Clarity in process flows of software development is becoming increasingly important, especially when it comes to object-oriented programming. A central aspect of this programming style is polymorphism. With a deeper understanding of your application logic, you can create more flexible and maintainable programs. Let’s dive together into the world of polymorphism and experience the concepts through practical examples.
Key findings
Polymorphism allows objects to present themselves in different forms. This occurs through method overriding and overloading. The key concepts include:
- Use of virtual methods in base classes
- Application of "override" in subclasses
- The concept of casting objects
- Extending functionalities by simply adding new functions
Step-by-step guide
Step 1: Introduction to polymorphism
To understand polymorphism in C#, let's first look at the underlying concept. The term comes from Greek and means "many forms." In object-oriented programming, it means that a method in a base class can be overridden and overloaded in subclasses. This allows you to utilize common interfaces while simultaneously redefining the implementation.
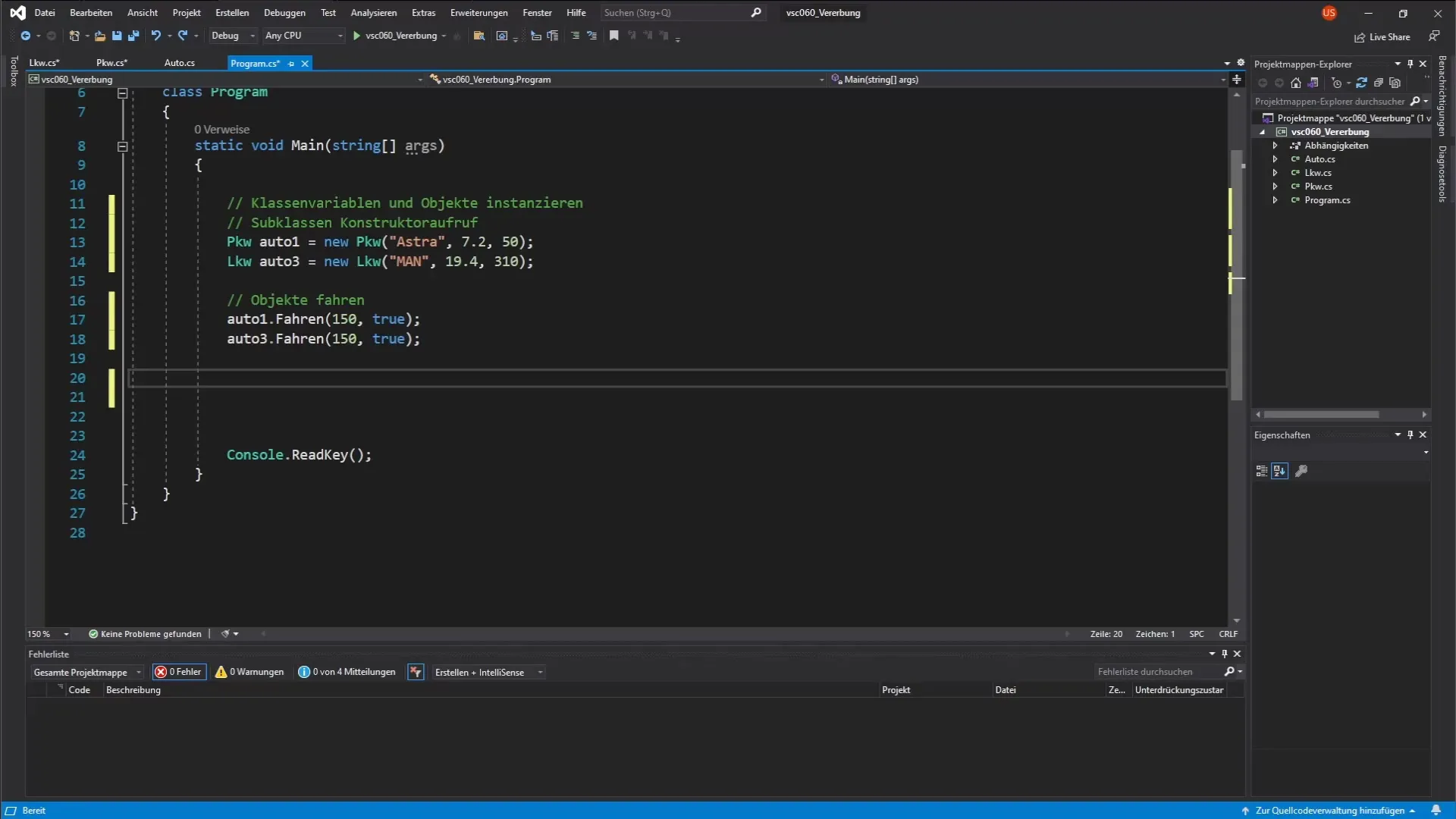
Step 2: Creating the structure
Start with a basic structure that defines a base class. In this case, we will use a class named Auto. This class should also contain a method called "TÜV." The method is declared in the base class but not yet implemented.
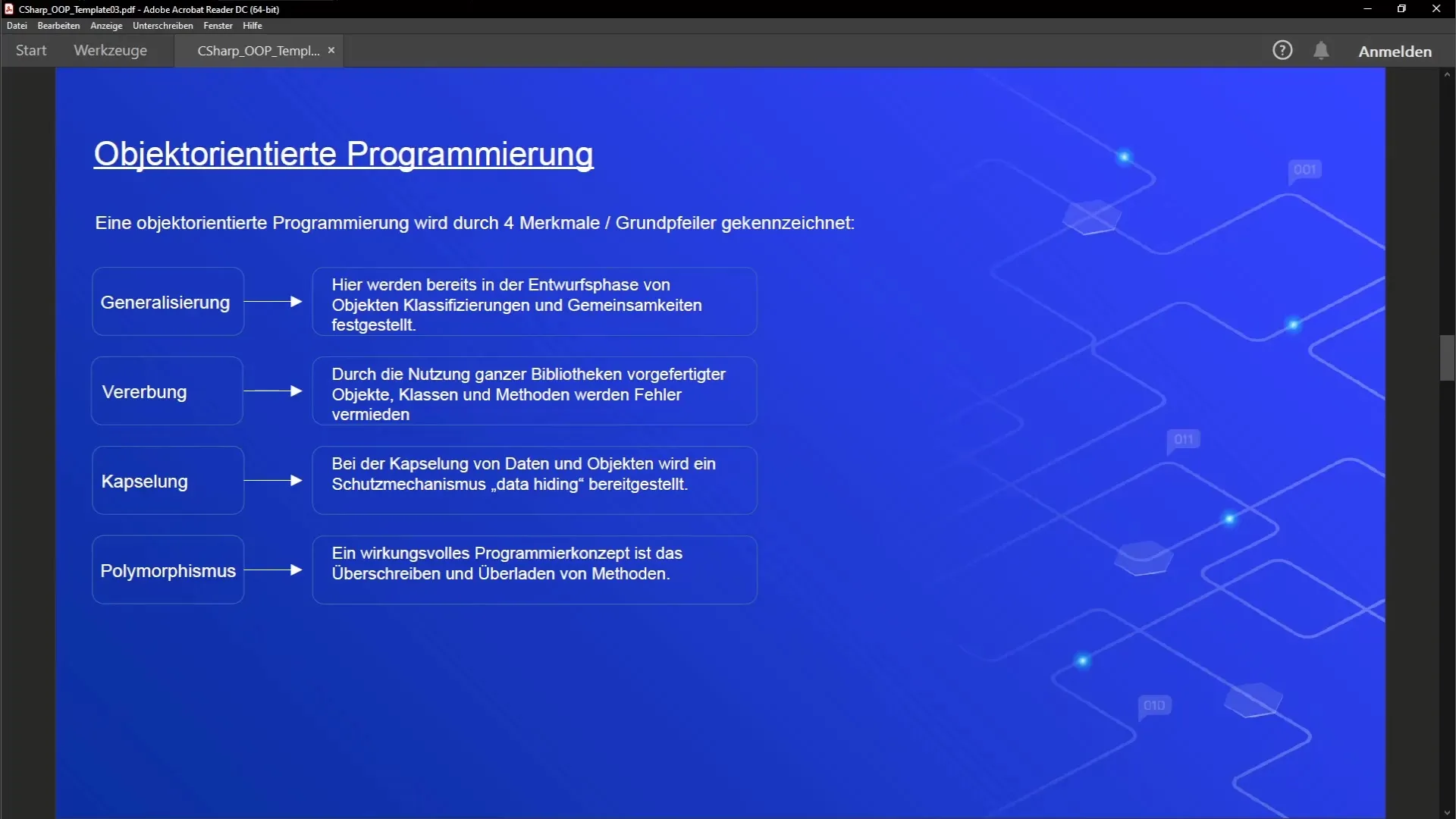
Step 3: Defining the subclasses
Now create the subclasses PKW and LKW. Each subclass should extend the base class Auto. In these classes, you will implement the TÜV method using the override keyword. This adapts the base class method so that each subclass can have its specific logic.
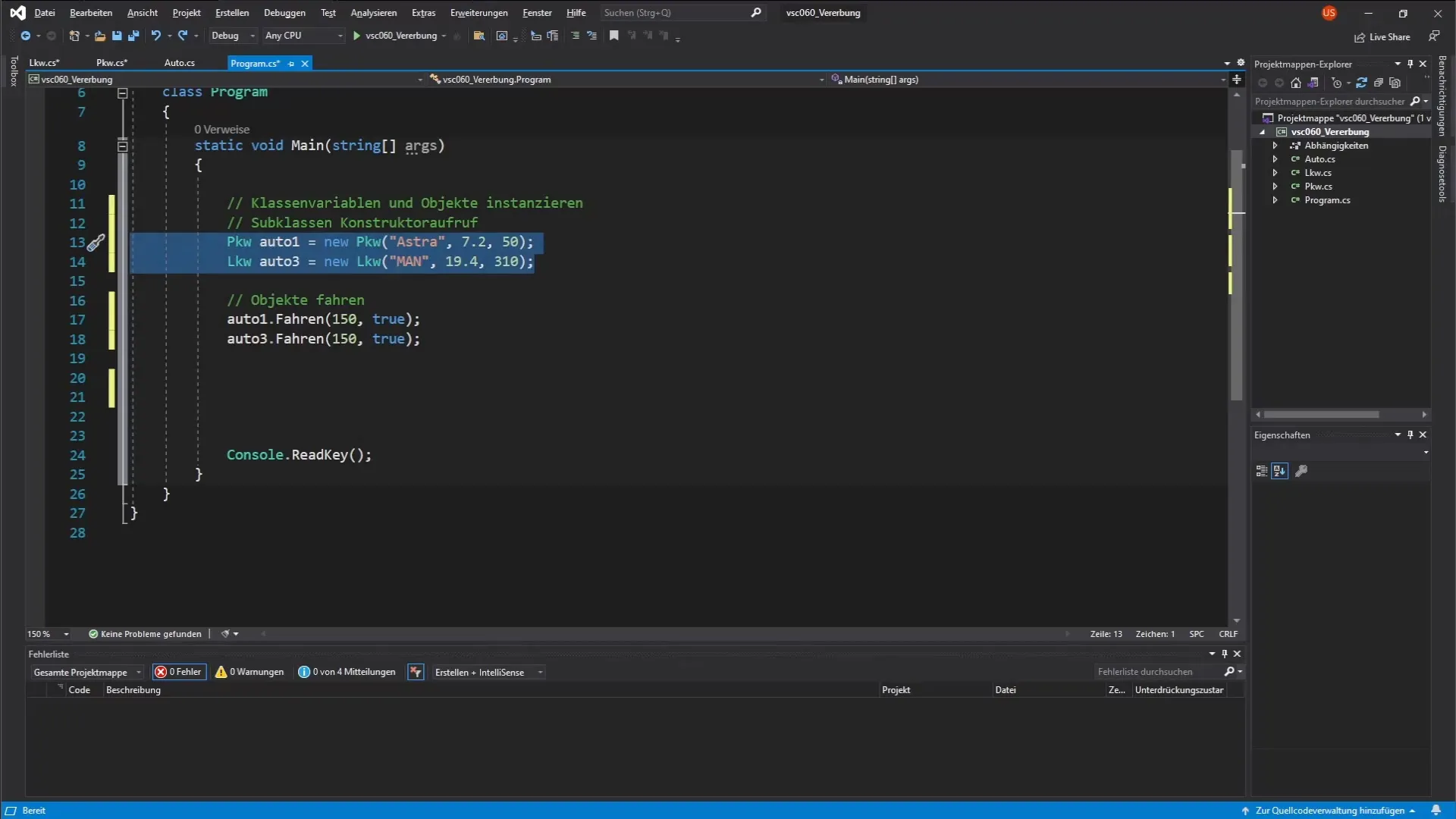
Step 4: Implementing the virtual method
Before overriding the TÜV method in the subclasses, the virtual keyword must be specified in the base class. This signals that the base class method can be overridden.
Step 5: Creating a list for objects
To further explore polymorphism, set up a list that can store both PKW and LKW objects. In C#, you can do this using the List data type. Ensure to add the correct using directive for generic collections.
Step 6: Adding objects to the list
In this list, you can then create multiple PKW and LKW objects. These objects will be separated by a comma in the collection. It is important to know that despite different types, all objects in the list share the type of the base class Auto.
Step 7: Calling polymorphism
Now that you have a list, create a foreach loop that iterates through all these objects. Each time an object is processed, call the TÜV method. Depending on the type of the object, the specific implementation of the subclass will be used.
Step 8: Analyzing the result
Let the program run and observe the output. It will show you which car was at TÜV when. Polymorphism ensures that the TÜV method of the respective subclass is called, not that of the base class.
Step 9: Testing the effects of "override"
Gain clarity on how the override keyword works by temporarily removing it from the PKW class. What do you see when the program runs again? The base class method should then be called, showing you how important override is.
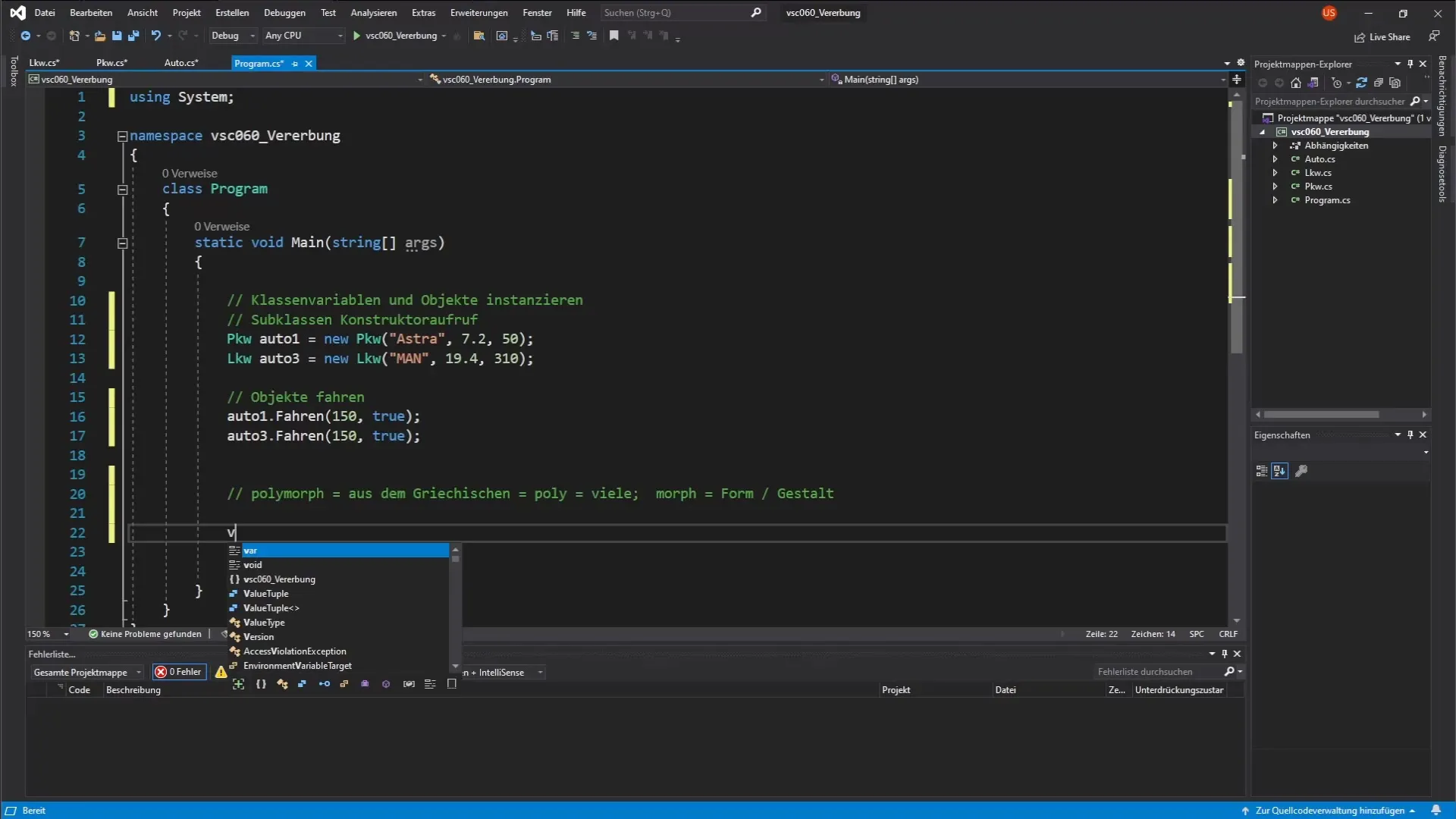
Step 10: Expanding your application
To deepen your understanding of polymorphism, you can create new methods like refuel and conduct similar steps. See how flexible your approach is and continue practicing the concepts.
Summary – Polymorphism in C#: A guide to understanding and practical application
Polymorphism is a fundamental concept of object-oriented programming that allows you to write flexible and extensible software. By using virtual methods and overriding subclass methods, the reusability and maintainability of your code are enhanced. Here, you learned how to create a base class and its subclasses, manage objects in a list, and effectively call them polymorphically.
Frequently asked questions
How do I use the virtual keyword?The virtual keyword is used in the base class to indicate that a method can be overridden in subclasses.
What does the override keyword do?override allows a subclass to redefine a method of the base class.
How do I create a list of objects with different types?You can use the List type to store both PKW and LKW objects in a list.
What happens if I remove override?Without override, the method of the base class is executed, not the implementation in the subclass.
How can I further test my application?You can create new methods and add different objects to see how polymorphism works.