Sets are an exciting and useful data structure in Python that helps you manage unique values and work efficiently with data. In this guide, you will learn everything about sets, how to create and manage them, and their advantages compared to lists. Let's dive right into the topic!
Key Insights
- Sets are a collection of unique elements.
- You can create sets in different ways: by assignment or by casting.
- Sets do not accept duplicate values, making them ideal for filtering duplicates.
Step-by-Step Guide to Working with Sets in Python
Step 1: Create a Set
To create a set in Python, you need a variable name.
This means that it can now be referenced as an empty set. Alternatively, you can also initialize the set with curly braces.
Both methods are valid, and it's a matter of personal preference which one you want to use.
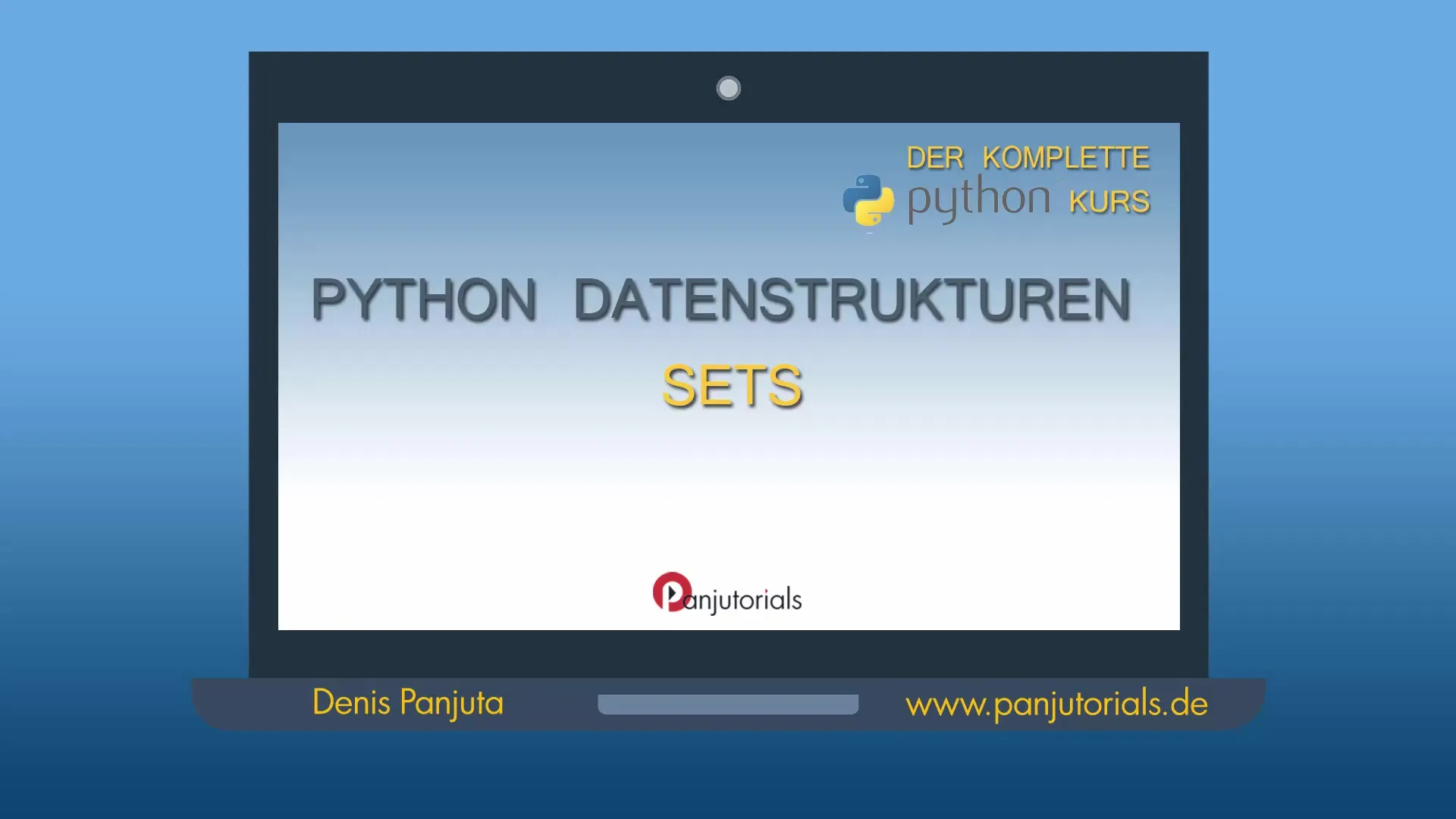
Step 2: Add Values to a Set
After you have created a set, you can add values. This is done using the add() method.
If you print it now, you will see that the values are represented in curly braces.
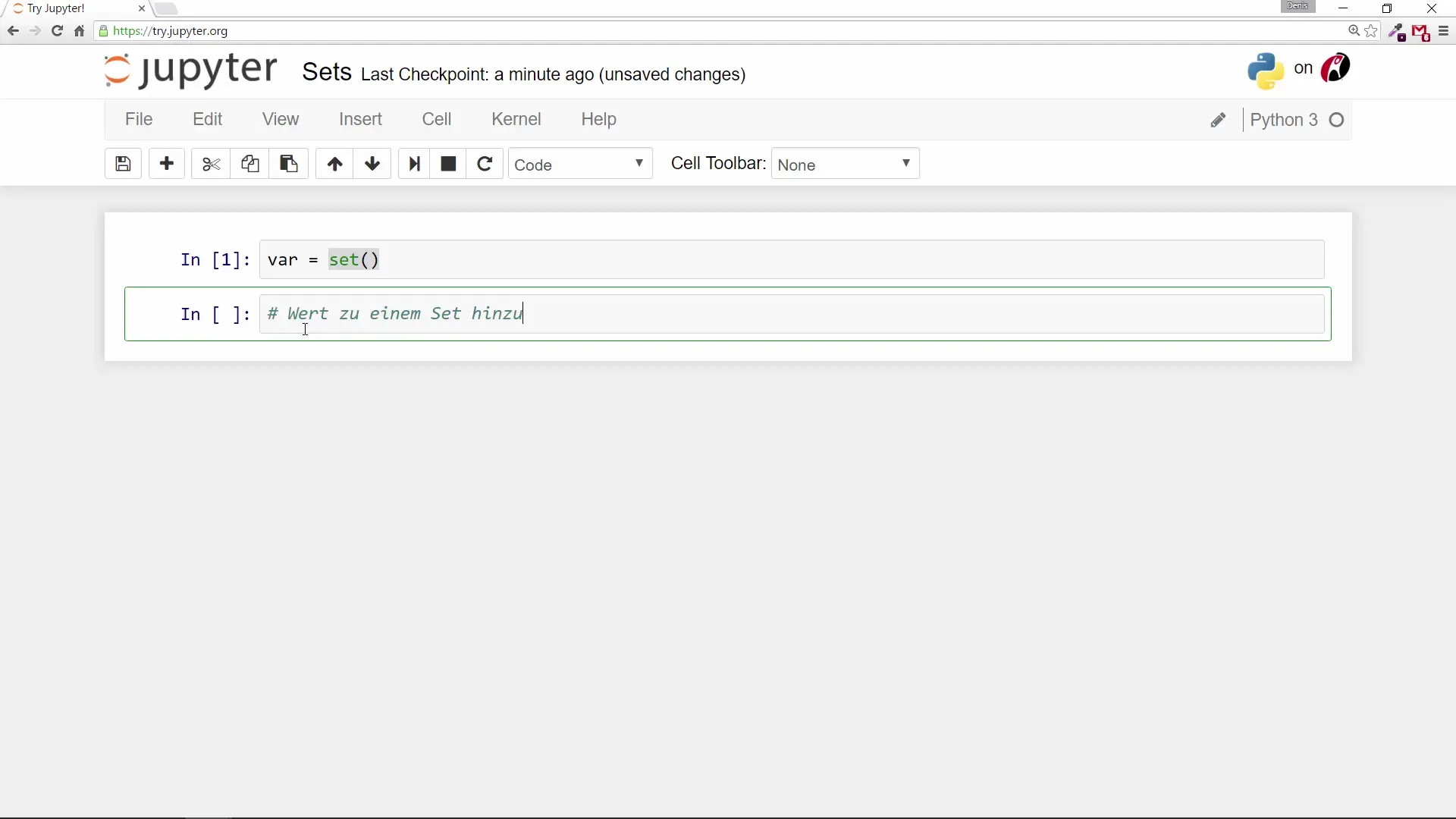
Step 3: Add Another Element
If you try to add an element that is already present in the set, it will not be added again.
the set does not change when we add the value 4 again. Check the result by printing the set again.
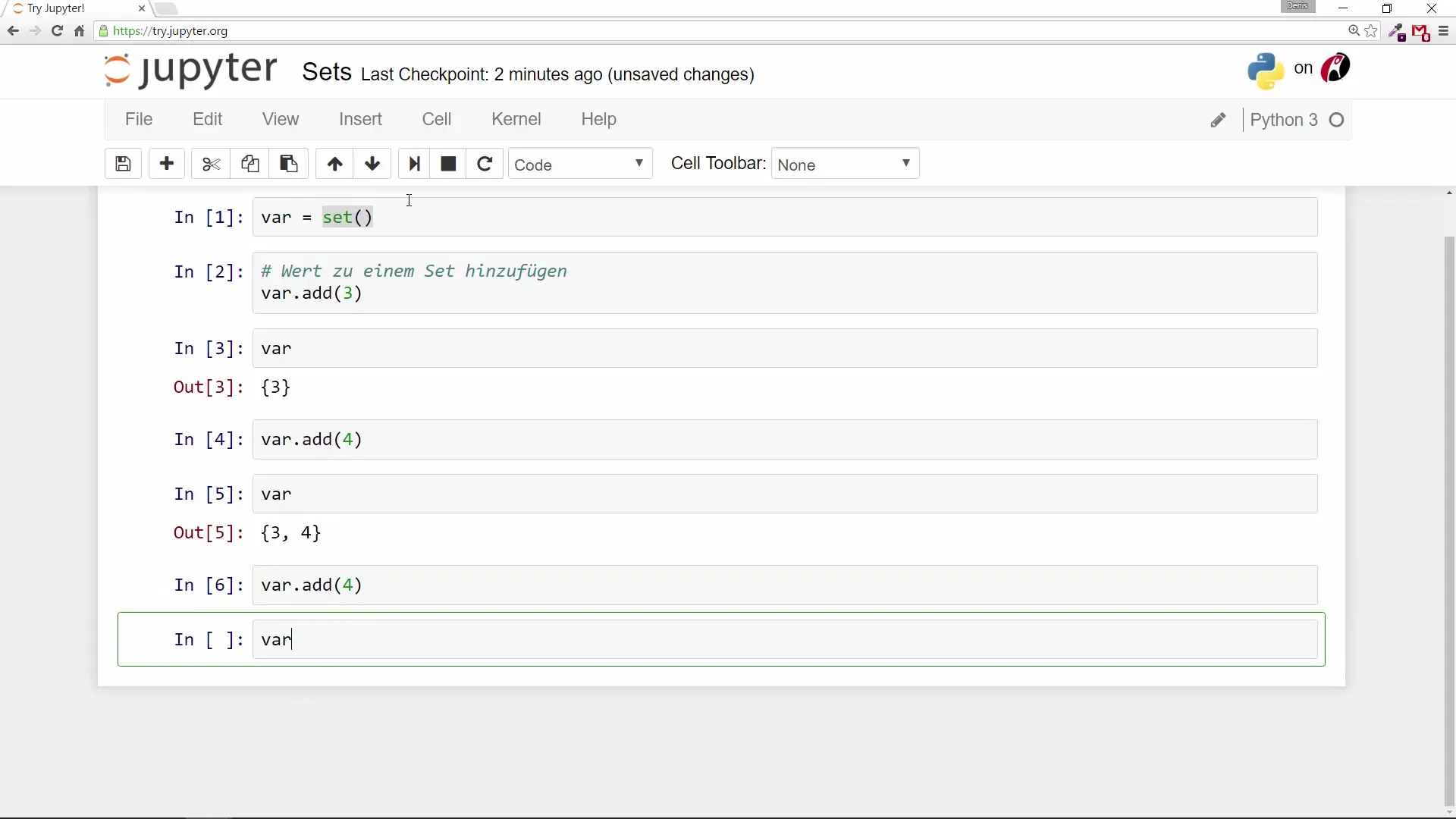
Step 4: Create Sets from a List
You can also convert a list into a set. This is called “casting”.
Now, set_list contains only the unique values.
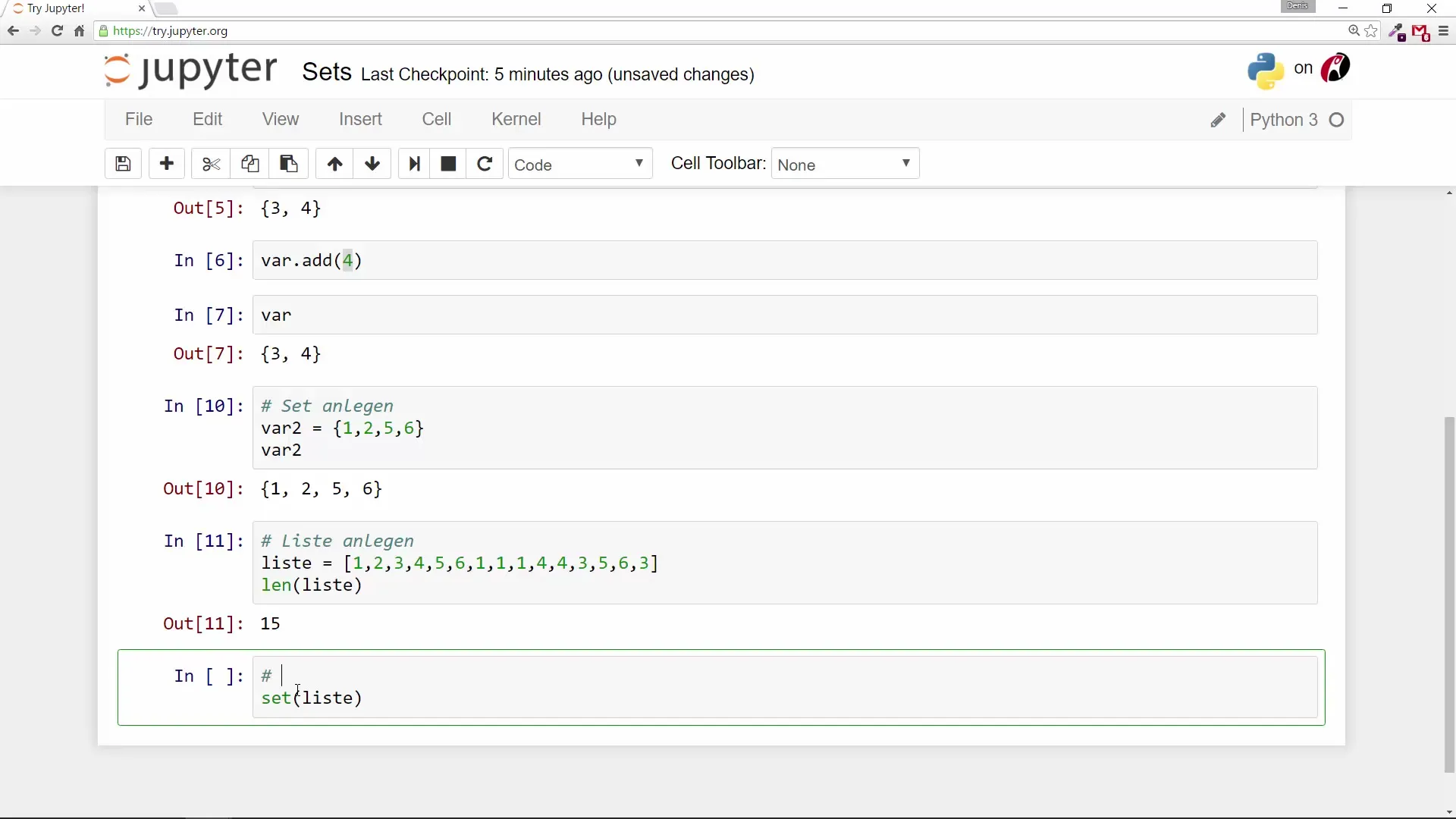
Step 5: Check the Number of Entries
Use the len() function to check the number of elements in your set.
This returns the number of unique elements in set_list, showing how effective sets are compared to lists in avoiding duplicates.
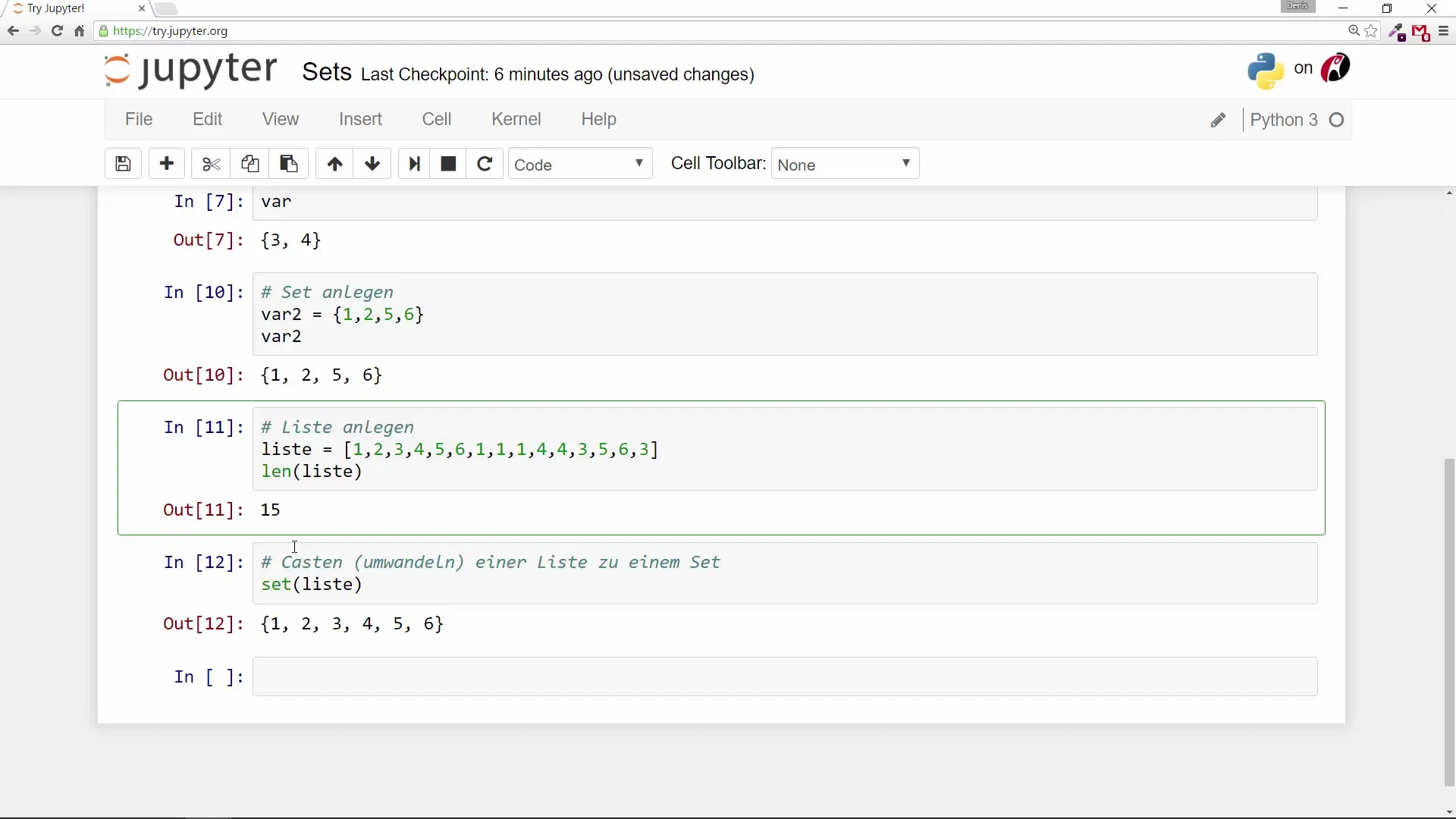
Step 6: Using Sets to Filter Duplicates
A set becomes particularly helpful when you have a large amount of data with many duplicates. With the set() function, you can easily remove duplicates to end up with a set containing only unique values. This helps you to create a clean dataset, which can be immensely beneficial in many application scenarios.
Step 7: Summary of Set Creation
In summary, you can create a set in two ways: by assigning a variable and by casting a list into a set. You also learned how to avoid duplicate values by storing only unique values in your set.
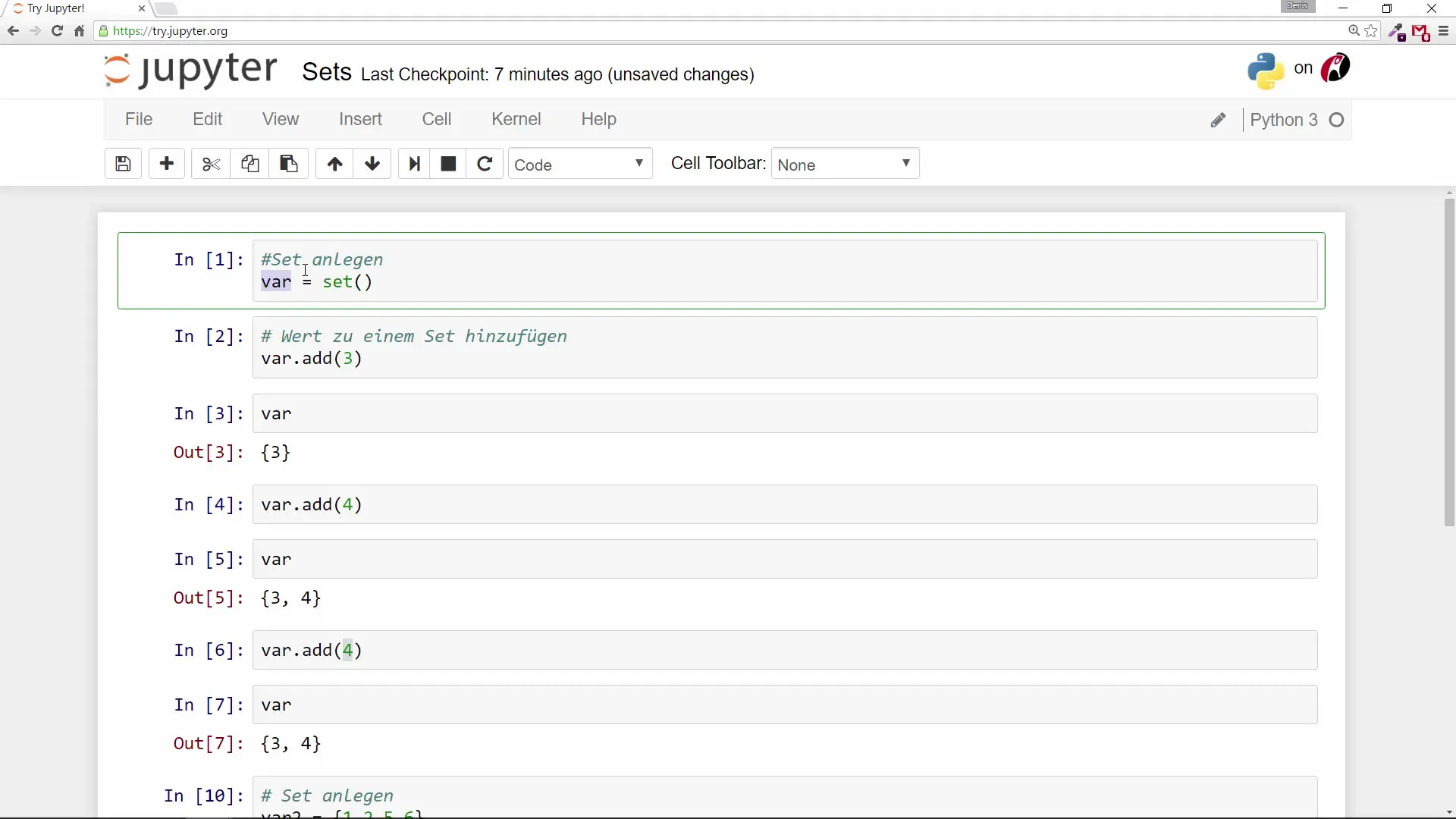
Summary – Introduction to Programming with Sets in Python
You have now learned the fundamentals of sets in Python. From creation to using them for filtering data, you have the necessary knowledge to use sets effectively in your projects.
Frequently Asked Questions
What are sets in Python?Sets are collections of unique elements that do not allow duplicate values.
How do I add values to a set?Use the add() method to add values to a set.
Can sets contain duplicates?No, sets can only contain unique values and ignore duplicates.
How do I create a set from a list?Use the set() function and pass the list as an argument.
How do I check the number of elements in a set?Use the len() function on your set.