The understanding of classes, instances, and methods is essential for anyone who wants to program in Python. A special role is played by static methods, which offer an efficient way to accomplish tasks without needing an instance of the class. In this article, I will show you how static methods work, what they are useful for and provide you with practical examples for implementation in your Python project.
Key insights
- Static methods are tied to the class, not the instance.
- You can use static methods to implement logical functions that do not access class or instance variables.
- They are particularly suitable for utility functions that are meant to work independently of instance states.
Step-by-step guide to static methods
1. Introduction to class methods
At the beginning, it is important to understand the structure of a class in Python.
Here you define a class Database with an initializer to set the connection variable and a method to establish a connection.
2. Instance variables and their usage
You create an individual connection for db1. Each instance has its own memory area that holds this connection. This means that you can have multiple instances of the class and use them independently of each other.
3. Counting connections with class variables
If you want to count the number of connections across all instances, it makes sense to use a class variable.
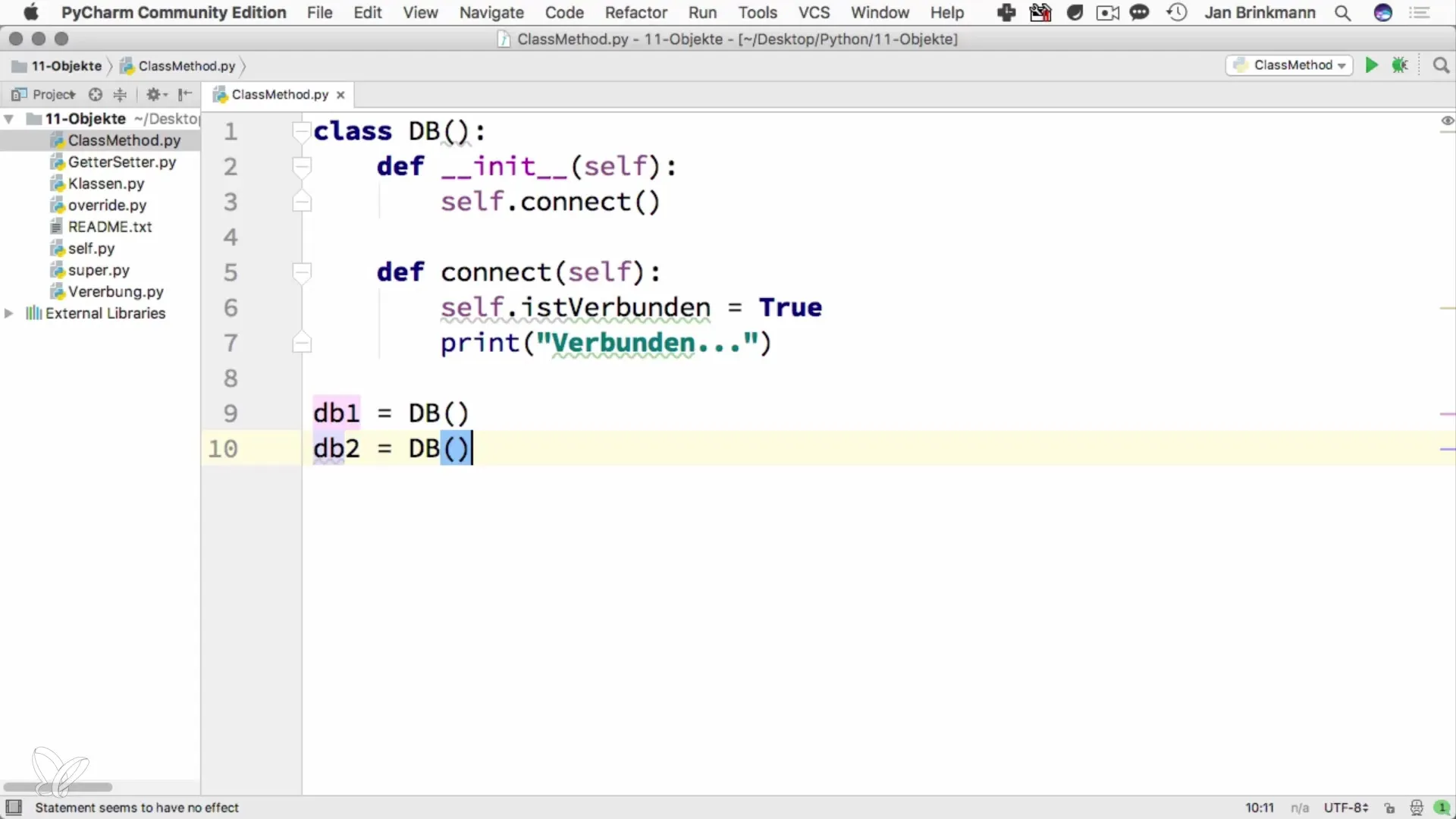
The variable connections belongs to the class itself and is incremented with each connection establishment. This way, you have access to the total number of connections at any time.
4. Implementing a class method
To output the number of connections, you can add a class method:
class Database: connections = 0
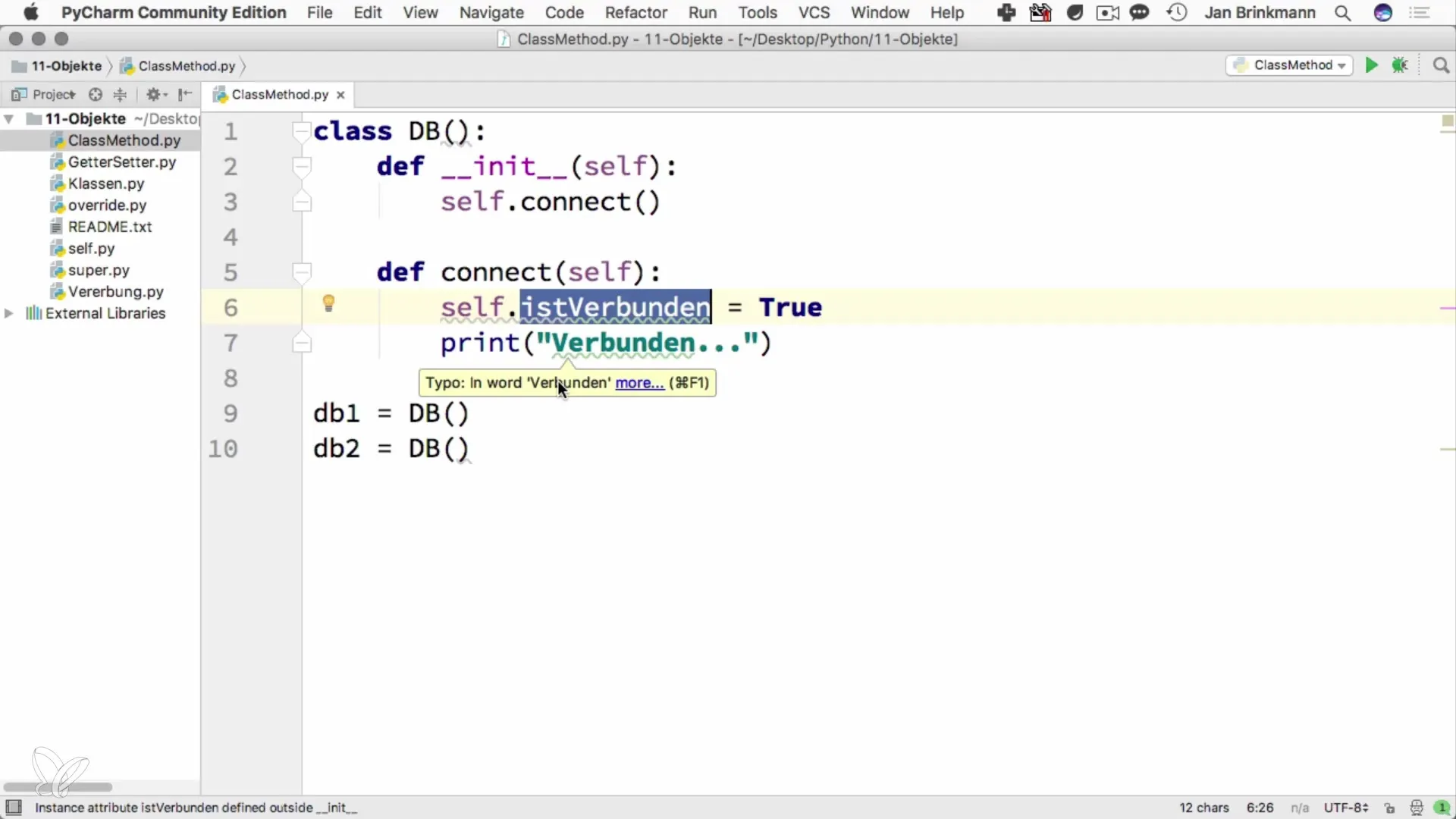
Here, the method number_of_connections is added, which outputs the total number of connections.
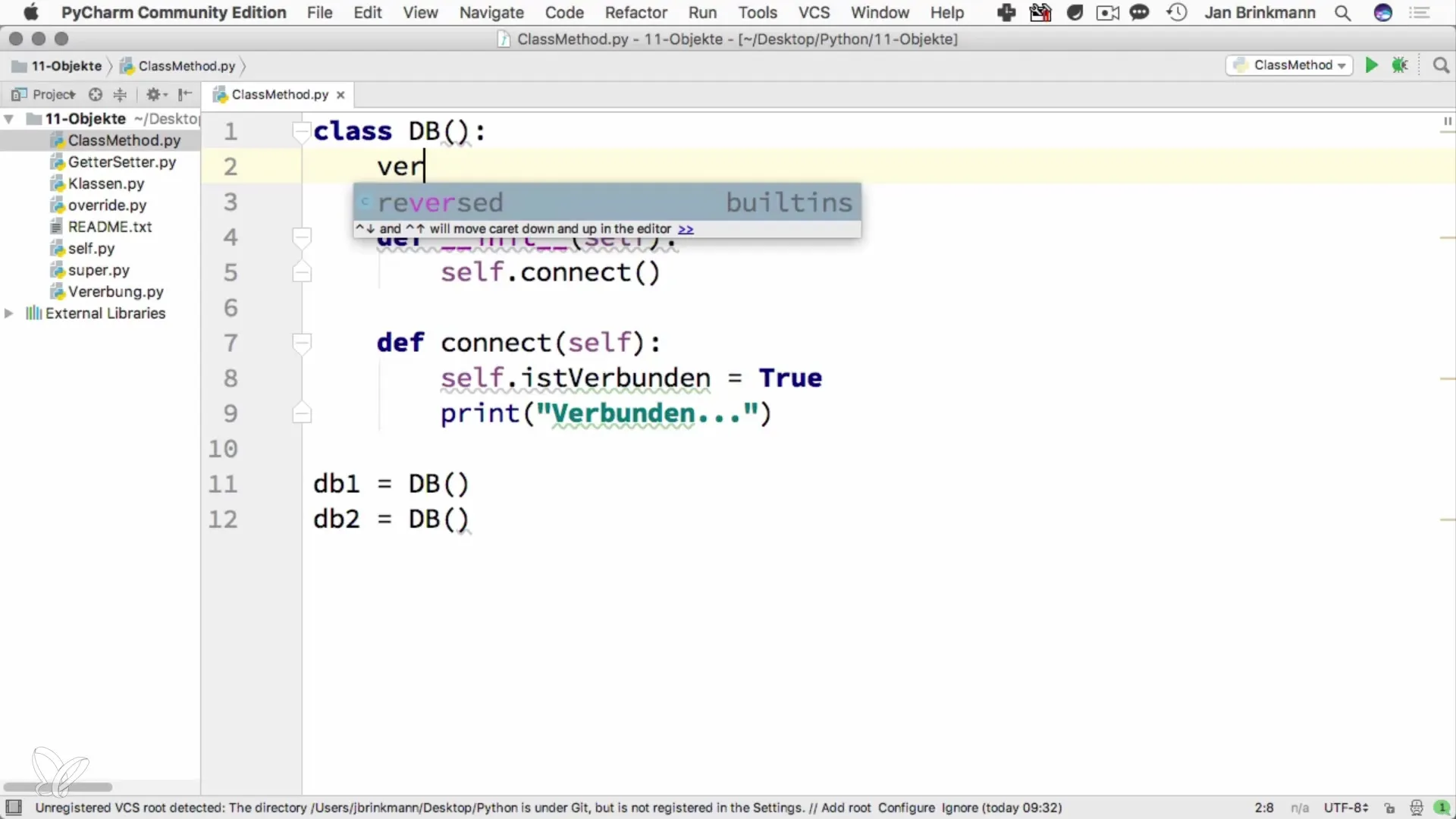
5. Introduction to static methods
Static methods differ from class methods in that they do not access class or instance variables. They are used to provide functions that do not depend on the state of an object.
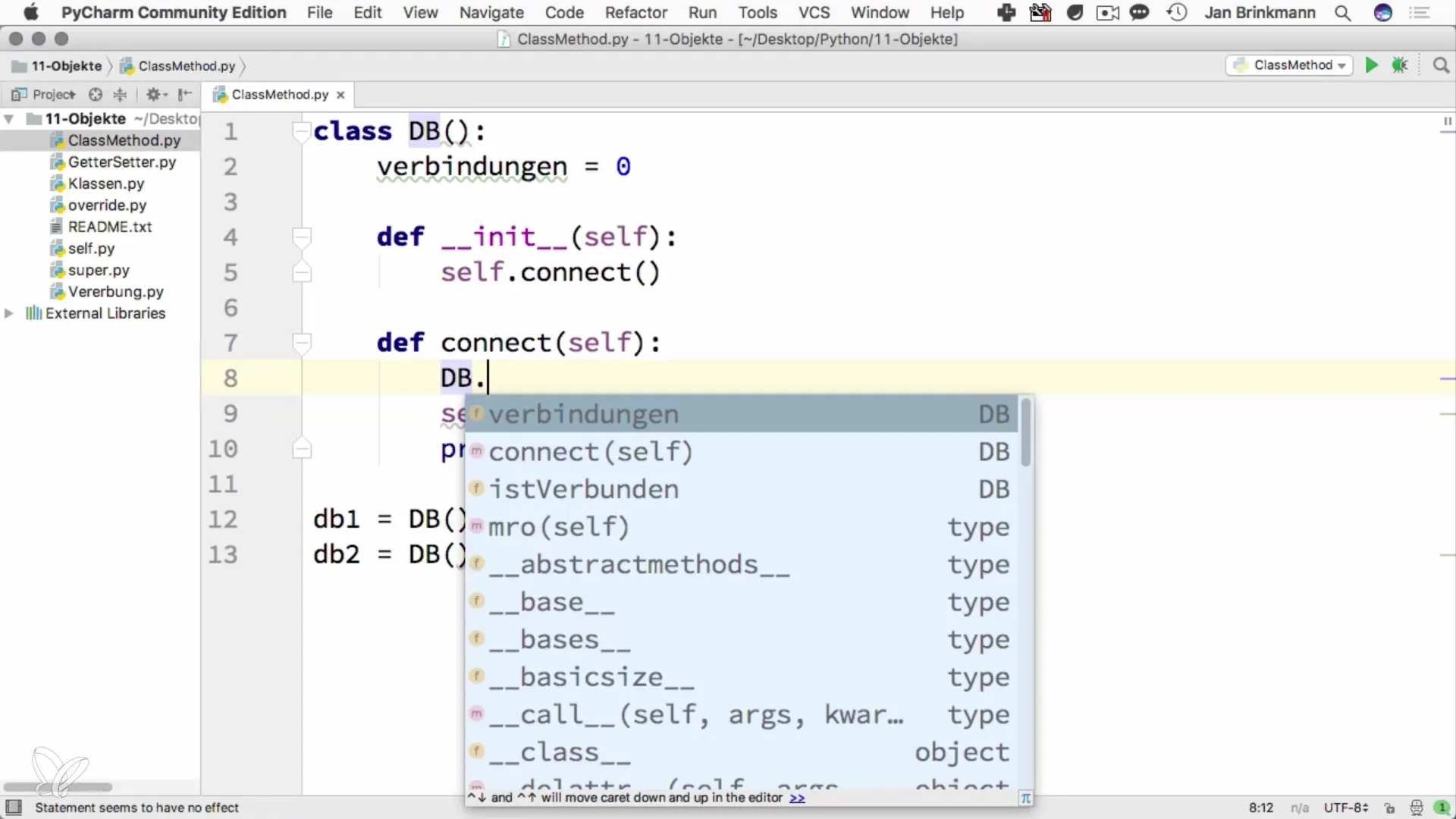
6. Useful application examples and conclusion
Static methods are particularly useful for utility functions that are neither in the context of a class nor an instance. They allow you to keep your code clean and tidy by providing reusable functions consistently in one place. In practice, you can use them, for example, for data formatting or mathematical calculations.
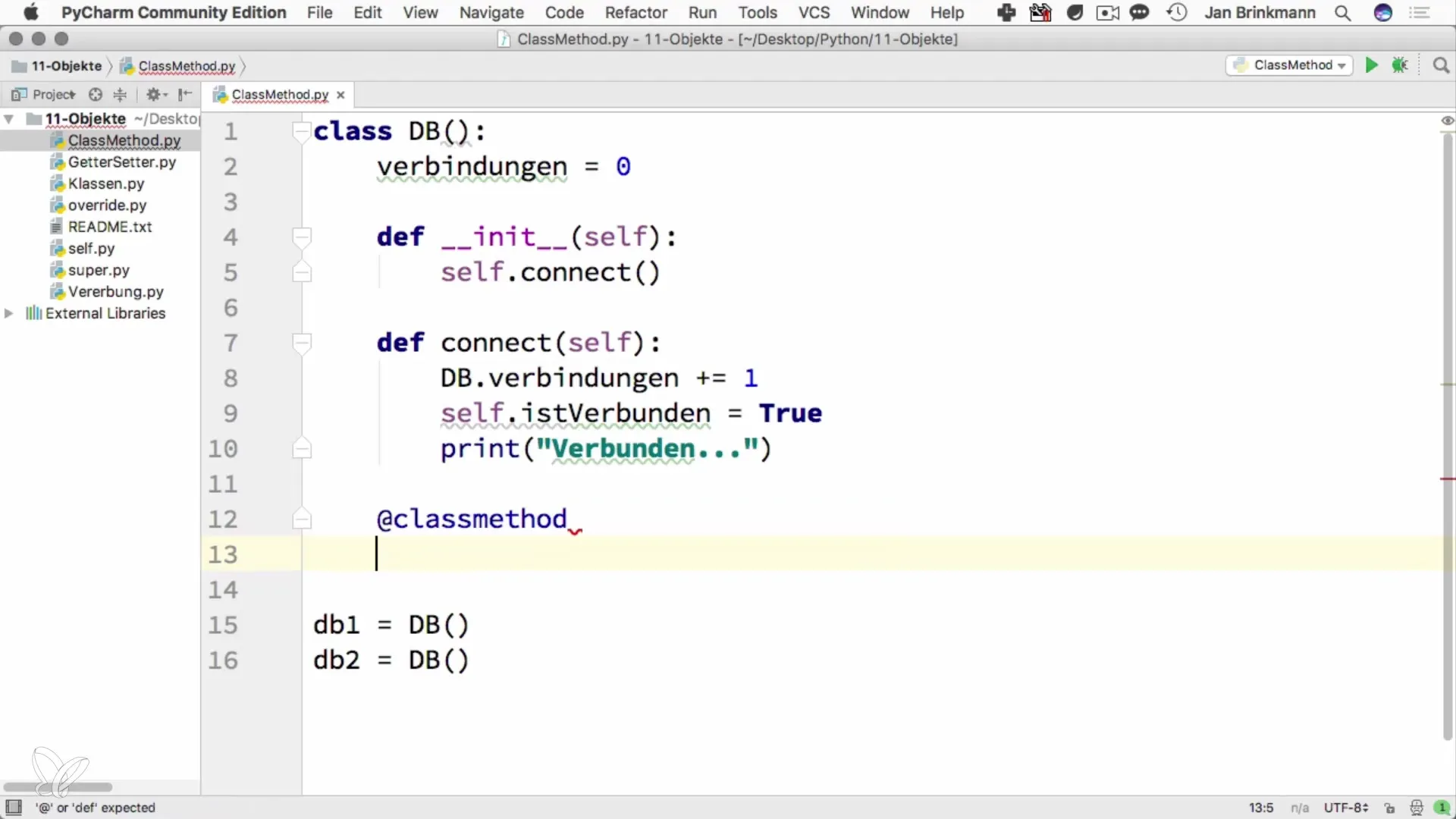
In this example, you calculate the hash value of a database entry without needing a specific instance.
Summary - Static Methods in Python: Usage and Implementation
Static methods provide you with a flexible way to improve the reusability and organization of your code. They allow access to logical operations without needing to create an instance. This not only makes your program more efficient but also more readable and maintainable.
Frequently asked questions
How do class methods and static methods differ?Class methods refer to the class and can access class variables, whereas static methods are independent of instances or class variables.
When should you use static methods?Static methods should be used when there is no dependency on instance or class variables.
Can I also call a static method as an instance method?Yes, static methods can be called both through the class and through an instance of the class.