The development of a To-Do-Liste is an excellent project to deepen your knowledge of JavaScript and jQuery. In this section, you will learn how to create a page that allows you to add new tasks. We will focus on the basic design and user interaction.
Key insights
- You will learn how to create a new task page that we can use to input new tasks.
- You will learn how to create and manage forms and input fields with jQuery.
- It will be explained how to bind button events to extend the functionality of your To-Do list.
Step-by-Step Instructions
Step 1: Preparing the new task page
First, you need to establish the structure for your new page. This page will serve as a form to add new tasks. It is important to design this page clearly so that users can quickly recognize where to input information.
To create the basic structure, start with the header. Copy the header from another page that you have already created and paste it into the new task page.
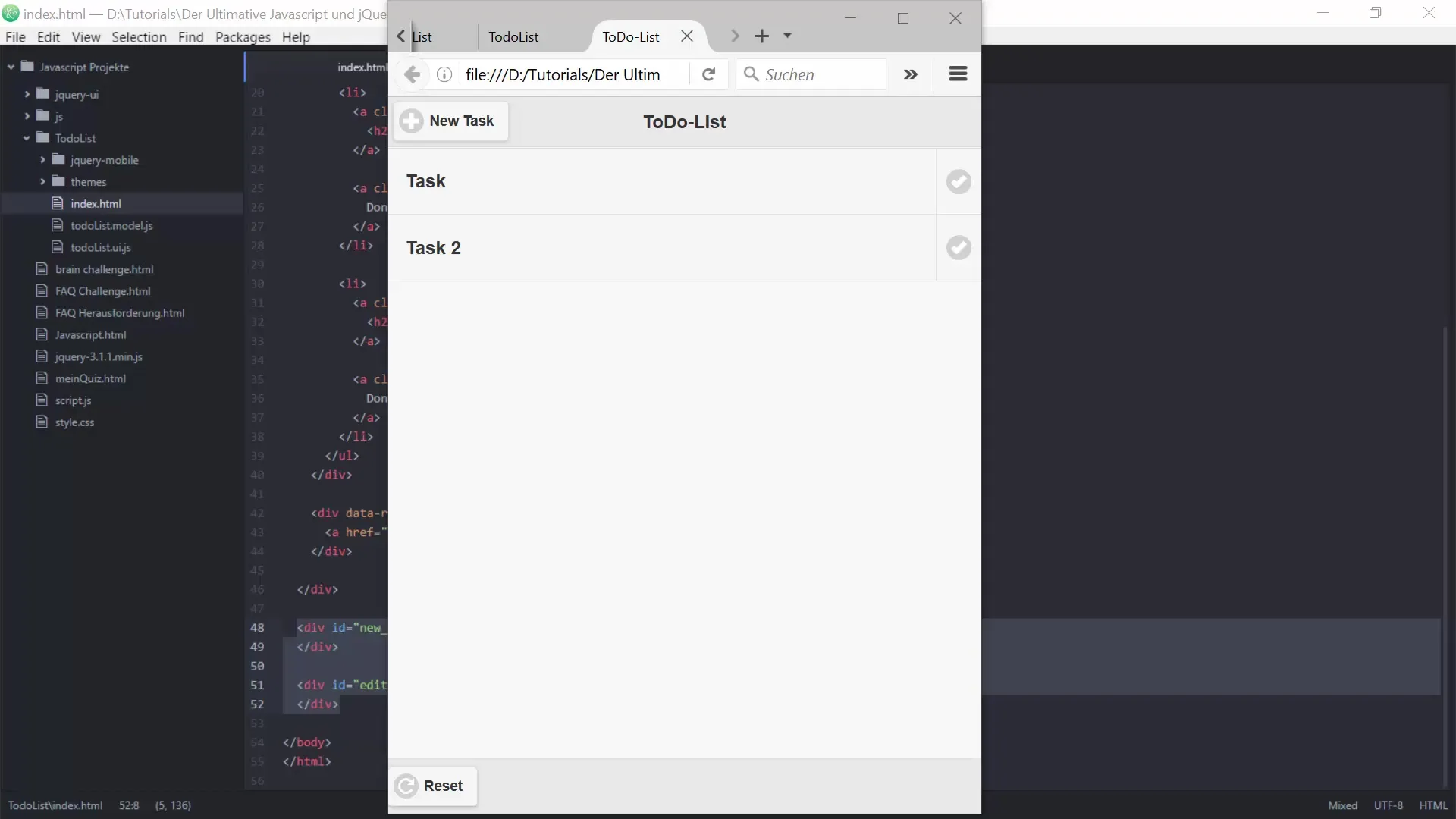
Step 2: Customizing the header
In the header, the button texts need to be adjusted. Instead of "New Task", change the text to "Cancel" to make the functionality clear. Also, add another button element for saving.
Use the following IDs and Data attributes to style the buttons and ensure they are interactive.
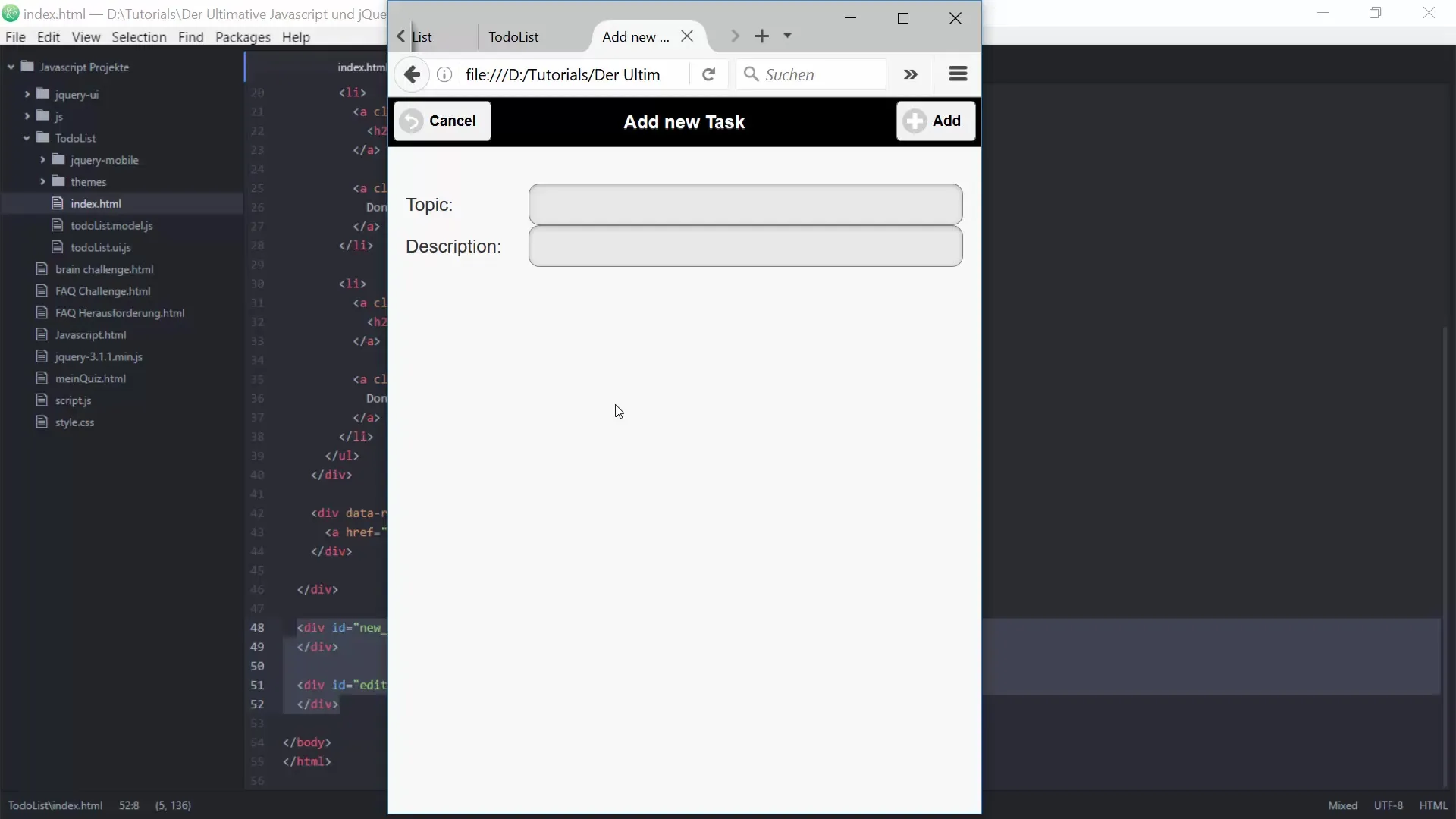
Step 3: Adding heading and content area
Now add a heading “Add New Task”. Then create a content area for the form where users can input the titles and descriptions of their tasks.
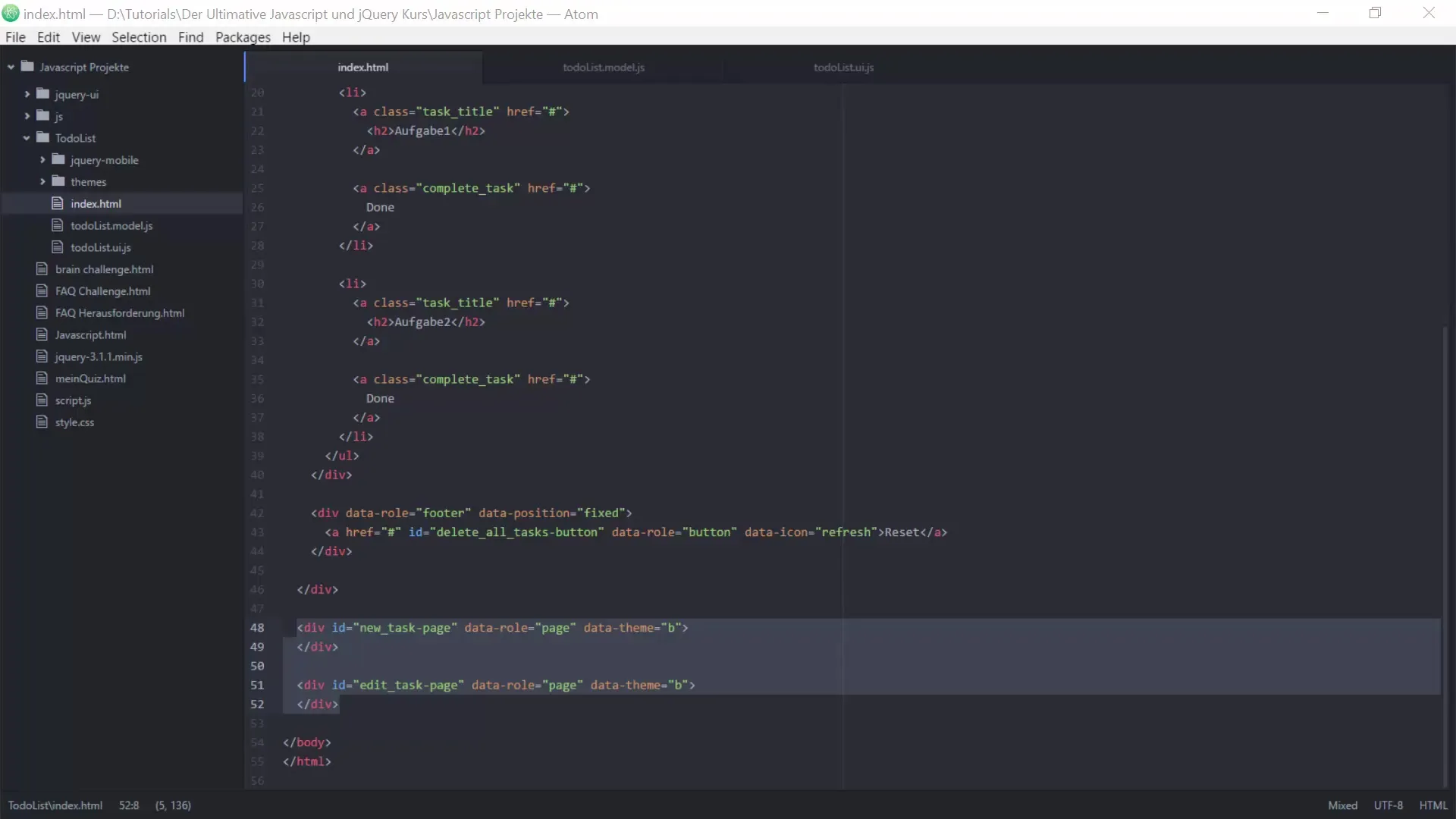
Step 4: Creating the form with input fields
In this step, you will build the form for your tasks. You will need two input fields: one for the task title and one for the description. Don’t forget to add appropriate labels to clearly identify the input fields.
Here you will also define the IDs and names of the input fields to easily query them later in the code.
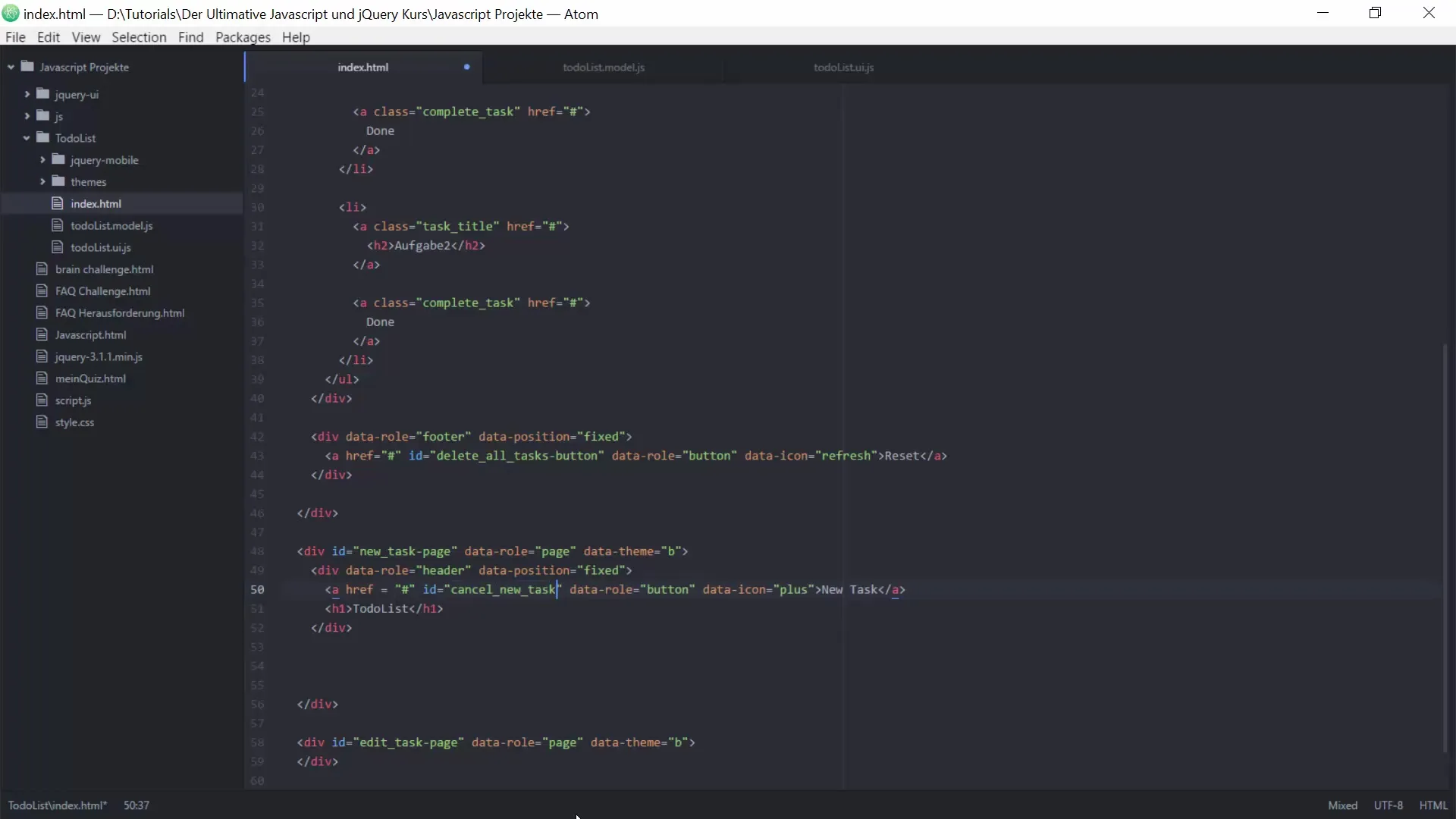
Step 5: Binding button events
Now it’s time to implement the interactions. You need to ensure that the buttons you created also have functionality. You can define the functions for the buttons in the appropriate JavaScript file.
Start with the Cancel button, which should bring the user back to the main page. Use the jQuery event system to bind the functionality.
Step 6: Function to add new tasks
The most crucial part of your page is the function that allows you to add new tasks to the list. You need to create a function that retrieves the input values from the fields when the Save button is clicked and adds them to the existing task list.
Make sure you capture both the title and the description and append them to the To-Do list when clicking on "Add".
Step 7: Transition between pages
To create a seamless user experience, you should implement a transition from the main page to the new task page. This is done with the jQuery Mobile function changePage, which provides a nice animation.
You can customize the type of transition so that the user receives visual feedback when switching between pages.
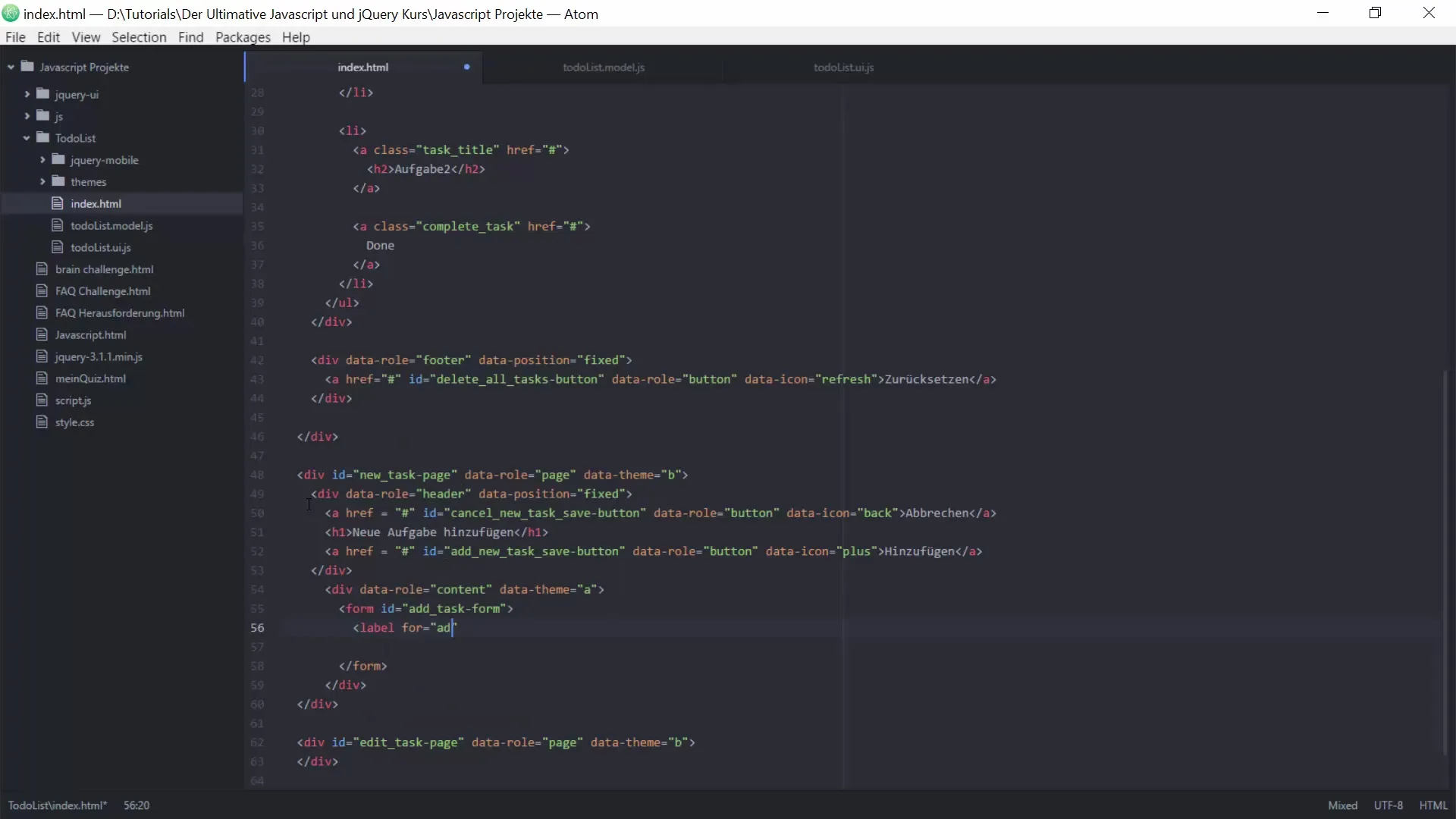
Step 8: Testing functionality
Before you complete the project, you should test all functions. Check if the Cancel button brings the user back to the main page and whether the Save button correctly adds the new tasks. Take notes if anything doesn’t work as expected, so you can fix it later.
Summary – Guide to Creating a To-Do List with JavaScript and jQuery – Adding Tasks and Pages
In this lesson, you learned how to add a new task to the To-Do list. You designed the structure of the page, created the HTML for the form, and configured the interactions with jQuery. The knowledge you have gained here provides a solid foundation to further develop your programming skills.
Frequently Asked Questions
What is jQuery Mobile?jQuery Mobile is a touch-optimized framework that helps developers create mobile websites and applications.
How do I add a form in jQuery?Use the -tag to create a form and use jQuery to process the inputs.
How can I customize page transitions in jQuery Mobile?Use the changePage function and customize the transition parameters like transition.
How can I retrieve the entered data from the form?Use jQuery's.val() method to get the value of the input fields.