You have already learned how a function with a single parameter works. Now we want to deal with the concept of functions with multiple parameters. This type of function is particularly useful when you want to combine or process data from different variables. A simple example is mathematics, where you add multiple values. Below, I will show you how to create and use such functions in JavaScript.
Key Insights
- Functions can accept multiple parameters.
- Each parameter can be used differently to perform complex calculations.
- Functions provide the ability to process and derive data dynamically.
Step-by-Step Guide
Step 1: The Basics of a Function
An important part of programming is understanding how functions work. A function is declared with the keyword function, followed by a name and parentheses that contain the parameters. You already learned how this works in the previous chapter. Now I will show you how to add multiple parameters to a function.
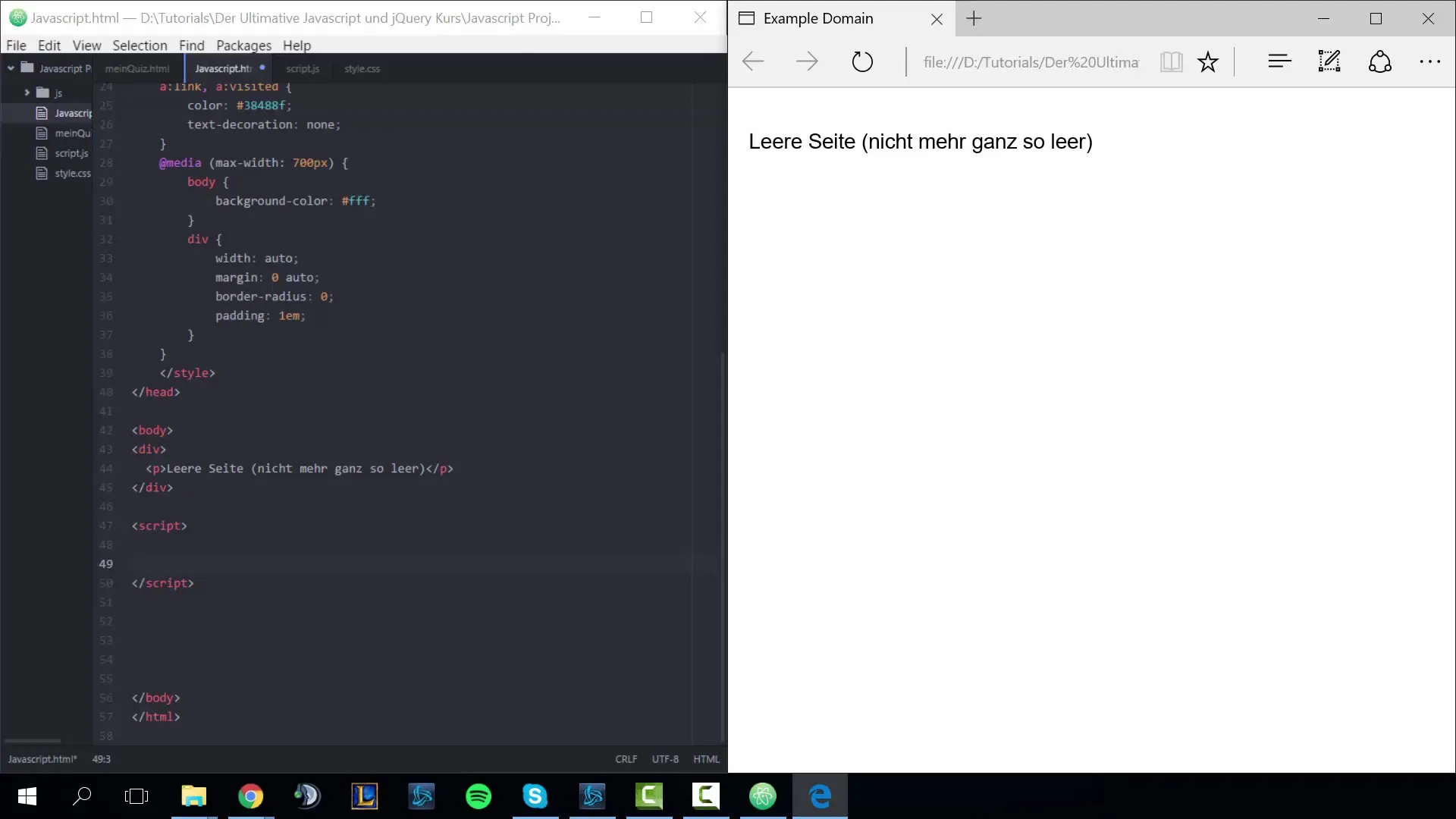
Step 2: Creating a Simple Function
Let's create a function that calculates the "score" of a couple in love. We will name this function Liebest. It takes two parameters, Name1 and Name2, which represent the names of the couple.
Step 3: Explanation of the Parameters
In the Liebest function, Name1 is your own name and Name2 is the name of the crush. The order does not matter much in this case; it is only important that you pass both names to perform the calculation.
Step 4: Calculating the Score
To calculate the score, we create a variable called Punkte. This is calculated from the length of both names. We use the.length property to determine the number of characters in the names. If Name1 is "Dennis" and Name2 is "Denise", the score will be 10, as both names consist of five letters.
Step 5: Displaying the Results
To display the results, you can use a console.log() statement to output the score and the names on the console. You combine the two names and the score in a readable form.
Step 6: Using the Function
To test the function, you call Liebest with two names, e.g., Liebest("Kaffee", "Kuchen"). This will return the score for the combination of these two names. Don't forget that accessing the.length property in JavaScript is simple because it is a property – you don't need parentheses for that.
Step 7: Adding More Parameters
You can expand the function by adding more parameters, such as Name3, Name4, and so on. In this case, you would implement an add function that adds multiple numbers by storing them in variables and outputting the result.
Step 8: Dynamically Calculating
Store the result of the addition or calculation in a variable called ergebnis. You can call the function with any four values to see the result – for example: addieren(123, 1003, 45, 11), and the program will return the sum of these values.
Summary – Functions with Multiple Parameters
In this guide, you have learned how to create and use functions with multiple parameters in JavaScript. The basics of functionality and data processing through parameters were illustrated in simple but effective examples. Use this knowledge to continue building your programming skills and develop more complex functions.
Frequently Asked Questions
How many parameters can a JavaScript function have?A JavaScript function can have as many parameters as you specify in the function definition.
Can I use optional parameters in a function?Yes, you can make parameters optional by setting default values for them.
How do I call a function with multiple parameters?To call a function with multiple parameters, you pass the values in the same order as the parameters are defined.
What happens if I pass fewer parameters than the function expects?In JavaScript, parameters that are not passed receive the value undefined.
Can I use different data types with functions that have multiple parameters?Yes, in JavaScript you can use parameters of different data types in a function, such as numbers, strings, or objects.