Working with key-value pairs is a common need in programming. The introduction of the Map data structure in JavaScript has many advantages over the previous use of objects as a sort of dictionary. In this guide, you will learn how to effectively use Maps for your purposes, and what possibilities and functions they offer.
Key insights Maps in JavaScript offer a flexible and type-safe method for storing key-value pairs. They allow the use of various data types and provide useful methods like set, get, and has to work with the data.
Using Maps
Step 1: Create a first Map object
To get started with Maps in JavaScript, you first need to create a Map object. This is done easily with the constructor function new Map().
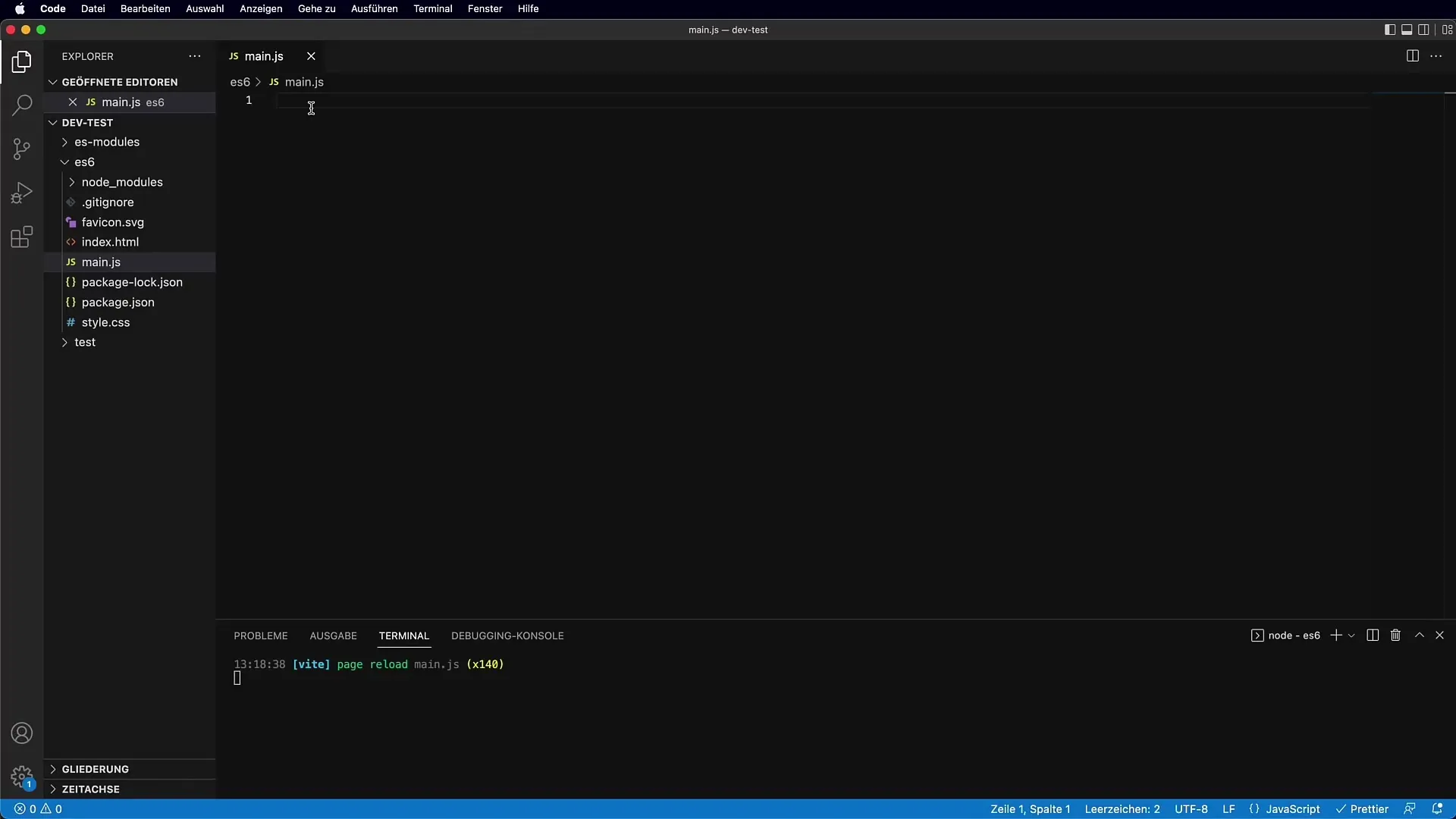
Step 2: Insert keys and values into the Map
After creating your Map, you can add keys and values using the set method. Note that the keys can have any data type, while the values can also be complex objects.
Here you set the key 1234 with the value "value one".
Step 3: Retrieve values from the Map
To retrieve a value from the Map, you use the get method. This method takes the key and returns the corresponding value.
Step 4: Check if a key exists
With the has method, you can check if a specific key is present in the Map. This function returns a boolean value (true or false).
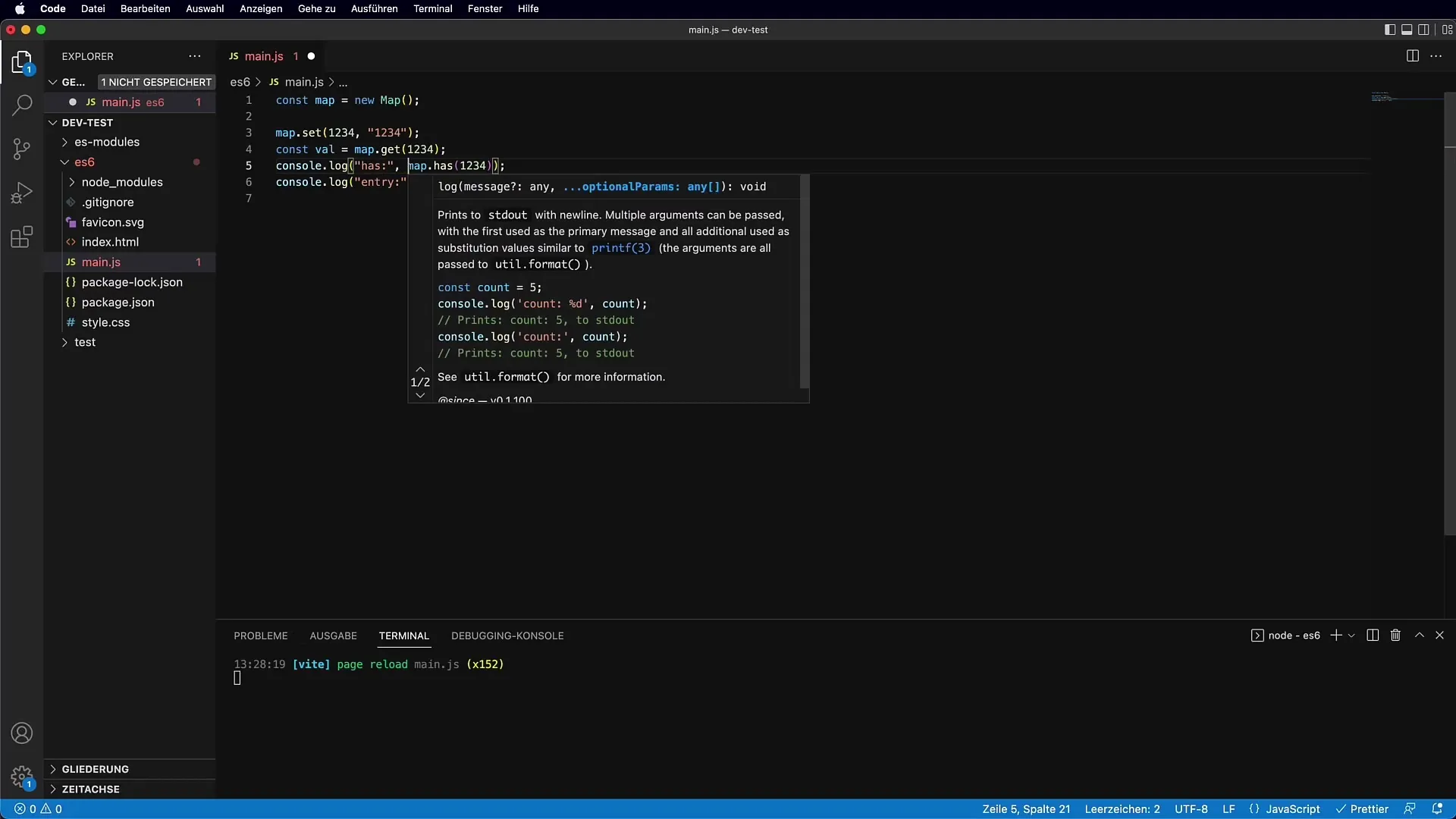
Step 5: Iterate over the keys and values
Maps have the capability to iterate over their keys and values. For iteration, you can use the keys(), values(), and entries() methods.
Step 6: Delete entries from the Map
Deleting an entry from the Map is done with the delete method. This method expects the key of the entry to be deleted.
Step 7: Delete all entries in the Map
If you want to delete all entries in the Map, you can use the clear method. This removes all entries at once.
Step 8: Query the size of the Map
With the size property, you can query the number of entries in the Map. This is useful to know how many key-value pairs you have stored.
Summary - JavaScript ES6–ES13: Using Maps as a Dictionary and Key-Value Store
By using Maps in JavaScript, you can implement a type-safe and flexible structure for your data. The methods and properties that Maps offer are not only intuitive but also make your code more maintainable and easier to understand.
Frequently Asked Questions
How do I create a Map in JavaScript?You can create a Map with new Map().
How do I insert entries into a Map?Use the set method to add keys and values.
How do I retrieve a value from a Map?Use the get method with the corresponding key.
What happens if the key does not exist?get returns undefined if the key does not exist.
How do I check if a key is present in the Map?You can do this with the has method.
How can I delete all entries from the Map?Use the clear method to remove all entries.