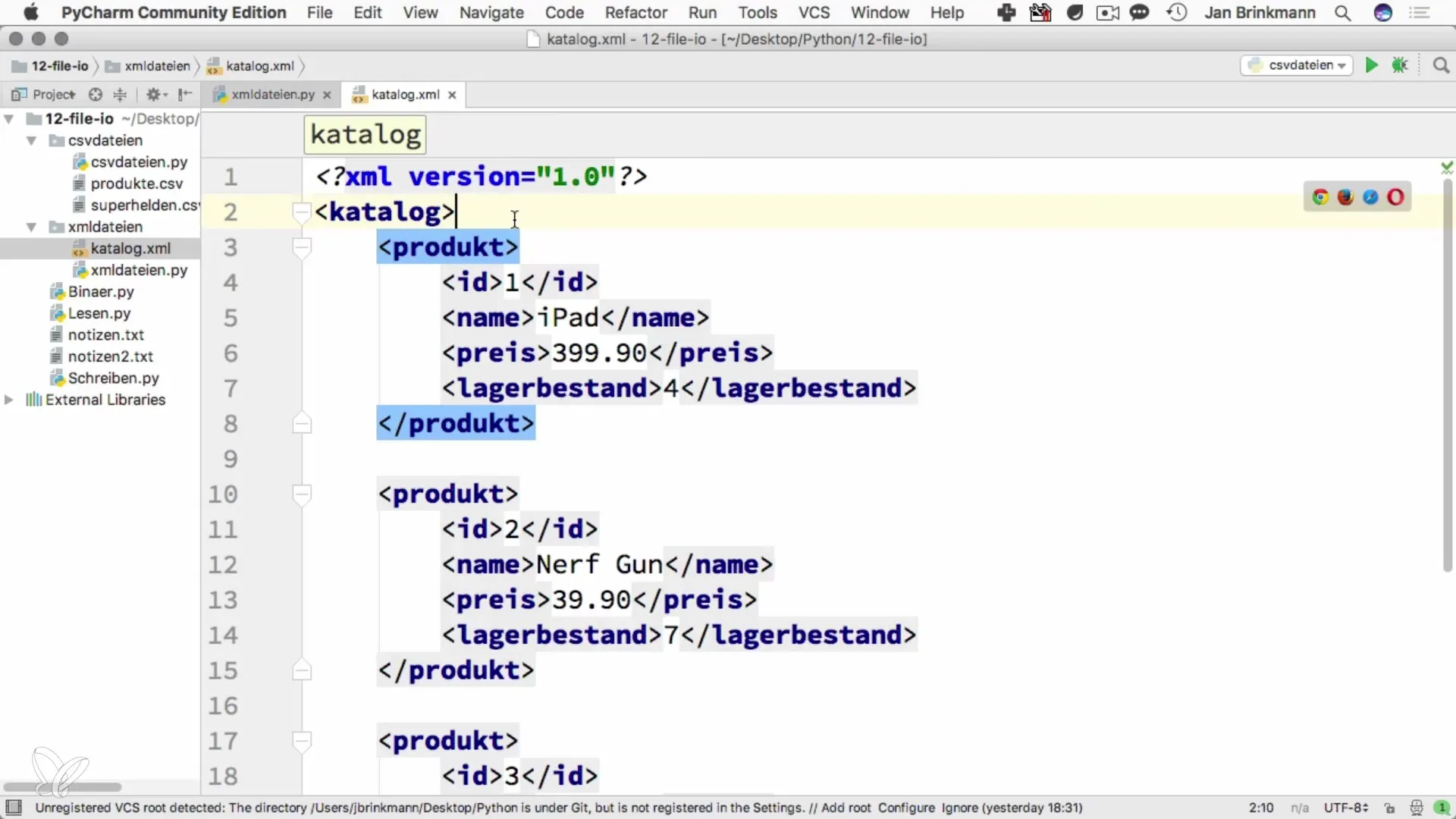
Step 3: Reading the XML file
Now you need to read the XML file that contains the data. This is done using the ElementTree method.
This reads the XML file and stores it as a tree structure in the tree variable. Now you have access to the entire structure.
Step 4: Finding the main node
To proceed, you need the root node of the XML data, which is crucial for your work.
The root represents the catalog node, from which all other nodes depend.
Step 5: Reading product data
Now you can iterate through the product nodes in a loop and query the child information.
This code will return all important information about each product in the catalog. Make sure that access to the properties exactly corresponds to the tag names.
Step 6: Processing attributes (optional)
XML allows the use of attributes in nodes. For example, if you have a product tag with an attribute, you could retrieve it using the get command.
This will return the ID as an attribute.
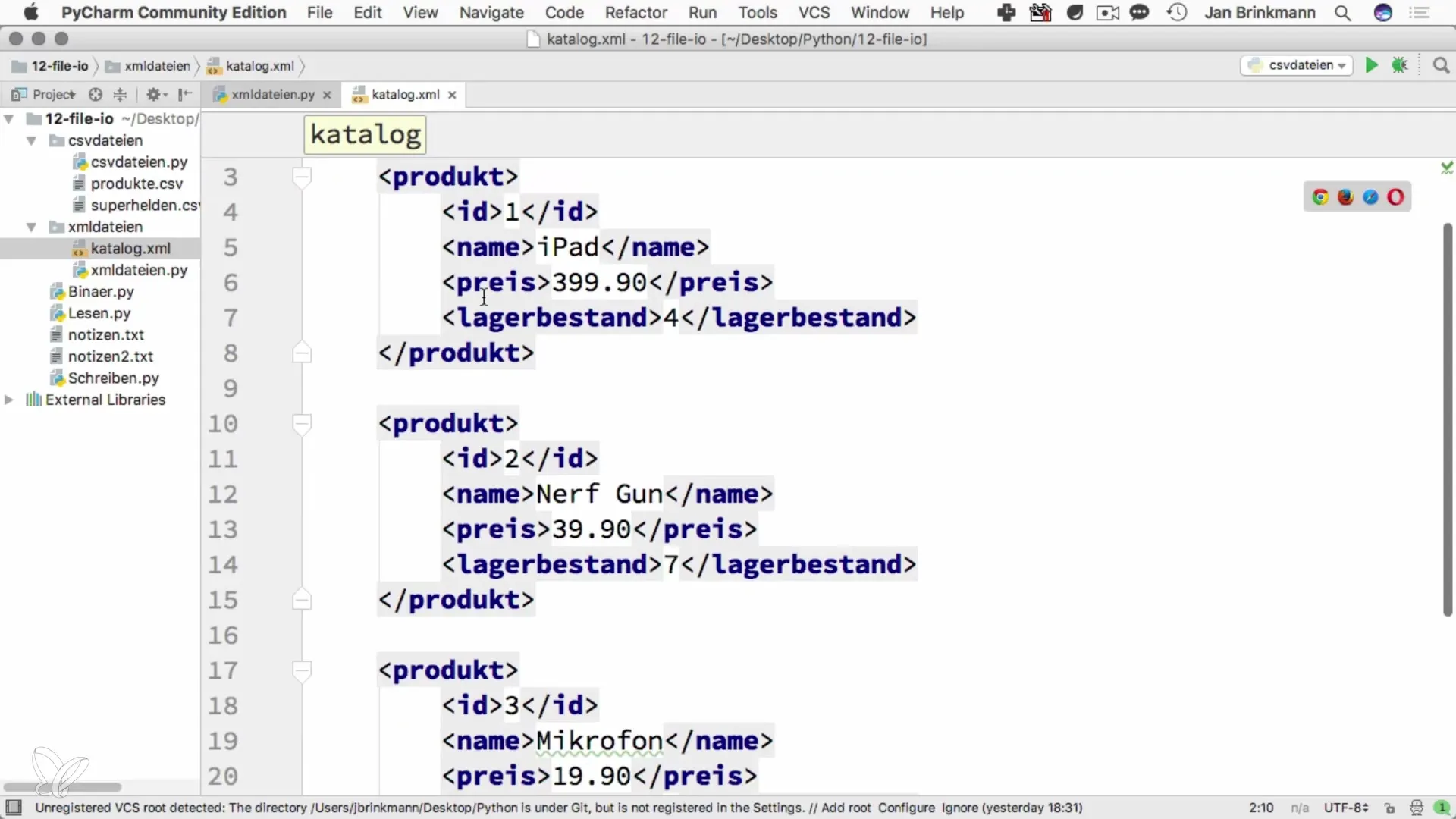
Step 7: Formatting output
To obtain a better overview when outputting the data, you can format the information.
This structure makes it easier to understand the extracted data.
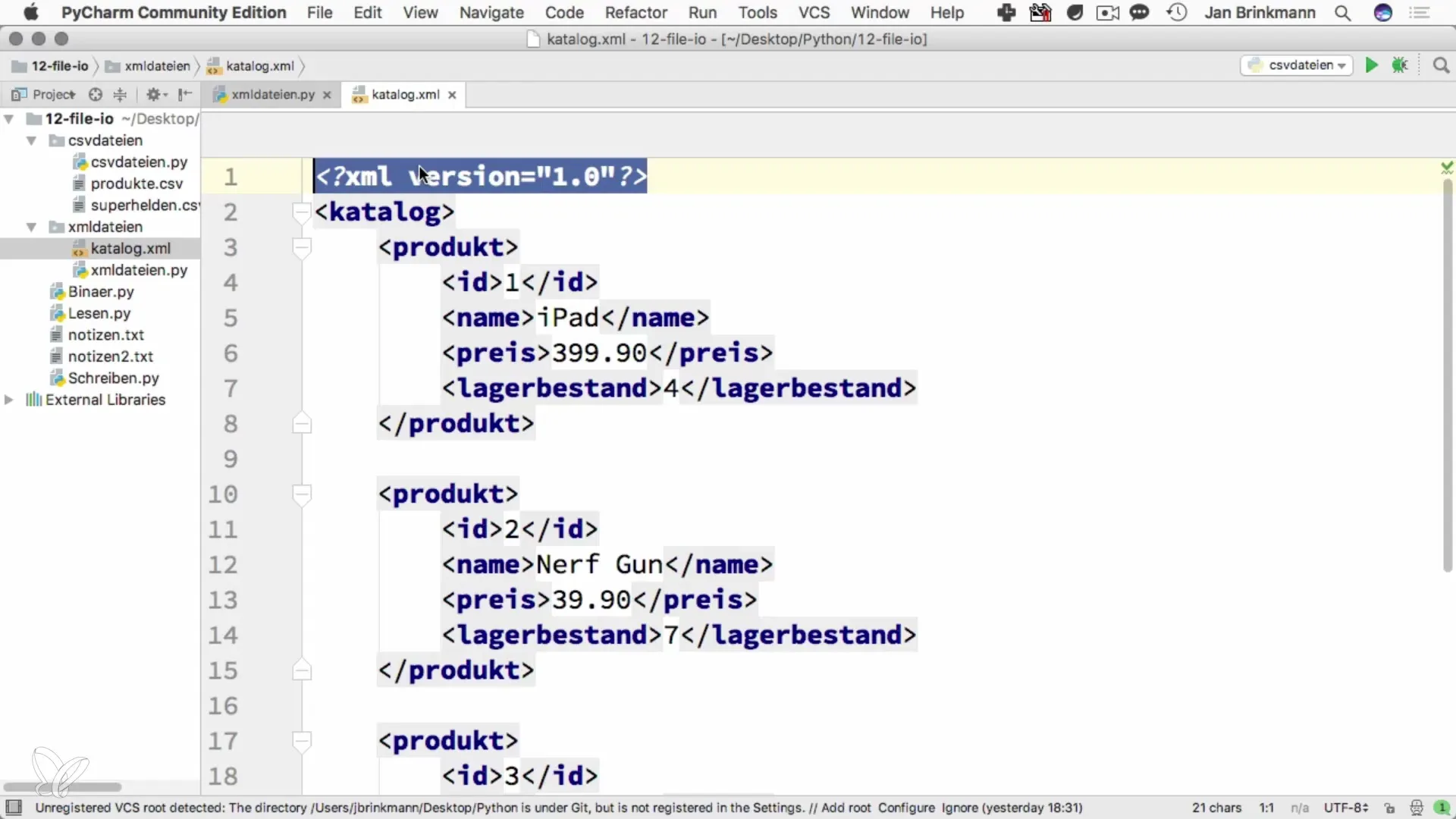
Summary – Mastering XML processing with Python efficiently
In summary, you have learned how to read and process XML data with Python. You now know the basic structure of an XML file, how to find central nodes, and how to effectively extract the information contained – all essential for important data manipulations in Python.
Frequently Asked Questions
How can I process XML data in Python?You can use the xml.etree.ElementTree module to read and manipulate XML data.
Can I read XML attributes?Yes, you can access attributes defined in XML tags using the get command.
Is ElementTree the only module for XML in Python?There are also other modules like minidom and lxml that offer different functionalities.
Can I modify XML data myself?Yes, you can also modify XML data by adding or deleting nodes.