Sets are a powerful data type in Python that allows you to work with unique values. In this guide, you will learn about the various methods of sets that help you efficiently store and work with unique values. From adding and removing elements to comparing and merging sets—here you will learn everything you need to know about sets.
Key Insights
- Sets in Python store unique values and provide various methods for manipulating these values.
- It’s important to know that a set cannot store duplicate values and you can effectively operate with functions like add(), remove(), copy(), difference(), intersection(), and many others.
- Working with sets can help you efficiently compare and analyze data.
Step-by-Step Guide
To make it easy for you to work with sets, I have summarized the key methods and their applications. Check out the following steps to leverage the full potential of sets in Python.
Creating a Set and Adding Values
First, let's look at how to create a set and add values to it. To create a set, you can use the set() function or simply write the values in curly braces.
By using add(), you can add elements to the set. When you print the set now, you will see that both values are included.
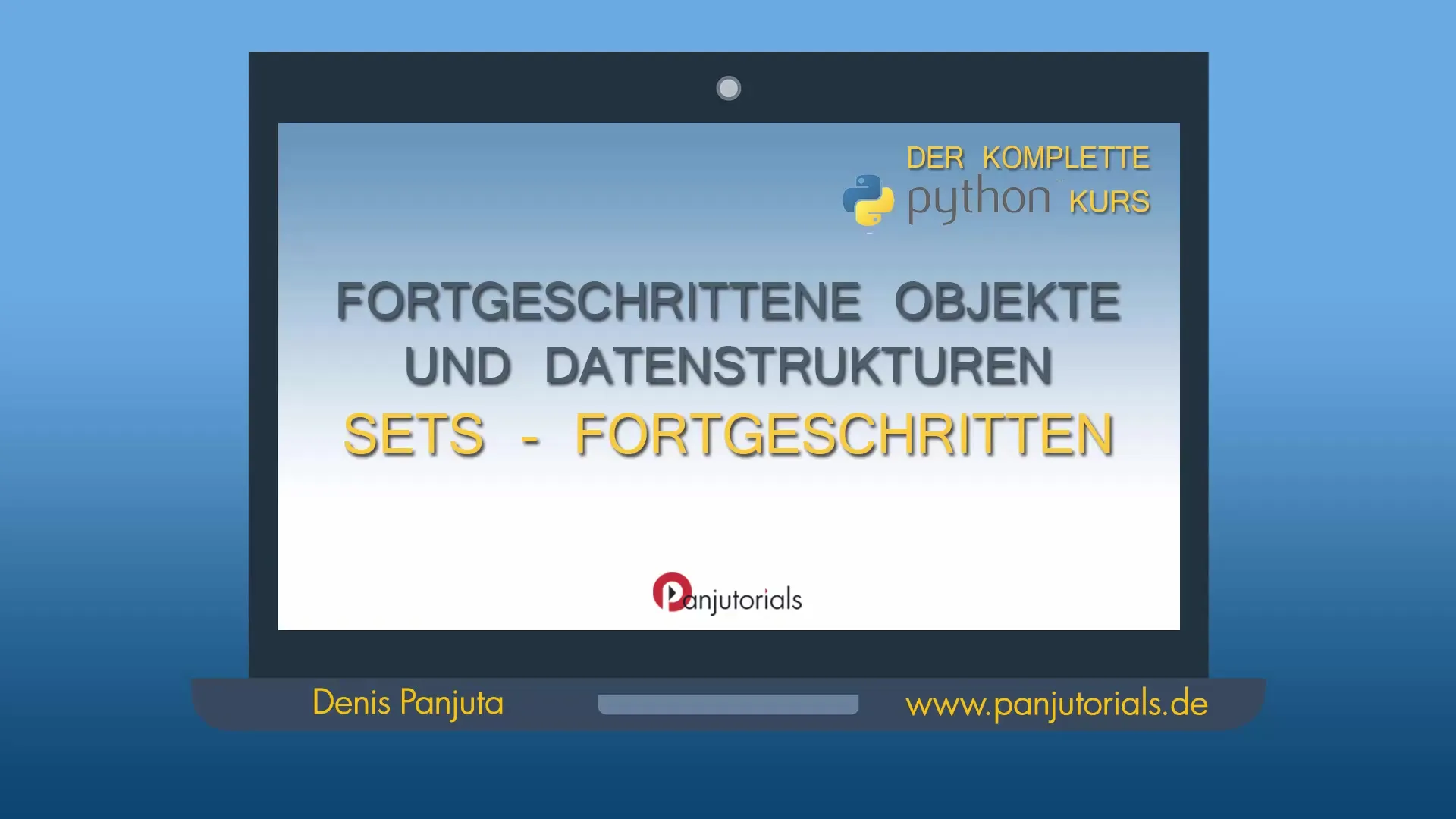
A set allows you to store only unique values. This means that if you try to add a value that already exists, it will be ignored.
Clearing a Set
Do you want to remove all values from a set? No problem! You can use the clear() method to empty the set.
When you print the set after this, you will see that it is empty.
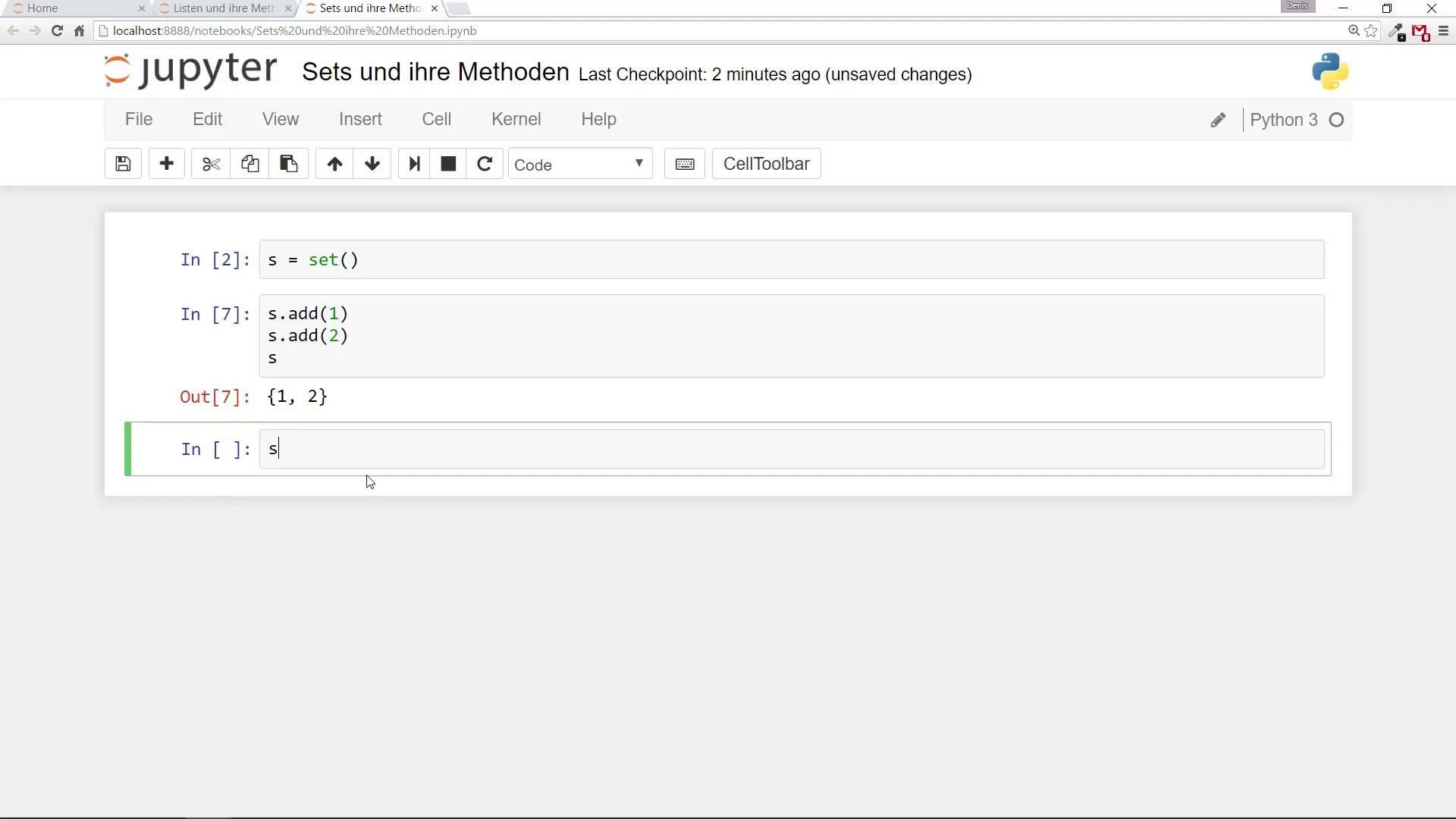
Copying a Set
If you want to copy the contents of one set into another, you can use the copy() method.
The copied set s2 now has the same values as s. However, it is a separate object, so changes to s2 do not affect s.
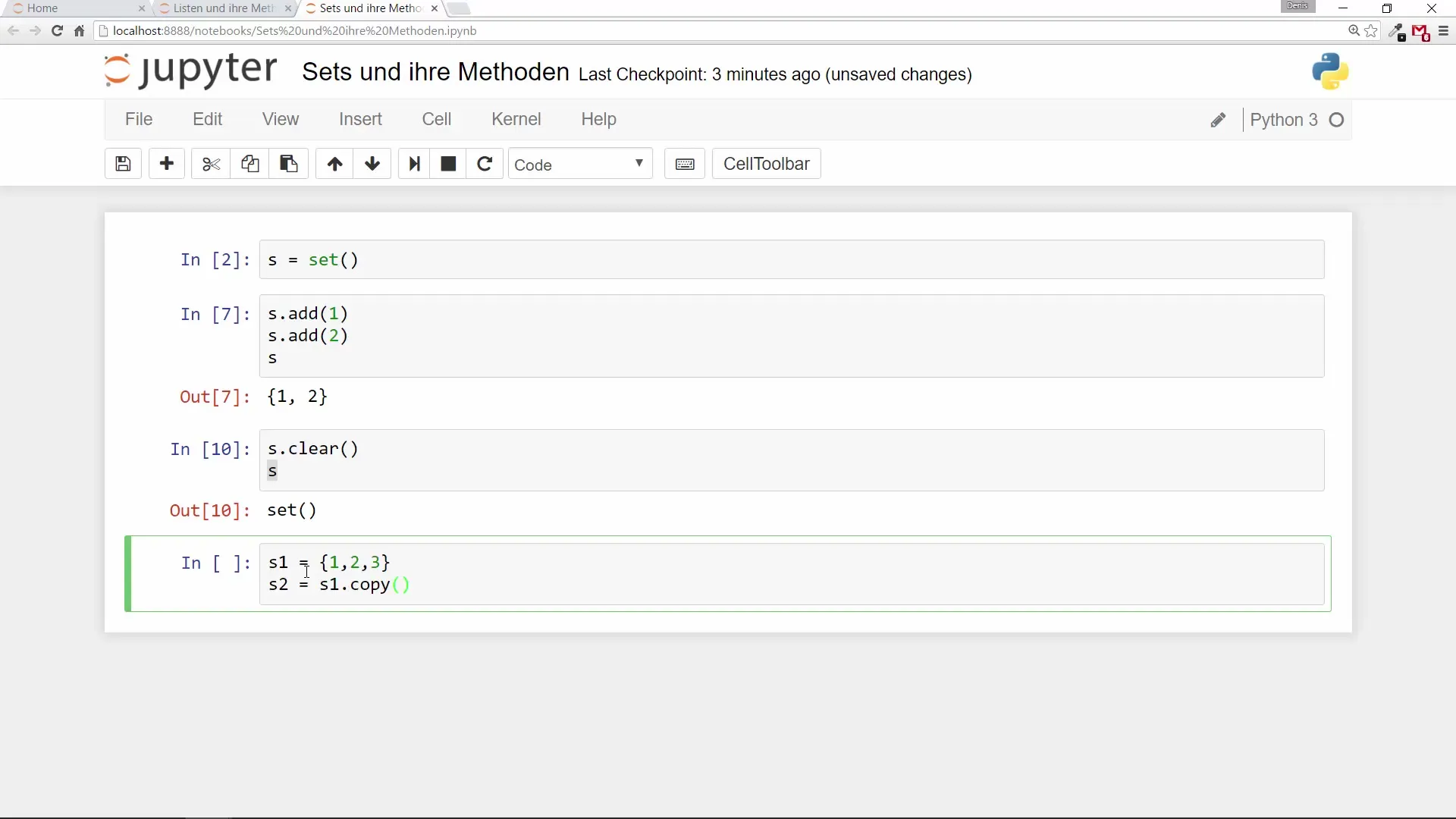
Finding Differences Between Two Sets
If you want to find the differences between two sets, use the difference() method.
You will get a set that contains only the values from s1 that are not present in s2.
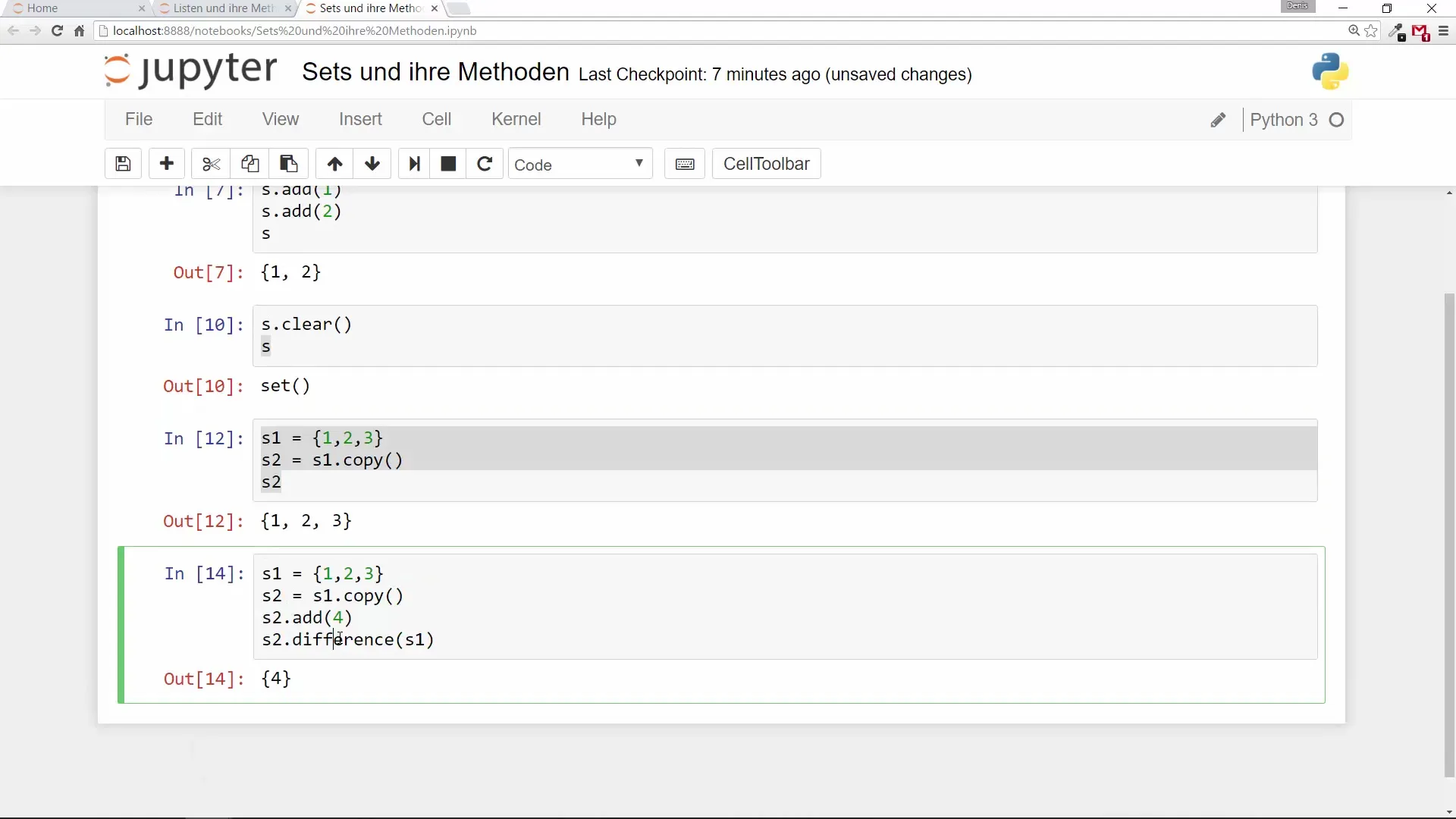
Updating Elements with the difference_update Method
If you want to update the elements in s1 so that only the values that do not exist in s2 remain, you can use the difference_update() method.
This means that after this call, only the elements of s1 that are not in s2 will remain in s1.
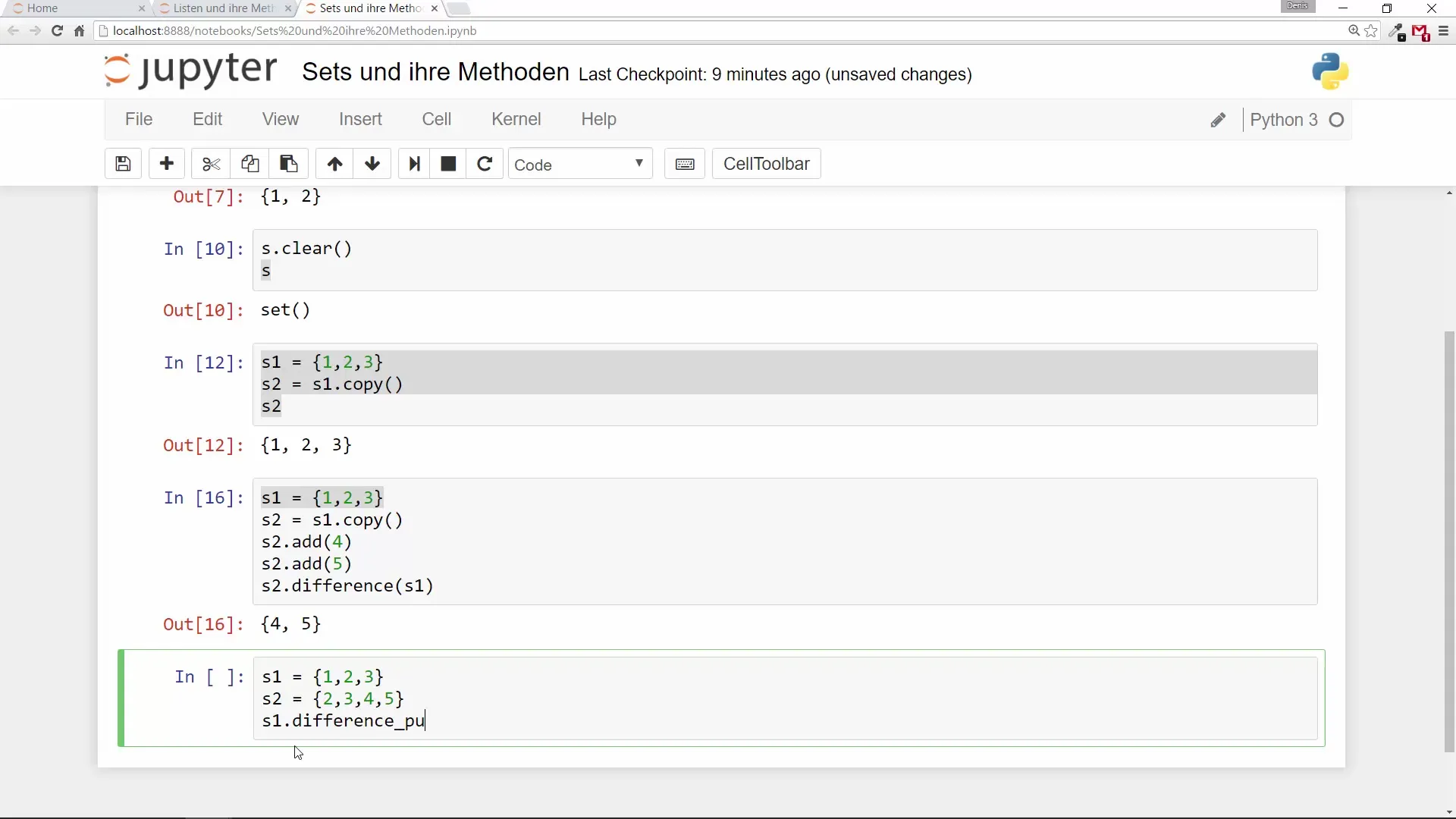
Finding Common Values
Do you want to find the common values of two sets? Use the intersection() method.
s3 contains only the values that are present in both sets.
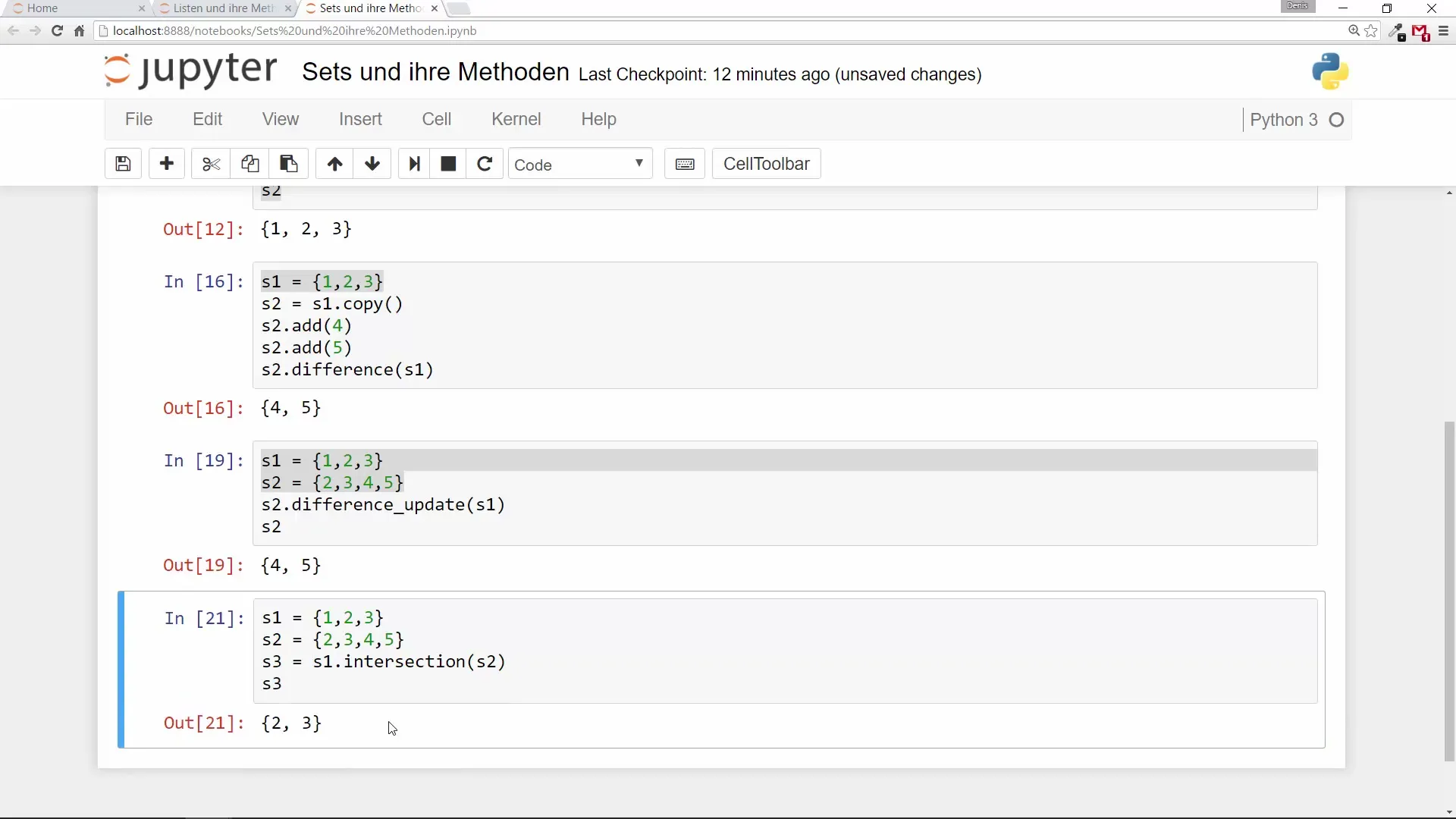
Set Union
To combine two sets into one, you can use the union() method.
The result is a new set that contains all unique values from both sets.
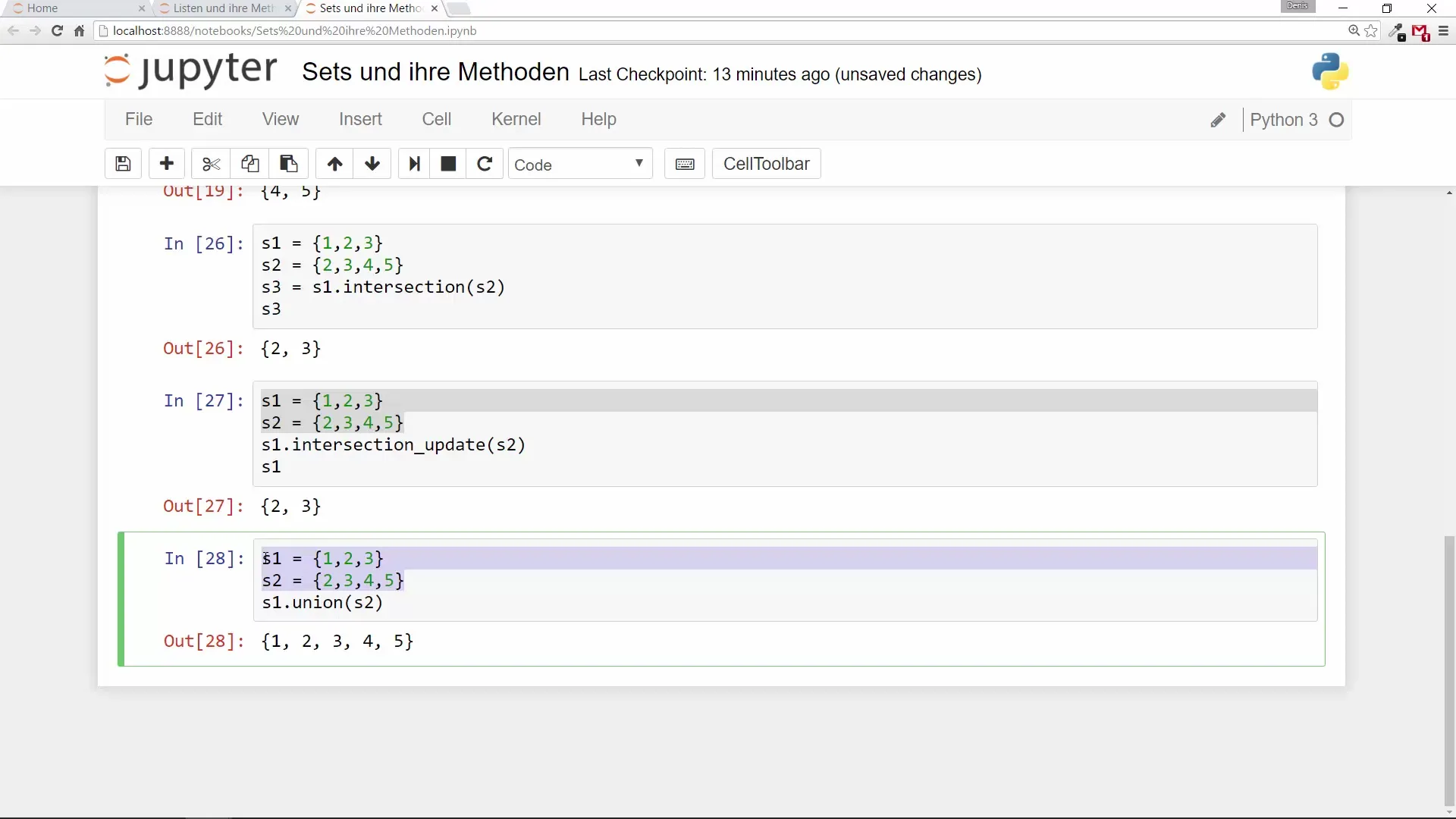
Checking for Overlaps
The isdisjoint() method is useful for determining whether there are overlaps between two sets.
This will return True if there are no common elements.
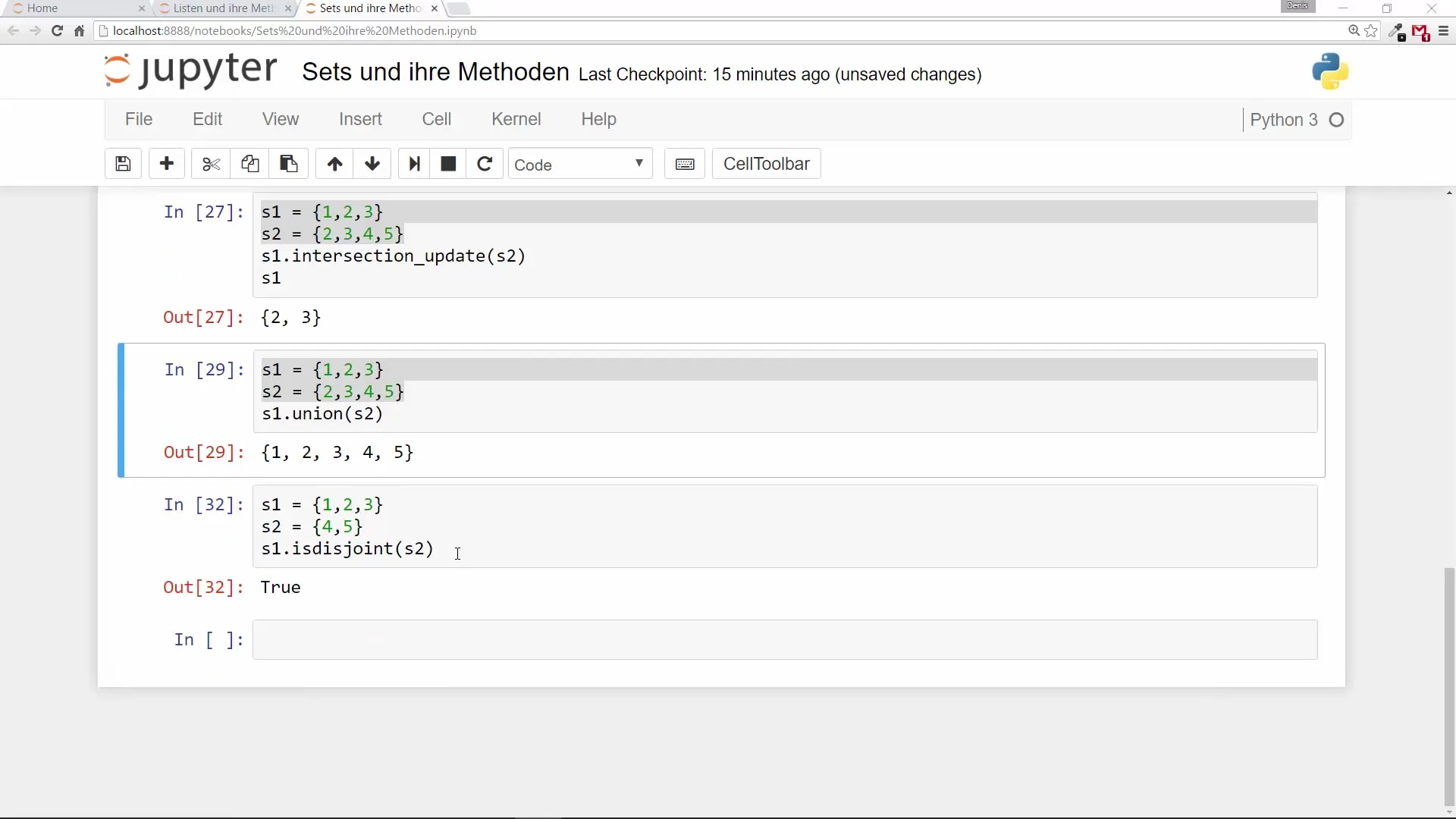
Checking for Subsets
Do you want to know if all values of one set are also present in another set? Then the issubset() method is helpful.
This returns True if every element of s1 is also present in s2.
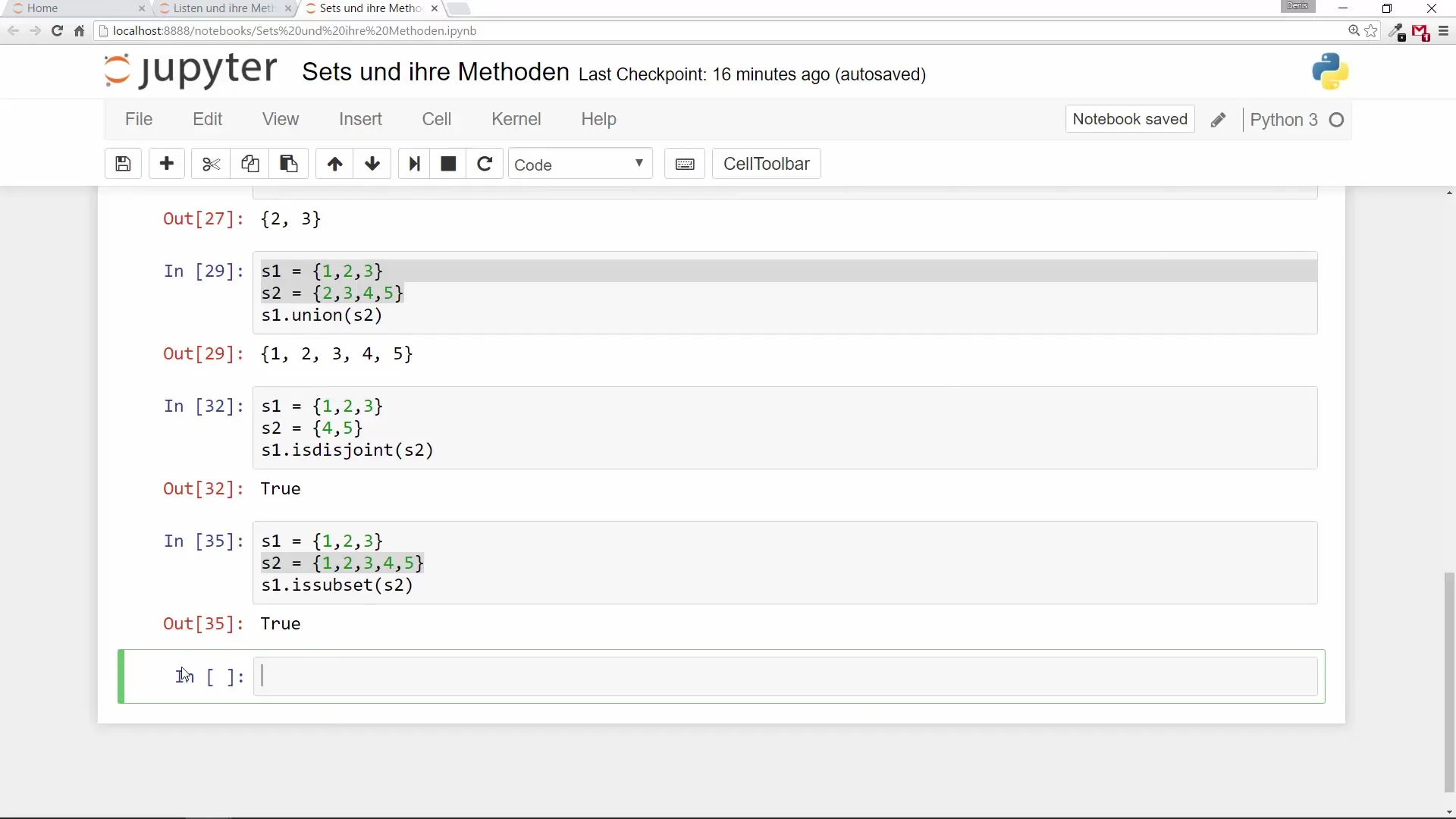
Symmetric Difference
The symmetric_difference() method returns the values that are present in only one of the two sets. This excludes common elements.
The result contains only the elements that do not appear in both sets.
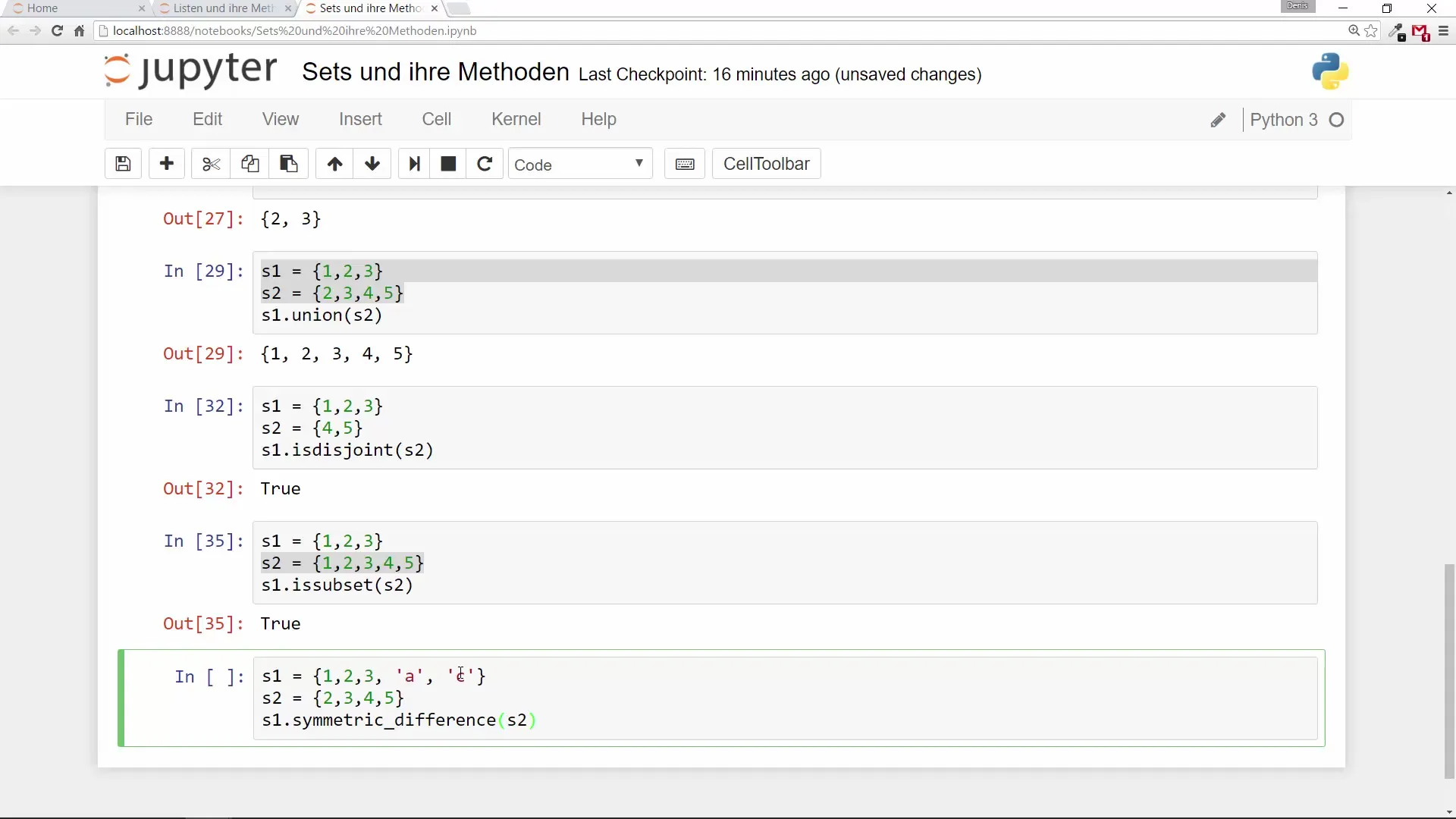
Summary – Python Programming for Beginners: Sets and Their Methods in Detail
Working with sets in Python expands your capabilities for data storage and management. Understanding methods like add(), remove(), difference(), and intersection() helps you manage data efficiently and make clear comparisons between collections. With these fundamentals, you can significantly improve your programming skills.
Frequently Asked Questions
What is a set in Python?A set in Python is a collection of unique values that does not contain duplicate elements.
What methods exist for sets?There are numerous methods, such as add(), remove(), clear(), copy(), difference(), intersection(), and many more.
How can I count the number of elements in a set?You can use the len() function to count the number of elements in a set.
Can I have sets with different data types?Yes, a set can contain elements of different data types as long as they are immutable.
How do I handle duplicate values in a set?Duplicate values are automatically ignored when adding, as a set does not allow duplicates.