The work with databases is a central aspect of software development. This tutorial is about modifying existing records in an SQLite database. You will learn how to give a user the ability to update data without compromising the integrity of the data.
Key insights
- Users can modify existing entries in the SQLite database.
- It is important to catch errors and design a reactive user interface.
- With the right SQL commands, you can update records specifically.
Step-by-step guide
Enable user interaction
First of all, you should ensure that the user has the ability to adjust the car brand they have added. To achieve this, you need to set up a mechanism that allows the user to change the current selection. This is done through a list box from which the user can select the car brand.
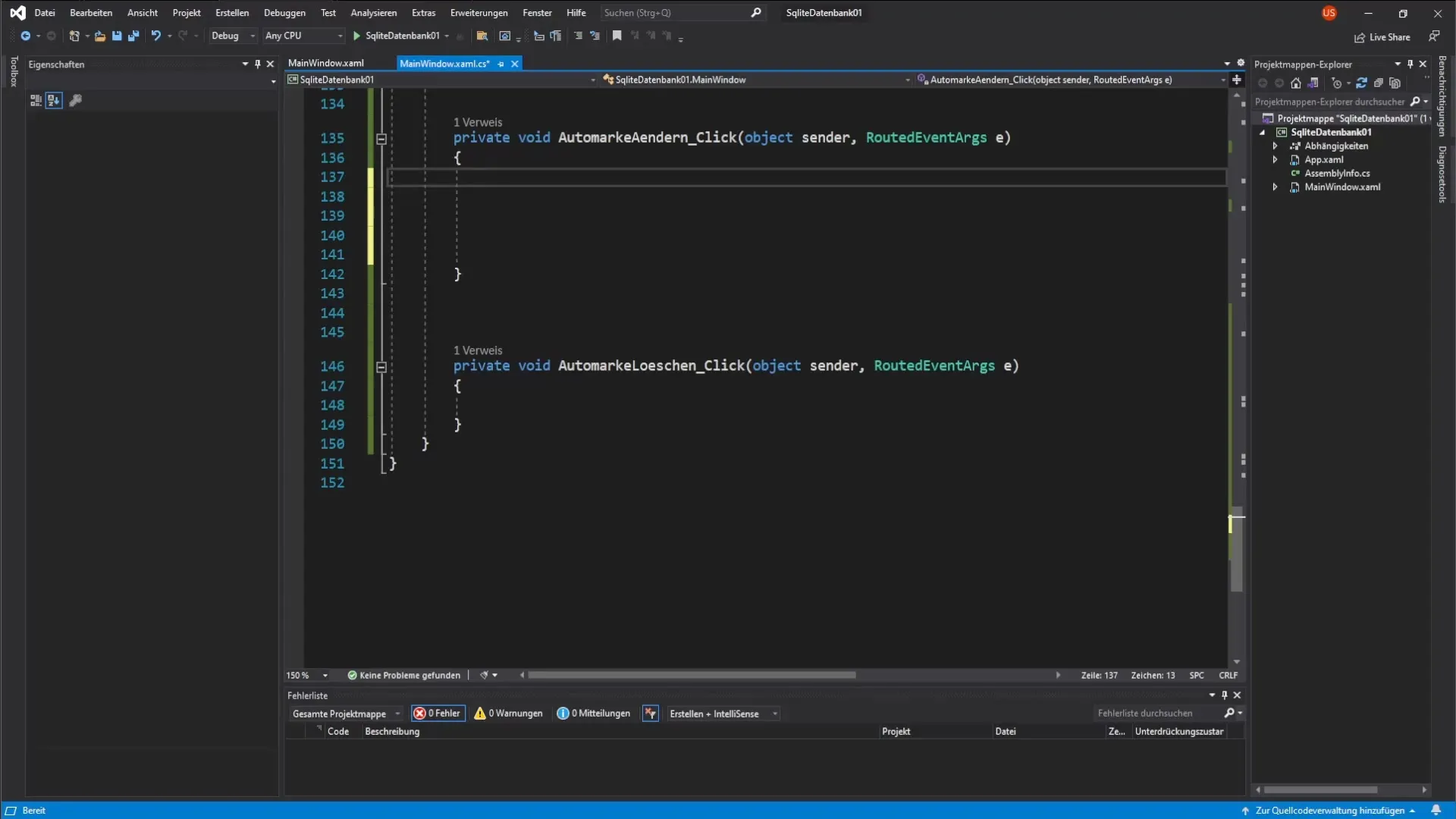
Implement error handling
To ensure a smooth experience for the user, you must catch errors. This means that you should check if an item in the list box has been selected before proceeding with the modification. If no car brand is selected, the method should be aborted early.
Enter changes into the database
Next, it’s time to enter the changes into the database. For this, you will use a try-catch block again to catch potential errors while accessing the database. It is advisable to also add a finally block to ensure that the database connection is always closed, regardless of whether an error occurred or not.
SQL command to update
After you have set up the error handling block, the next step is to formulate the corresponding SQL command. You will use the UPDATE command, followed by specifying the table, here "Automarken". It will then be necessary to use the SET keyword to indicate which column should be updated.
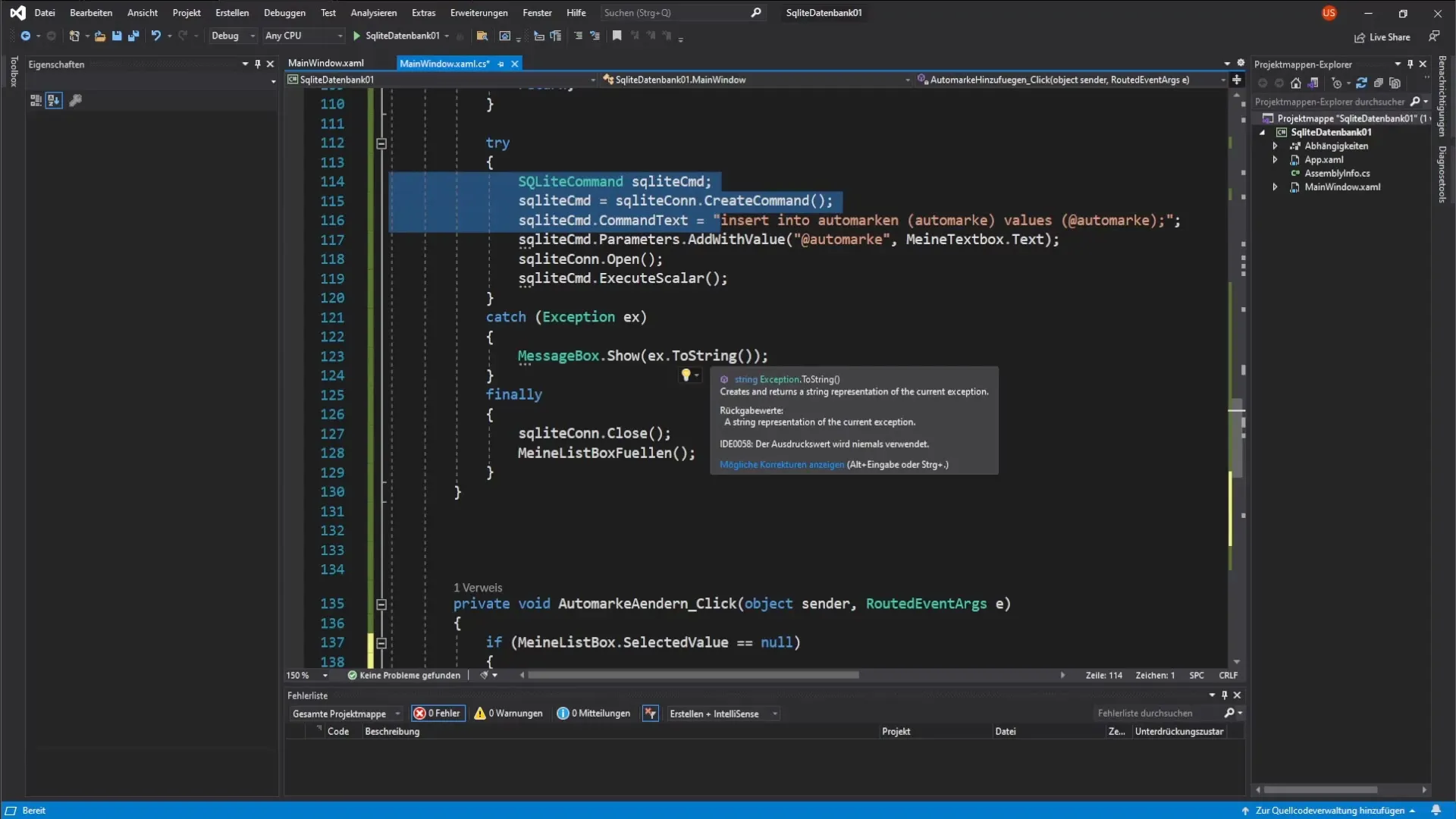
Add parameters for the update command
For the UPDATE command, you need two parameters: the new car brand you want to set and the ID of the record you want to change. The ID is crucial to uniquely identify the specific record. This ensures that the correct car brand is modified.
Filling in the parameters
Now is the time to set the parameters for the UPDATE command. You take the ID from the selected list box and the car brand from the input box (Textbox). These two pieces of information are needed to resolve the corresponding conflict in the database and allow you to make the desired changes.
Open database connection and execute command
As in the previous examples, you need to open the database connection before executing the change. Make sure that all parameters are passed correctly. This is a critical step to ensure the integrity of the data.
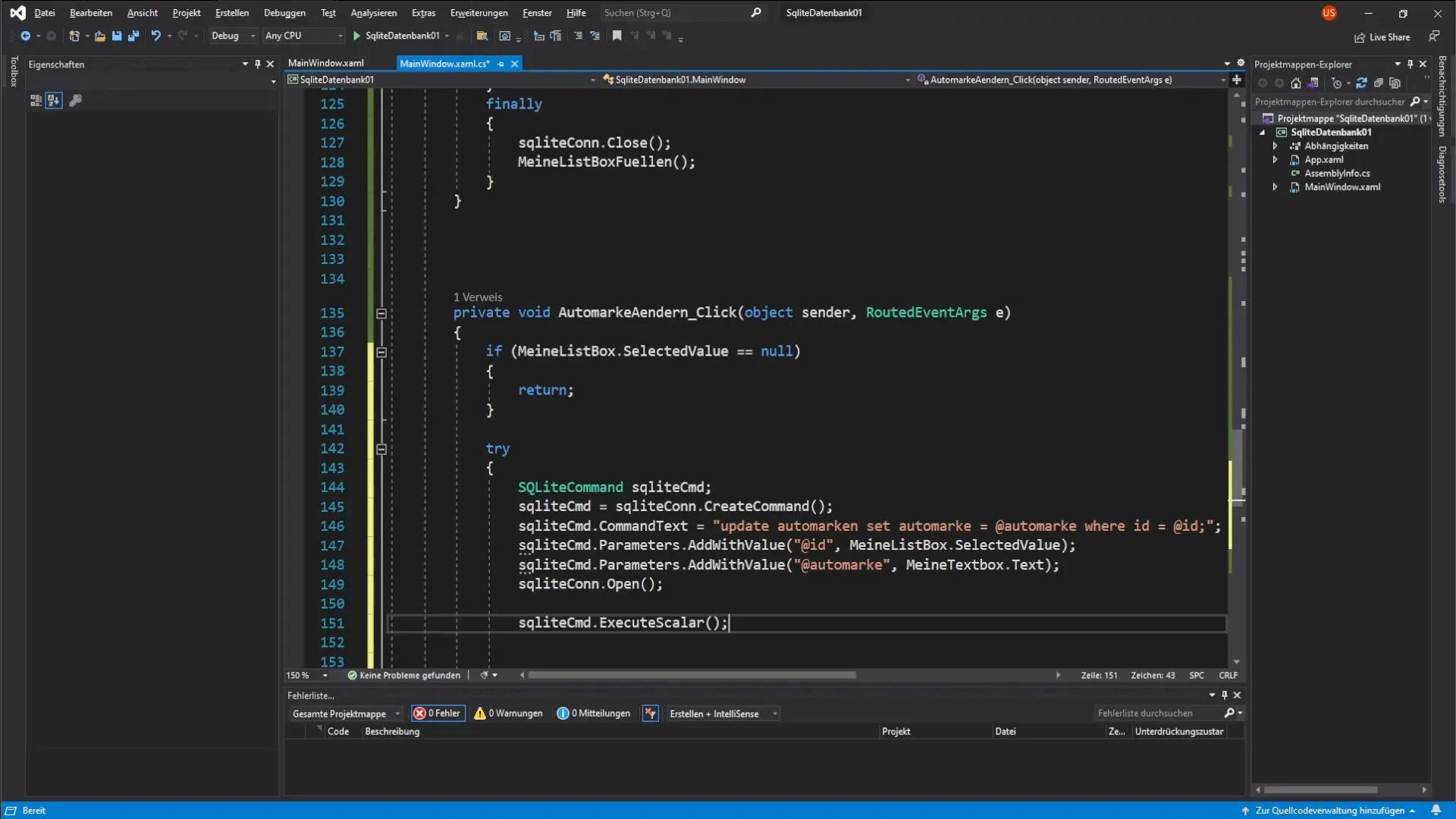
Update list box
After the change has been made, it is important to update the list box so that the user can see the new car brand in the user interface. This greatly improves user friendliness and ensures that the user can see the current state of the data at any time.
Conduct application tests
Finally, you need to test the entire system to ensure that everything works as expected. Start the application, select an entry in the list box, change the car brand, and check if the list box is correctly updated after the change. These tests are crucial to ensure that everything functions without errors.
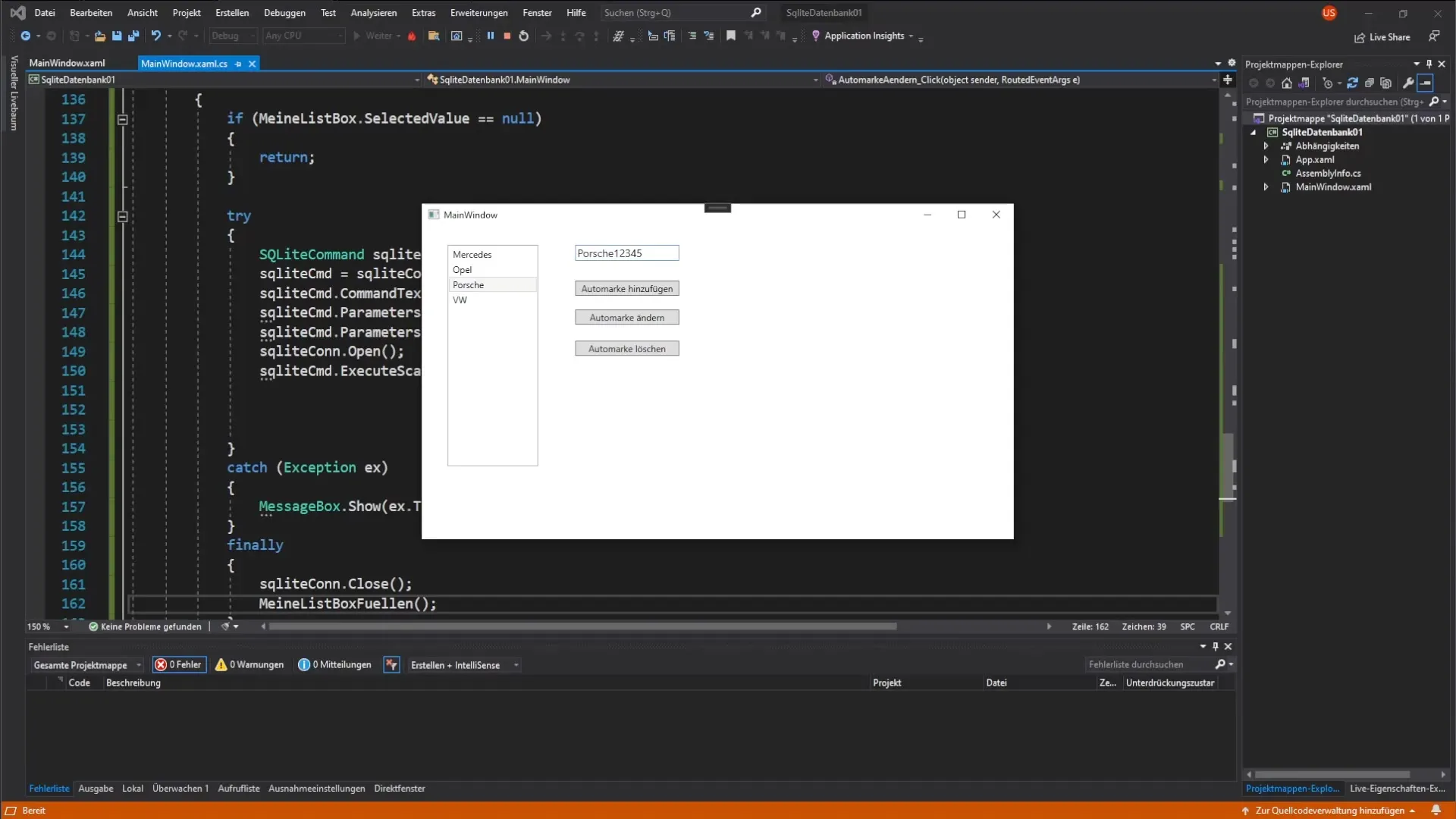
Summary – Changing data in an SQLite database
In this guide, you have learned how to change existing records in an SQLite database. The implementation of error handling, the construction of SQL commands, and user interaction are central aspects that you have considered. These skills are essential for programming robust applications.
Frequently Asked Questions
How can I catch errors when modifying a record?You should use a try-catch block to log and handle errors when accessing the database.
Which SQL commands are necessary to change a record?You use the UPDATE command, followed by SET, to update the necessary columns.
How can I update the user interface after a change?By reloading the available entries in the list box after the changes have been made in the database.