The concepts of scope and namespaces are central to effective programming in Python. These concepts help manage the scope of variables and their visibility, which is particularly important when you are working with functions.
Key insights
- The scope of a variable determines in which part of the code the variable can be accessed.
- Local variables exist only within the function in which they are defined.
- Global variables are visible throughout the entire module unless they are overridden within a function.
- The global keyword allows access and manipulation of global variables within a function.
- Incorrect use of global variables can lead to error-prone and hard-to-follow code.
Introduction to scopes
In the following section, you will learn how scopes work in Python. We will look at how local and global variables are defined and used, and how you can ensure that your code remains clear and maintainable.
Definition of scopes
Let’s start with a simple example. Suppose you have a variable weather that holds the value “sun”. This might look like this:
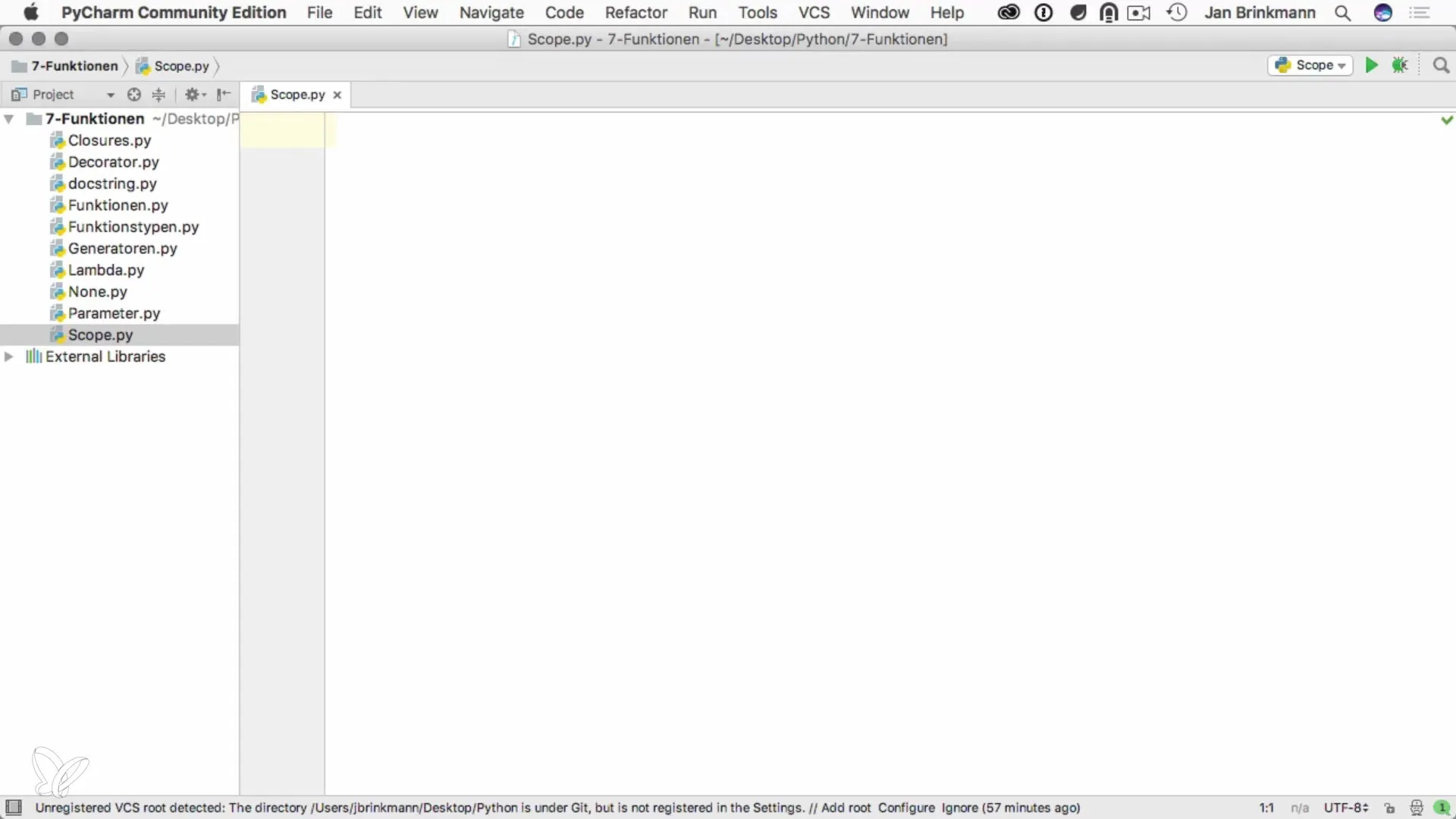
Now we want to create a function that changes the weather, for example, a function rain:
In this function, we set the variable weather to “rain” and expect the result to be visible in the main function.
Local versus global variables
When you call the function and set the variable weather within the function, you will notice that the value of weather in the global environment does not change. Instead, the original value “sun” remains. There is a simple reason for this: Python treats the variable weather within the function as a local variable.
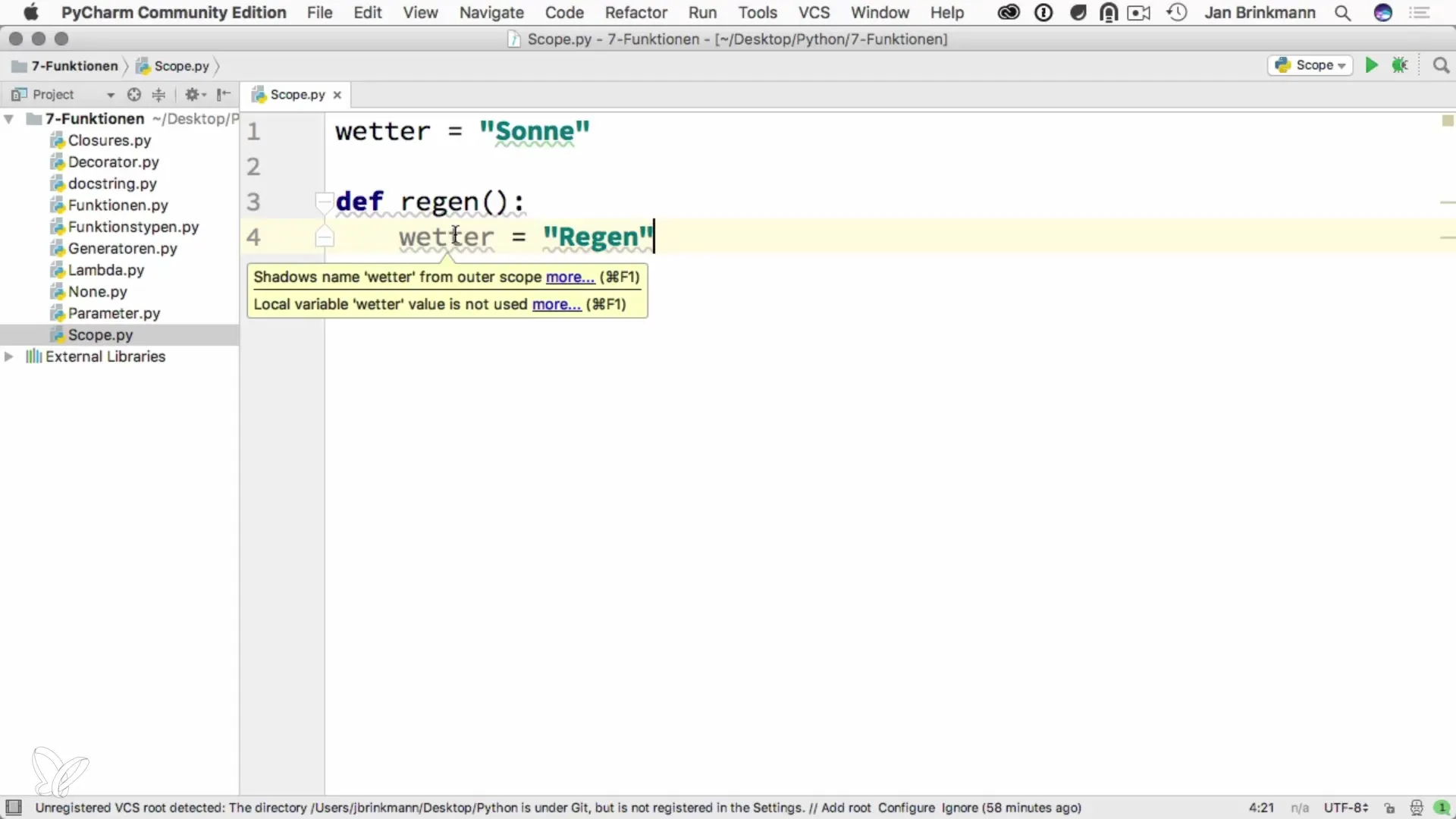
This means the local variable overshadows the global variable with the same name. This is clearly expressed in the error message: "local variable 'weather' not used". This indicates that the local variable was created within the function's scope and cannot access the global variable.
Changing the value of a global variable
To change the value of a global variable within a function, you must use the global keyword:
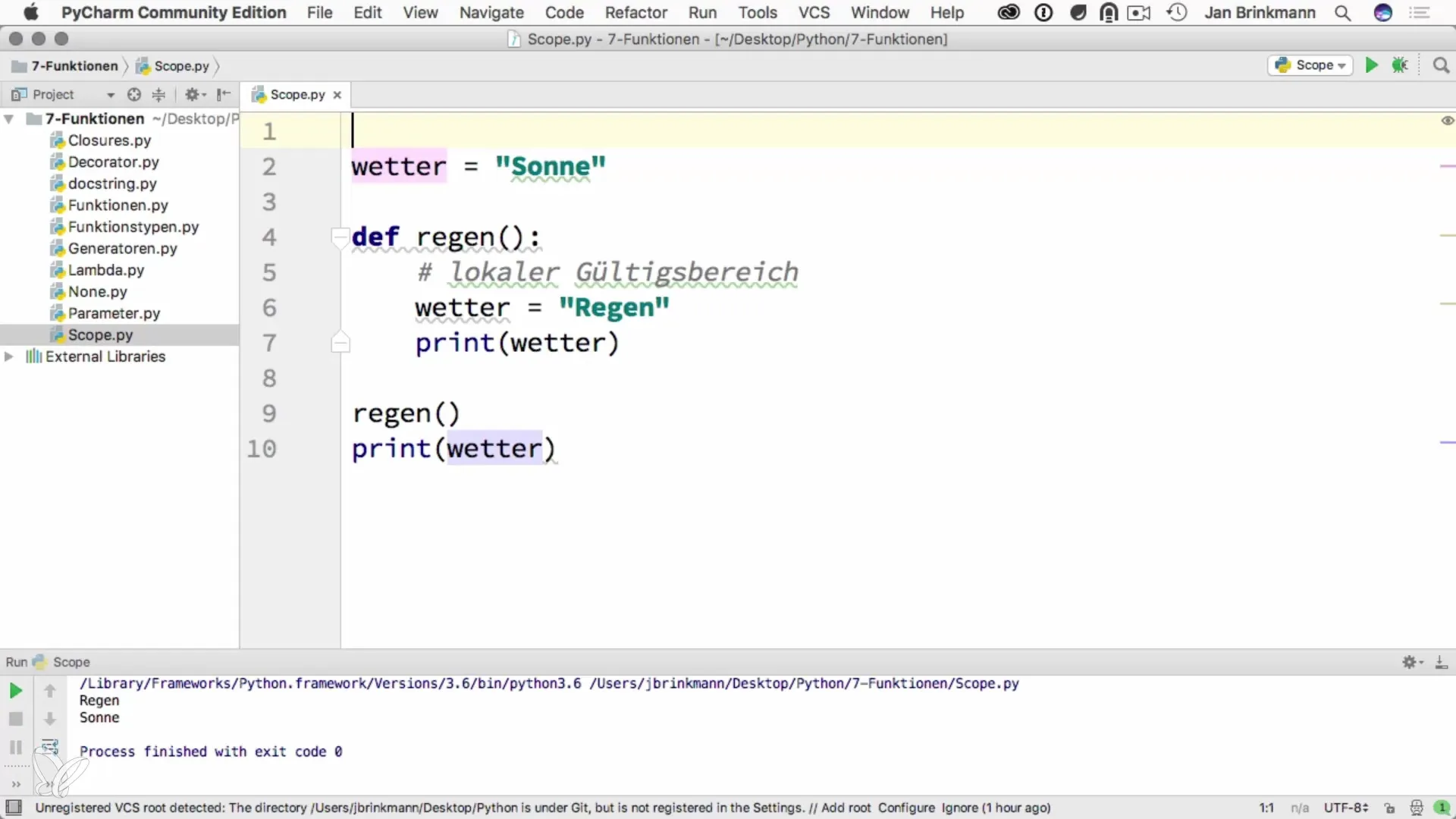
When you use the global keyword, you inform Python that you want to access and modify the already defined global variable. When you then call the function rain again, the value of weather is indeed changed to “rain”. This allows you to use the function to manage global states.
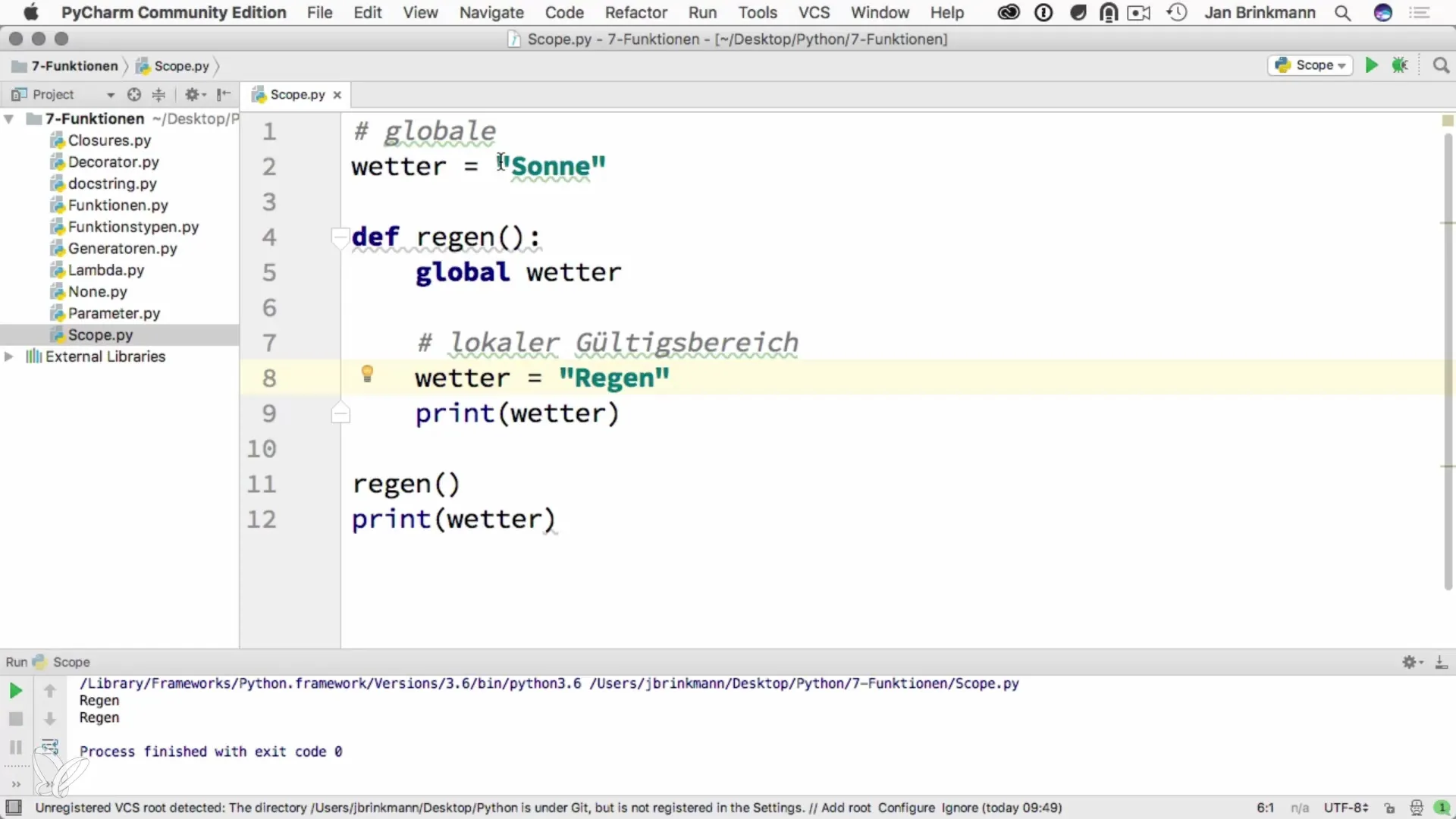
Using local variables
It is important to note that local variables exist only within their defined function. For example, if you define a local variable wind_strength in the function rain, it will only be recognized within that function.
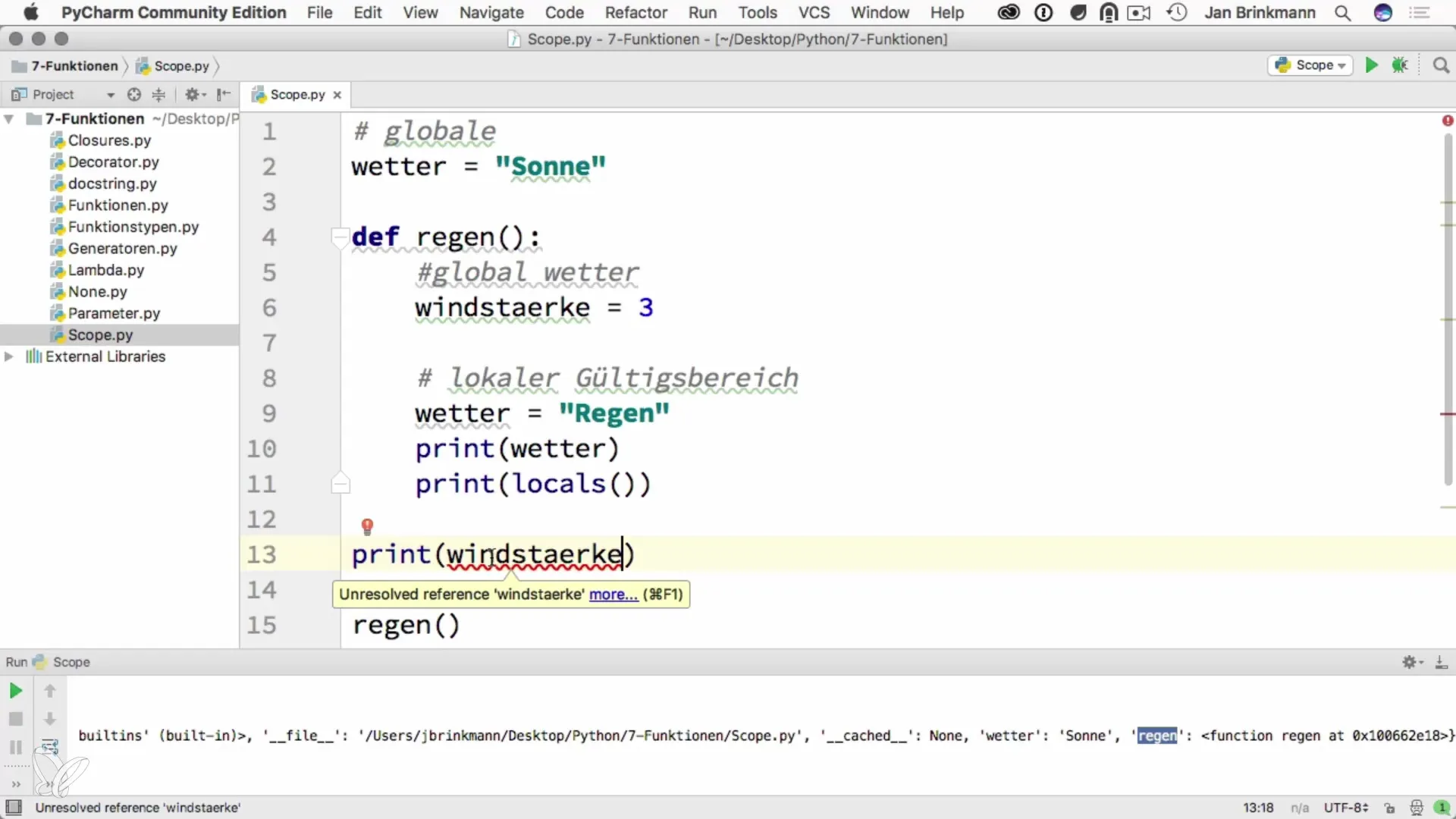
Accessing wind_strength outside of that function will not be possible. Instead, you will receive an error message that there is no reference to wind_strength in the global namespace.
Comparison of local and global variables
Let’s summarize the differences between local and global variables:
- Global variables: Visible throughout the entire module. They can be read and altered anywhere as long as you use the global keyword in a function.
- Local variables: Visible only within the function in which they are defined. They are not accessible outside that function.
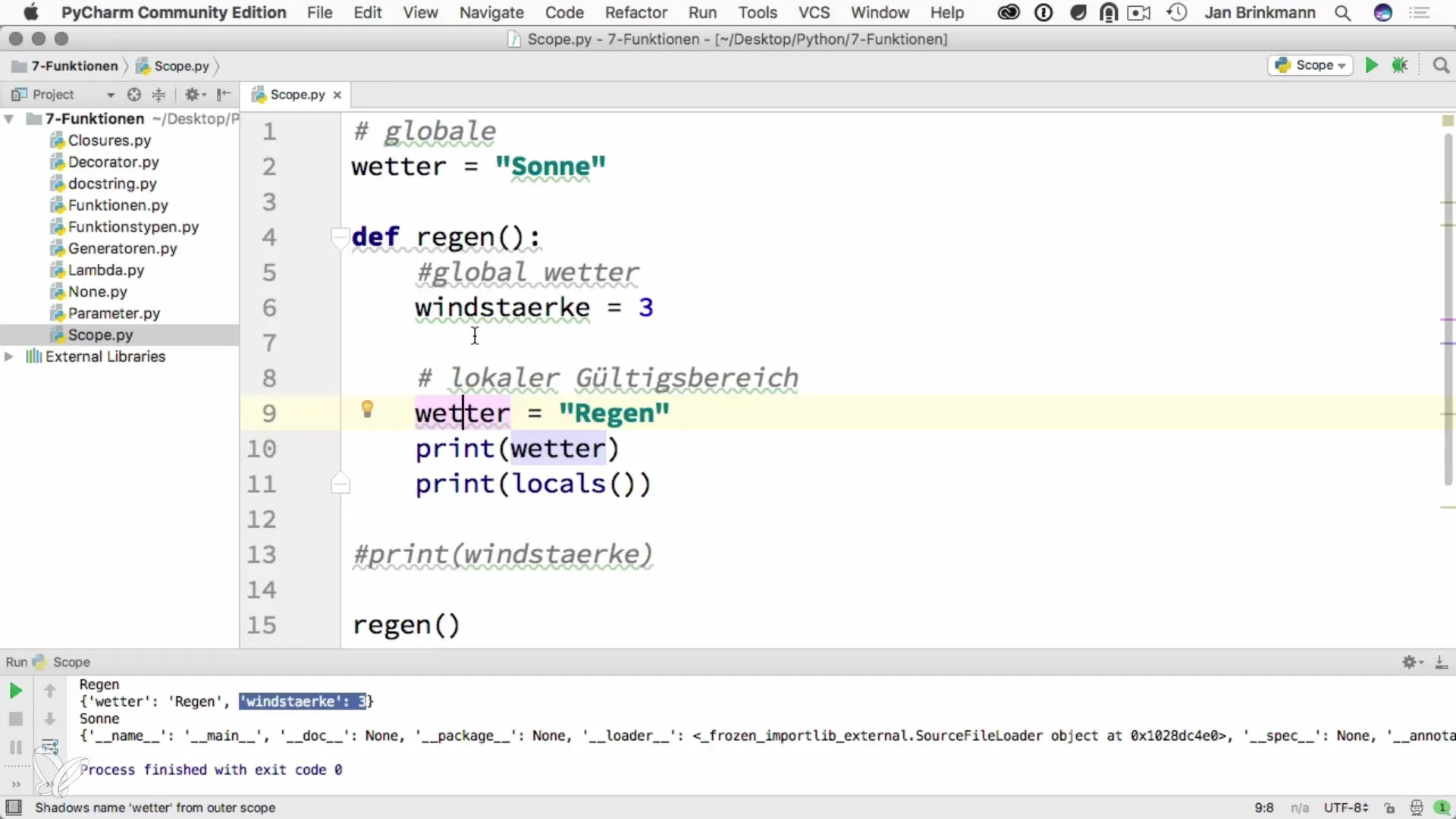
Scopes and best practices
It is important to be cautious when using scopes. Overreliance on global variables can lead to unclear and hard-to-maintain code. A good practice is to avoid global variables unless absolutely necessary. Instead, functions and methods should use parameters and return results. This makes your code clearer and easier to follow.
Additional considerations are important: Ensure that any function that uses data from the global environment is clearly named and well documented. This minimizes potential sources of error.
Summary - Understanding scopes and namespaces in Python
The concepts of scopes and namespaces are crucial for clean programming practices in Python. Understand the difference between local and global variables and apply best practices. This will create a robust and maintainable code design.
Frequently Asked Questions
What is a scope in Python?A scope in Python defines where a variable exists in the code and can be accessed.
How can I use a global variable in a function?To access a global variable in a function, the global keyword must be used.
Why should I use local variables?Local variables prevent conflicts with global variables and make the code clearer and easier to maintain.
What happens if I try to access a local variable outside its function?You will receive an error since local variables only exist within the function and are not visible from outside.
Why are global variables problematic?Global variables can make the code less predictable and harder to understand, which reduces maintainability.