The do/while loop is an excellent choice when you need a repetition that must run at least once, regardless of the condition. In this tutorial, you will learn how to apply this loop and recognize its advantages compared to the classic while loop. We will also go through practical examples to better understand the concept.
Main Insights
- The do/while loop guarantees that the loop content is executed at least once.
- It is particularly useful when the condition is checked only after the first execution.
- This loop reduces code duplication and improves readability.
Step-by-Step Guide
1. Initial Situation: The While Loop
To understand the advantages of the do/while loop, let's first look at the classic while loop. Suppose you want to generate a random number between 1 and 3 and ensure that you get the number 2. Let's start with this simple task.
To generate a random number, we use the Math.floor() and Math.random() functions. Here is the basic code you can use:
If you run this code, you might get different results. Each time x is not equal to z, another random number is generated and displayed.
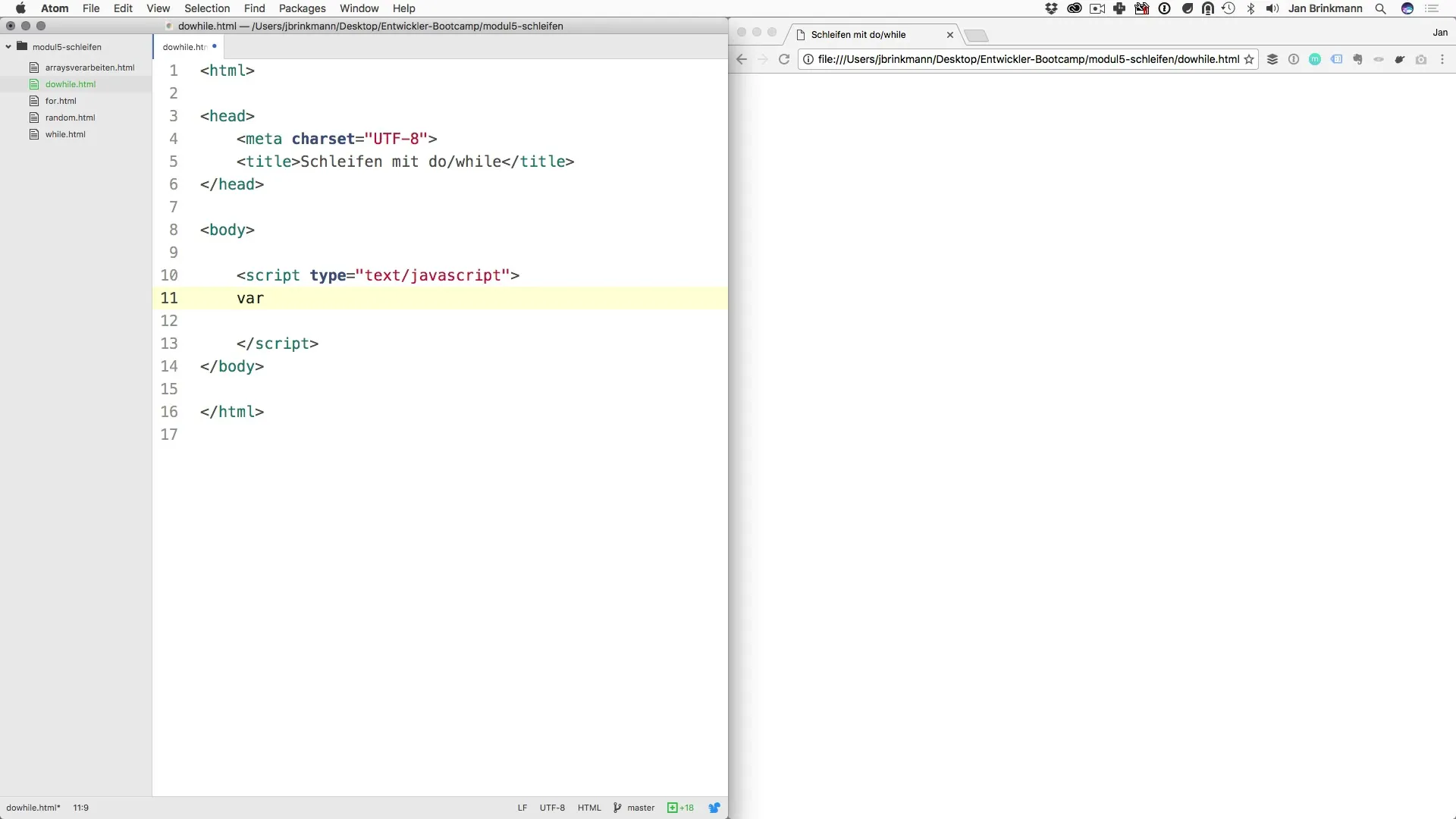
However, a problem could arise: It is possible that you receive the number 2 on the first try. In such cases, there is no reason to continue executing the loop.
2. The Disadvantage of the While Loop
The code we wrote with the while loop is not particularly elegant. We need to ensure that we generate the random number only once instead of recalculating it after the first run. Here are the possible results you would see if you run the loop multiple times.
The problem with this is that we waste time and resources. Our loop could be more efficient if we used a different structure. This is where the do/while loop comes into play.
3. Introduction to the Do/While Loop
The do/while loop has the advantage of executing the block before checking the condition. This means that the code in the block is executed at least once, no matter what.
By using do {... } while (condition), the random number is generated and output before the condition is checked.
This way, we can ensure that the number is generated and displayed at least once before the loop condition is validated.
4. Advantages of the Do/While Loop
The biggest advantage of the do/while loop is readability and reducing duplicates in the code. You don't have to create the random number before the loop, but can easily do that within the loop. This avoids writing the same code multiple times and improves maintainability.
Additionally, the do/while loop allows for a more elegant handling of scenarios where the condition only becomes relevant after the first execution. This is useful not only in JavaScript; many programming languages offer similar functionality.
5. Conclusion: The Do/While Loop as an Elegant Solution
In summary, the do/while loop offers a clear advantage in code structure and efficiency when execution must be guaranteed at least once. It allows you to write simpler and less error-prone programs.
It is recommended to use the do/while loop when this logical structure best fits your problems. You can subsequently deepen your knowledge with exercises to ensure that you fully understand the warnings and advantages of this loop.
Summary - Do/While Loops: Elegant and Efficient Programming for Beginners
You learned in this tutorial how to use the do/while loop in programming. You discovered its advantages over the traditional while loop and were able to get to know the structure that can help you write readable and efficient code.
Frequently Asked Questions
What is the main difference between a while loop and a do/while loop?The while loop checks the condition before executing the block, while the do/while loop executes the block at least once before checking the condition.
When should I use a do/while loop?You should use a do/while loop when you want to ensure that a block is executed at least once, regardless of the condition.
Are there alternatives to the do/while loop?Yes, you can also use recursive functions in various situations; however, do/while loops are often clearer and more structured for simple repetitions.
In which programming languages is the do/while loop available?The do/while loop is present in many programming languages, including JavaScript, C++, Java, and C#.