The understanding of the Return-Statement is essential for working with functions in JavaScript and jQuery. Functions not only serve to perform operations but can also return values that can be used in other parts of the code. In this guide, we will delve deeply into the Return-Statement, how it works, and how you can effectively use it in your own code.
Main insights
- The Return-Statement returns a value from a function.
- The function can return various data types, including numbers and text.
- By using Return-Statements, complex calculations and program flows can be better structured.
Step-by-Step Guide
Let's start by creating a simple example to demonstrate how the Return-Statement works in JavaScript.
- Create a function with a Return-Statement First, we will define a function that we will call "derReturn". Inside this function, we will create a simple Return-Statement that returns a text. You can implement this in your JavaScript editor.
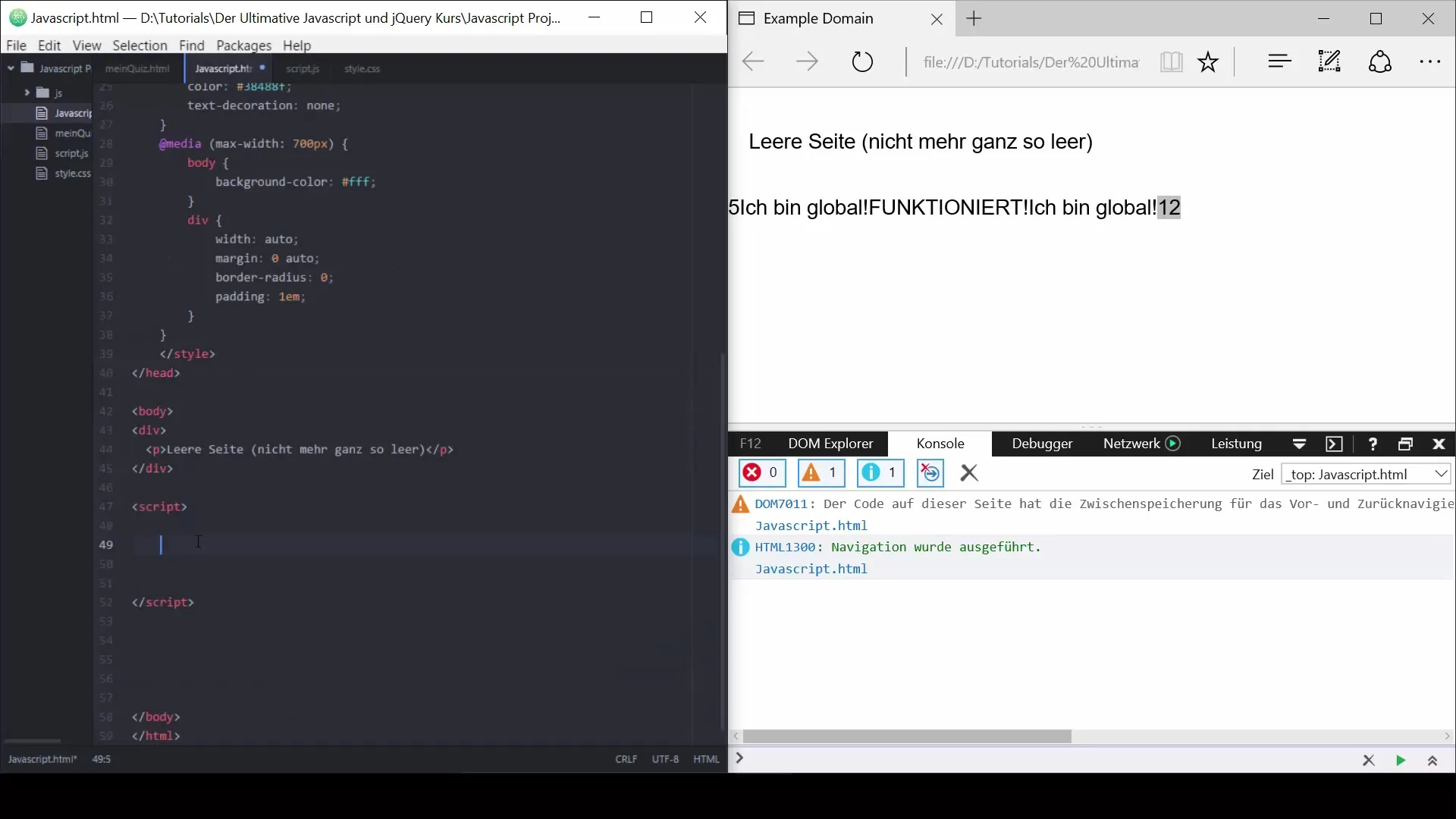
- Call the function Once you have defined the function, you can call it in your code. To display the returned result, use document.write(). This way, you can directly see what the function returns. Don’t forget to save your code after the changes and refresh the page.
- Return values Let’s continue and create a function to add two numbers. The function will accept two parameters – num1 and num2. With the Return-Statement, we will return the sum of these two numbers. You should ensure that you call the function correctly and insert the arguments.
- Error handling When passing numbers, ensure that both values are numeric. Otherwise, unexpected results, such as "NaN" (Not a Number), may occur. Check the inputs and include error handling in the function to ensure that users enter the correct values.
- Variables and return values An elegant solution is to store the result of a calculation in a variable before returning it. This improves the readability of your code. You can store the result of the addition in the variable "Ergebnis" and then return this result.
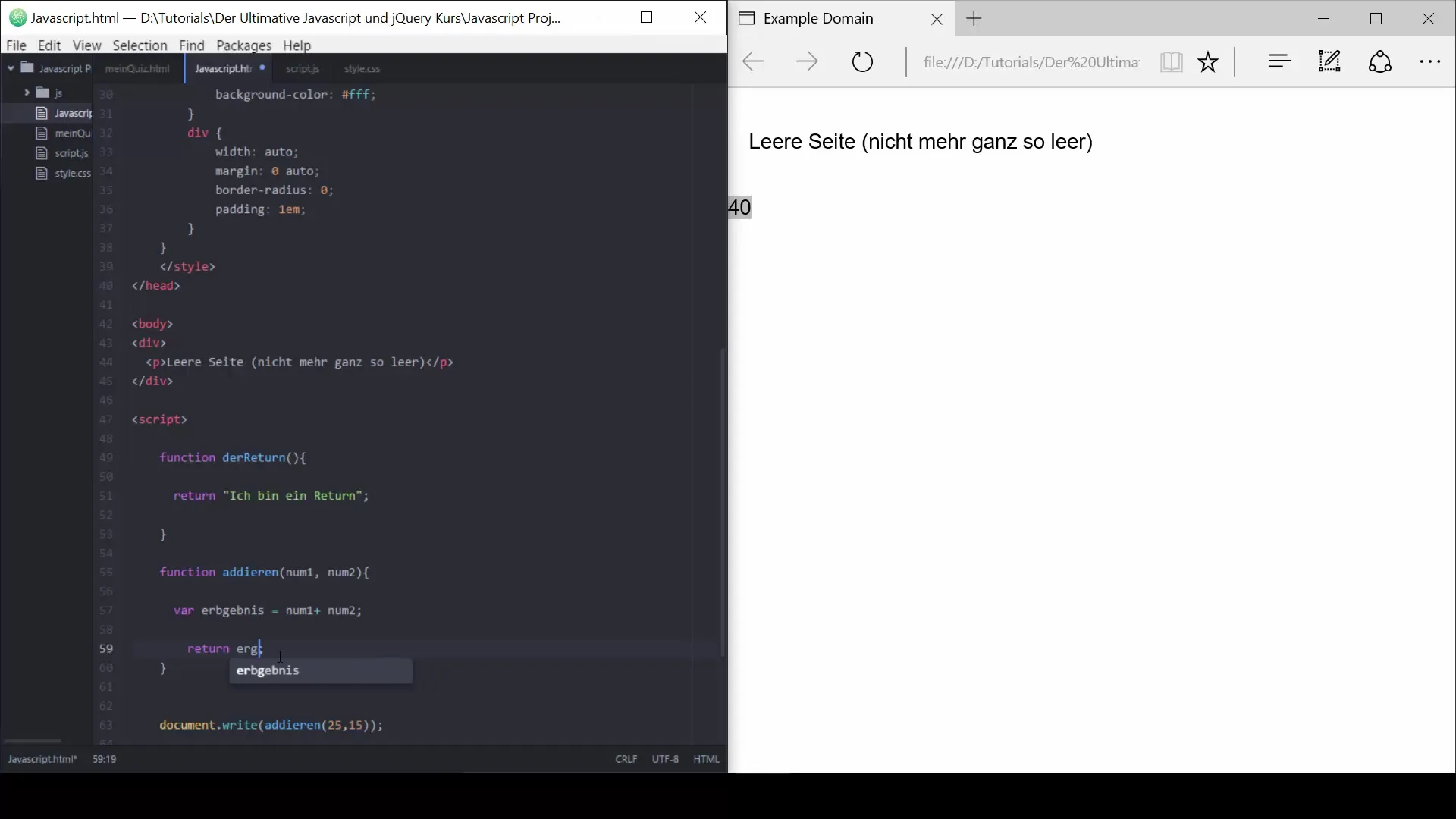
- Creative challenges Now that you have understood the basic concept, it’s time to get creative. Try writing a function that returns a person with a name and an age. Make sure to format the return values well to enhance readability. Ensure that you successfully call the function and display the result on the webpage.
- Additional formatting You can further improve the format of the returned string. By inserting arithmetic in the return value, such as "Alter Jahre alt", you will get a clearer output. This offers an opportunity to test your skills in string manipulation.
Summary - The Return-Statement in JavaScript and jQuery
This comprehensive guide has introduced you to the basics of the Return-Statement in JavaScript. You should now be able to create functions that return values and effectively use those return values in your code.
Frequently Asked Questions
How does the Return-Statement work?The Return-Statement returns the value defined in the function when the function is called.
Can a function return multiple values?A function can only return one value with the Return-Statement, but you can combine multiple values into an array or an object.
What happens if I omit the Return-Statement?If you omit the Return-Statement, the function returns "undefined".
Can I use multiple Return-Statements?Yes, you can use multiple Return-Statements within a function to return different values depending on conditions.
What data types can I use with the Return-Statement?You can return numbers, strings, objects, arrays, and other data types with the Return-Statement.