The use of generators is an excellent method for saving memory resources and increasing the efficiency of your code. In this guide, you will learn how to utilize generators in Python to handle large amounts of data without exceeding the memory limits of your system. The concepts are illustrated with practical examples, so you can quickly and efficiently dive into the topic.
Key Insights
Generators allow you to produce large amounts of data without storing them all in memory at once. Instead of generating a complete list of values, you can provide values on demand using generator functions. This is particularly useful for iterative processes that need to generate large or infinite sequences of data.
Step-by-Step Guide
Introduction to the Generator
Start by understanding the basic idea behind generators. Imagine you have a large quantity of numbers you want to generate. For example, a list of numbers from 1 to 1,000,000. If you were to use a regular list in Python, you would have to store the entire list in memory. This could already pose a problem with a million integer values. To illustrate how common this situation is, consider what would happen if you wanted to print all these values at once. Instead of doing that, we use generators that generate values one by one, thus saving you resources.
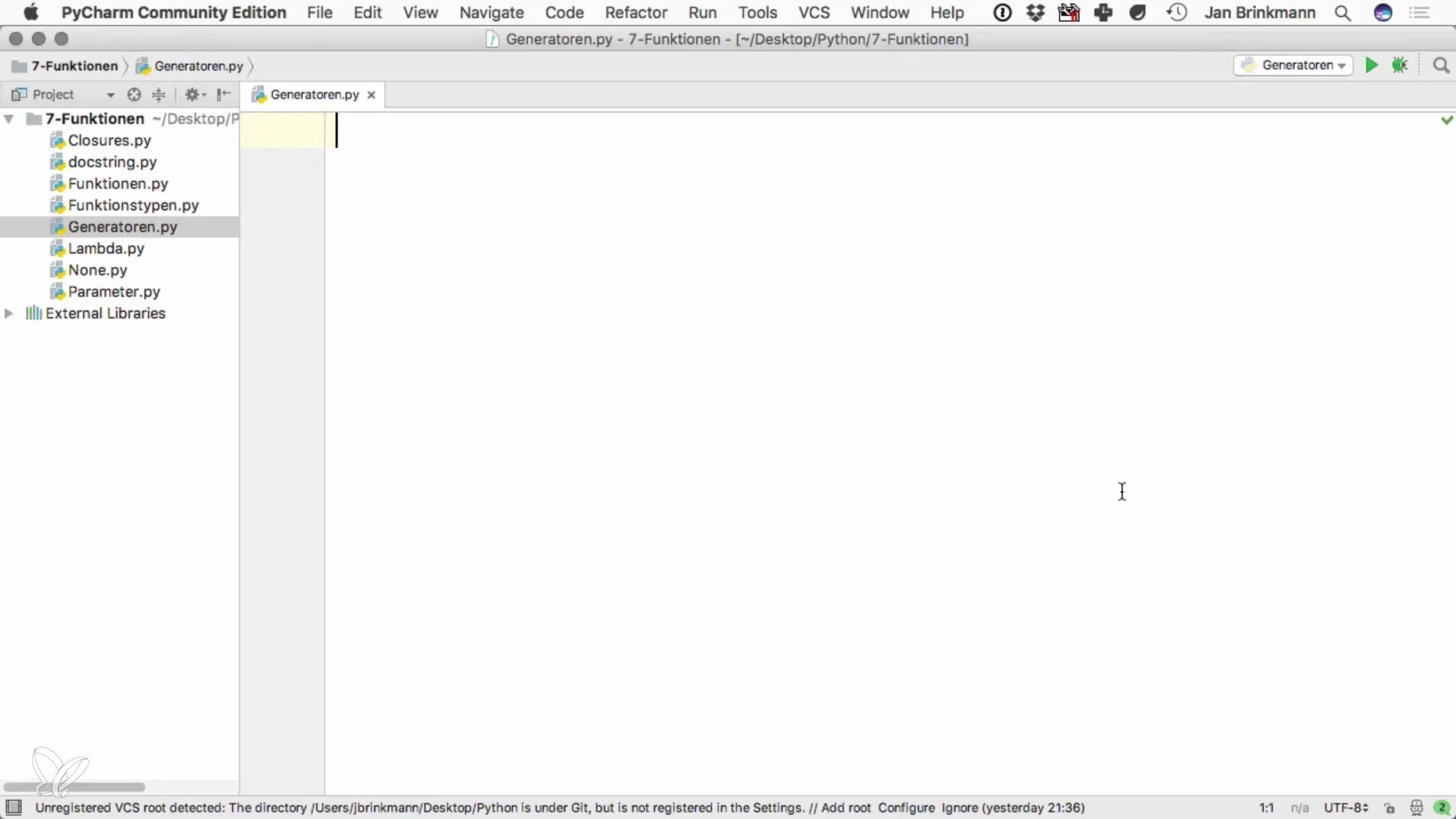
Creating a Generator Function
Now let's move on to creating your own generator function. The first step is to outline the structure of your generator.
This is a function that generates numbers from a start value to a stop value. The keyword yield is crucial for the functioning of generators. At this point, the current value is returned, and the function's state is preserved. When you request the next number, the execution of the function continues at the point where it was previously paused with yield.
Using the Generator
To use the function, you can call it within a loop.
With this example, you want to print only a few values, specifically those that are divisible by 10,000. With a million numbers, you will thus only get 100 outputs, which significantly increases performance and reduces memory consumption. Instead of holding all numbers in memory at once, the generator produces them gradually.
Advantages of Using Generators
A significant advantage of using generators is the reduction of memory consumption. Unlike traditional lists that store all values simultaneously in memory, a generator only requires memory for the current value. This is a major advantage, particularly when dealing with large datasets or infinite sequences. This efficiency enables you to work with substantial amounts of data without your program becoming slow or inefficient.
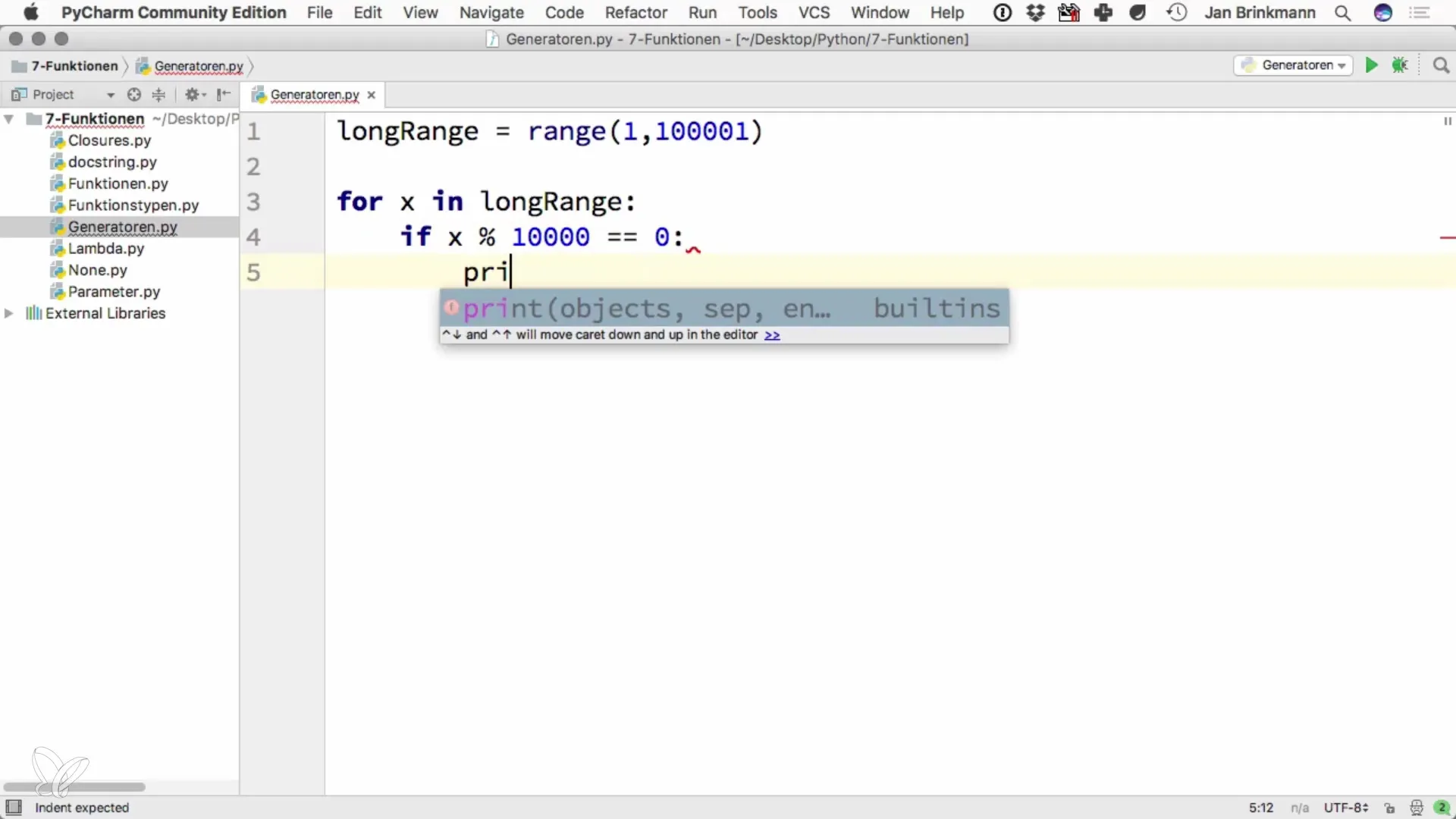
Conclusion and Next Steps
After understanding the basics and the practical application of generators in Python, you can now start generating and processing your own data streams. To deepen your knowledge, try working with more complex generators or combine generators with other Python constructs that can help you in your projects.
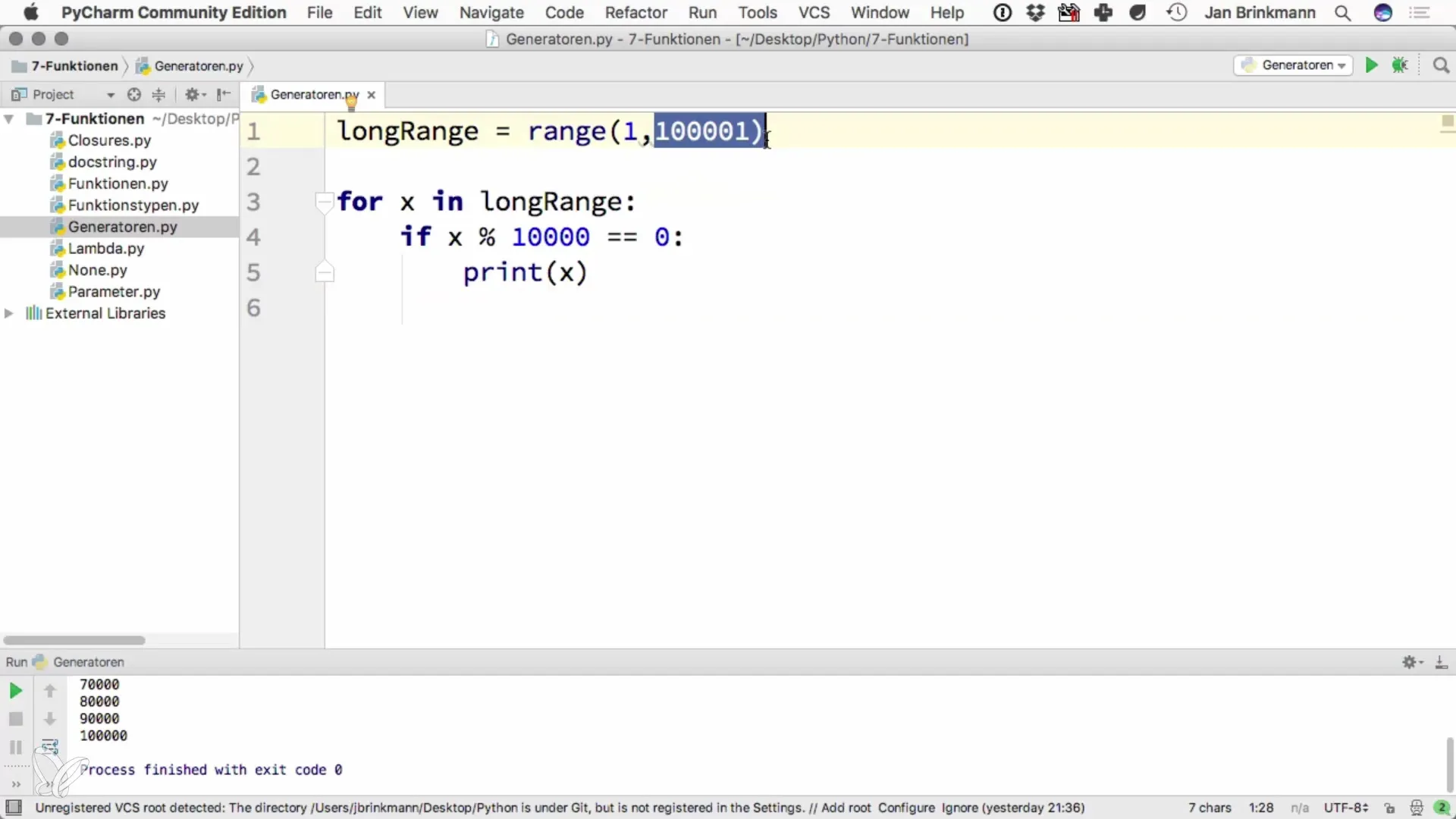
Summary – Generators in Python: How to Use Them Effectively
Generators offer a powerful way to process data efficiently by minimizing memory usage and increasing performance. You have learned how to create your own generator functions and effectively implement them in your projects. Future experiments and experiences will show you how versatile and useful generators truly are.
Frequently Asked Questions
What is a generator in Python?A generator in Python is a special type of function that produces values on-the-fly without holding all values in memory at once.
How does the keyword 'yield' work?The keyword 'yield' returns a value and pauses the function so that it can be resumed at exactly that point during the next call.
When should I use a generator?Generators should be used when working with large datasets where you do not want to hold all data in memory simultaneously.
Can I use generators instead of lists?Yes, you can use generators instead of lists, especially when memory consumption could pose a problem.
How do I start with generators in my project?Start by creating simple generator functions and using them in loops to generate and process data.