Counter in Python are very popular. This powerful tool allows you to count how often certain values appear in an object. If you work with data and its analysis, you will quickly notice the advantages a Counter can offer in programming. In this guide, you will learn the different methods to initialize a Counter and how to use it effectively in your projects. Let’s dive right in!
Key Insights
- Counters are containers that count the frequency of values.
- There are various ways to initialize a Counter.
- With the update method, you can extend an existing Counter.
- The Counter can work with characters as well as whole words or numbers.
- The most_common feature allows you to quickly identify the most frequently occurring elements.
Step-by-Step Guide
To use a Counter, you first need to import the collections module. This is done easily by writing this command in your Python script.
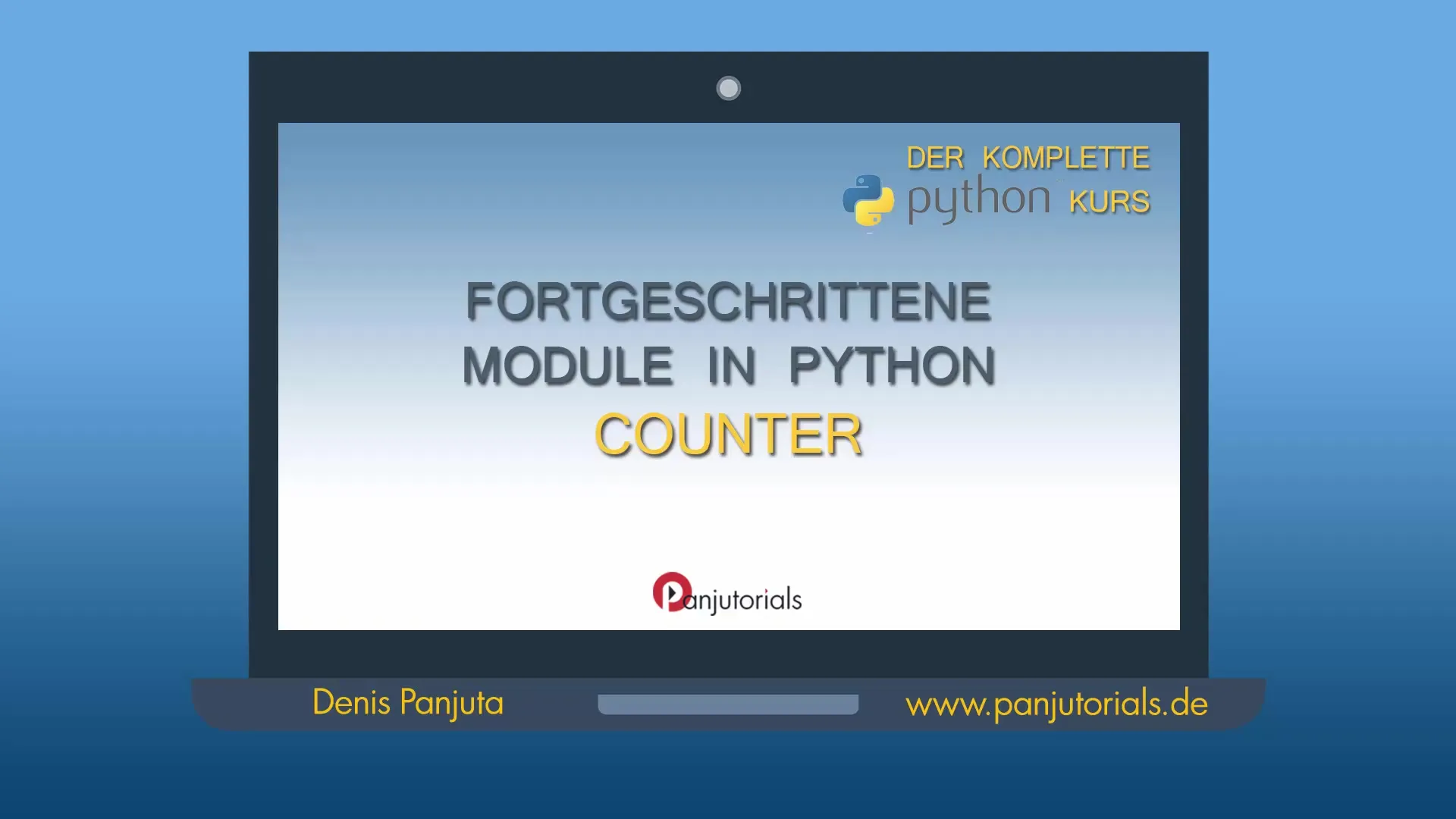
Once the module is imported, there are different ways to initialize a Counter. One way is to pass the values directly as a list. For example, if you create a list of characters containing the letters "A", "B", "C" along with some repetitions, you can do it like this.
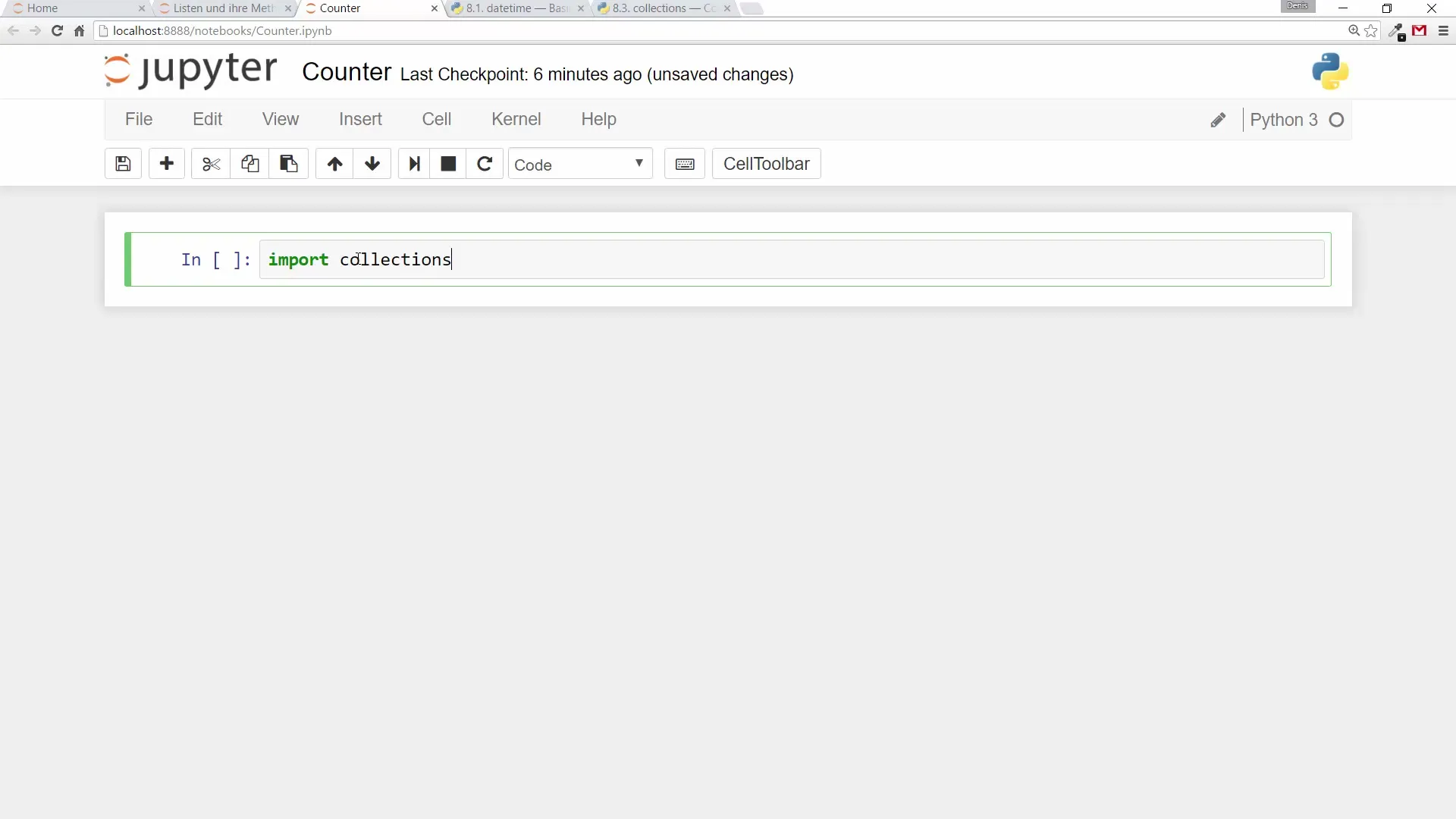
You will now see that the count of occurrences is displayed for each letter. In this example, the Counter shows: "A" appears twice, "B" three times, and "C" once.
Another example for initializing the Counter is to use empty curly braces to create an empty Counter. This is especially useful if you want to add values later.
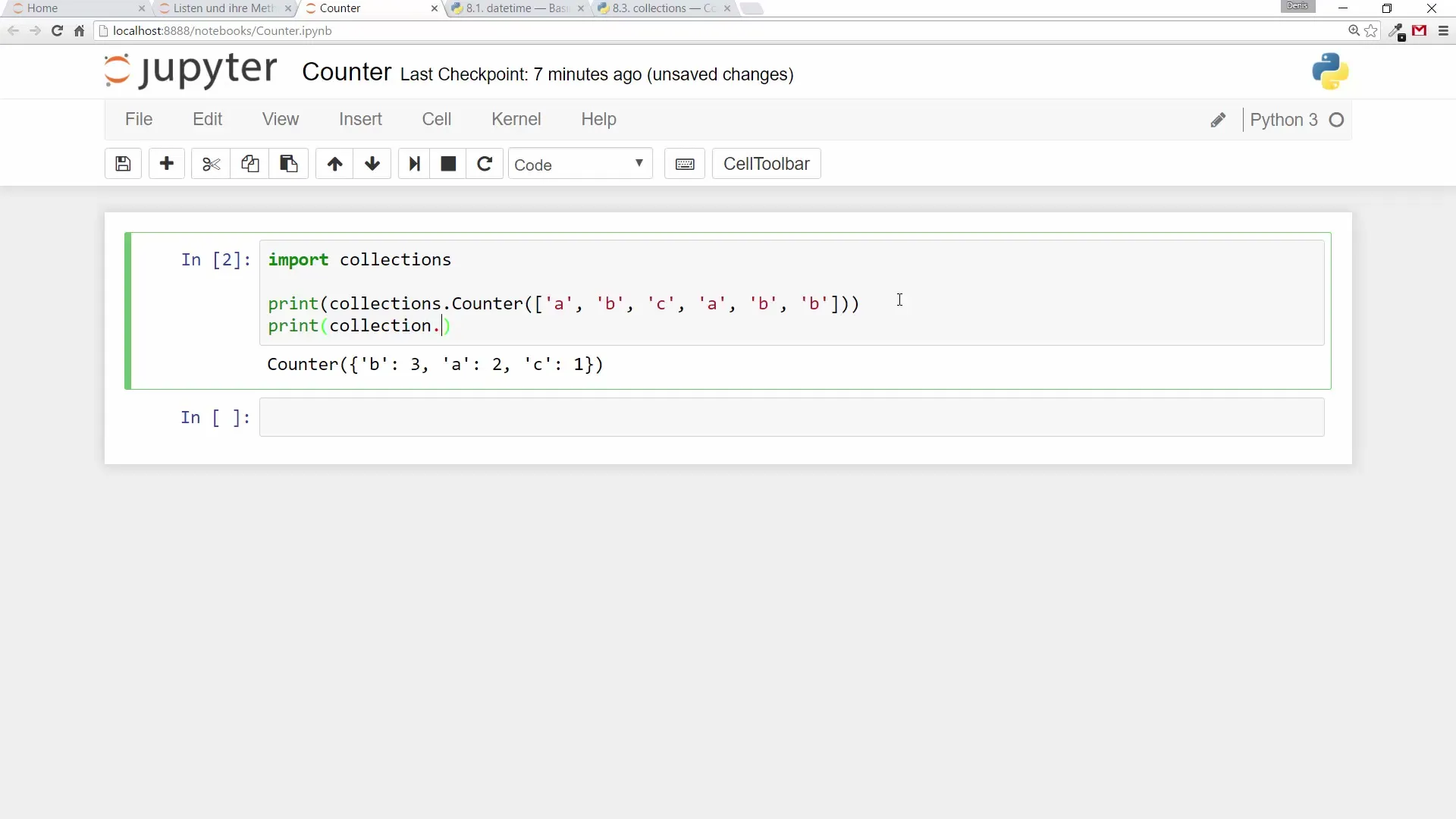
To update this empty Counter, you can use the update method. Suppose you want to add the letters "A", "B", "C", "D" and some repetitions. The update would be done like this:
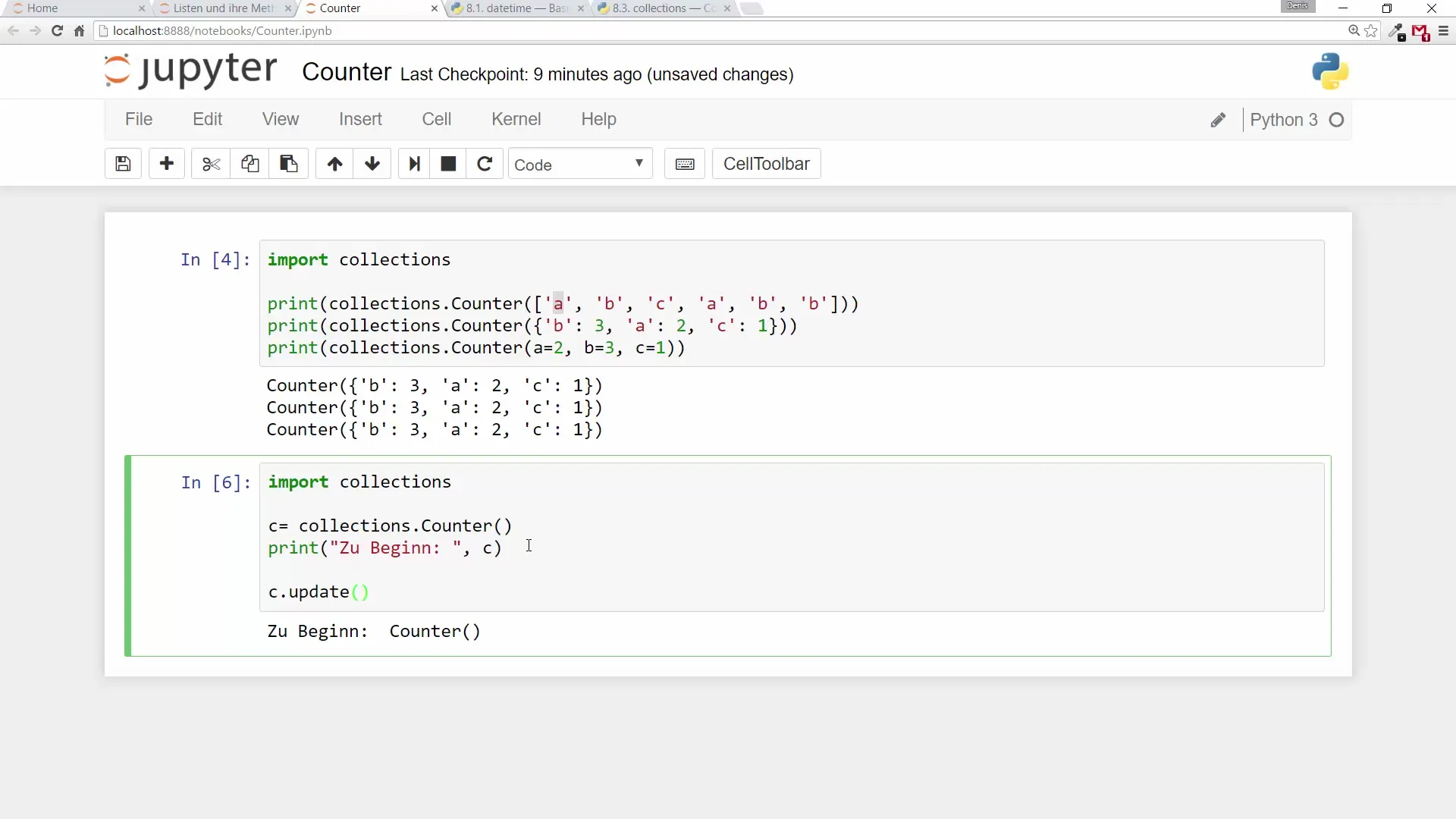
When you print the result, you will see that the Counter now correctly displays the frequencies of the letters.
In addition to letters, you can also use a Counter to count the frequency of words in a sentence. For this, you can simply split the sentence and count the individual words.
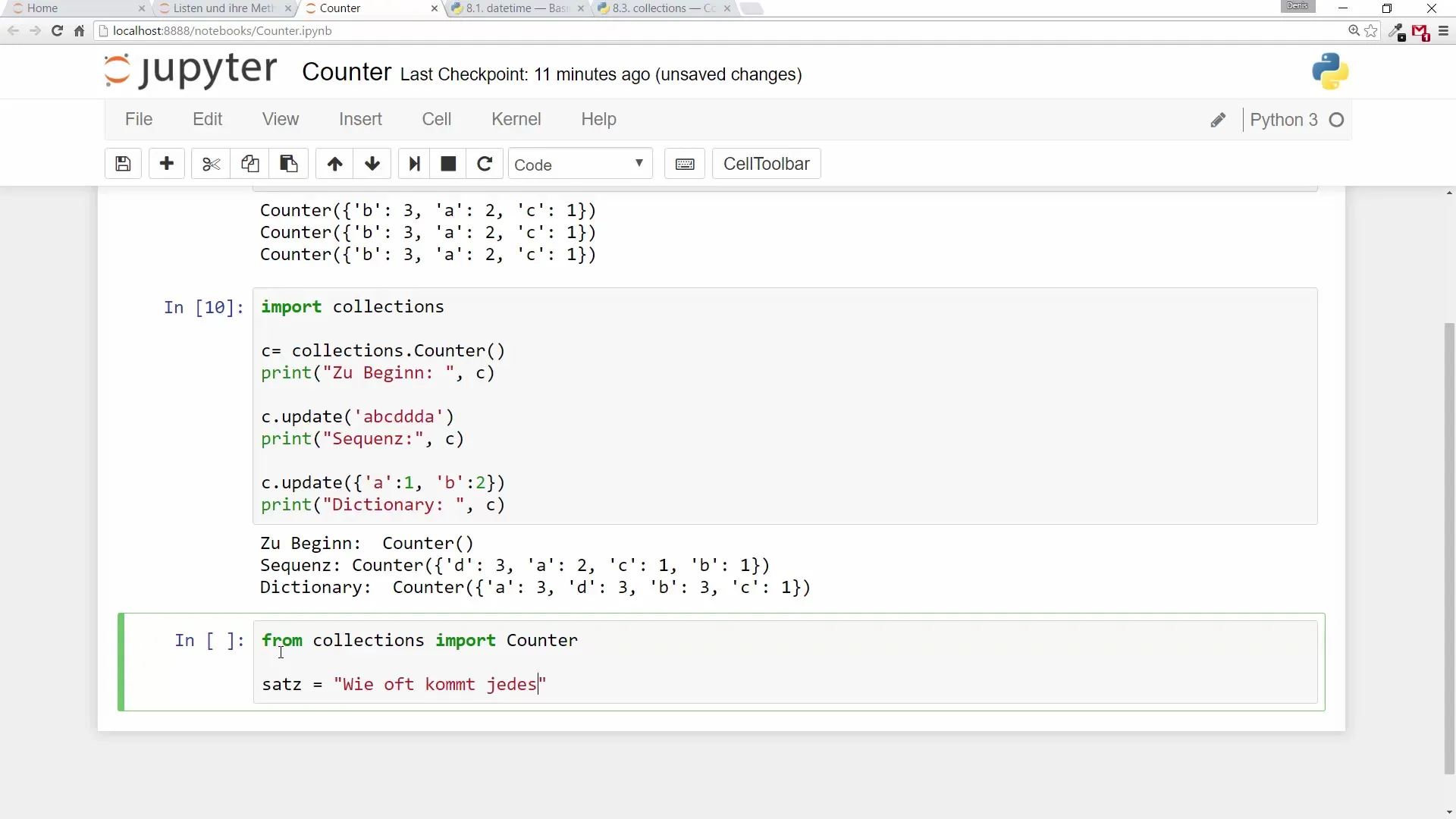
You can then apply the Counter to the list of words and check how often each word occurs.
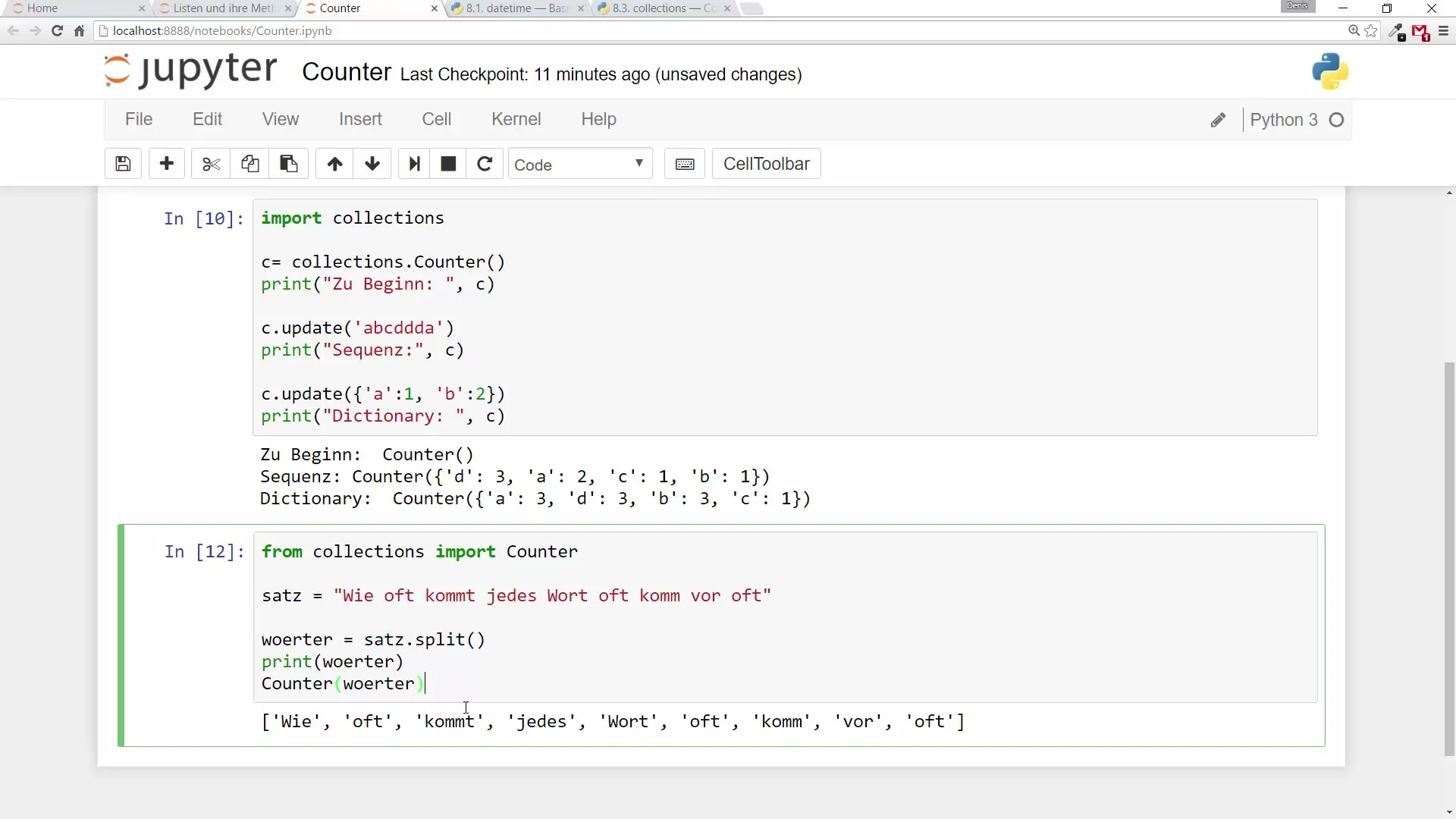
If you analyze a large text file and want to know which word occurs most frequently, you can use the most_common method for this. This will return the most common words in descending order.
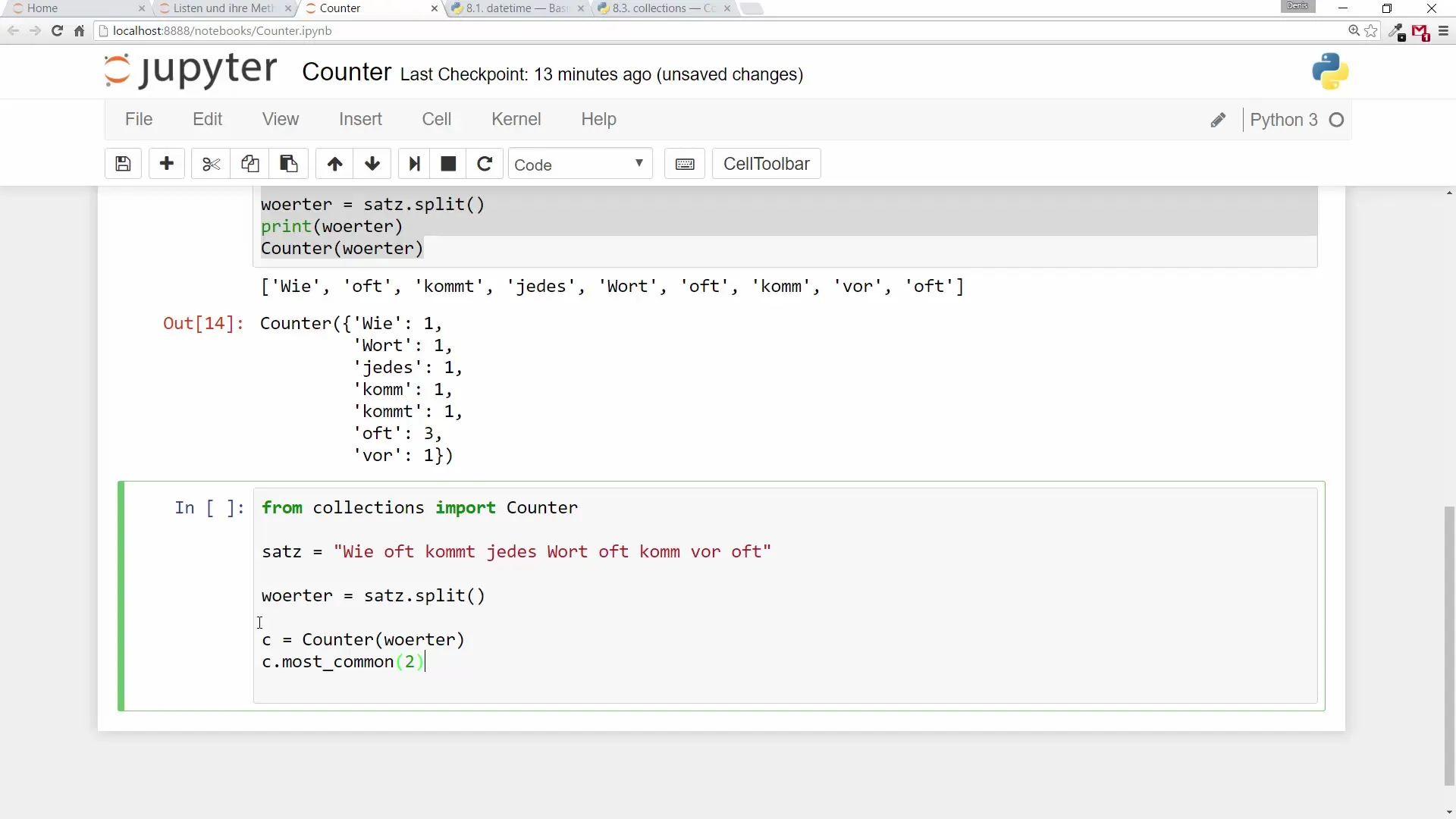
For example, if you want to find out the two most common words, you can implement it like this:
Now you can observe that the word "Often" is the most common word in the previously used sentence. This gives you a very useful overview of the most frequent terms in your text.
The Counter can also be applied to numbers. You can create a list of numbers and count the frequency of each individual value.
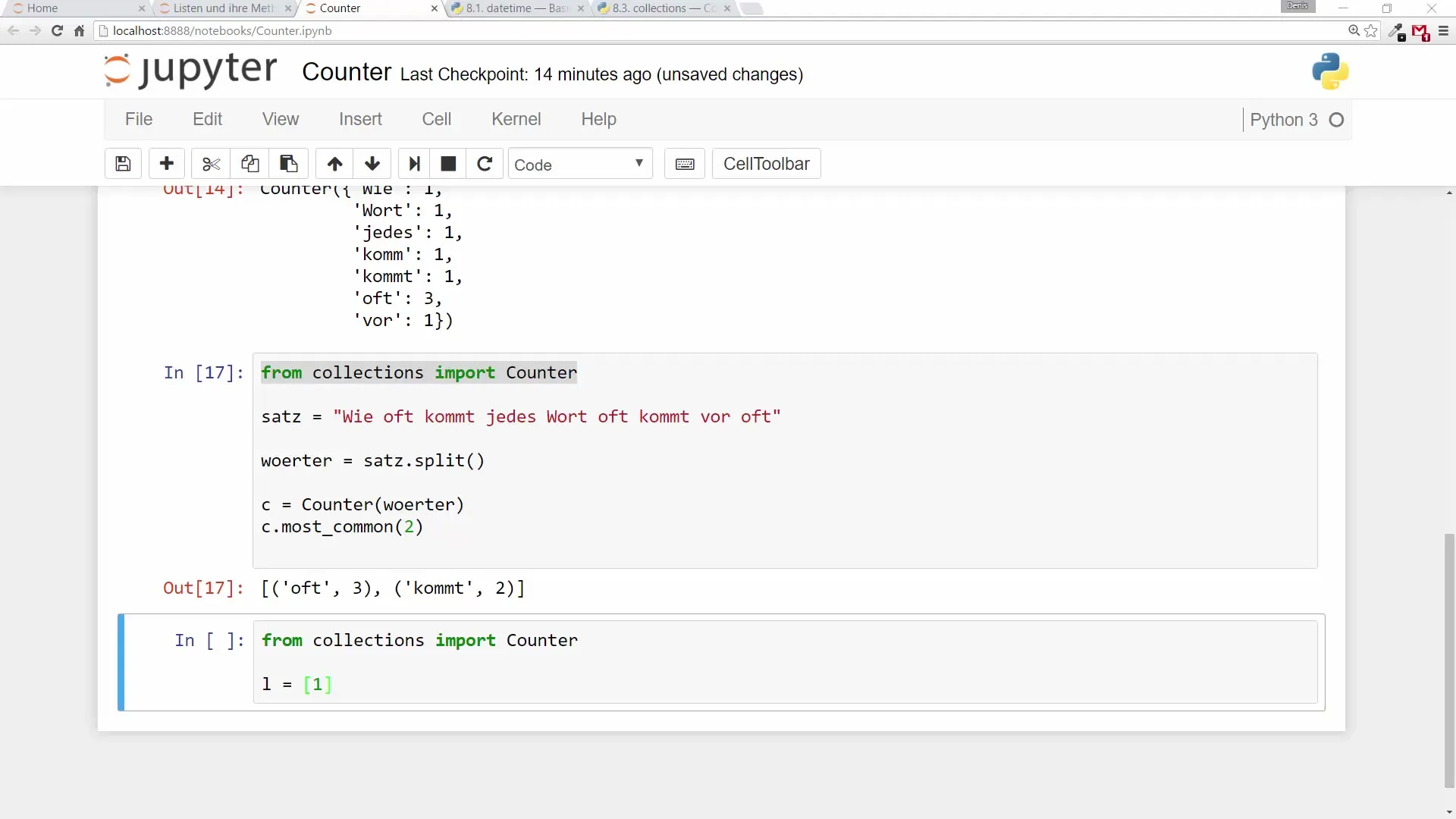
When you analyze the list, you see along with the counters how often each number occurs.
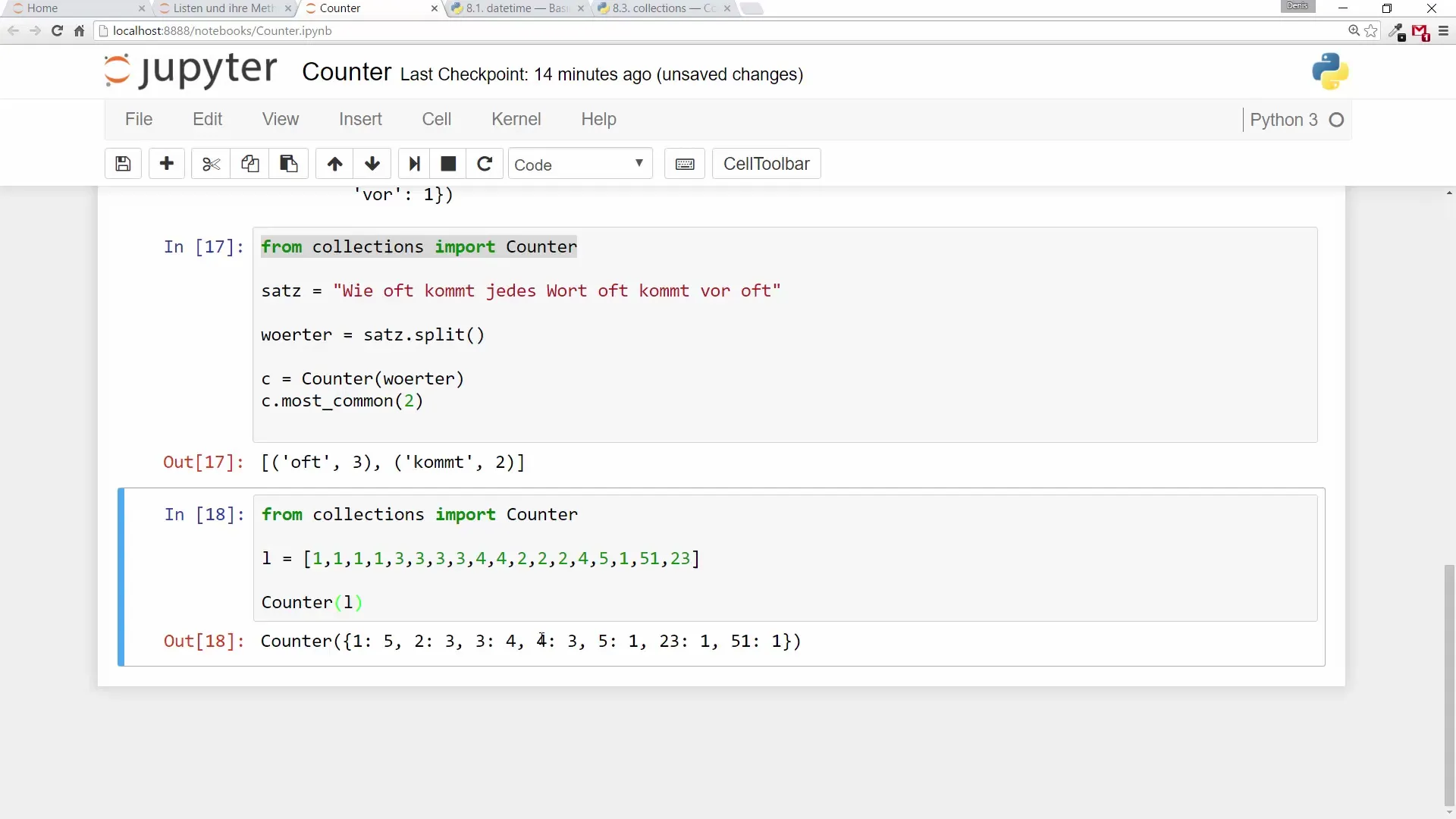
This method is particularly helpful when analyzing data such as grades or survey results.
In summary, Counters provide an excellent way to analyze the frequency of elements. Whether with letters, words, or numbers – the application is diverse and straightforward.
Summary – Python Counter: Count Your Values Effortlessly
In this guide, you learned how to work with the Counter in Python. You now know the various initialization options, the use of the update method, and the application to word and number analysis. The tools available to you can help you to process and analyze data efficiently.
Frequently Asked Questions
How do I import the collections module?You can import the module using import collections.
How can I create an empty Counter?An empty Counter can be created with collections.Counter().
How do I use the update method?The update method is called on a Counter object, followed by the values you want to add, e.g., counter.update(['A', 'B', 'C']).
Can I also use the Counter with words?Yes, you can use the Counter very effectively to count the frequency of words in a text.
What is the most_common method?The most_common method returns the most frequently occurring elements in descending order.