You have the desire to learn the basics of Python programming and want to deepen your knowledge through practical applications? In this guide, you will learn how to program a simple Black Jack game in Python. Through defined functions and logical decisions, you will grasp the dynamics of this well-known card game and take your programming skills to the next level.
Main insights
- The use of functions to modularize the code.
- The importance of logic and decision-making in the game.
- Basics of card creation and score calculation.
Step-by-step guide
1. Generate random cards
To start your game, you first need to generate some random numbers that will serve as cards. For this, you will import the random module.
It is important to create a function that draws a random card between 1 and 14. The values represent the card values, including the face cards.
def random_card(): value = random.randint(1, 14) if value == 11: return 'Jack' elif value == 12: return 'Queen' elif value == 13: return 'King' elif value == 14: return 'Ace' else: return value
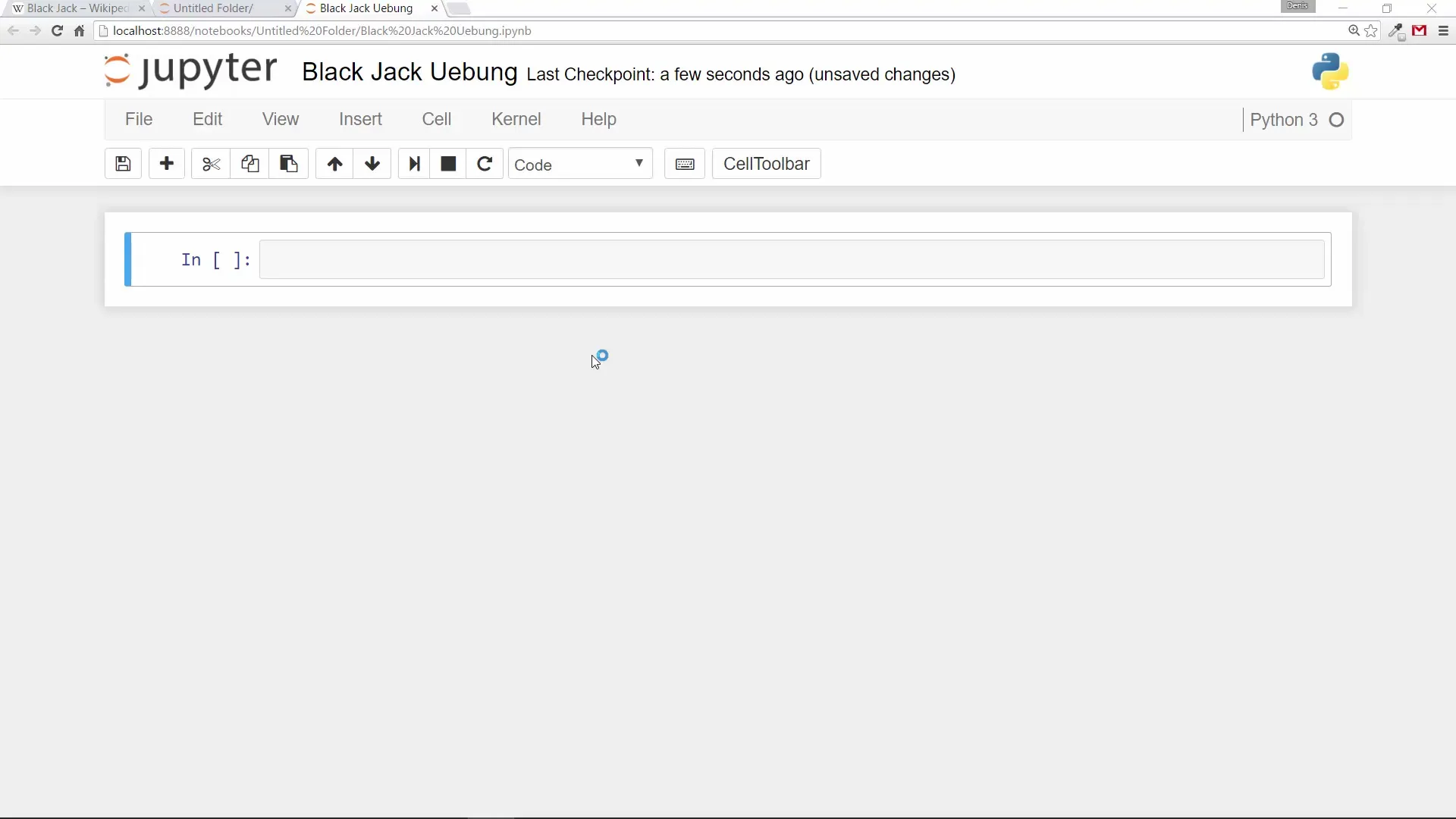
2. Calculate score
Once you can draw cards, you need to calculate the points for a player's hand. For this, you can implement a function called calculate_score.
This function takes a list of cards and returns the total sum of points. Keep in mind the special rules for the Ace, which can be worth 1 or 11 points depending on the game situation.
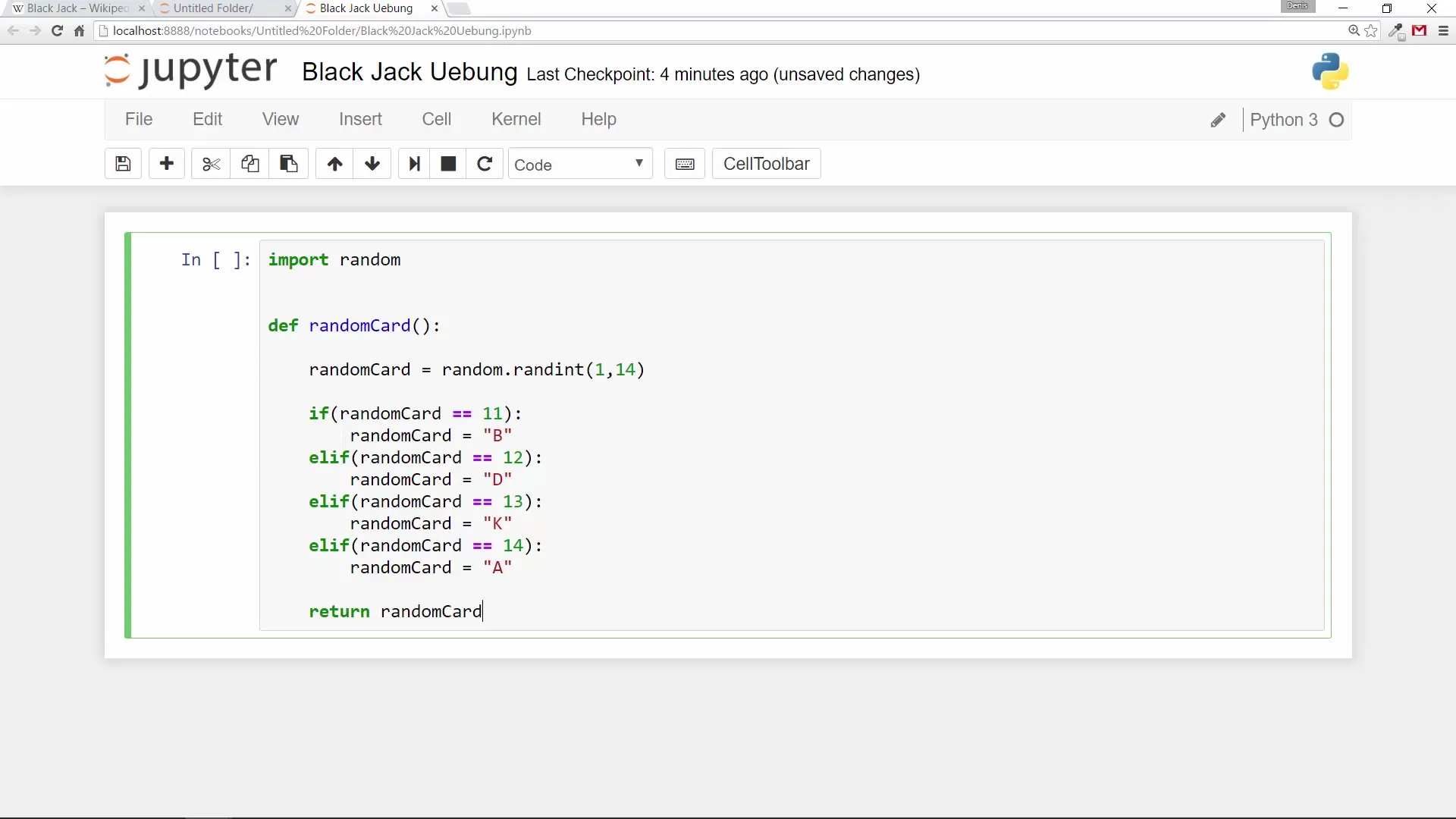
3. Determine the winner
To recognize who has won the game, you need a function that compares the scores of both hands.
This will determine if one of the players has more than 21 points and thus lost or who has more points.
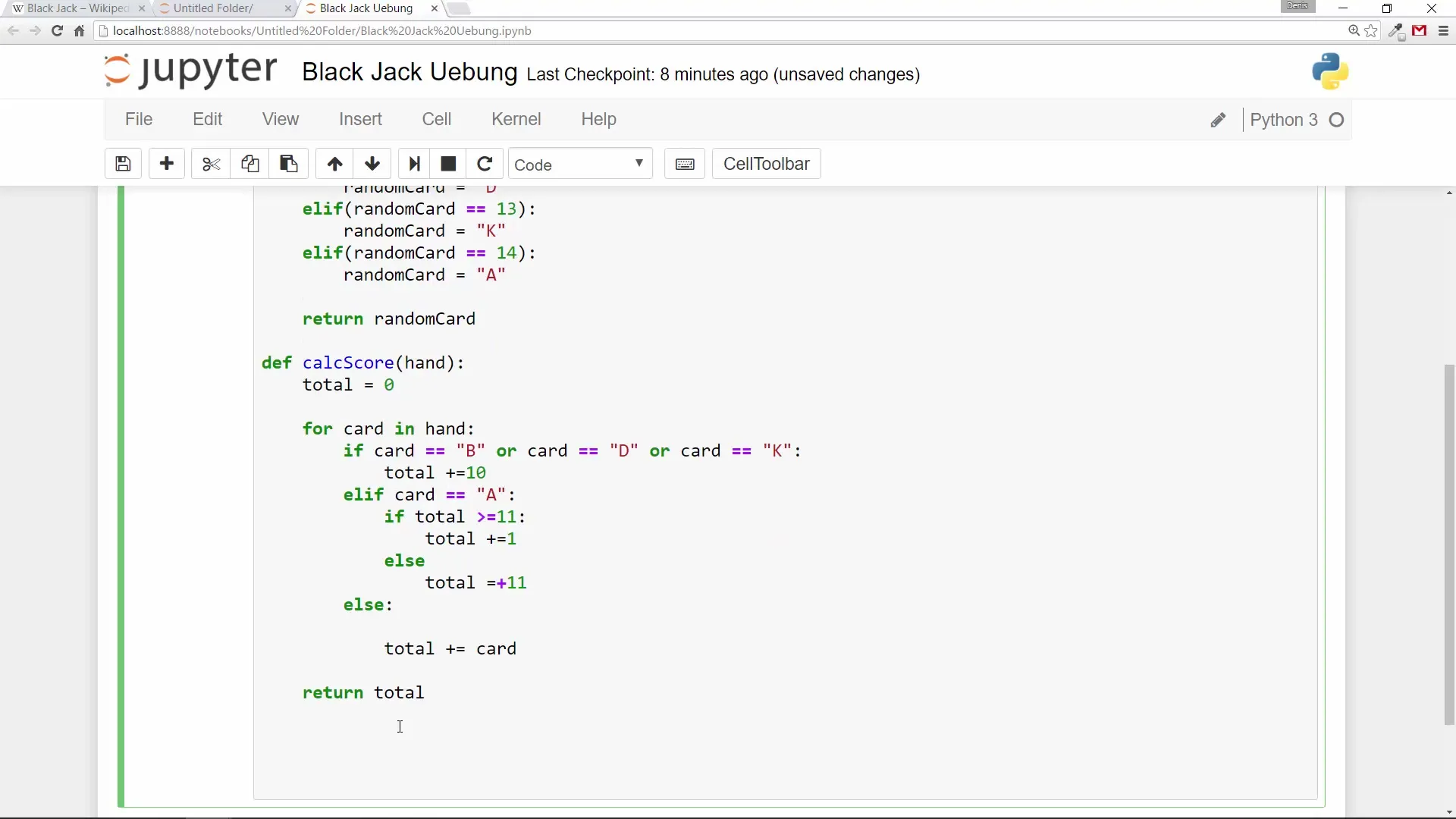
4. Drawing a card (Hit)
If the player wants to draw another card, a hit function is needed that adds a new card to the player's hand.
5. Computer strategy
The computer's decision-making on whether to draw another card can be established with an ai_strategy function.
A simple rule could be implemented here: The computer draws cards up to a certain score.
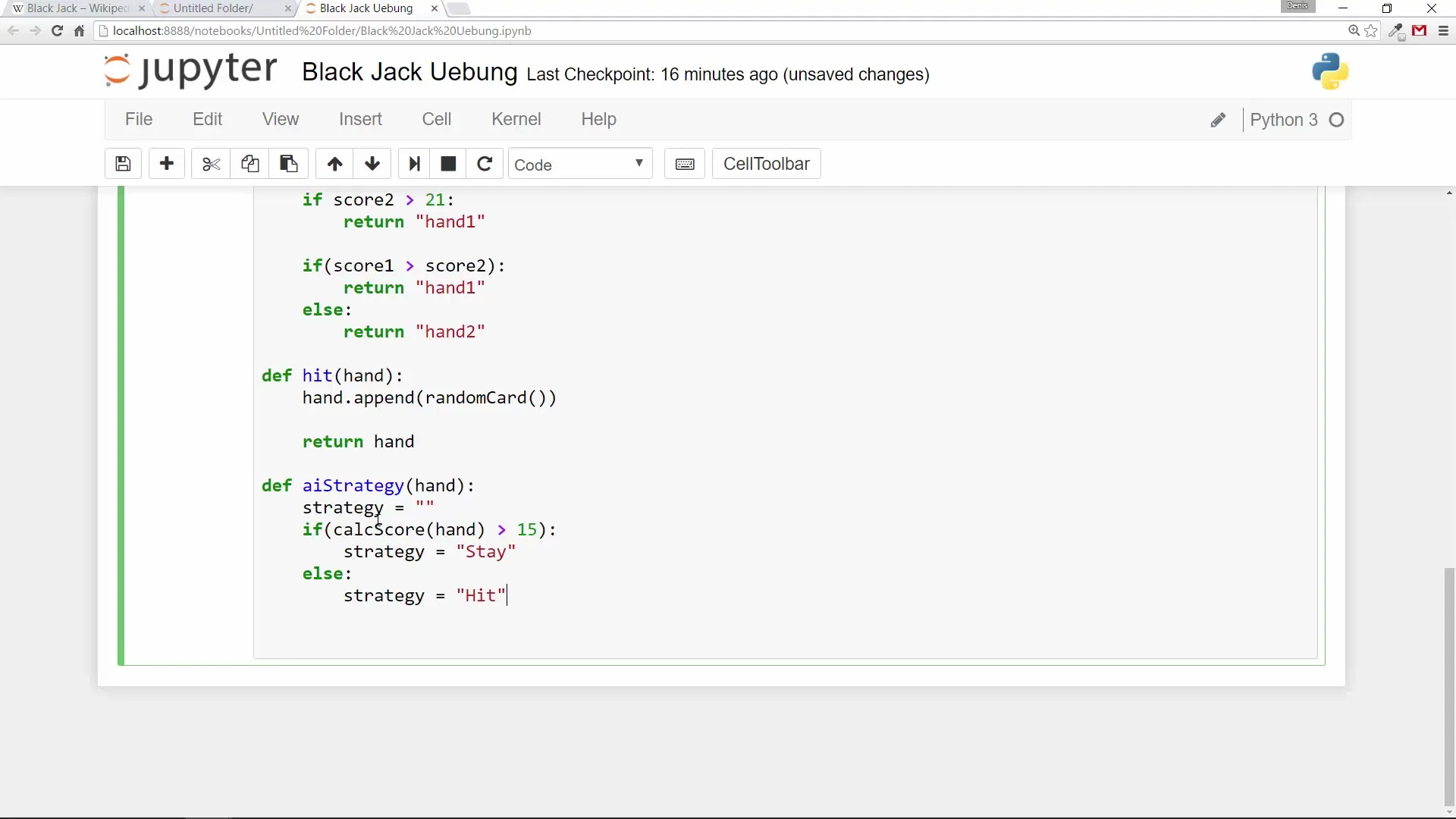
6. Deal hands
There is another useful function that provides the initial hands for the player and the computer.
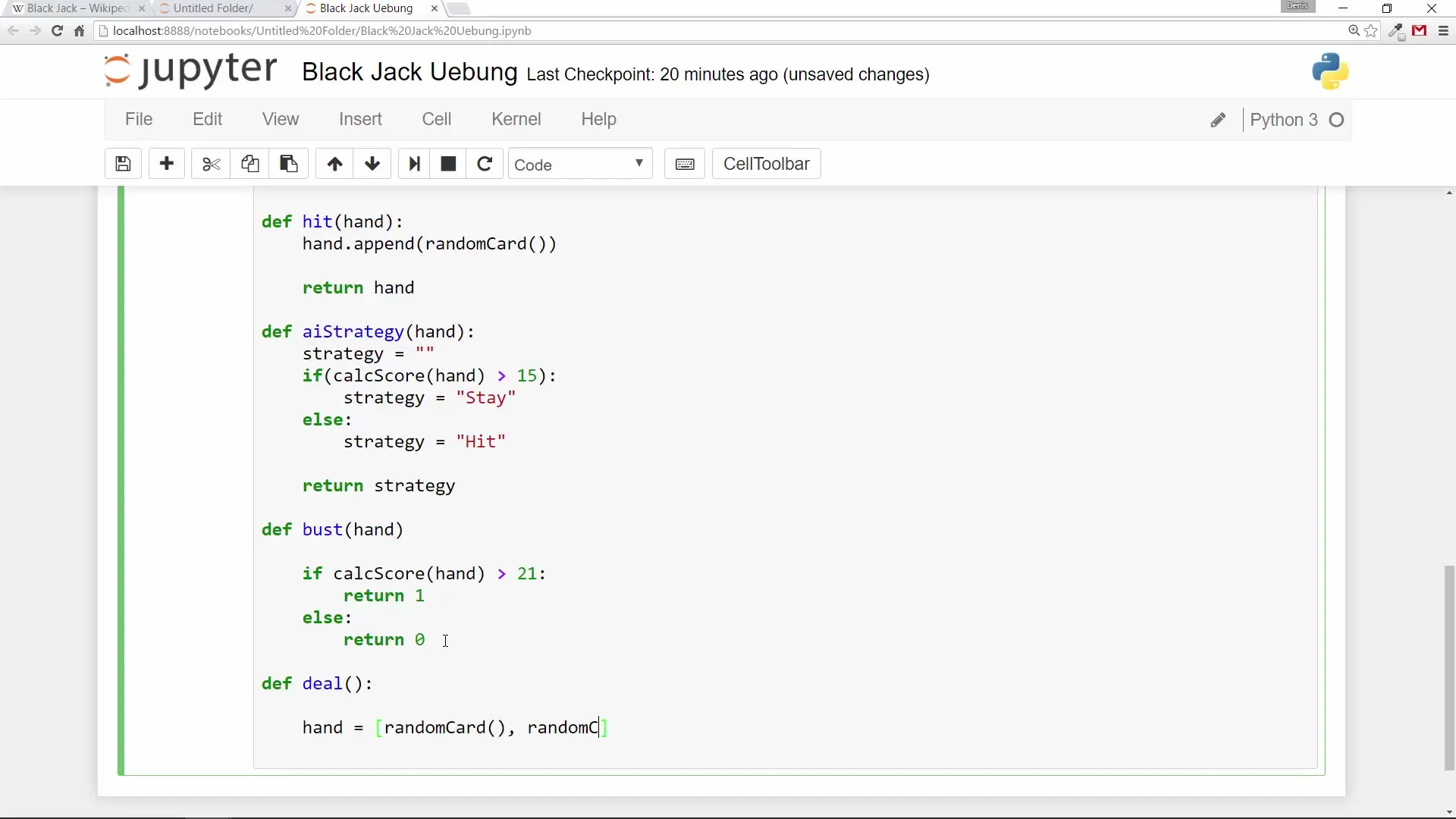
7. Control the main game
Now we need a main function that controls the game. Here you can deal cards and implement prompts for the player's inputs.
8. Play again
To give the player the option to play the game again, we will add a prompt.
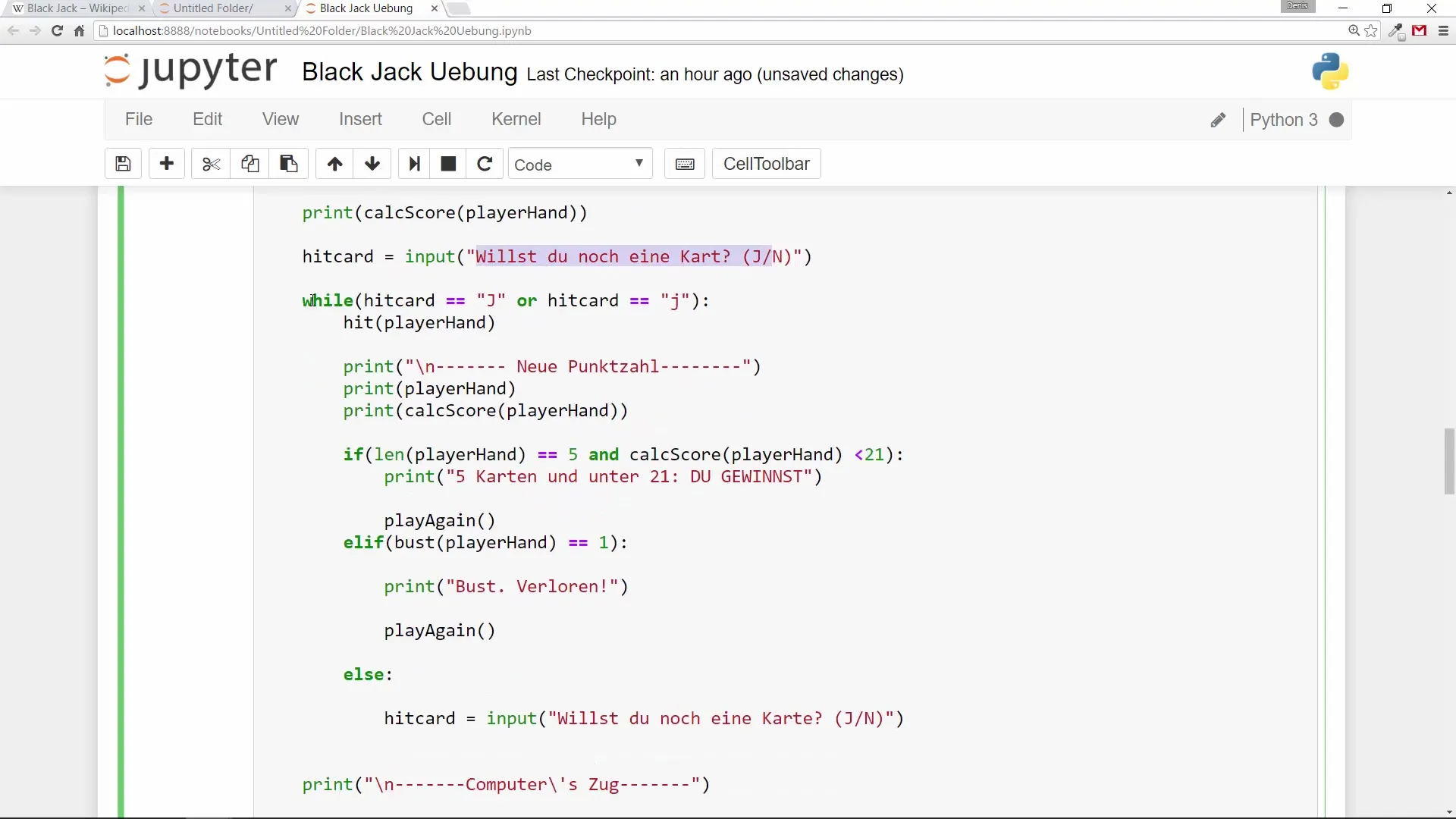
Summary – Programming Black Jack with Python
This guide takes you through creating a simple Black Jack game in Python. You have learned how to create basic functions, implement the logic for the game, and effectively control the gameplay. Use your newly acquired knowledge to develop more complex games or applications, and let your creativity run wild!
Frequently Asked Questions
How can I improve the user interface of the game?A graphical user interface (GUI) can be created using libraries such as Tkinter or Pygame.
Can I play the game online?Yes, you can implement it in a web framework like Flask or Django for web applications.
How can I customize the game rules?Simply change the conditions in the corresponding functions that determine how the game is won.