JavaScript has evolved significantly over the years, providing developers with increasingly powerful tools. One of the most significant improvements in recent versions is the introduction of Arrow-Functions (=>) with ES6. This new syntax not only makes functions more compact but also resolves issues related to the context of this. In this guide, I will teach you the basics of Arrow Functions and show you how to use them effectively in your code.
Key Insights
- Arrow Functions provide a shorter syntax for declaring functions and capture the context of this in an intuitive way.
- They are particularly useful in functionalities that expect return values in the form of expressions.
- Using Arrow Functions can make the code more readable and maintainable.
Step-by-Step Guide
Arrow Functions have their own syntax, which differs from traditional function declarations. Let’s go through various aspects of this syntax together so you can understand how it works and where you can use it.
First of all, traditional functions in JavaScript. Here you see an example of how a function is declared using the function keyword:
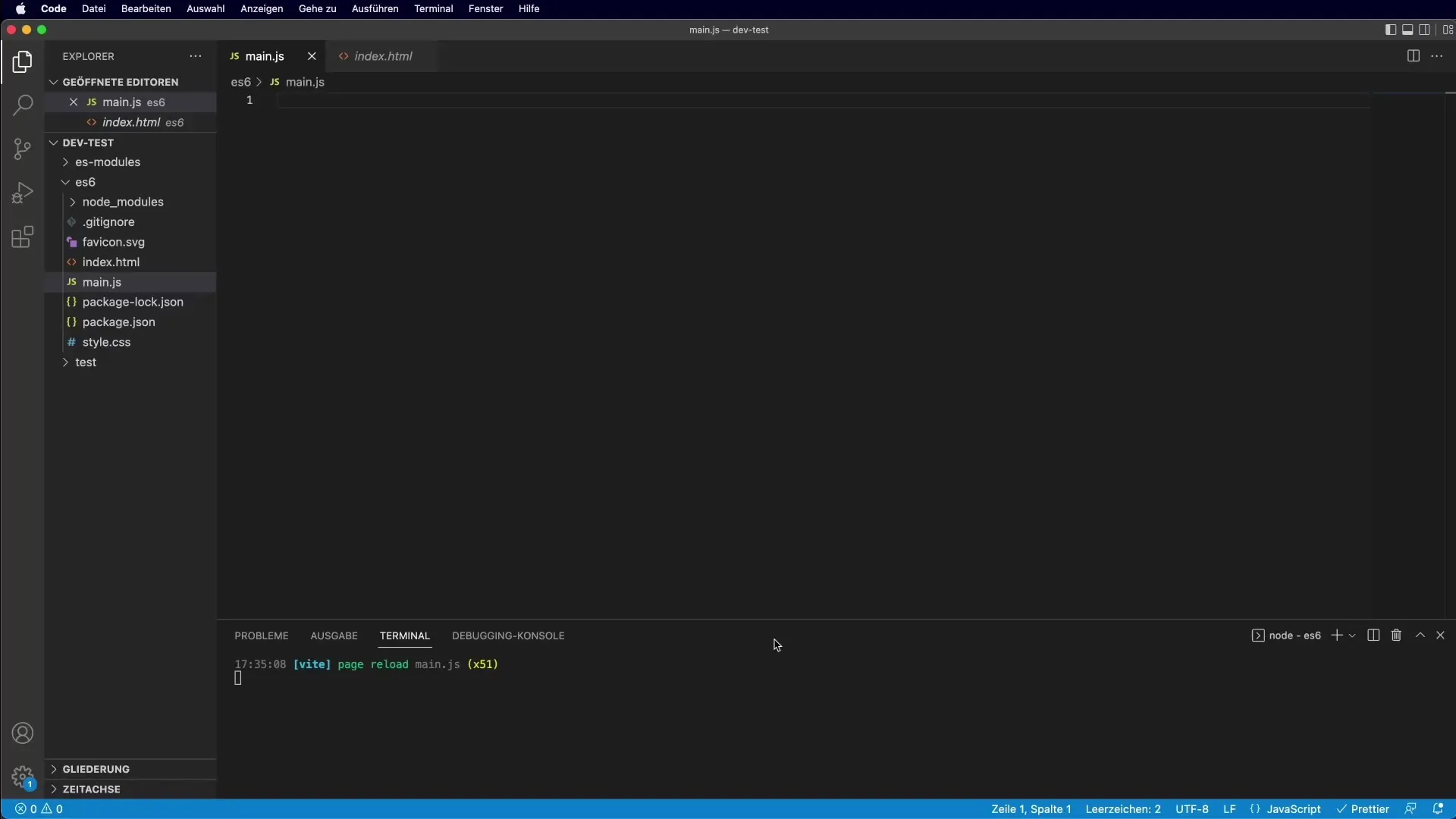
We pass this function, for example, to setTimeout to execute an anonymous function after one second:
This is the standard method for using functions in JavaScript. But we can improve this syntax by omitting the function keyword and instead using an Arrow Function. It achieves the same goal but requires fewer lines of code. The syntax looks like this:
If we take a practical example to illustrate this. I declare an array that contains the values 1 to 5:
Now we can use the findIndex method to find the index of a specific value (e.g., 3) in the array. When using traditional functions, the code looks like this:
This works, but let’s use the Arrow Function to improve the code. We remove the function keyword and use the Arrow syntax:
We can even further shorten the function body by omitting the curly braces and the return keyword. This results in an even more compact form:
This works perfectly, and we get the desired index.
The Arrow Function is compact and significantly simplifies the syntax. Another advantage is the handling of this. Let’s look at an example with objects. You have an object that has a method accessing a property in the object:
If you call this method with a setTimeout and write it as a traditional function, this will lead to a problem. The output of this will no longer be the expected object:
This will be undefined. However, if you replace the function keyword with an Arrow Function, this remains anchored in the surrounding context:
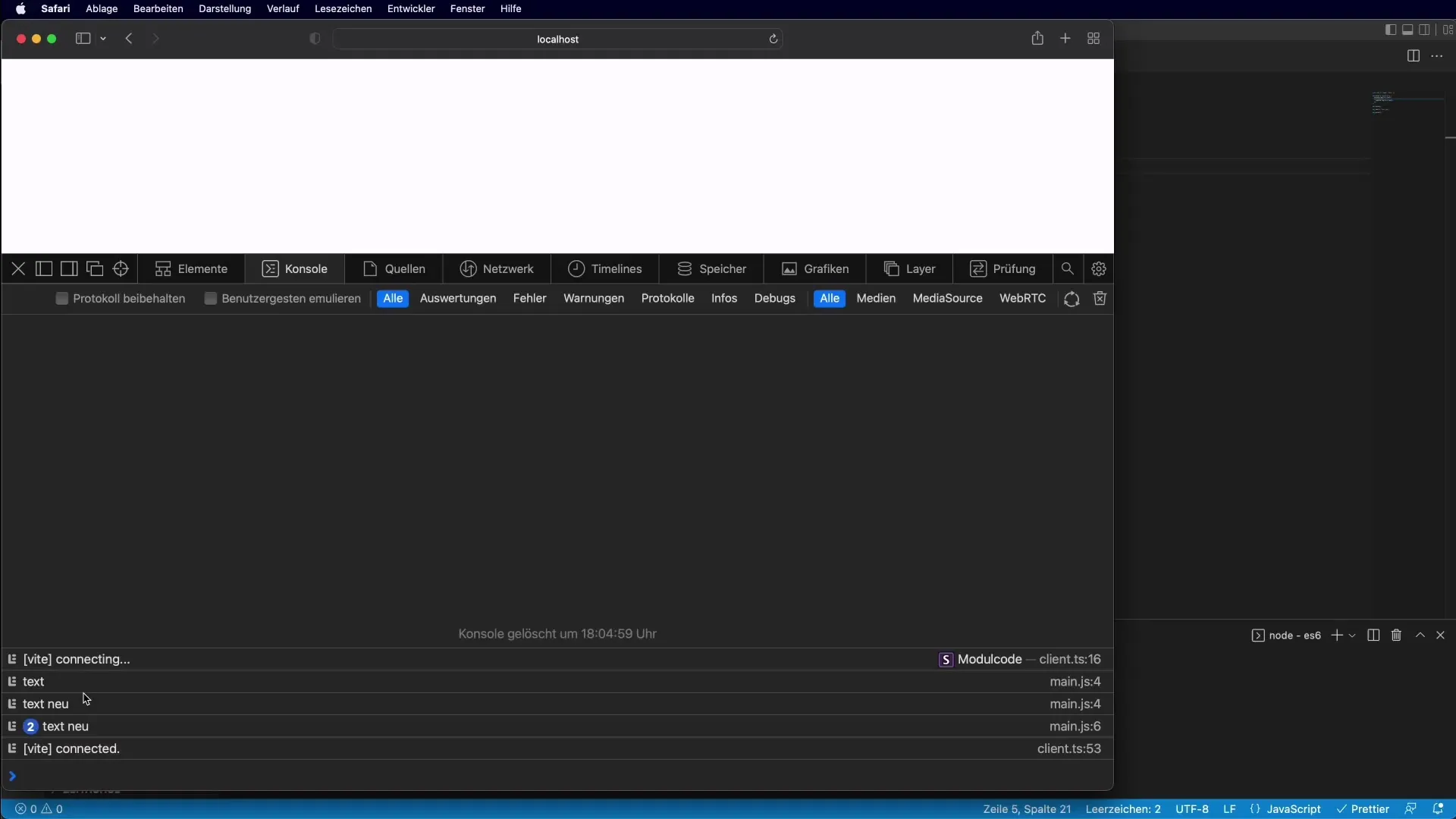
Here, the output should be correct and display the text in the console. The Arrow Function has mitigated the loss of context of this in this example.
Where else can we use Arrow Functions? They are particularly handy when you want to pass functions to methods like map, filter, or reduce. These methods expect a function as an argument and benefit from the shorter syntax. Here’s a simple example with map that squares the elements of an array:
Another useful feature of Arrow Functions is that you don't need curly braces when your function only returns an expression. This makes the code even simpler and clearer:
However, note that in certain situations, such as with multi-line function bodies, using the function keyword remains mandatory. So it’s up to you to choose the appropriate method based on the context.
Summary - Arrow Functions in JavaScript Explained Clearly
Arrow Functions are a valuable addition in JavaScript that not only reduces writing effort but also improves the readability of the code. Thanks to their intuitive handling of this, they are particularly useful in modern web applications. By using Arrow Functions appropriately in your code, you can benefit from a significant improvement in maintainability.
Frequently Asked Questions
What are Arrow Functions in JavaScript?Arrow Functions are a compact syntax for declaring functions that were introduced in ES6.
How do Arrow Functions differ from traditional functions?Arrow Functions use a different syntax and maintain the context of this, while traditional functions do not.
When should I use Arrow Functions?Use Arrow Functions when writing short functions or passing functions as arguments to other functions.
Are Arrow Functions a complete alternative to traditional functions?Not always. In some cases, such as with methods that rely on the context of this, you must use traditional functions to achieve the desired functionalities.
Are there any drawbacks to Arrow Functions?Yes, Arrow Functions cannot be used as Constructors, and they cannot be used in objects or classes where this has a specific meaning.