This tutorial guides you through the process of building an architecture for a To-do-list using JavaScript and jQuery. You will learn how to create the basic elements for your application using an object-oriented approach. Here, we particularly focus on the structure and organization of the code to create a user-friendly and extendable application.
Key Insights
- You will create a clear structure for your To-do application.
- You will learn how to use jQuery Mobile to design an appealing user interface.
- Using object-oriented programming will make your code more robust and maintainable.
Step-by-Step Guide
1. Create the Header of the To-do List
Start by revising the header of your To-do list. You will insert a button that allows users to add new tasks. Implement this by creating a link that is assigned an ID. You will name this button "new task Button" and give it an appropriate data attribute.
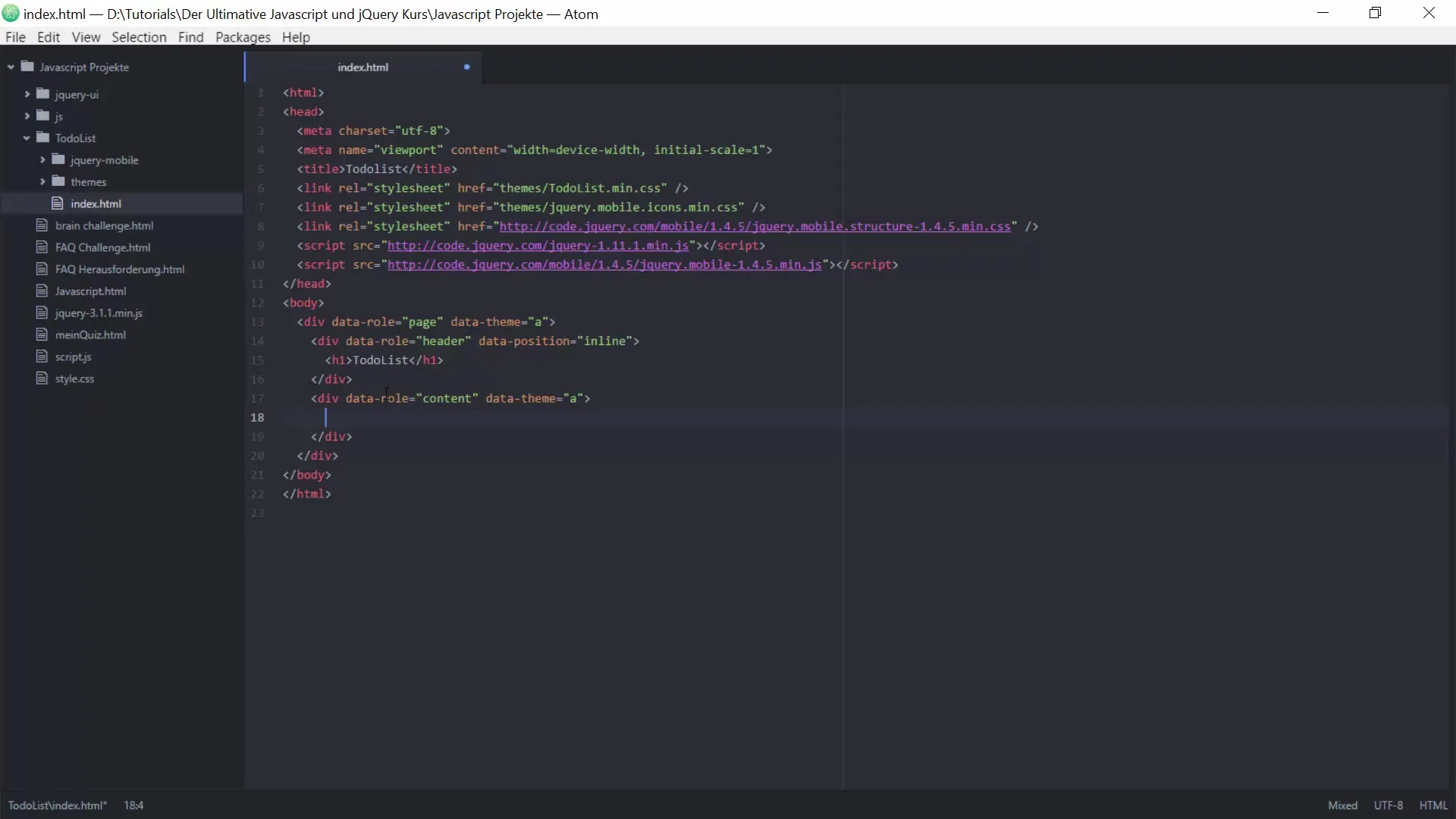
The button behaves like a typical button and changes color when hovered over, thanks to the data-icon that you add. You can choose different icons from the jQuery Mobile Icon catalog to enhance the user interface.
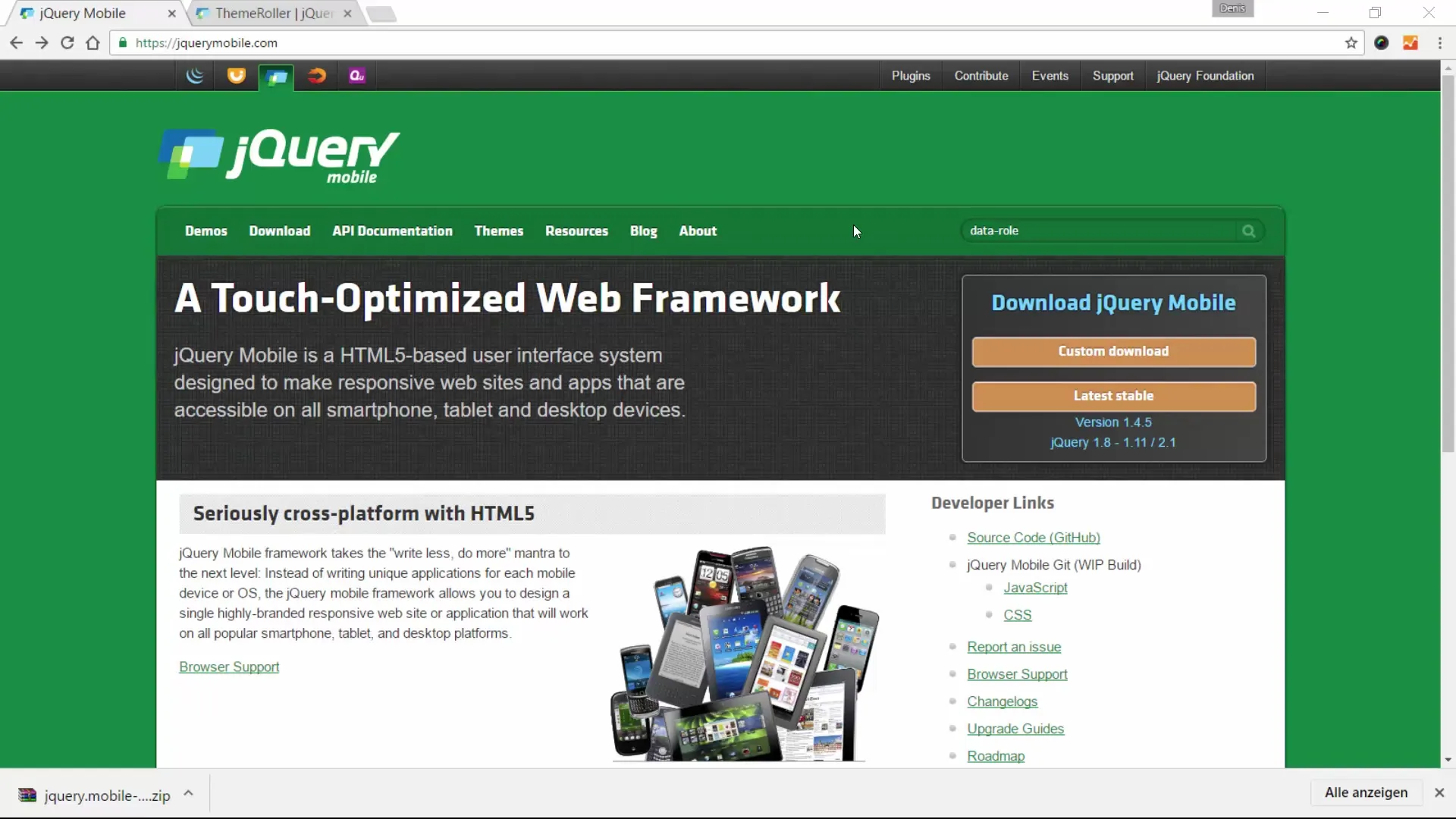
2. Create a List for Tasks
In the next step, you will add an unordered list for the tasks. This list will receive an ID so you can access it later, and a data-role attribute of "listview". This way, jQuery Mobile knows that it is a listed overview.
To fill the list with content, you will create list items. Start with the first list item and insert a link that receives a special class for the title of the task. Additionally, you will add a button that indicates whether the task is completed or not.
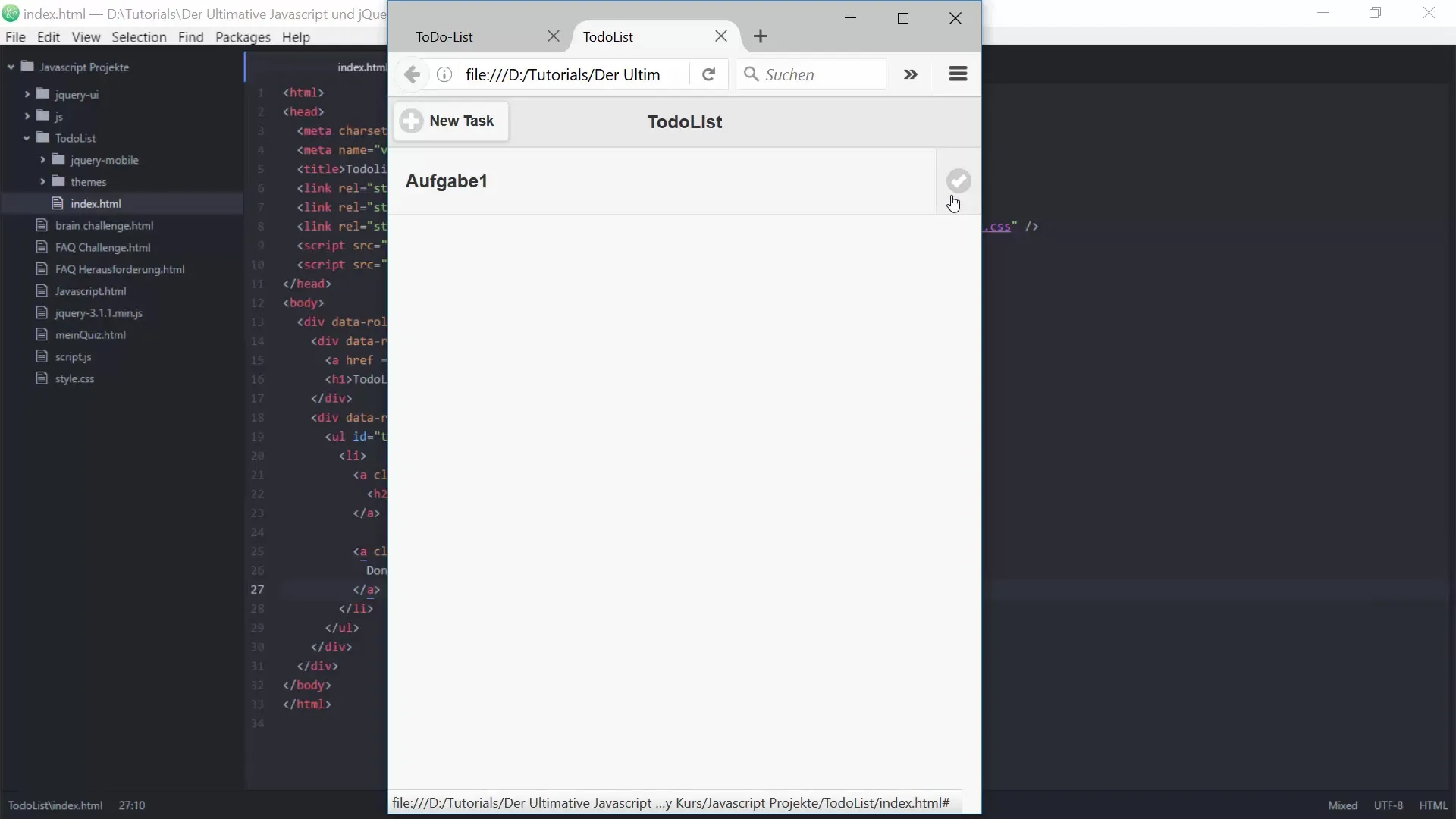
3. Insert Footer with Reset Button
The footer of the application should also contain a reset button. This button allows users to delete all tasks with one click, enhancing usability. Use the data attribute "footer" for this and ensure that the footer is fixed, so it never disappears, regardless of how many tasks are added to the list.
4. Page Structure for Adding and Editing Tasks
Now, define two more pages: one for adding new tasks and one for editing existing tasks. Each of these pages will have a data attribute of type "page," which gives them the correct representation within the jQuery Mobile environment.
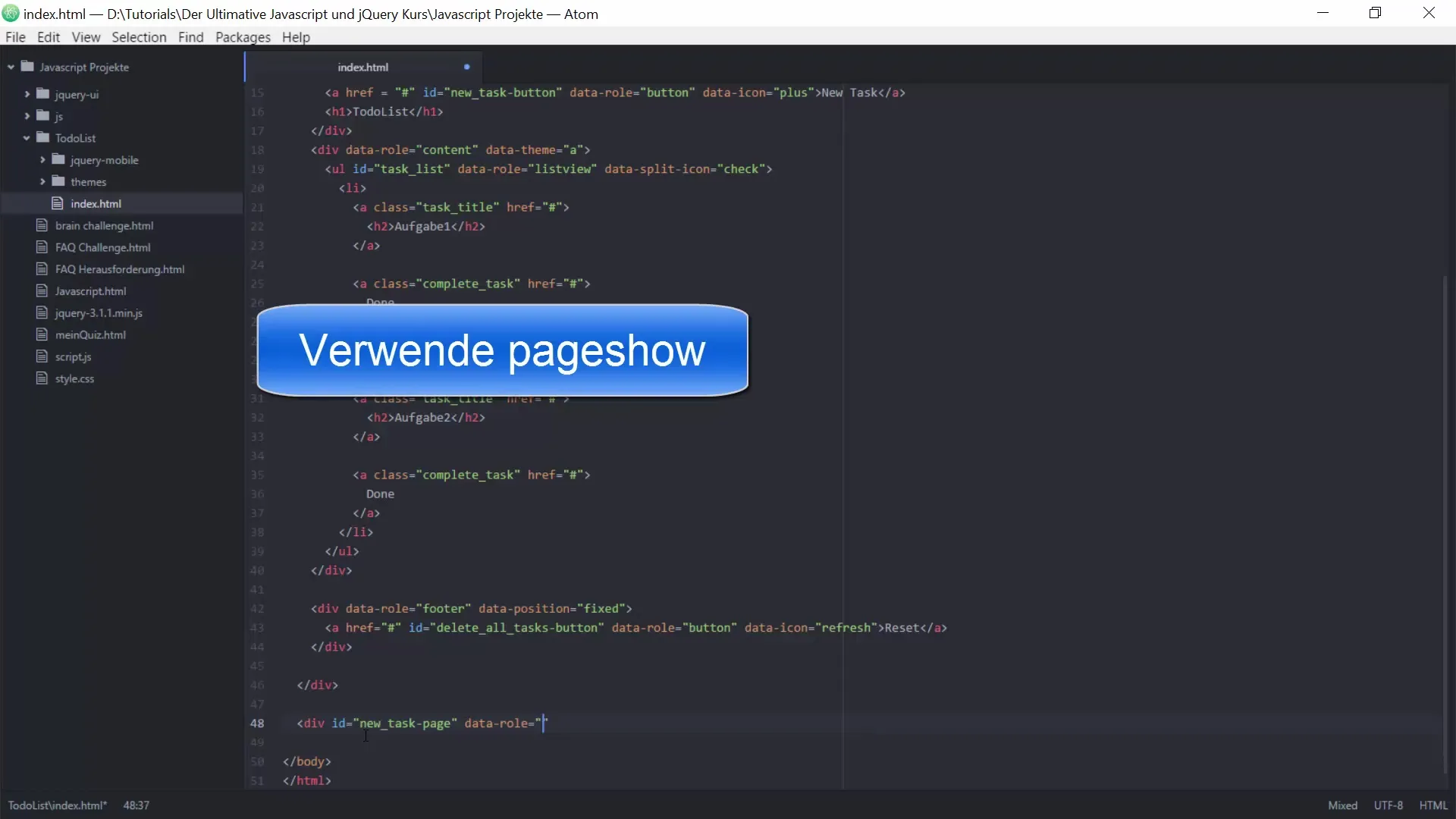
Each of these pages will receive specific attributes that point to the future functionalities that will be implemented here. This ensures that your layout is already prepared for all upcoming features.
5. Set Up JavaScript Architecture
In the next step, you will create the basic structure of your JavaScript files. Start by creating the file “todoList.model.js,” which will serve as the model for your To-do list. In this file, you will define how your data structure should be built and what functions are needed to add, delete, or retrieve tasks.
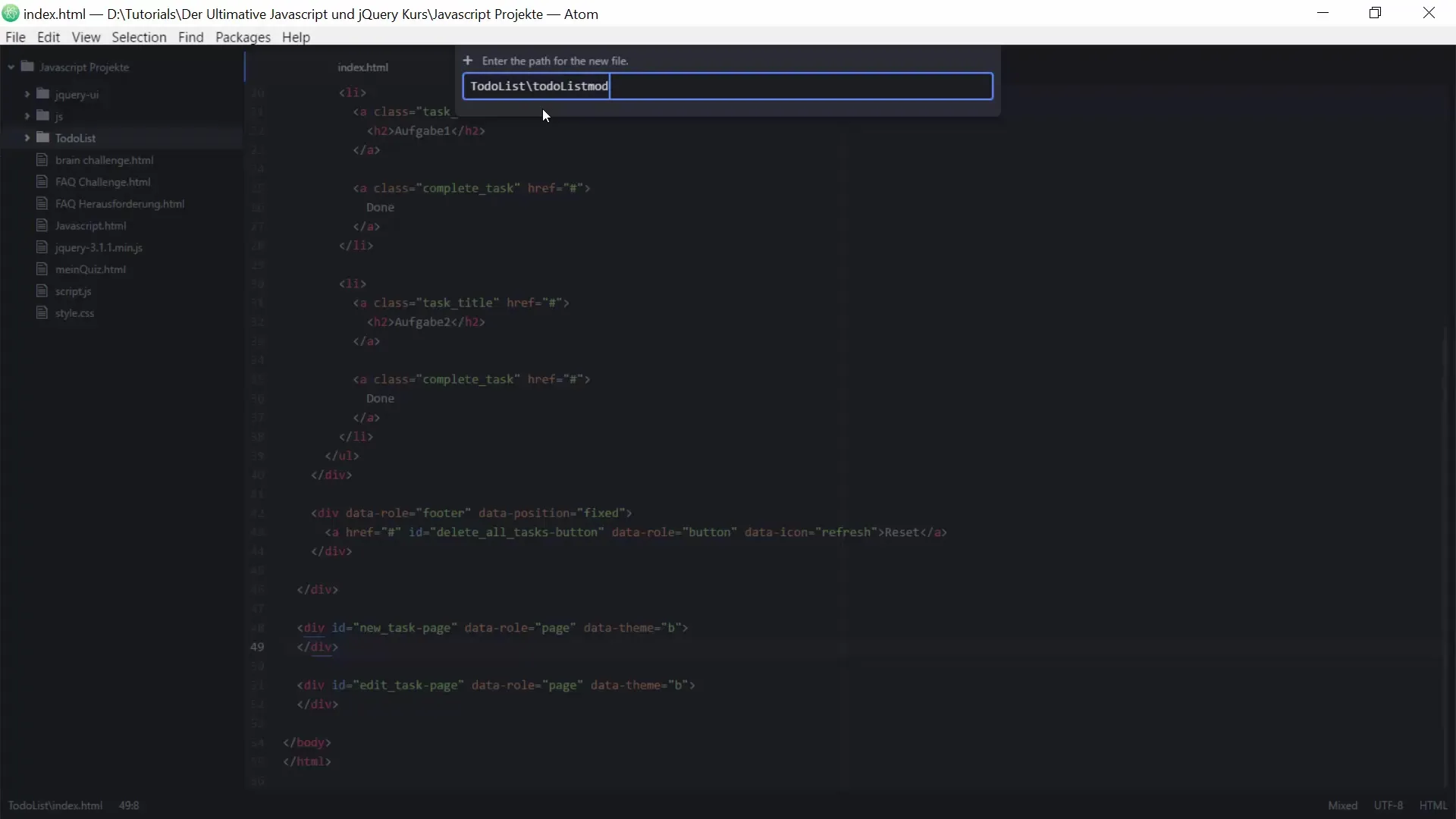
Then create a second file named “todoList.ui.js.” In this file, you will take care of the user interface. Here, you will implement object-oriented approaches that will make your code clearer and more maintainable.
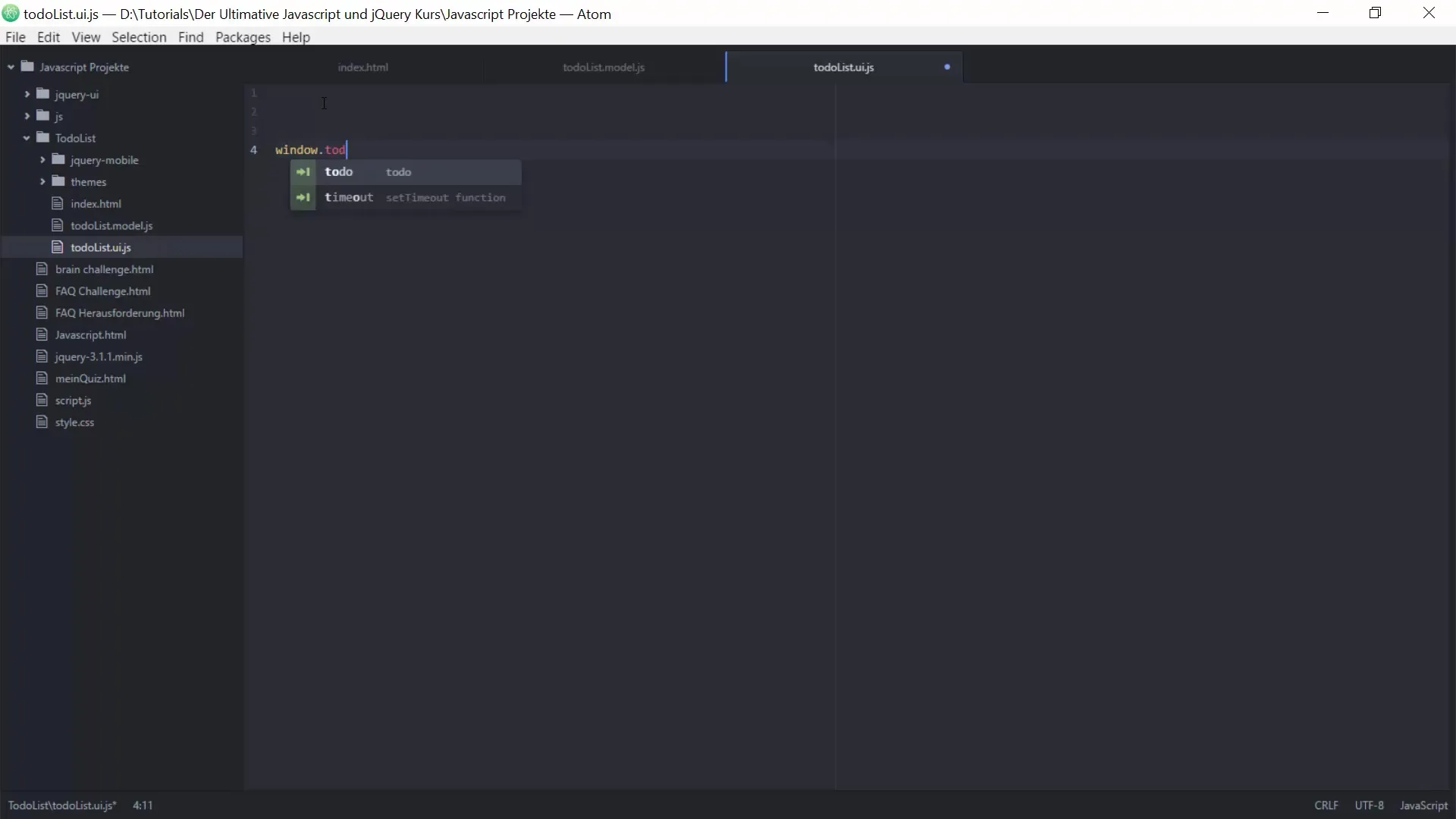
6. Create Basic Functions
Now it's time to create the first functions in your JavaScript code. You will define an addTask function to add a new task, as well as a deleteTask function to remove a task. Additionally, getTask and getTasks are important to specifically retrieve tasks or display all tasks at once.
This structure allows you to clearly separate the logic of the program, which significantly simplifies the maintenance and expansion of the application in the future.
7. Apply Object-Oriented Approaches
Additionally, you will specify that the todoList model should exist to store data in a structured manner. You will check if it already exists and create it again if necessary. This way, you ensure that your code becomes more robust and better organized.
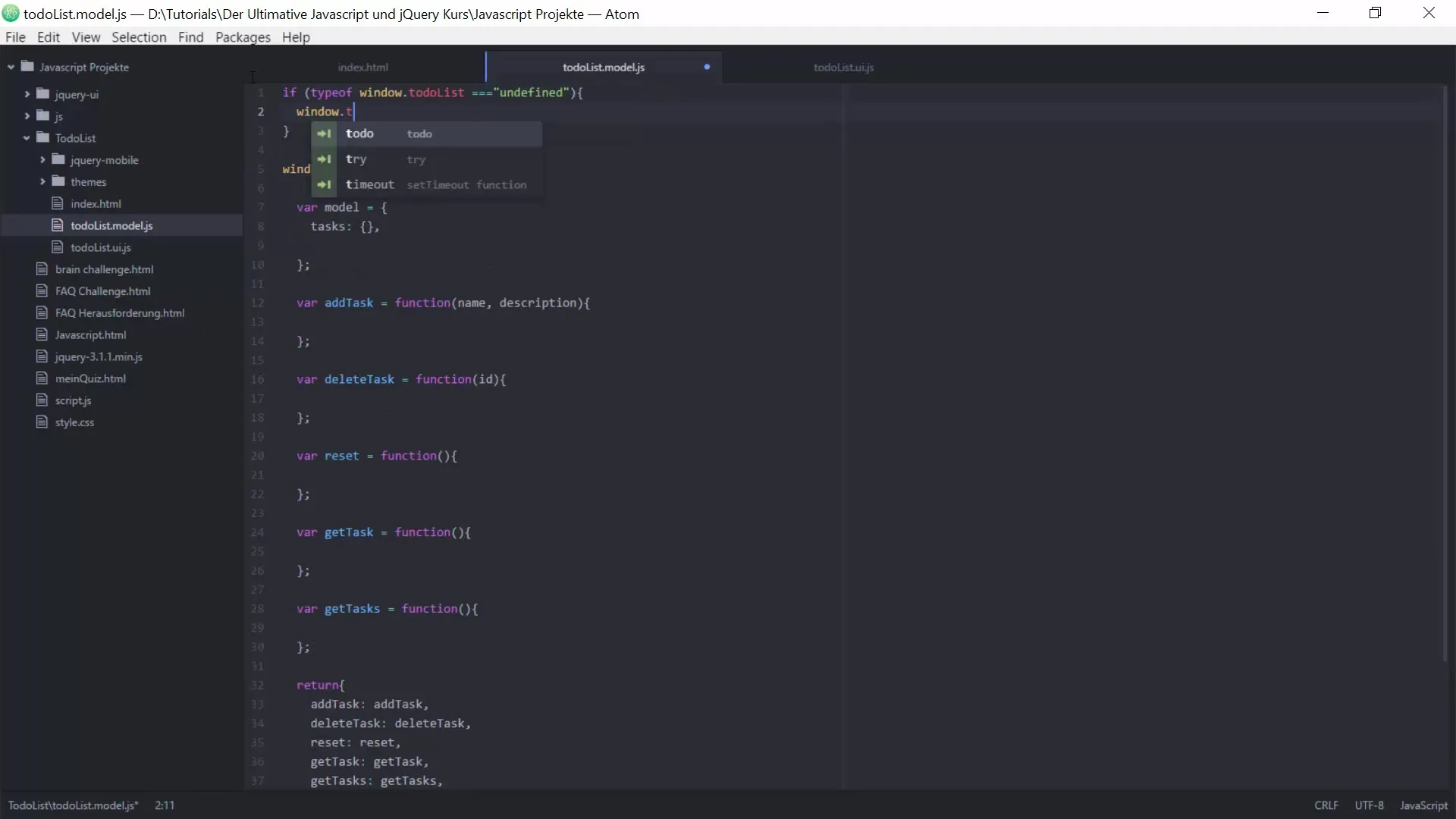
Summary – Architecture for a To-do List in JavaScript and jQuery
In this tutorial, you were able to learn how to build a structured architecture for a To-do list in JavaScript and jQuery Mobile. You established the foundations for the user interface and the necessary functionality to manage tasks effectively.
Frequently Asked Questions
How do I add new tasks?To add new tasks, use the addTask function in your JavaScript logic.
What should I do if I want to delete a task?Use the deleteTask function and pass the ID of the task you want to delete.
How do I make my application responsive?Use the features of jQuery Mobile to ensure that your application adapts to different screen sizes.
In what format can I store my data?You can use JSON or similar data structures to store and retrieve your tasks efficiently.