The dynamic importing of modules in JavaScript allows you to load modules at runtime based on conditions or events. This improves the performance of your applications since not all modules need to be loaded at the beginning. In this tutorial, you will learn how to effectively use dynamic imports to optimize your web applications.
Key Insights
- Static imports are required at the beginning of a file and cannot be used within functions.
- Dynamic imports allow loading modules at any point in the code and handling returned promises.
- Using await simplifies promise handling and enables a more readable syntax.
Step-by-Step Guide
1. Static Imports vs. Dynamic Imports
Before you start with dynamic imports, you should be aware of the differences between static and dynamic imports. Static imports must be at the top of a file and can be used throughout the module. They are easy to identify and provide clear dependencies. Dynamic imports, on the other hand, allow you to load modules flexibly when they are needed. This capability is referred to as "Lazy Loading" and can significantly reduce the loading time of your application.
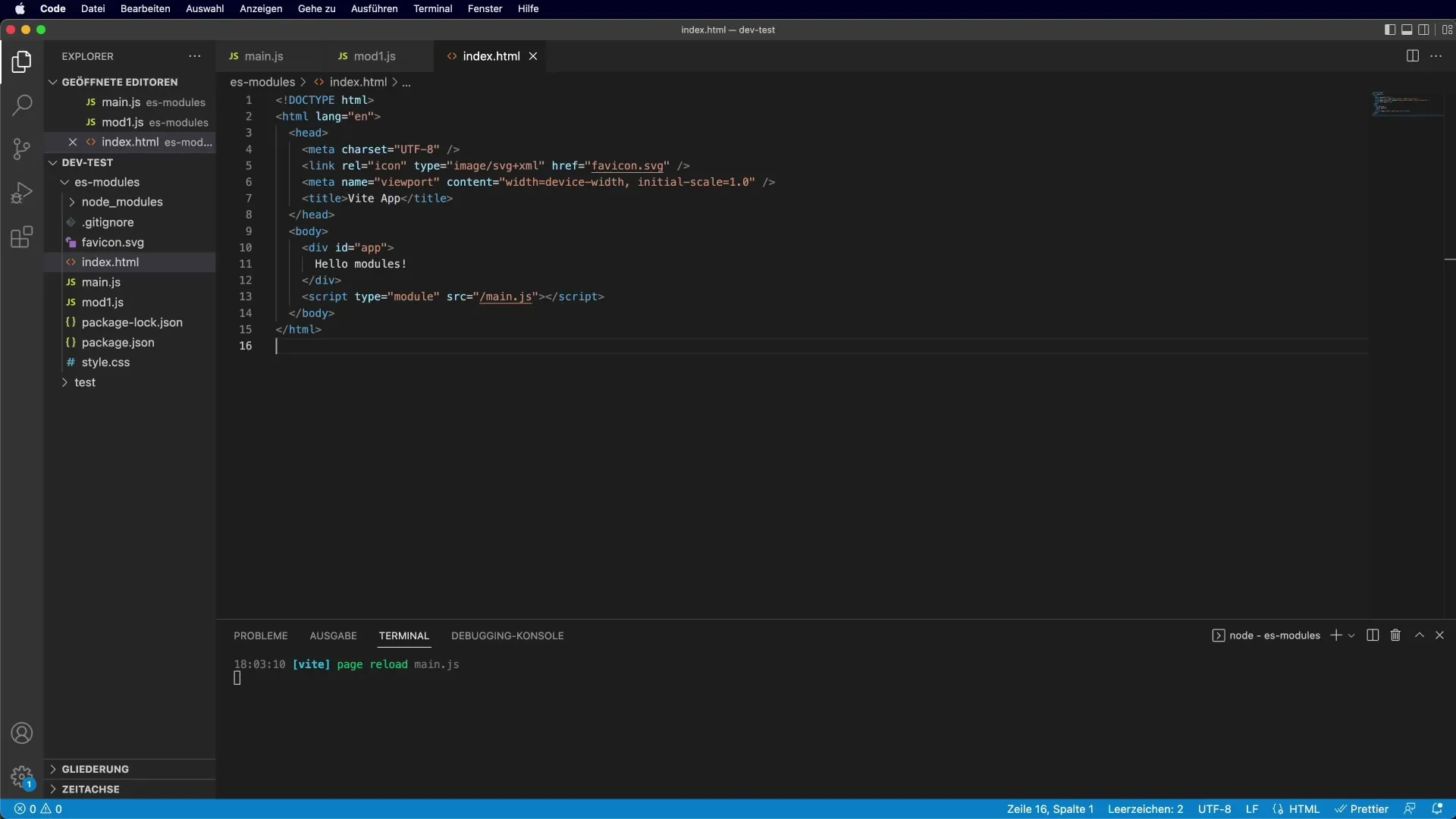
2. Introduction to Dynamic Importing
To start with the dynamic import of modules, you can use the import() function. This function returns a promise, meaning you can work with the module as soon as the promise is fulfilled. At this point, you will create a function where you perform a dynamic import. This gives you the flexibility to load modules based on user actions or certain conditions.
3. Creating a Function for Dynamic Import
Now you can create your function. Let's call it main. In this function, you will dynamically import the module. Don't forget to specify the corresponding path to your module.
This function will load the module only when main() is called, and not during the initial loading of the page.
4. Processing the Imported Module
Once the module is successfully loaded, you can access the exported elements of the module. Note that the import() command returns a promise. This means that you define a callback function with.then() that will be executed when the promise is fulfilled.
Here you have your dynamically loaded module and can access it as if it had been statically imported.
5. Using async/await for Simplified Syntax
To make handling the promise easier, you can use the async/await syntax. To do this, you need to add the async keyword before the function keyword.
This method is particularly readable, as the code looks as if the import was executed synchronously.
6. Integration into the Application
Suppose you only want to load a module under certain conditions – for example, when the user clicks a button.
This way, the module is only loaded when the user clicks the button, which improves the performance of your application.
7. Set Timeout
To make the dynamism even clearer, you can also use a timeout to delay the loading of the module.
Here, main is called after 2 seconds, which dynamically imports the module.
Summary – Dynamic Importing of Modules in JavaScript
In this tutorial, you have learned how to implement dynamic imports in JavaScript. You have learned the differences between static and dynamic imports, grasped the basics of the syntax, and seen how to work with promises and async/await. These techniques help you make your web applications more effective and efficient.
Frequently Asked Questions
How does dynamic importing work in JavaScript?Dynamic importing is done through the import() function, which returns a promise and allows modules to be loaded at runtime.
When should I use dynamic imports?Dynamic imports are ideal when you want to load modules only when needed to shorten the initialization time of your application.
What is the difference between static and dynamic imports?Static imports must be at the top of a file and are loaded immediately at compile time, while dynamic imports can be used at any point in the code and are loaded at runtime.