Arrays are a central concept in programming, especially in JavaScript. They provide you with the ability to store multiple values in a single variable, which is efficient and makes sense for organizing and managing data. In this Tutorial, you will learn how to declare, initialize, and use arrays in JavaScript.
Key Insights
- Arrays are variables that can store multiple values.
- There are several ways to create and work with arrays.
- Accessing elements in an array is done through indexes, starting at zero.
Step-by-Step Guide
Basics of Arrays
To create an array in JavaScript, you need a variable name and the method new Array(). You can declare and initialize your first array in one line of code.
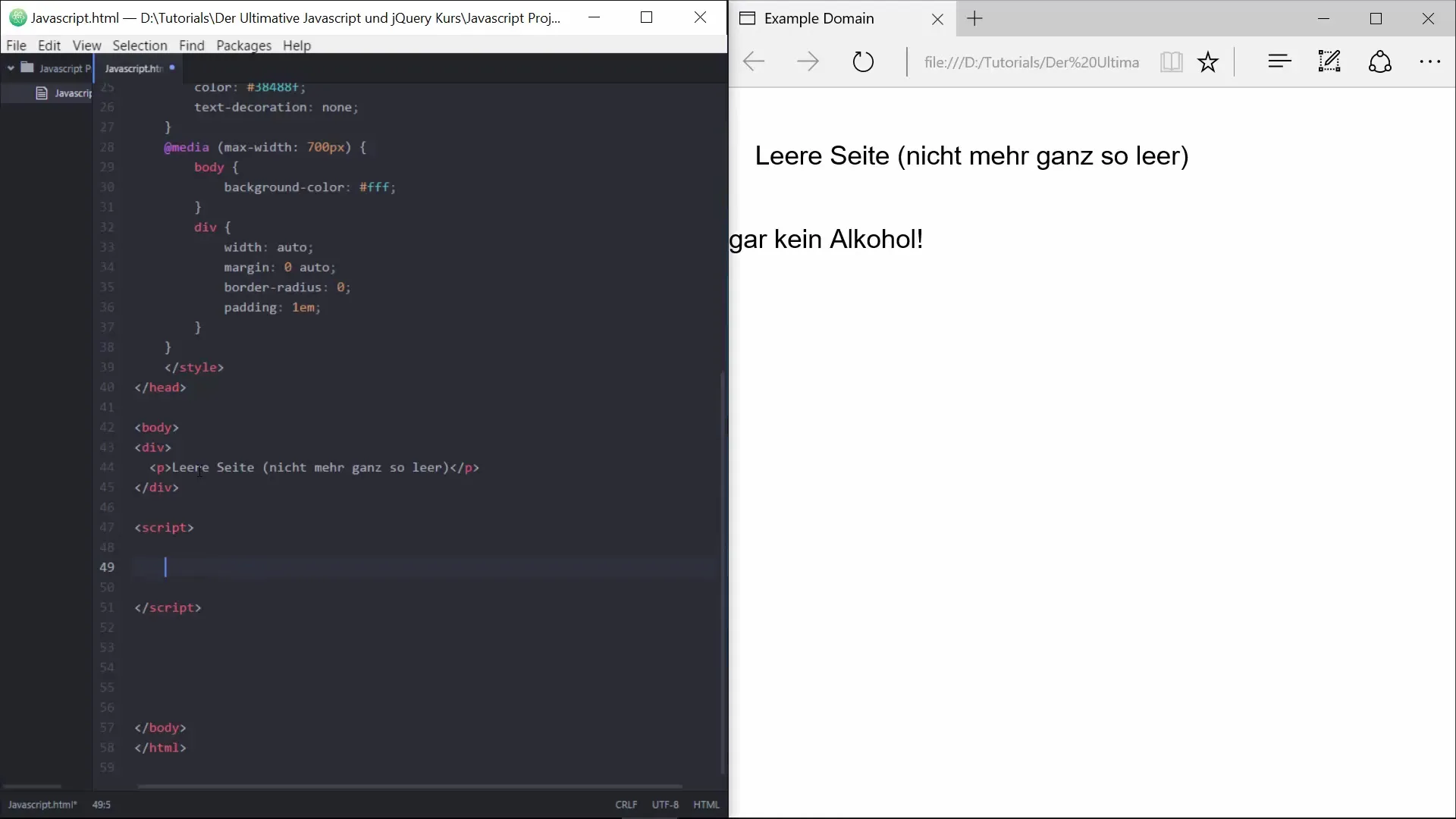
Here’s a simple example of how you can create a number array. Let’s call this array zahlenarray. After initializing the variable with new Array(), you can add values like the numbers from 0 to 9.
Creating Text Arrays
Similar to numbers, you can also store texts in an array. You create a new array for names by again using new Array() and then adding some entries. This is a simple way to store multiple names in a variable.
Once you have made the entries, you can access them. For example, you can output the first name in the array by using the respective index.
This index is crucial, as arrays in JavaScript are zero-based. This means the first entry in the array has index 0. So if you retrieve the name at position 1, you get the second name.
Dynamic Arrays
Please note that you can also declare arrays without a fixed number of values. If you are not sure how many values your array will have, you can simply create an empty array.
You then add values flexibly over time. Suppose you want to create an array for roommates; you can start by leaving the array empty and later add the roommates.
Accessing Array Content
To access or output the content of your array, you use the method document.write(). If you want to output the third element of your mitbewohner array, you specify the corresponding index.
If you want to see the entire content of your array, you can simply use the array name without an index. This gives you an overview of all contained values.
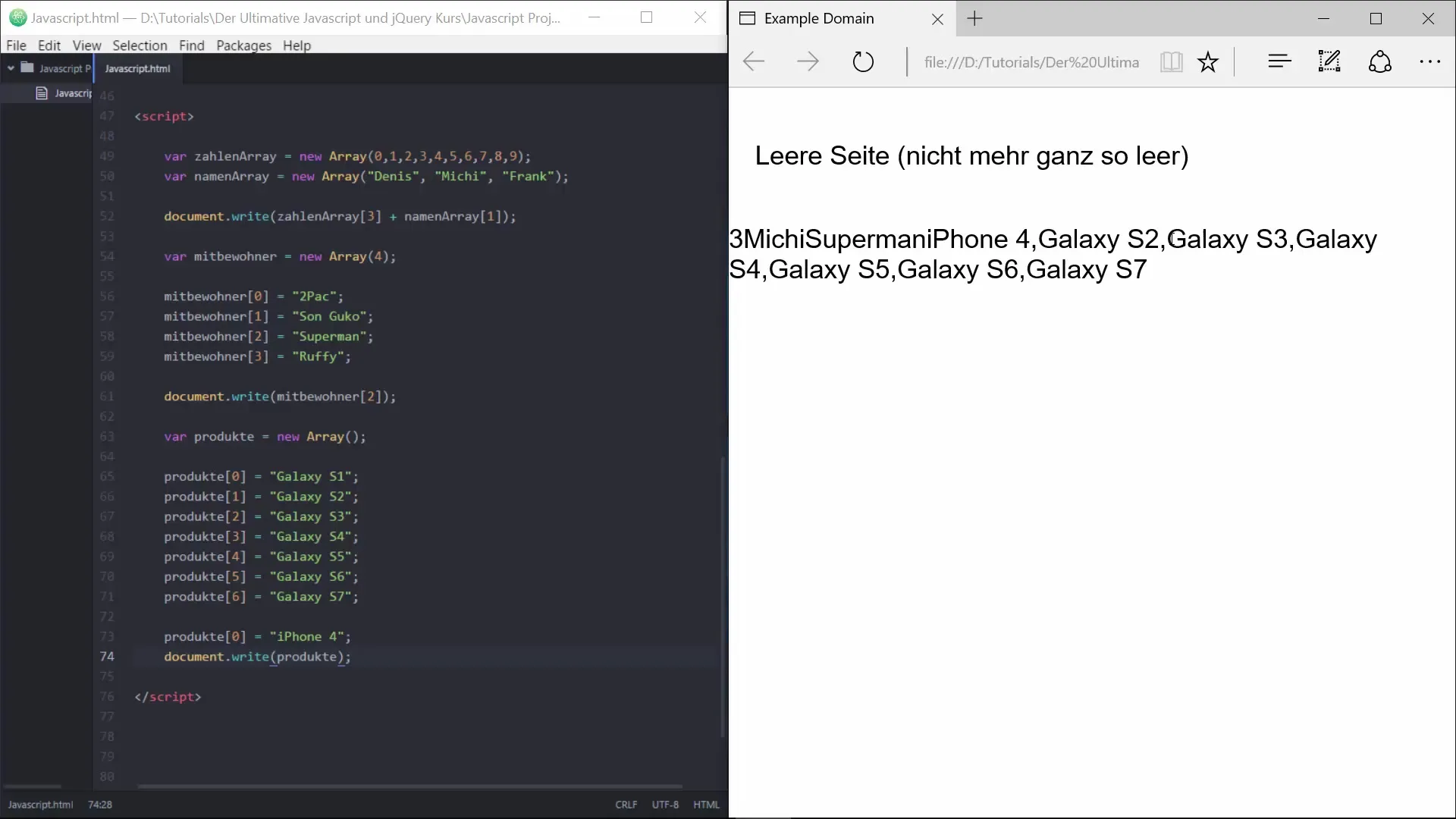
The Flexibility of Arrays
Another advantage of using arrays is flexibility. You can update or replace the values in your array at any time, easily through the index. So, for example, if you want to change an existing value in the array, you can do this by assigning the new value.
After updating, you can output the entire array again to ensure that the changes were successful.
Summary – Arrays in JavaScript: A Comprehensive Guide
Arrays are a powerful tool in JavaScript for managing data in an organized and efficient manner. You have learned how to create arrays, add values, access them, and update them. Understanding arrays is fundamental to your journey into programming as they form the basis of many larger and more complex data structures.
Frequently Asked Questions
How do you create an array in JavaScript?You can create an array in JavaScript either with new Array() or with square brackets [].
Can I fill an array with different data types?Yes, an array can store values of different types, including numbers, strings, and objects.
How do I access elements in an array?You can access array elements by their index, with the first index being 0.
Can I change the size of an array dynamically?Yes, arrays in JavaScript are dynamic; you can add or remove elements without needing to set the size in advance.