Strings are a central part of any programming, and Python offers you a variety of methods to manipulate and analyze these strings. In this guide, you will learn how to work effectively with strings in Python. You will discover how to create strings, edit them, access components, and apply basic string functionalities. Let's dive right in!
Main Insights
- Strings in Python can be processed multiple times.
- You can access and manipulate individual characters of a string.
- There are a variety of methods to change, replace, and analyze strings.
- You can create substrings and query specific characters using indices and step sizes.
- The length of a string can be easily determined.
Step-by-Step Guide to Working with Strings in Python
Strings and Their Concatenation
Strings can be easily created and modified in Python. A particularly fascinating feature is the ability to multiply strings. For example, if you multiply the letter "h" four times, you get "hhhh". This is not a numeric multiplication, but rather a string concatenation.
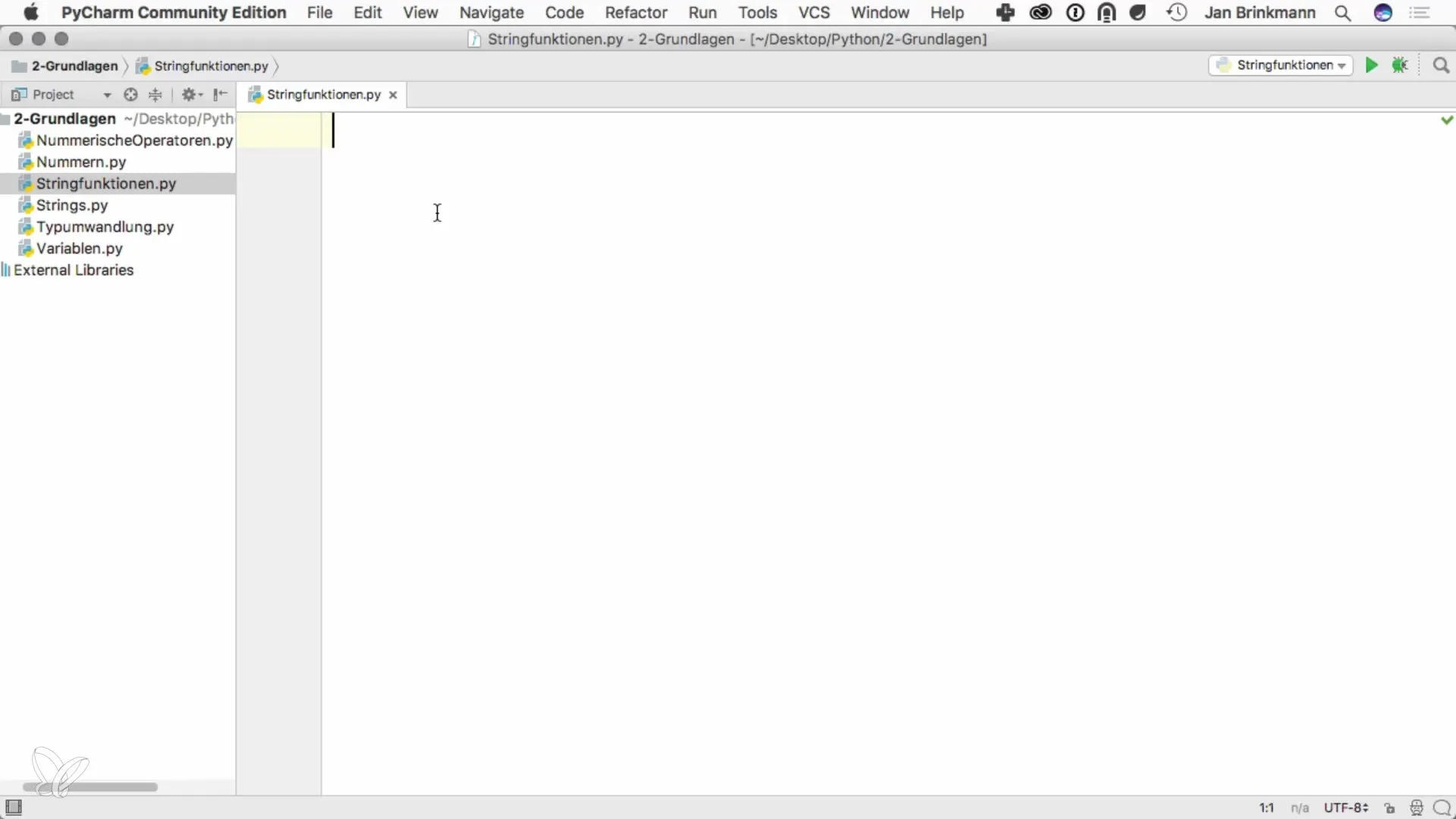
You will see that Python effectively utilizes this method to join strings together.
Accessing Individual Characters
Another exciting aspect is accessing individual letters within a string. This is done through indices, where the first index always starts at 0.
You can retrieve the first element, "A", with index 0, the second element, "B", with index 1, and so on.
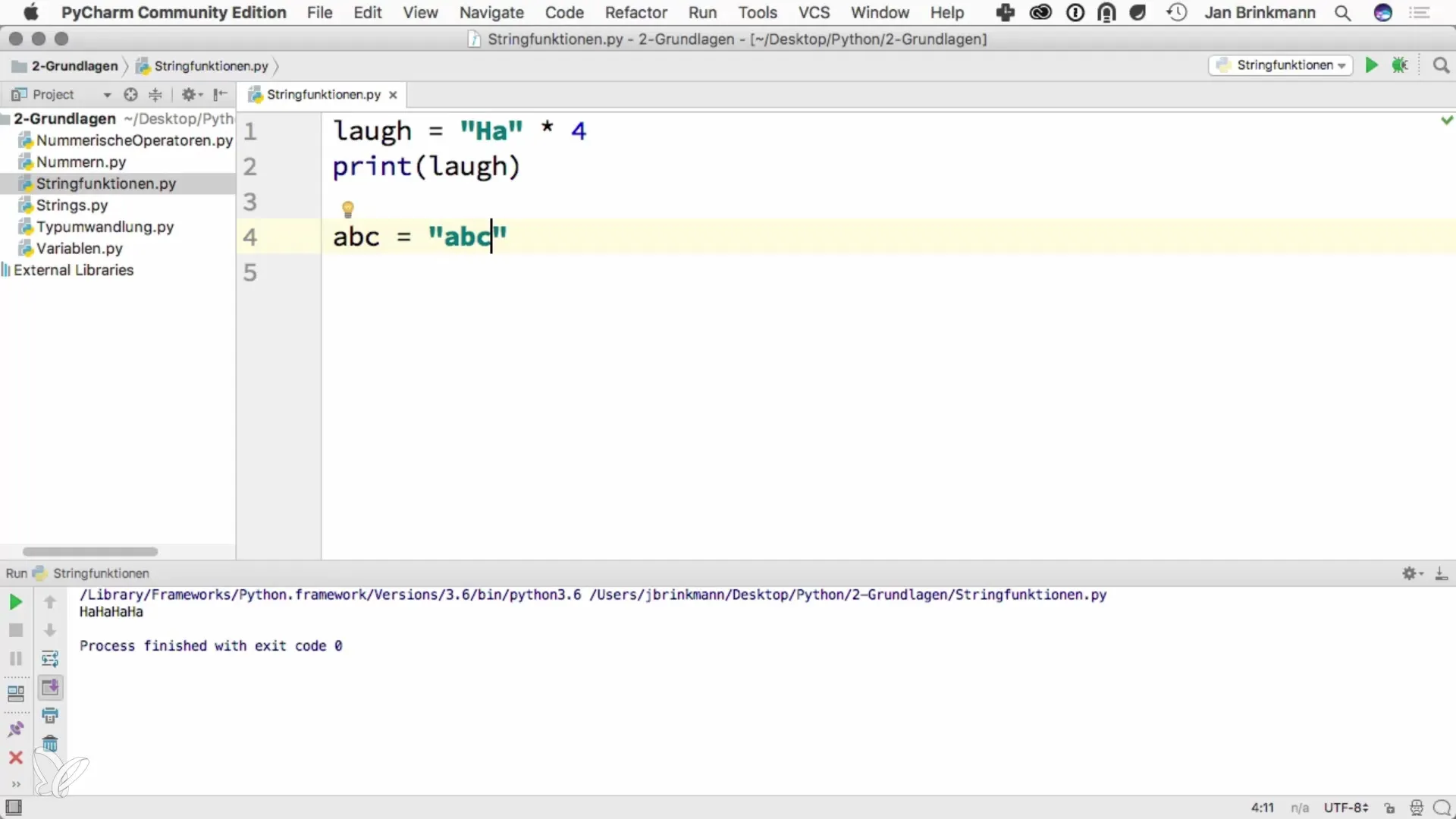
This has the advantage of allowing you to access characters flexibly.
Reverse Access
In addition to forward access to letters, it is also possible to access the letters in reverse order. The index -1 returns the last element, -2 returns the second to last, and so on.
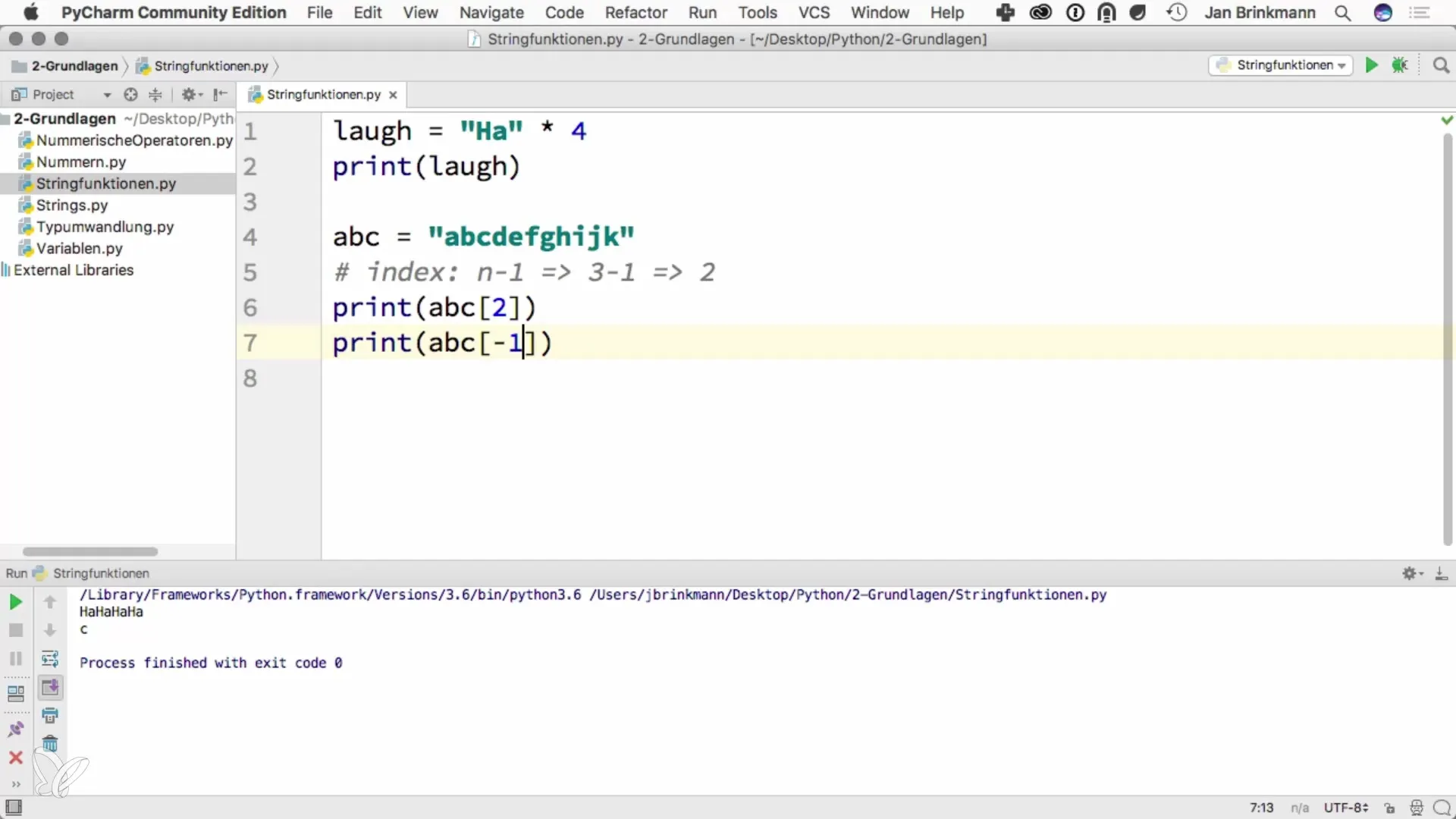
Replacing Substrings
A common requirement when working with strings is replacing certain characters or substrings. Python offers a simple method that you can use: replace().
For example, if you want to change the string "Star Trek" to "Star Trek TNG", you can do so as follows:
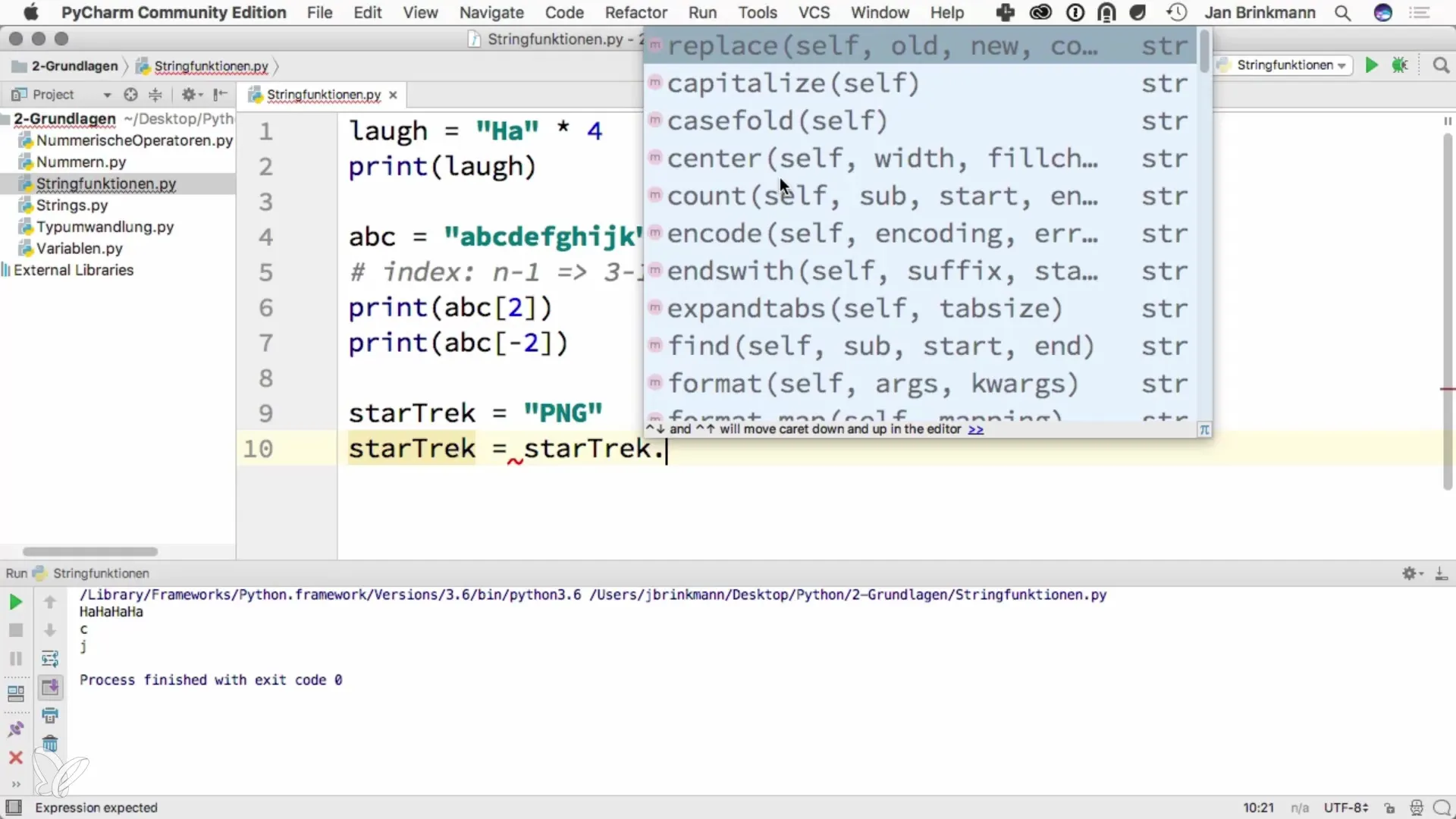
Creating Substrings
Often, it is helpful to extract only a part of a string – for this, you use substrings. You can specify with indices where you want to retrieve the characters from.
Assuming you have the string "Hello World" and want to extract only "Hello":
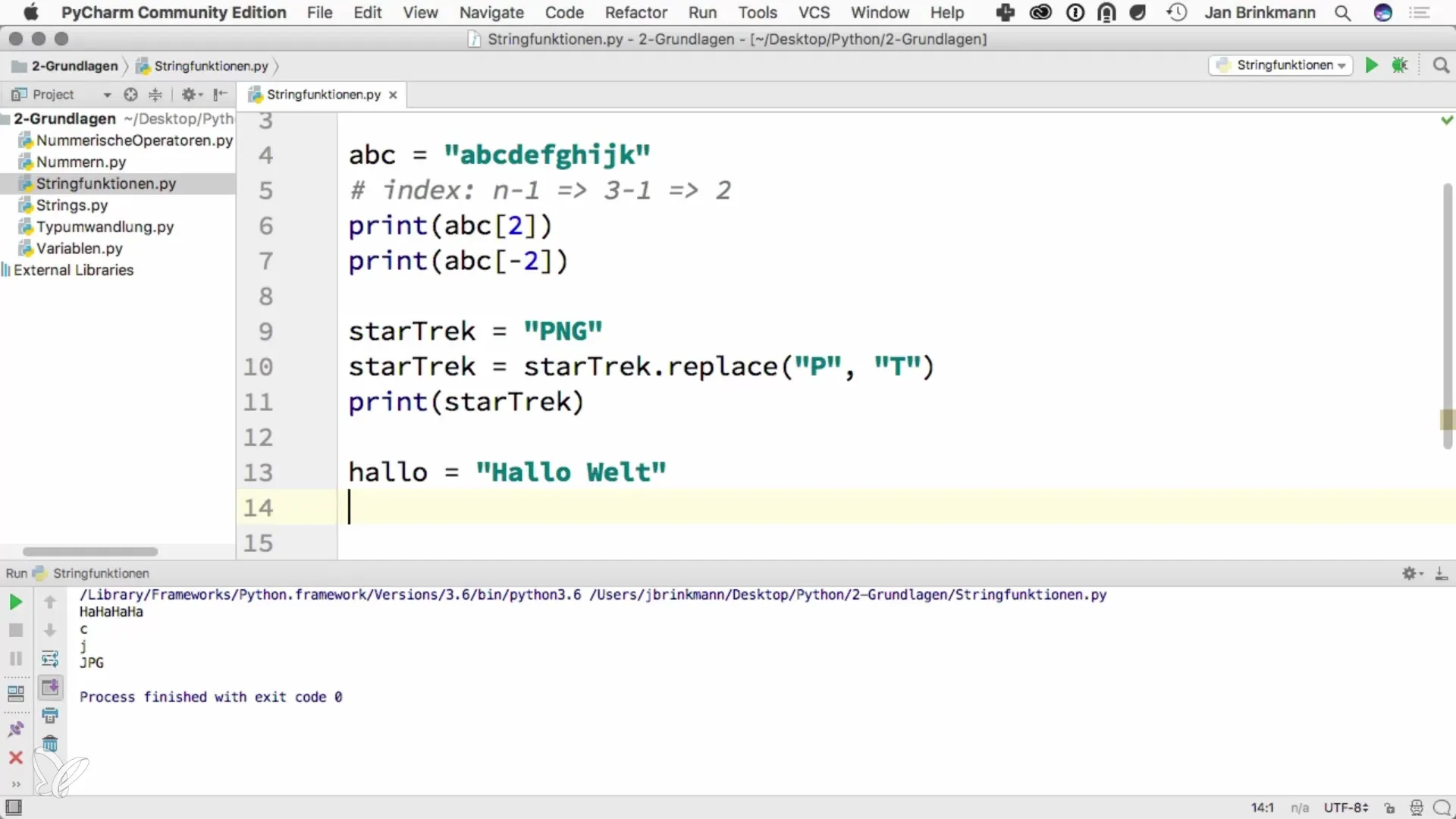
Here, text[0:5] means that you start at index 0 and go up to index 5, but do not include it.
Using Steps
An interesting feature is the ability to extend access to strings using step sizes. With this method, instead of looping through every character, you can selectively query, for example, every second character.
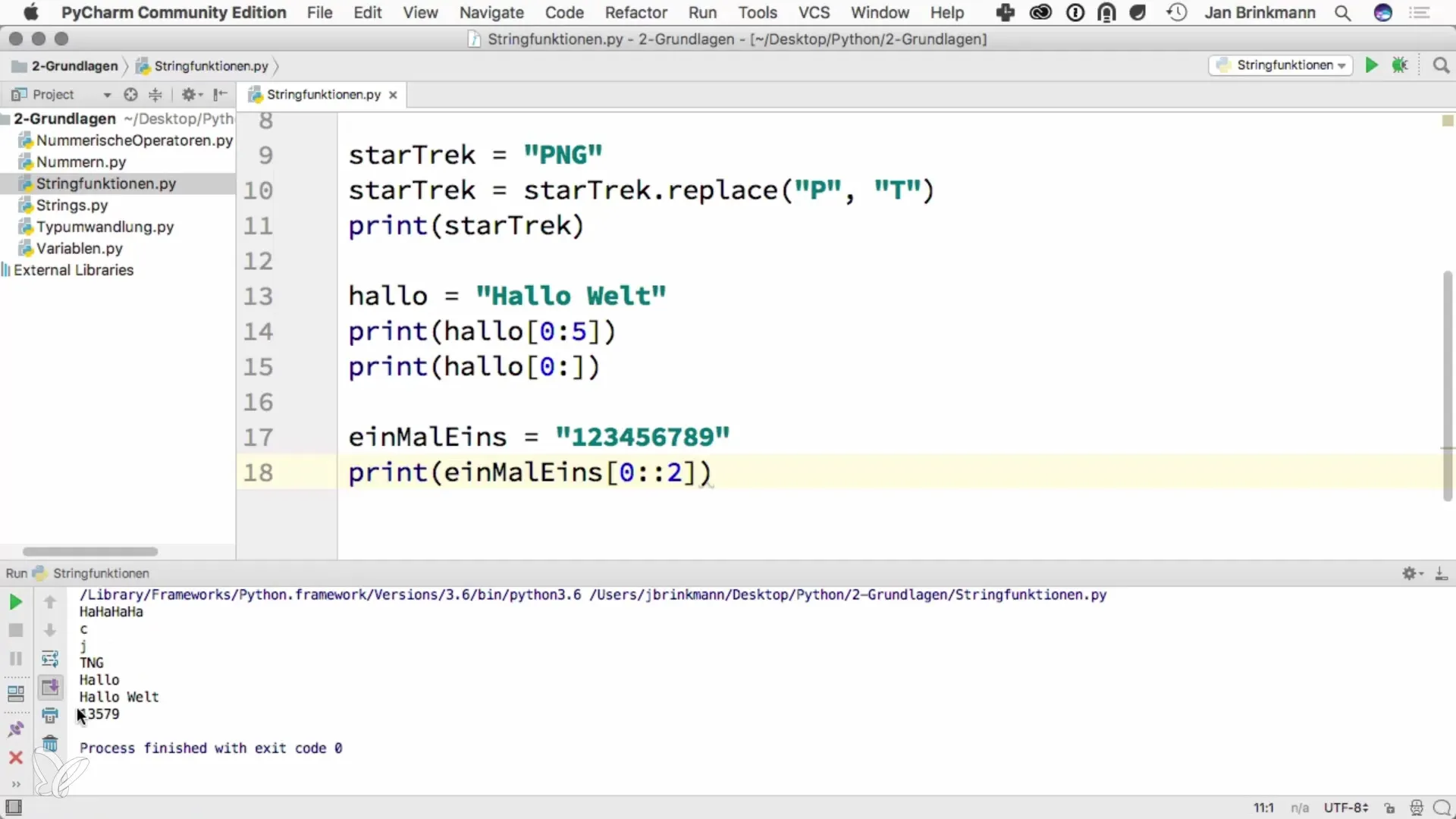
In the above example, the code returns the odd numbers.
Determining String Length
Determining the length of a string is done with the built-in function len(). This method counts the number of characters in the string, including spaces.
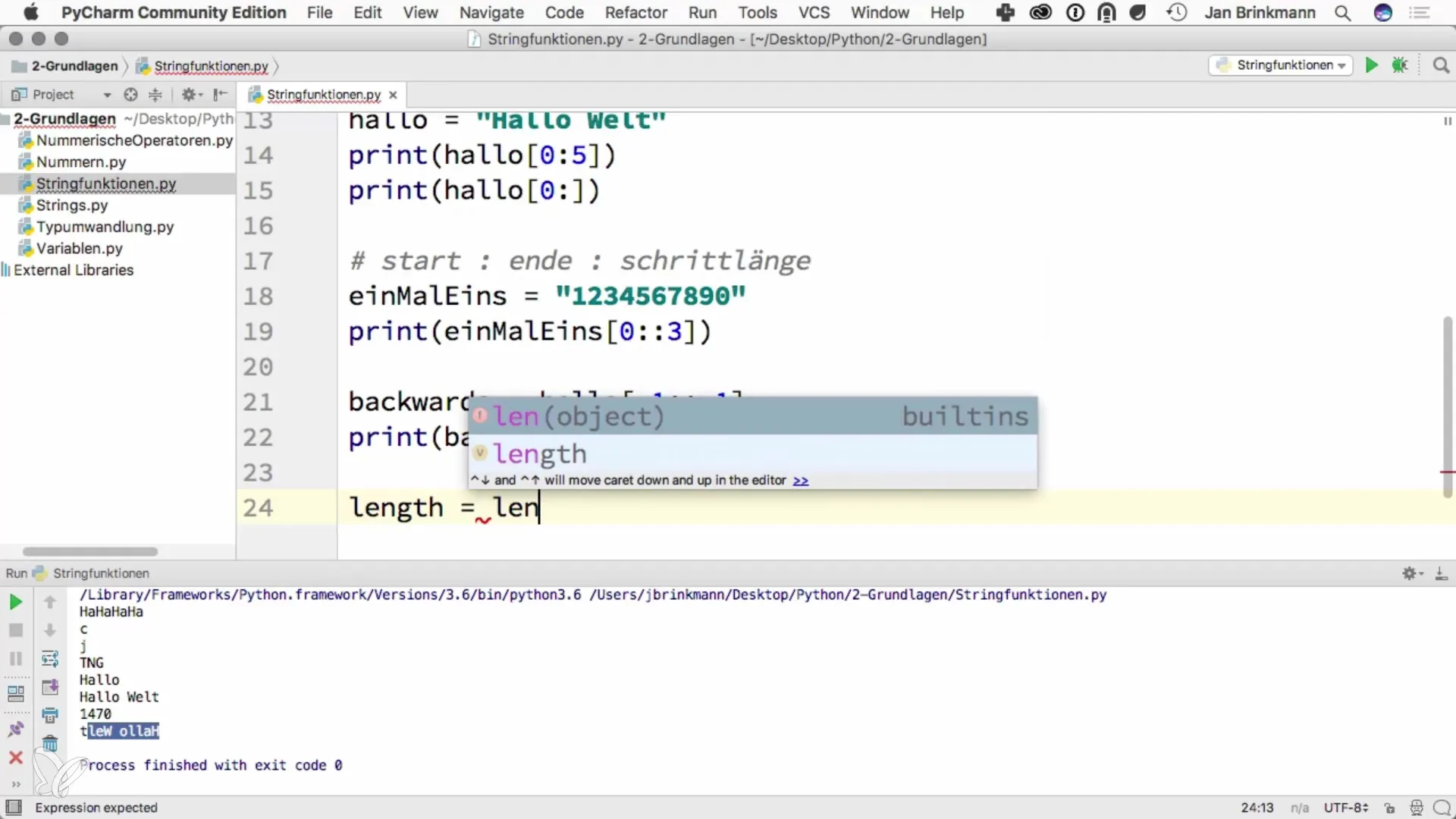
Experimenting with Strings
Of course, there are many other methods and functions in Python that you can try out. Be creative, test different possibilities, and take a look at the official Python documentation to learn more about the various methods available to you.
Summary - Using Python Strings Effectively
You have now learned how to work with strings in Python. You can create them, manipulate them, retrieve parts of them, and replace them. Use the methods presented to further deepen your programming skills and experiment with your own ideas.
Frequently Asked Questions
How can I create a string in Python?A string is simply created by placing text within quotes: my_string = "Hello World".
What is the difference between replace() and substring?replace() replaces a part of a string with another, while a substring extracts a part of the original.
How do I access the last character of a string?You use index -1, for example: my_string[-1].
How can I count the characters of a string?With the len() function, you can determine the number of characters in a string.
Can I perform arithmetic with Python strings?While strings cannot be added like numbers, you can multiply them to repeat them, e.g. my_string * 3.