The Do-While-loop in C# gives you the opportunity to execute a code block at least once before the condition is checked. This makes it particularly suitable for situations where at least one execution of the code is necessary before deciding whether it should be executed again or not. In this guide, I will show you how the Do-While loop works, how to apply it effectively, and when its use is meaningful.
Key takeaways
- The Do-While loop executes the code at least once.
- The condition is at the end of the loop.
- A break command can be used to terminate the loop prematurely.
- Examples of applications include user inputs in console applications.
Step-by-step guide
1. Basic understanding of the Do-While loop
First, it is important to understand how the Do-While loop is structured. The loop begins with the keyword do, followed by a code block that will be executed. At the end of the code block, the keyword while is followed by the condition in parentheses.
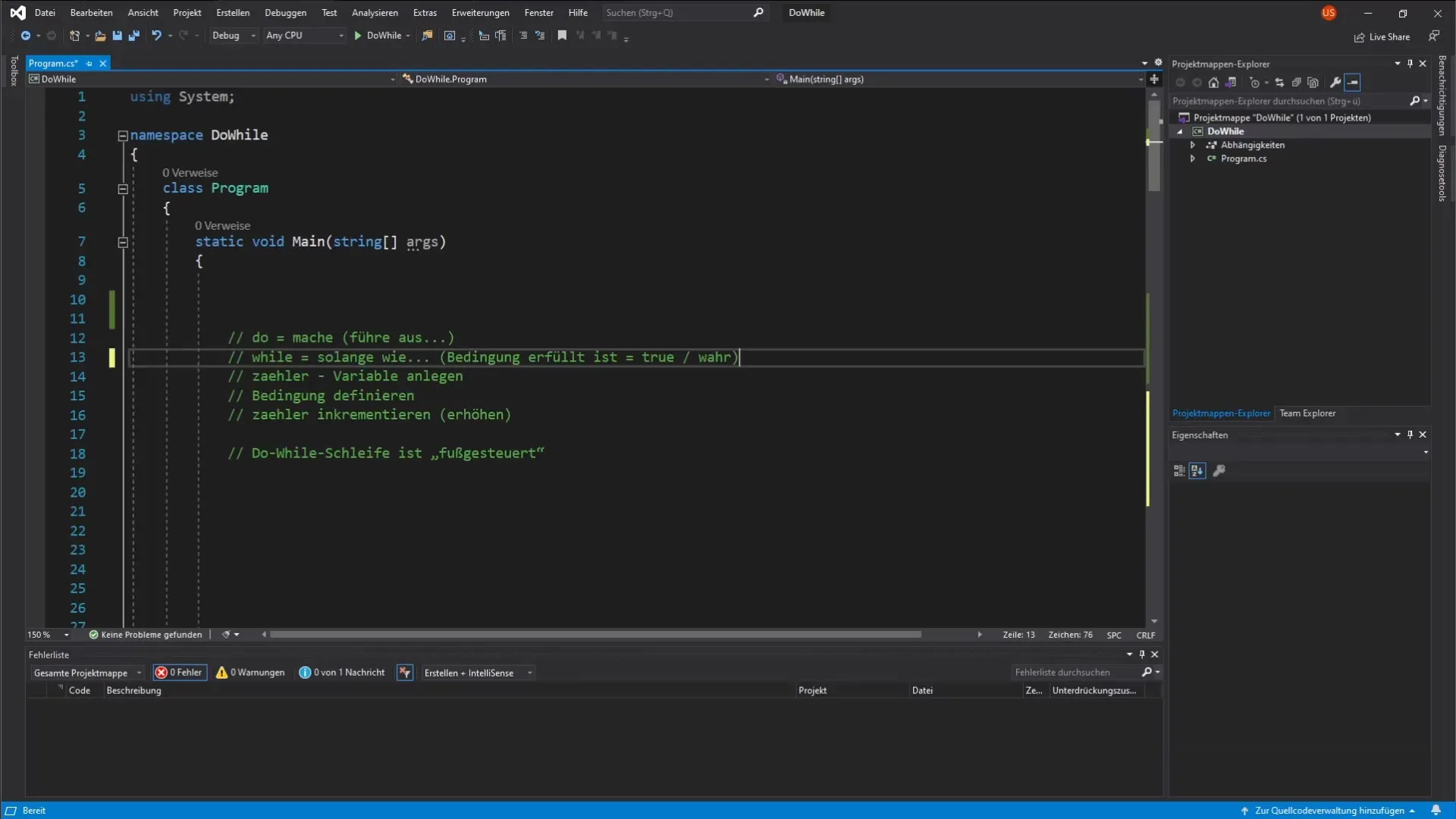
2. Declaration of the counter variable
Before you start with the Do-While loop, you need a counter variable. This variable is used to increment a counter, allowing you to control the number of iterations. For example, you could declare and initialize a counter variable as Integer zähler = 0.
3. Implementing the loop
Start with the keyword do, followed by the code that should be executed. In this code block, you can output the counter variable using Console.WriteLine(zähler) and then increment it with zähler++. This way you can track the number of loop iterations.
4. Set the condition at the end
At the end of the code block comes while followed by a condition. This condition determines whether the loop should continue or not.
5. Run and test the loop
When you run the code block now, you should be able to see the counter count from 0 to 9. The loop will stop at a counter of 10. Make sure you increment the counter variable correctly.
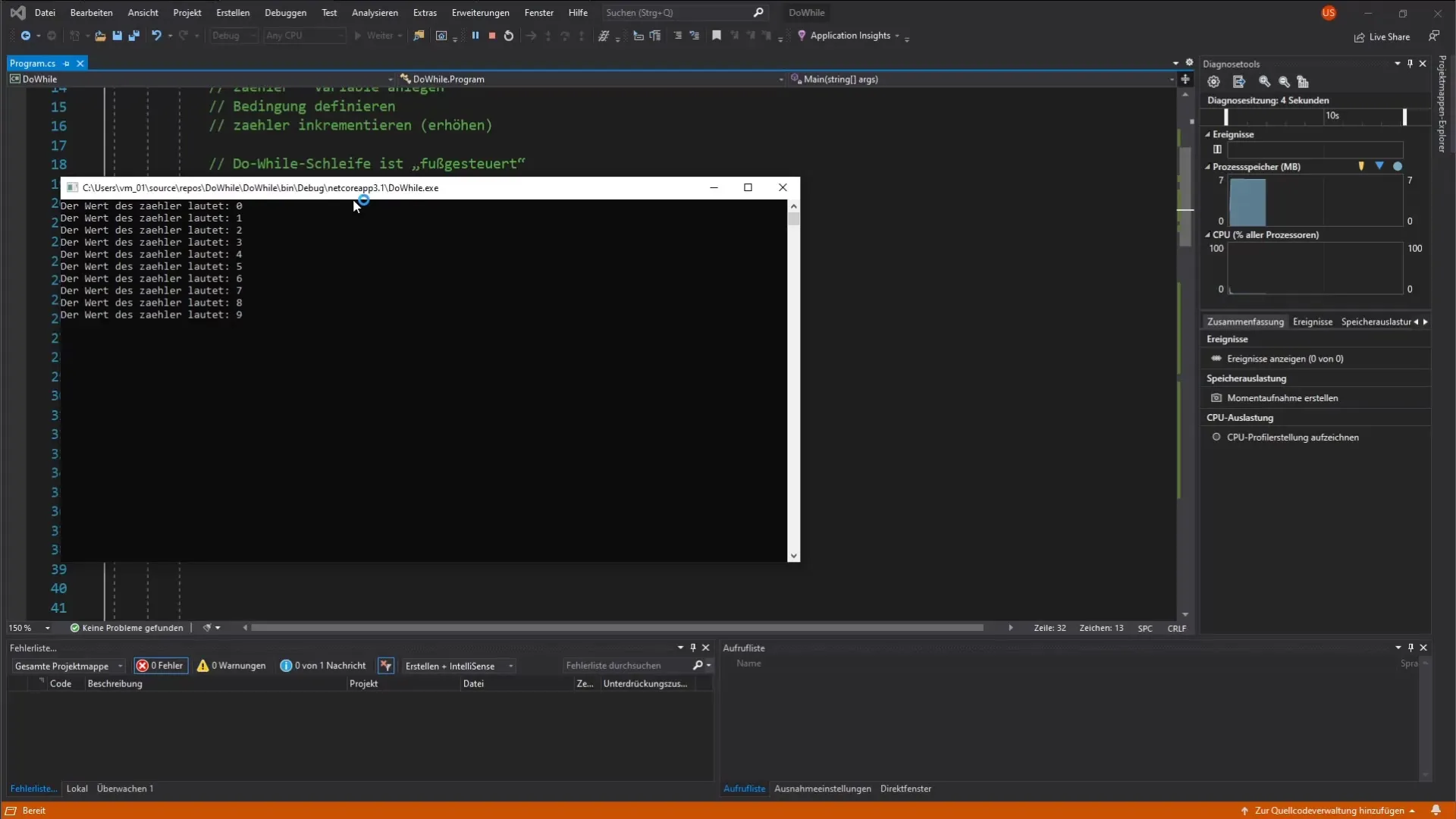
6. Special feature of the Do-While loop
One of the special properties of the Do-While loop is that it is always executed at least once, even if the condition is not met at the beginning. This differs from the conventional While loop, where the condition is checked first. Consequently, the Do-While loop is used in situations where you want to ensure that the code is initiated in any case.
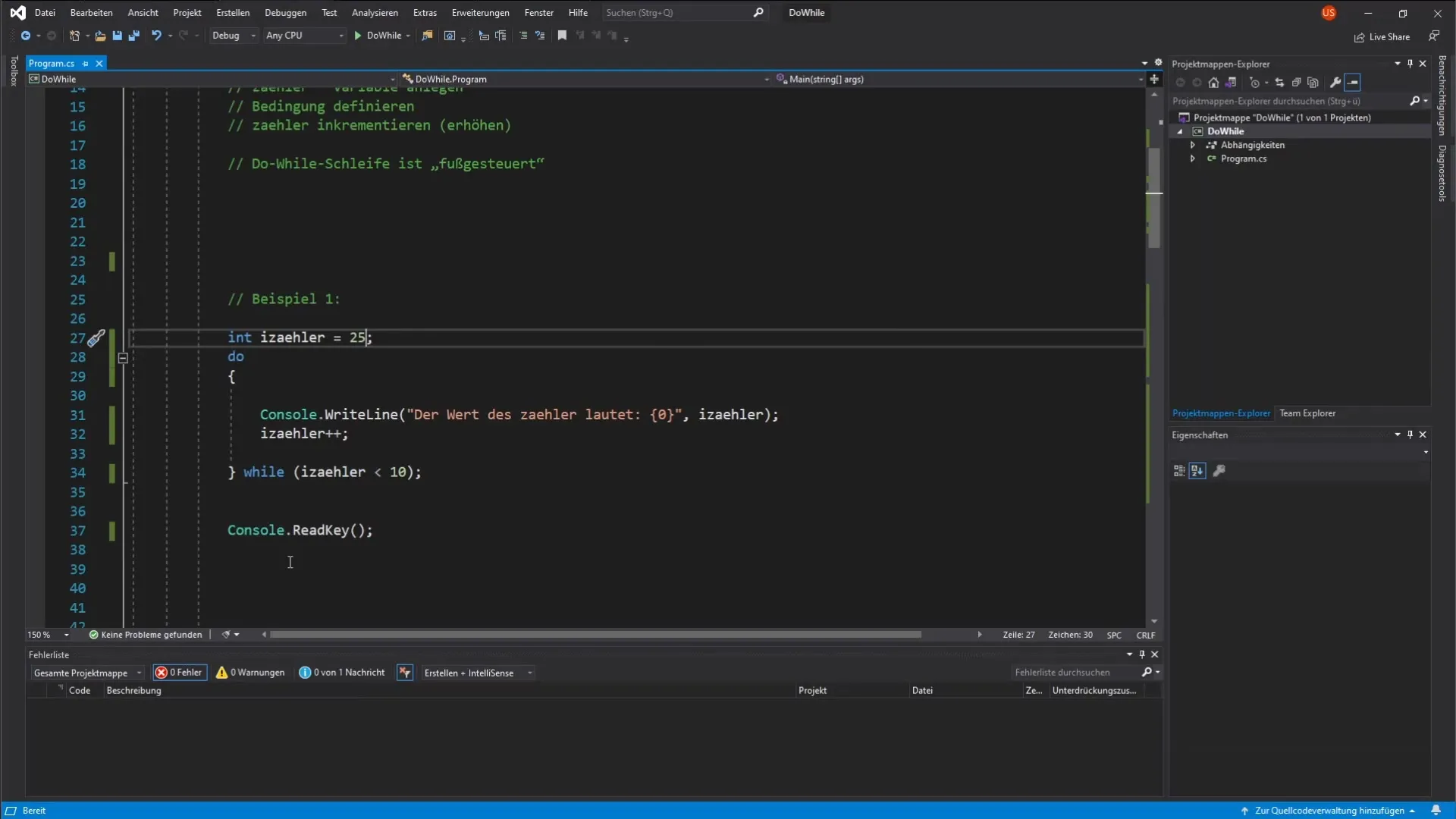
7. Example application with user interactions
A typical example of using a Do-While loop can be found in a vending machine. When a user is supposed to make a choice, the loop could run until a valid input is made. Here, you can use an if statement to validate the input. If the user makes an invalid input, signal with an error message that a new input is required.
8. Using the break command
Additionally, you can use the break command to end the loop prematurely if the input was valid. In the if statement, it is checked whether the input was correct. If so, the loop is exited with break.
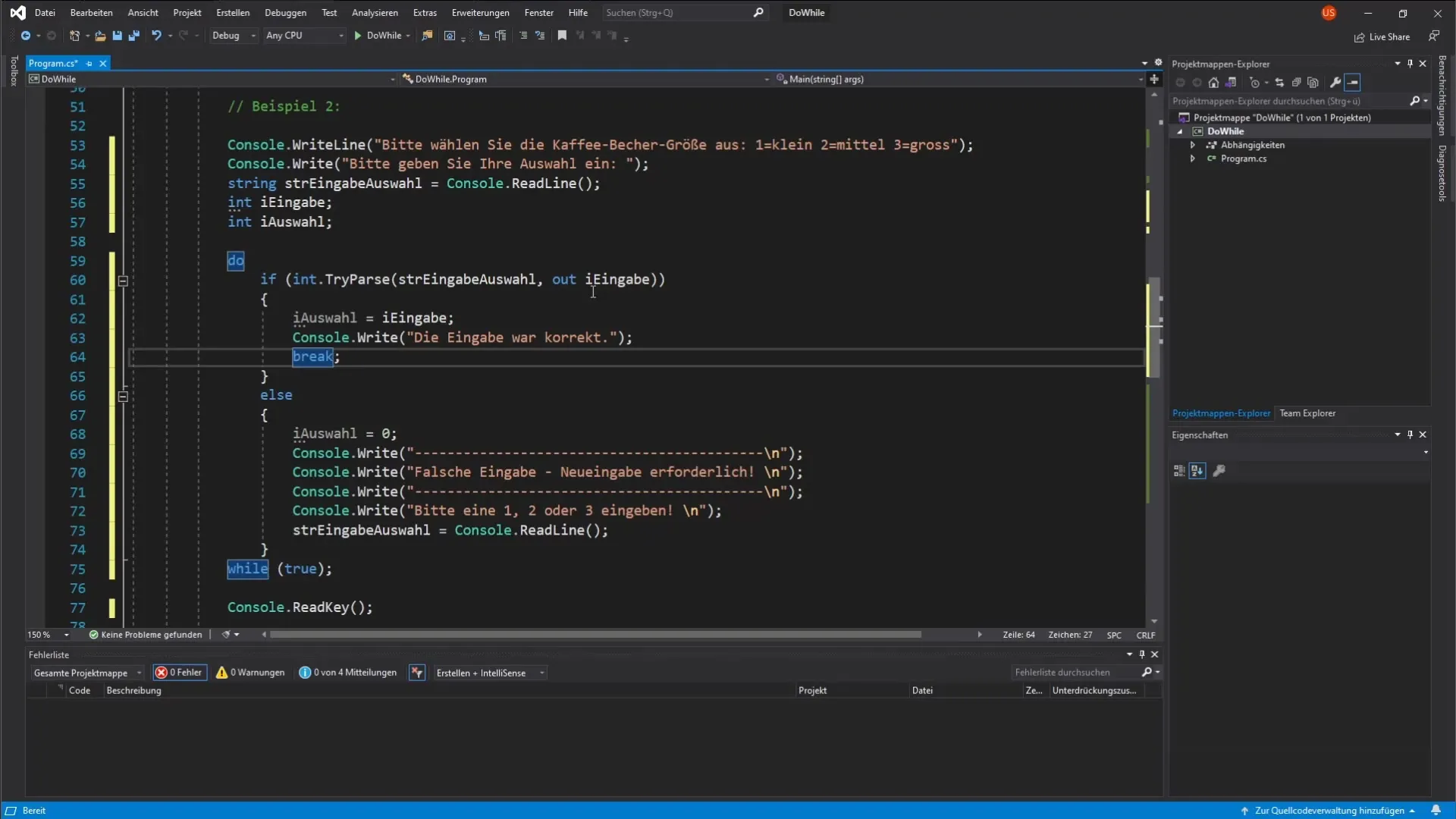
9. Expressing user preferences
For example, if the user is to enter a size for their drink choice (e.g., "1 for small, 2 for medium, 3 for large"), they will be prompted to enter. If an invalid input occurs, a clear error message should appear, and the user should be prompted to enter again.
10. Error diagnosis and improvement
It is important to ensure that your program does not create infinite loops. Make sure that the condition in the Do-While loop is realistic and that inputs are validated correctly. One possible improvement would be to ensure that only the values 1, 2, or 3 are accepted.
11. Practice tasks
A practice task for you would be to extend the code so that only inputs of 1, 2, or 3 are accepted. Ensure that the program catches all other inputs and encourages the user to retry while the cup has not yet been dispensed.
Summary – Do-While loop in C#: Effectively use and master
The Do-While loop is a powerful and versatile tool in C# that allows you to execute code at least once while seamlessly integrating user interactions. By using it, you can create clear prompts for input and efficiently validate user inputs. Take advantage of the ability to terminate the loop with the break command to optimize the user experience.
Frequently asked questions
What is a Do-While loop?A Do-While loop executes a code block at least once before the associated condition is checked.
When should I use a Do-While loop?When you want to ensure that a code block is executed at least once before a condition decides whether to continue or not.
How does the break command affect the Do-While loop?The break command causes the loop to terminate prematurely, allowing the code after the loop to execute.
Can a Do-While loop be used without a counter variable?Yes, it is possible to use a Do-While loop without a counter variable if you want to use other conditions than a counter.
How do I prevent my Do-While loop from becoming an infinite loop?Make sure that the condition of your loop is realistic and that inputs are validated correctly.