You want to create a FAQ page that is not only informative but also interactive and user-friendly? In this guide, you will learn how to implement an engaging FAQ page using HTML, CSS, JavaScript, and jQuery. We will walk through the basic components and show you how to create an accordion for the questions and answers that allows visitors to see only the information that interests them.
Key Insights
- Basics for structuring the FAQ page with HTML.
- Creating interactive elements with JavaScript and jQuery.
- Designing the user interface using CSS.
Step-by-Step Guide
Creating an FAQ page involves several steps from the basic structure to full functionality. Let’s start with the first step.
1. Create Basic Structure
First, you need to create the HTML file for your FAQ page. Open your code editor and create a new file named FAQ_Challenge.html. Start with the basic HTML elements:
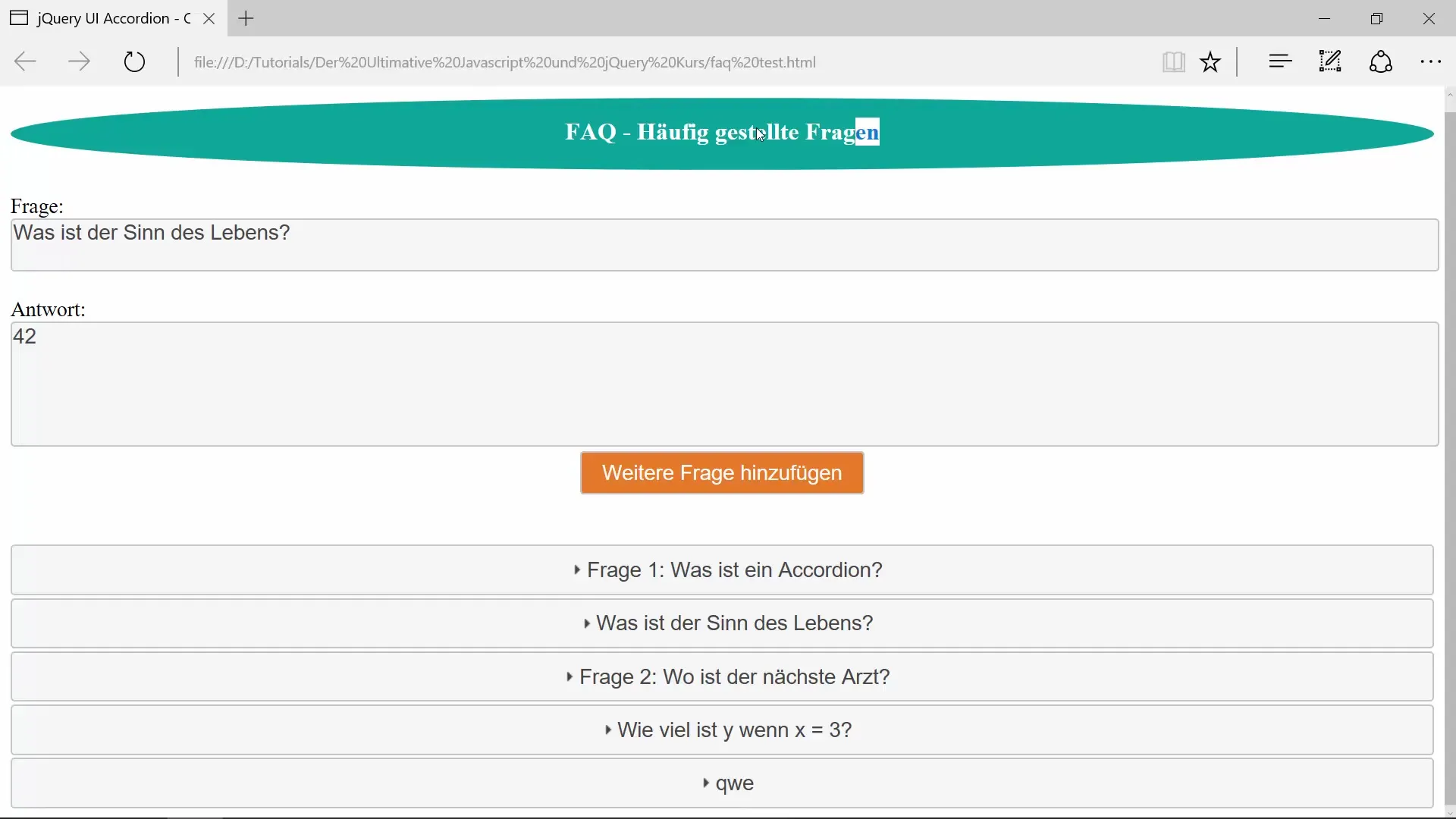
2. Add Textareas for Questions and Answers
Add two textareas: one for the question and one for the answer. These will be labeled to enhance usability.
3. Button to Add Questions
Below the textareas, place a button that can be used to add new questions and answers. Make sure that the button has a clear connection to its function.
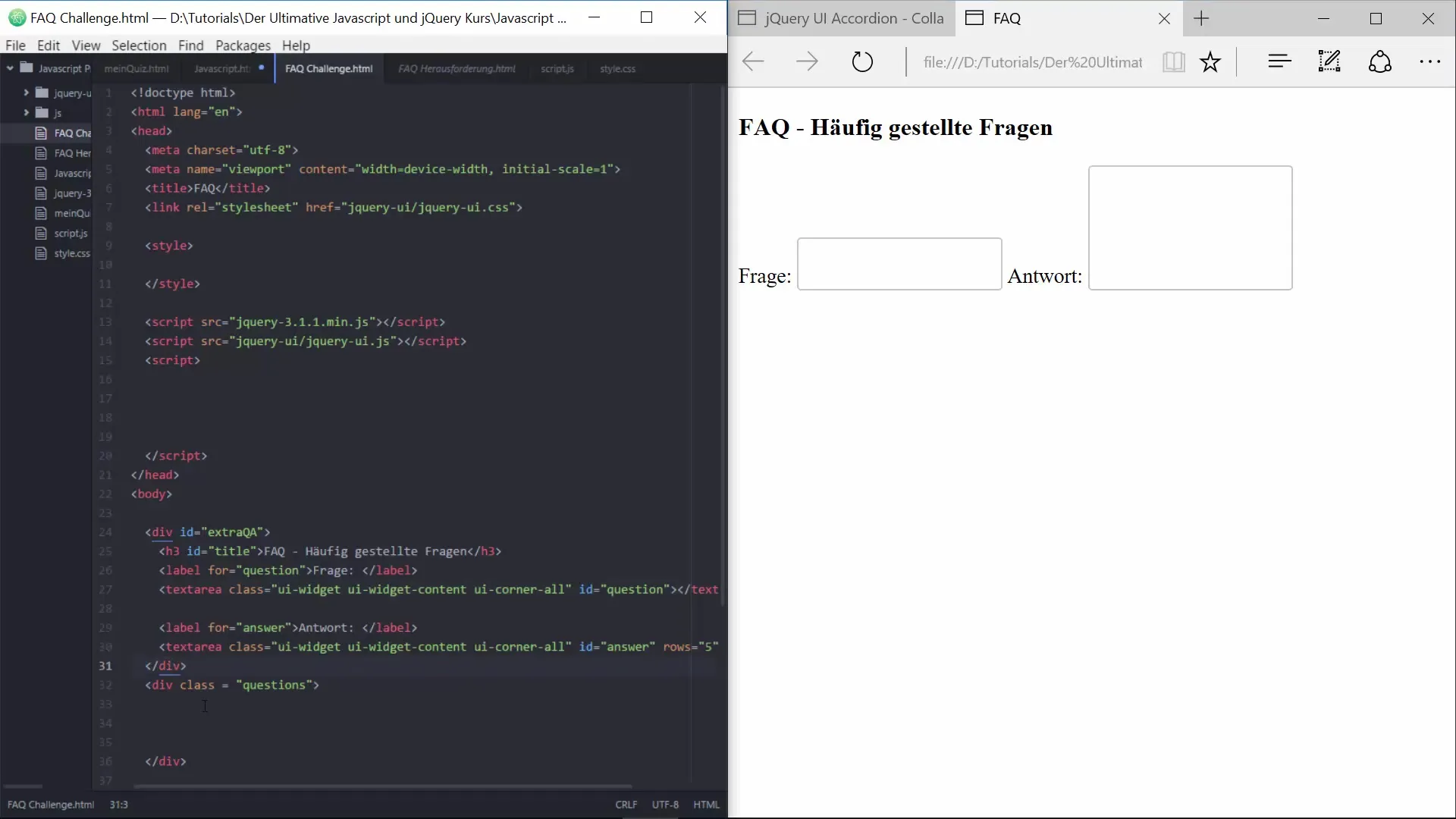
4. Create the Accordion
To create the accordion, you need a container where the questions and answers will be placed. Each question will be wrapped in its own div, which can be shown or hidden when clicked.
5. Add JavaScript for Interactivity
Now comes the interactive component! With JavaScript and jQuery, you will now add logic to add the new questions and answers. This means that the entered questions and answers will be inserted into the accordion when the button is pressed.
6. Implement Accordion Functionality
Once the questions are added, you need to ensure that they also function like an accordion. This can easily be achieved with jQuery. Make sure to define the actors to control the behavior.
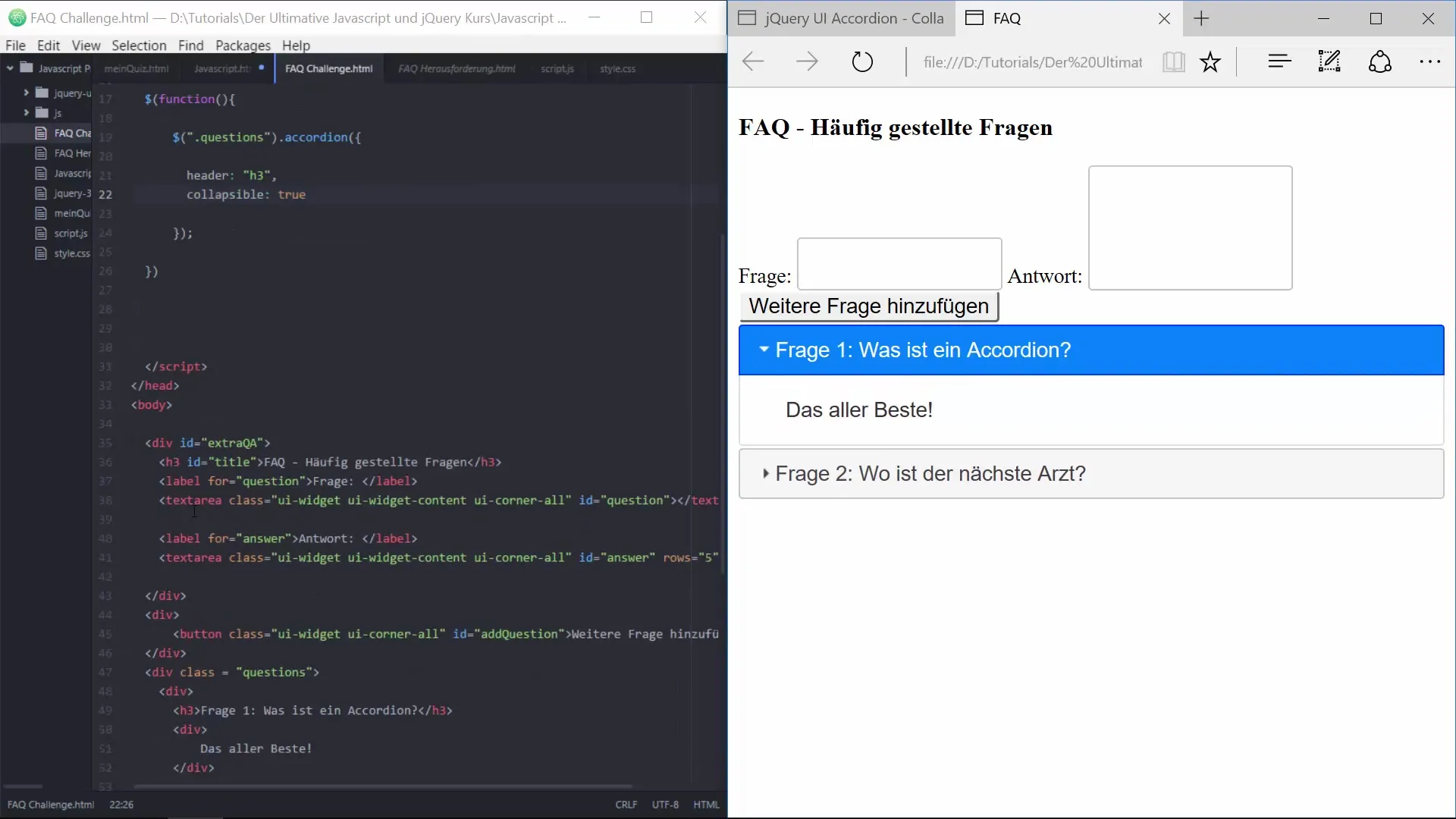
7. Styling with CSS
To make the FAQ page visually appealing, add CSS. Ensure that the textareas take full width of the container and that the elements are arranged attractively.
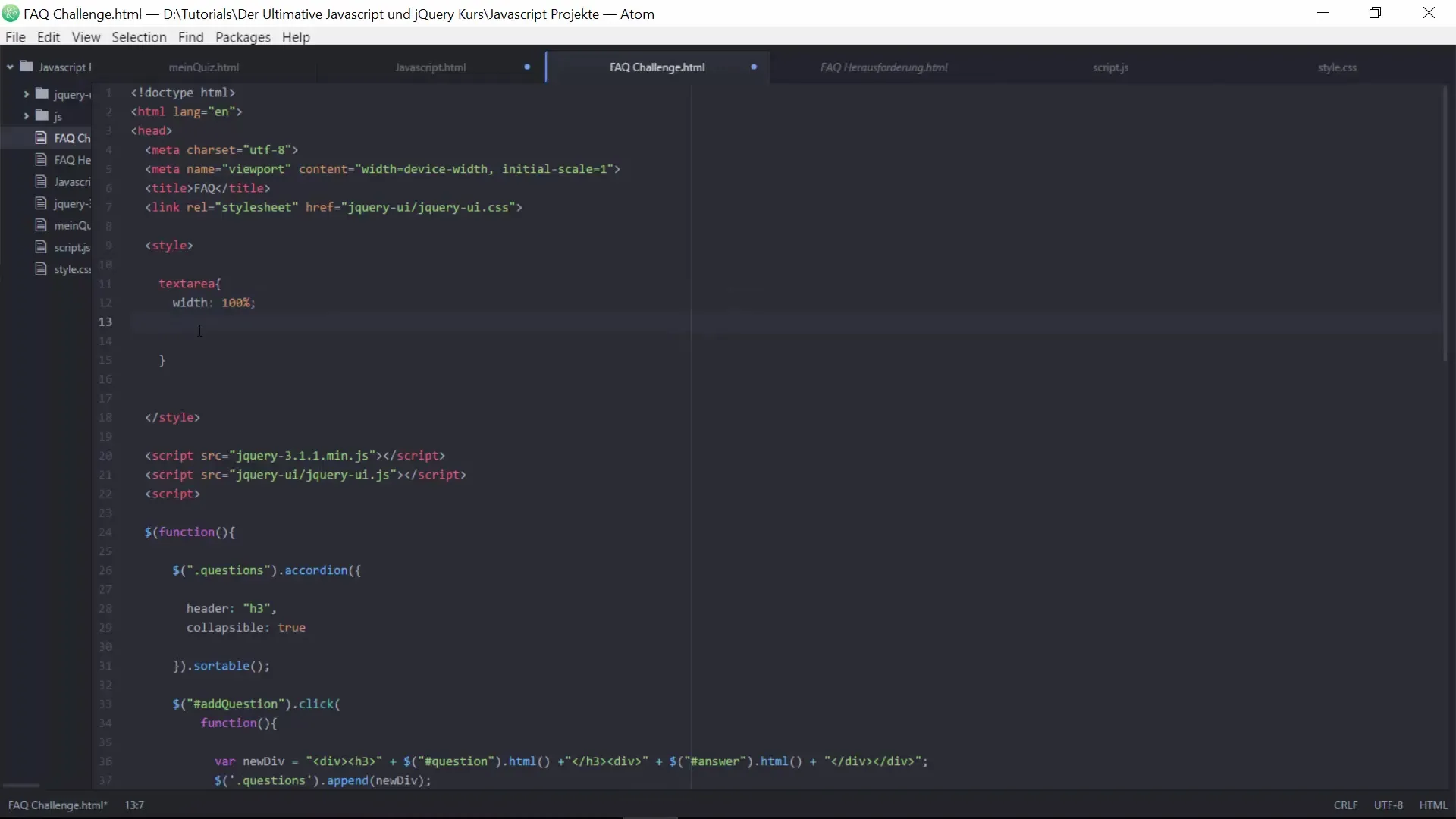
8. Test the Functionality
After completing all the steps, test your FAQ page. Add some questions and make sure everything works as expected. Check the interactivity of the accordion and the user interface.
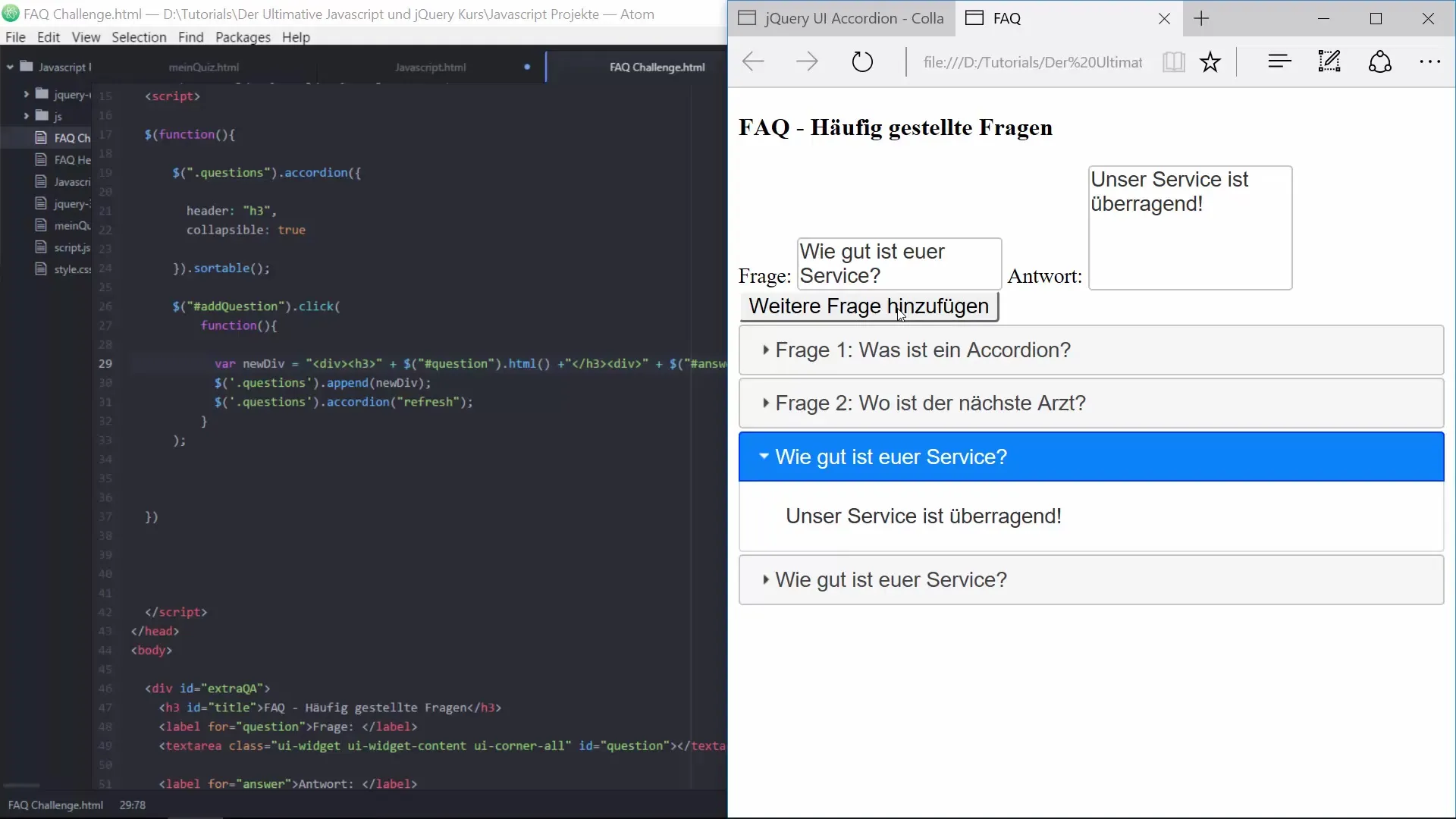
9. Troubleshooting
If something doesn't work as desired, go through the code step by step. Check the console in your browser for any JavaScript error messages and correct them accordingly.
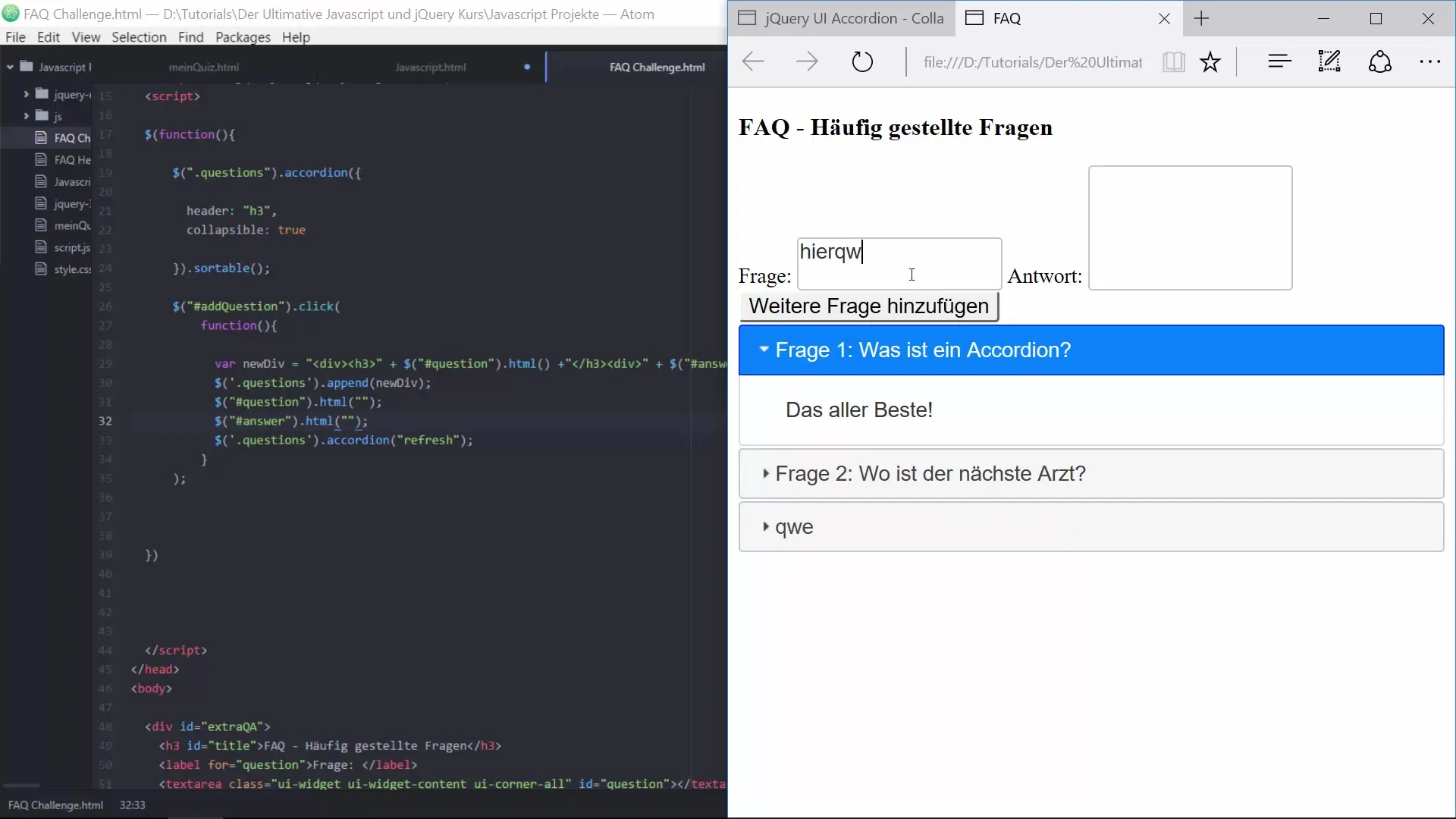
10. Finalization and Refinement
When everything works, focus on further enhancing the design and optimizing the code. Pay attention to responsiveness and user efficiency to get the most out of the FAQ page.
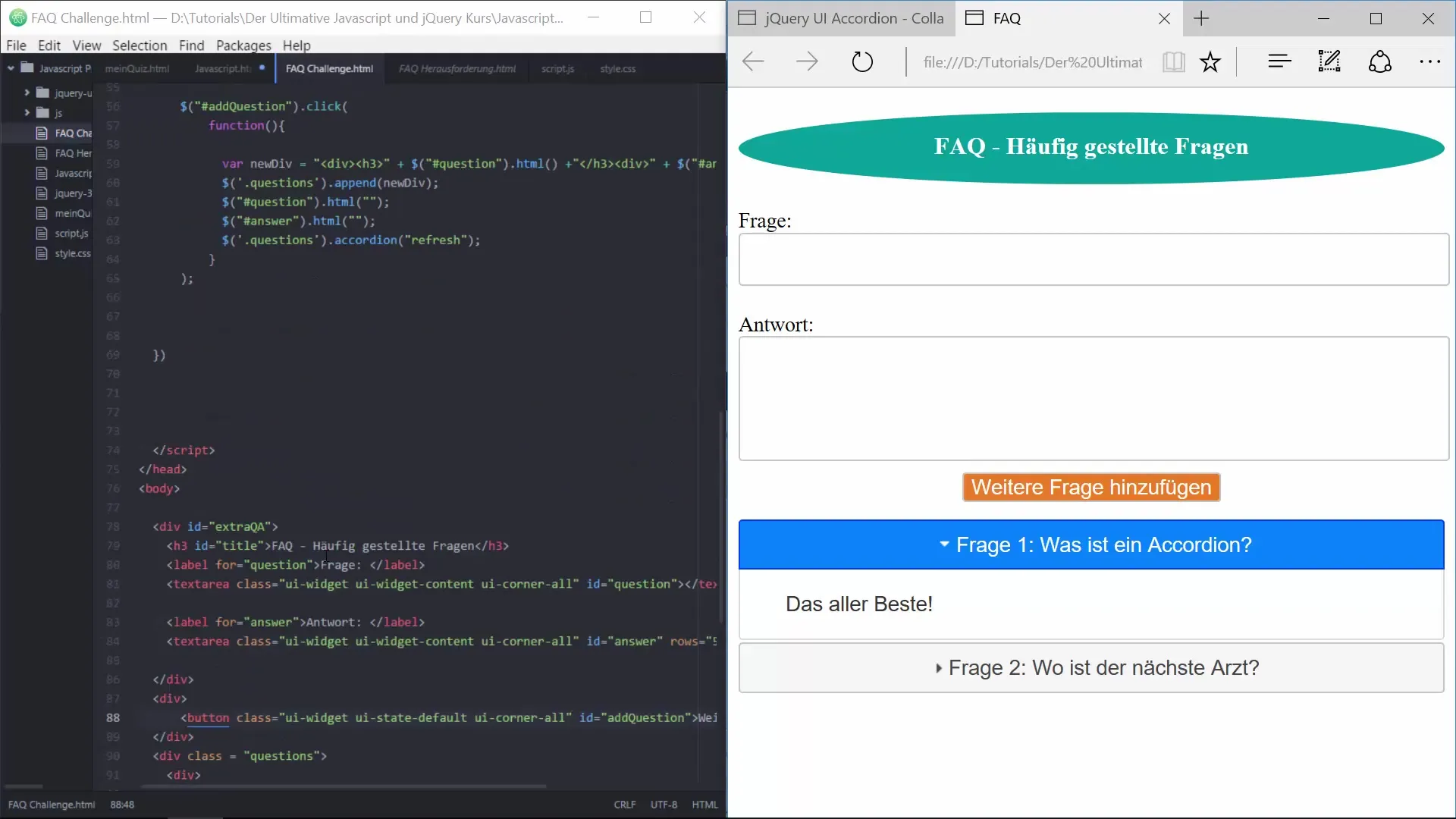
Summary – FAQ Page with JavaScript and jQuery
You have now learned step by step how to create an FAQ page with interactive elements. From the basic HTML structure to full functionality with jQuery and CSS, you have implemented all the essential learning yourself.
Frequently Asked Questions
How can I make the FAQ page responsive?Use CSS media queries to optimize the design for different screen sizes.
Can I further customize the design?Yes, you can adjust the CSS styles to your needs to change the appearance.
What should I do if jQuery doesn't work?Make sure jQuery is correctly included in your project and that no JavaScript errors are being displayed in the console.