Variables in JavaScript are essential for storing and processing data. It is important to understand where these variables are valid and which areas of your code they can access. Given the different types of variables – global and local – understanding the “scope” is fundamental. Therefore, let's dive in and find out what global and local variables are all about.
Key insights
- Global variables are accessible throughout the code, while local variables are only visible within their function.
- Defining a variable outside of a function makes it global, while defining it within a function makes it local.
- For a clear and maintainable structure of the code, it is beneficial to declare global variables at the beginning of the script.
Step-by-Step Guide
Defining Variables and Their Visibility
In the first step, we will look at how you can create a variable and what its visibility means. When you declare a variable outside of any function, as shown in the following example, it is global.
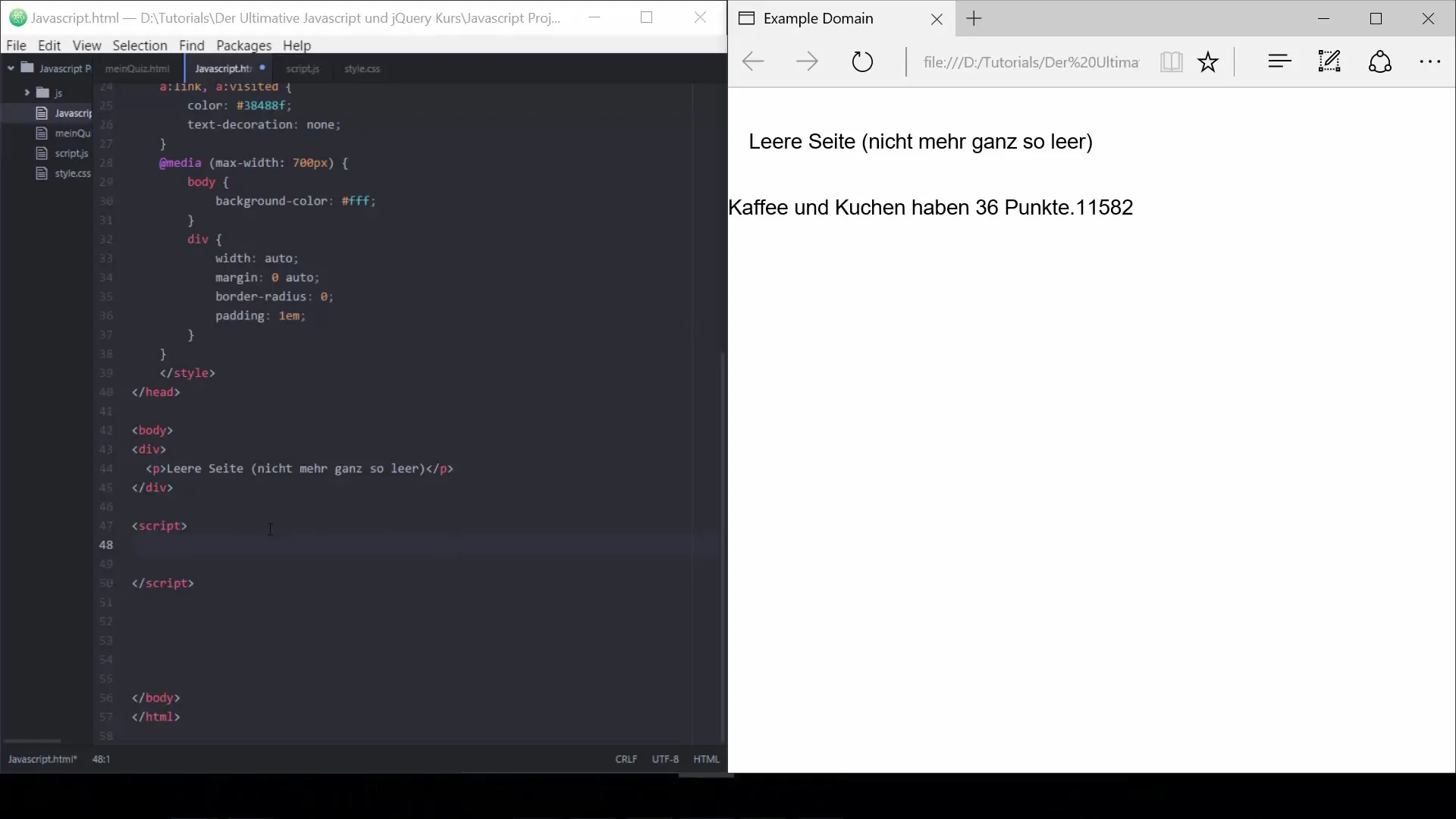
Here we create a global variable called meineVariable.
Creating Functions with Local Variables
Next, we create two functions. We call one function lokaleFunktion, in which we create a local variable. This variable is only visible within the function. Let's define our function.
In the lokaleFunktion, you create a local variable lokaleV with the value 5 and log it out.
Calling the Functions
Now we want to call the lokaleFunktion to log out the number 5. After we have invoked the function in the code, the output looks like this:
Testing the Visibility of Local Variables
Now we will try to use the local variable lokaleV in another function. You will find that this does not work. Let's make a call to the andereFunktion and see what happens.
We receive an error because the variable lokaleV is only visible within the lokaleFunktion.
Checking Errors in the Console
To better understand why the error occurs, let's examine the code in the console. By right-clicking and selecting “Inspect,” you can open the DOM Explorer and the console to analyze the error.
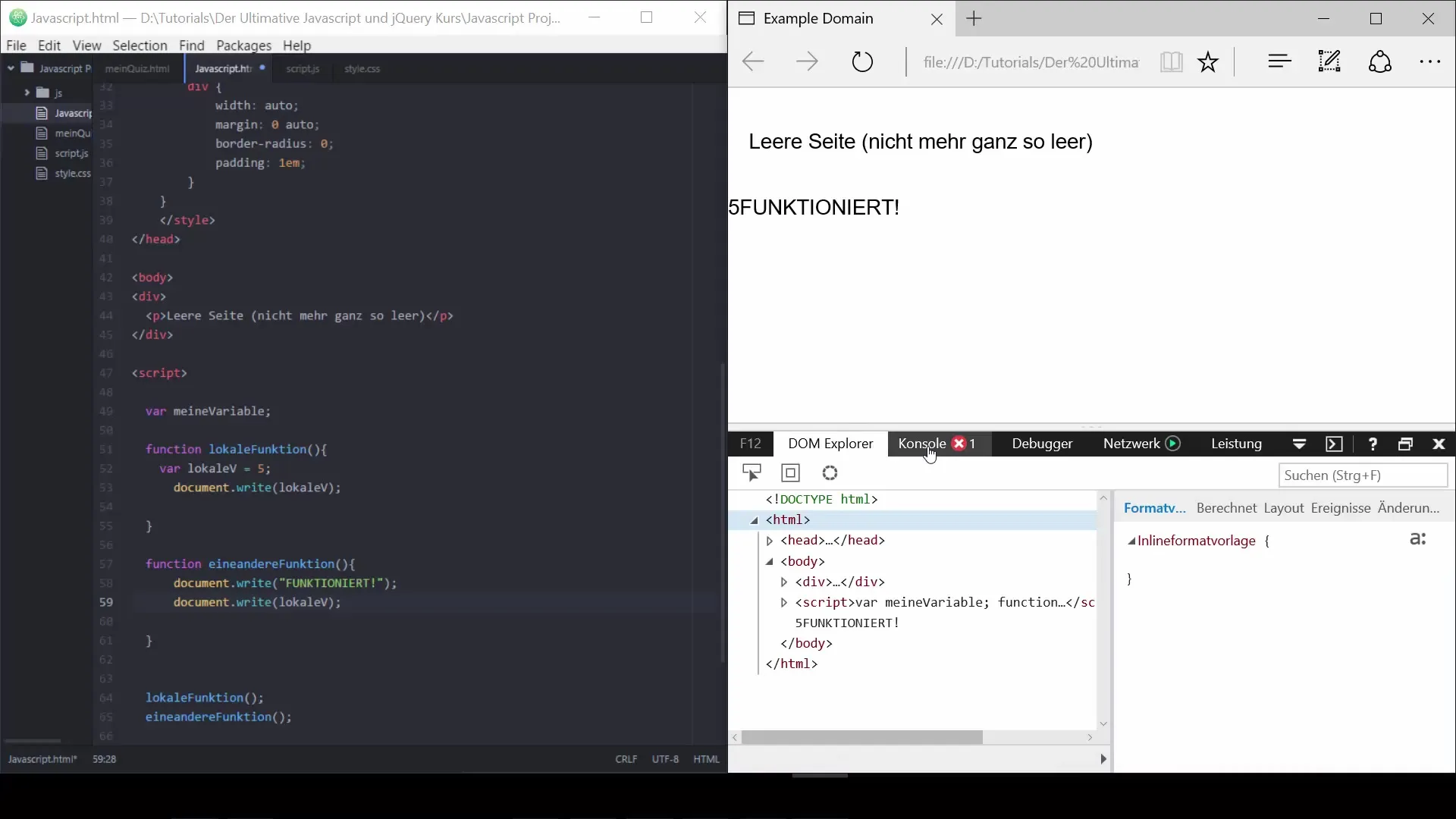
It shows that lokaleV is undefined. This reinforces the assumption that the variable does not exist outside its function.
Defining a Global Variable
Let’s now create a global variable that we can use in both functions. We will call this variable globaleVariable and give it a simple text value.
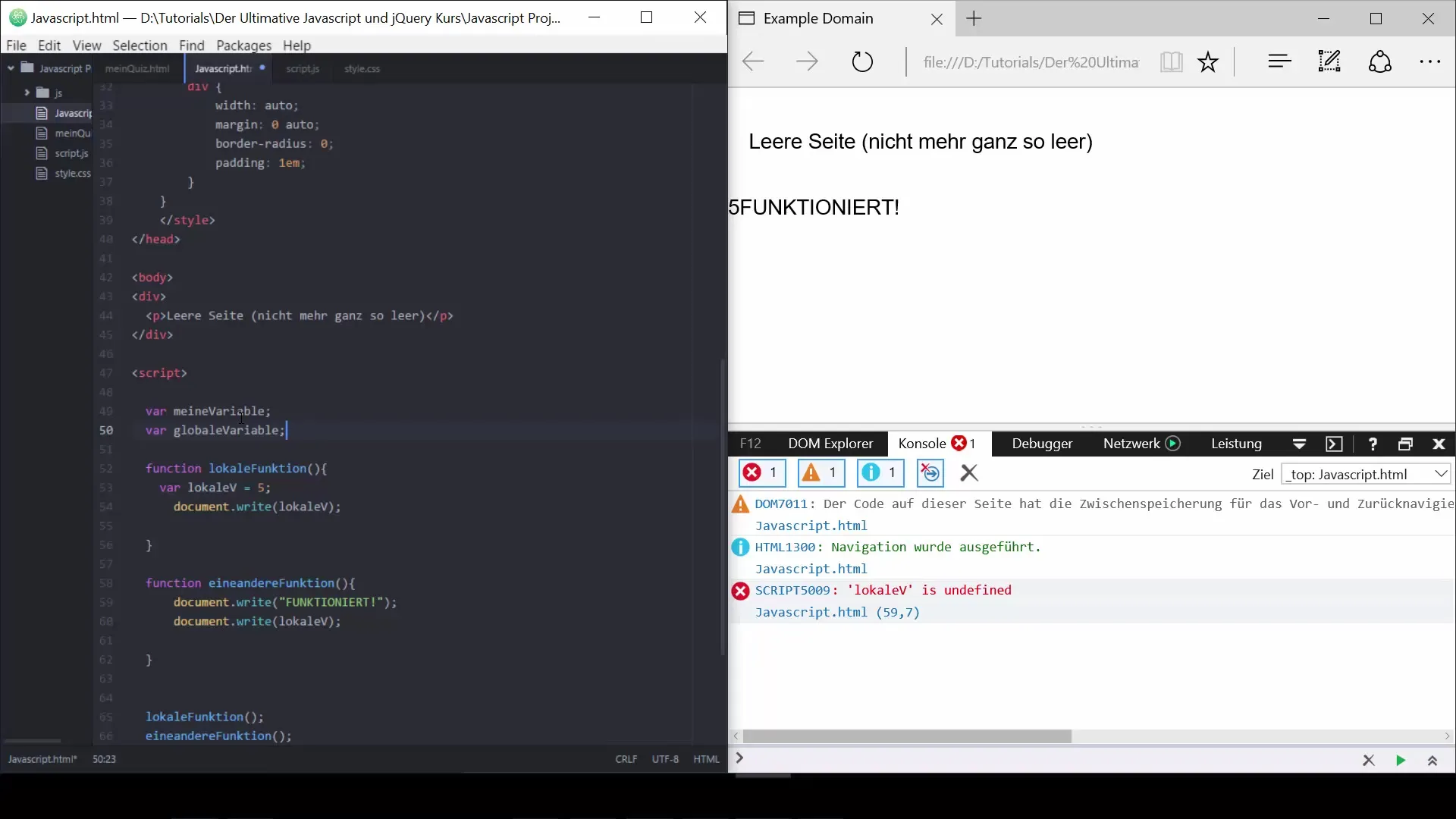
Now this global variable can be called in both functions, and the output will be the same. Let’s test that out.
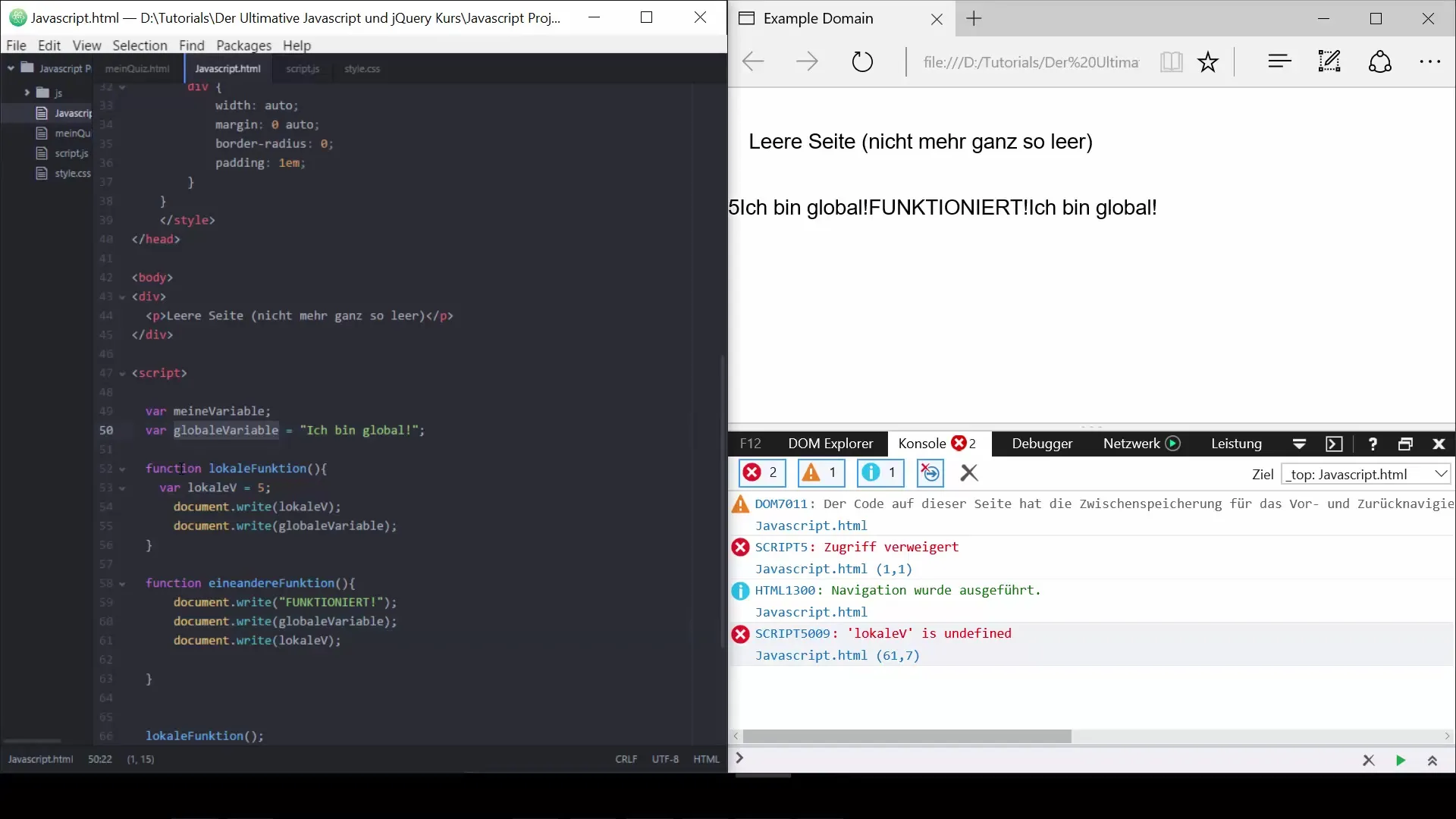
Variable Definition and Call Order
It’s important to note that the order of variable and function definitions is crucial. If you create a global variable below its usage, this will lead to an undefined problem.
This is because the function that requires the global variable calls it before its definition. To avoid this, it is advisable to define global variables at the top of your code.
More About Local Variables and Their Use
Now we will again create a local variable in another function. Here we will call it lokaleVariable and set its value to 12.
We can also use this local variable, but only within its own function, not in others. Using both local variables may require patience and organization in your programs, depending on complexity.
Summary of Concepts
You have now understood the basic concepts of visibility and scope of variables in JavaScript. Global variables are visible to all functions, while local variables only exist within their function. It is advisable to define global variables in a centralized location in the code to improve readability and maintainability.
Summary – The Visibility of Variables in JavaScript
In summary, you have learned that the way you define variables has a direct impact on their visibility and scope. Always pay attention to where you place your variables to avoid errors.
Frequently Asked Questions
What are global variables?Global variables are variables that are declared outside of functions and thus are accessible throughout the entire script.
What are local variables?Local variables are variables that are created within a function and are only visible within that function.
How can I best use global variables?It is recommended to define global variables at the beginning of the script to ensure a clear structure and better maintainability.
Can I use local variables outside of their function?No, local variables are only visible within the function in which they were defined.
How do I deal with undefined errors?Make sure in which order you define variables and functions to ensure that the required variables are defined before their use.