The understanding of scopes is a fundamental skill in software programming. In programming, a scope refers to the area in your code where a variable is visible, i.e., where you can access a variable. In this guide, you will explore the concept of scopes, particularly in JavaScript, and learn how to handle global and local namespaces.
Key Insights
- A scope determines where a variable is accessible within the code.
- There are global and local scopes. Global variables are accessible everywhere in the code, while local variables are only accessible within their function.
- Each function call in JavaScript has its own local scope.
Step-by-Step Guide
Step 1: Create Global Variables
Start by creating a global variable. You can do this by declaring a variable outside of any function. In our example, we will call the variable Version and set it to 1.2. This variable is now accessible in the global namespace, meaning it can be accessed by any function within your script.
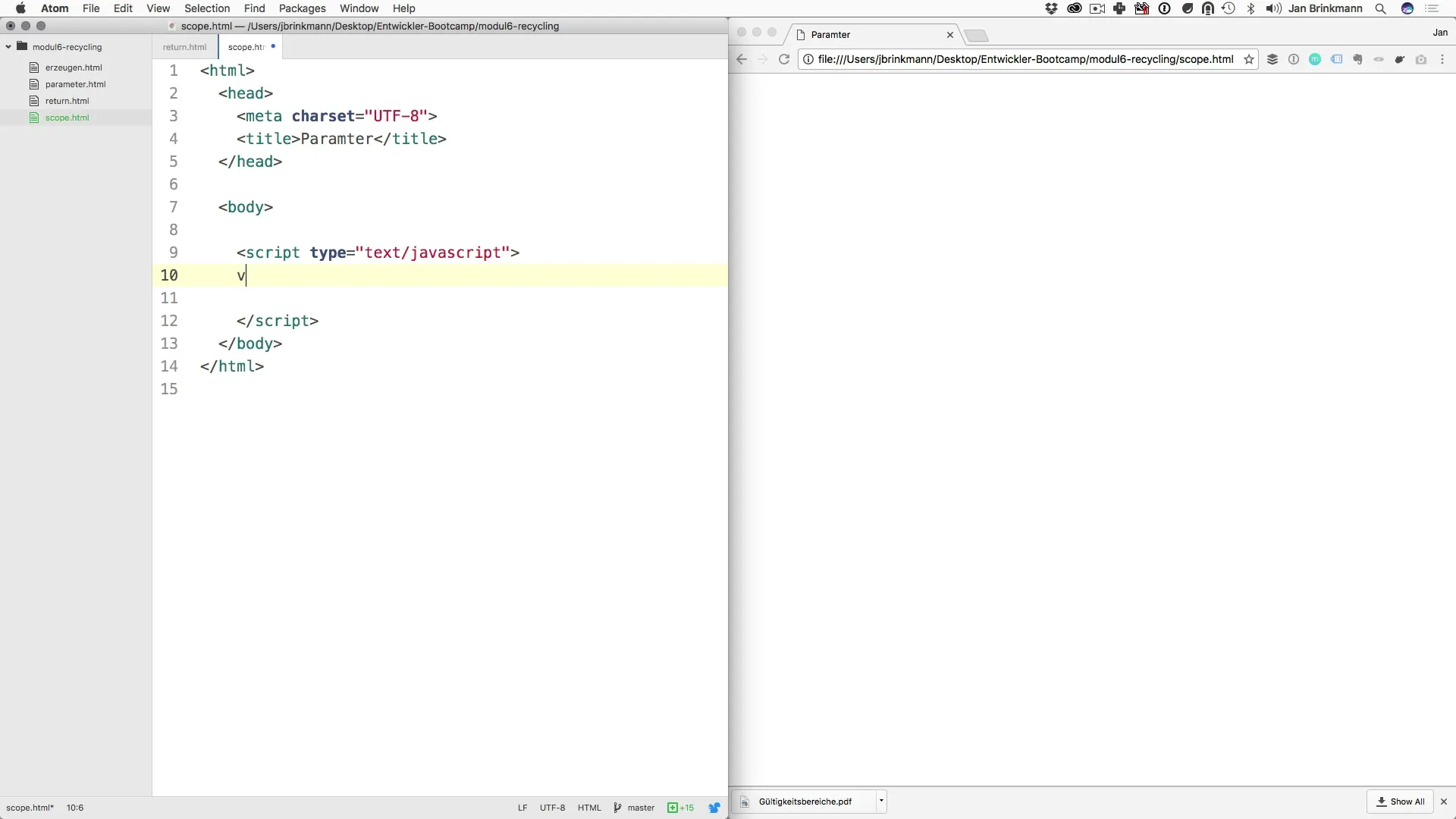
Step 2: Use Global Variables in Code
Now you can use the global variable in your code. Create a simple function that we will call Function Test. Within this function, you can access the global variable and use it, for example, in an alert command. When you refresh the page after calling the function, the version will be displayed correctly.
Step 3: Create Local Variables
Now it’s time to declare a local variable. Inside your Function Test, add a variable called Name and assign it the value “Terminator.” This variable is now only visible within the function and cannot be accessed outside of it.
Step 4: Access Local Variables
Before testing access to the local variable, try accessing it from outside the function. You will see that it does not work since the variable is only available in the local namespace within the function. So remove the code that attempts to access the local variable from outside.
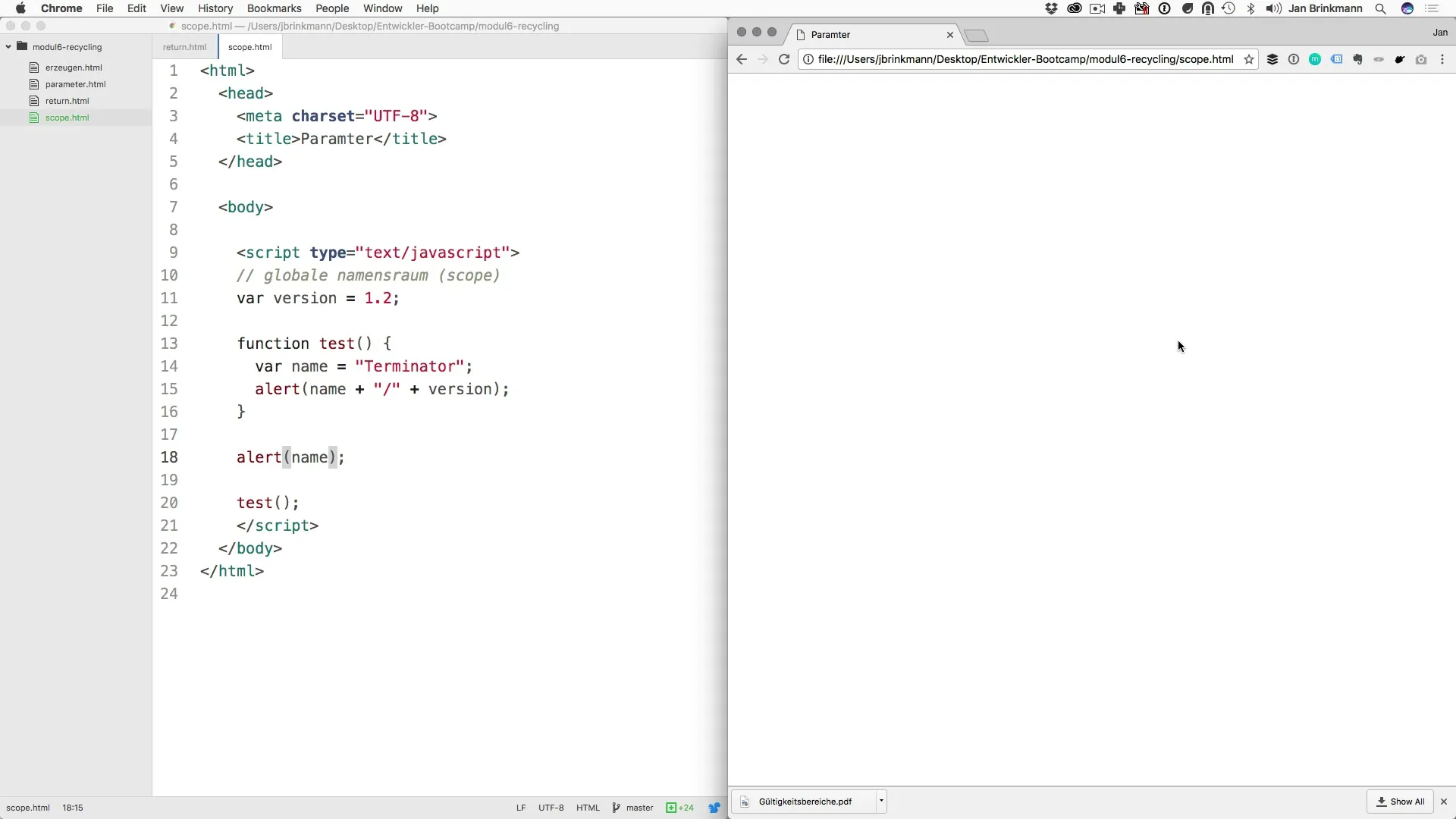
Step 5: Illustrate Scopes
A better understanding of the different scopes can be achieved with a visual representation. In this graphic, the green area represents the global namespace where the version is defined, while the yellow area illustrates the local namespace of your function. Functions have their own namespace, and variables in that namespace are not accessible from outside.
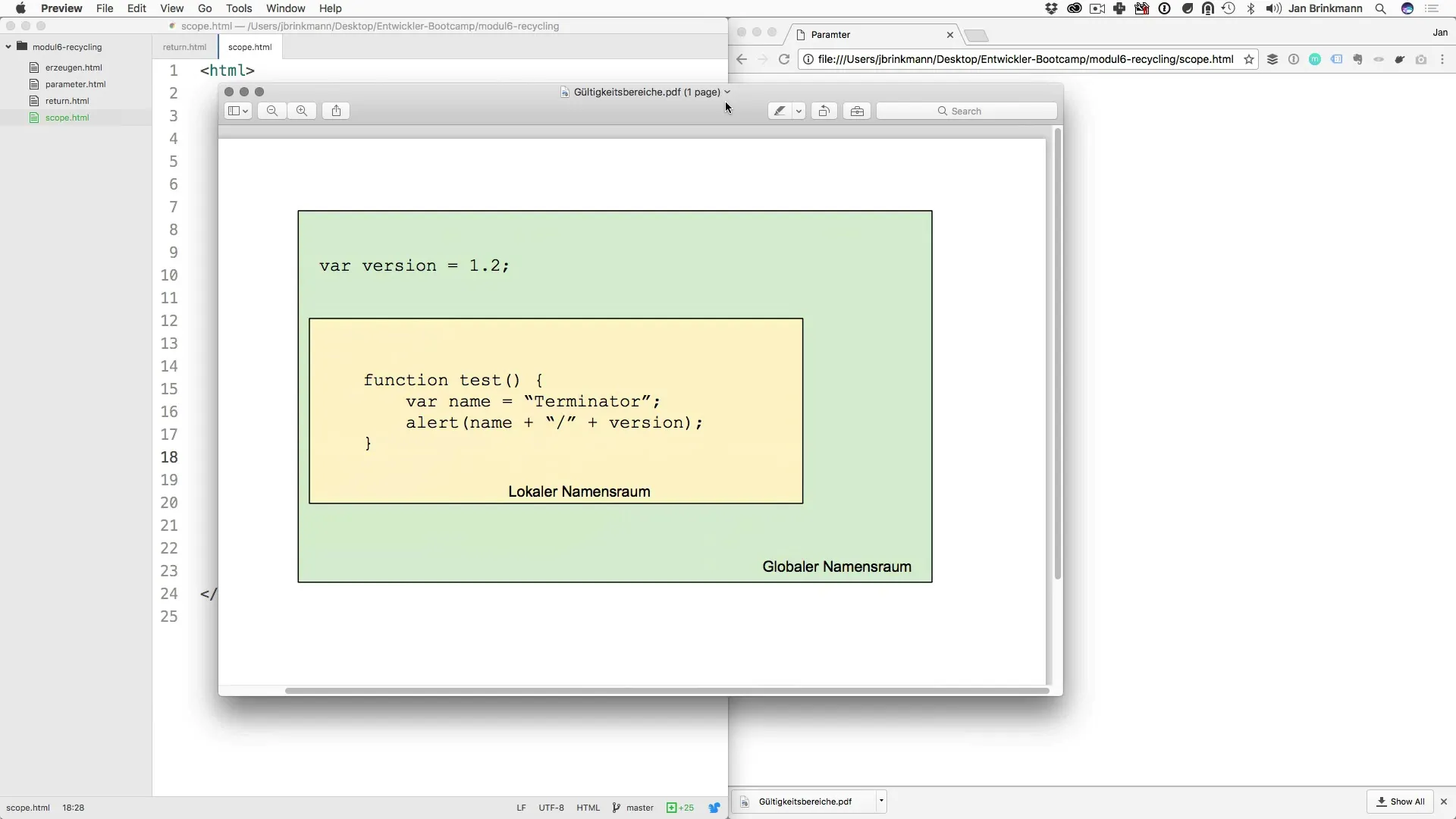
Step 6: Practical Application
To deepen your understanding of scopes further, you should create more functions and assign each their own local variables. Note that each time you declare a new function, a new, isolated local namespace is created. Experiment with different variables and function calls to better understand the behavior of scopes.
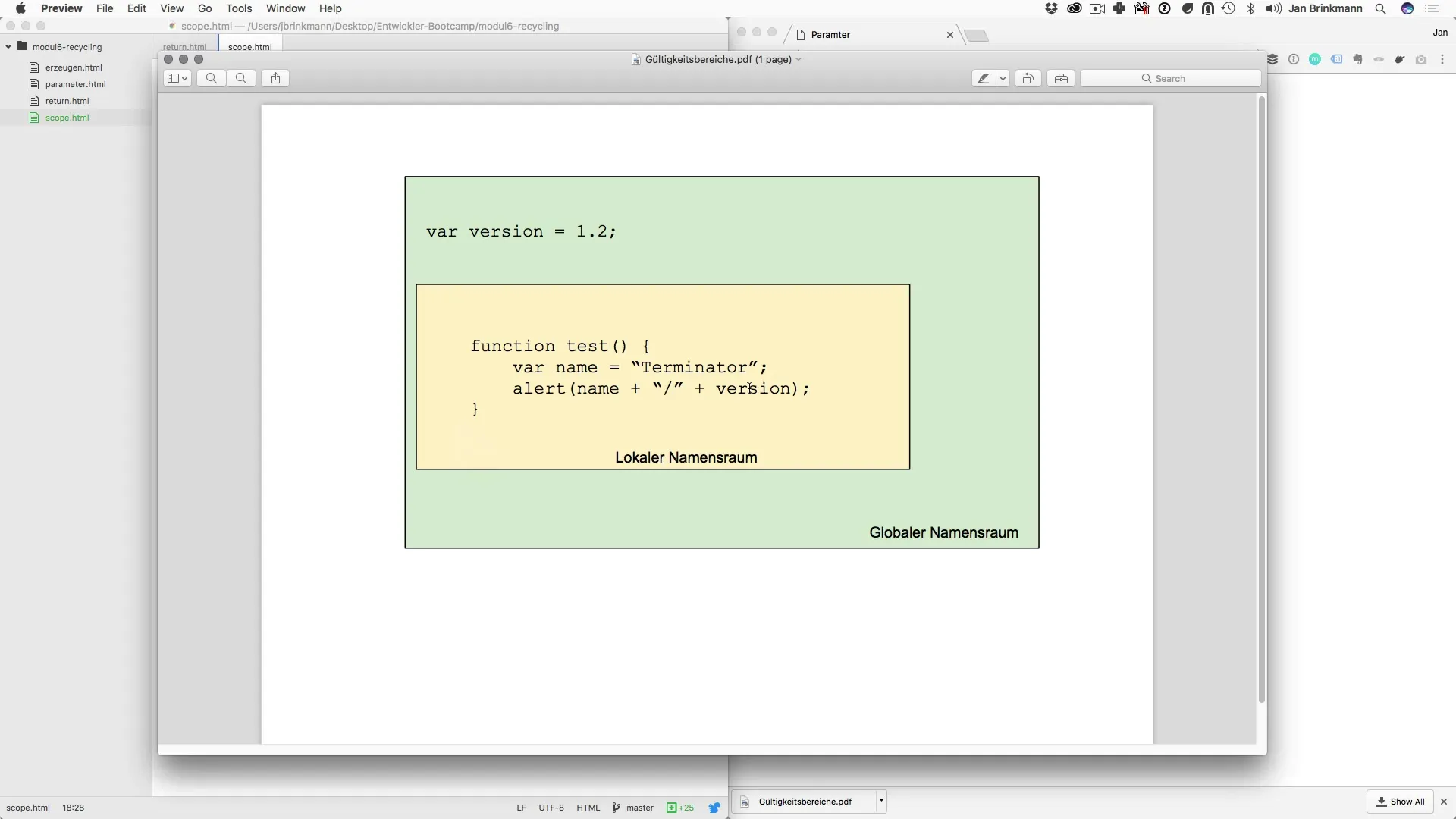
Summary – Scopes in Programming: A Beginner's Guide
Scopes are an essential part of programming, and a deep understanding of them will help you code more effectively. You have learned how global and local variables work and how they are accessible within their respective areas.
Frequently Asked Questions
What are global variables?Global variables are accessible everywhere in the code and can be used by all functions.
What are local variables?Local variables are only visible within the function in which they are defined.
How can I create a global variable?A global variable is effectively created by declaring it outside of all functions.
Can I access a global variable within a function?Yes, within any function you can access global variables.
Why are local variables important?Local variables help avoid naming conflicts and better structure the code.