Python is a programming language known for its readability and simplicity. A central concept that you must understand when programming in Python are spaces used to define code blocks. In this guide, you will learn how to use spaces and indentation in Python effectively and which common mistakes to avoid.
Key takeaways
- Spaces are crucial for the structure of your code in Python.
- Indentation defines which statements belong to a block.
- There are no curly braces to delineate code blocks, only spaces.
- Consistent use of spaces makes the code easier to read and understand.
Step-by-Step Guide
Step 1: Create a New Python Project
Start by creating a new directory for your project. Then open your preferred development environment and create a new Python file. You could name it, for example, “my_app.py”. This file will be the main file of your project.
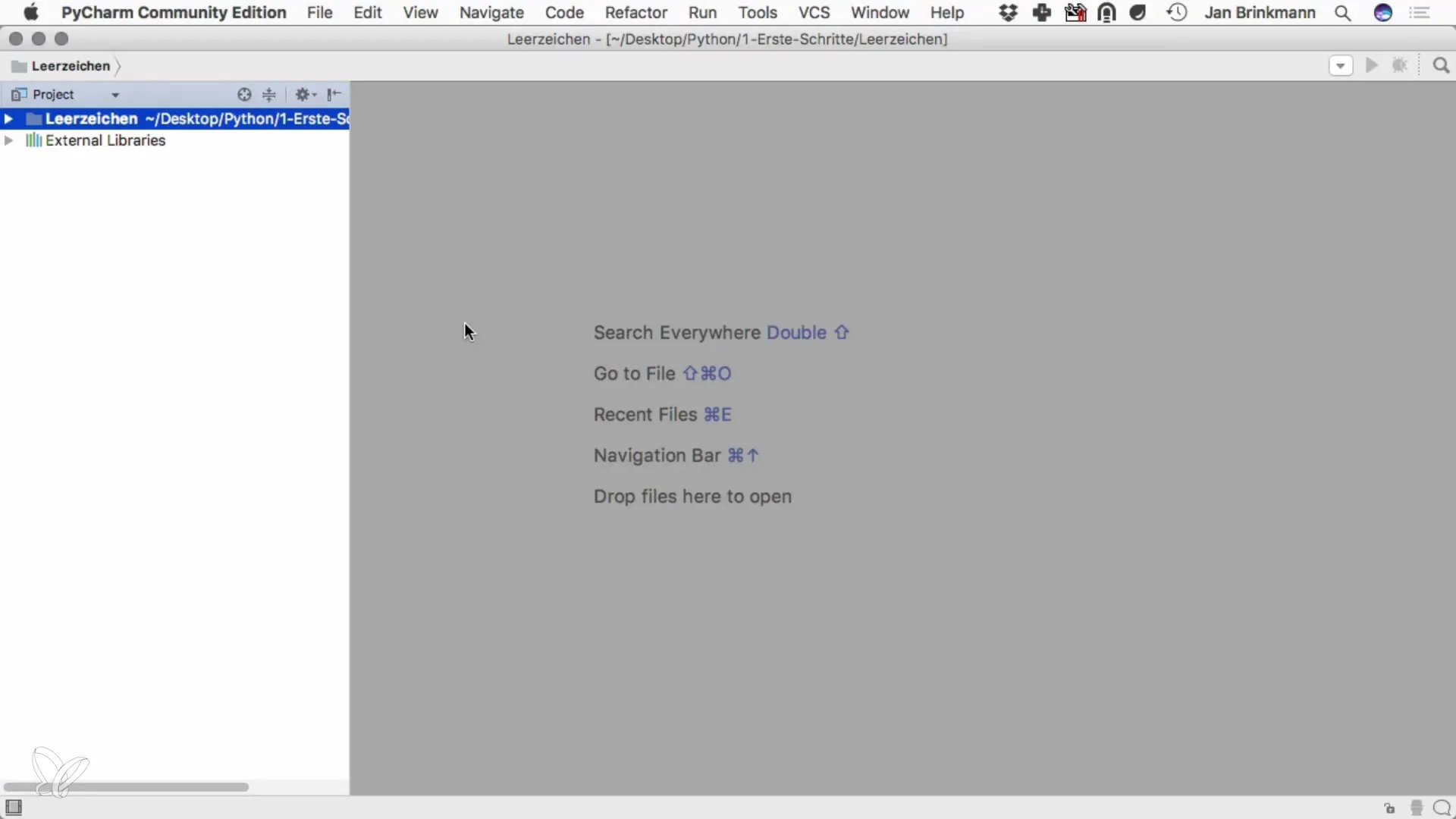
Step 2: Define a Function
To start programming functions, write the definition of a function using the def keyword. For example, you could create a function named sag_hallo. The function is defined in Python by indenting code blocks, which we will look at in more detail in the next step.
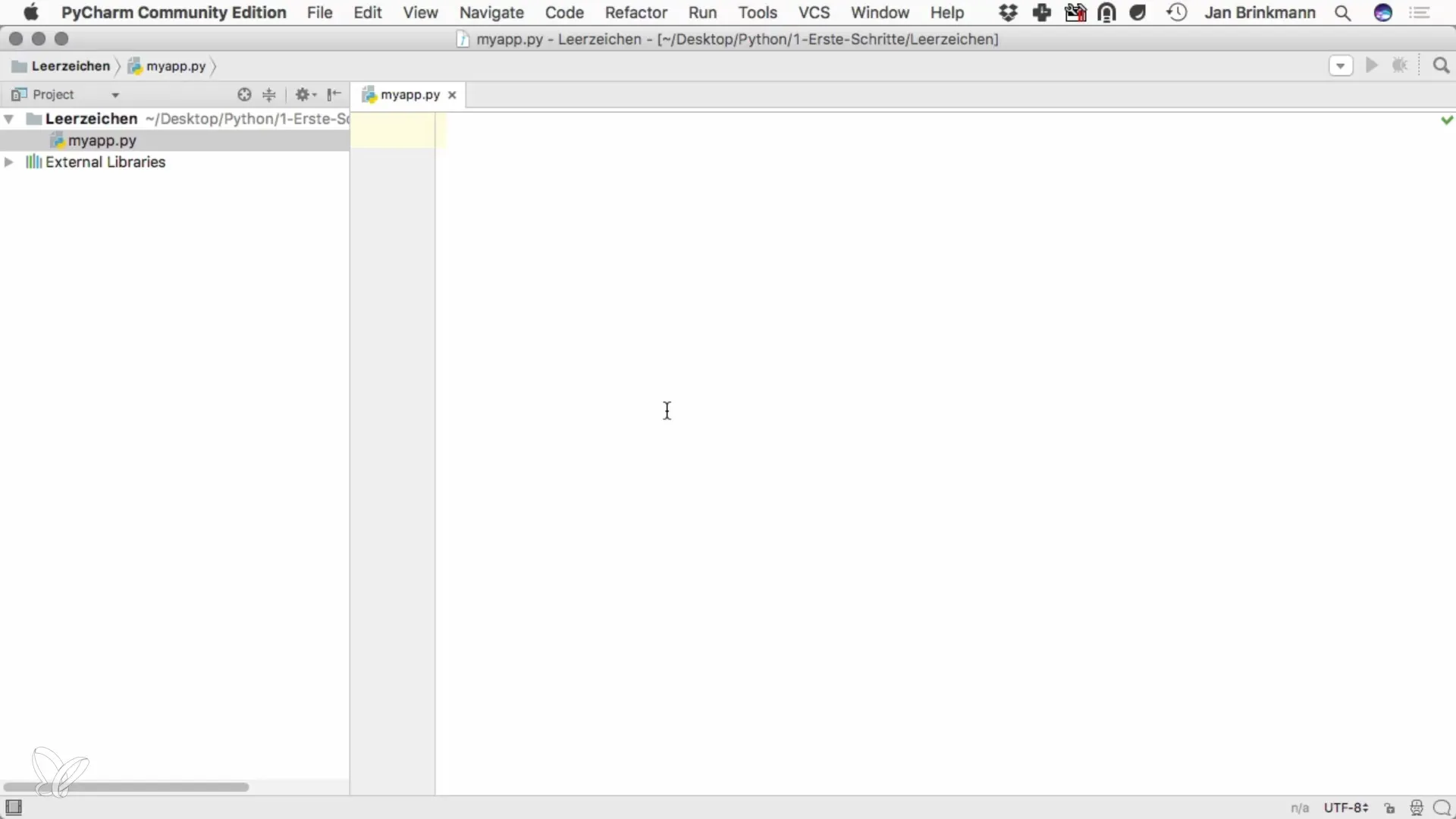
Step 3: Enter Code for the Function
After you have defined the function, add a colon to mark the start of the code block. Then press Enter to move to the next line. You will notice that the cursor shifts slightly to the right. This means you are now inside the function. Here you can add a print statement to output a message to the screen.
Step 4: Understand the Structure of the Function
In other programming languages like PHP, code blocks are delimited by curly braces ({}). In Python, however, this delimitation is done by indentation. It is important to see what belongs to the function and what does not. The context of your function is made clear by the indentation. For example, if you write print("Hallo, Jan") inside the function, this statement is part of the function.
Step 5: Call the Function
To test the function, you can simply call it at the end of your file, as long as you are at the same indentation level as the function definition. When you run the file, the output will be displayed.
Step 6: Adjust Indentation and Spaces
It is important to learn how to use spaces correctly. In Python, the number of spaces you use matters. You have the flexibility to use either five or four spaces, but you should always use the same number of spaces consistently to keep the code clear and understandable.
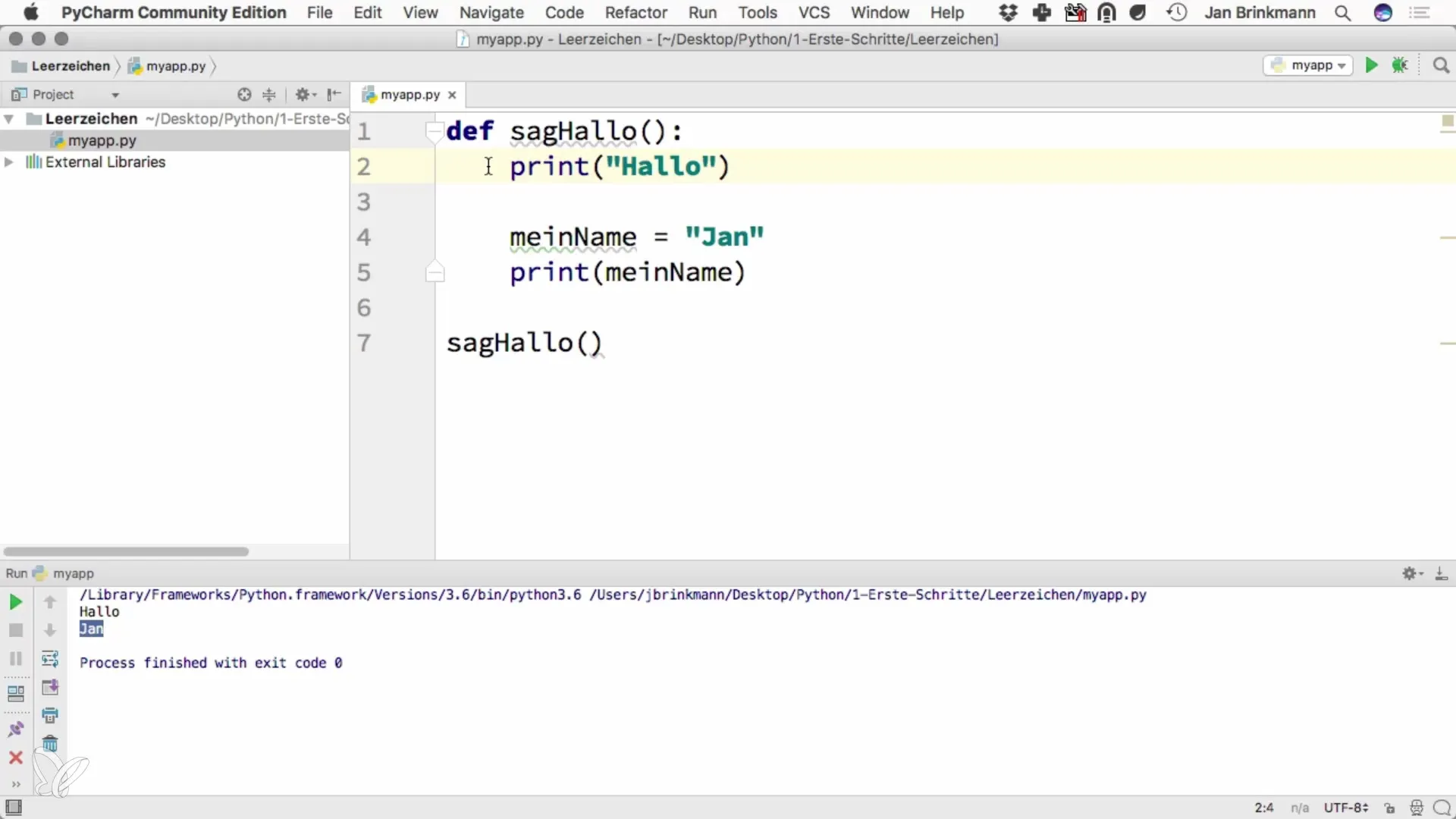
Step 7: Tips and Errors
Make sure that the number of spaces in your code is consistent. For example, if you get an error message saying that the indentation is not a multiple of four, it means you should check the number of spaces. Too many or too few spaces can lead to unexpected errors.
Step 8: A Consistent Style
Be sure to use a consistent style for indentation to keep the code readable. It is recommended to always use four spaces, as this is the standard in many code editors like PyCharm. This convention helps maintain a clear structure in the code.
Summary – Programming with Python: Understanding Spaces
In this guide, you learned how important spaces and indentation are in Python. These basic elements help you structure your programs clearly and avoid misunderstandings. Make sure to apply the principles of indentation to write clean and maintainable code.
Frequently Asked Questions
How do I define a function in Python?You use the def keyword followed by the function name and a colon.
Can I use different amounts of spaces?Yes, but it’s important to remain consistent to avoid errors.
Why are spaces important?They indicate which statements belong to a block and support readability.
What happens if I don’t indent properly?It can lead to errors in your code, as Python uses spaces for structuring.
How many spaces should I use?Usually, four spaces are recommended to improve readability.