Working with CSV-files (Comma-Separated Values) is a common task in programming that manifests in many practical applications. Whether you are retrieving data from APIs or managing inventory — the ability to read and write CSV files is indispensable. In this guide, you will learn how to effectively process CSV files with Python. We will look at the creation of CSV files, reading data, and manipulating that data.
Main findings
- Module overview: Python offers a built-in CSV module that provides CSV readers and writers.
- Data structure: You can use both lists and dictionaries to store and process data.
- Efficient I/O handling: Use with open(...) for file access management to avoid worrying about closing the files.
Step-by-step guide
Create a directory and generate a CSV file
To begin, create an empty directory. In this directory, you should create a file named csvdateien.py. This file will be your main script for working with CSV files.
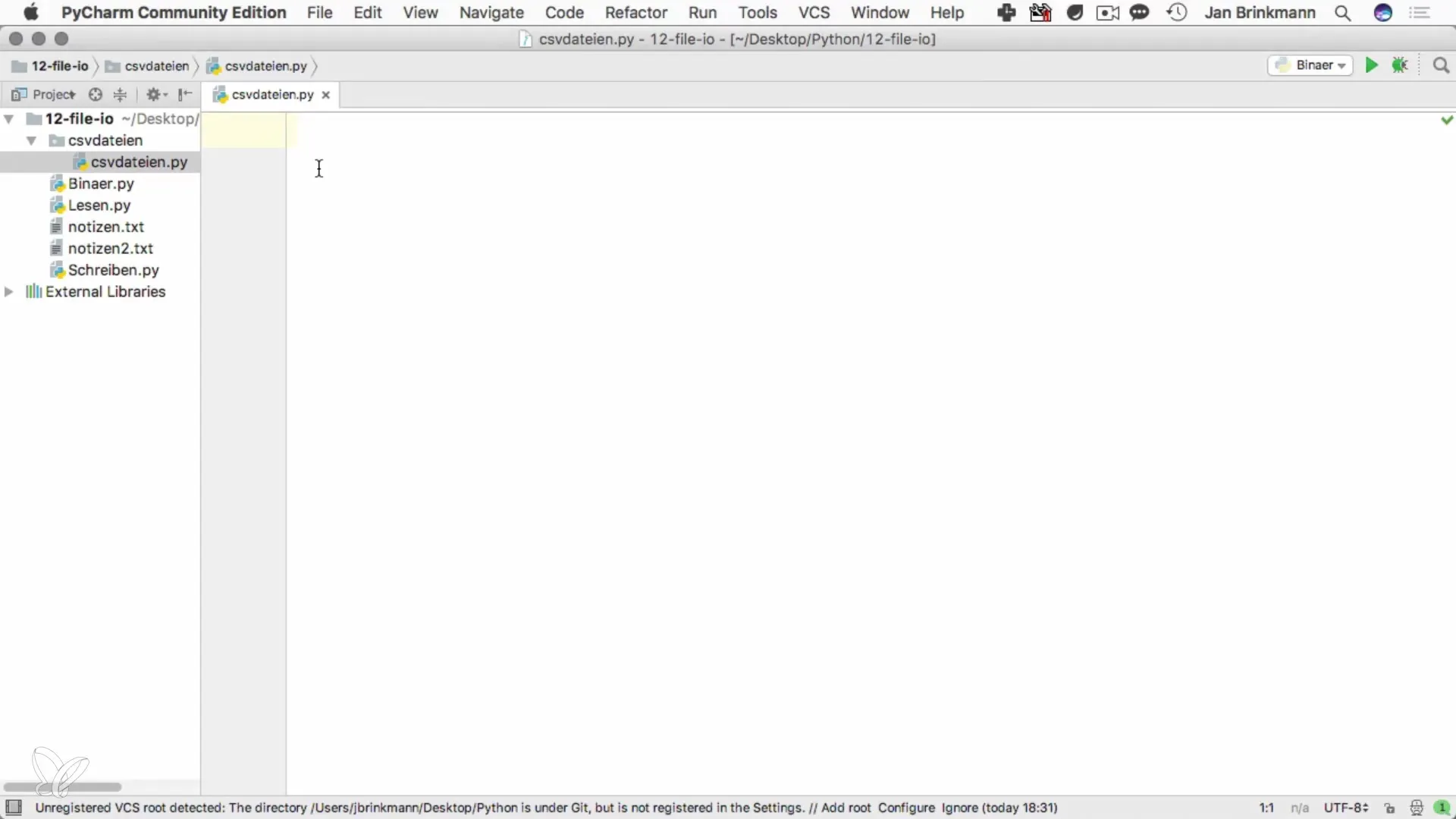
Within your Python file, you can create the CSV file using the name produkte.csv. In this file, you will define the columns with the headers Product ID, Name, Price, and Stock.
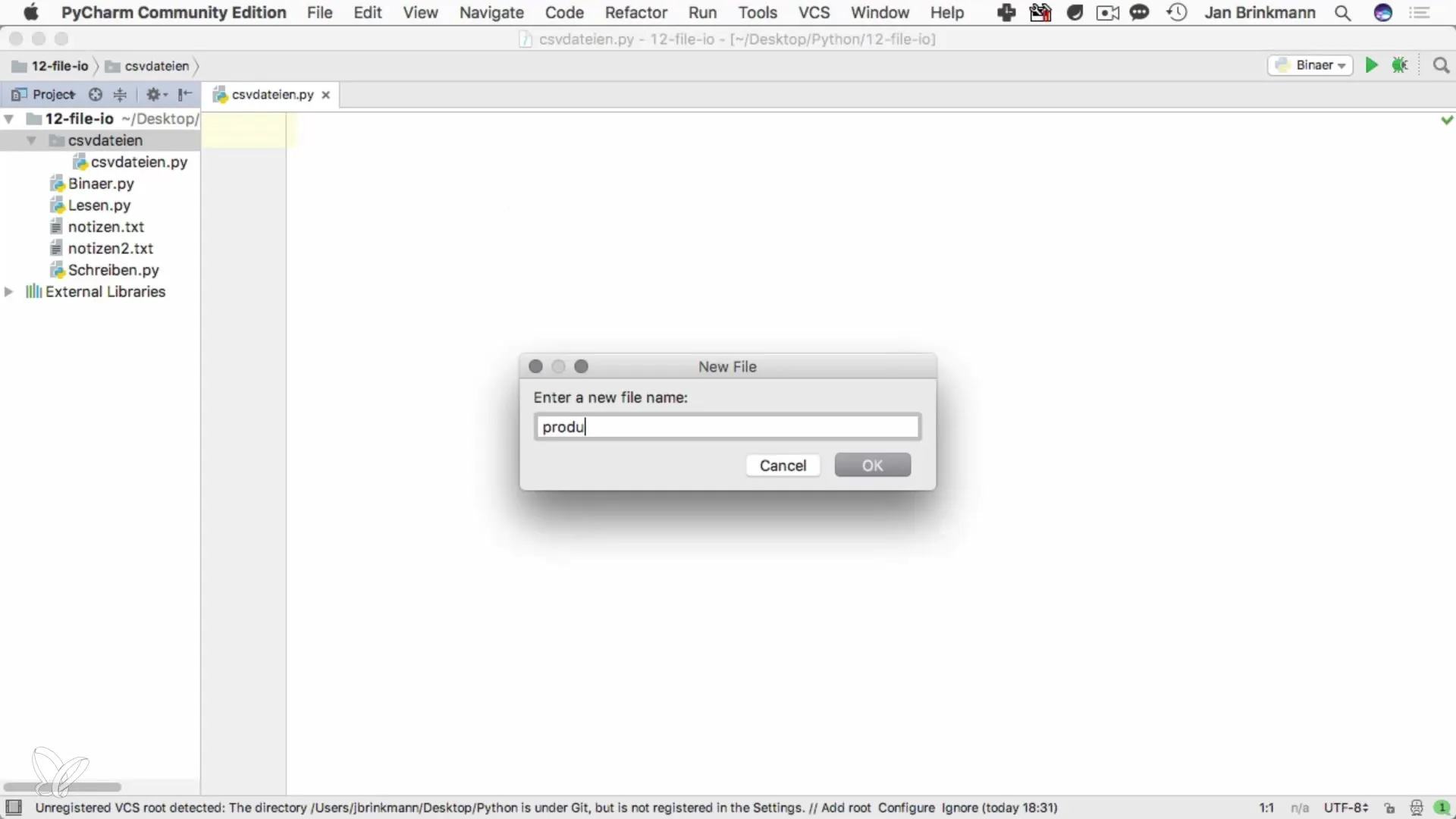
The CSV file might look something like this:
Product ID,Name,Price,Stock 1,iPad,399,50 2,Nerfgun,29.99,100
Read CSV file
To read data from the produkte.csv file, you need the CSV module. The module allows you to process CSV data efficiently. You can convert the data into a readable format by using with open(...). This avoids the manual closing of file objects and makes your code cleaner and safer.
In the next step, you will create a CSV reader. This allows you to iterate through the file line by line and extract the data:
with open('produkte.csv', newline='', encoding='utf-8') as csvfile: csvreader = csv.reader(csvfile) for row in csvreader: print(row)
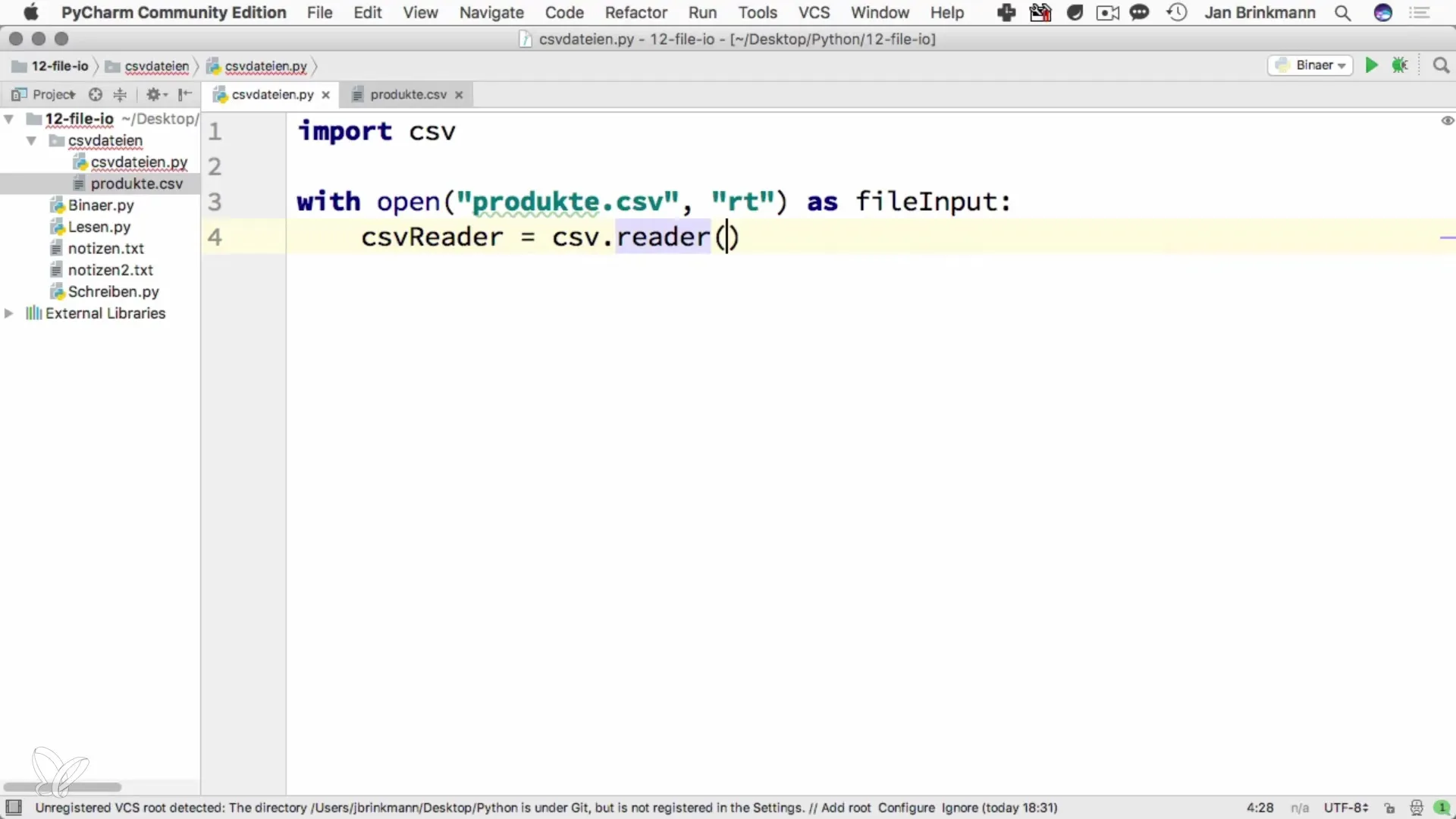
Here, each line is read as a list, indicating that the data is structured as expected. Each line corresponds to a product and contains information about ID, Name, Price, and Stock.
Using dictionaries for more intuitive data processing
To handle the data more simply and intuitively, you should use the dictionary format. With a dictionary reader, you can make accessing your data easier using the field names.
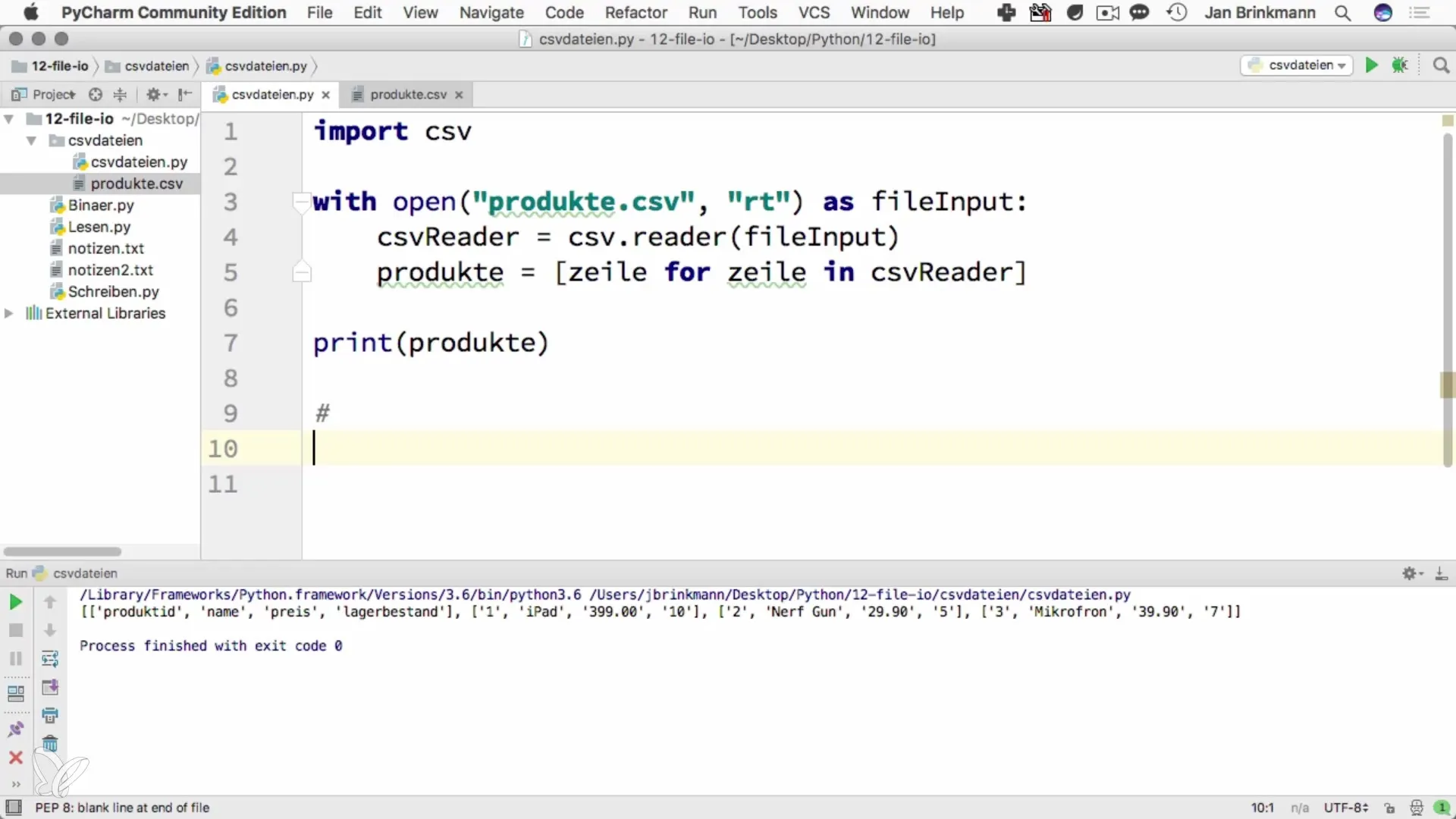
Here, you also need with open(...) to open the file and create the CSV reader. Make sure to specify a list of the fields you want to read:
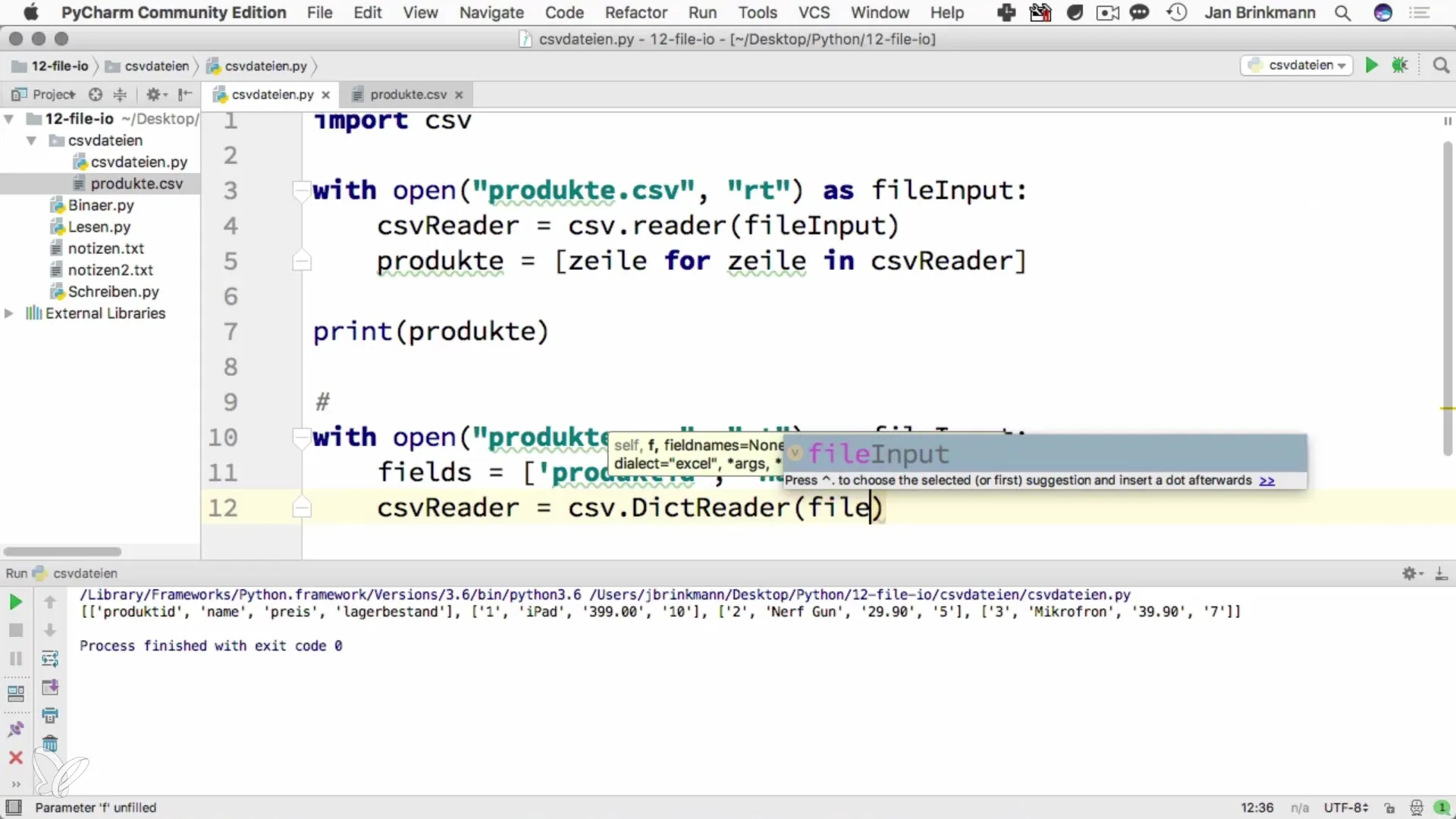
Now you can easily access the fields by their names. This makes your processing significantly more accessible.
Writing CSV file
To write your own data to a CSV file, you can use a dictionary writer object. You have the option to create a list of dictionaries that represent your data.
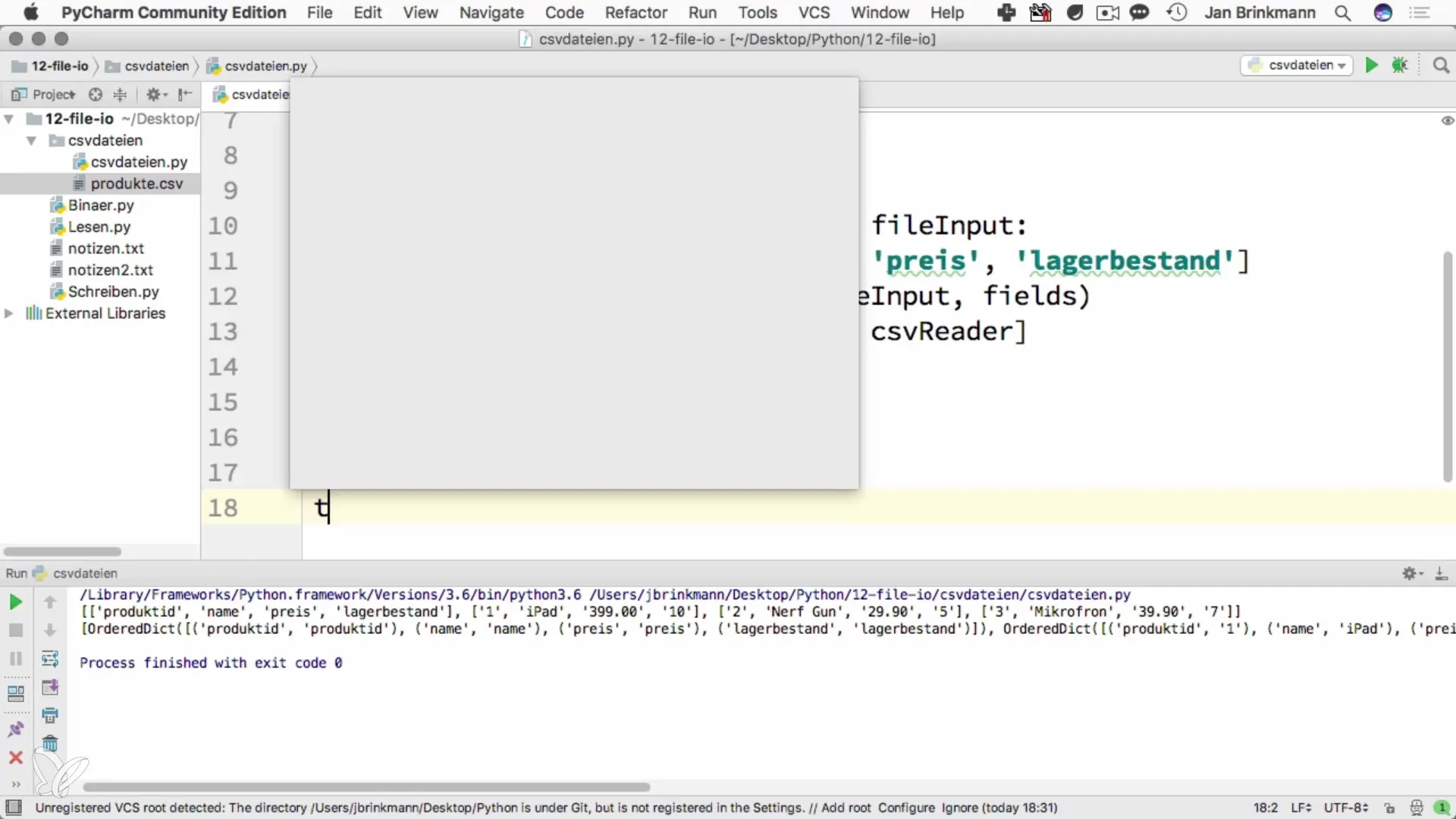
Here is a simple example of how to save a list of superheroes as a CSV file:
with open('superhelden.csv', 'w', newline='') as csvfile: fieldnames = ['Name', 'Alias'] writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
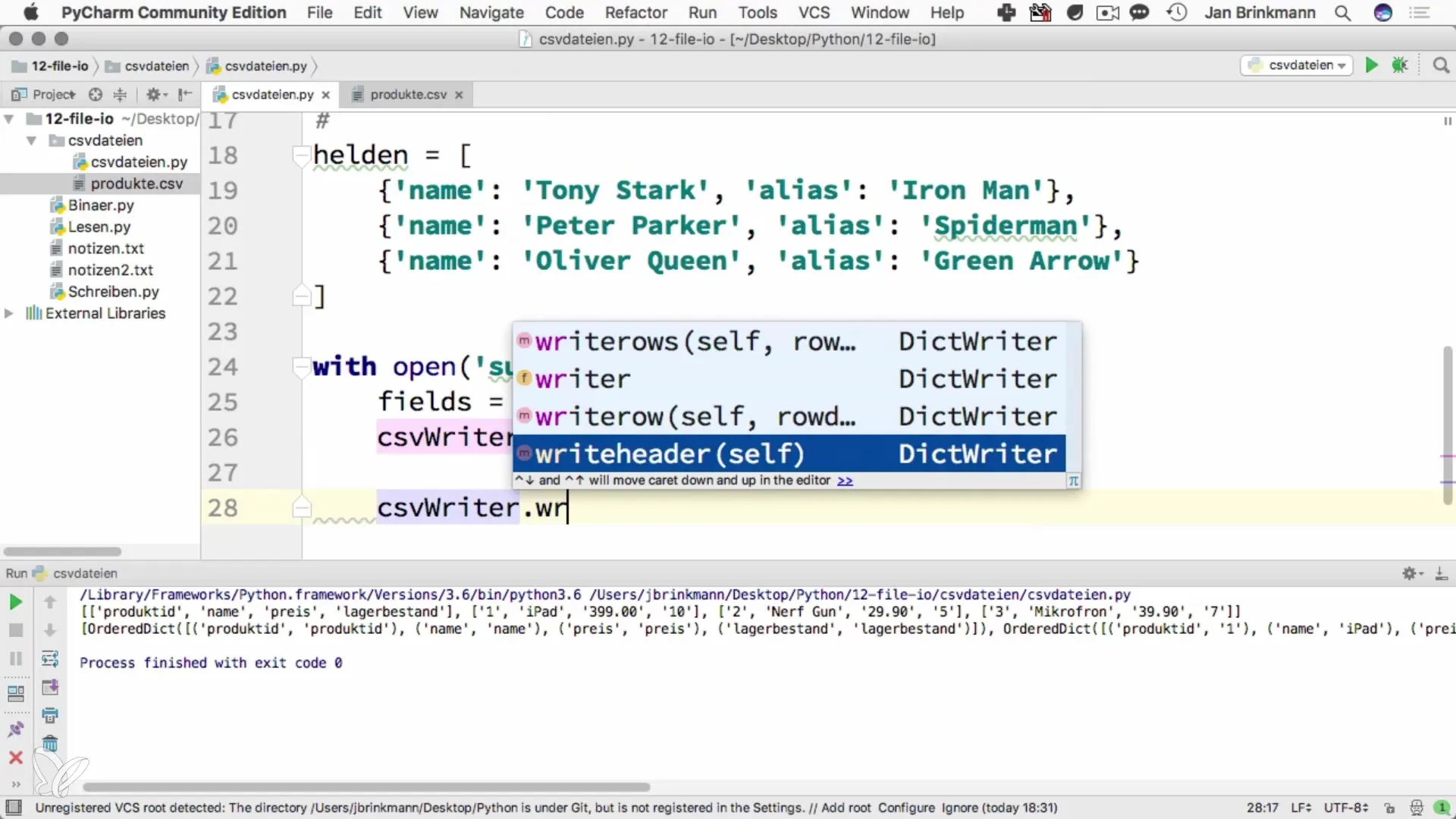
The file superhelden.csv will now be created and will contain the data of the superheroes in a well-structured, readable format.
Conclusion and practical application
CSV files are a valuable tool for data exchange that is often required in practice. This guide provides you with the basics for processing CSV files with Python. You have learned how to read data from CSV files, process it in Python, and write your own data to the CSV format.
Summary – Processing CSV files with Python
CSV files are an important format for data exchange in many applications. With Python, you can work with these files efficiently and easily. Once you understand the basics, you can easily apply these techniques to complex data applications.
Frequently Asked Questions
How do I open a CSV file in Python?You can open a CSV file in Python with with open('filename.csv', 'r'):
How can I write data to a CSV file?For that, you use the csv.writer or csv.DictWriter object to format and write the data to the file.
What is the difference between csv.reader and csv.DictReader?The csv.reader reads the data as lists, while csv.DictReader outputs the data as dictionaries with field names as keys.
How can I set the header in a CSV file?The header can be specified using writeheader() while writing a CSV file through the writer.
How do I read a CSV file with custom field names?Pass a list of the desired field names when creating a csv.DictReader.