Creating and initializing lists in Python can often be a tedious and time-consuming task. This is where list comprehensions come into play, an elegant and efficient method that allows you to easily generate and transform lists. In this guide, you'll learn how list comprehensions work and how to effectively use them in your Python projects.
Key Takeaways
- List comprehensions provide a concise and clear syntax for creating and transforming lists.
- You can create not only simple lists but also complex structures.
- You can integrate conditions and loops into list comprehensions to filter and transform data as desired.
Step-by-Step Guide
1. Basics of List Comprehensions
List comprehensions are a concise way to create lists in Python. They consist of an expression and a loop.
With a list comprehension, you can now create a new list that contains, for example, the numbers 1 through 5.
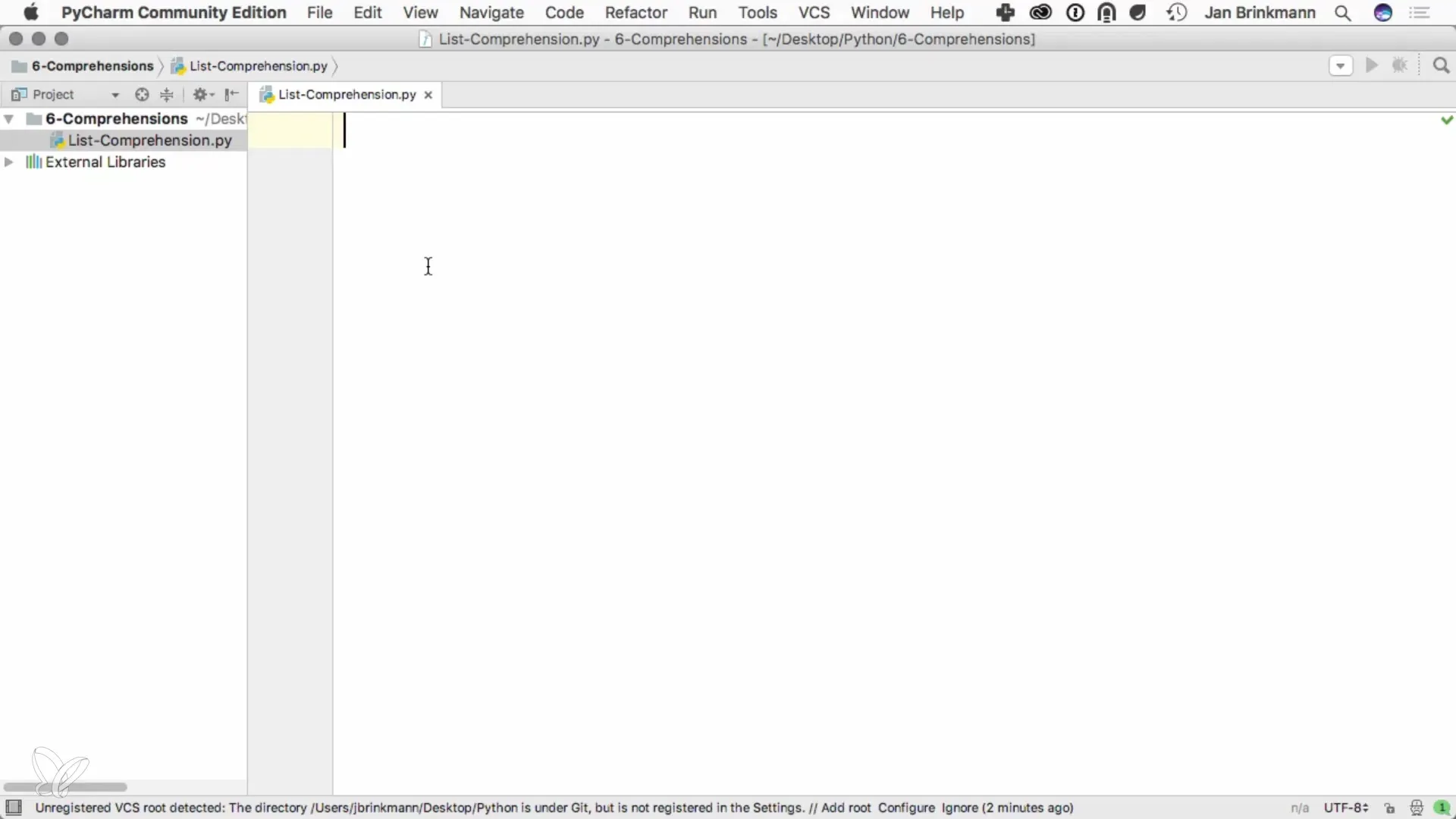
2. Using range() in List Comprehensions
With the range() function, you can generate a list of numbers.
This produces a list with the numbers 1 through 5.
3. Transforming List Elements
You can also use list comprehensions to transform the elements of a list.
Here, the triple of each number from 1 to 20 is calculated and stored in a new list.
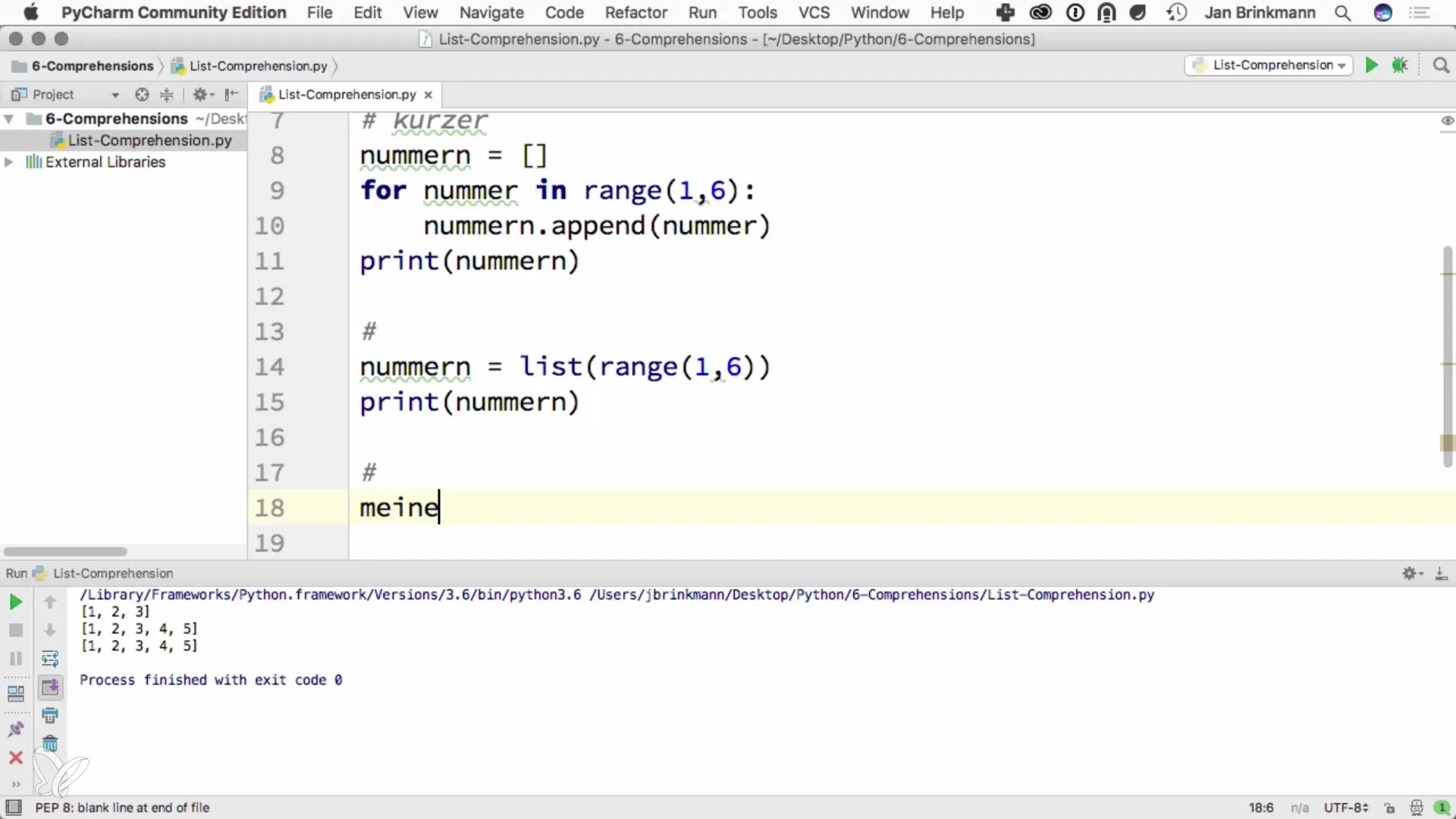
4. Filtering with Conditions
List comprehensions also allow you to add conditions.
Now even_numbers contains only even values.
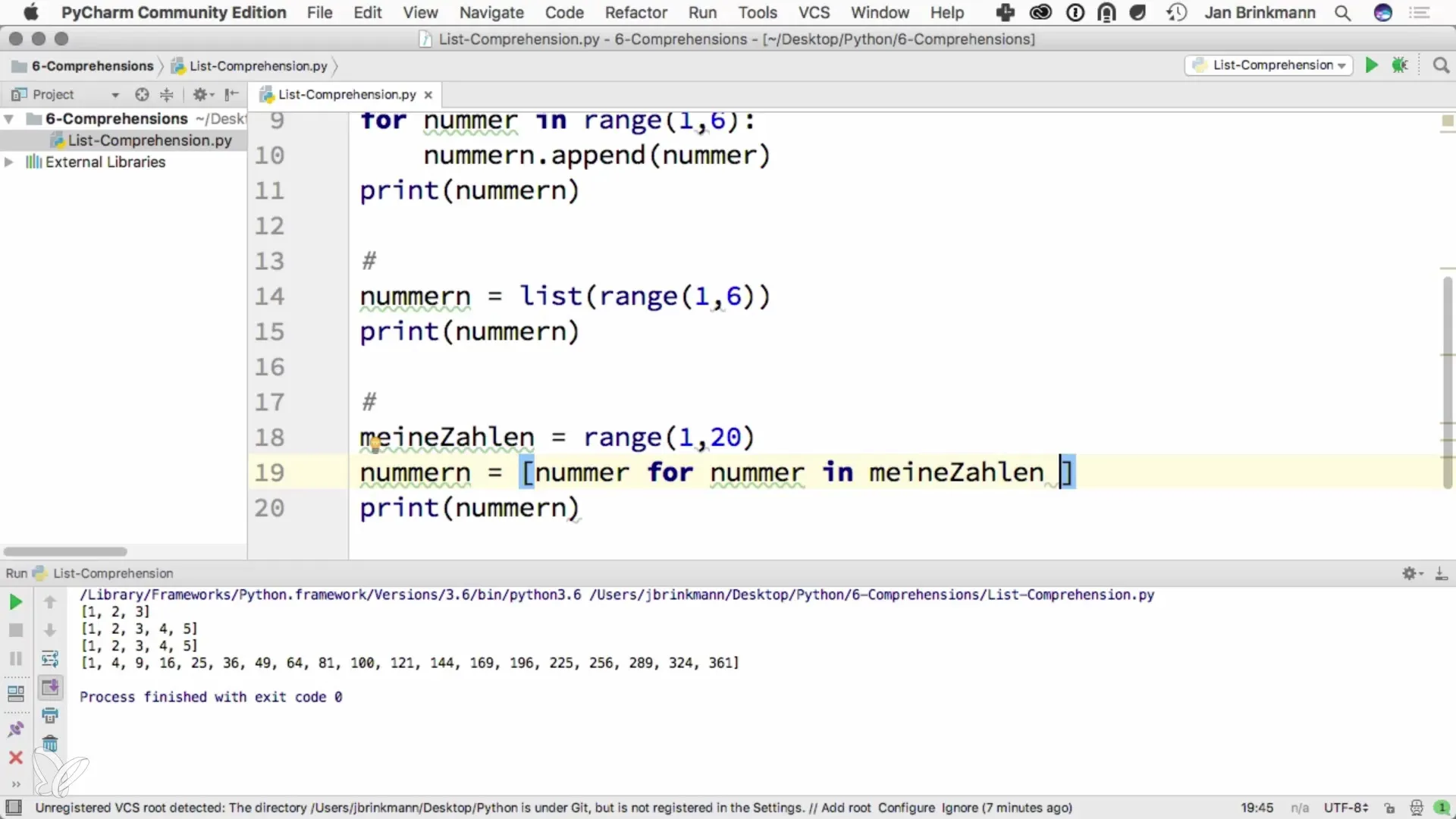
5. Nested List Comprehensions
It is also possible to nest list comprehensions.
This yields a list of tuples for each combination of row and column.
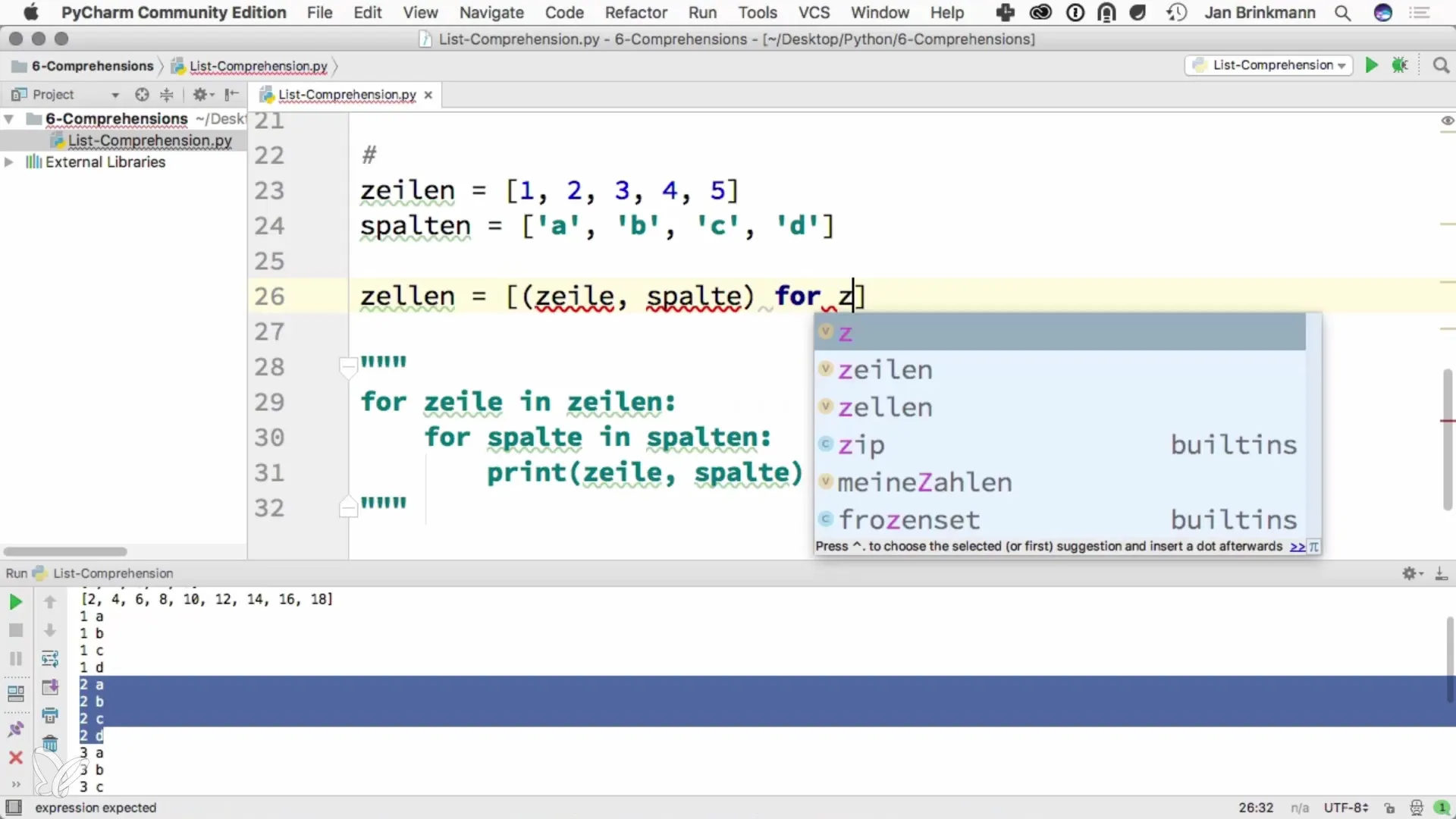
6. Displaying the Result
This gives you a clear display of the generated list.
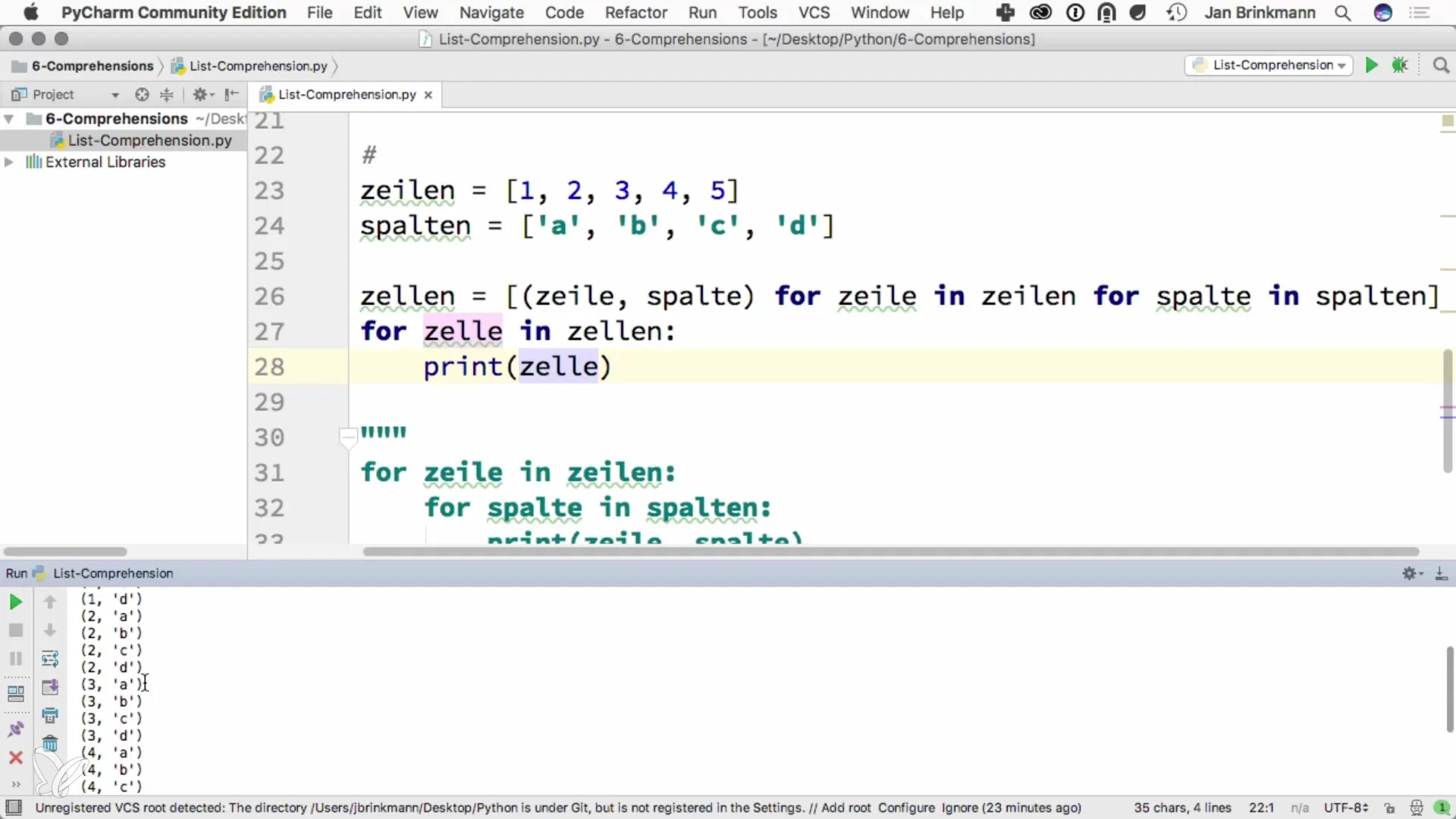
Summary – List Comprehensions in Python: Efficient and Practical
List comprehensions are a powerful tool in Python that allow you to efficiently create and transform lists. You have the ability not only to generate data but also to filter and manipulate it, making your code more elegant and concise.
Frequently Asked Questions
What advantages do list comprehensions offer?List comprehensions enable a concise syntax for creating lists and avoid unnecessary loops.
Can I use list comprehensions in nested for loops as well?Yes, list comprehensions can be easily nested to create more complex data structures.
Where can I find more information about list comprehensions?The official Python documentation provides comprehensive information about list comprehensions and their usage.