If you are already working with JavaScript and jQuery, it's time to expand your knowledge with an essential application: creating a To-do-List. The structure and the architecture of your application are especially important in this context. In this guide, you will learn how to build the basic components of your To-do list. We will focus on the model that manages all tasks.
Key insights
- The right data structure is crucial for managing tasks.
- Methods for managing task IDs are necessary to add new tasks and manage existing ones.
- Getters and setters are useful for accessing and modifying the properties of a task element.
Step-by-step guide
Step 1: Create the basic structure of the To-do model
Before you start programming, it's important to understand the structure of your To-do model. This model essentially consists of an object that organizes the To-do elements. So you first create the basic structure for your model.
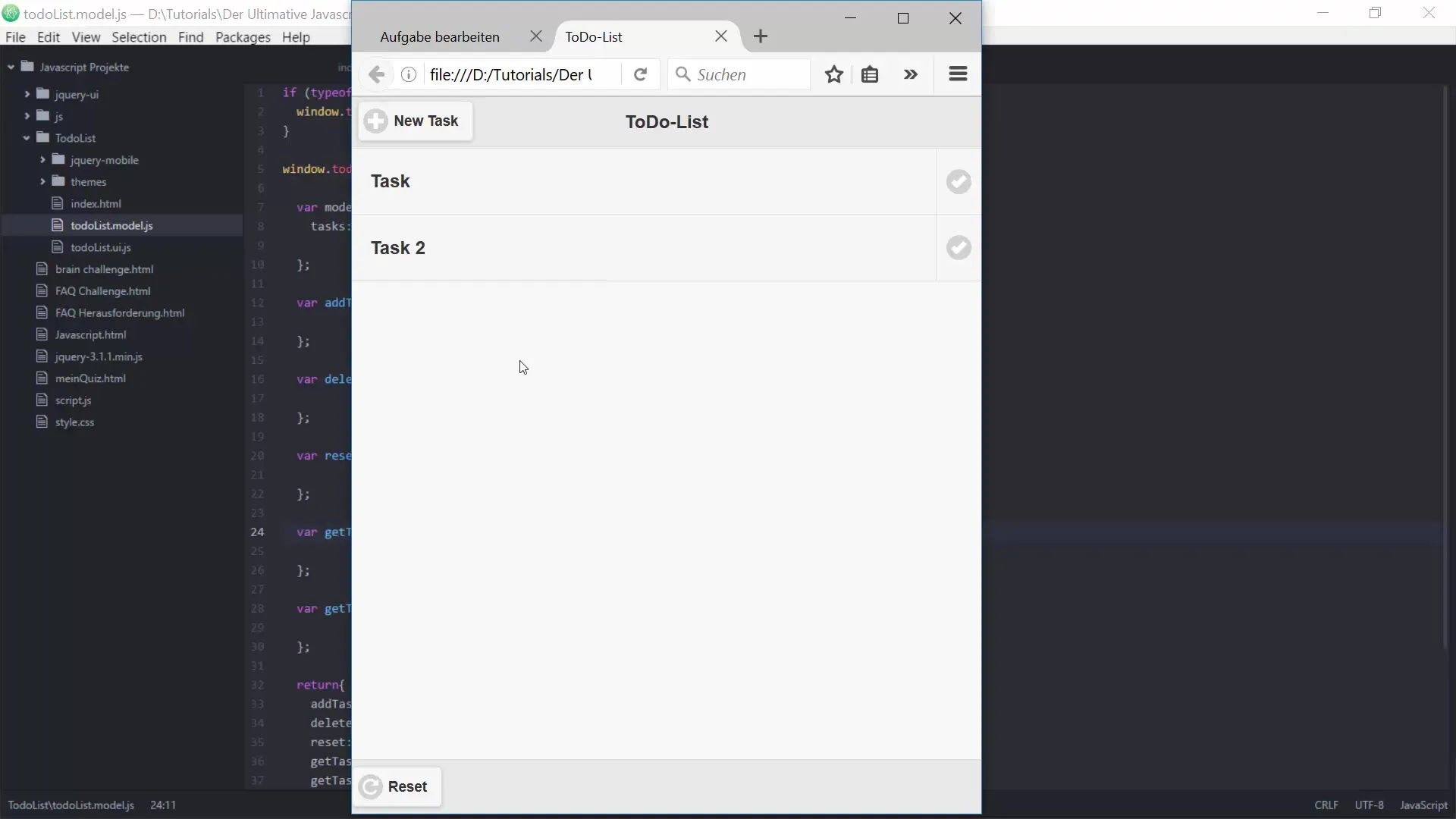
The model will consist of a collection of tasks that we will manage in an array. Each task needs a unique ID that you will store in the variable currentTaskID. This takes into account how many tasks we already have and what ID the next task should receive.
Step 2: Manage the task ID
Add a variable for nextTaskID, which is initially set to 1. This ID will be incremented when a new task is added. This allows you to number new tasks consistently.
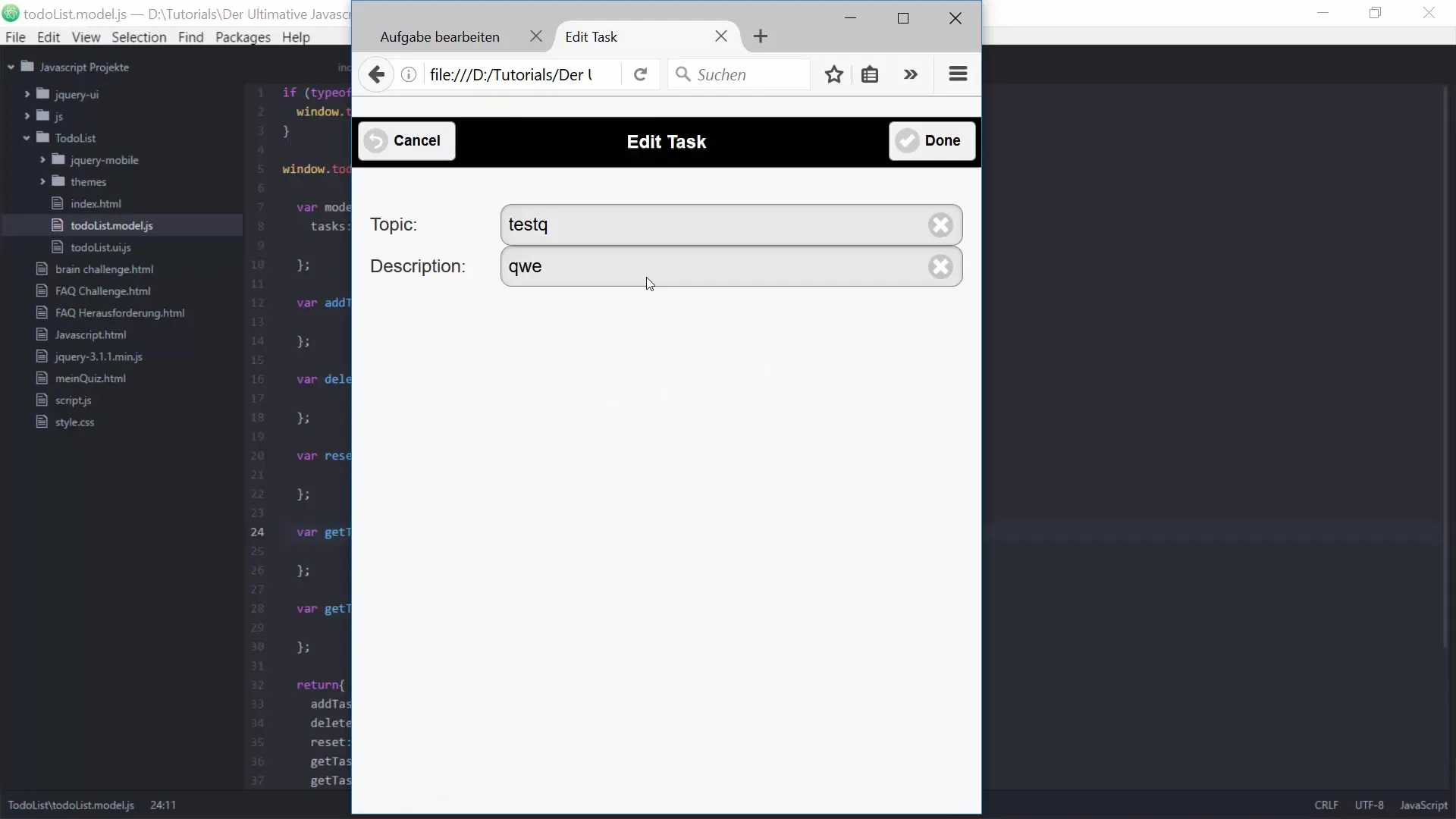
Additionally, you should create getters and setters for the current task ID. The getter allows you to retrieve the current ID, while the setter is used to update the current ID.
Step 3: Define the task structure
The next major element you will need is the structure of a single task. A task typically consists of the following properties: ID, name, and description. You should therefore define the structure of your task object.
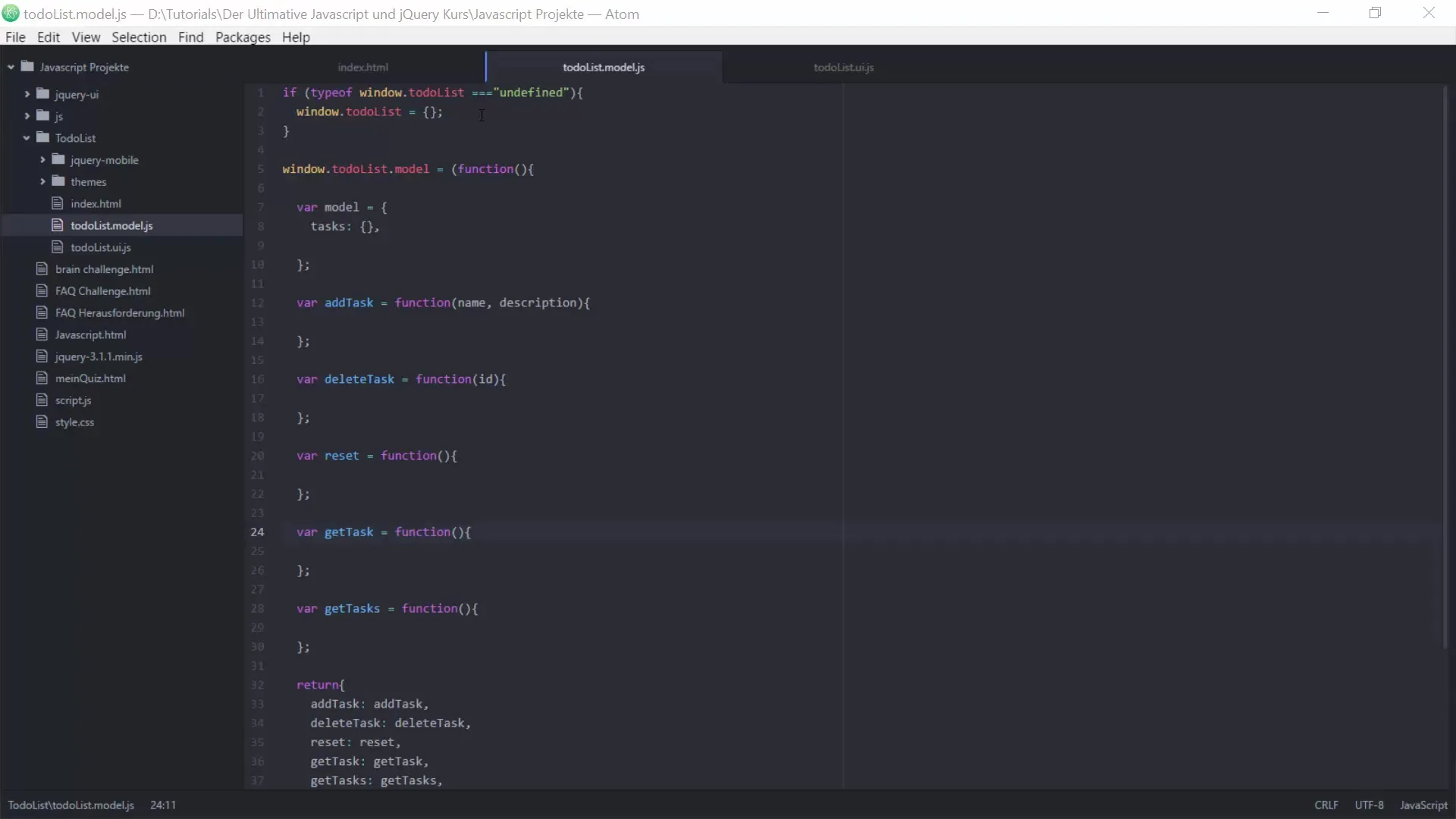
In this context, you are able to create getters and setters for all required elements. With these methods, you can easily query or modify the name or description of a task. Remember to use these properties efficiently.
Step 4: Add tasks
If you want to add a new task, you need a function that creates a new task. Here, you access the nextTaskID and assign this ID to the new task. You then store the new task in your model.
You can achieve this by instantiating a new task object and passing the relevant properties. Ensure that the new task is also correctly added to the modeled task array.
Step 5: Delete tasks
To improve the usability of your application, you also need to be able to delete tasks. Create an appropriate function that removes a specific task based on its ID.
Here, it will first check whether the task actually exists in the model. If so, it will be removed from the array.
Step 6: Return all tasks
A function that returns all tasks is also essential. This method iterates through your task array and returns all tasks.
With this function, you can display all tasks on the user interface, making them easily accessible and significantly improving the user experience.
Step 7: Update tasks
Sometimes you need to update existing tasks. Implement getters and setters for the properties of your tasks to enable this. For example, you can change the name or description of a task at any time.
By incorporating this flexibility, you can develop your To-do list into a sustainable solution.
Step 8: Storing the data
Although we have created the architecture and basic data structure for your To-do list in this phase, the next step is to store the data persistently. This is done with an appropriate storage format like JSON.
By storing the data, you can ensure that users can find their tasks again after reloading the page.
Summary – Architecture and Data Structure for an Effective To-do List
In this guide, you have learned how important architecture and data structure are for a To-do list. You have learned how to define the model for your application, add and remove tasks, and how to store them persistently.
Frequently Asked Questions
What is the main goal of this guide?You will learn how to develop an effective architecture for a To-do list and manage the basic data structure.
How can I add tasks to my list?By creating a function that creates a new task with a unique ID, a name, and a description.
How can I delete tasks?Create a function that removes a task from your model based on its ID.
Do I have to manage the task ID manually?No, there are mechanisms that automatically increment the ID, so you don't have to worry about that.
How can I save my data?You can use JSON to store your tasks so they are available the next time the page loads.