Strings are extremely important in programming as they are used for processing and displaying text. In this guide, you will learn how to effectively utilize various string methods in C# to search and modify texts. Here, we will specifically deal with the methods that allow you to retrieve information from strings and manipulate them.
Key Findings
- Strings are case-sensitive.
- Methods such as Contains, StartsWith, and EndsWith check the contents of strings.
- The use of IndexOf and LastIndexOf helps determine the position of characters in the string.
- With Substring, you can extract parts of a string.
- Insert and Remove allow editing of strings.
Step-by-Step Guide
Strings and Their Initialization
Before we delve into the methods in detail, you should ensure that you master the basics. Strings in C# are typically declared and initialized with double quotation marks.
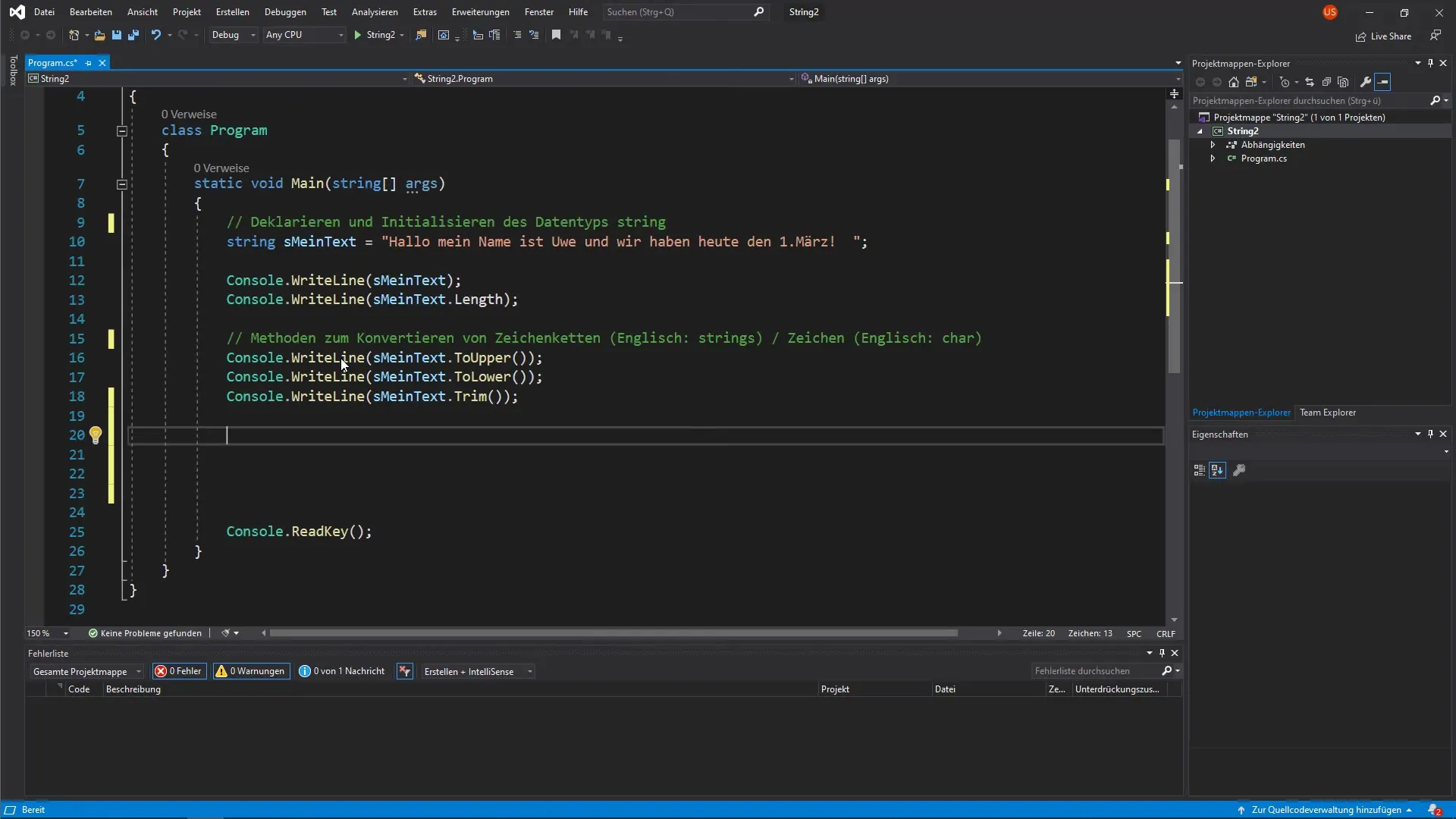
Checking the Contents of a String
To determine whether a certain text or word is contained within a string, you can use the Contains method.
It is important to note that this method is case-sensitive. If you search for "hello", the result will be false since the capitalization does not match.
Check the Beginning and End of a String
In addition to checking if a string contains a certain word, you can also check whether a string begins or ends with a specific character or string. For this, you have the methods StartsWith and EndsWith.
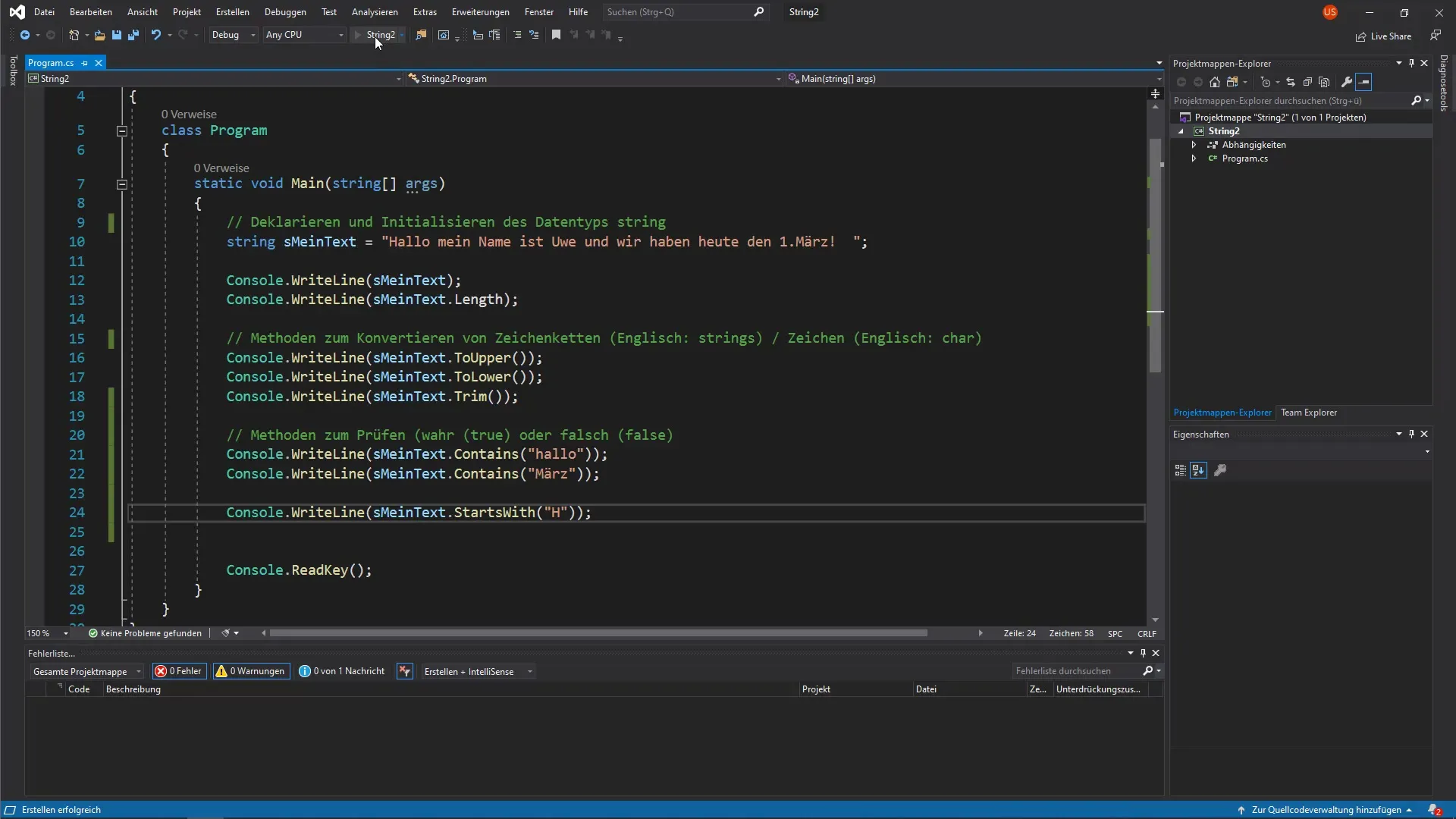
If you see that EndsWith returns false when you expected a period, make sure there are no extra spaces or characters at the end.
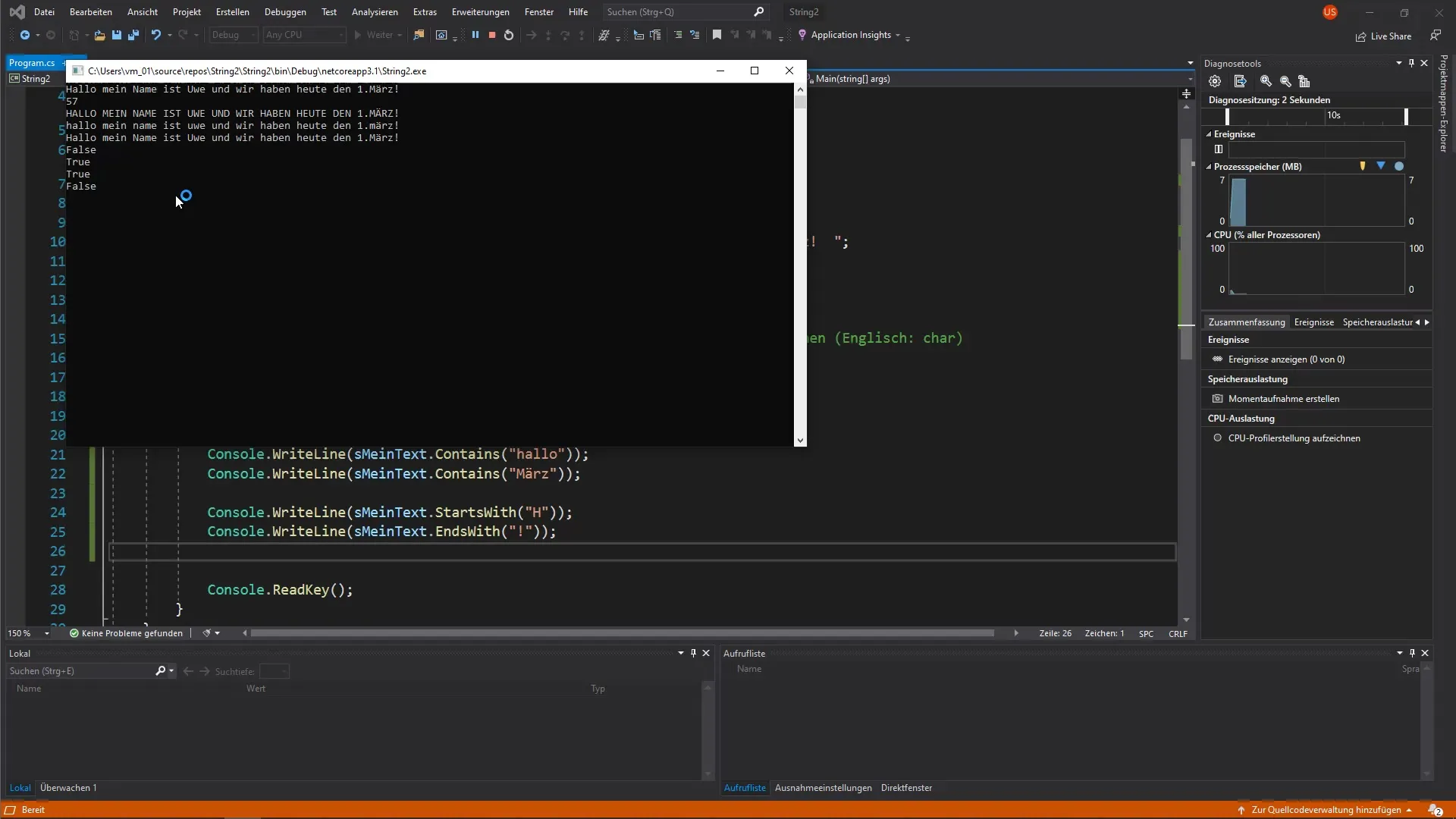
Finding the Position of a Character
If you want to know the position of a specific character or word in a string, IndexOf is the correct method.
Remember that counting positions starts at 0. If the letter is at position 20, IndexOf will return the value 20.
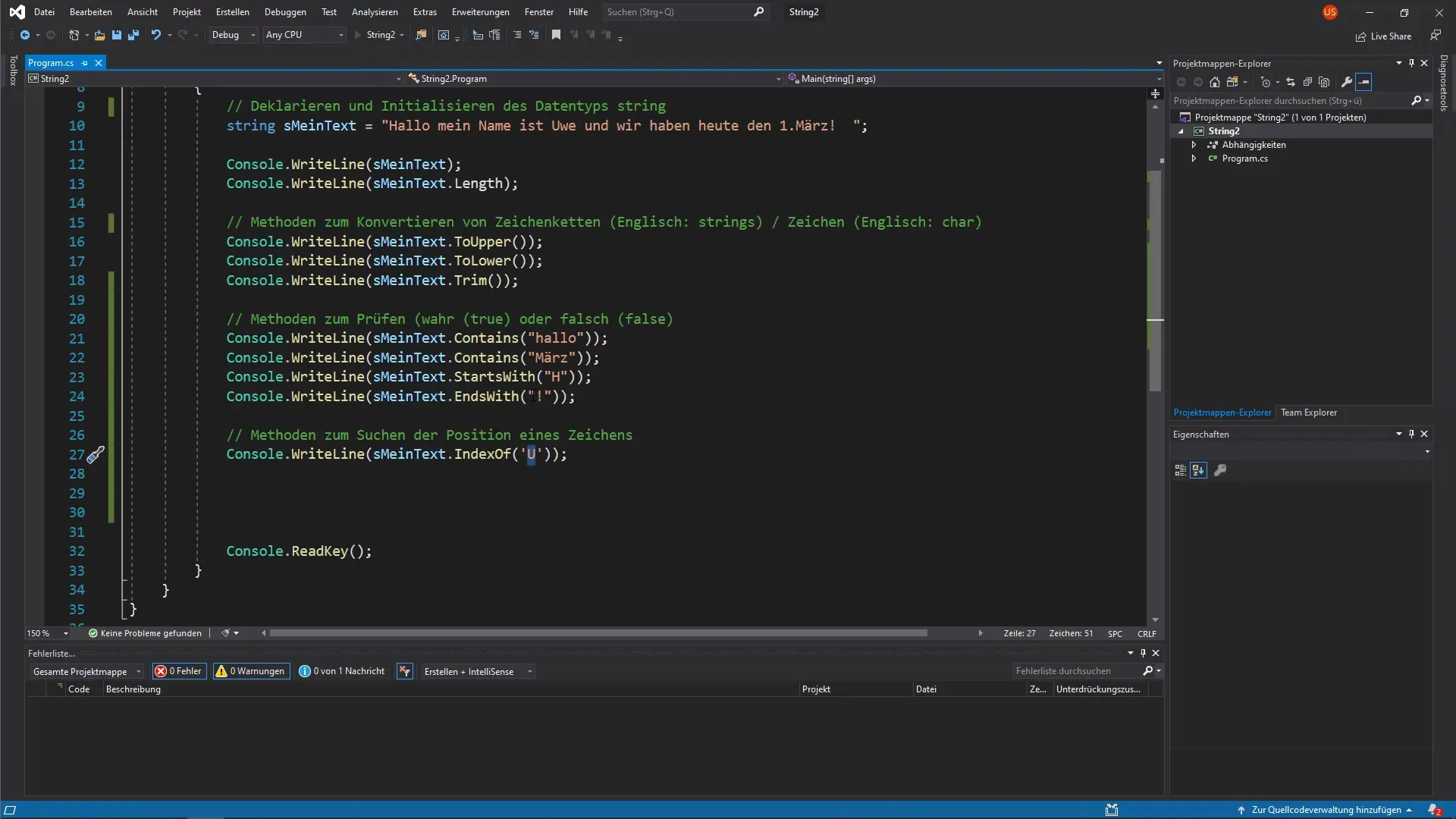
To find the last position of a character, you can use LastIndexOf. This gives you the last occurrence of a character in a string.
Determining Parts of a String
If you want to extract parts of a string, you use the Substring method. Here, you specify the starting position and optionally the length of the substring.
In this case, you start at index 20 and want to extract 3 characters (U, w, e).
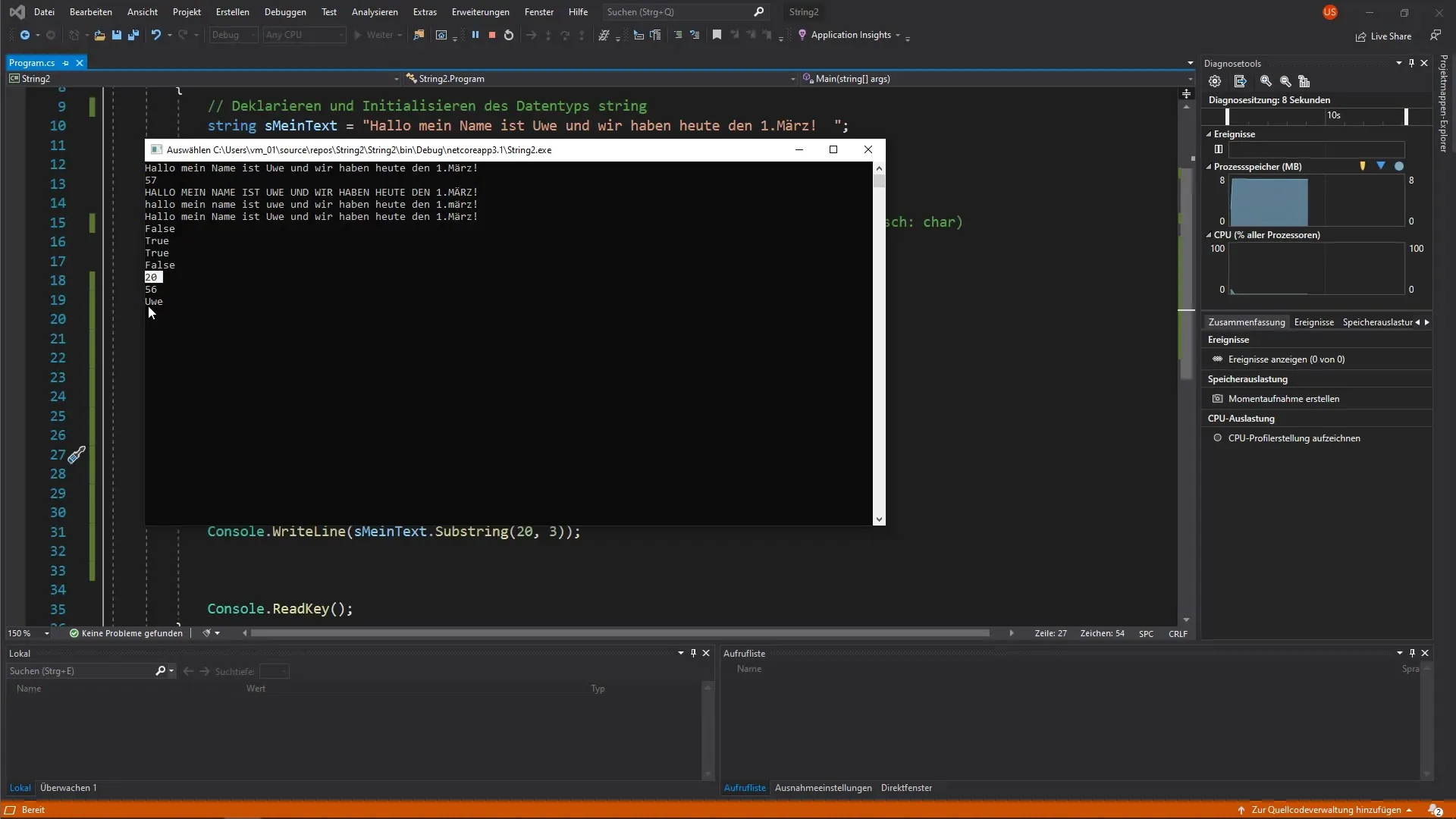
Modifying Strings: Adding and Removing Characters
Sometimes it is necessary to modify a string by adding or removing characters. With Insert, you can add new text at a specific position.
This would add the text "2" before "March".
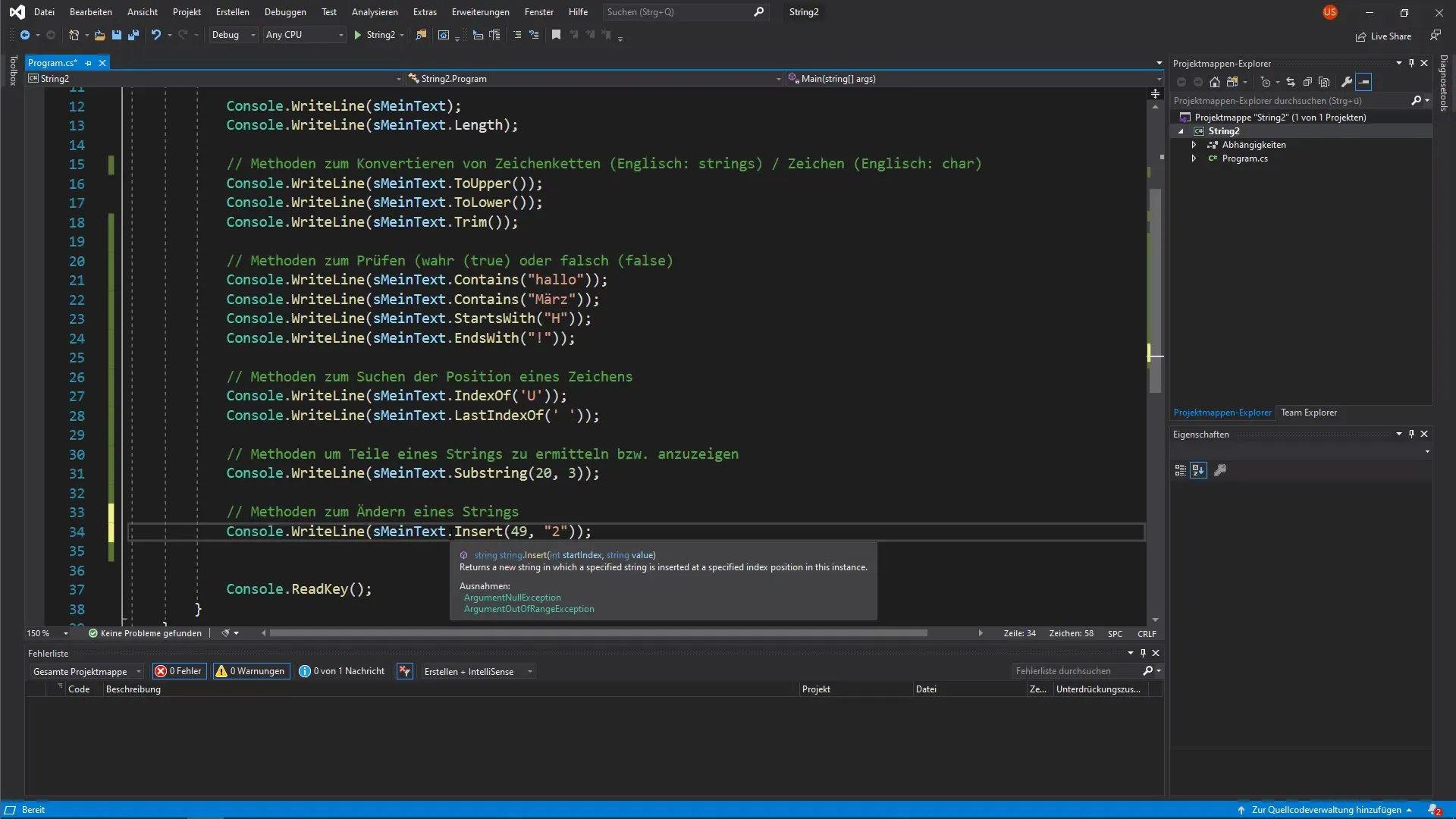
If you want to remove a character, you can use the Remove method.
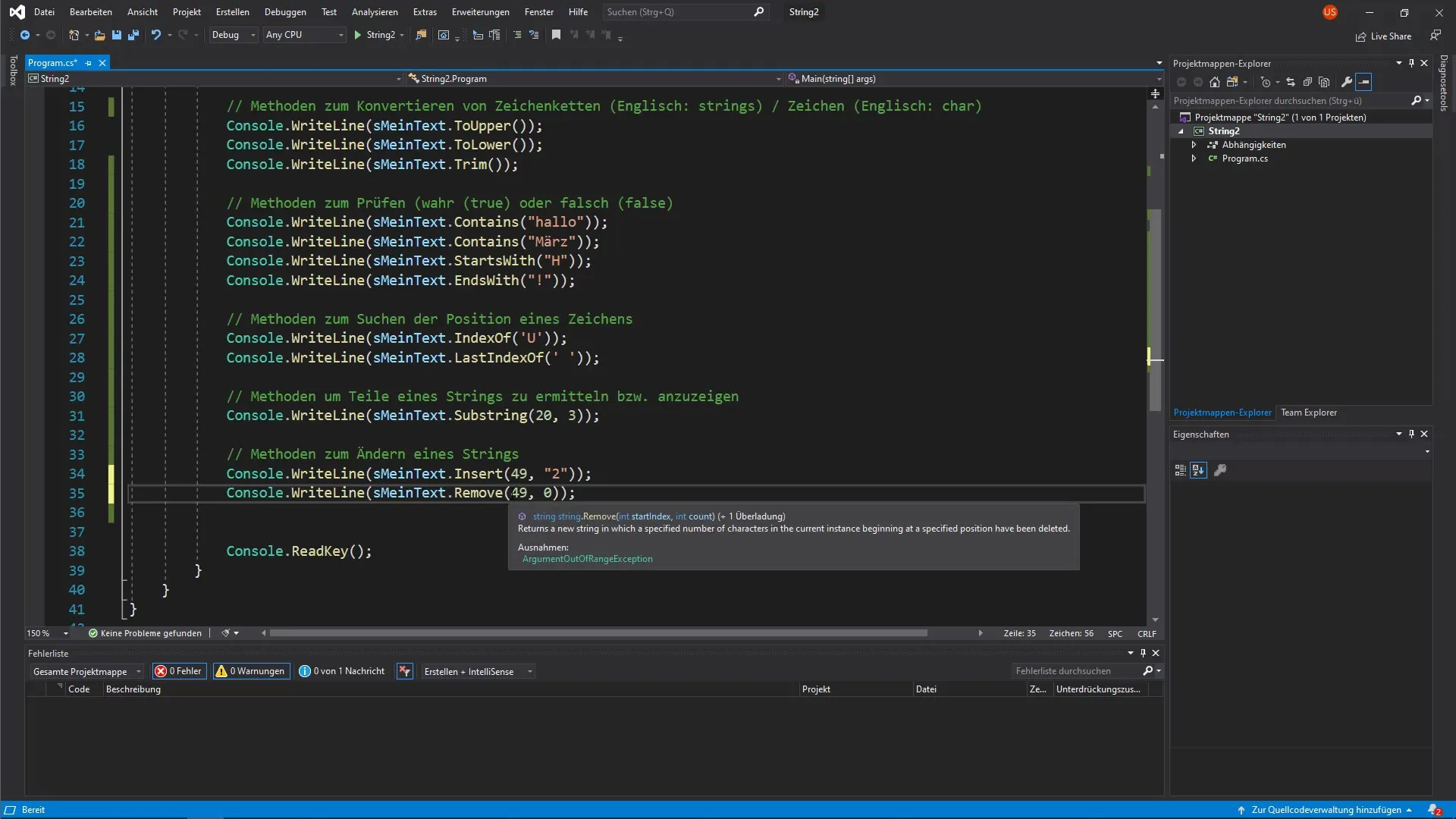
Exercise: Testing Your Own Strings
Now it’s your turn! Try to incorporate your own name into a string and output it to the console. Experiment with generating substrings and using the various methods. This will help you get a feel for the possibilities with strings.
Summary – C# Programming: String Methods Searching and Modifying in Detail
In this guide, you have gained extensive insights into the use of string methods in C#. You now know how to search for text elements, find their positions, and edit strings. Experiment with the methods to deepen your programming skills.
Frequently Asked Questions
What is case-sensitive in the context of strings?Case-sensitive means that capitalization is considered when checking strings.
How do I find the index of the first occurrence of a character?Use the IndexOf method to find the first occurrence of a character in the string.
What does the LastIndexOf method do?With LastIndexOf, you can determine the position of the last occurrence of a specific character in the string.
How can I extract a part of a string?Use the Substring method to extract a part of the string starting from a certain position.
Can I also modify a string?Yes, you can use methods like Insert and Remove to add or remove characters.