ArrayLists are a powerful tool for managing data in C#, especially when you work with variable amounts of information. Unlike traditional arrays, ArrayLists are dynamic and offer numerous advantages, including the ability to store elements of different types. In this guide, we illuminate the usage of ArrayLists through clear examples and practical explanations.
Key insights
- ArrayLists dynamically adjust to the number of elements.
- They can store heterogeneous data types like strings, integers, and doubles.
- Adding, deleting, and outputting elements is intuitive and flexible.
Step-by-Step Guide
1. Declaring an ArrayList
To create an ArrayList in C#, we begin with its declaration. This is done by specifying the type of list and importing the necessary namespace.
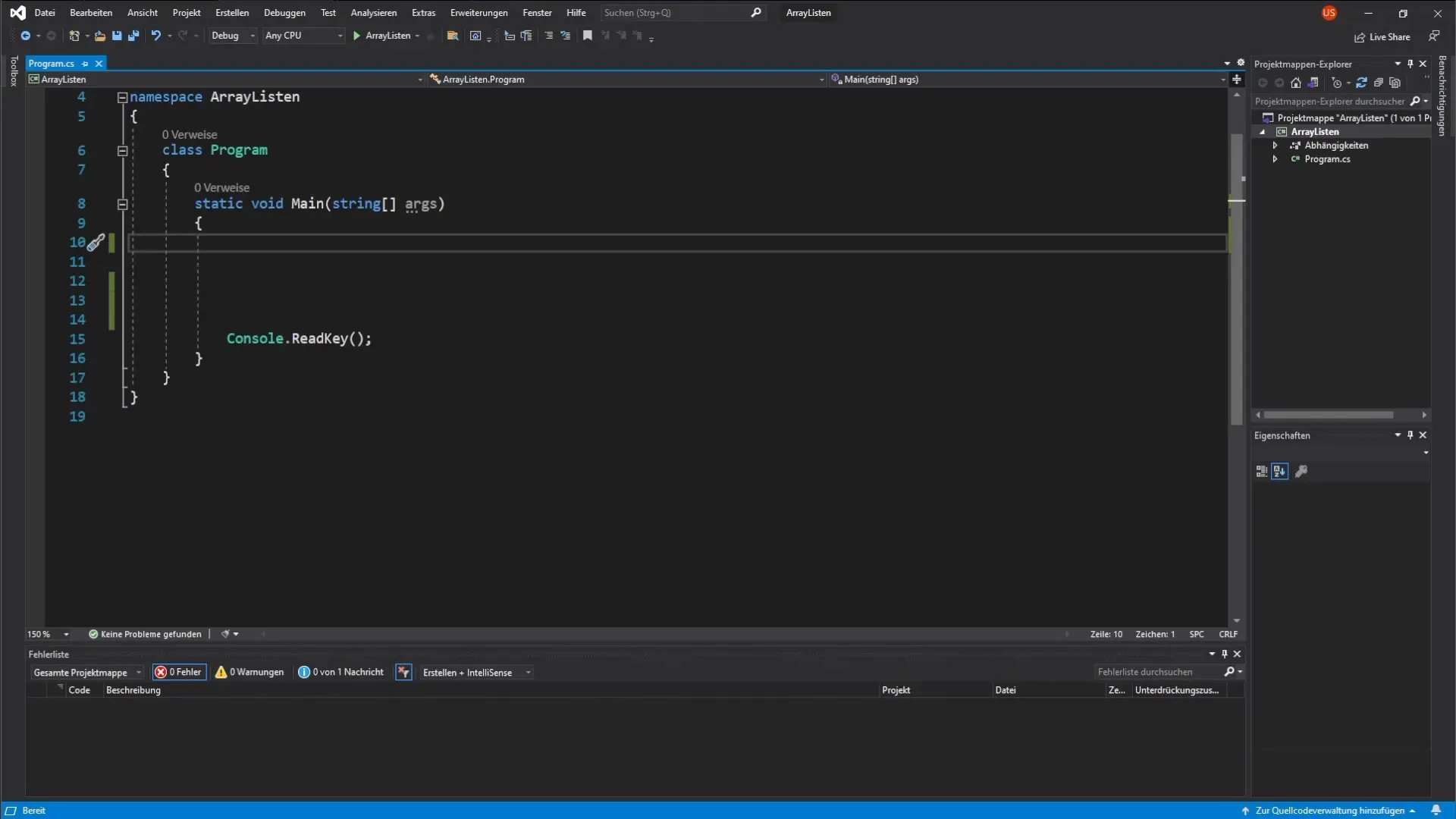
Alternatively, you can create the list directly to fill it later with various data types:
2. Adding Elements
The next step is to add elements to your ArrayList. This is done using the Add() method. For example, we first add a string:
You can also store different data types in one list. Here we add an integer to our existing list:
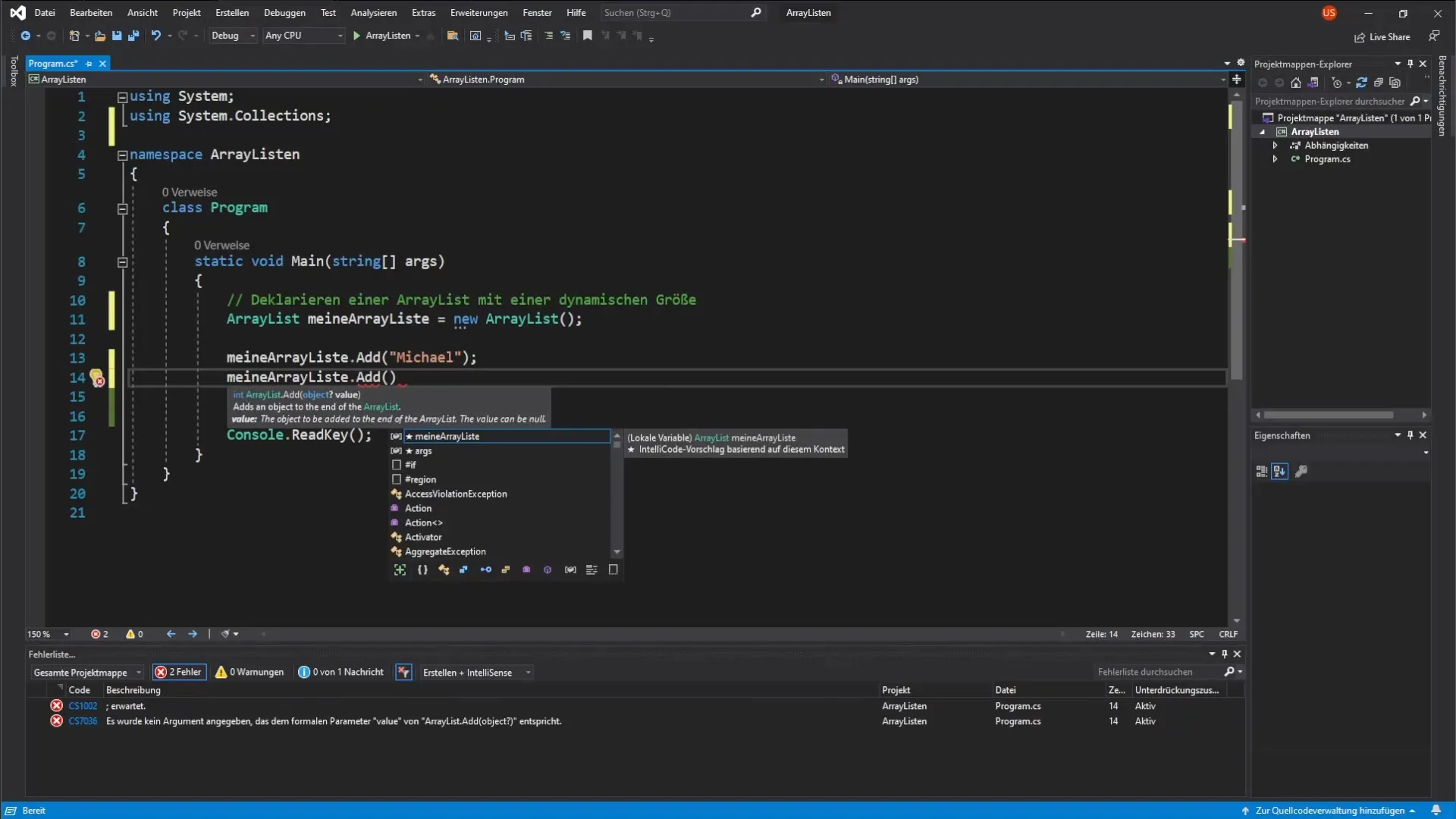
3. Counting Elements
Collecting data in your ArrayLists is useless if you don’t know how many elements you have. You can use the Count method to quickly and easily read the number of elements.
4. Outputting Elements
There are several ways to output the elements in the ArrayList to the console. A common method is to use a for loop:
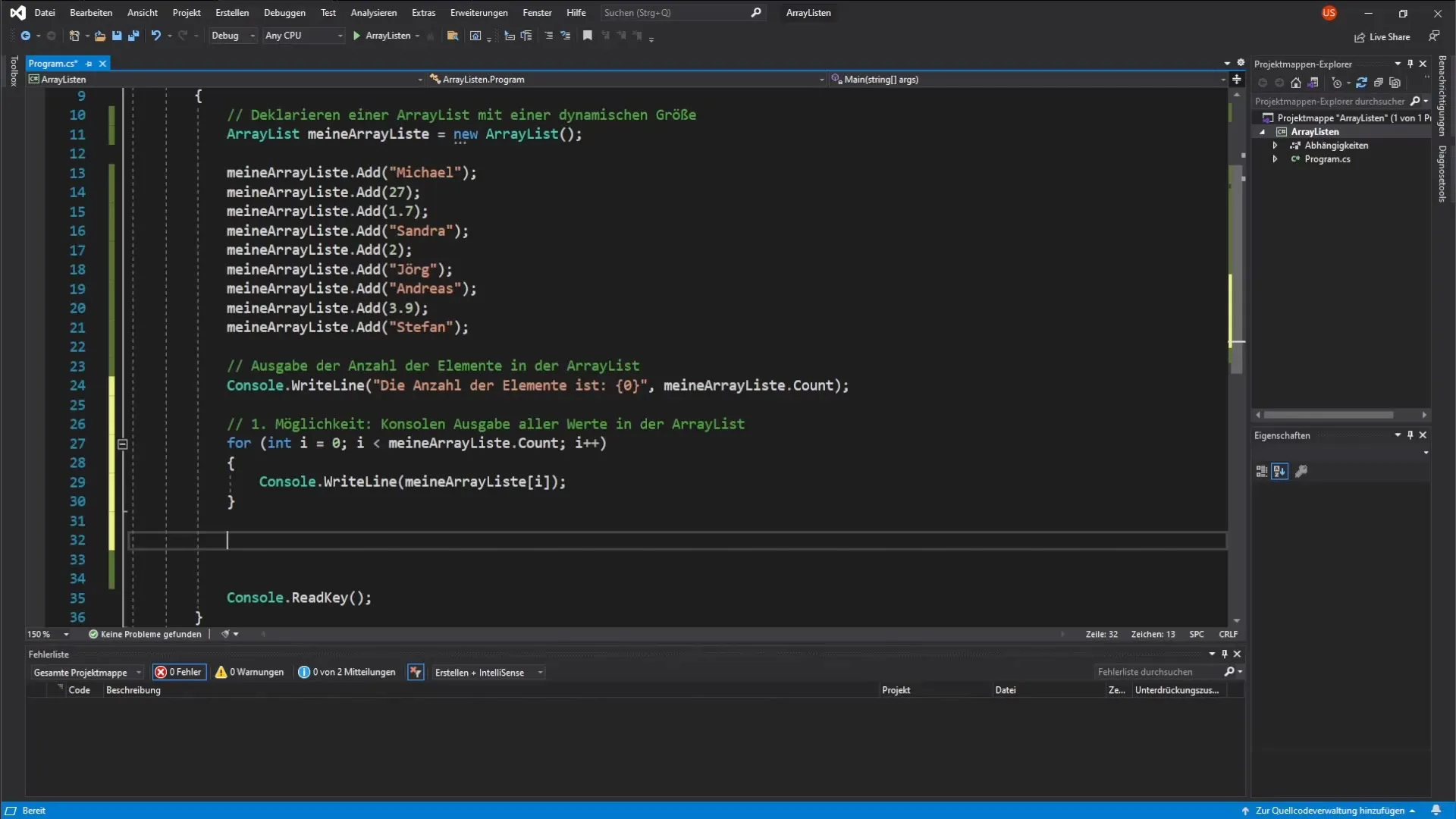
A more elegant option is to use a foreach loop, which makes it easier for you to iterate through the list:
A more modern syntax is to apply object-initialization type inference to automatically recognize types:
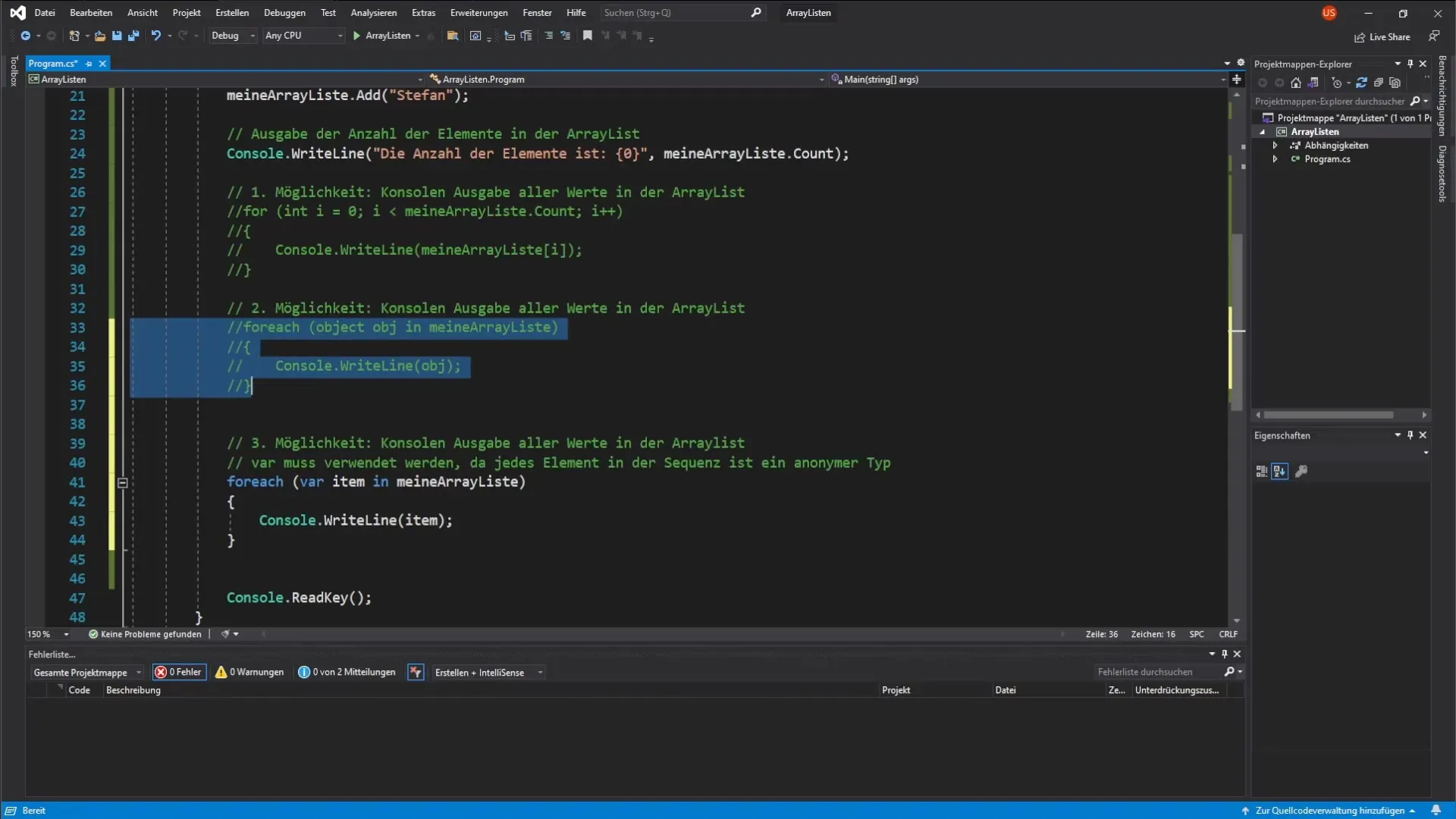
5. Filtering Specific Data Types
If you only want to filter specific data types from your ArrayList, you can do this using a loop and a condition check. Here we only output the strings by checking if each element is of type string:
6. Summing Integer Values
A common scenario is summing values in an ArrayList. Here we use the foreach loop to collect and sum all integer values:
7. Deleting Elements
If you want to remove a specific element, you use the Remove() method. Here you directly name the element to be deleted:
Alternatively, you can delete an element based on its index. Here’s an example:
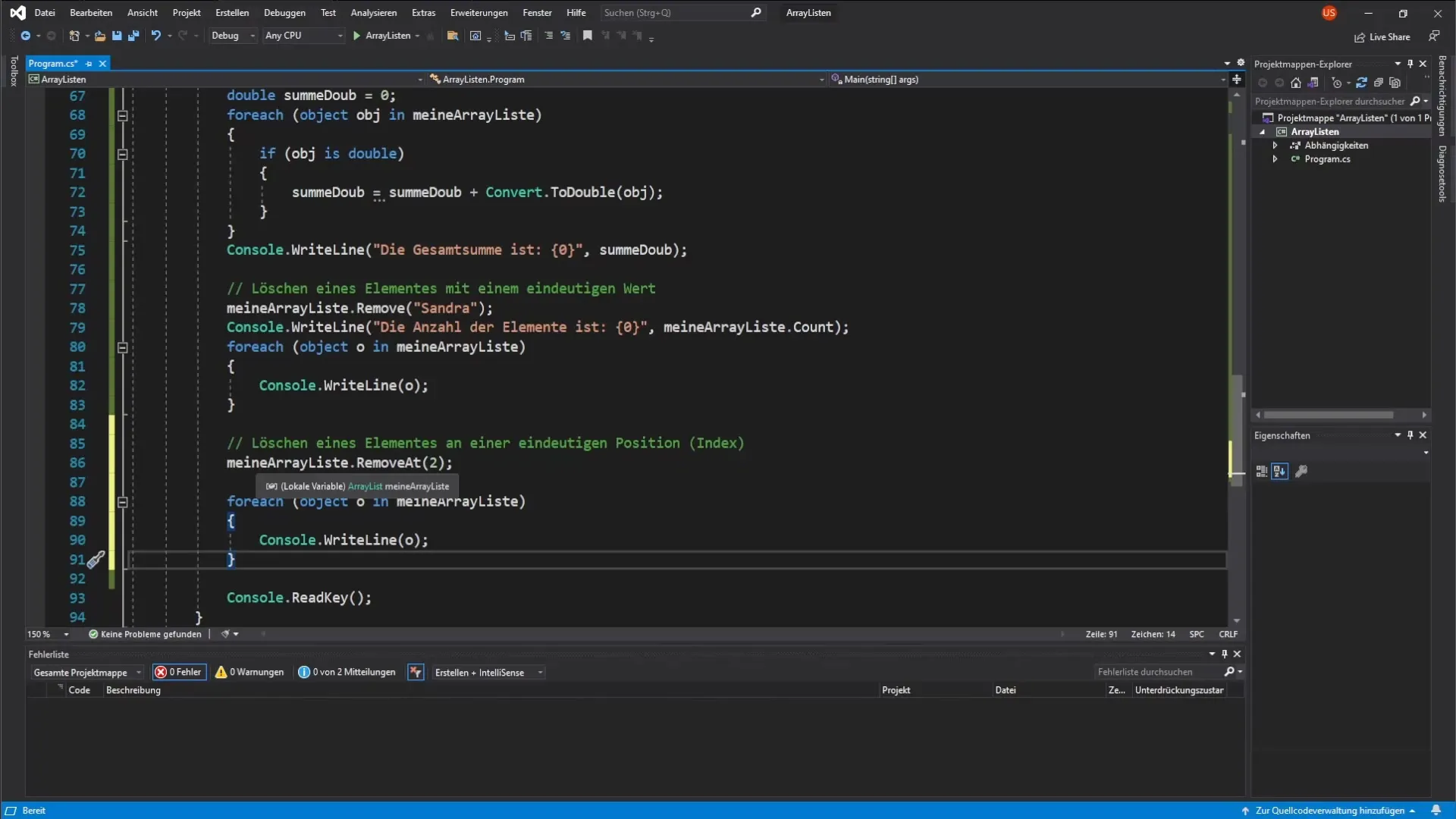
8. Inserting Elements at Specific Positions
If you have deleted an element and want to insert a new value at that position, you can do so using Insert(). Here we add the element "Sandra" at a specific index position:
9. Conclusion and Further Remarks
In this guide, we covered basic operations with an ArrayList, including declaring, adding, counting, outputting, and deleting elements. ArrayLists offer high flexibility and efficiency in managing data sets that can be both homogeneous and heterogeneous.
Summary - Eloquent Work with ArrayLists in C
In this step-by-step guide, you have learned the fundamentals of working with ArrayLists – from initialization to managing data. These concepts are the foundation for many programming applications and help you to work effectively with dynamic datasets.
Frequently Asked Questions
How can I declare an ArrayList in C#?Use using System.Collections; and then create the ArrayList with ArrayList meineArrayList = new ArrayList();.
Can an ArrayList contain heterogeneous data types?Yes, ArrayLists can store different data types, such as strings, integers, and doubles.
How do I count the elements in an ArrayList?Use the Count property: int elementCount = meineArrayList.Count;.
How can I output elements in an ArrayList?Use a loop, e.g., foreach or for, to iterate through the ArrayList.
Which method do you use to remove an element from an ArrayList?Use the Remove() or RemoveAt(index) method to delete an element.