JavaScript is indispensable in modern web development. It allows you to create interactive web pages and generate dynamic content. In this tutorial, you will learn how to implement click events and work with variables. The focus is on a simple, practical application so that you can get started immediately.
Key Takeaways
- You will learn how to handle clicks on buttons or other elements in JavaScript.
- You will discover how to store values with variables and retrieve them later.
- The combination of click events and variables opens up numerous possibilities in web development.
Basics of Clicks in JavaScript
To make a button interactive, you should first have the basic structure of HTML and JavaScript. In this step, I will show you how to create a button that displays an alert when clicked.
Next, you need to reference this button in your JavaScript file and assign it a function that triggers when you click it. The getElementById method is used to select the button.
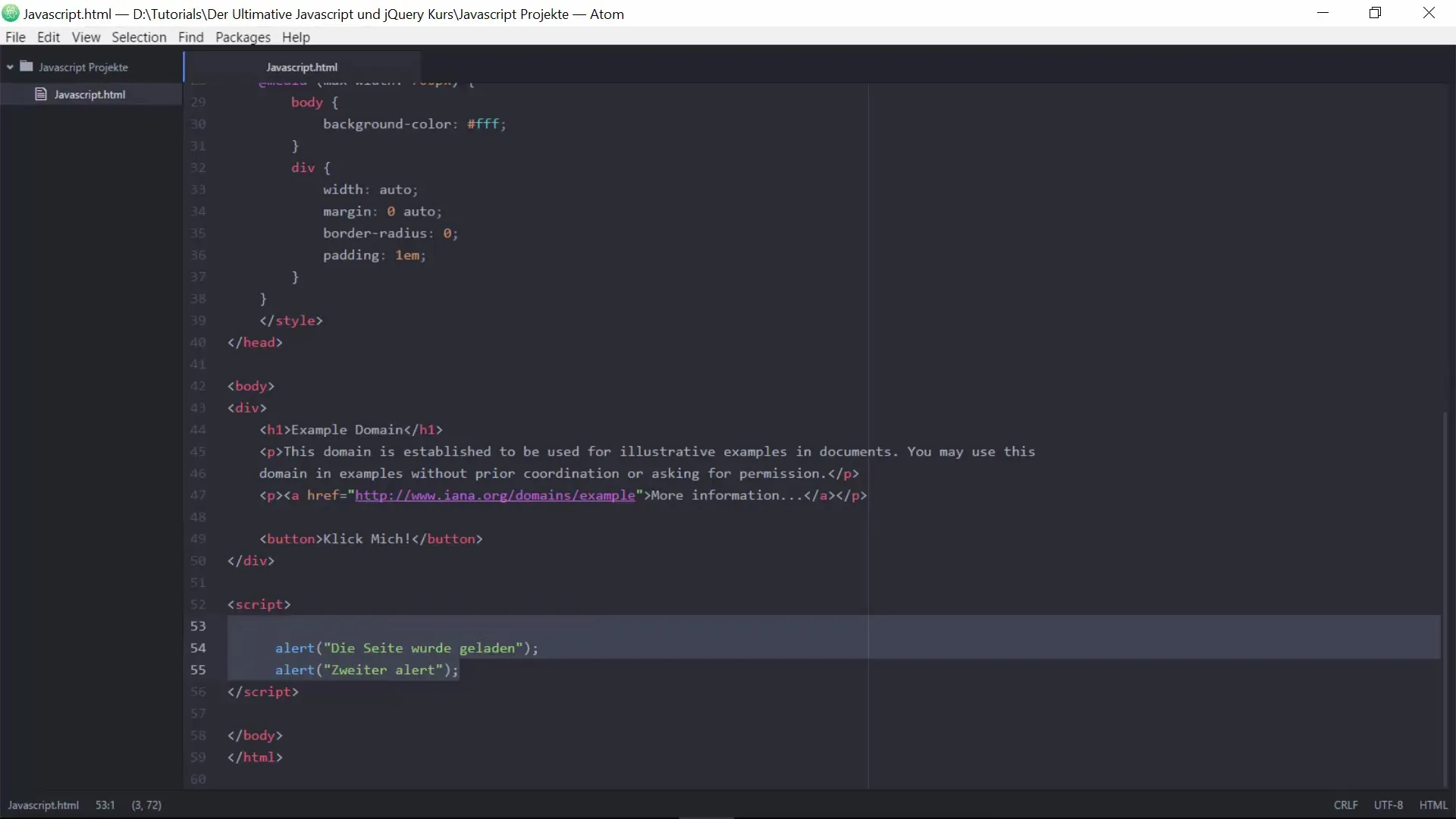
Now you can implement the click event.
In this example, the alert indicates that the button was successfully clicked. Now test your implementation and ensure that the alert appears when you click the button.
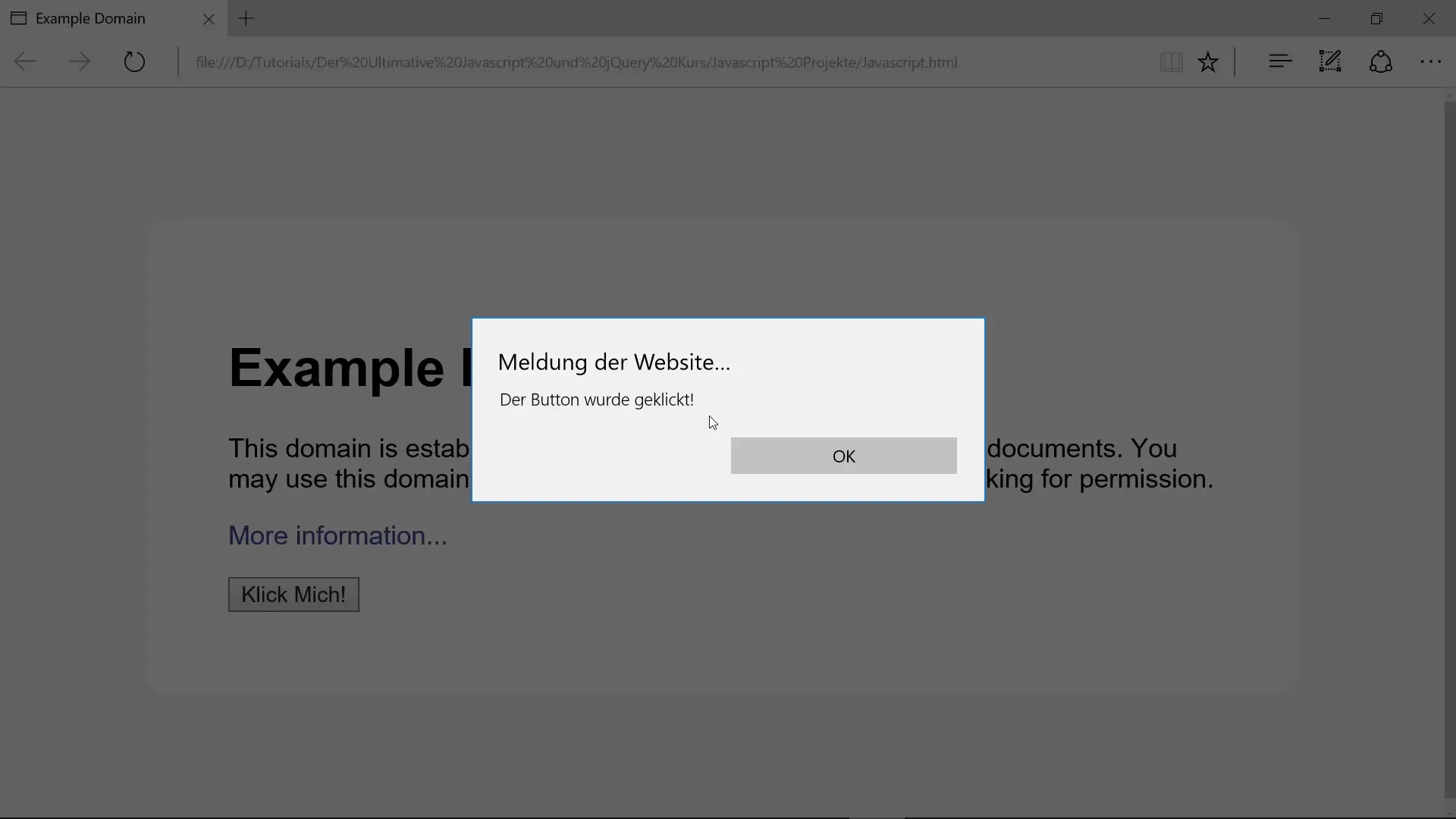
Using Variables
Variables are a central concept in programming. They allow you to store data and reuse it later. Let's create a variable and see how we can use it in conjunction with your previous implementation.
Create a new variable that stores a specific value.
You can then use this variable to change the text in your alert.
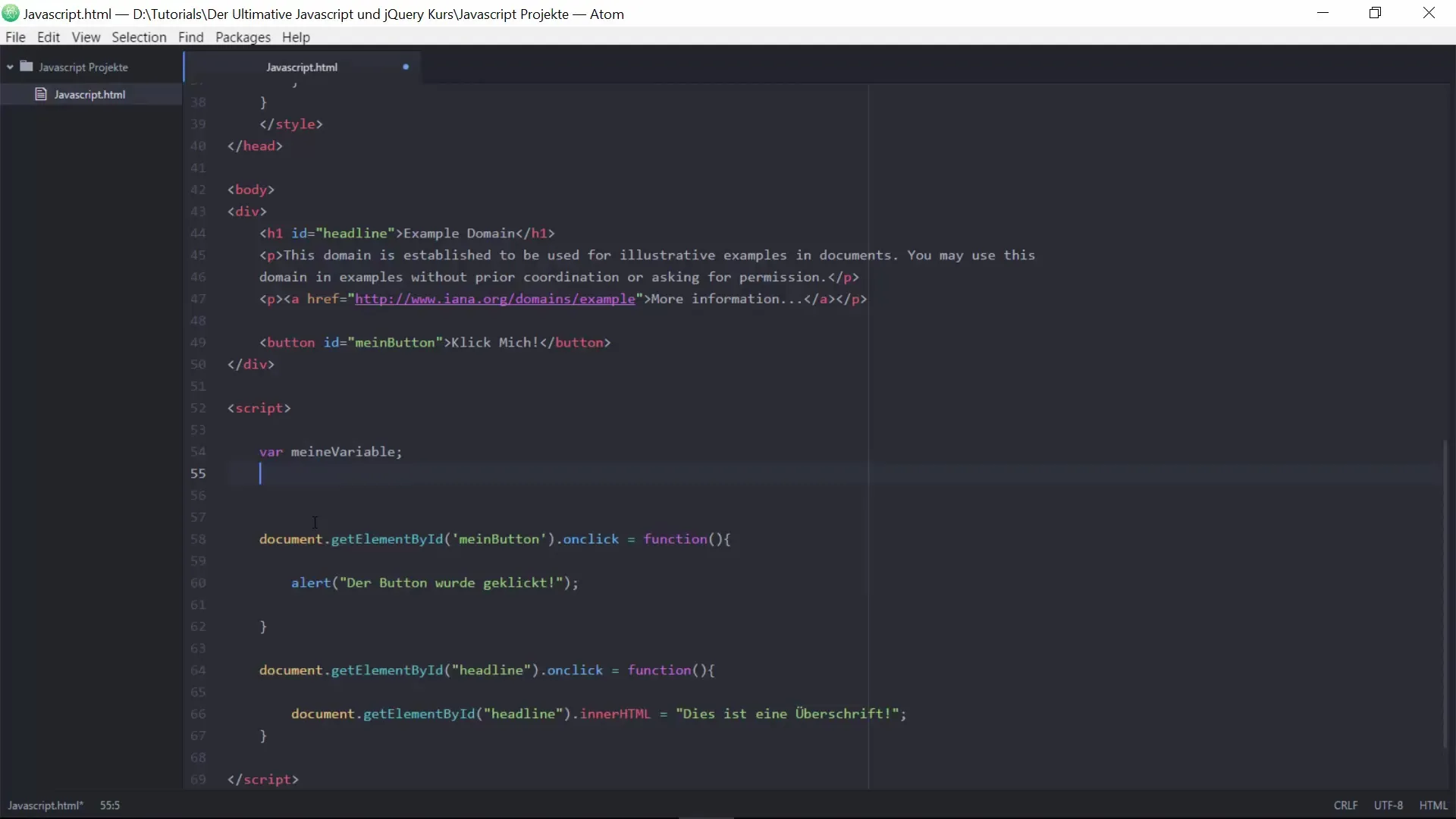
Now let's change the alert so that instead of a fixed number, the value of the variable is displayed.
When you click the button now, the alert looks like this: "The value is: 15". Feel free to experiment with the value of the variable and see what happens.
Creating Interactive Web Pages
Now let's see how we can create an interactive web page using click events and variables. You might want to change the text of an H1 element when you click your button.
In your JavaScript, you can then update the inner HTML content of this H1 element with a new variable.
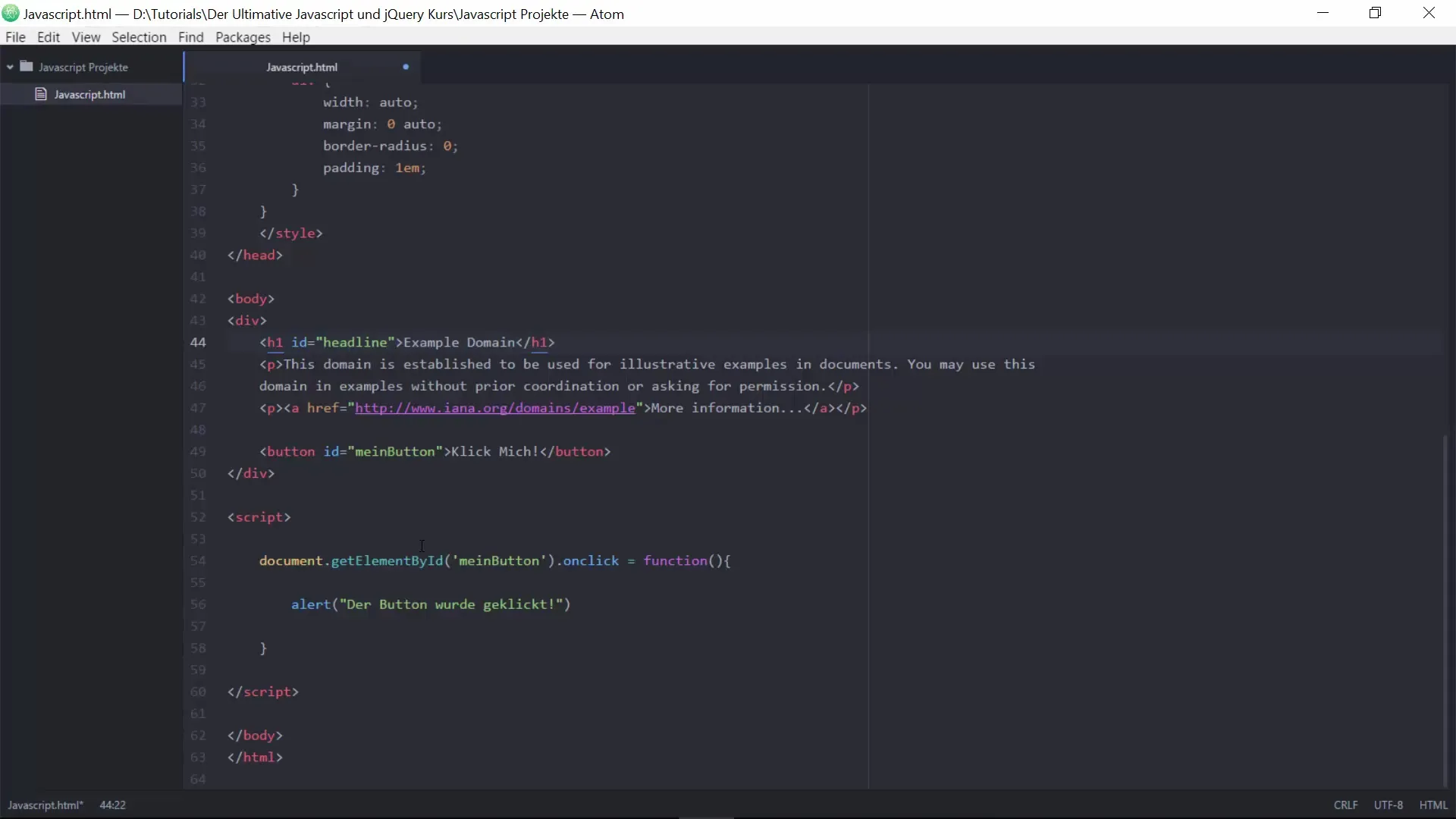
When you click the button now, the text of the heading changes. This makes the page interactive and more appealing to users.
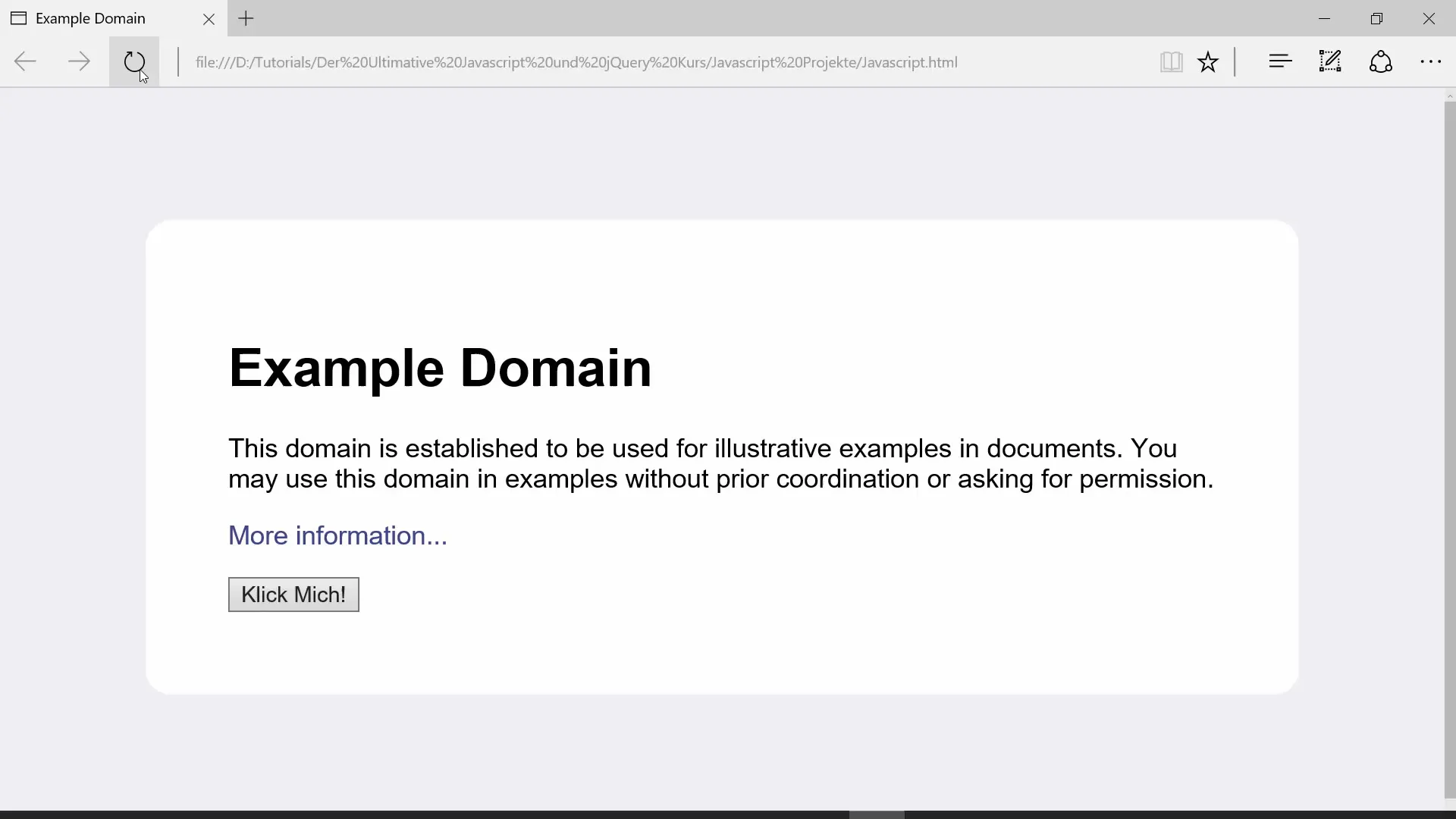
Using Variables with Various Data Types
Another important aspect is the different data types in JavaScript. In addition to numbers, you can also store text or boolean values. Here are some examples:
The way you define a variable affects how you can use it later.
When you click the button, the text of the heading changes according to the content of newText.
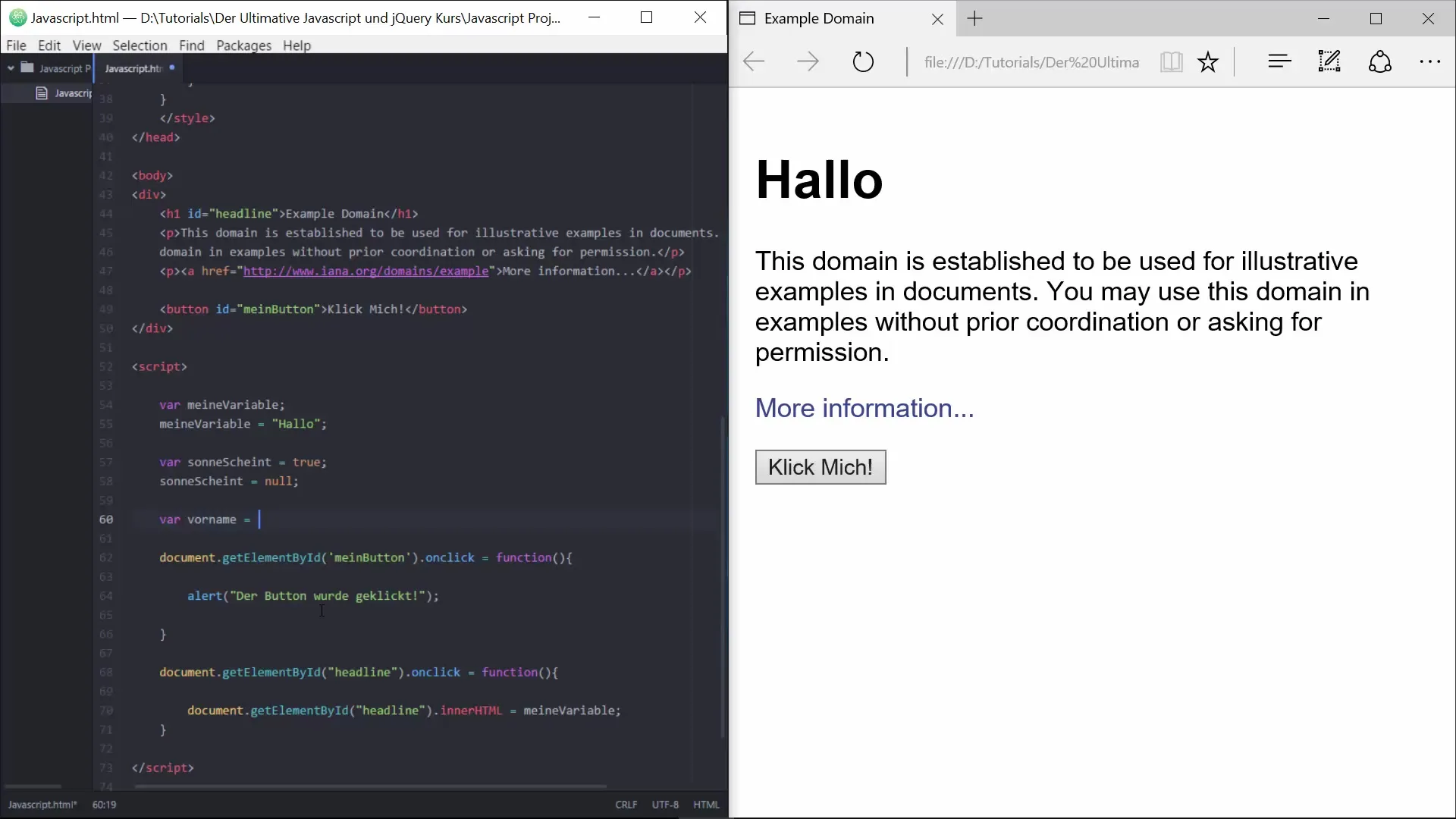
Summary – Efficient Use of JavaScript Clicks and Variables
You have learned the basics of implementing click events in JavaScript and using variables to create dynamically interactive elements. As you continue to experiment and deepen your understanding of the concepts, you will be able to develop more complex functionalities.
Frequently Asked Questions
What is the difference between a number and a string in JavaScript?A number is processed as a numeric value, while a string is text that is enclosed in quotes.
How can I change the value of a variable?You can simply overwrite the value of a variable with an assignment, e.g., myVariable = 20.
Do I need a semicolon at the end of a line in JavaScript?In most cases, it's recommended to use semicolons as they clearly define where a statement ends.
What happens to variables when I refresh the page?When the page is refreshed, variables are reset and lose their content, as they only exist in the context of the current session.
How can I use multiple parameters in my function?You can simply define additional parameters during the function call, e.g.: function myFunction(param1, param2).