Handling user input is a fundamental skill in programming, especially when it comes <strong> to creating interactive programs. In this excursus you will learn how to read inputs on the command line in Python. This is particularly useful for developing dynamic applications and enriching the user experience.
Key takeaways
- The input() function in Python allows reading user input on the command line.
- Inputs can be processed as strings and used for logical evaluations.
- Interactive programs promote better user engagement and make applications more lively.
Step 1: Introduction to input in Python
To begin, let’s look at how you can implement user interaction in Python. The simplest method for this is using the input() function. This function allows you to ask a question to the user and capture their response.
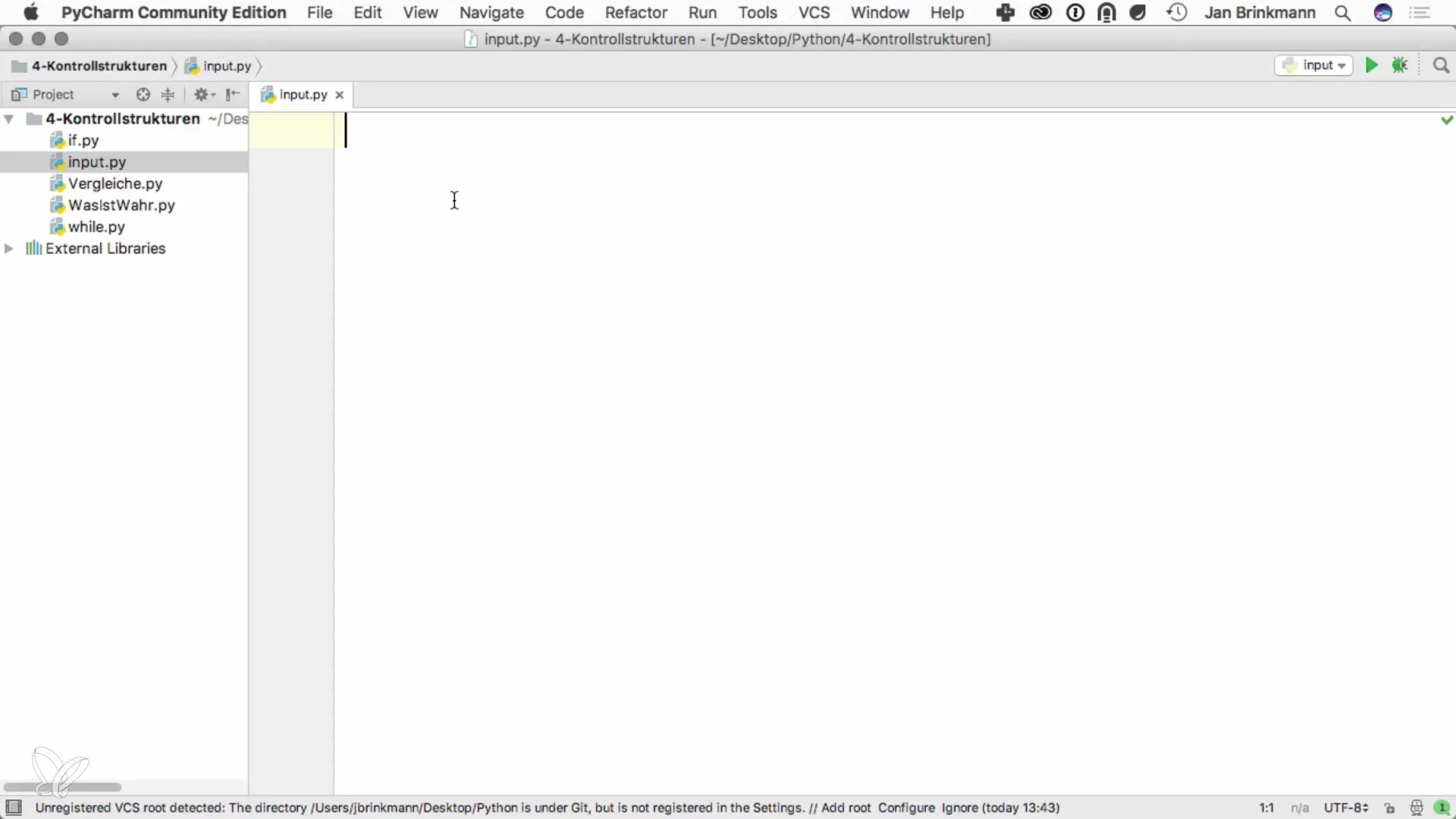
Step 2: Defining a user prompt
Suppose you want to ask a simple quiz question: "Which door do you choose, door 1, 2, or 3?". To allow the user to answer, you formulate the prompt accordingly. It’s important to make the question clear and understandable so the user knows what is expected of them.
Step 3: Processing the input
After the question is asked, you need to read the user input with the input() function. This input is always processed as a string, so it’s important to possibly evaluate the input later to determine whether the user has won or lost. For example, if the user chose "door 3", your logic could look like this:
Step 4: Logic for evaluating the answer
Now the evaluation of the input comes into play. If the user chose "door 3", you have the option to output: "Won". If another option is chosen, the response would be "Not won". This provides clear feedback to the user and rounds off the interactive experience.
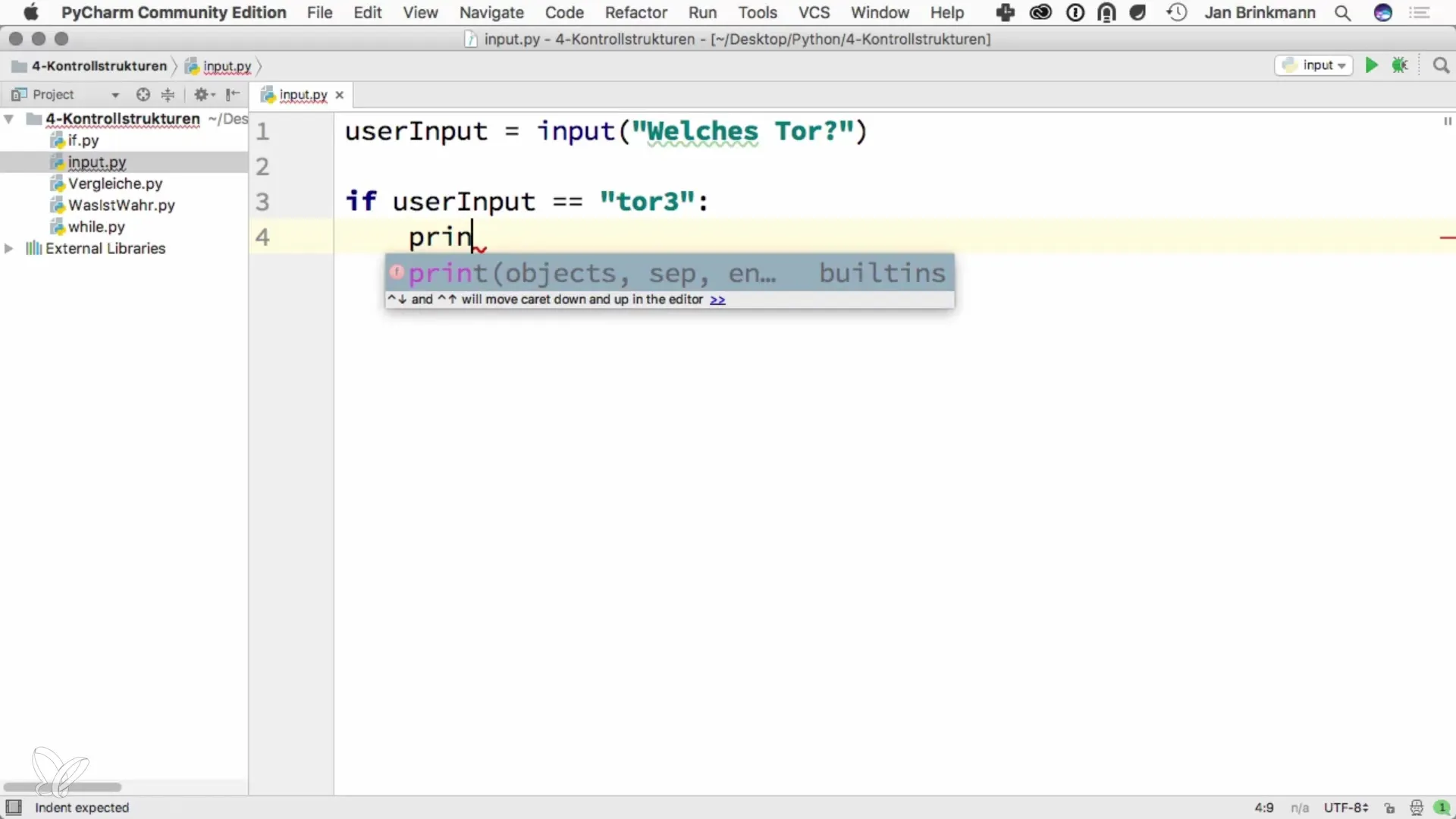
Step 5: Repetition and dynamic input
Finally, it is possible to design this logic so that the user has multiple attempts. After the first input has been evaluated, you could ask the user if they want to play the quiz again. This creates a dynamic and engaging user experience.
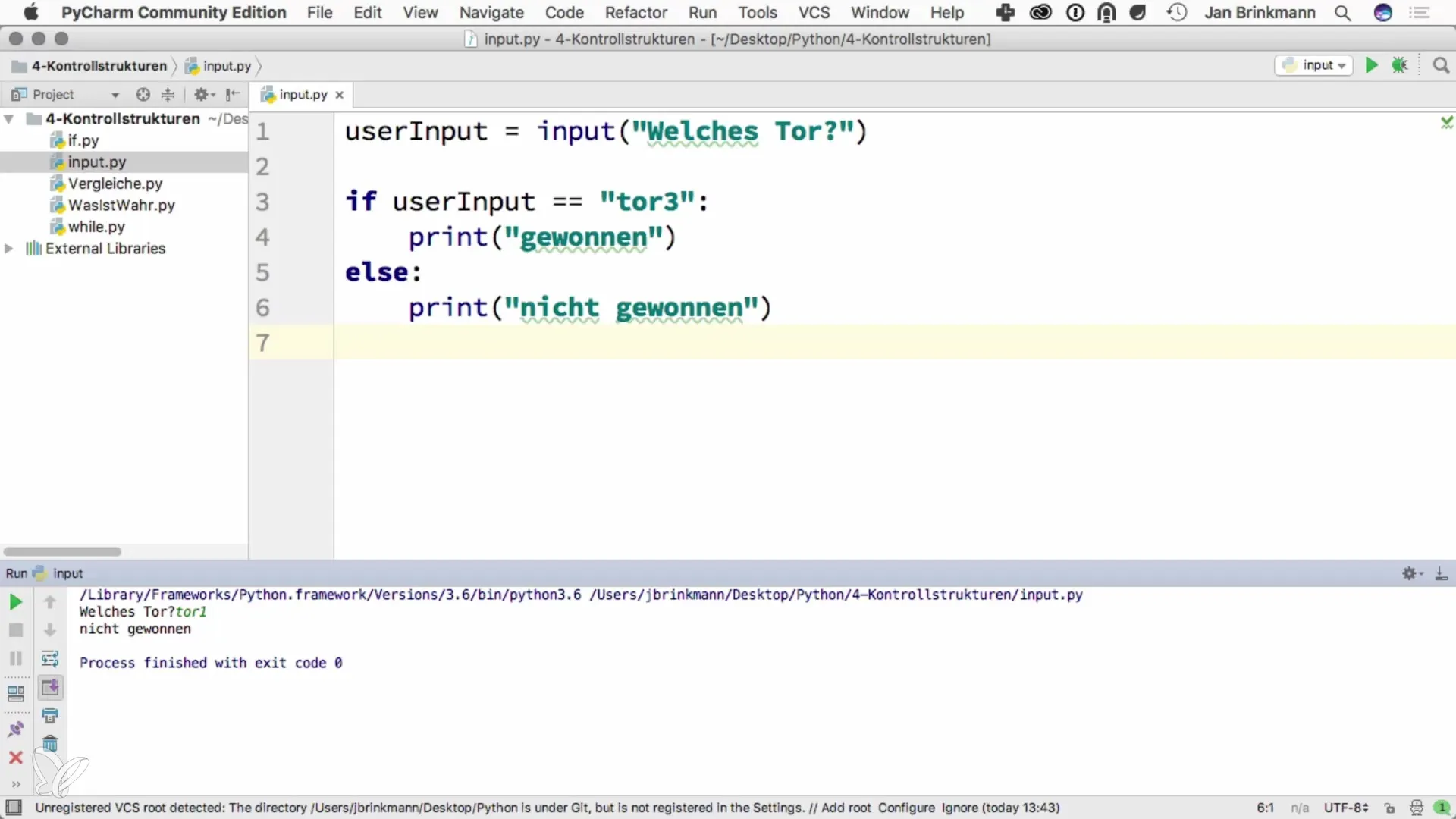
Summary – Programming with Python: Inputs on the command line
In this short excursus, you have learned how to effectively read user input on the command line in Python using the input() function. You have seen how important it is to formulate prompts clearly and how to handle user responses to create an interactive program.
Frequently Asked Questions
How does the input() function in Python work?The input() function reads a user’s input from the command line and returns it as a string.
Can I check the user’s response for a specific type?The response is processed as a string. However, you can convert it to other data types if necessary, for example using int() for integers.
How can I give feedback to the user?You can use the print() function to output a message showing the result of the user’s input, e.g. whether they won or lost.