Programming is often a complex matter, where one can quickly lose track. Especially in large projects that consist of hundreds of lines of source code, it can be challenging to understand the code and to follow the programmer's intentions. This is where comments come into play. They are not only helpful for yourself but also for colleagues who may work on your code or need to maintain it in the future. In this guide, you will learn how to effectively insert comments into your C# source code and what forms of comments exist.
Key insights
- Single-line comments begin with two slashes //.
- Multi-line comments are created with /* and */.
- Comments contribute to better readability and traceability of the code.
Simple single-line comments
To insert a simple comment, you place two slashes // before the text you want to note as a comment. This is especially useful for quickly explaining what a line of source code does.
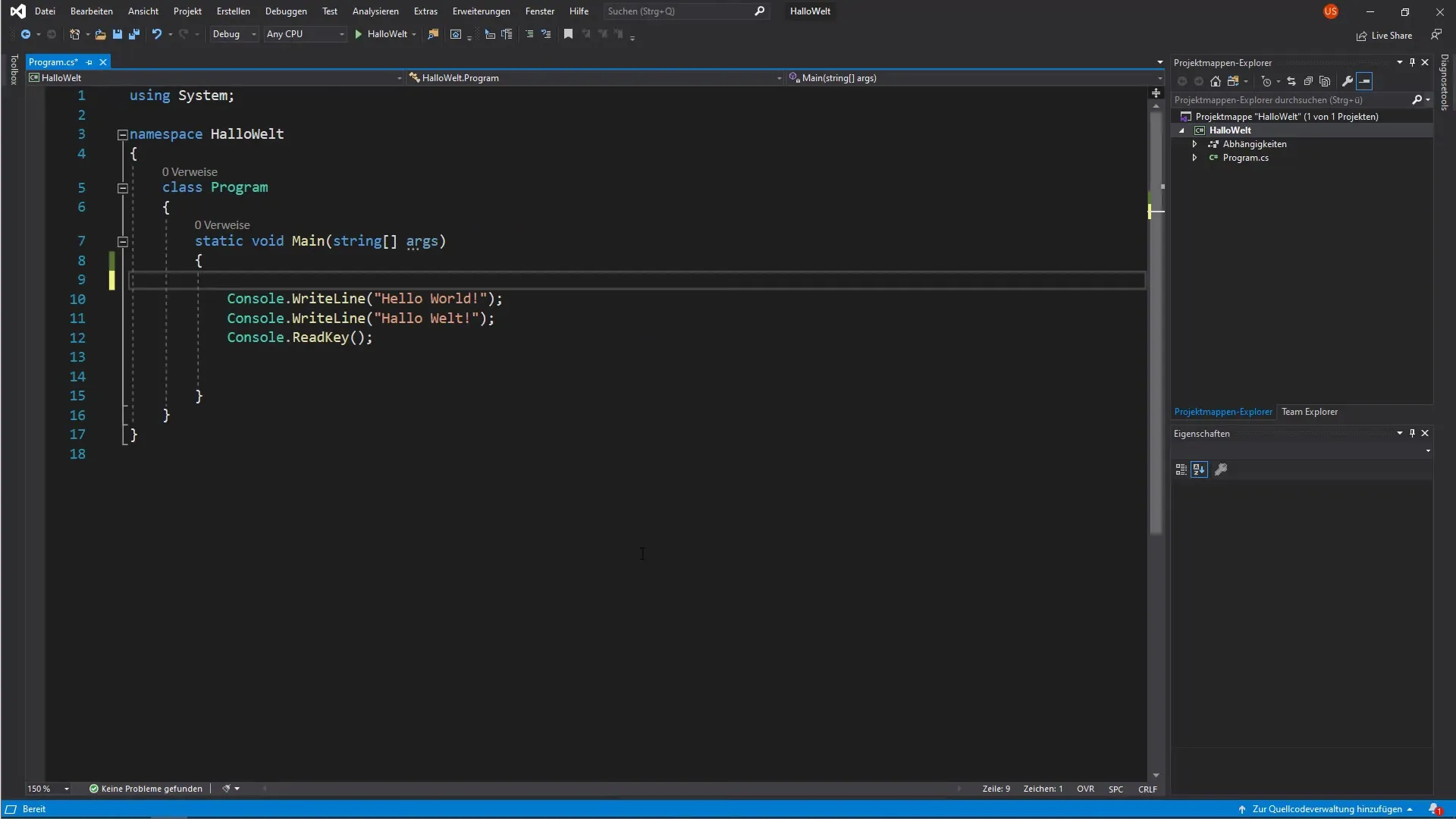
Here it helps the reader to immediately understand that the following line is responsible for outputting the text "Hello World".
Using multi-line comments
Sometimes you want to comment on more than just one line. In such cases, multi-line comments come into play. You start this comment with /* and end it with */. This allows you to have multiple lines in the comment.
This way, you can provide more detailed explanations or notes without interrupting the flow of your code.
The use of summary tags
In addition to the comments mentioned above, there are also special tags you can use to provide additional information, especially for documentation purposes. summary is also a member type that you can use for documenting your methods.
The summary tag gives other developers a clear idea of what the method does without having to read the source code itself.
Conclusion on comments in source code
Inserting comments into your source code is an effective way to clarify your intentions and increase the maintainability of your software. Good commenting not only promotes collaboration within the team but also ensures that you will spend less time in the future deciphering your own code.
—
Summary – Comments in C#: How to leave clear traces in the source code
Inserting single-line and multi-line comments into your source code is a simple yet effective way to improve the understandability of your code. Remember not to overdo comments – they should be helpful but not overwhelming. Use summary tags for methods when you want to document what they do.
Frequently Asked Questions
What are single-line comments in C#?Single-line comments begin with two slashes // and comment on a single line of code.
How do I create multi-line comments in C#?Multi-line comments start with /* and end with */, allowing you to comment on multiple lines at once.
When should I use comments in my code?Comments should be used to provide explanations for important parts of code, represent their function or intention, and leave notes that are important for maintenance.
What is the purpose of summary tags?Summary tags are used to provide information about classes or methods that can subsequently be used in documentation.