In programming, it is important to handle user inputs. Often it can happen that the user enters something that does not meet expectations – for example, letters instead of numbers. This can lead to program crashes. In this guide, we will deal with the use of if conditions and the TryParse method in C# to effectively manage such situations. You will learn how to avoid errors when converting strings to integers and make your program robust.
Main insights
- The TryParse method allows for a conversion to be performed while simultaneously checking if it was successful.
- With if conditions, you can decide what should happen in the event of an invalid input.
- By using default values, you can ensure that your program continues to run even with invalid inputs.
Step-by-step guide
Capturing user input
To begin, capture the room temperature as a number.
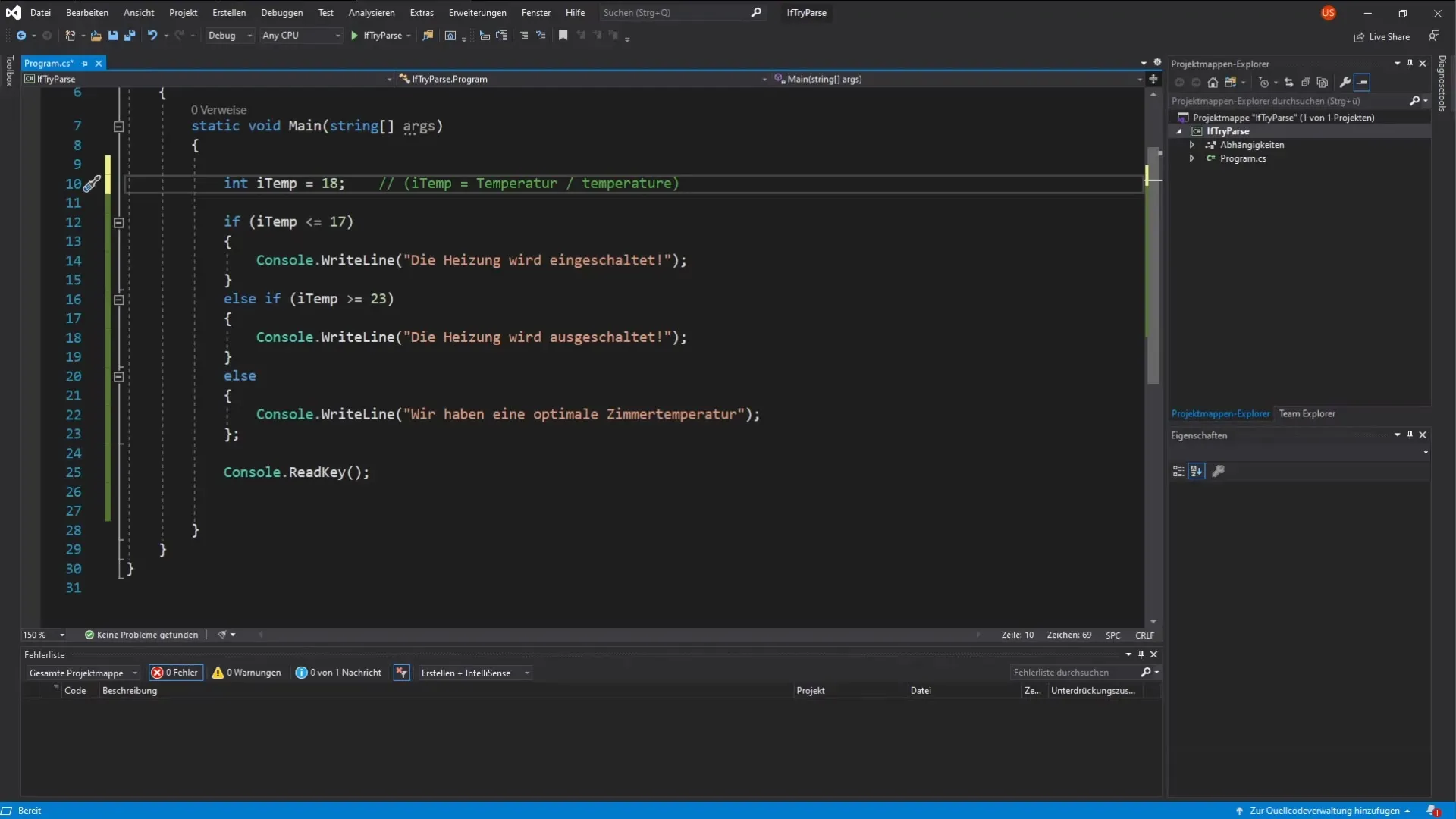
Here, the user is prompted to make an input, which is saved in the variable tempEingabe as a string. It is important to note that all inputs are returned by the console as strings. Therefore, we need to convert the input later.
Converting the input with TryParse
Now, to convert the user's input into an integer, we use the TryParse method. This allows us to ensure that the conversion only occurs if the input is actually a number.
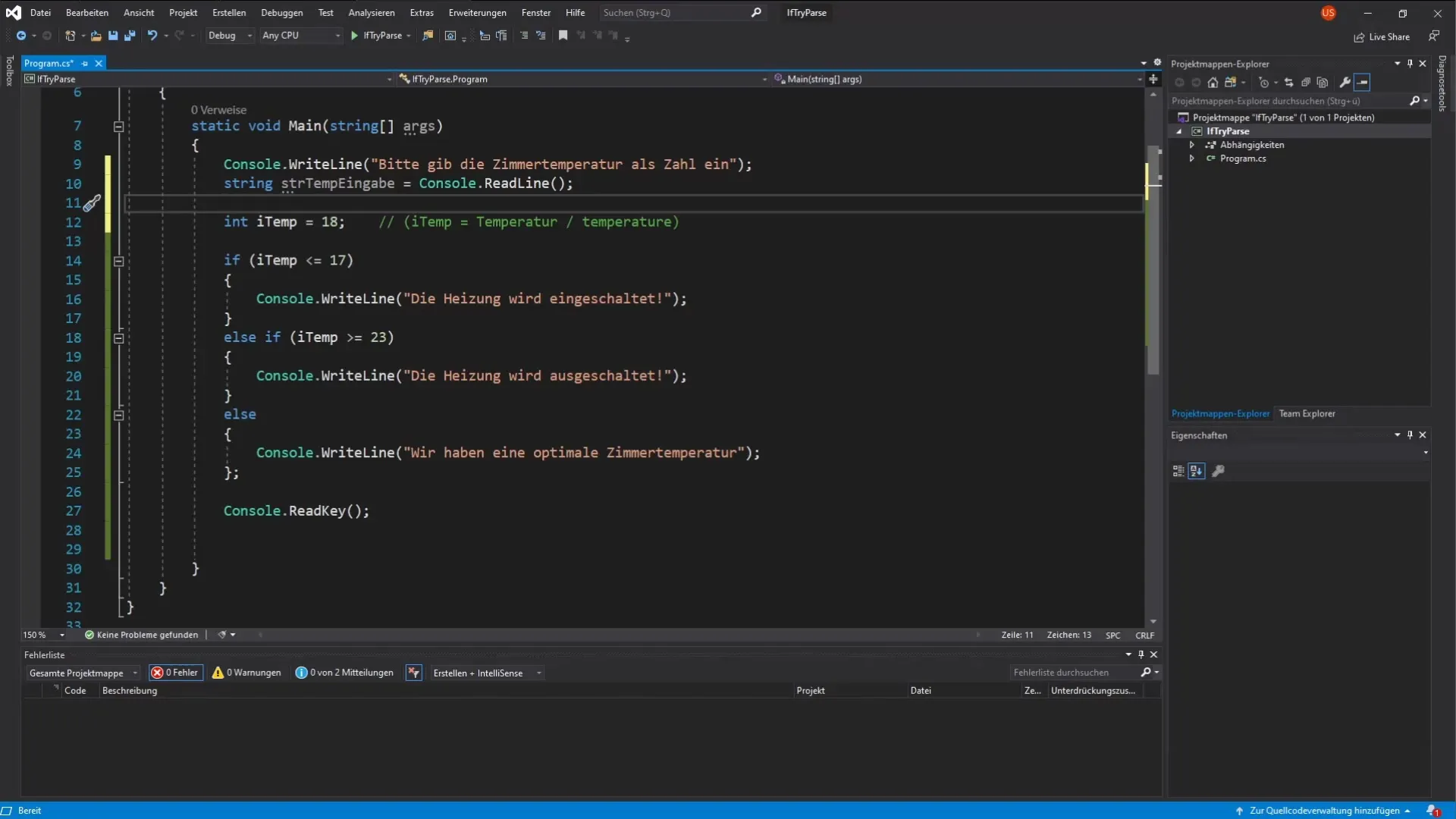
The TryParse method returns a boolean value. This indicates whether the conversion was successful. out temp allows us to store the converted number in the variable temp if the input was valid.
Applying the if condition
Now you come to the decision of what to do if the conversion was not successful.
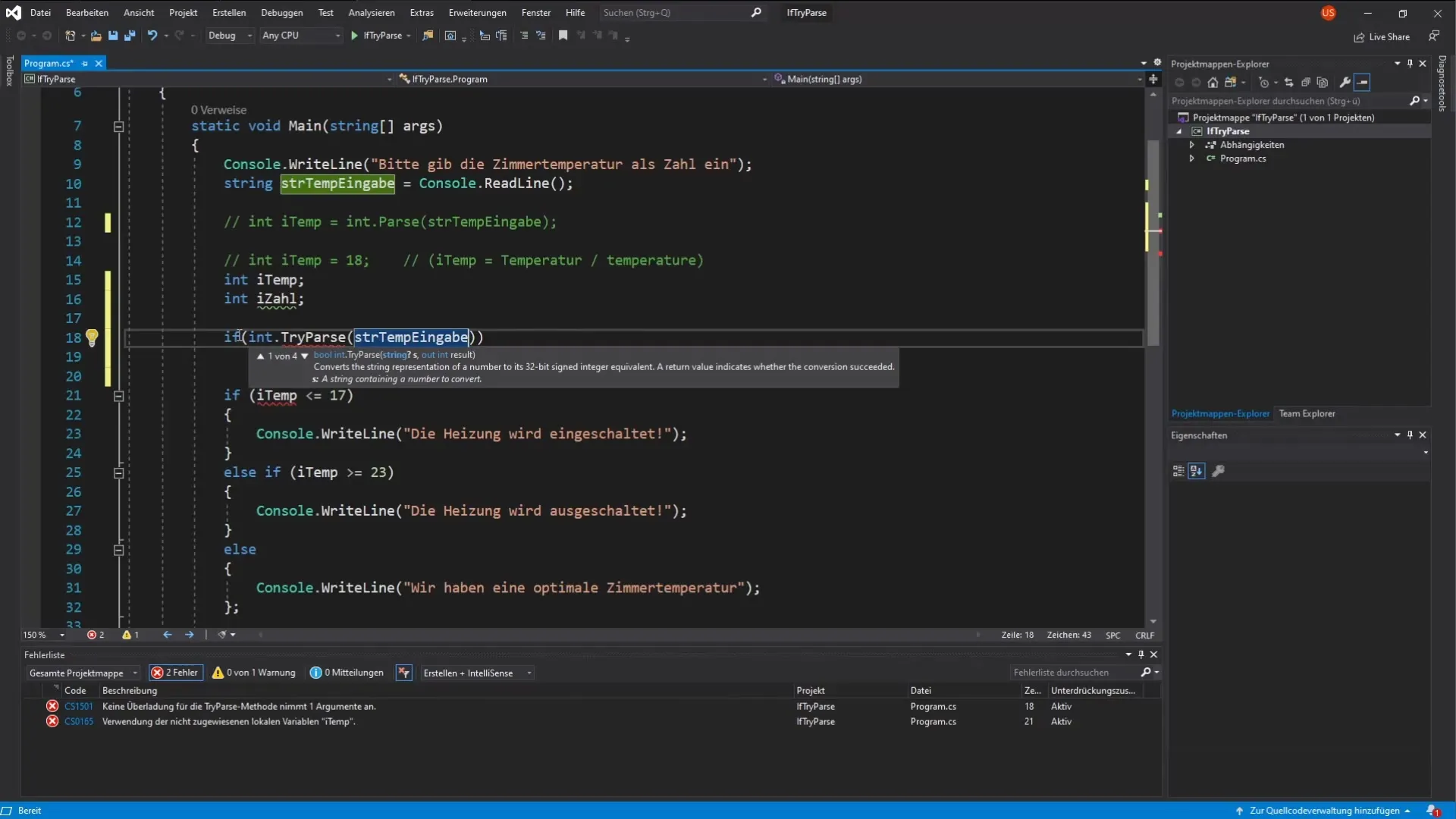
In the if block, the code executes if the input is valid. In the else block, you can set a default value or output an error message.
Setting a default value
In the case that the user's input is invalid, you probably want to use a default value.
In this example, the default value of the variable temp is set to 0 if the input is erroneous. This prevents your program from crashing due to an invalid input.
Executing and verifying the total code
Put all the parts together and execute your entire code.
In this complete implementation, you should now ensure that your application is robust and functions smoothly even with invalid user inputs.
Summary – Understanding If Conditions and TryParse in C# Programming
In this guide, you learned how to handle user inputs in C# and the TryParse method. You have learned step by step how to validate, convert inputs and handle errors so that your program remains stable. The combination of if conditions and TryParse allows you to implement effective error handling.
Frequently Asked Questions
What is the function of TryParse?TryParse attempts to convert a string into an integer and returns whether this was successful or not.
How do I handle invalid user inputs?With an if statement, you can check if the input is valid and take appropriate actions, such as setting a default value.
Where do I set default values in my code?Default values should be set in the else block of the if statement when the input is invalid.
How can I ensure that my program does not crash?Use TryParse and manage incorrect inputs with if conditions to ensure that your program continues running.
What happens if the user inputs letters?If letters are input instead of numbers, the TryParse method will return false, and you can set a default value in the else block.