How often have you asked yourself while programming what data type a function expects? Type Hints in Python are a powerful tool that helps you significantly improve the readability of your code and utilize autocompletion in development environments. They not only assist you but also other developers who need to read or use your code. Let's find out together how you can effectively apply Type Hints in your code.
Key insights
- Type Hints increase the readability and understandability of the code.
- They facilitate autocompletion in IDEs.
Step-by-Step Guide
What are Type Hints?
Type Hints are annotations about the data type that are used in Python to declare what kind of data is expected in variables, functions, or methods. Let's start with a simple example to illustrate this.
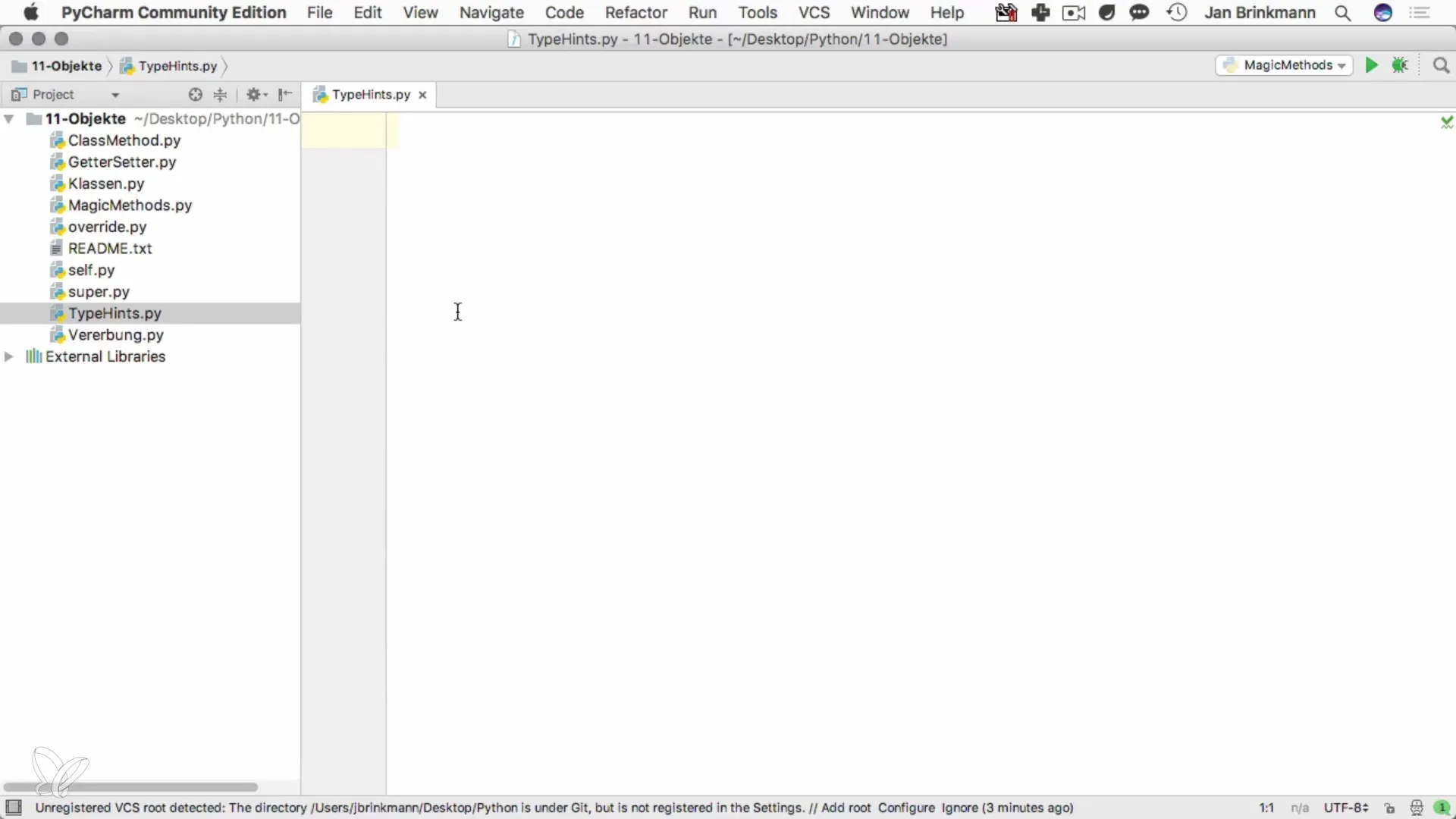
Usage in a Function
Imagine you have a class called Adapter that includes a method get_entries. This method could help retrieve data from a database. To apply Type Hints correctly, you need to ensure that the function clearly describes what it returns.
Here we indicate that get_entries returns a list of entries.
Passing Parameters
Consider how you can create a method like list_output that expects an adapter as a parameter. By specifying the type of the parameter, it becomes clear what this method expects.
Such a type hint ensures that you immediately recognize what kind of object is required when you call the function.
Improving Autocompletion
When you ask in the code what methods or attributes the adapter contains, you immediately see all available options as soon as you activate autocompletion.
For example, if you type adapter., get_entries and other methods will immediately appear to assist you while programming.
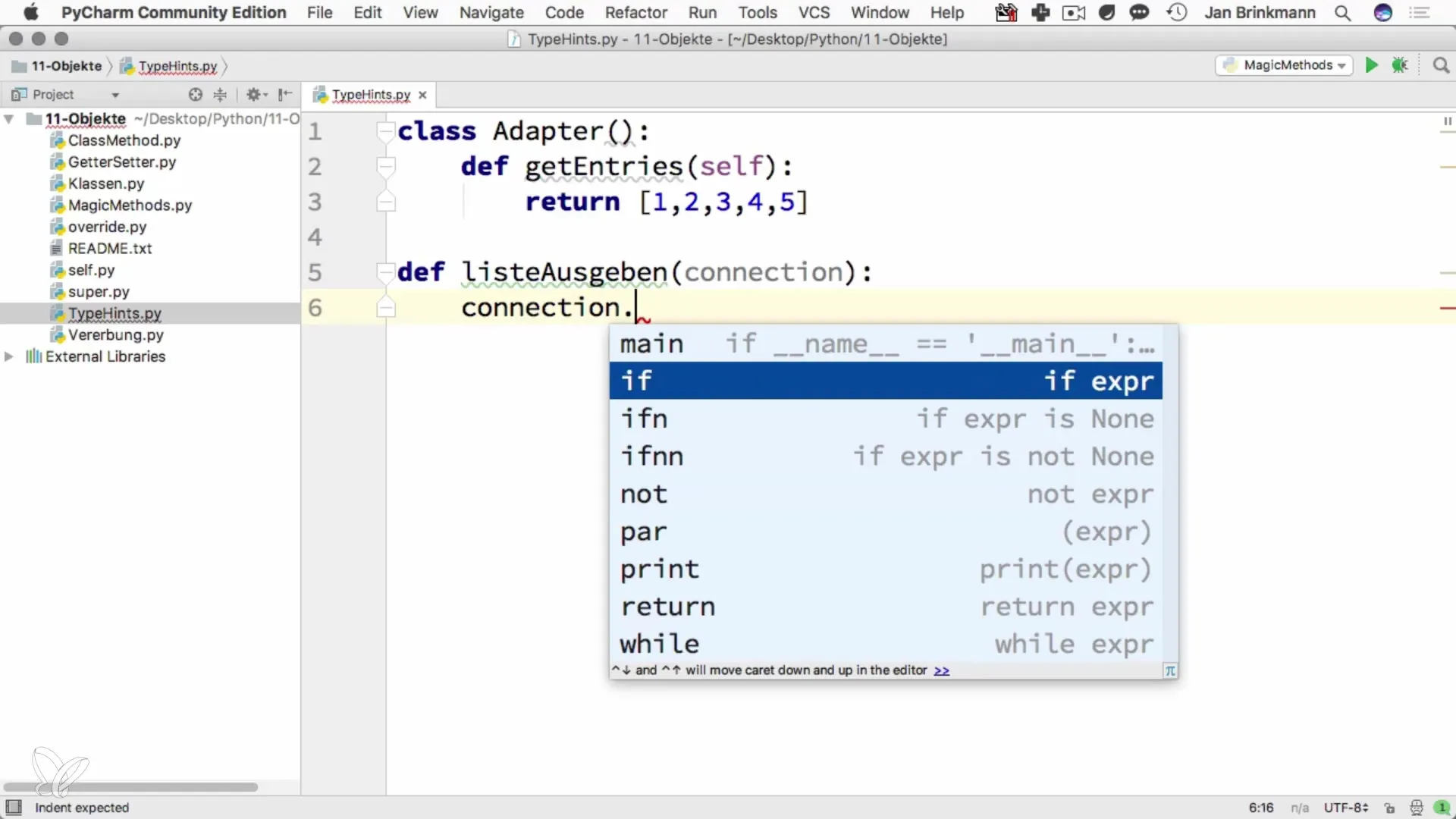
Avoiding Errors
Although the use of Type Hints is not mandatory, they help you avoid bugs and misunderstandings in the code. Instead of hunting for errors based on assumptions, you can rely on static type checking, especially in IDEs.
By defining the parameter and return types, the likelihood of accidentally passing the wrong data type is reduced.
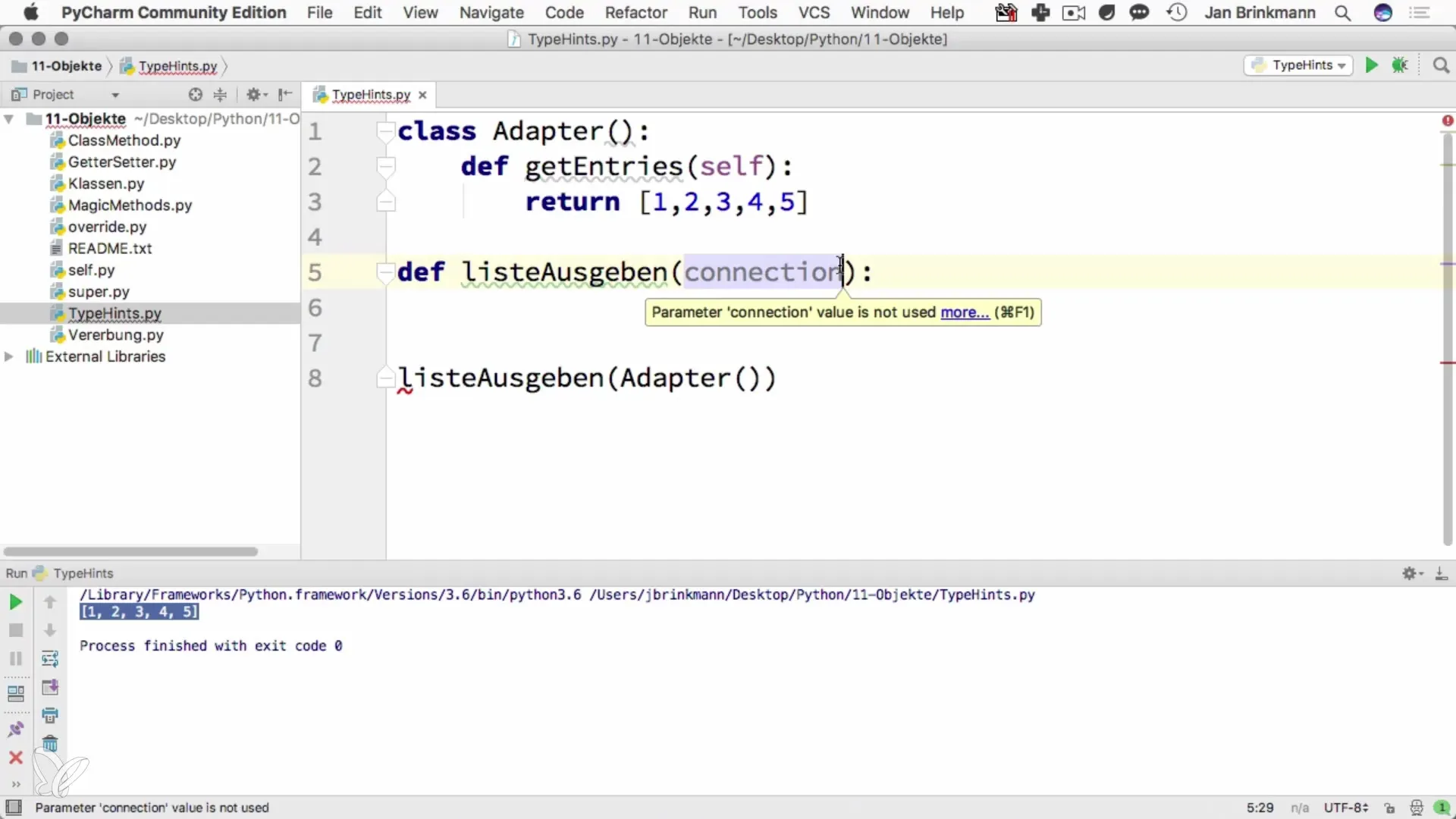
Conclusion for Type Hints
Type Hints in Python are a small but powerful tool that helps you not only structure your code better but also ensures that it is more understandable to others. It is a practical method for dealing with types and optimizing autocompletion, thereby increasing your efficiency in programming.
Summary – Type Hints in Python – How to Improve Your Code Readability
Implementing Type Hints is key to improving the readability and maintainability of your code. Use them to clarify what data types are expected in your functions and methods. While they do not alter how your code actually works, they provide tremendous benefits during development.