You are at the beginning of your Python journey and want to learn the basic mathematical operations in programming? Programming numerical calculations is the first step into this fascinating world. In this tutorial, you will learn everything important about numerical operators in Python. You will not only learn the standard operations such as addition and subtraction but also more specialized functions like integer division, modulo, and exponents. A solid foundation in these concepts will help you develop more complex programs efficiently.
Key Takeaways
- Python supports various numerical operators for mathematical calculations.
- The basic operations include addition, subtraction, multiplication, and division.
- Special operators like modulo and exponents offer advanced options for processing numbers.
- Integer division treats the results of divisions differently and is important to understand in order to avoid errors.
- Writing calculations compactly with so-called Compound Assignments makes the code cleaner and easier to read.
Step-by-Step Guide
1. Addition – Adding Values
The simplest mathematical operation is addition. In Python, you use the plus operator (+) to add two numbers together.
Here, a will have the value 15.
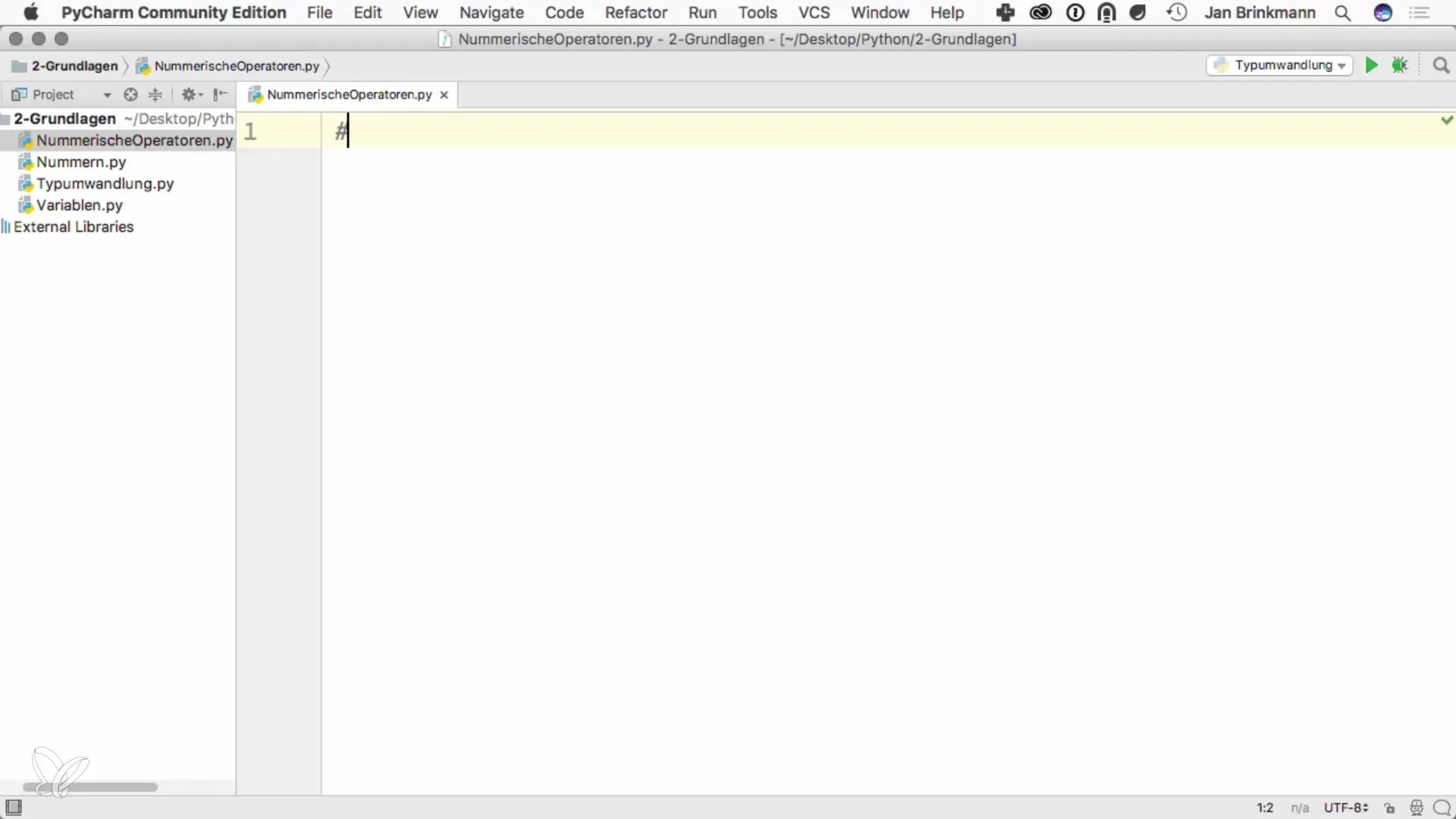
2. Subtraction – Subtracting Values
Subtraction in Python is done with the minus operator (-).
The result for b will be 5.
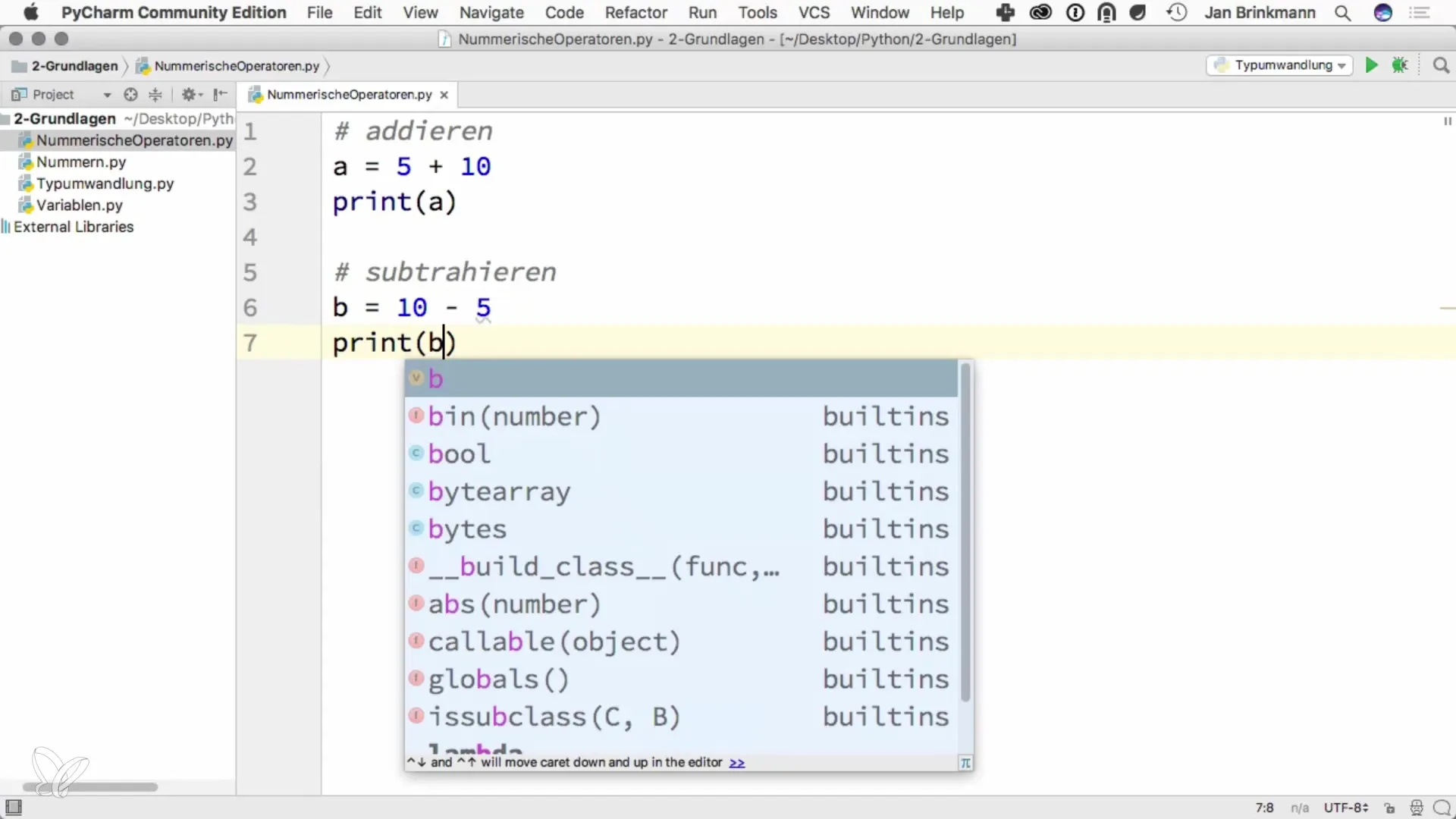
3. Multiplication – Multiplying Values
For multiplication, Python uses the asterisk (*).
The result is 12.
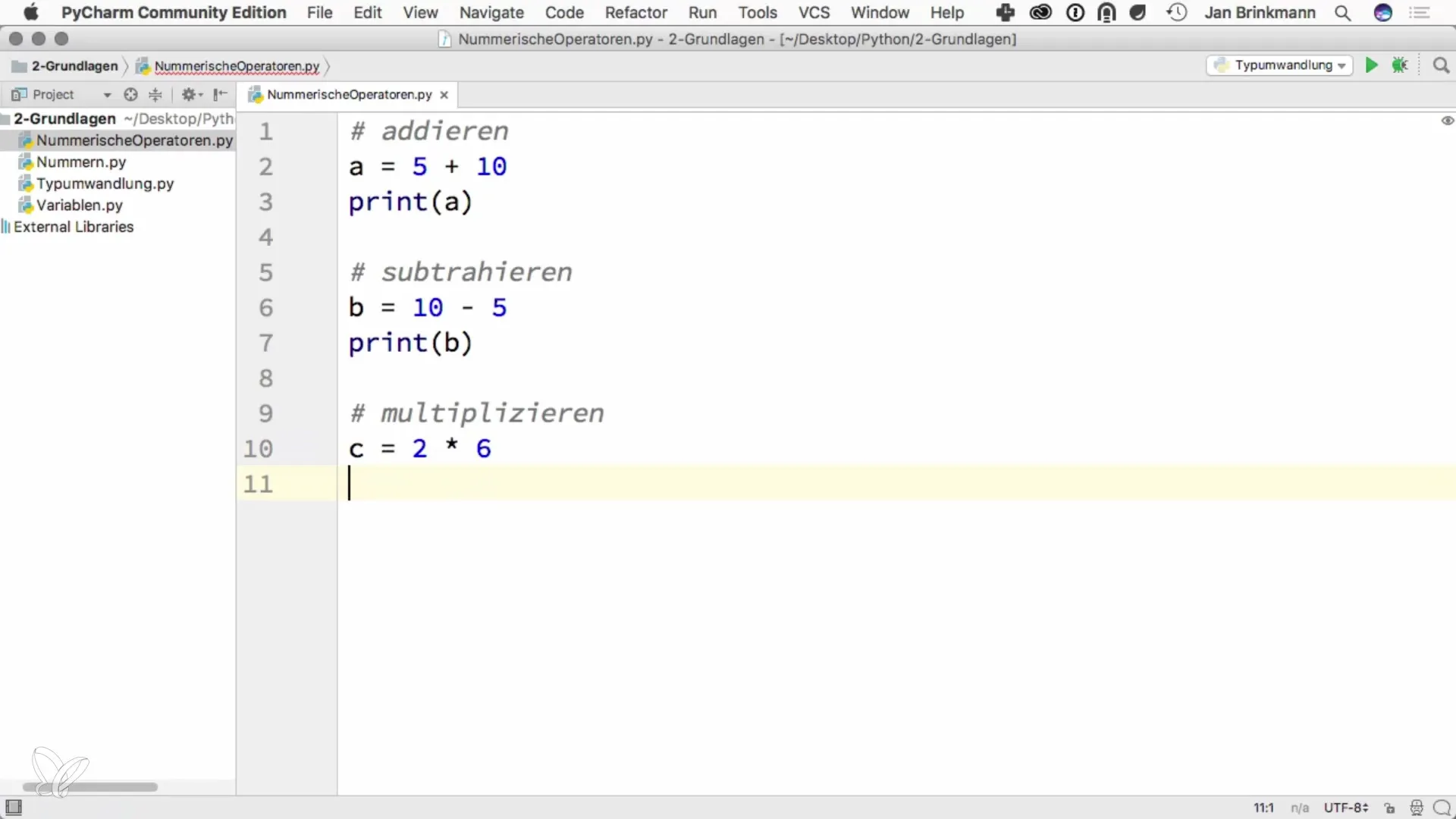
4. Division – Float Division versus Integer Division
Python distinguishes between float and integer division. In float division, the result is given as a decimal number.
The result is 2.5.
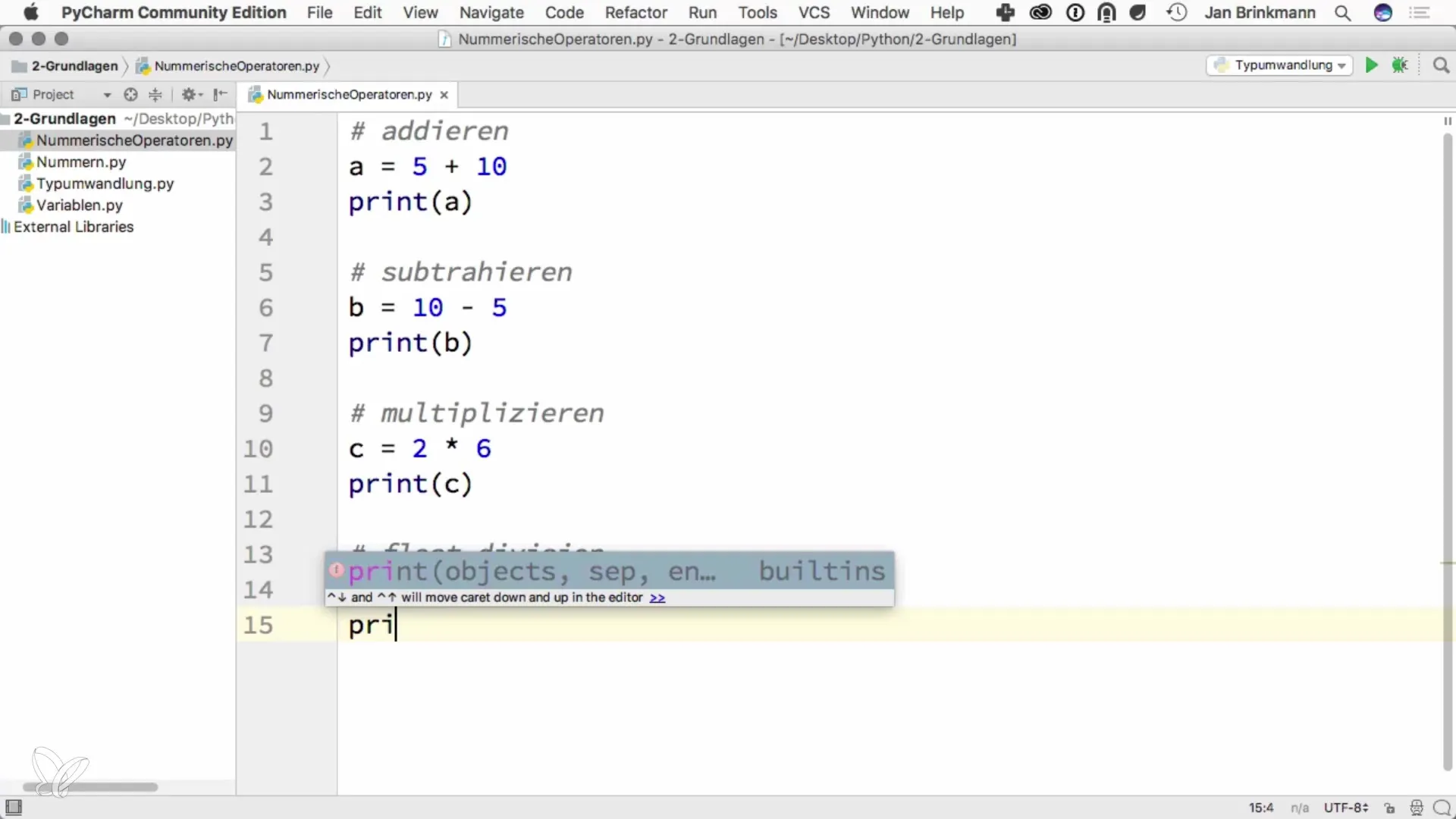
In contrast, integer division (whole number division) with two slashes (//) gives a different result.
Here, the decimal point is cut off, so e has the value 2.
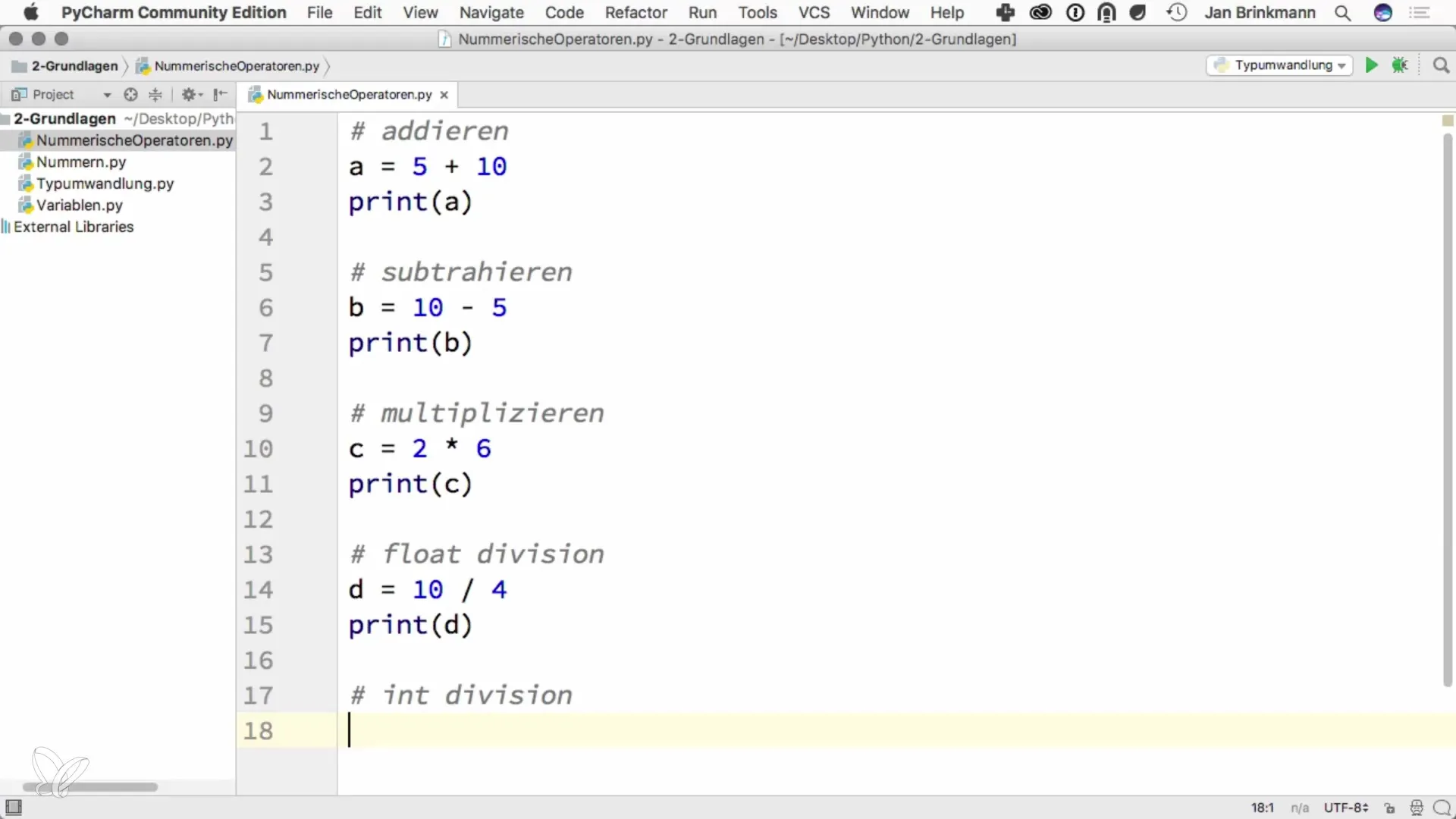
5. Modulo – The Remainder
The modulo operator (%) calculates the remainder of a division.
Here you check how many times 2 fits into 9. The remainder you get is 1.
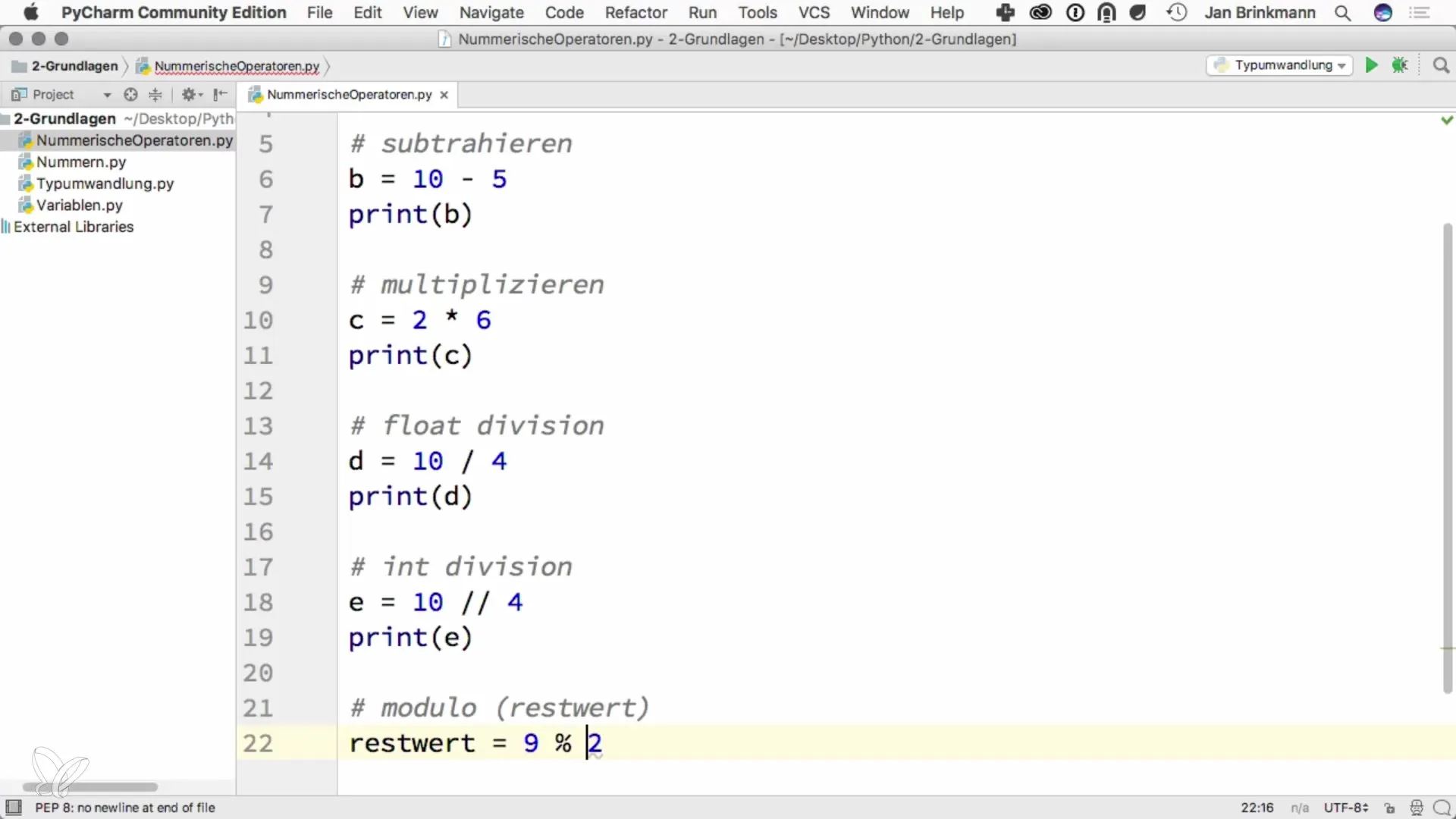
You can also use the modulo operator to check whether a number is even or odd. For an even number, the result of number % 2 equals 0.
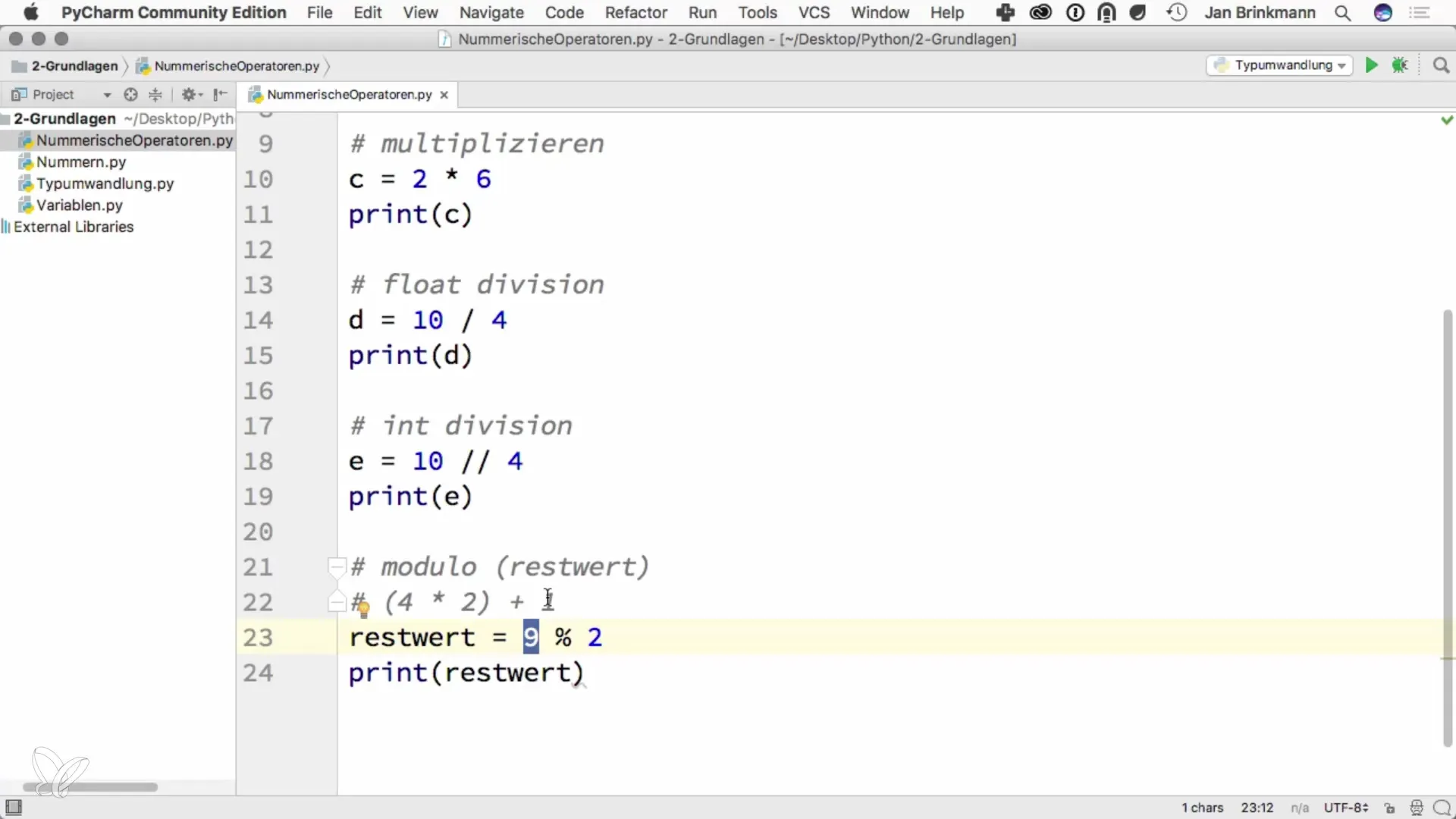
6. Exponents – Calculating Powers
With the exponent operator (**), you can raise a number to a power.
The result is 27, because 3 to the power of 3 equals 3 * 3 * 3.
7. Compact Writing with Compound Assignments
To make calculations more compact, you use compound assignments.
This corresponds to x = x + 2 and the result will be 3.
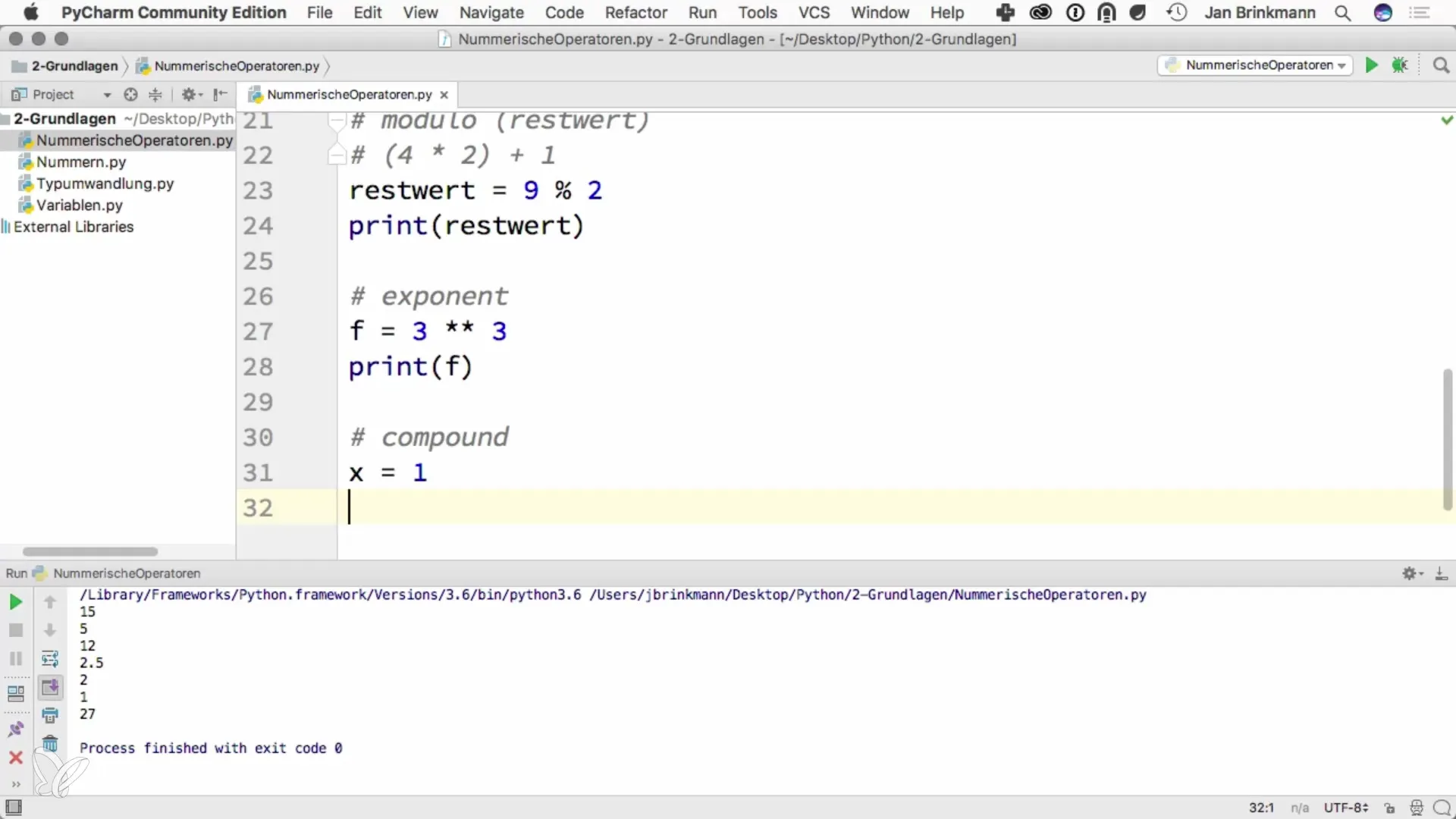
8. Order of Operations – Pay Attention to Parentheses
The mathematical order of calculations is crucial. Note that parentheses are evaluated first, followed by multiplication and division.
Here, the expression results in 25 (not 30), as multiplication takes precedence.
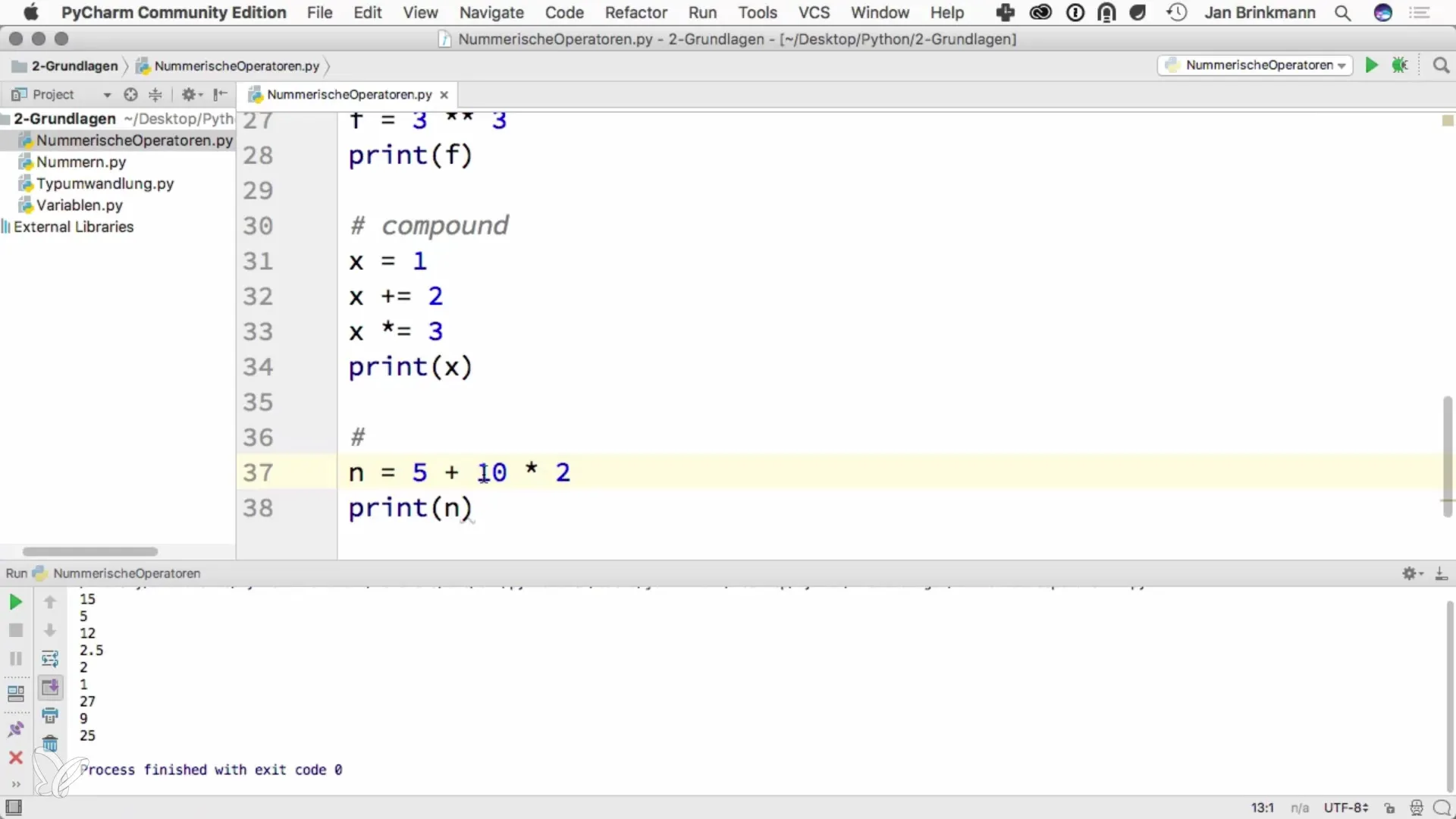
You should use parentheses to influence the operations.
Now you will get 30.
Summary – Successfully Mastering Numerical Operators in Python
In this tutorial, you have learned the most important numerical operators in Python. You now know how addition, subtraction, multiplication, division, modulo, and exponents work. With these basic operations, you can start writing more complex programs. Use the programming power that these operators offer you to solve mathematical problems and further expand your skills.
Frequently Asked Questions
How do I perform a simple addition in Python?Use the plus operator (+), e.g., a = 5 + 10.
What is the difference between float and integer division?Float division returns the result as a decimal number, while integer division truncates the decimal places.
How can I calculate the remainder of a division?Use the modulo operator (%), to get the remainder, e.g., 9 % 2.
How do I correctly use exponents in Python?Use two asterisks (), e.g., 3 3 equals 27.
What do compound assignments do in Python?They allow for a compact way to write calculations, e.g., x += 2 instead of x = x + 2.