If you work in programming with Python, it is essential to handle errors effectively. Often, the standard error handling is not sufficient, so you should define your own exceptions. This gives your code even more meaning and structure. In this tutorial, you will learn how to create and manage your own exceptions in Python.
Main insights
- Creating your own exceptions is done through class definition.
- Exceptions can easily be raised using the raise keyword.
- Using try and except blocks allows for specific error cases to be handled.
- A differentiated error handling enables you to respond appropriately to different situations.
Step 1: Basics of Exceptions
First, you should understand what exceptions are. An exception is essentially a type of error that can occur during the execution of a program. Python has many built-in exceptions, but sometimes you have no choice but to define your own exceptions. To do this, you can create a class that inherits from the base Exception class.
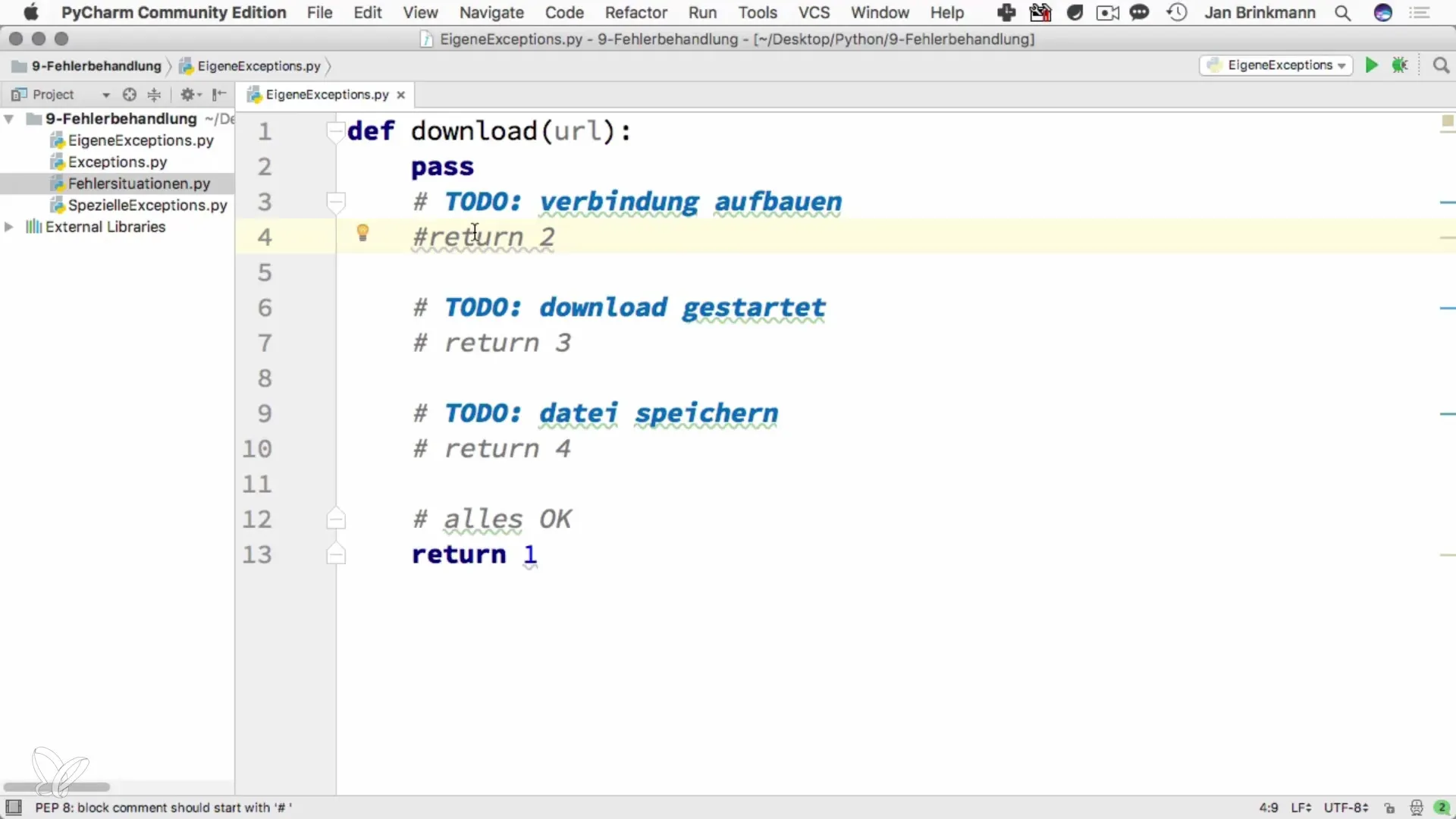
Step 2: Define your own Exception
Create a class for your own exception. For example, you can define a class ConnectionException. The name should start with a capital letter to adhere to Python's style.
From now on, you can use the ConnectionException anywhere in your program when there is a connection error.
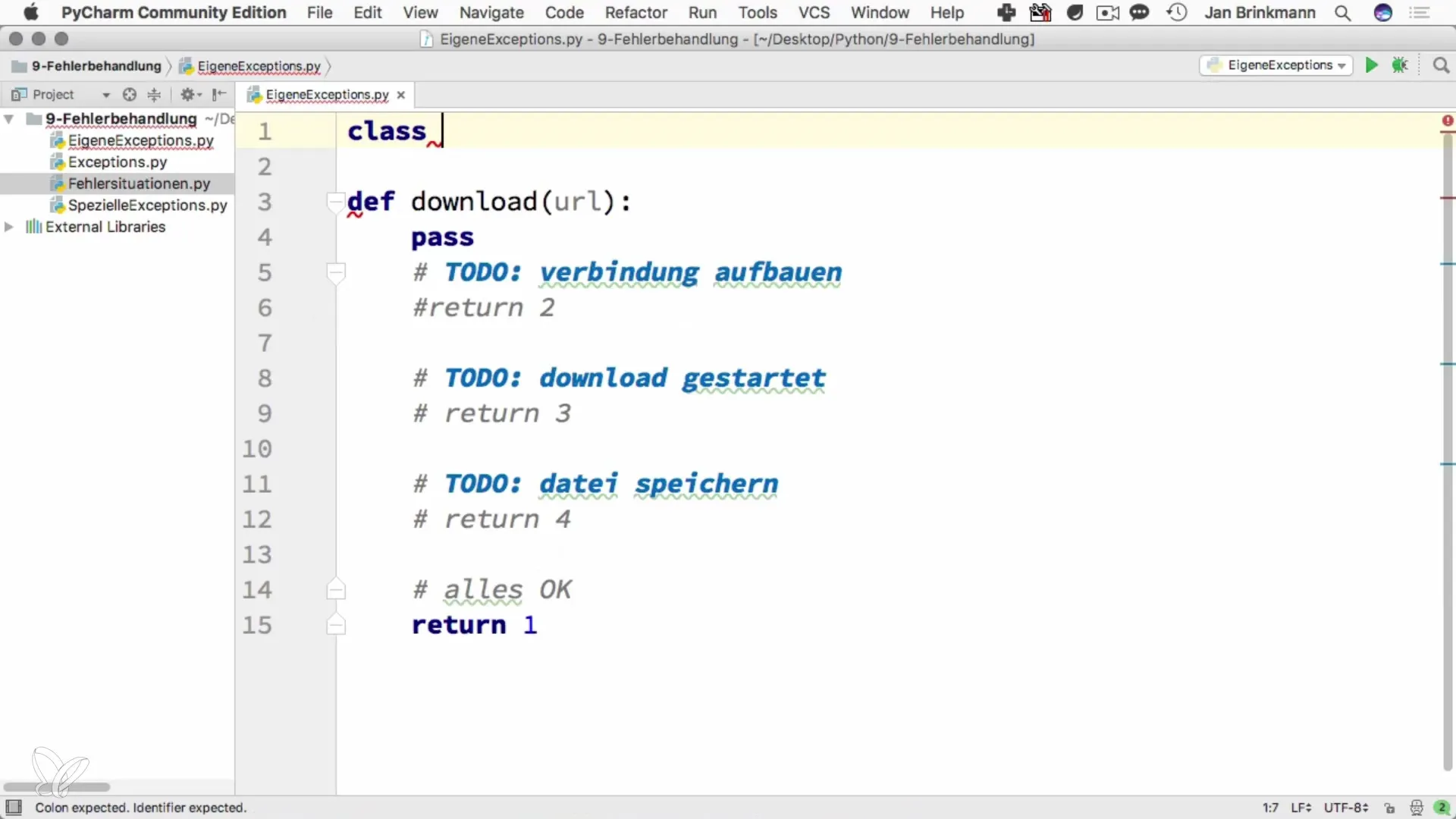
Step 3: Using the Exception in your code
Now that you have defined your own exception, you can implement it in your code. Use the raise keyword to raise the exception.
This way, the function signals that an error has occurred, without abruptly terminating the program.
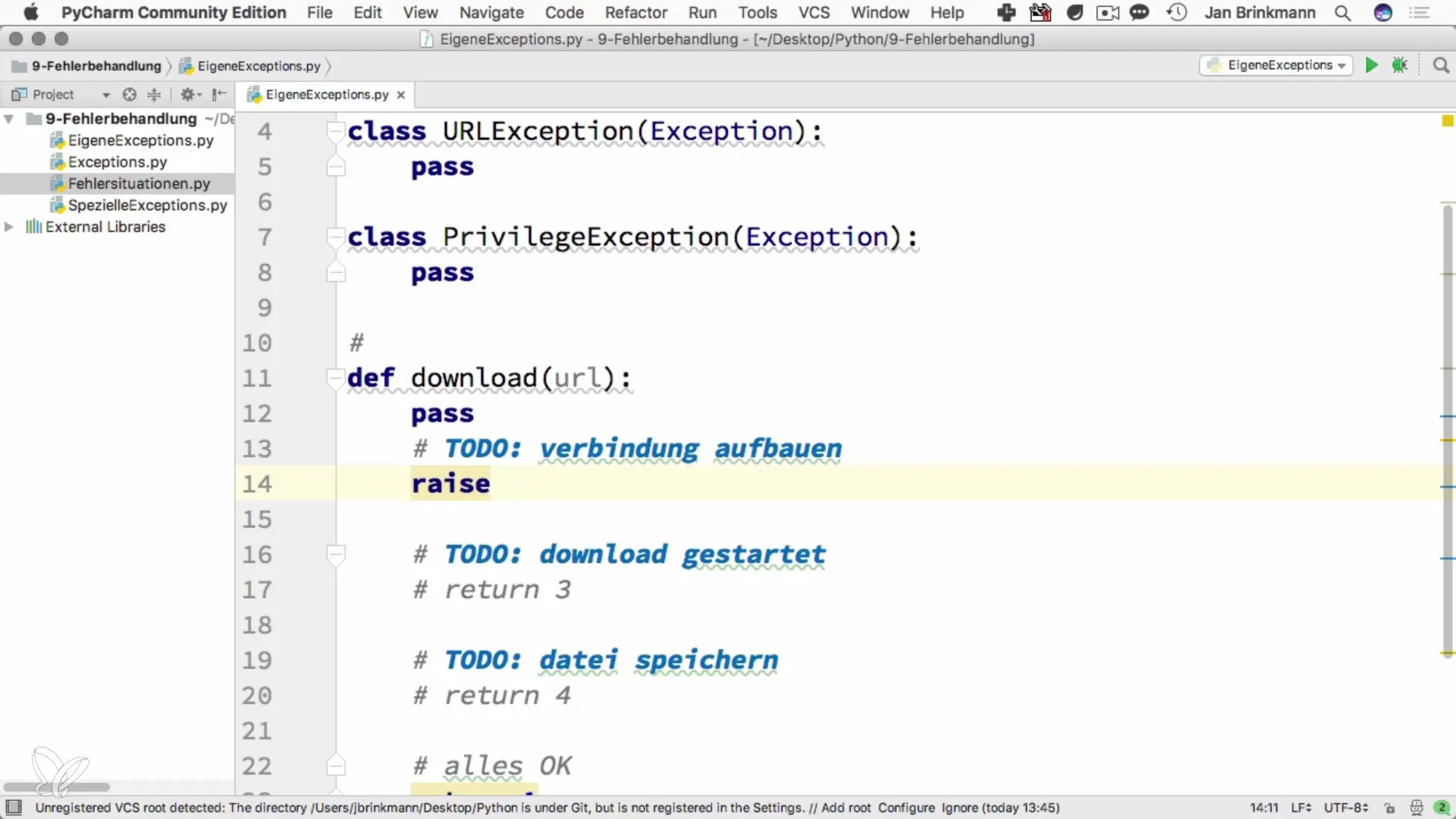
Step 4: Catching errors with try and except
To prevent your program from crashing unexpectedly when exceptions occur, you should use try and except to handle errors.
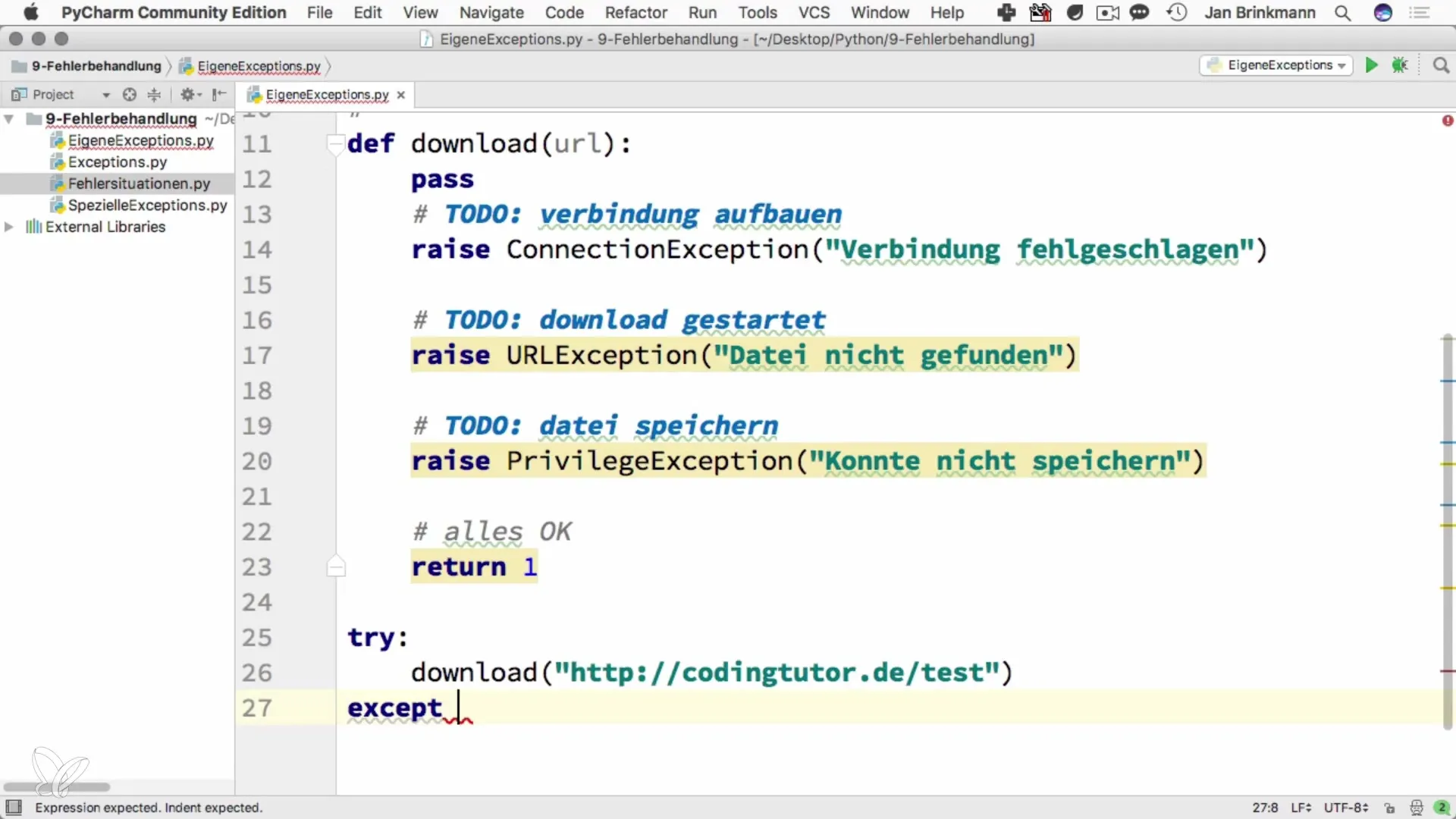
Step 5: Multiple types of Exceptions
You can define multiple types of your own exceptions to handle different errors that may occur in your program. For instance, you could also create a URLException:
After that, you would use it in a similar way:
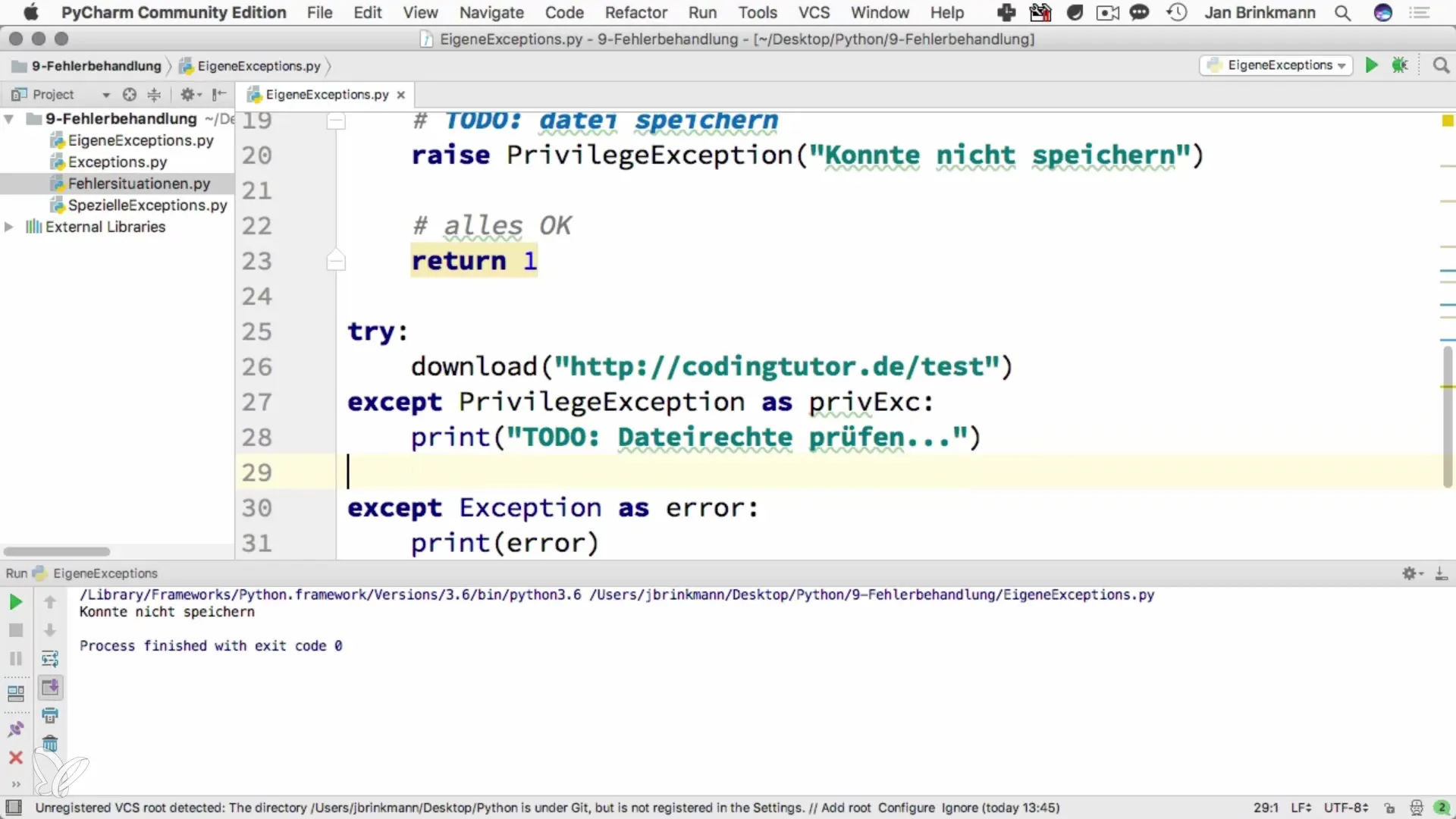
Step 6: Specific error handling
Sometimes you want to handle specific errors differently. You can use different except blocks for different exceptions.
With this type of error handling, you create more clarity in your code, as you can specifically address different types of errors.
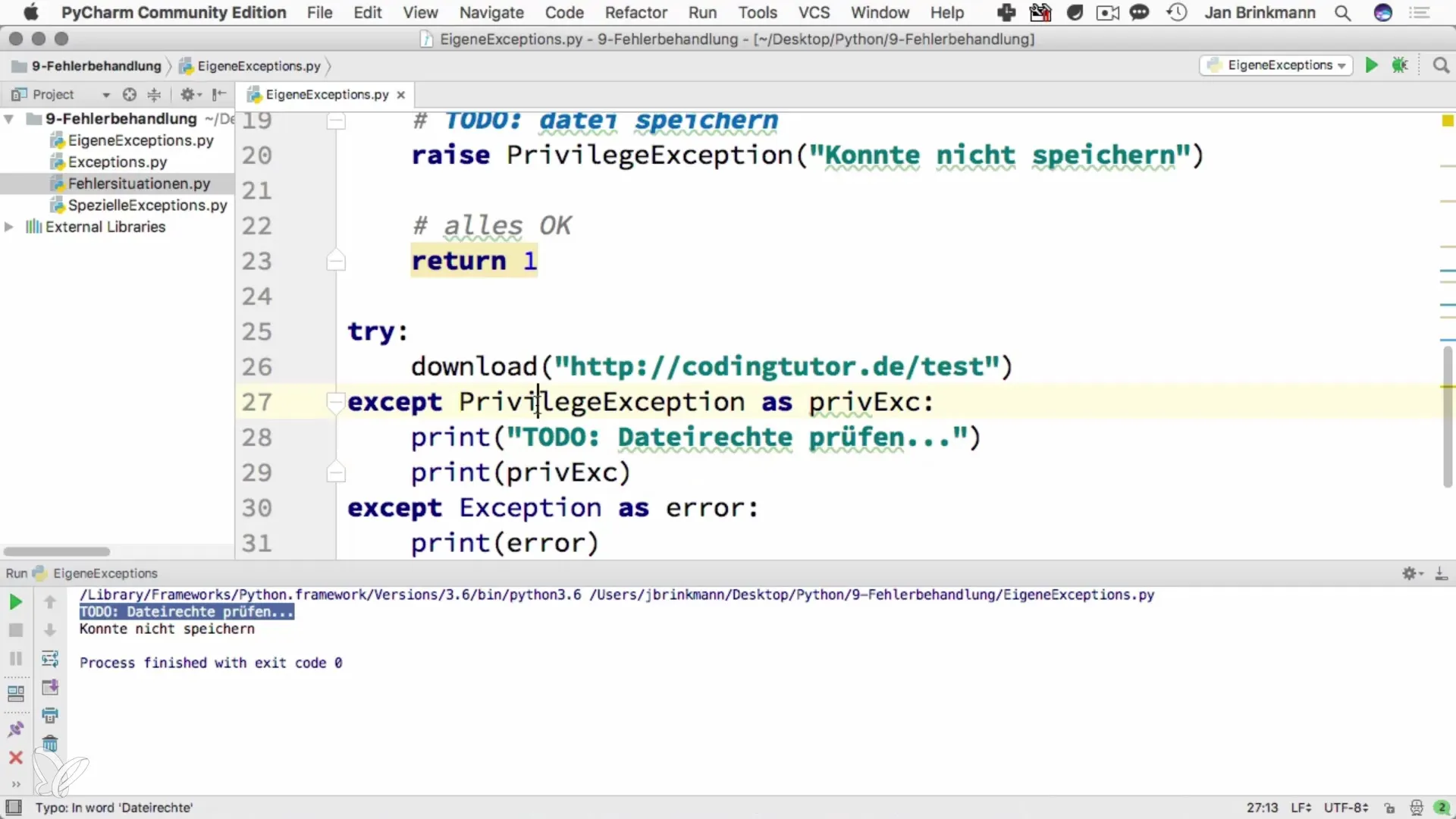
Step 7: Testing and validation
It is advisable to test your exceptions and ensure they are raised in the right context. Adjust your code to check when and where errors may occur, and make sure that the outputs make sense.
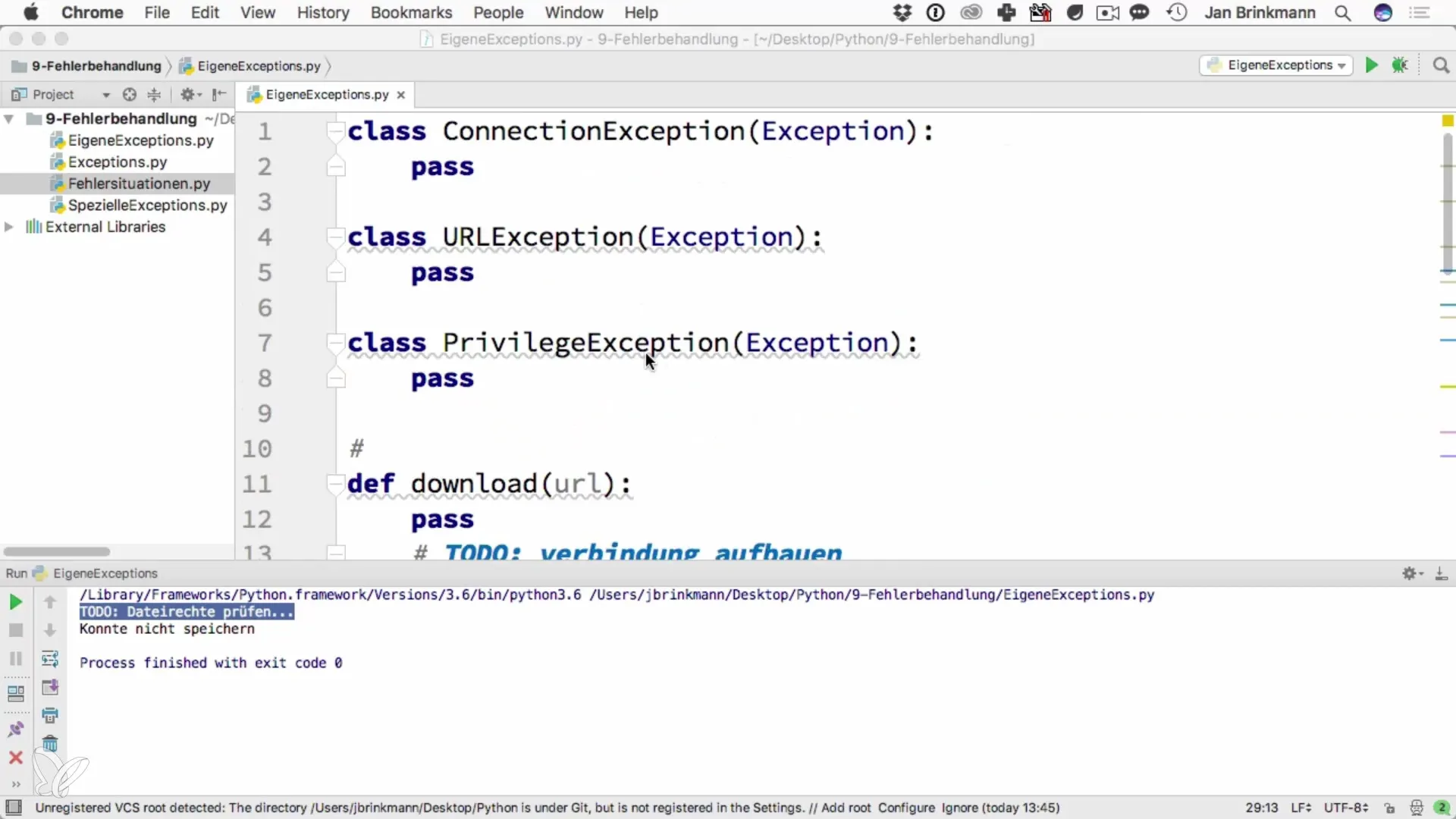
Summary – Defining your own Exceptions in Python
Creating your own exceptions in Python is a simple yet effective step to optimize error handling in your code. With the help of classes, you can define specific types of errors and trigger them with raise. try and except blocks allow you to implement robust error handling tailored to the different needs of your program.
Frequently Asked Questions
How do I define my own Exception in Python?You can define your own Exception by creating a class that inherits from the Python Exception class.
How do I raise an Exception in my code?Use the raise keyword followed by the Exception you want to raise.
Can I have multiple custom Exceptions in a program?Yes, you can define as many custom Exceptions as you like to handle different errors.
How can I handle errors specifically?Use different except blocks to handle different types of errors separately and take specific actions.