Delegates are fascinating building blocks in C# programming that allow you to link methods in a flexible and dynamic way. This functionality is especially valuable when you are writing a program that is very modular and extensible. In this guide, you will learn what delegates are, how they are declared and instantiated, and how you can effectively use them to make your programs clearer and more adaptable.
Main insights
- Delegates are references to methods that can be assigned and invoked at runtime.
- They are typed and allow parameters to be passed or not.
- Delegates can be chained together, enabling multiple methods to be combined in a single delegate instance.
- Event handlers are special delegates used in many applications to respond to events.
Step-by-step guide
1. Declare a delegate
The first step when working with delegates is the declaration. You place this in an appropriate location, typically under the namespace and before the class. Use the syntax delegate [return value] [delegateName]([parameters]). In our example, it looks like this:
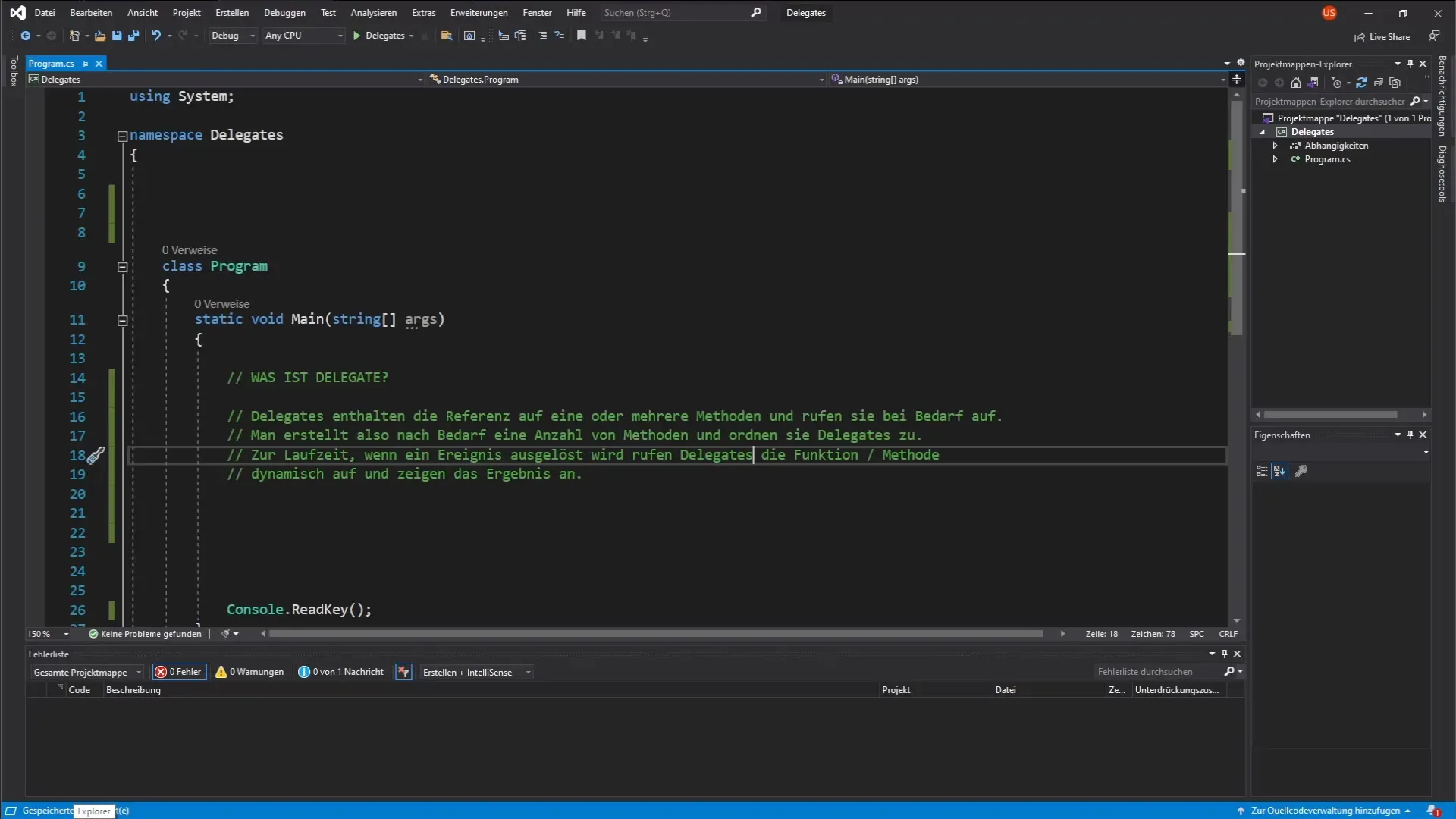
2. Instantiate a delegate
After declaring the delegate, you need to create an instance. You do this by assigning a method to a delegate variable. It is important that the methods you assign match the delegate's signature. In our example, we instantiate our delegate as follows:
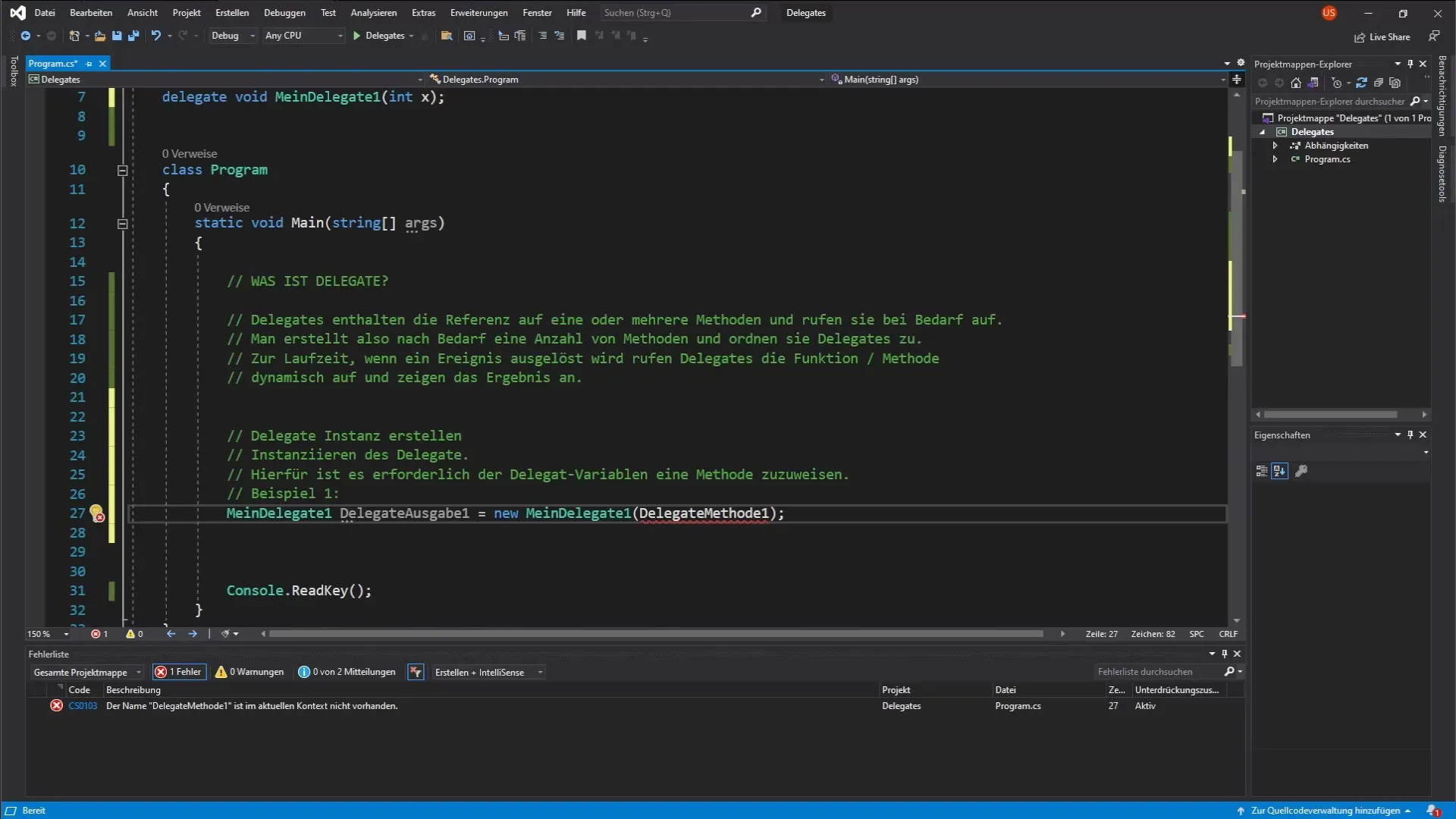
3. Create a method
Now you need to define the method that you previously assigned to your delegate. The method should have the same signature as the delegate. Here is a simple example:
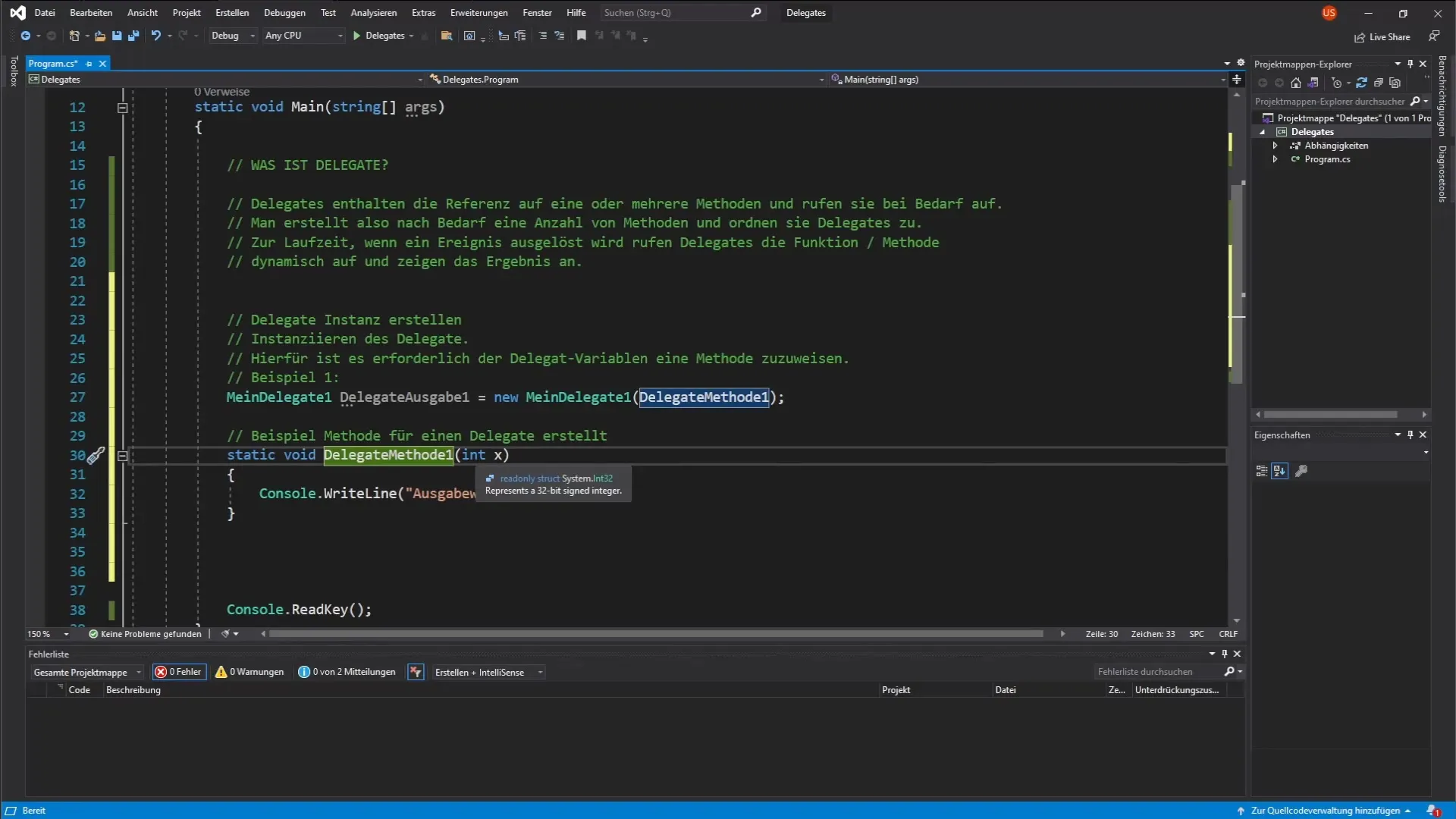
4. Call a delegate
So far, we have declared and instantiated the delegate, but we have not called it yet. To do this, you need to invoke the delegate with a value.
When you run the program, you will see that the value 12 is output on the console.
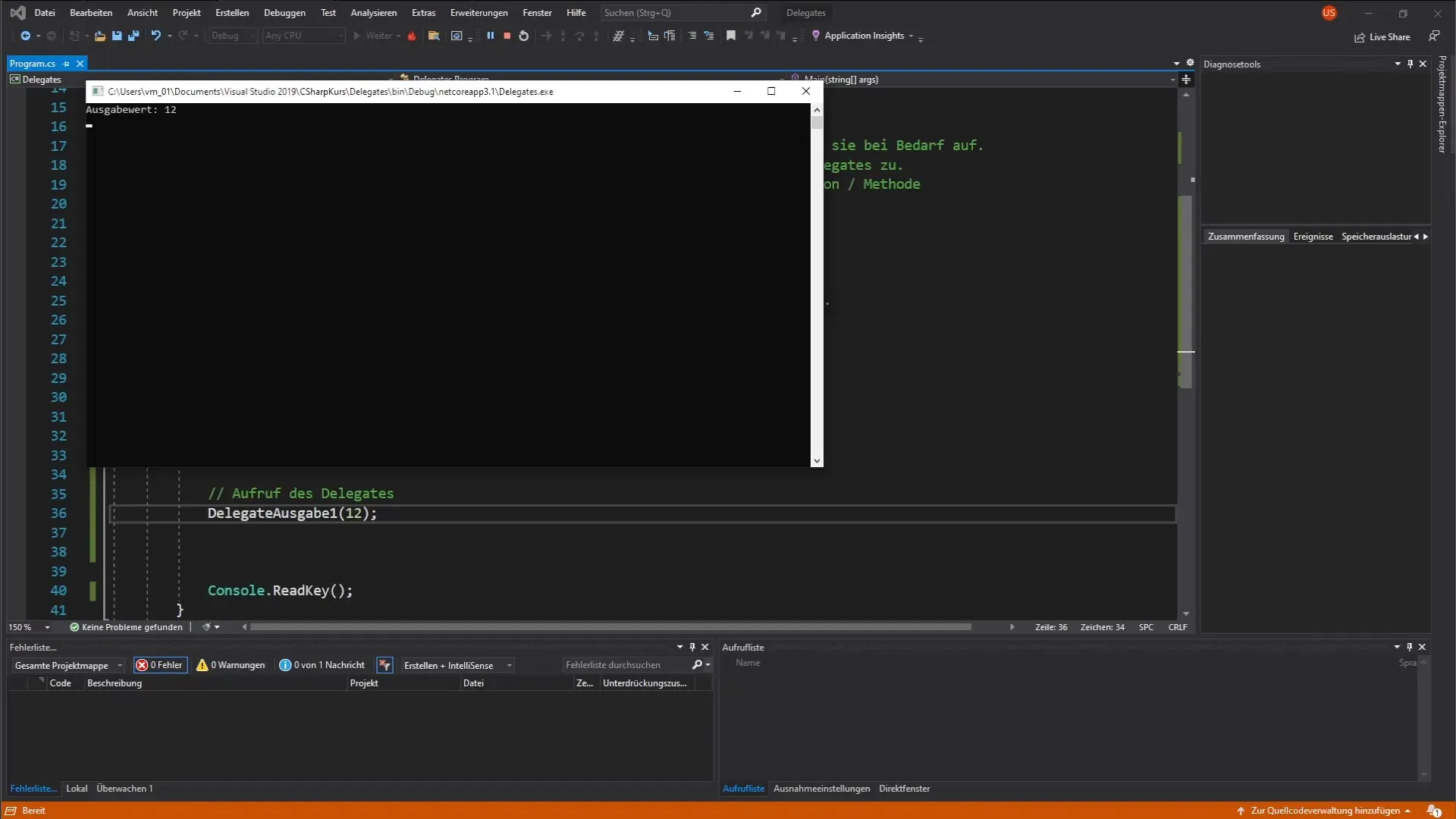
5. Delegate without parameters
Delegates do not always have to accept parameters. You can also declare a delegate without parameters.
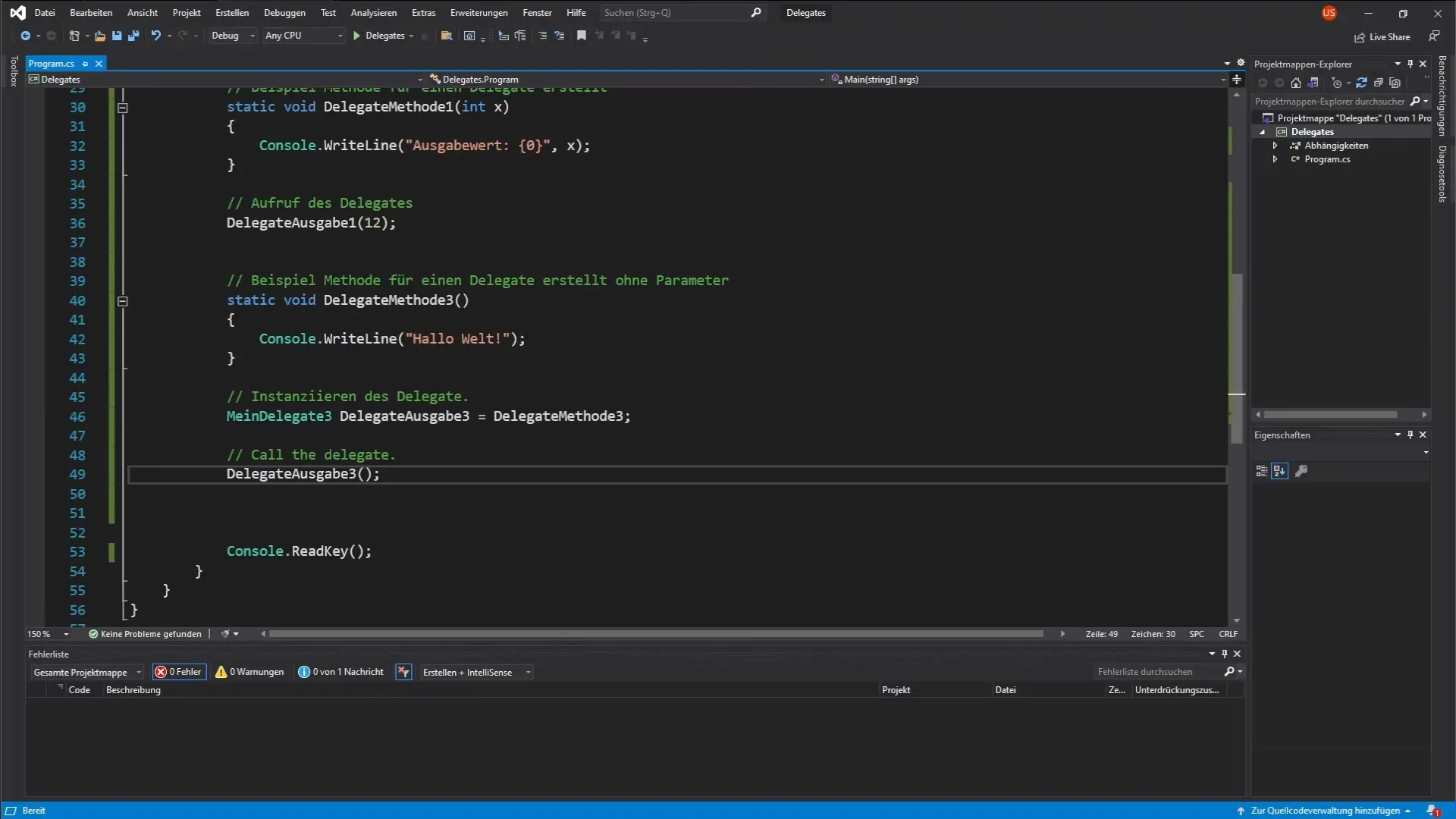
6. Chain delegates
A particularly interesting feature of delegates is the ability to chain them. You can do this with the += operator.
When you run the program, each linked method will be called in turn, and their results will be displayed.
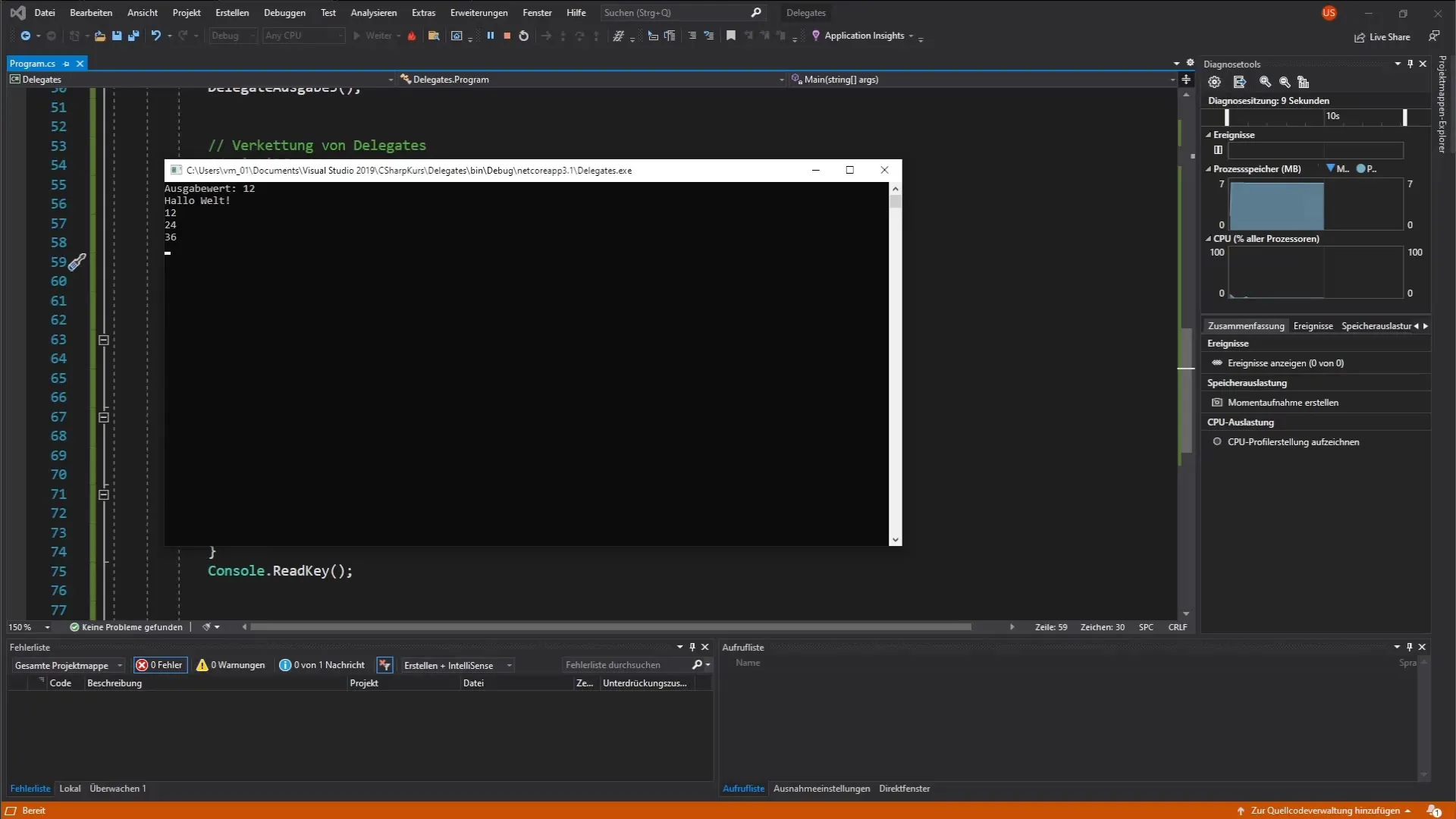
7. Understanding types and return values
Delegates are strongly typed. This means that the delegate can only assign methods with a specific signature. You can also use return values by adjusting the method signature accordingly.
Here, you can define methods that simply return values.
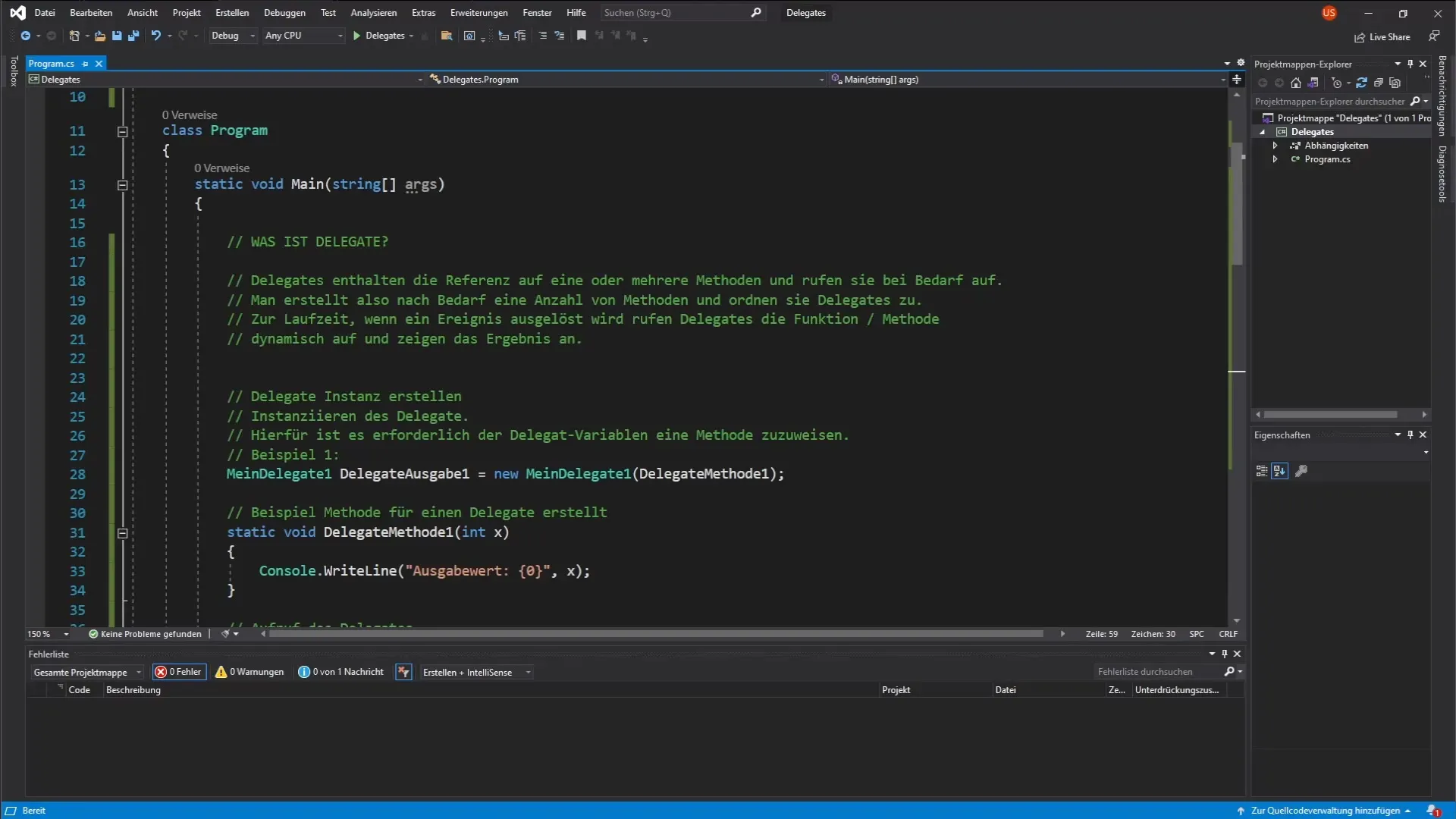
Summary – Introduction to C# Delegates: Efficiently Link Methods
Delegates are a valuable tool in C# that allows you to dynamically link methods and make them flexible. With the ability to instantiate, invoke, and chain them, they open up many application possibilities in your programming practice. To fully harness the potential of delegates, it is important to understand how they work and apply them in your projects.
Frequently Asked Questions
What are delegates in C#?Delegates are types that represent references to methods with a specific parameter list and optional return values.
How do I declare a delegate?A delegate is declared using the syntax delegate [return value] [delegateName]([parameters]).
Can a delegate have parameters?Yes, a delegate can have parameters, or there can also be a delegate without parameters.
How do I invoke a delegate?A delegate is invoked just like a method by using its instance with the required parameters.
What does it mean to chain delegates?Chaining delegates allows you to link multiple methods to a delegate so they are called one after the other.