Do you want to implement a simple project in Python to deepen your programming skills? In this tutorial, you will learn how to read values and calculate the average of these values. The project is practical and will help you effectively apply the fundamentals of programming in Python.
Main Insights
- Using inputs to collect multiple values
- Calculating the average from the entered values
- Applying basic programming structures in Python
Step-by-Step Guide
First, you define how the values are entered. You can use a loop that allows you to collect multiple values until you decide to end the input. The input occurs via the console, so you can enter temperature values, e.g., in degrees Celsius.
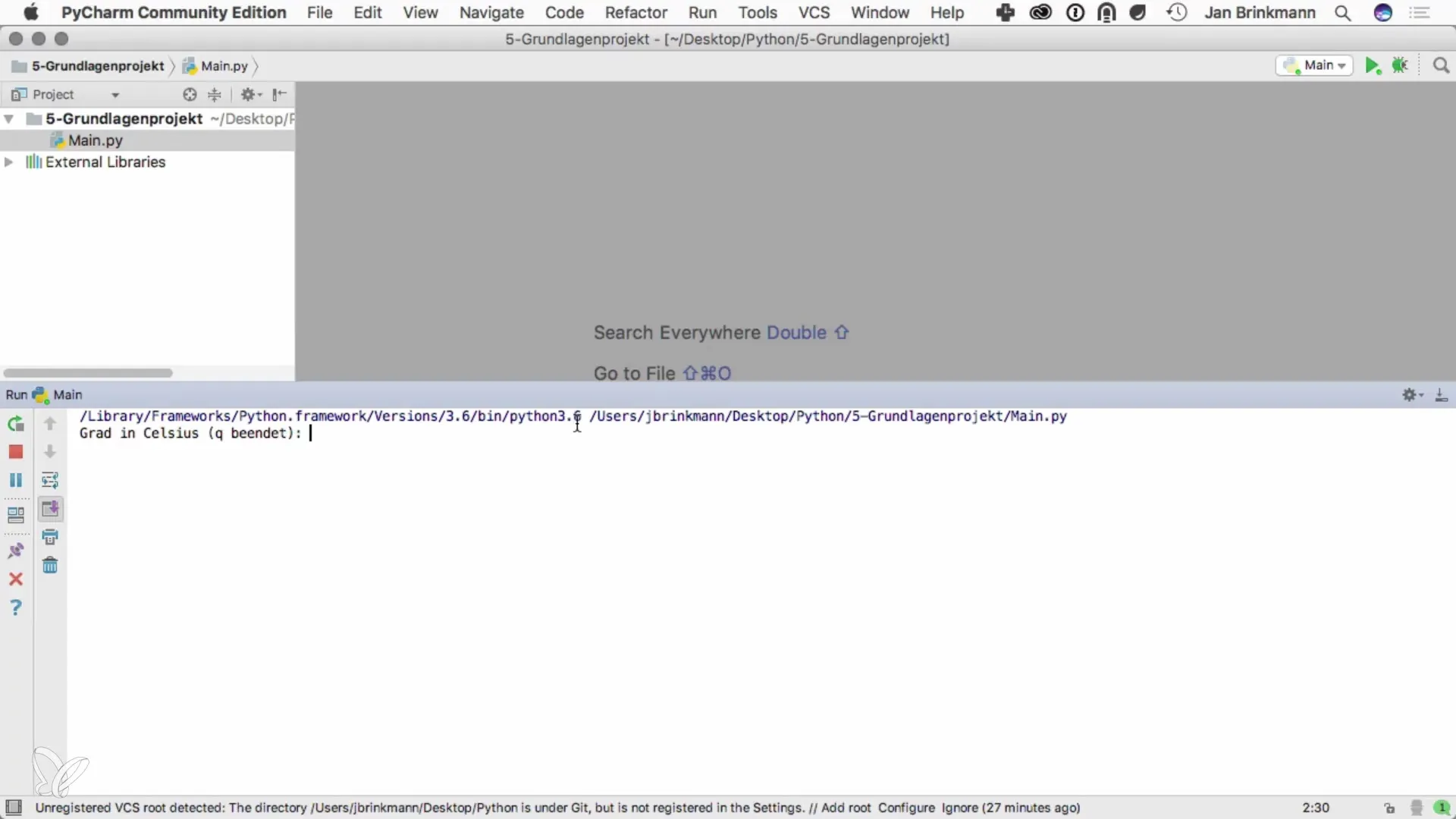
When the user is ready to end the input, they can enter a specific command – in this example, the character "Q". This character is used to terminate the loop and stop the input. In practice, this means that you can confirm the values after input by pressing the "Enter" key.
In the next step, you should ensure that you can store the values as floating-point numbers in order to perform an accurate average calculation. It is important that the input values are stored in an appropriate data structure type like a list, so that you have access to them later and can carry out the average calculation.
A very important part of the implementation is the ability to capture decimal numbers. If the user wants to enter, for example, 15.6 or 13.7, the program must be able to correctly interpret these values and convert them into the appropriate format.
After all the values have been collected, you can calculate the average temperature. To do this, you sum all the entered values and divide the sum by the number of values. This is done in a clear, easy-to-understand step to ensure that the calculation is comprehensible for everyone.
The result is then output. Choose a meaningful output that shows the user what average temperature has been calculated based on the entered values. Make sure that the representation of float values is done through the standard output in the terminal and that it is understandable and clear.
The entire program runs in an infinite loop, which means that you can enter multiple values without having to restart the program. This allows you to test the application and try different invocation patterns to obtain various average values.
At the same time, any feedback is implemented so that the user receives a helpful message in case of incorrect inputs or unexpected data. This enhances user-friendliness and helps address minor errors in the input.
Once all steps for input and average calculation have been successfully implemented, the project is complete. You have now created a simple Python application that puts basic programming knowledge into practice.
Summary – Reading Values and Calculating Average with Python
In this tutorial, you learned how to read values with Python and calculate the average of these values. You discovered how to use an infinite loop to enable data input and how to ensure that the data is handled correctly. Use this knowledge to develop further projects and expand your programming skills.
Frequently Asked Questions
How can I start the program?The program runs in a Python environment.
Which data structures should I use?A list is best suited for storing the values.
How can I ensure that I enter floating-point numbers?Use the input function to ensure that decimal numbers are read correctly.
What happens if I make an incorrect input?The program should provide feedback if inputs are not in the expected format.
Which command do I use to end the input?Just enter the character "Q" to end the input.