Text files are a fundamental element in programming. They allow you to store data between sessions, keep logs, or persist user inputs. In this guide, you will learn how to create, describe, modify, and append text files in C#. Through clear steps and practical examples, you will be able to successfully manipulate text files.
Key insights
- You can create and manipulate text files in C# using various methods.
- The methods File.WriteAllLines, File.WriteAllText, and File.AppendAllText are essential for writing and appending to files.
- The StreamWriter offers additional flexibility for saving text, especially for specific requirements such as character encoding.
Step-by-step guide
Writing text to a new file with File.WriteAllLines
In the first step, we will create a simple text file and write lines to it. You will start by setting up the necessary directories and adding the using directive for System.IO.
Now you can run the program. After running, you should be able to find the file "meineNeueTextdatei.txt" in the solution explorer.
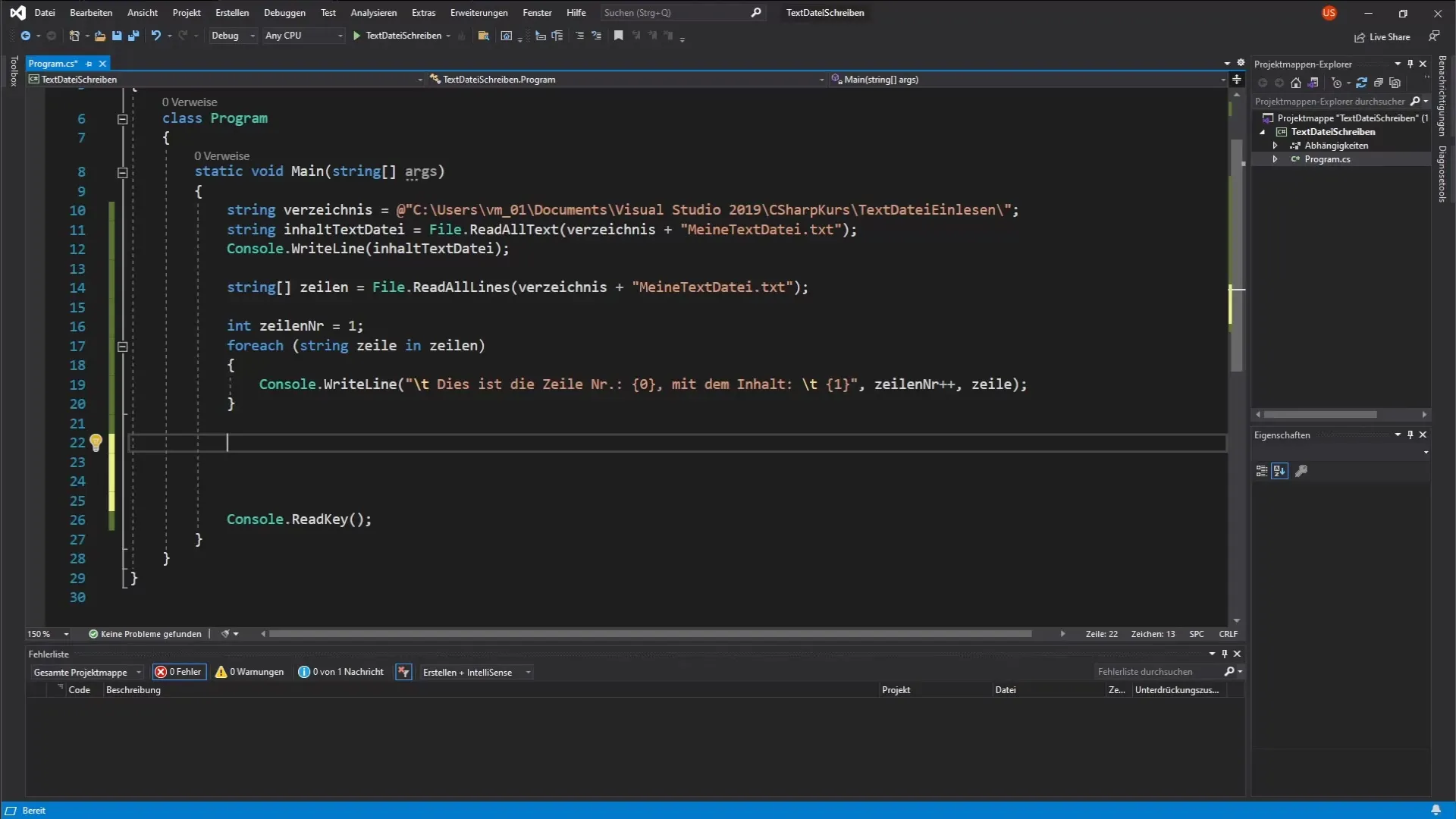
Writing completely with File.WriteAllText
The second option uses the File.WriteAllText method to write all data to a file.
Again, run the program and see the new file appear in the explorer.
Appending text to an existing file
Now you may want to add text to an already existing file instead of overwriting it. For this, we use the File.AppendAllText method.
Start the program and open the file again to check the newly added text.
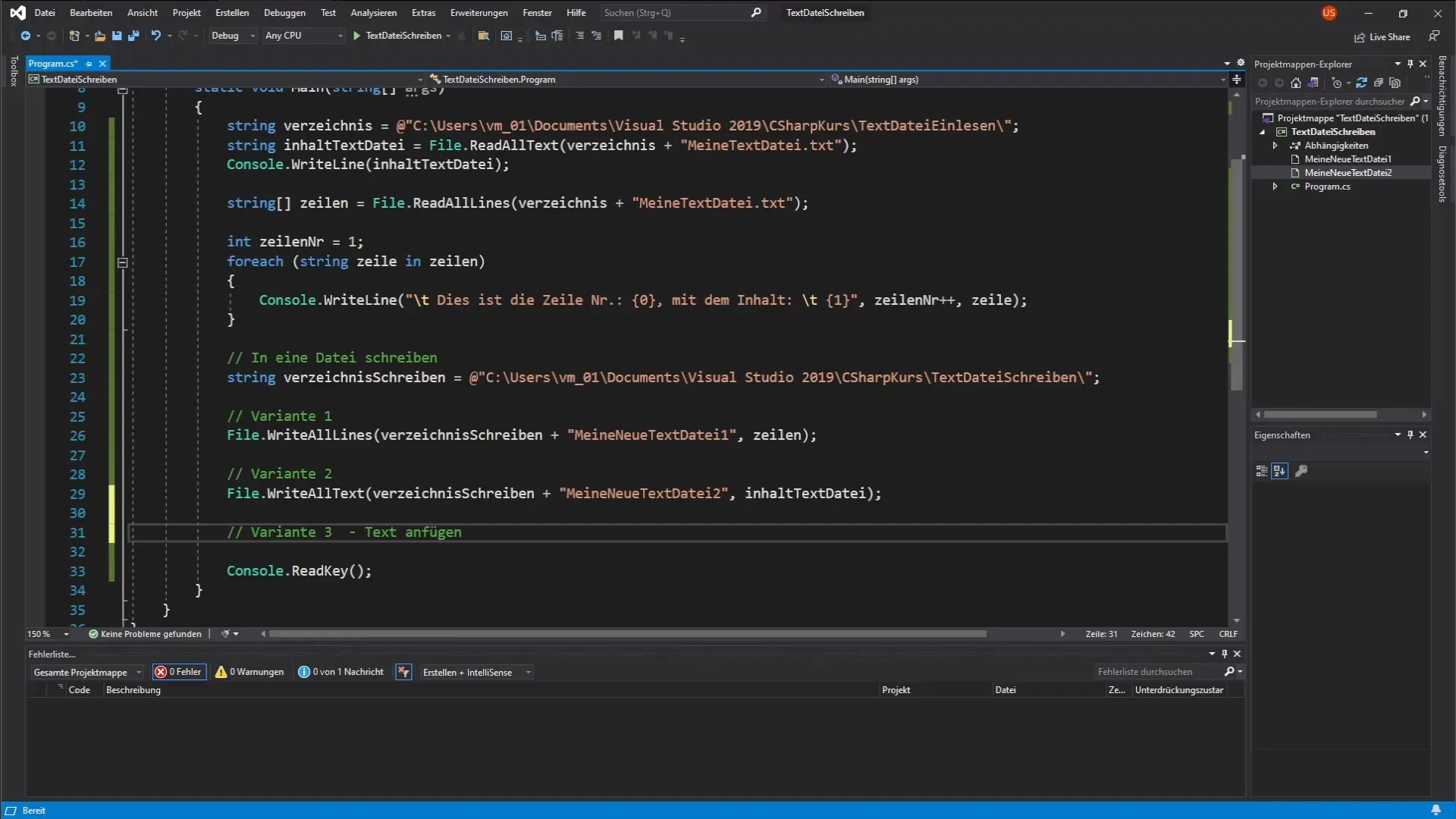
Using StreamWriter
Using StreamWriter gives you additional functions. It allows you not only to write but also to manage specific encodings.
The using statement ensures that resources are managed properly. Run the program and check if your file was created successfully.
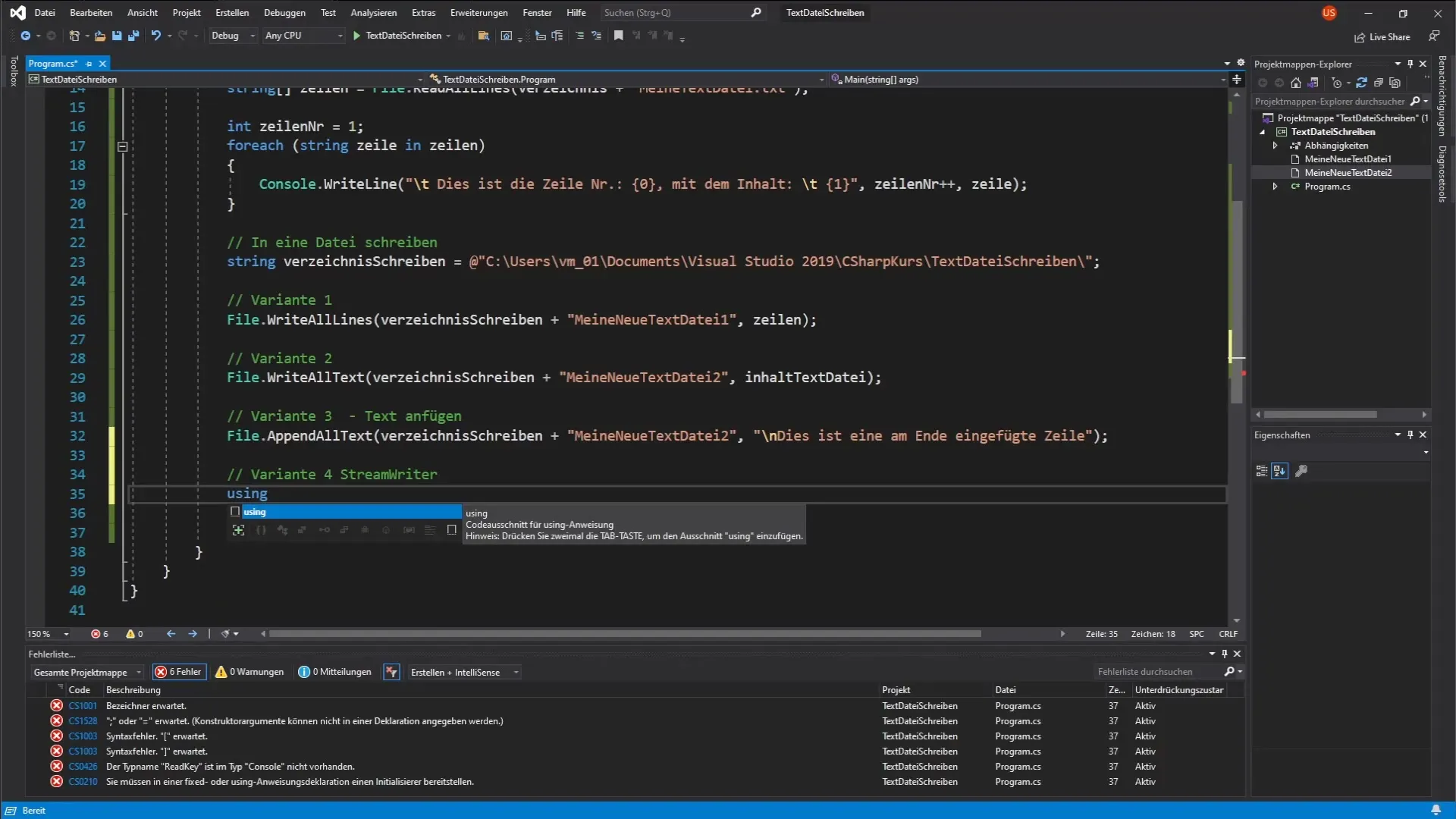
Text processing with StreamWriter without overwriting
To append to an existing file with StreamWriter, you share the constructor in the using statement with true.
This code ensures that the text is not overwritten, but appended.
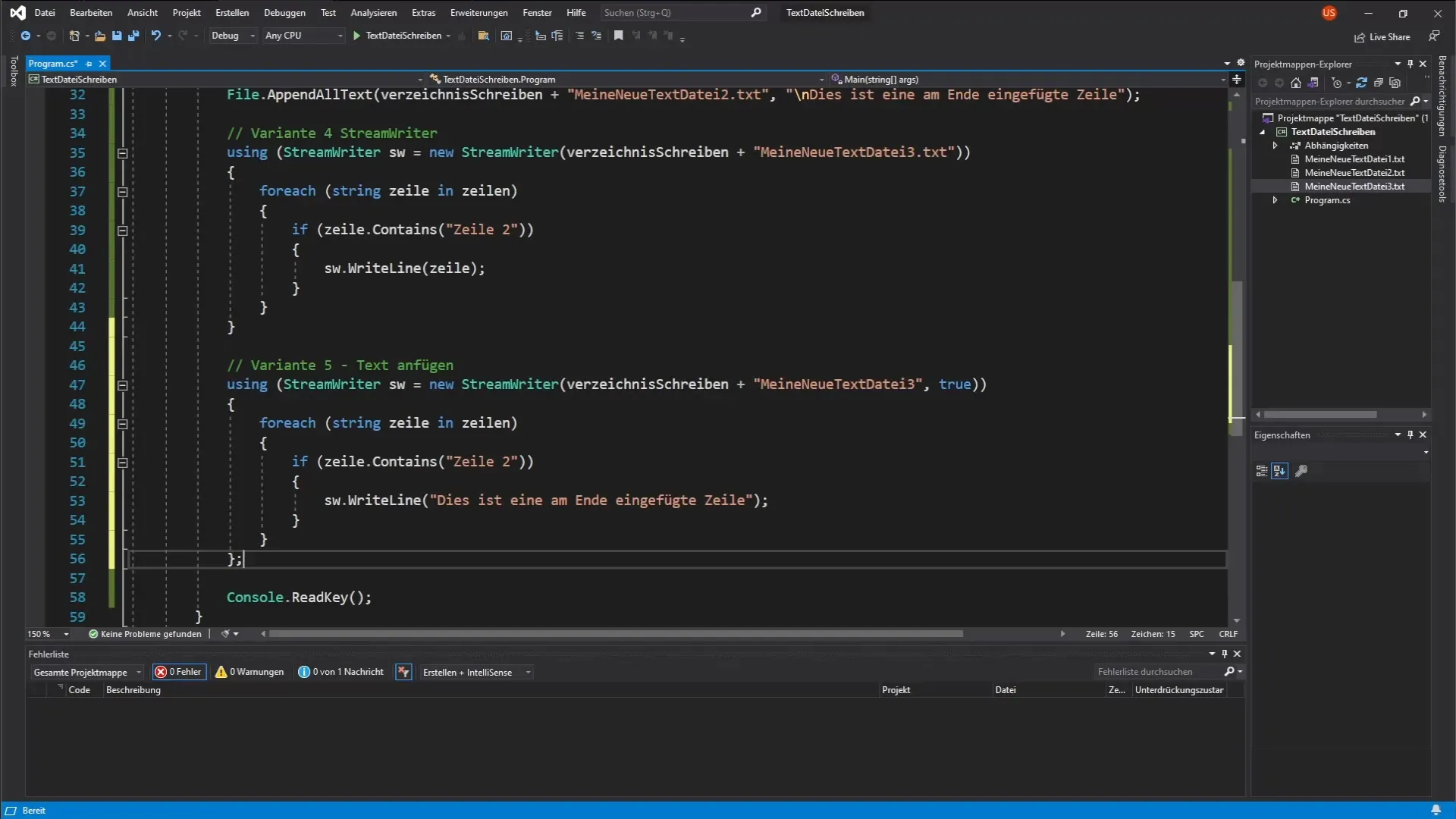
Summary – Writing text files effectively in C
You have reviewed the various ways you can write, create, modify, and append text in C# to files. Whether you want to create a new file or append to existing files, C# provides you with the necessary tools for that. With the examples in this guide, you should be able to expand or customize your application as needed.
Frequently asked questions
How do I create a new text file in C#?You can create a new text file using File.WriteAllLines or File.WriteAllText.
How do I append text to an existing file?Use File.AppendAllText or StreamWriter with the true option in the constructor.
How do I manage files in different directories?Make sure to include the full path of the file in your code.
What is the advantage of using StreamWriter?StreamWriter allows you to use specific encodings and ensures clean resource management.
How do I read content from a file?Use methods like File.ReadAllLines or File.ReadAllText for that.