Functions are central to programming and play a crucial role in structuring your code. They allow you to write reusable code, separate tasks, and enhance the readability of your program. In this guide, you will learn how to define functions in Python, how to pass parameters, and how return values work.
Key takeaways
- Functions are defined using the keyword def.
- A function can accept parameters and return values.
- The return value is specified with the keyword return.
- Indentation in Python is important for defining the block of a function.
Basics of Function Definition
You start a function in Python with the keyword def, followed by the function name and an empty parameter list in parentheses. Here is an example of how to define a simple function called Feierabend:
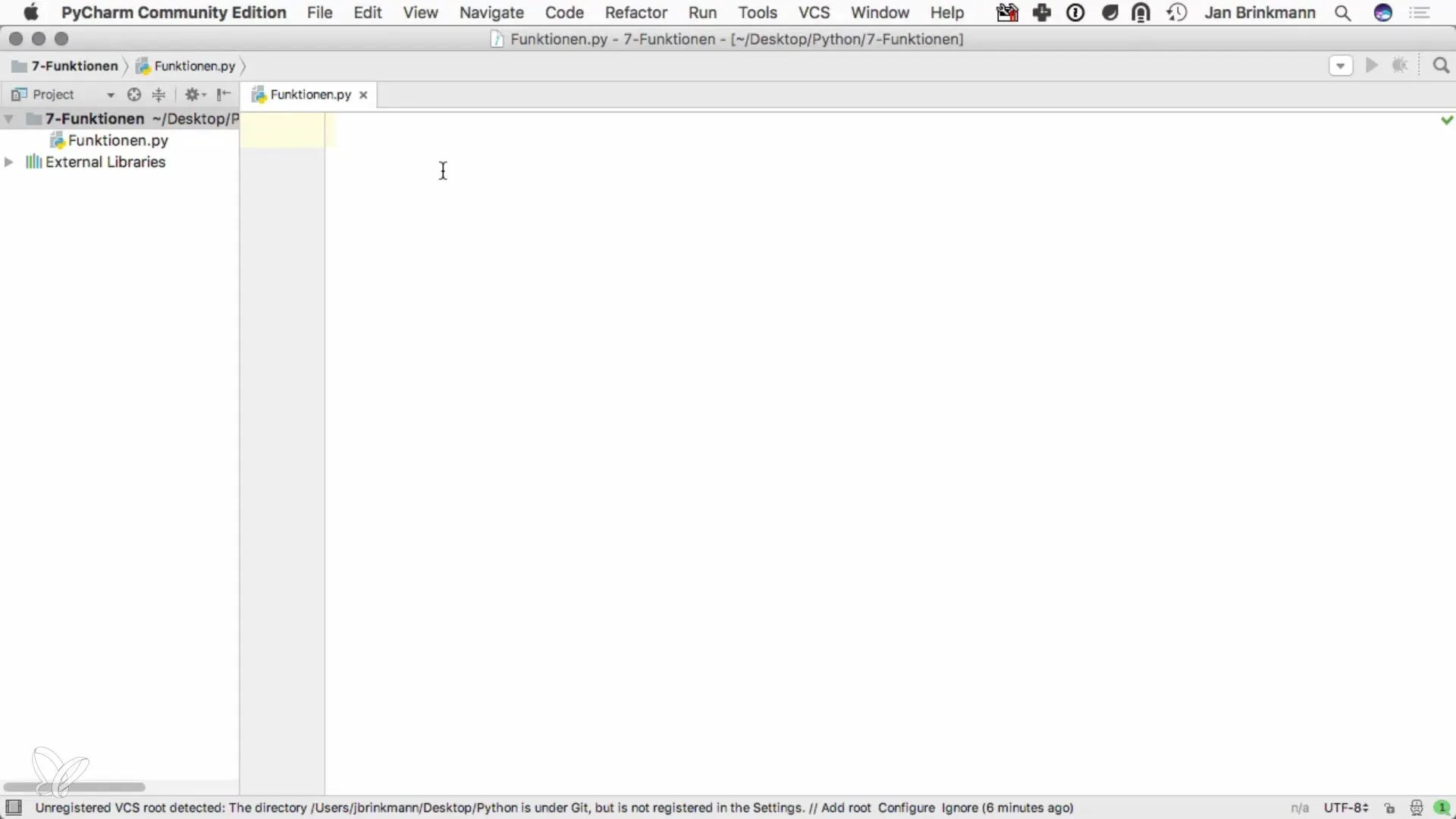
You can also define an empty function, but you must use the keyword pass to tell Python that the function is empty and contains no specific code.
To execute your function, simply call its name followed by the parameter list. If the function does not contain return values, initially nothing visible happens until you implement the function.
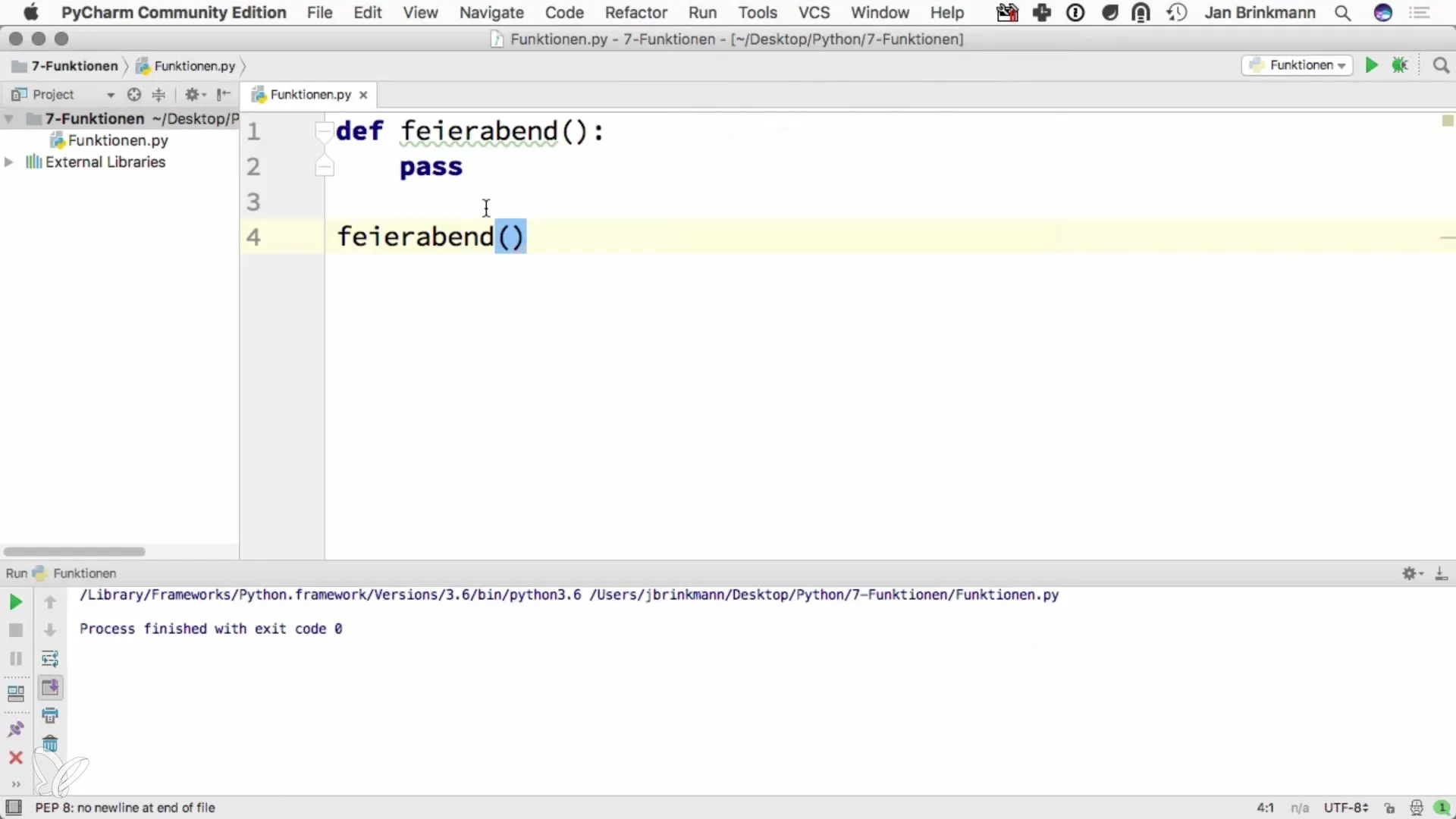
Functions with Return Values
A great way to enhance the functionality of your programs is to use return values. These values can then be utilized in other parts of your code. For example, let's say you want to create a function that returns age. You can do this with the keyword return.
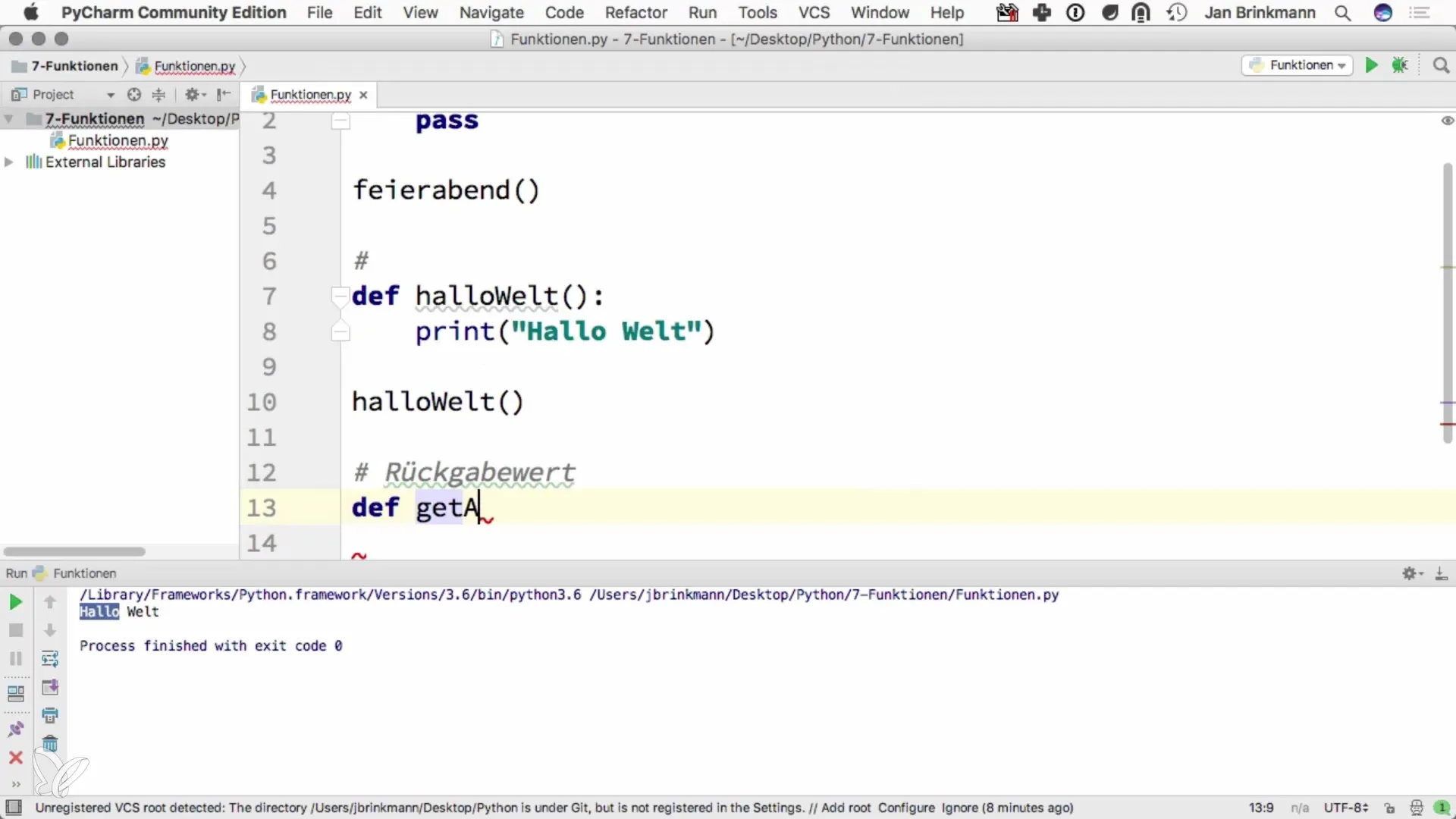
Here is an example of a function get_alter that returns a fixed value, such as 21. This return value can then be checked in conditions.
For example, you can check if the returned number is greater than or equal to 18 and generate an output accordingly.
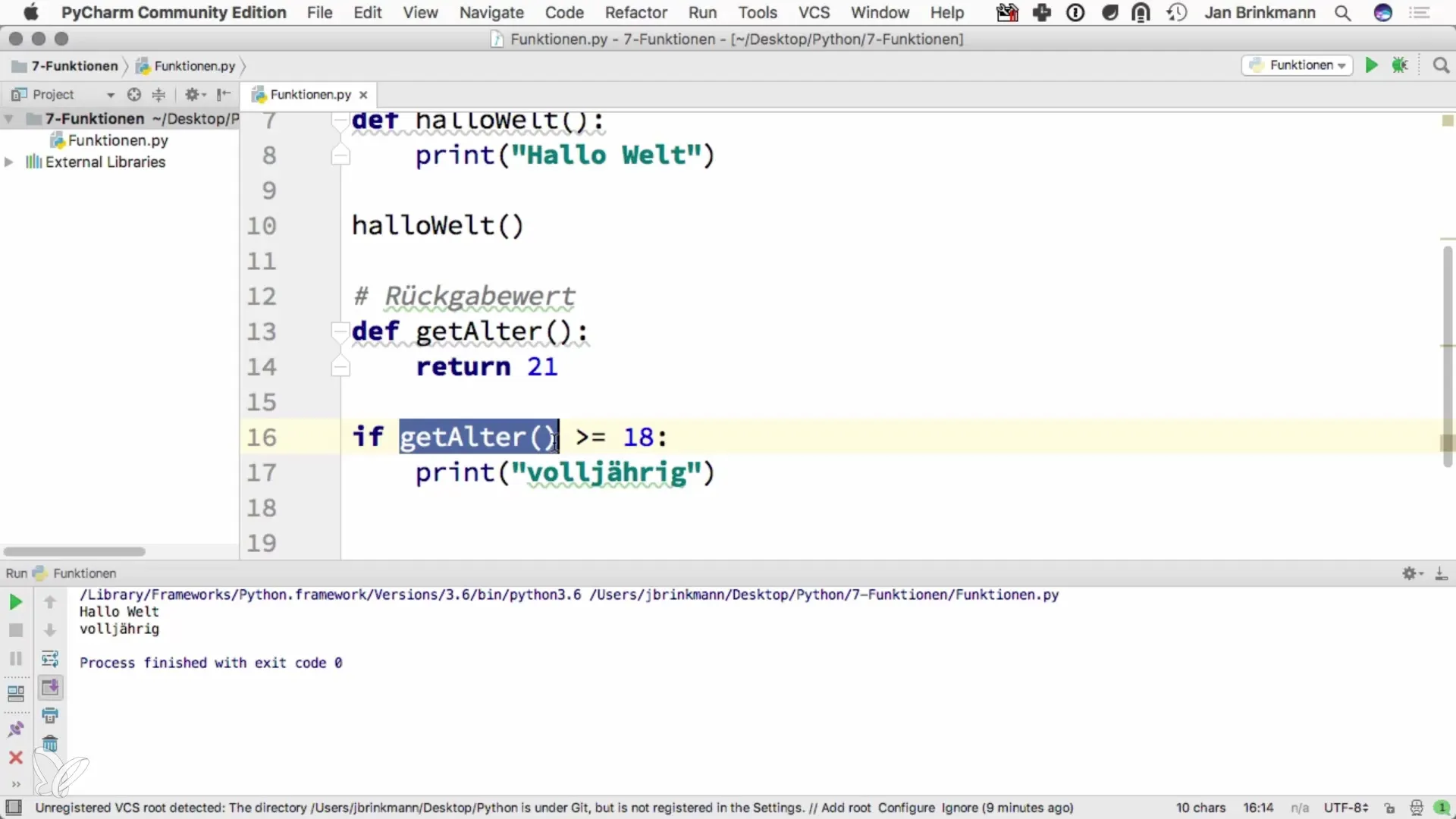
You also have the option to store the return value of a function in a variable. This allows you to reuse the value multiple times.
Functions with Parameters
To increase the flexibility of your functions, you can use parameters. Parameters are essentially variables that are passed to your function. For example, if you want to create an add function to add two numbers, define the function with parameters.
When you call the function, these parameters can be used to return the result. For example, you can define x + y in your function to compute the sum of the two values.
Remember that a function can also contain multiple lines of code, so you can incorporate more complex logic into your function.
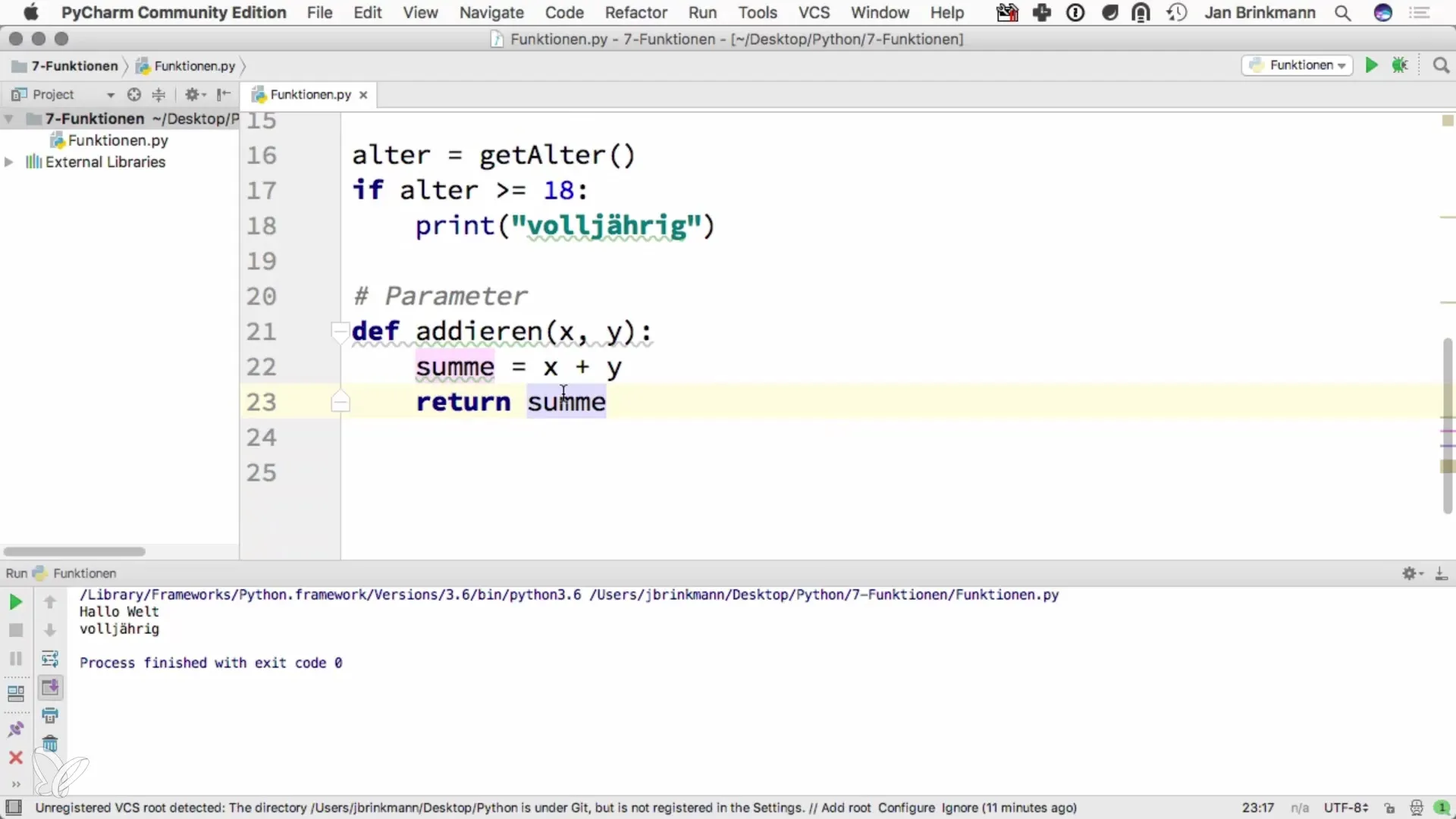
Indentation in Python
An important concept in Python is indentation. It is not only stylistic but also functional – the compiler uses indentation to recognize the block of a function.
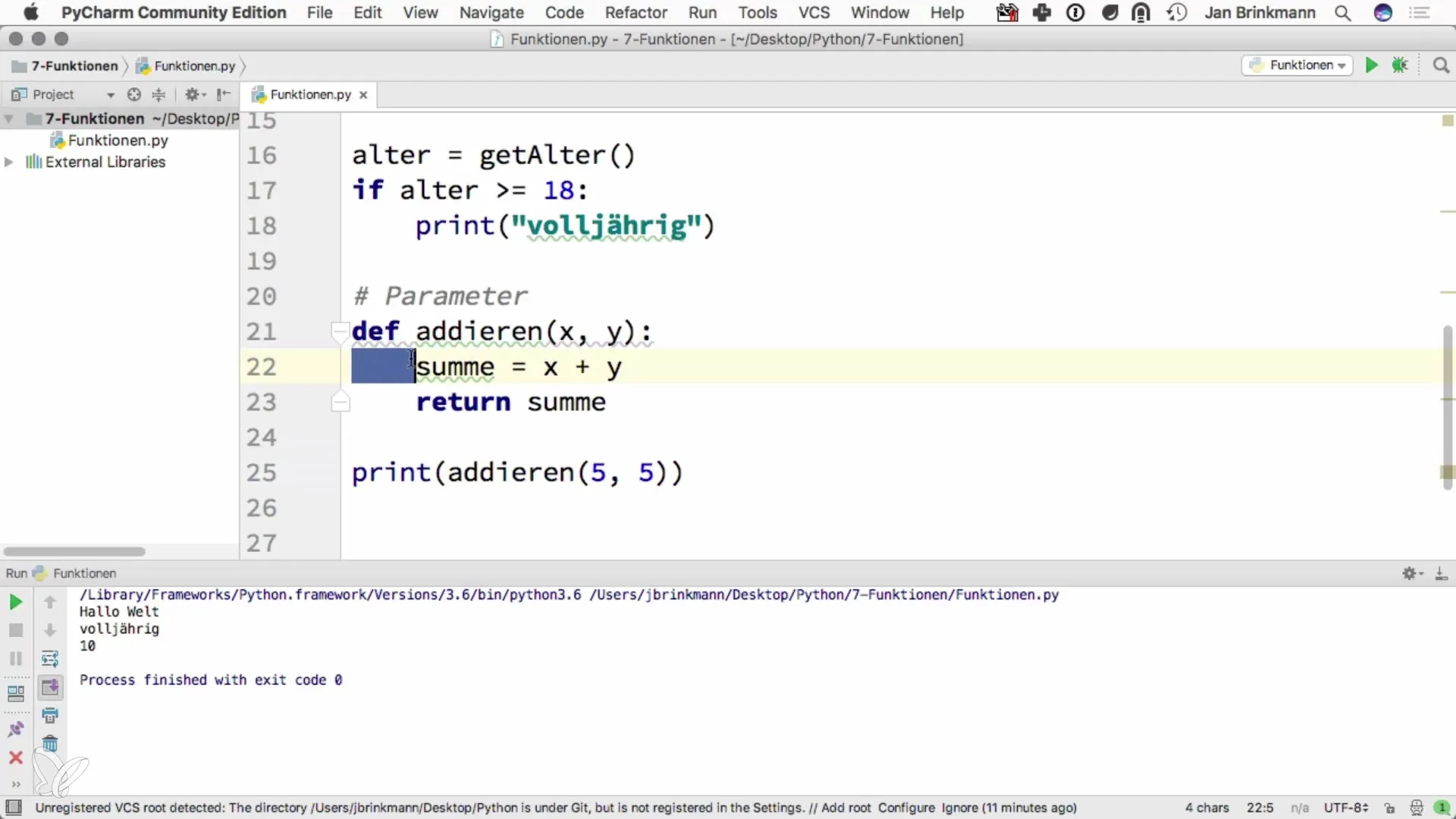
If you do not indent the code correctly, you may receive an error message indicating that a statement is not in the correct context.
Make sure that all statements belonging to a function are correctly indented and placed in the right block. This ensures that your code executes as intended.
Summary – Python Programming: Functions with Parameters and Return Values
The use of functions in Python is fundamental to programming. You have learned how to define functions, how they can accept parameters and provide return values, as well as the importance of indentation in your code. These concepts are essential for writing efficient and readable code.
Frequently Asked Questions
What is a function in Python?A function in Python is a block of reusable code that you can define and execute.
How do I define a function?A function is defined with the keyword def, the name of the function, and a parameter list in parentheses.
What is the difference between parameters and return values?Parameters are variables passed into the function, while return values are the values returned from the function.
What happens if I forget the return of a function?If you forget return in your function, the return value will be None unless you simply output another value.
Why is indentation important?Indentation is important because it defines the structure of your code and tells the interpreter which lines belong to which functions.